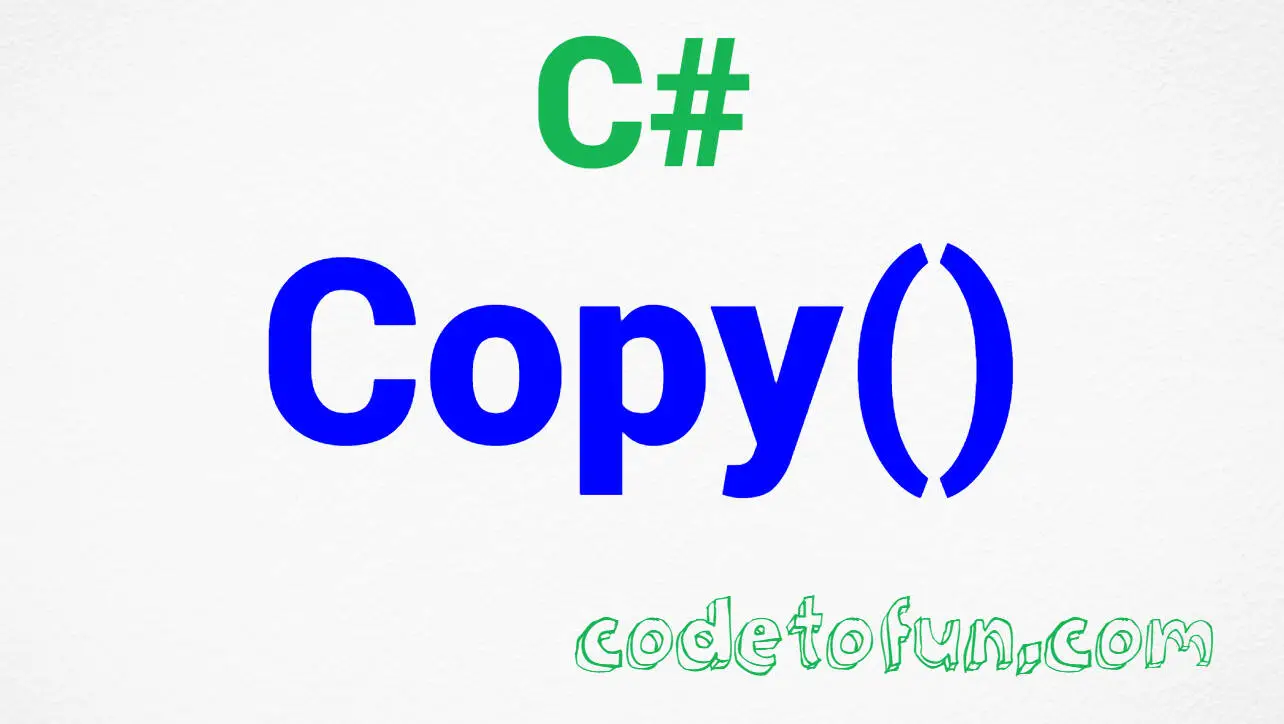
C# Basic
C# String CopyTo() Method
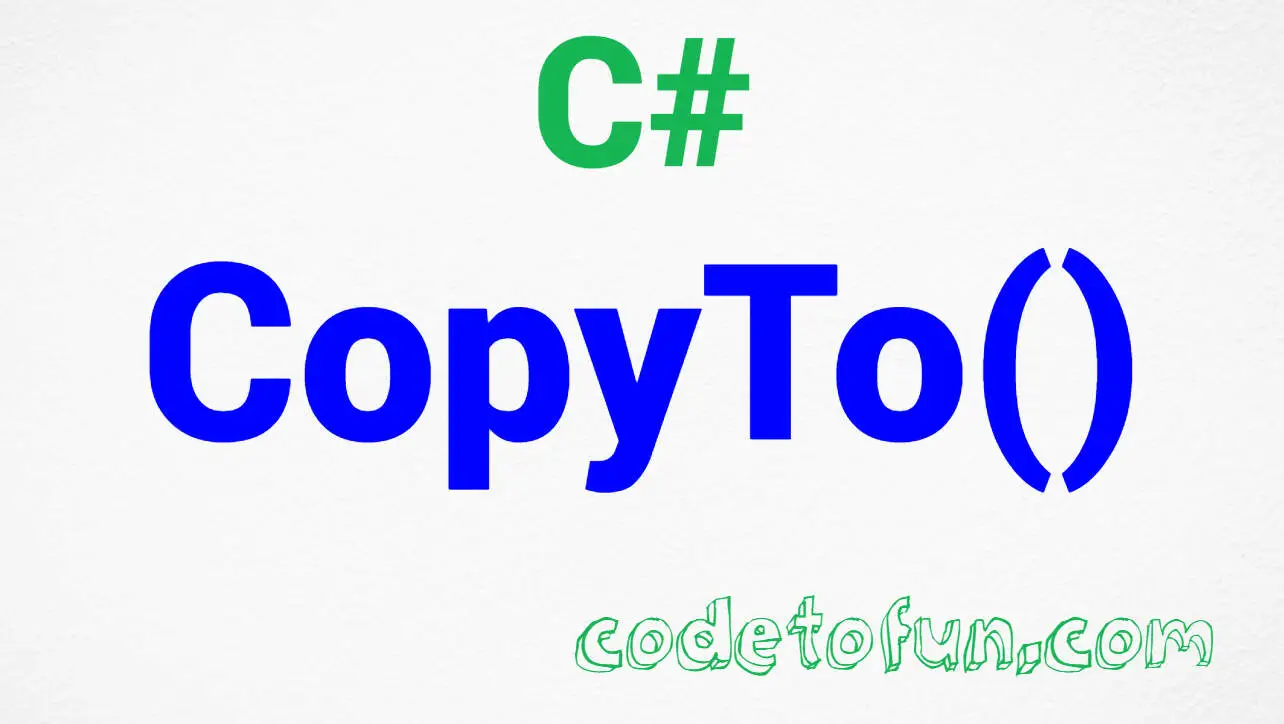
Photo Credit to CodeToFun
đ Introduction
In C# programming, the System.String class provides various methods for string manipulation.
The CopyTo()
method is one such method that allows you to copy the characters of the string to a character array.
In this tutorial, we'll explore the usage and functionality of the CopyTo()
method in C#.
đĄ Syntax
The syntax for the CopyTo()
method is as follows:
public void CopyTo(int sourceIndex, char[] destination, int destinationIndex, int count);
- sourceIndex: The starting index of the string from which to begin copying characters.
- destination: The character array to which characters are copied.
- destinationIndex: The starting index in the destination array where copying begins.
- count: The number of characters to copy.
đ Example
Let's dive into an example to illustrate how the CopyTo()
method works.
using System;
class Program {
static void Main() {
string sampleString = "C# is powerful!";
// Create a character array with enough space
char[] charArray = new char[sampleString.Length];
// Copy characters from the string to the array
sampleString.CopyTo(0, charArray, 0, sampleString.Length);
// Output the copied characters
Console.WriteLine("Copied Characters:");
Console.WriteLine(charArray);
}
}
đģ Testing the Program
Copied Characters: C# is powerful!
đ§ How the Program Works
In this example, the CopyTo()
method is used to copy all characters from the string "C# is powerful!" to a character array, and the result is then printed.
âŠī¸ Return Value
The CopyTo()
method does not return a value (void).
đ Common Use Cases
The CopyTo()
method is useful when you need to manipulate or process the characters of a string individually. Copying characters to an array allows for more flexible handling, such as modification or analysis of specific characters.
đ Notes
- The method throws ArgumentNullException if the destination array is null.
- It throws ArgumentOutOfRangeException if any of the indices or the count is outside the valid range.
đĸ Optimization
The CopyTo()
method is optimized for efficient character copying. Ensure that the destination array has sufficient space for the specified number of characters to avoid exceptions.
đ Conclusion
The CopyTo()
method in C# is a valuable tool for copying characters from a string to a character array. It provides flexibility in handling individual characters and is particularly useful when more advanced string manipulations are required.
Feel free to experiment with different source indices, destination arrays, and counts to explore the behavior of the CopyTo()
method in various scenarios. Happy coding!
đ¨âđģ Join our Community:
Author
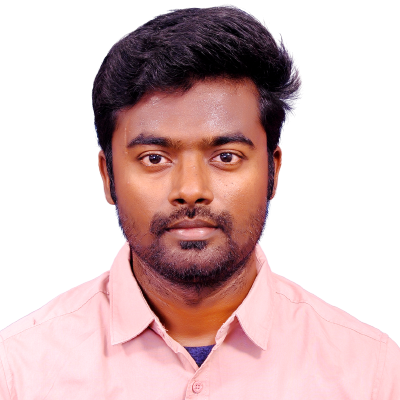
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C# String CopyTo() Method), please comment here. I will help you immediately.