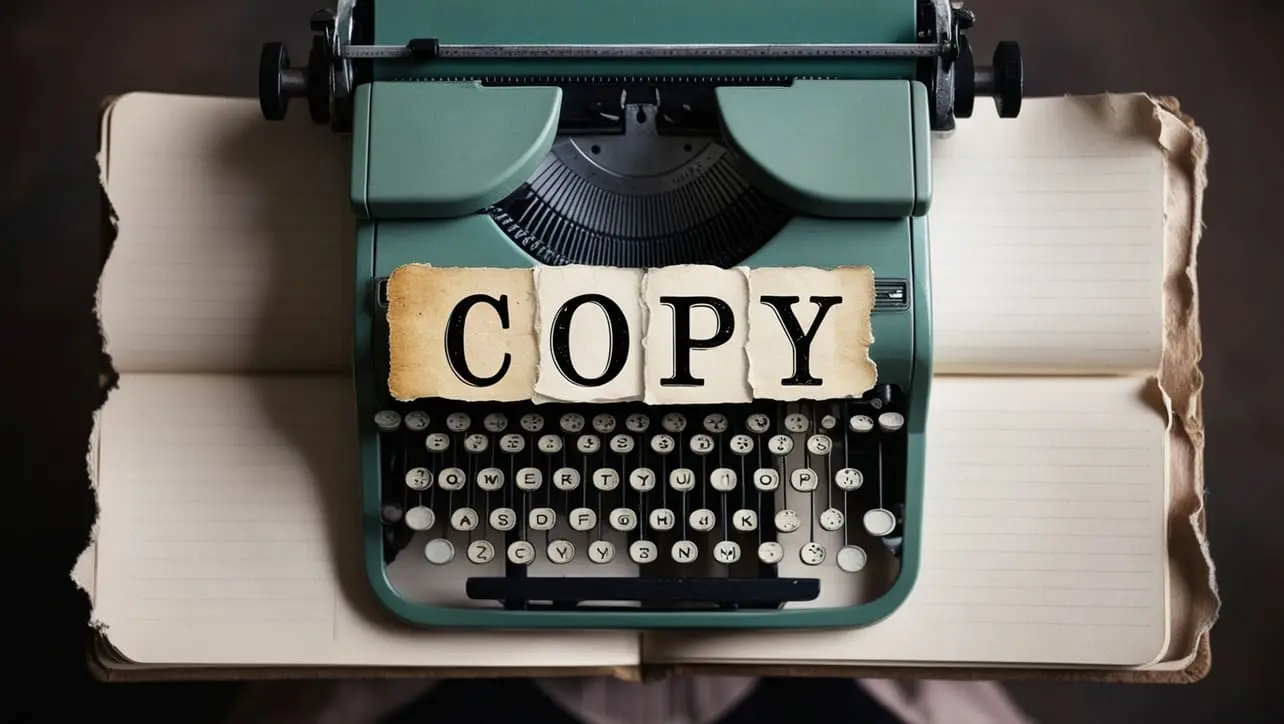
C# String Methods
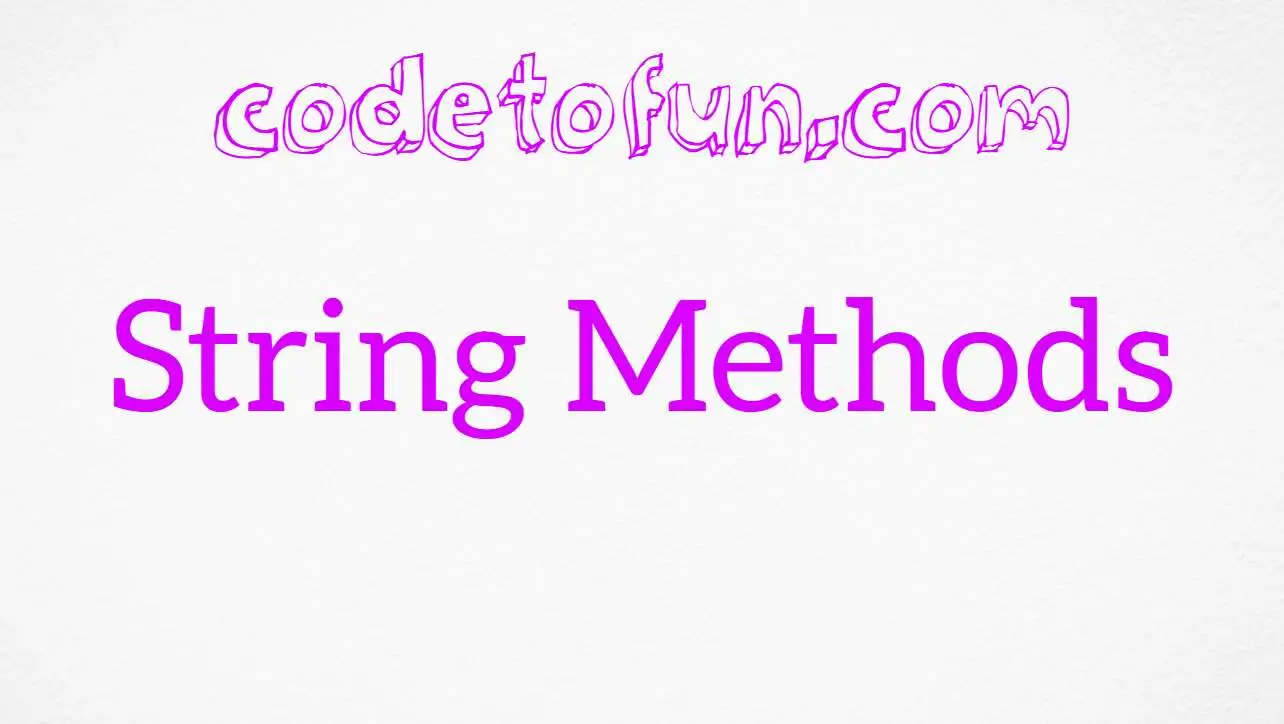
Photo Credit to CodeToFun
🙋 Introduction
C# provides a comprehensive set of built-in methods for working with strings. These methods empower developers to manipulate, analyze, and format strings efficiently.
In this reference guide, we'll explore commonly used C# string methods to enhance your understanding and utilization of strings in C#.
📑 Table of Contents
Some of the commonly used string methods in C# includes:
Methods | Explanation |
---|---|
Clone() | Creates a shallow copy of the string, providing a new reference to the same content. |
Compare() | Used to compare two strings based on their lexicographical order. |
CompareOrdinal() | Compare two strings using ordinal (binary) comparison, returning an integer that indicates their relative position in lexicographical order. |
CompareTo() | Compare the current string with another string, providing a numeric indication of their relative order in lexicographic (dictionary) comparison. |
Concat() | Used to concatenate two or more strings and returns a new string containing the combined text. |
Contains() | Checks if a specific substring is present within a given string and returns a boolean value indicating the result. |
Copy() | Used to create a new string with the same content as the original string. |
CopyTo() | Copies a specified number of characters from the current string to a character array, starting at a specified position in the array. |
EndsWith() | Determine whether a string instance ends with a specified suffix and returns a boolean value indicating the result. |
Equals() | Determine whether two strings have the same content, returning true if they are equal and false otherwise. |
Format() | Used to create formatted strings by replacing placeholders (specified by curly braces {}) with the values of corresponding arguments. |
GetEnumerator() | Returns an enumerator that iterates through each character in the string, facilitating sequential access for enumeration or iteration purposes. |
GetHashCode() | Returns a unique numeric hash code representing the content of the string, facilitating efficient data retrieval and comparison in hash-based data structures. |
GetType() | Used to obtain the runtime type information of an instance of a string, returning a Type object representing its class. |
GetTypeCode() | Returns the TypeCode enumeration value corresponding to the underlying type of the string, indicating whether it represents a numeric, string, or other type. |
🚀 Usage Tips
Enhance your C# string manipulation skills with these tips:
- StringBuilder: For extensive string manipulation, consider using the StringBuilder class to improve performance.
- String Interpolation: Leverage C#'s string interpolation feature for cleaner and more readable string formatting.
- Exception Handling: Be cautious with methods that can throw exceptions; consider implementing appropriate error handling.
- Globalization: Some methods have overloads that allow you to specify culture and case sensitivity, providing flexibility for globalization scenarios.
📄 Example
Let's explore a practical example of using C# string methods to manipulate a string:
using System;
class Program {
static void Main() {
// Example: Formatting a string using C# string methods
string originalString = "hello world";
string formattedString = originalString.Replace("hello", "greetings").ToUpper();
Console.WriteLine($"Original String: {originalString}");
Console.WriteLine($"Formatted String: {formattedString}");
}
}
💻 Testing the Program
Original String: hello world Formatted String: GREETINGS WORLD
🧠 How the Program Works
This example demonstrates using the Replace and ToUpper methods to transform the original string. Experiment with different methods and discover the power of C# string manipulation.
🎉 Conclusion
This reference guide offers an overview of essential C# string methods. Incorporating these methods into your code will empower you to efficiently handle and manipulate strings. Experiment with these methods to enhance your C# programming skills. For more in-depth information, consult the official Microsoft documentation.
👨💻 Join our Community:
Author
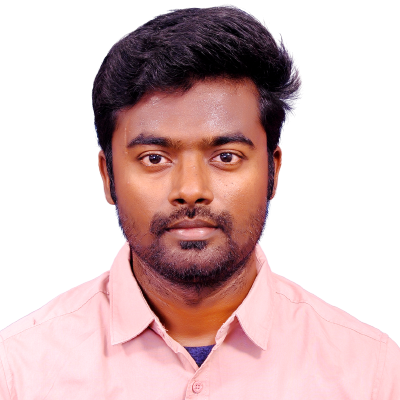
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C# String Methods), please comment here. I will help you immediately.