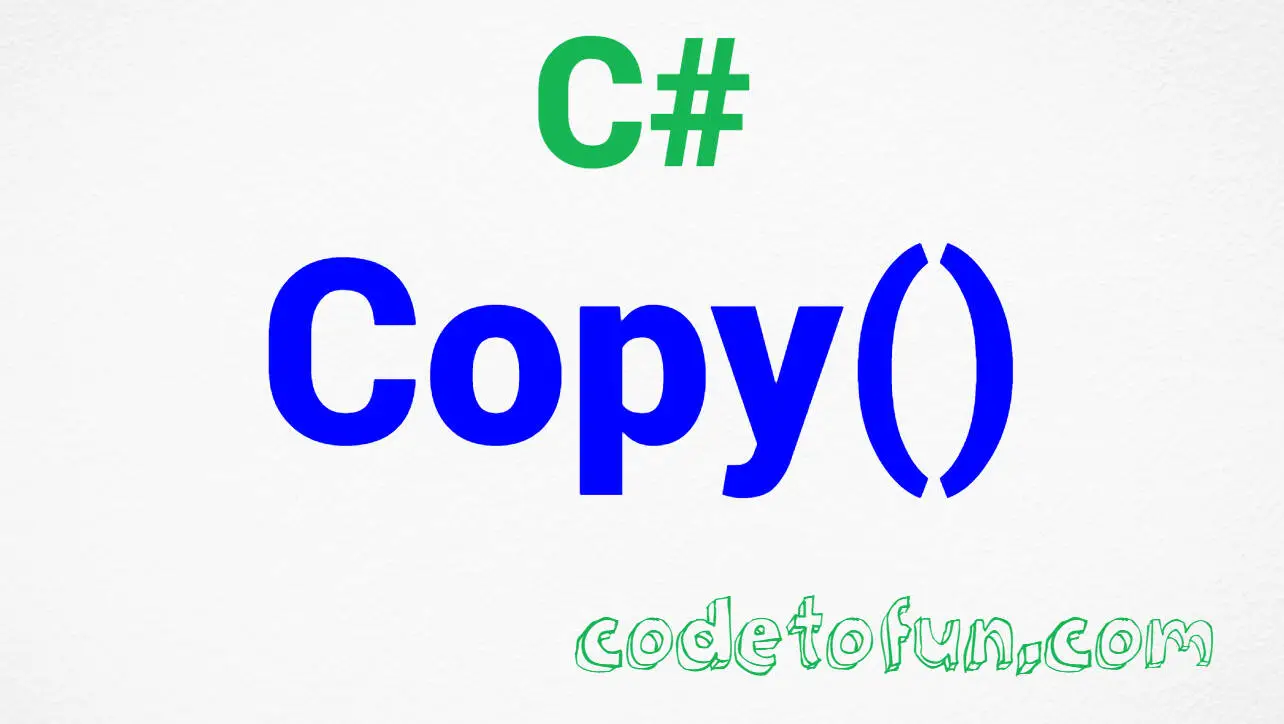
C# Basic
C# String Concat() Method
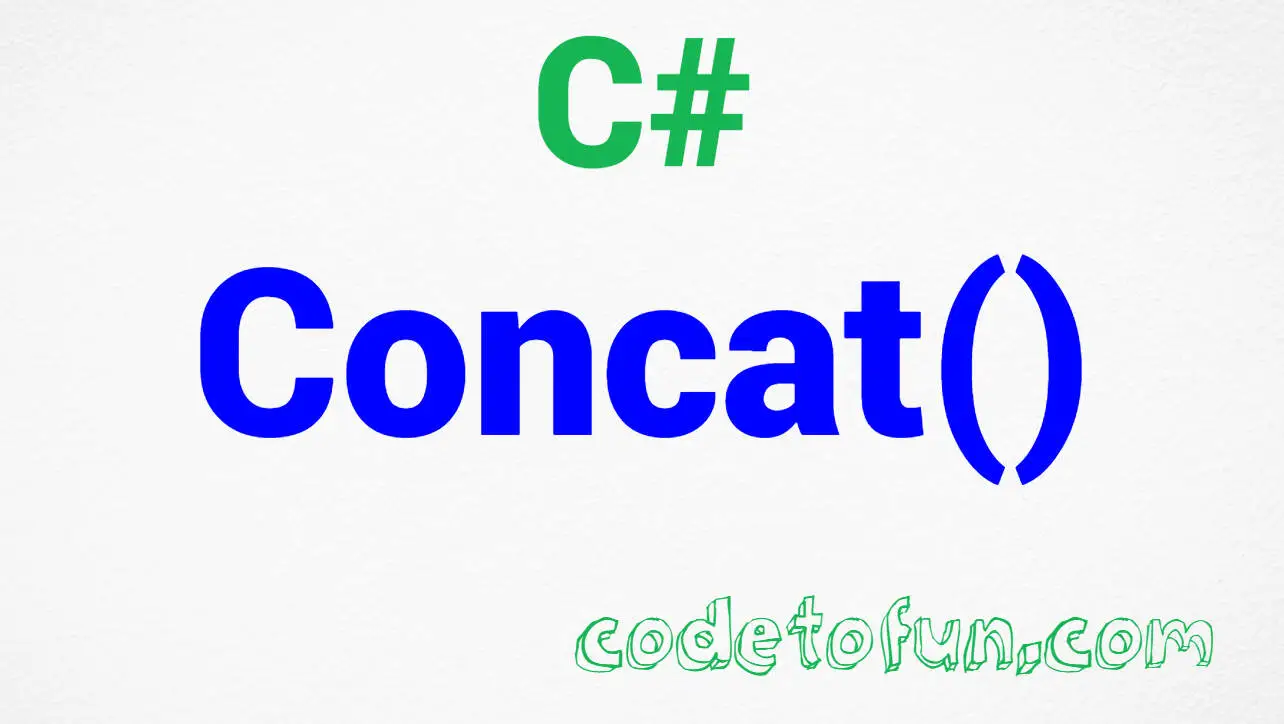
Photo Credit to CodeToFun
đ Introduction
In C# programming, strings are essential for handling and manipulating text.
The Concat()
method is a fundamental string method provided by the System.String class. It is used for concatenating multiple strings into a single string.
In this tutorial, we'll explore the usage and functionality of the Concat()
method in C#.
đĄ Syntax
The syntax for the Concat()
method is as follows:
public static string Concat(string str0, string str1, ...);
The method is a member of the System.String class and is declared as a static method. It takes one or more string parameters and returns a new string that is the result of concatenating the input strings.
đ Example
Let's dive into an example to illustrate how the Concat()
method works.
using System;
class Program {
static void Main() {
string firstName = "John";
string lastName = "Doe";
// Concatenate strings
string fullName = string.Concat(firstName, " ", lastName);
// Output the result
Console.WriteLine($"Full Name: {fullName}");
}
}
đģ Testing the Program
Full Name: John Doe
đ§ How the Program Works
In this example, the Concat()
method is used to concatenate the firstName and lastName strings, separated by a space. The result is then printed.
âŠī¸ Return Value
The Concat()
method returns a new string that is the result of concatenating the input strings.
đ Common Use Cases
The Concat()
method is useful when you need to combine multiple strings into a single string. It is commonly used to build formatted strings, construct messages, or concatenate variable values with fixed text.
đ Notes
- The
Concat()
method can concatenate not only strings but also other data types. It automatically converts non-string arguments to strings before concatenation. - For large-scale concatenation operations, consider using the StringBuilder class, which provides better performance.
đĸ Optimization
While the Concat()
method is efficient for a small number of concatenations, for scenarios involving a large number of concatenations in a loop, consider using StringBuilder for improved performance and memory efficiency.
using System;
using System.Text;
class Program {
static void Main() {
StringBuilder resultBuilder = new StringBuilder();
for (int i = 0; i < 1000; i++) {
resultBuilder.Append($"Value {i}, ");
}
string result = resultBuilder.ToString();
Console.WriteLine(result);
}
}
đ Conclusion
The Concat()
method in C# is a straightforward and effective way to concatenate strings. It plays a crucial role in string manipulation and is widely used in various scenarios.
Feel free to experiment with different strings and explore the behavior of the Concat()
method in different concatenation scenarios. Happy coding!
đ¨âđģ Join our Community:
Author
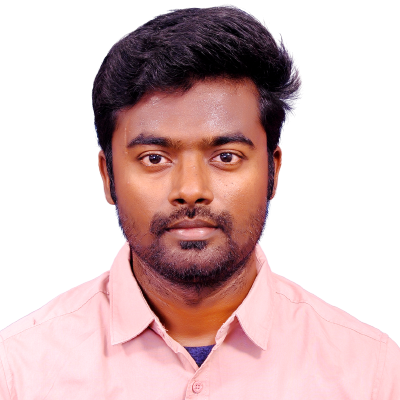
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C# String Concat() Method), please comment here. I will help you immediately.