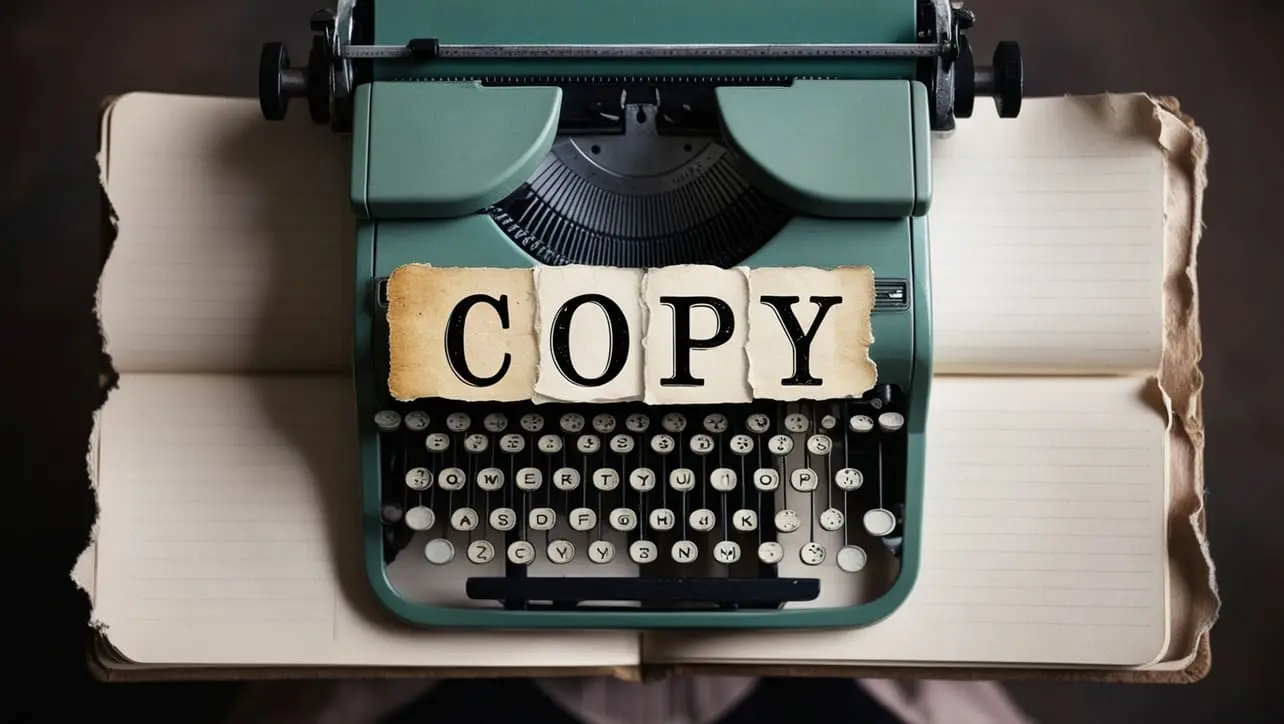
C# String EndsWith() Method
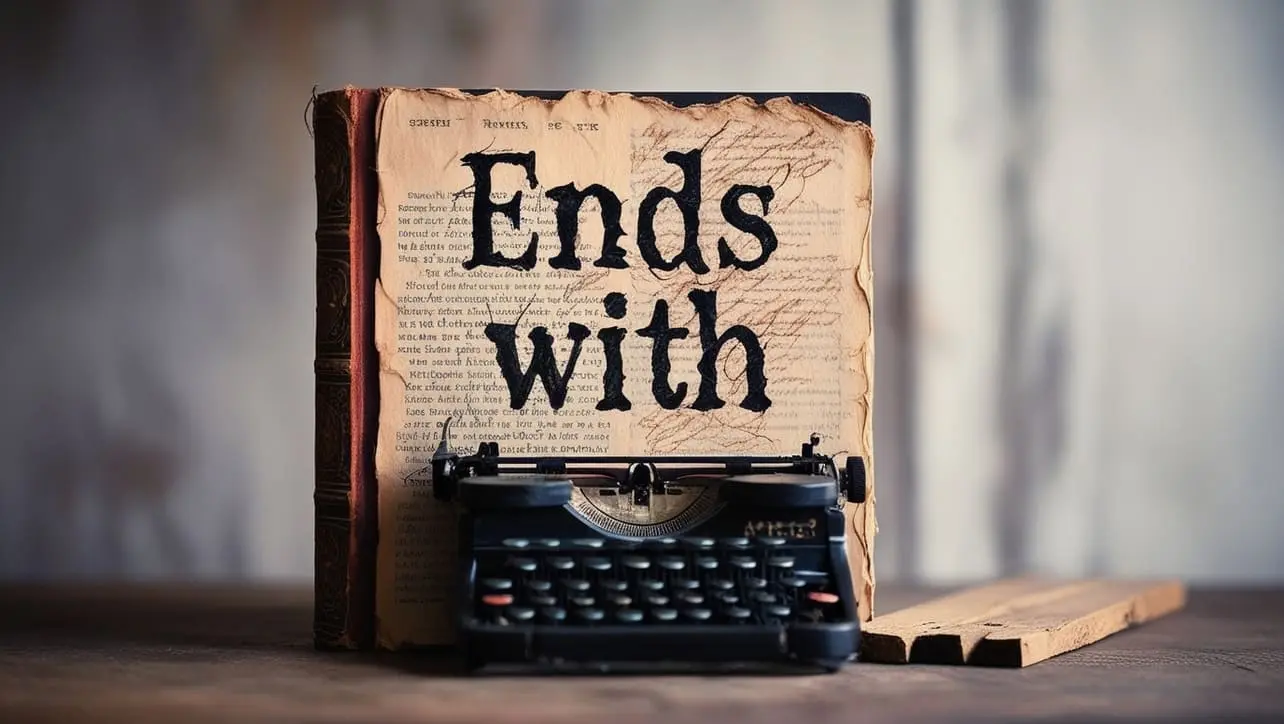
Photo Credit to CodeToFun
đ Introduction
In C# programming, working with strings is a common and essential task.
The EndsWith()
method is a member of the System.String class and is used to determine whether the end of the string instance matches a specified substring.
In this tutorial, we'll explore the usage and functionality of the EndsWith()
method in C#.
đĄ Syntax
The syntax for the EndsWith()
method is as follows:
public bool EndsWith(string value);
- value: The substring to compare.
The method returns a bool value, indicating whether the string ends with the specified substring.
đ Example
Let's dive into examples to illustrate how the EndsWith()
method works.
using System;
class Program {
static void Main() {
string sampleString = "Hello, World";
// Check if the string ends with "World"
bool endsWithCSharp = sampleString.EndsWith("World");
Console.WriteLine($"Example 1: {endsWithCSharp}");
// case-insensitive check
bool endsWithWorld = sampleString.EndsWith("world", StringComparison.OrdinalIgnoreCase);
Console.WriteLine($"Example 2: {endsWithWorld}");
}
}
đģ Testing the Program
Example 1: True Example 2: True
đ§ How the Program Works
In Example 1, the EndsWith()
method is used to check if the string "Hello, World" ends with the specified substring World.
In Example 2, a case-insensitive check is performed to see if the string ends with the substring world.
âŠī¸ Return Value
The EndsWith()
method returns true if the string instance ends with the specified substring; otherwise, it returns false.
đ Common Use Cases
The EndsWith()
method is particularly useful when you need to perform conditional logic based on the ending characters of a string. It's commonly used in scenarios where you want to check file extensions, domain names, or specific patterns.
đ Notes
- The comparison is case-sensitive by default, but you can use overloads that allow you to specify whether the comparison should be case-insensitive.
- If the specified substring is an empty string, the method returns true.
đĸ Optimization
The EndsWith()
method is optimized for efficiency, and no specific optimizations are typically needed. If you're performing multiple checks with the same substring, consider storing the result in a variable to avoid redundant computations.
đ Conclusion
The EndsWith()
method in C# provides a straightforward way to check whether a string ends with a specific substring. It's a valuable tool for string manipulation tasks and conditional logic.
Feel free to experiment with different strings and substrings to deepen your understanding of the EndsWith()
method. Happy coding!
đ¨âđģ Join our Community:
Author
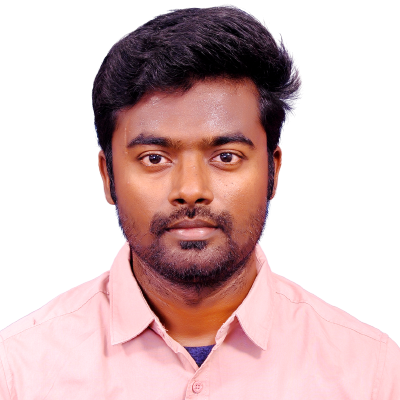
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C# String EndsWith() Method), please comment here. I will help you immediately.