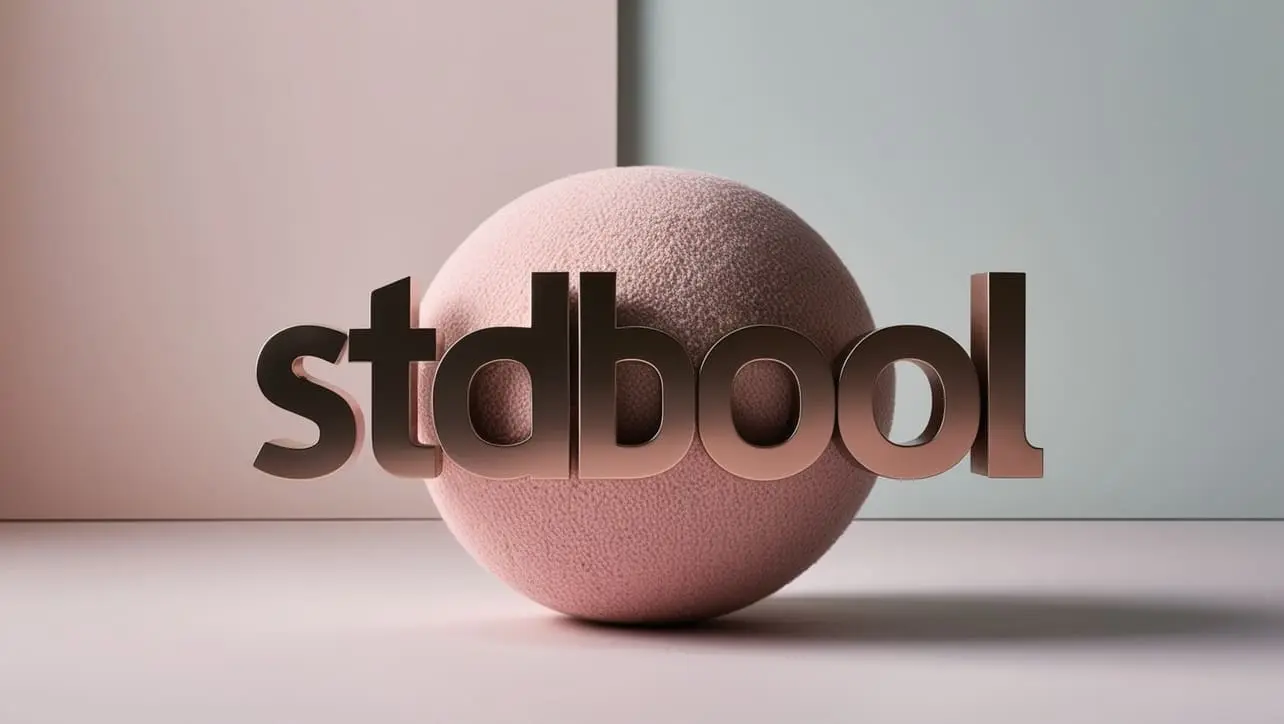
C Basic
C Math Functions
C atanh() Function
.webp)
Photo Credit to CodeToFun
π Introduction
In C programming, the atanh()
function is part of the <math.h> library, and it stands for arc hyperbolic tangent.
This function calculates the inverse hyperbolic tangent (or arctangent) of a given value. In mathematical terms, it finds the value whose hyperbolic tangent is the specified number.
In this tutorial, we'll explore the usage and functionality of the atanh()
function in C.
π‘ Syntax
The syntax for the atanh()
function is as follows:
double atanh(double x);
- x: The value whose inverse hyperbolic tangent is to be calculated.
The function returns the hyperbolic tangent value.
π Example
Let's dive into an example to illustrate how the atanh()
function works.
#include <stdio.h>
#include <math.h>
int main() {
double x = 0.5;
// Calculate inverse hyperbolic tangent
double result = atanh(x);
// Output the result
printf("Inverse hyperbolic tangent of %f: %f\n", x, result);
return 0;
}
π» Output
Inverse hyperbolic tangent of 0.500000: 0.549306
π§ How the Program Works
In this example, the atanh()
function is used to calculate the inverse hyperbolic tangent of the value 0.5, and the result is printed.
β©οΈ Return Value
The atanh()
function returns the inverse hyperbolic tangent of the given value x in radians.
π Common Use Cases
The atanh()
function is particularly useful in mathematical and scientific calculations involving hyperbolic functions. It can be used, for example, in statistics, physics, or any domain where hyperbolic functions are relevant.
π Notes
- The argument x must be in the range [β1,1] to avoid undefined results.
- The result is in radians. If degrees are needed, you can convert the result using the formula: result_degrees=result_radiansΓ(180/Ο).
π’ Optimization
The atanh()
function is typically optimized for performance and accuracy. Ensure that the input values are within the valid range to avoid undefined behavior.
π Conclusion
The atanh()
function in C provides a powerful tool for calculating the inverse hyperbolic tangent. It is a valuable asset in mathematical computations that involve hyperbolic functions.
Feel free to experiment with different values of x to observe the behavior of the atanh()
function in various scenarios. Happy coding!
π¨βπ» Join our Community:
Author
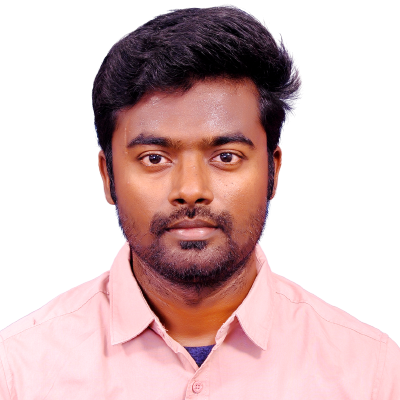
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C atanh() Function) please comment here. I will help you immediately.