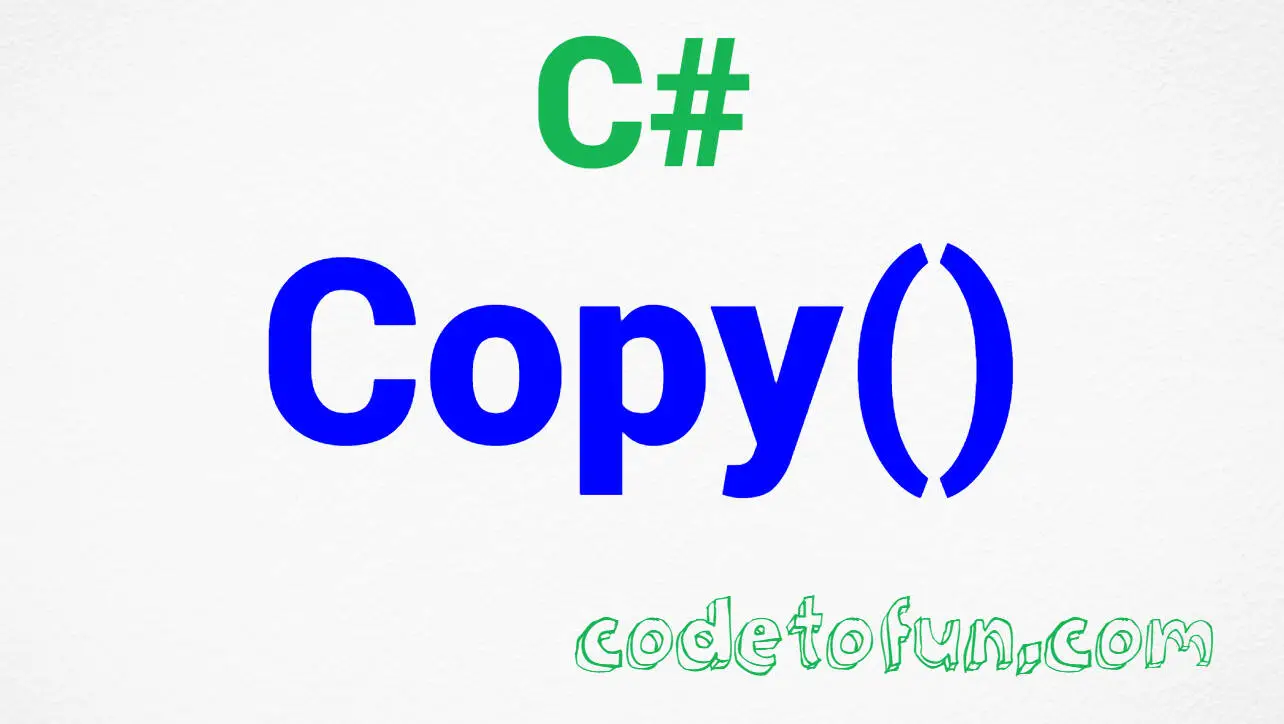
C# Basic
C# String Equals() Method
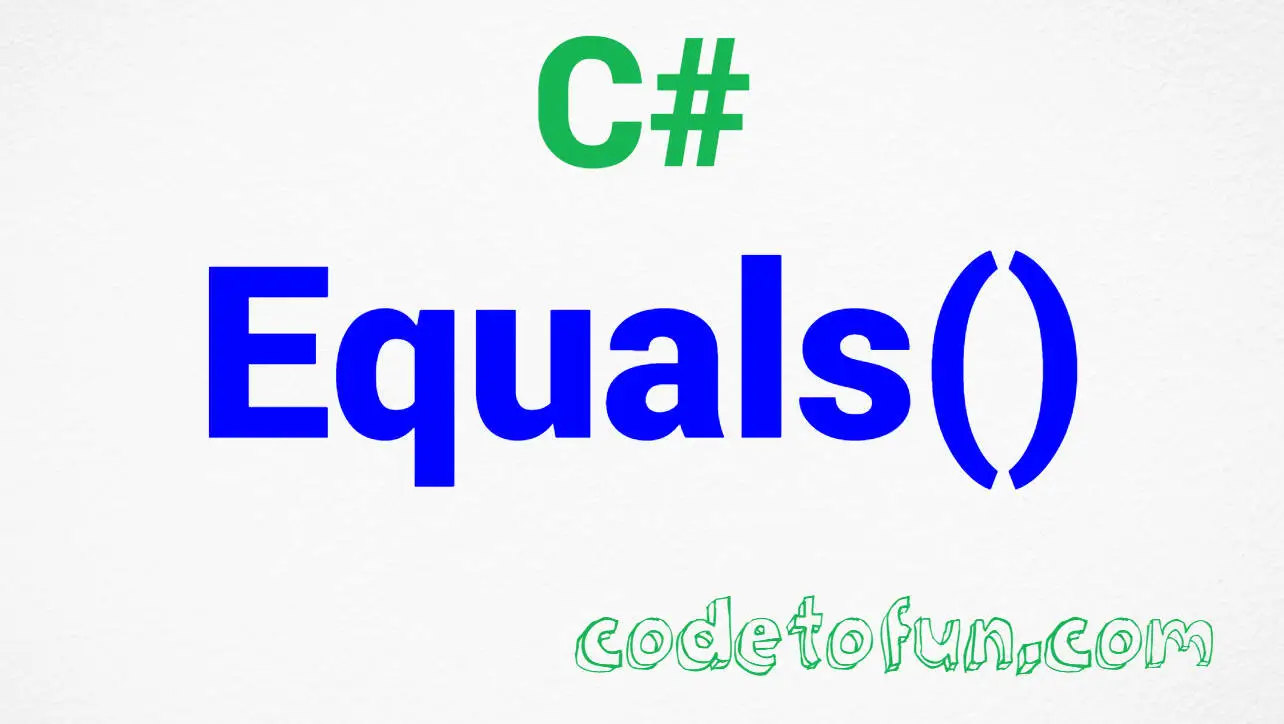
Photo Credit to CodeToFun
đ Introduction
In C# programming, string comparison is a common operation, and the Equals()
method is a fundamental tool for comparing strings for equality.
The Equals()
method is a member of the System.String class and is used to determine whether two strings have the same value.
In this tutorial, we'll explore the usage and functionality of the Equals()
method in C#.
đĄ Syntax
The syntax for the Equals()
method is as follows:
public bool Equals(string value);
The method is a member of the System.String class and takes another string as a parameter. It returns a boolean value indicating whether the two strings are equal.
đ Example
Let's dive into an example to illustrate how the Equals()
method works.
using System;
class Program {
static void Main() {
string firstString = "Hello, C#!";
string secondString = "Hello, C#!";
string thirdString = "Greetings!";
// Compare firstString and secondString
bool isEqual1 = firstString.Equals(secondString);
Console.WriteLine($"Are firstString and secondString equal? {isEqual1}"); // Output: true
// Compare firstString and thirdString
bool isEqual2 = firstString.Equals(thirdString);
Console.WriteLine($"Are firstString and thirdString equal? {isEqual2}"); // Output: false
}
}
đģ Testing the Program
Are firstString and secondString equal? True Are firstString and thirdString equal? False
đ§ How the Program Works
In this example, the Equals()
method is used to compare two pairs of strings and determine whether they are equal.
âŠī¸ Return Value
The Equals()
method returns a boolean value (true or false) indicating whether the specified string is equal to the current string.
đ Common Use Cases
The Equals()
method is useful when you need to perform case-sensitive or case-insensitive string comparisons and determine if two strings have the same content. It's commonly employed in scenarios where precise string equality is required.
đ Notes
By default, the
Equals()
method performs a case-sensitive comparison. To perform a case-insensitive comparison, you can use an overload of the method that accepts a StringComparison parameter.StringComparisonCopiedbool isEqualIgnoreCase = firstString.Equals(secondString, StringComparison.OrdinalIgnoreCase);
đĸ Optimization
The Equals()
method is optimized for performance and should be the preferred choice for string comparisons.
If you are comparing strings for equality multiple times and are concerned about performance, consider using the == operator, which is also optimized by the C# compiler.
đ Conclusion
The Equals()
method in C# is a fundamental tool for comparing strings for equality.
Whether you need case-sensitive or case-insensitive comparisons, the Equals()
method provides a reliable means of determining if two strings have the same content.
Feel free to experiment with different strings and explore the behavior of the Equals()
method in various comparison scenarios. Happy coding!
đ¨âđģ Join our Community:
Author
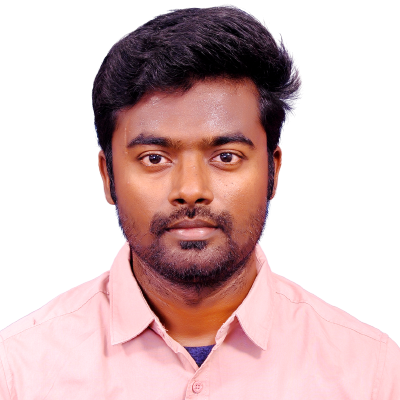
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C# String Equals() Method), please comment here. I will help you immediately.