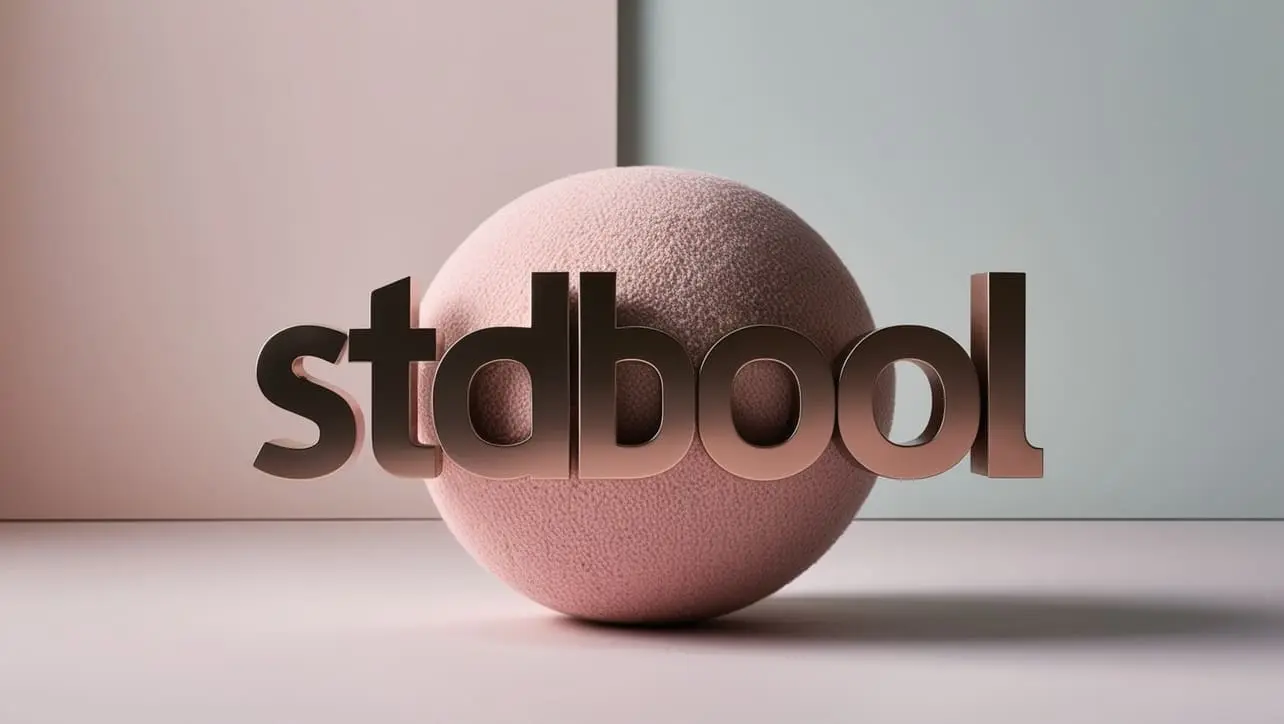
C Basic
C Interview Programs
- C Interview Programs
- C Abundant Number
- C Amicable Number
- C Armstrong Number
- C Average of N Numbers
- C Automorphic Number
- C Biggest of three numbers
- C Binary to Decimal
- C Common Divisors
- C Composite Number
- C Condense a Number
- C Cube Number
- C Decimal to Binary
- C Decimal to Octal
- C Disarium Number
- C Even Number
- C Evil Number
- C Factorial of a Number
- C Fibonacci Series
- C GCD
- C Happy Number
- C Harshad Number
- C LCM
- C Leap Year
- C Magic Number
- C Matrix Addition
- C Matrix Division
- C Matrix Multiplication
- C Matrix Subtraction
- C Matrix Transpose
- C Maximum Value of an Array
- C Minimum Value of an Array
- C Multiplication Table
- C Natural Number
- C Number Combination
- C Odd Number
- C Palindrome Number
- C Pascalβs Triangle
- C Power of 2
- C Power of 3
- C Pronic Number
- C Perfect Number
- C Perfect Square
- C Prime Factor
- C Prime Number
- C Smith Number
- C Strong Number
- C Sum of Array
- C Sum of Digits
- C Swap Two Numbers
- C Triangular Number
C Program to Condense a Number
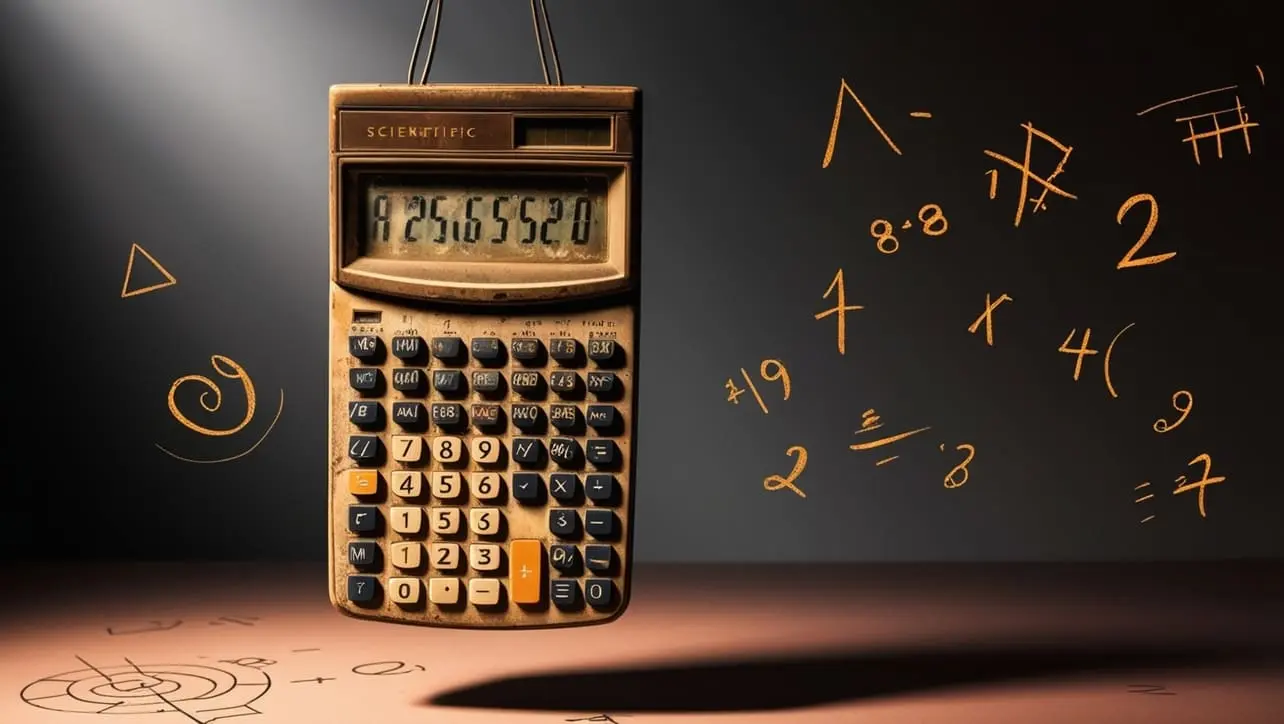
Photo Credit to CodeToFun
π Introduction
In the realm of programming, various tasks involve manipulating and transforming numbers. One interesting problem is condensing a number, which means reducing a number by summing its digits until a single-digit number is obtained. This process is often referred to as finding the digital root or digit sum.
In this tutorial, we will explore a C program designed to condense a given number. The program involves repeatedly summing the digits of the number until a single-digit result is achieved.
π Example
Let's delve into the C code that accomplishes this task.
#include <stdio.h>
// Function to condense a number
int condenseNumber(int number) {
// Continue summing digits until a single-digit number is obtained
while (number > 9) {
int sum = 0;
// Sum the digits of the number
while (number > 0) {
sum += number % 10;
number /= 10;
}
// Update the number with the sum
number = sum;
}
// Return the condensed number
return number;
}
// Driver program
int main() {
// Replace this value with the number you want to condense
int number = 9875;
// Call the function to condense the number
int condensedNumber = condenseNumber(number);
// Display the result
printf("The condensed form of %d is: %d\n", number, condensedNumber);
return 0;
}
π» Testing the Program
To test the program with different numbers, modify the value of number in the main program.
The condensed form of 9875 is: 2
π§ How the Program Works
- The program defines a function condenseNumber that takes an integer number as input and condenses it by summing its digits until a single-digit result is obtained.
- Inside the main program, replace the value of number with the desired number you want to condense.
- The program calls the condenseNumber function and prints the result.
π§ Understanding the Concept of Condensing a Number
Before delving into the code, let's understand the concept behind condensing a number. The process involves repeatedly summing the digits of the number until a single-digit result is achieved.
For example, consider the number 9875. The program would calculate 9 + 8 + 7 + 5 = 29, then 2 + 9 = 11, and finally 1 + 1 = 2. The condensed form is 2.
π’ Optimizing the Program
While the provided program is straightforward, consider exploring and implementing alternative approaches or optimizations for condensing numbers.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
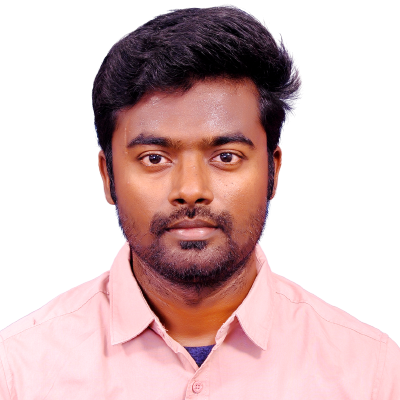
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C Program to Condense a Number) please comment here. I will help you immediately.