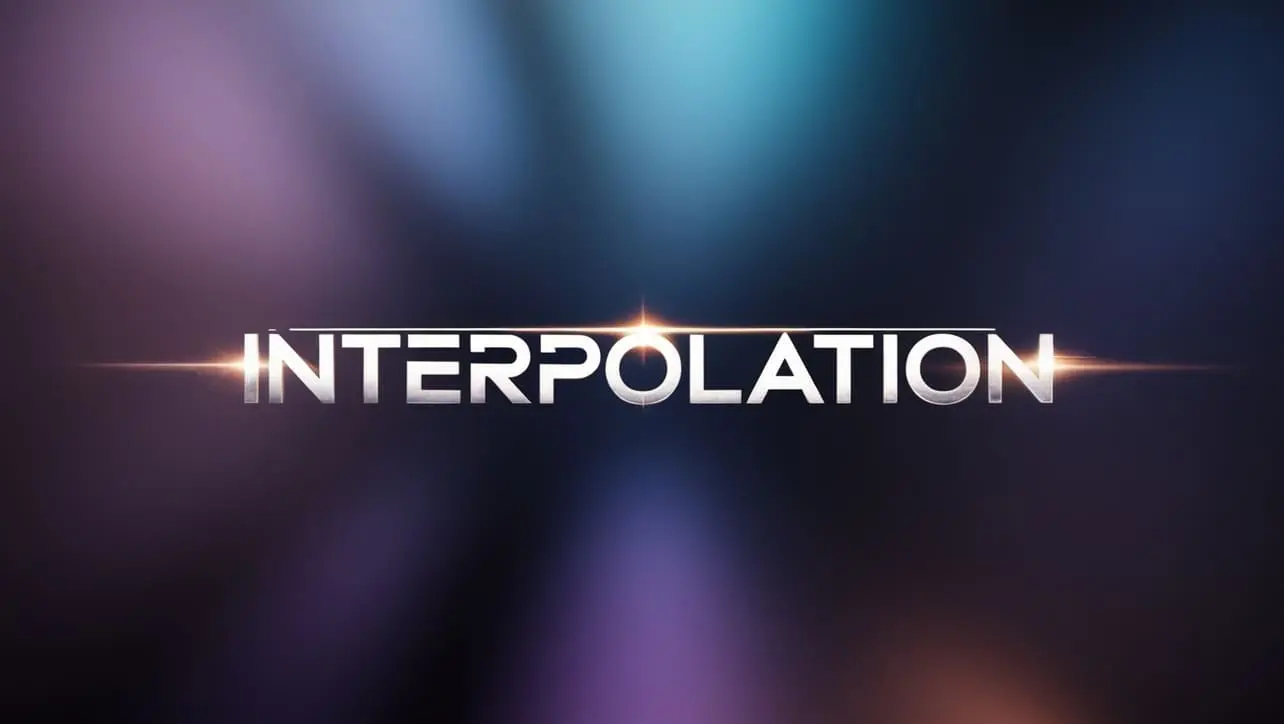
Sass at-rules
Sass @use
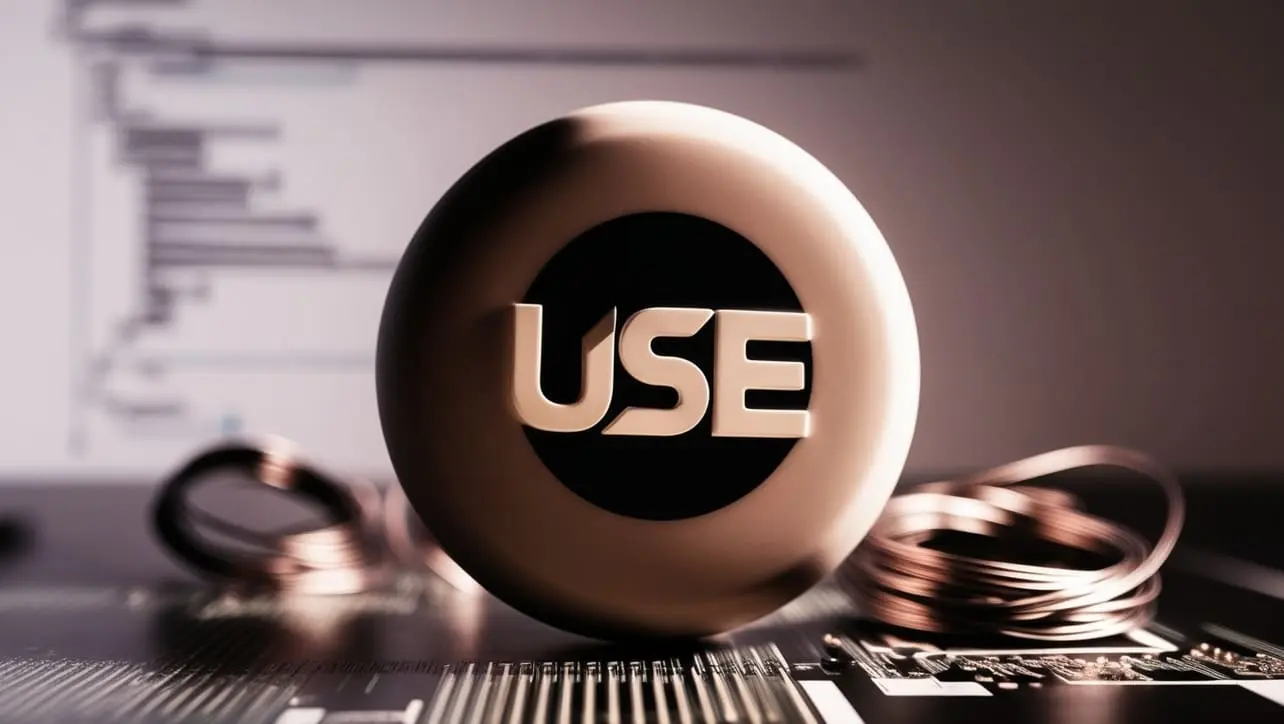
Photo Credit to CodeToFun
π Introduction
The @use
rule in Sass is a modern way to include and use styles from other Sass files. It introduces a more manageable and modular approach to organizing your stylesheets compared to the older @import rule.
The @use
rule ensures better encapsulation, avoids naming conflicts, and improves code maintainability by allowing you to load and configure Sass modules efficiently.
π‘ Syntax
The syntax for the @use
rule is as follows:
@use 'path/to/module' [as namespace];
π’ Parameters
- path/to/module: The relative or absolute path to the Sass file you want to use.
- namespace (optional): A namespace for the module to avoid naming conflicts and clarify which module a particular style or variable belongs to.
β©οΈ Return Value
The @use
rule doesnβt return a value. Instead, it makes the styles, variables, mixins, and functions from the specified module available in the current stylesheet.
π Example Usage
Let's look at some practical examples to see how the @use
rule can be implemented.
π Example 1: Basic Usage
Consider you have a Sass file named _colors.scss with the following content:
// _colors.scss
$primary-color: #3498db;
$secondary-color: #2ecc71;
You can use these variables in another Sass file with the @use
rule:
// styles.scss
@use 'colors';
body {
background-color: colors.$primary-color;
color: colors.$secondary-color;
}
In this example, the @use
rule loads the _colors.scss module, and the variables are accessed with the colors namespace.
π Example 2: Using a Custom Namespace
You can also define a custom namespace to make the code clearer:
// styles.scss
@use 'colors' as myColors;
body {
background-color: myColors.$primary-color;
color: myColors.$secondary-color;
}
Here, colors is given the namespace myColors, allowing you to refer to its variables with myColors.
π Example 3: Configuring Modules
Modules can be configured using the @use
rule to set variables directly:
// _buttons.scss
$button-color: #2980b9 !default;
@mixin button-style {
background-color: $button-color;
}
// styles.scss
@use 'buttons' with ($button-color: #e74c3c);
.button {
@include buttons.button-style;
}
In this example, the @use
rule is used with the with keyword to configure the $button-color variable, overriding its default value.
π Conclusion
The @use
rule in Sass is a powerful feature that enhances the modularity and organization of your stylesheets. By adopting @use
, you can improve code maintainability, avoid naming conflicts, and manage your styles more efficiently.
The @use
rule not only simplifies how you include and configure Sass modules but also encourages a more structured approach to writing and maintaining CSS. Whether you're working on a small project or a large-scale application, leveraging @use
can significantly streamline your styling process and enhance the overall quality of your codebase.
Experiment with @use
in your projects to see how it can improve your Sass workflow and contribute to cleaner, more organized stylesheets.
π¨βπ» Join our Community:
Author
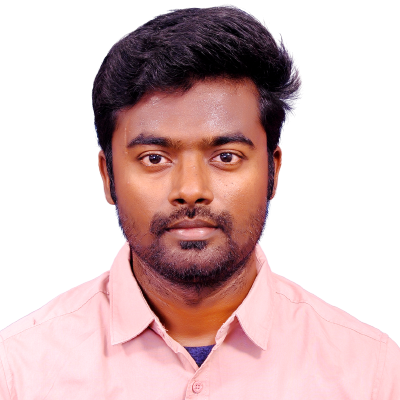
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Sass @use), please comment here. I will help you immediately.