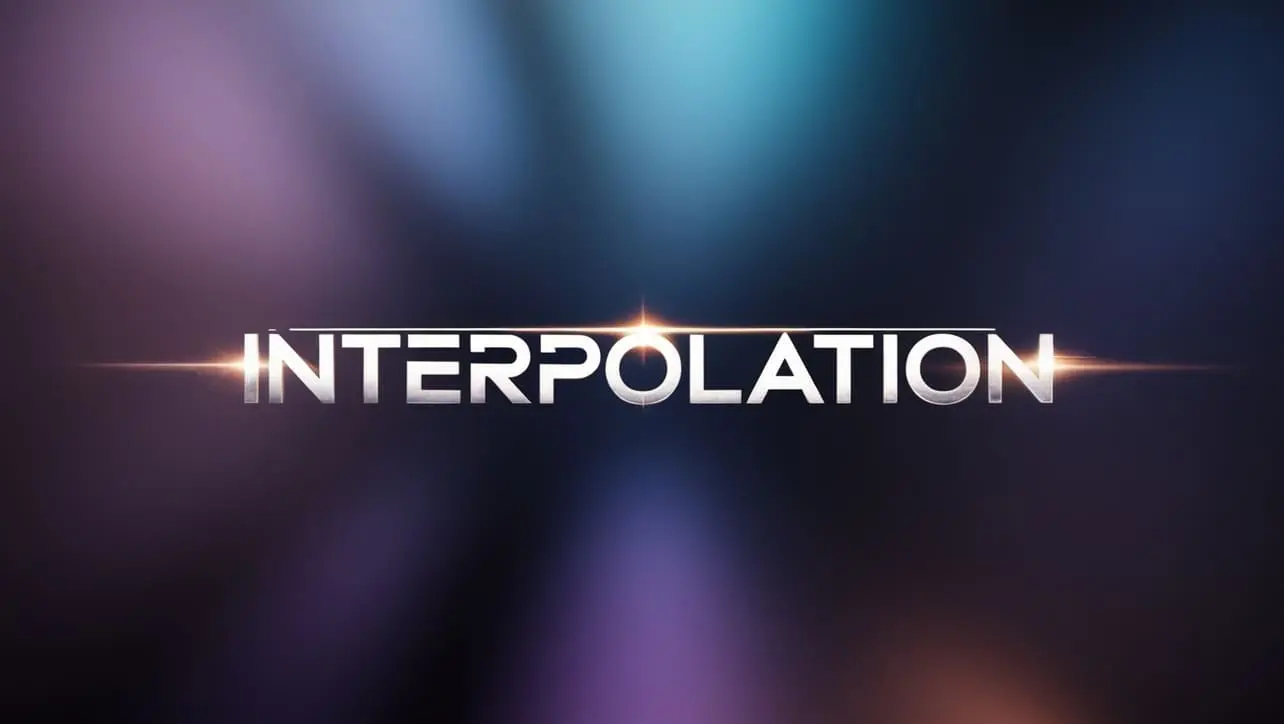
Sass Topics
- Sass Introduction
- Sass Installation
- Sass Nested Rules and Properties
- Sass Variables
- Sass @ Rules
- Sass Flow Control
- Sass Operators
- Sass Comments
- Sass Color Functions
- Sass Selector Functions
- Sass Introspection Functions
- Sass Map Functions
- Sass List Functions
- Sass Numeric Functions
- Sass String Functions
Sass abs() Function
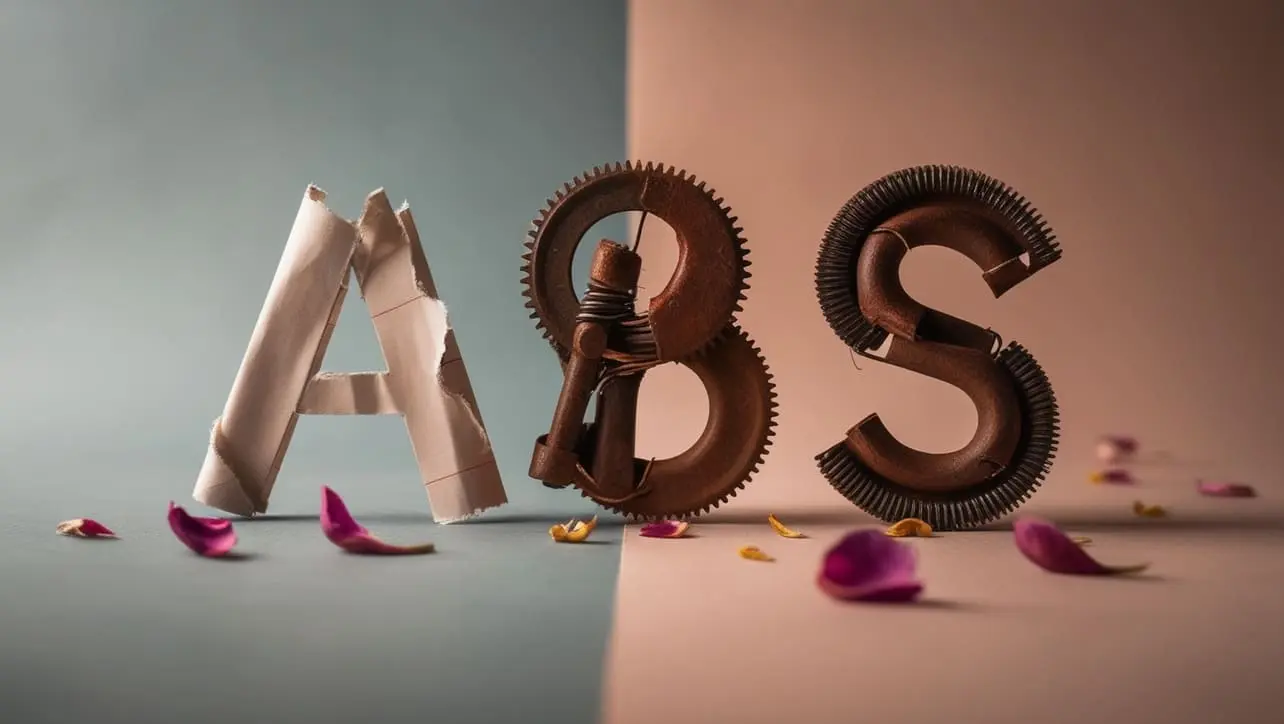
Photo Credit to CodeToFun
đ Introduction
The abs()
function in Sass is a simple yet essential tool for working with numeric values. It returns the absolute value of a given number, which means it converts any negative number into its positive equivalent.
This function is particularly useful in mathematical calculations where only positive values are desired, regardless of the input.
đĄ Syntax
The syntax of the abs()
function is straightforward, requiring just a single numeric argument:
abs(number)
đĸ Parameters
- number: The number (either positive or negative) from which you want to obtain the absolute value.
âŠī¸ Return Value
The function returns the absolute value of the input number. If the input number is positive or zero, it returns the same value. If the input number is negative, it returns the positive equivalent.
đ Example Usage
Let's explore some examples to see how abs()
can be applied in different scenarios.
đ Example 1: Basic Usage
$positive-number: 25;
$negative-number: -40;
$result1: abs($positive-number); // 25
$result2: abs($negative-number); // 40
.container {
width: abs(-100px); // 100px
height: $result2 * 1px; // 40px
}
In this example, abs()
converts the negative number -40 to its absolute value 40, which is then used for setting the height of a container.
đ Example 2: Using abs() in Calculations
$number1: -15;
$number2: 10;
$total: abs($number1) + $number2; // 25
.progress-bar {
width: $total * 1%;
}
Here, the absolute value of -15 is calculated and added to 10, resulting in a width of 25% for a progress bar.
đ Example 3: Applying abs() in Loops
@for $i from -3 through 3 {
.item-#{$i} {
margin-left: abs($i) * 10px;
}
}
In this loop, the abs()
function ensures that all margin values are positive, regardless of the loop's negative iterations.
đ Conclusion
The abs()
function in Sass is an indispensable tool when working with numbers, especially when you need to ensure positive values in your stylesheets. It simplifies the process of handling numeric data, making your Sass code more robust and predictable.
Whether you're setting dimensions, performing calculations, or iterating through loops, abs()
provides a reliable way to manage numeric values without worrying about negative numbers affecting your layout. By integrating the abs()
function into your Sass workflow, you can write cleaner, more maintainable code that behaves consistently across different scenarios.
đ¨âđģ Join our Community:
Author
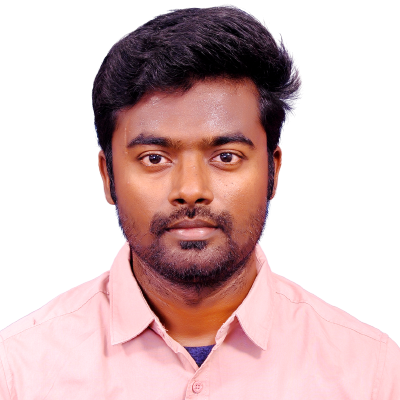
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Sass abs() Function), please comment here. I will help you immediately.