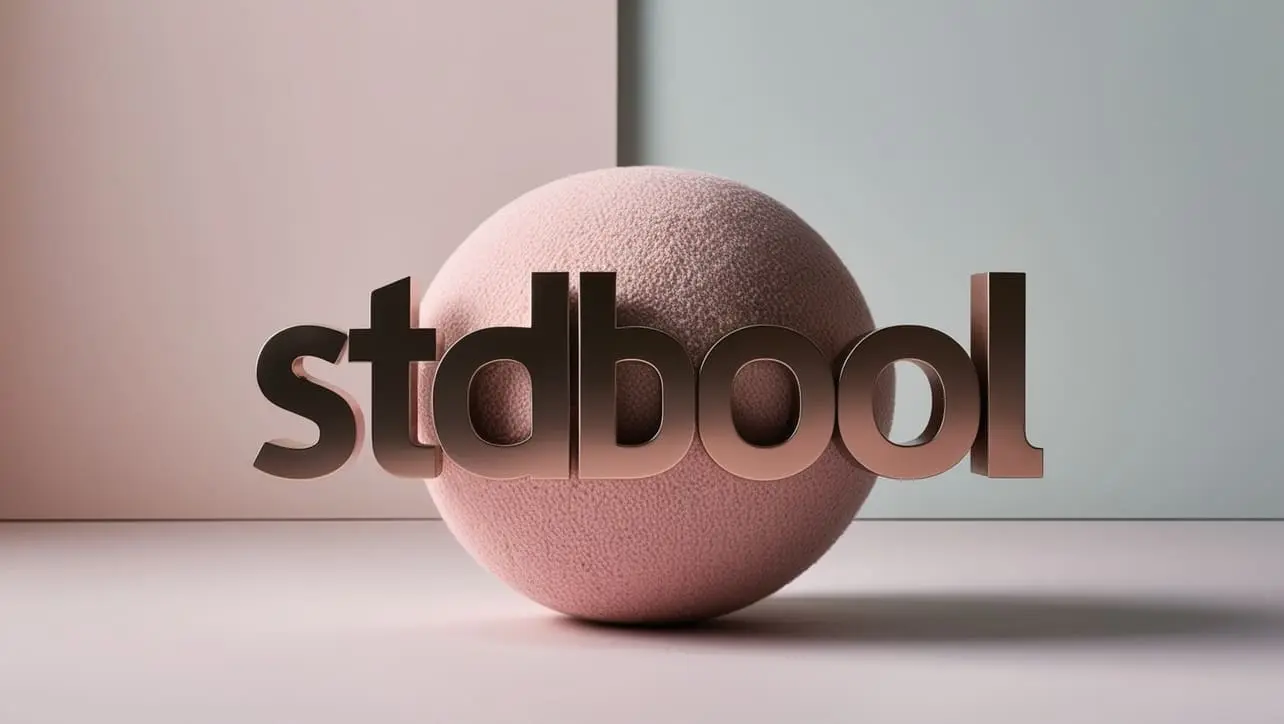
C Library – assert.h
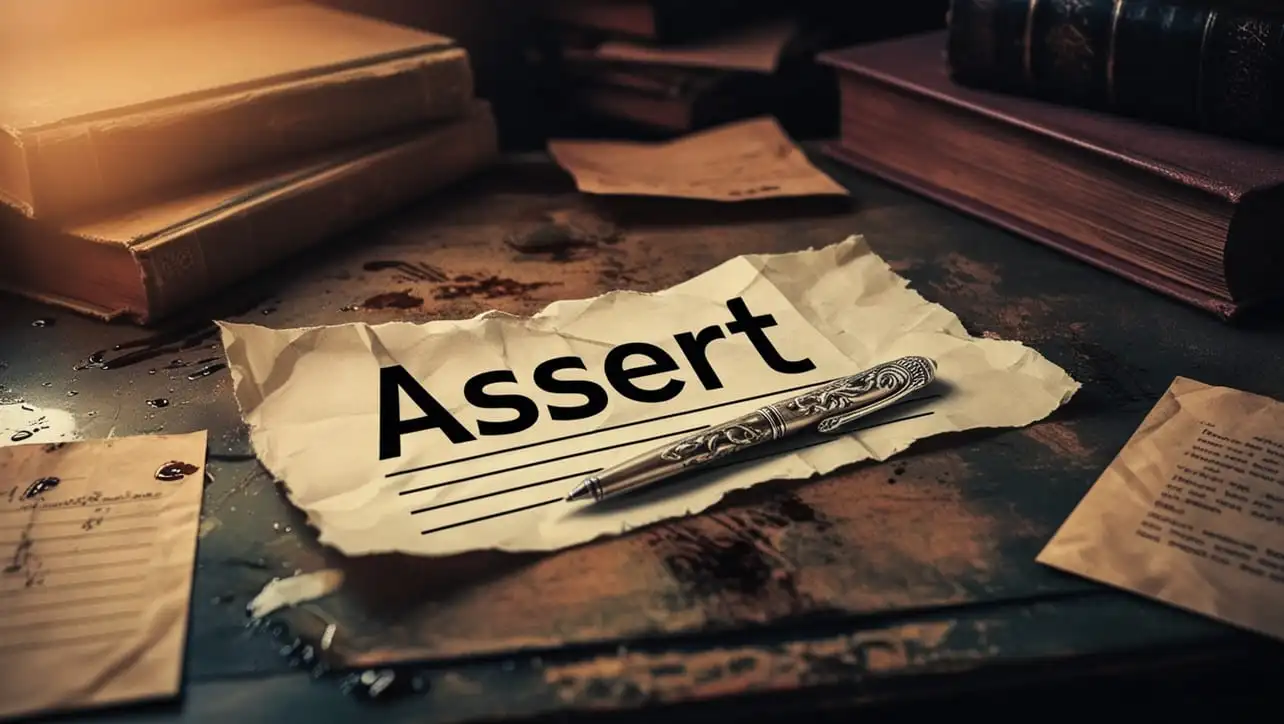
Photo Credit to CodeToFun
🙋 Introduction
The assert.h
header in C provides a mechanism for adding diagnostics to your programs. It defines a macro assert which can be used to verify assumptions made by the program and to detect logical errors and bugs. When the expression passed to assert evaluates to false, the macro writes an error message to the standard error stream and terminates the program.
💡 Syntax
To use the assert.h
library, you need to include it in your program:
#include <assert.h>
Macros Defined in assert.h
The assert.h
header defines the following macro:
- assert: This macro tests the given expression and triggers an error message if the expression is false.
Syntax of the assert Macro
void assert(expression);
- expression: The condition to be tested. If the expression evaluates to zero (false), assert will output an error message and terminate the program.
📄 Example
Below is a simple example demonstrating how to use the assert macro to verify assumptions in your code. This example includes a function that calculates the square root of a number, using assert to check that the input is non-negative.
#include <stdio.h>
#include <math.h>
#include <assert.h>
// Function to calculate the square root of a number
double calculate_sqrt(double number) {
// Ensure the number is non-negative
assert(number >= 0);
return sqrt(number);
}
int main() {
double num1 = 25.0;
double num2 = -4.0;
printf("Square root of %.2f is %.2f\n", num1, calculate_sqrt(num1));
// This will cause the program to terminate with an assertion failure
printf("Square root of %.2f is %.2f\n", num2, calculate_sqrt(num2));
return 0;
}
💻 Output
Square root of 25.00 is 5.00 a.out: main.c:8: calculate_sqrt: Assertion `number >= 0' failed.
🧠 How the Program Works
- Function Definition: The calculate_sqrt function takes a double number and returns its square root.
- Using assert: The assert macro checks that number is non-negative. If number is negative, assert will print an error message and terminate the program.
- Calling the Function: In the main function, calculate_sqrt is called with a positive number and a negative number. The first call succeeds, while the second call triggers an assertion failure, demonstrating the use of assert.
🚧 Common Pitfalls
- Side Effects in Expressions: Avoid using expressions with side effects (e.g., function calls) in assert statements, as these may not be executed if assertions are disabled.
- Disabling Assertions: In production code, assertions can be disabled by defining the NDEBUG macro. This can lead to different behavior between debug and release builds if assert is used for essential checks.
- Performance Overhead: Excessive use of assert in performance-critical sections can introduce overhead. Assertions should be used judiciously.
- Terminating the Program: Remember that assert will terminate the program if the expression is false. This behavior is not suitable for handling recoverable errors in production code.
✅ Best Practices
- Use for Debugging: Use assert primarily for debugging and development to catch logical errors and invalid assumptions early.
- Clear Error Messages: Ensure that the error messages produced by assert are clear and provide enough context to understand the failure.
- Avoid in Production: Avoid relying on assert for error handling in production code. Use proper error handling mechanisms instead.
- Document Assertions: Document the conditions being checked by assert to improve code readability and maintainability.
- Disable in Release Builds: Consider disabling assertions in release builds by defining NDEBUG to avoid unnecessary performance overhead.
🎉 Conclusion
The assert.h
header is a valuable tool for C programmers, providing a simple way to verify assumptions and detect logical errors during development.
By using the assert macro, you can ensure that your code behaves as expected and catch bugs early in the development process. However, it is essential to use assert judiciously and follow best practices to avoid common pitfalls and ensure that your code remains robust and maintainable in production.
👨💻 Join our Community:
Author
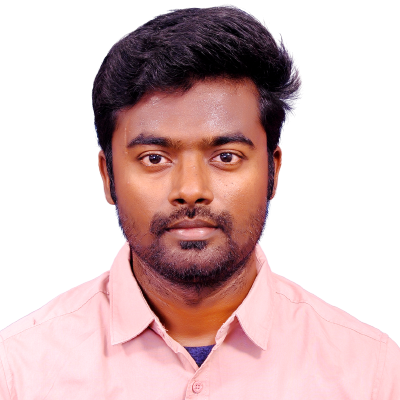
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C Library – assert.h), please comment here. I will help you immediately.