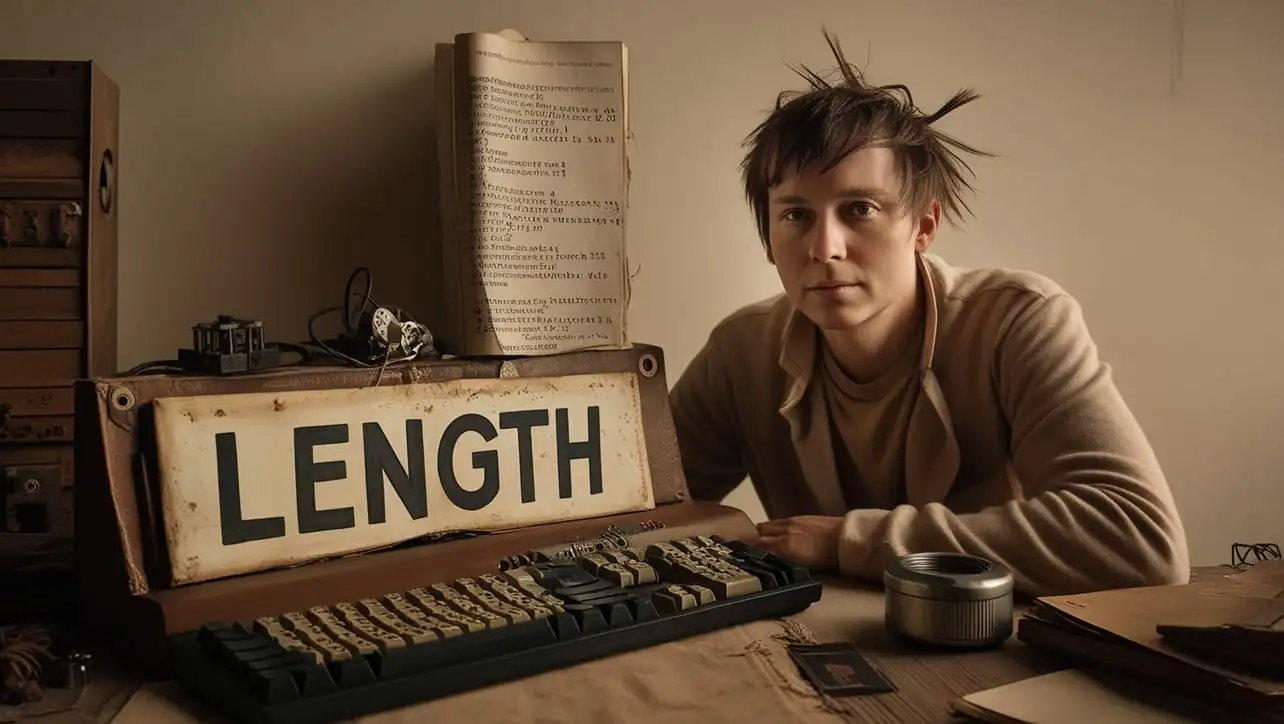
JS String Properties
JavaScript String length
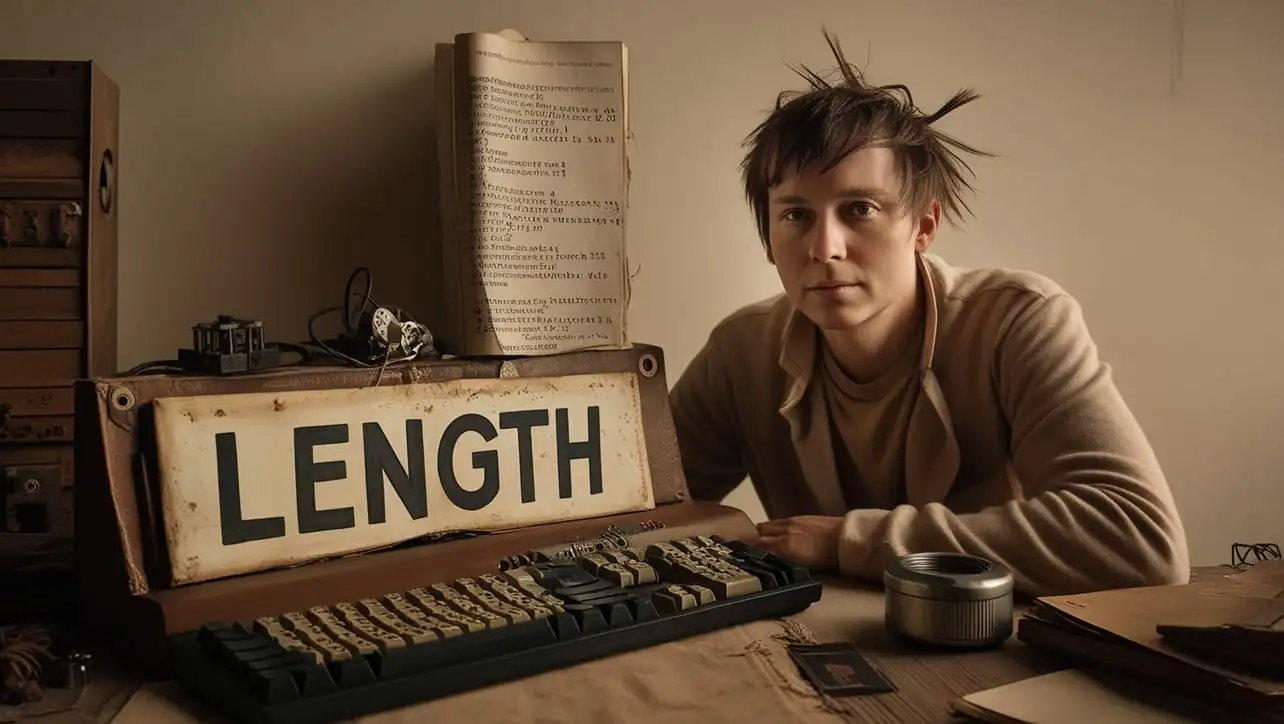
Photo Credit to CodeToFun
Introduction
The length
property in JavaScript is a built-in property that returns the number of characters in a string.
It's a simple and effective way to determine the size of a string, making it useful for tasks like input validation, string manipulation, and more.
Understanding the length Property
The length
property is an integer that reflects the number of 16-bit units (or characters) present in a string. This includes letters, digits, symbols, whitespace, and any special characters, with each counting as a single unit.
const str = "Hello, World!";
console.log(str.length); // Output: 13
How to Use the length Property
Using the length
property is straightforward. It can be accessed directly on any string and is often used to determine if a string meets a specific length requirement.
const password = "mypassword";
if (password.length >= 8) {
console.log("Password length is sufficient.");
} else {
console.log("Password is too short.");
}
Common Use Cases
- Input Validation: Check if user input meets required length criteria.
- Substring Extraction: Determine how much of a string to extract or manipulate.
- Looping Over Characters: Use
length
in a loop to iterate over each character in a string. - Truncating Strings: Shorten strings that exceed a specific length.
Important Considerations
Multibyte Characters:
length
counts UTF-16 code units, so characters like emojis or special symbols that use surrogate pairs may not be counted accurately. For instance:JavaScriptCopiedconst emoji = "π"; console.log(emoji.length); // Output: 2
Whitespace:
Spaces, tabs, and newline characters all contribute to a string's
length
.Immutable Property:
The
length
property is read-only. You cannot directly change the length of a string as strings in JavaScript are immutable.
Example
Hereβs a basic example that demonstrates how to use length
to create a character counter for a text input:
<!DOCTYPE html>
<html>
<head>
<title>String Length Example</title>
</head>
<body>
<h1>Character Counter</h1>
<textarea id="textInput" rows="4" cols="50"></textarea>
<p id="charCount">Characters: 0</p>
<script>
const textarea = document.getElementById('textInput');
const charCount = document.getElementById('charCount');
textarea.addEventListener('input', () => {
const length = textarea.value.length;
charCount.textContent = 'Characters: ' + length;
});
</script>
</body>
</html>
Conclusion
The length
property is an essential tool for handling and manipulating strings in JavaScript. With its simple syntax and versatility, it's a go-to for many string-related tasks. Understanding its behavior, especially with multibyte characters and whitespace, will help you use it effectively in your projects.
π¨βπ» Join our Community:
Author
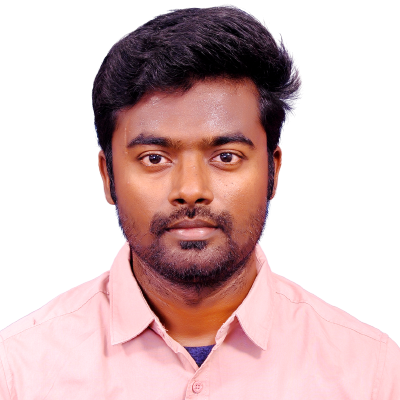
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee