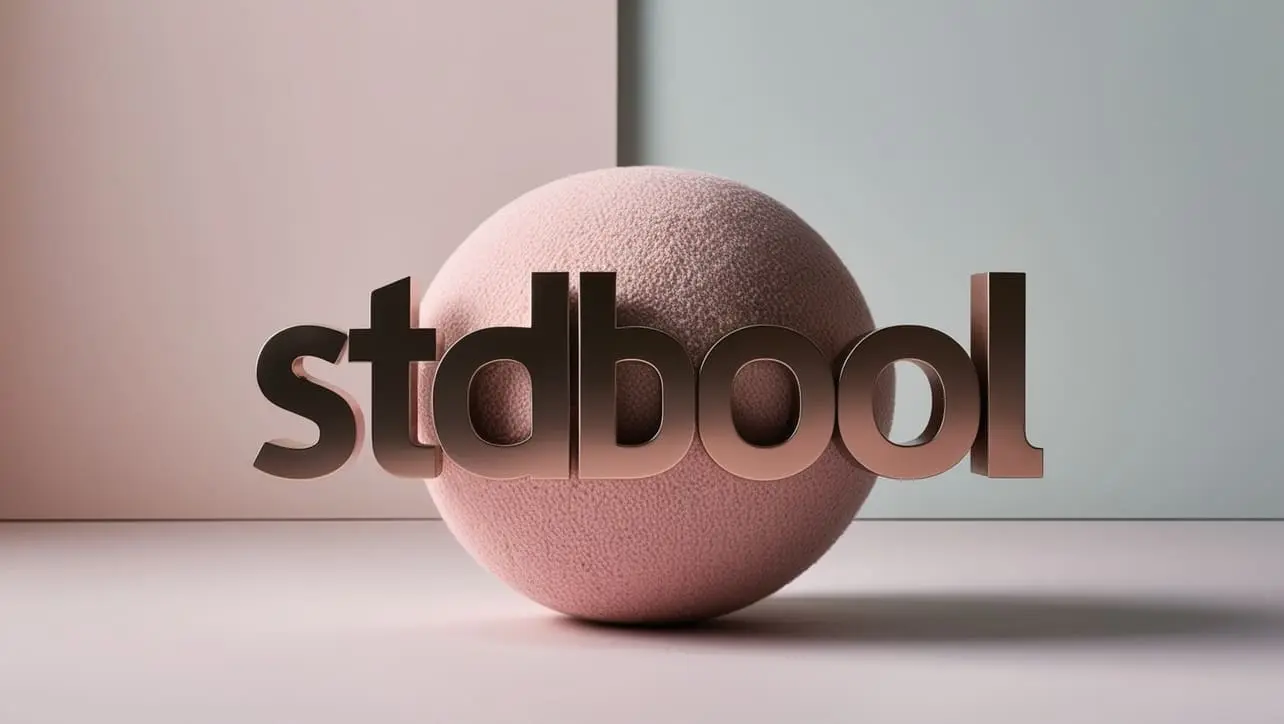
C Header Files
C Library – wctype.h
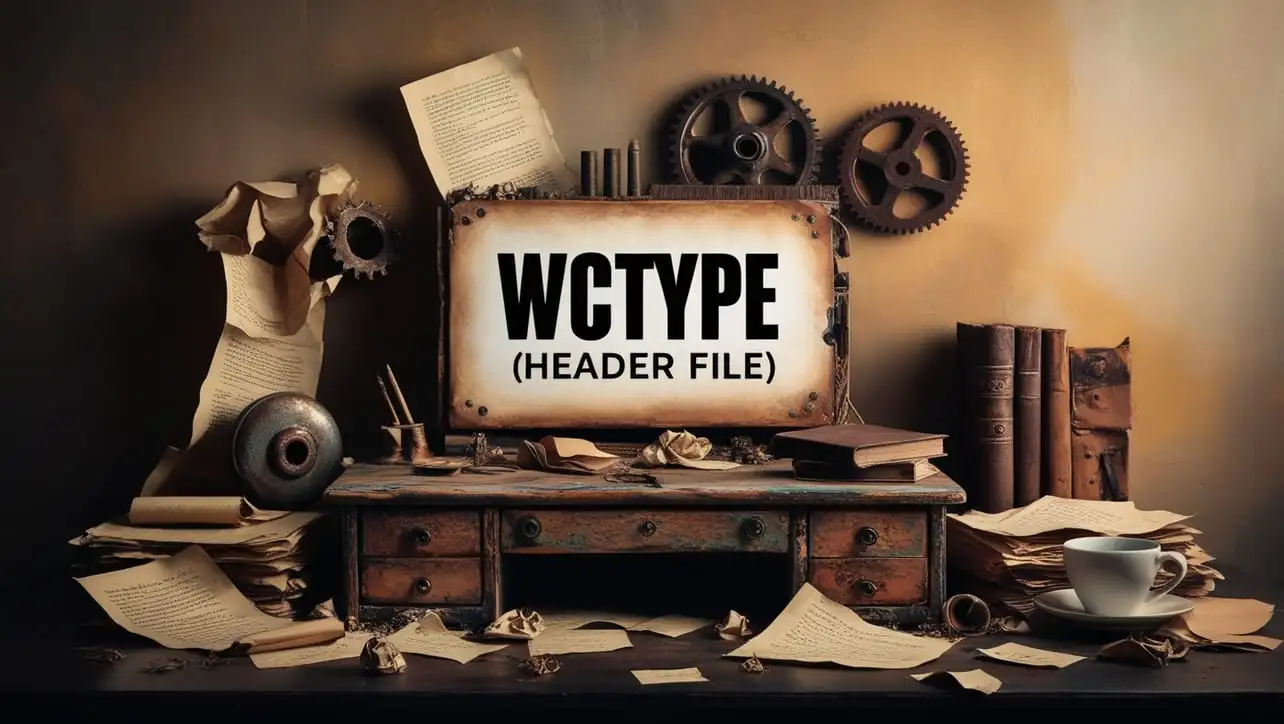
Photo Credit to CodeToFun
🙋 Introduction
The wctype.h
header in C provides a set of functions and macros for classifying and mapping wide characters. These functions are the wide character equivalents of the functions in ctype.h and are essential when working with wide characters and internationalization in C programs. Wide characters are typically used to represent characters from large character sets, such as Unicode.
💡 Syntax
To use the wctype.h
library, you need to include it in your program:
#include <wctype.h>
📘 Functions Defined in wctype.h
The wctype.h
header defines several functions for character classification and conversion. Here are some commonly used functions:
Character Classification Functions:
iswalpha: Checks if a wide character is an alphabetic letter.
example.cCopiedint iswalpha(wint_t wc);
iswdigit: Checks if a wide character is a decimal digit.
example.cCopiedint iswdigit(wint_t wc);
iswspace: Checks if a wide character is a whitespace character.
example.cCopiedint iswspace(wint_t wc);
iswpunct: Checks if a wide character is a punctuation character.
example.cCopiedint iswpunct(wint_t wc);
🔄 Character Conversion Functions
towlower: Converts a wide character to its lowercase equivalent.
example.cCopiedwint_t towlower(wint_t wc);
towupper: Converts a wide character to its uppercase equivalent.
example.cCopiedwint_t towupper(wint_t wc);
📄 Example
Below is a simple example demonstrating how to use the wctype.h
functions to classify and convert wide characters.
#include <stdio.h>
#include <wctype.h>
#include <wchar.h>
int main() {
wchar_t wc = L'Ä';
// Check if the character is alphabetic
if (iswalpha(wc)) {
wprintf(L"%lc is an alphabetic character\n", wc);
} else {
wprintf(L"%lc is not an alphabetic character\n", wc);
}
// Convert the character to lowercase
wchar_t lower_wc = towlower(wc);
wprintf(L"Lowercase of %lc is %lc\n", wc, lower_wc);
// Convert the character to uppercase
wchar_t upper_wc = towupper(lower_wc);
wprintf(L"Uppercase of %lc is %lc\n", lower_wc, upper_wc);
return 0;
}
💻 Output
? is not an alphabetic character Lowercase of ? is ? Uppercase of ? is ?
🧠 How the Program Works
- Character Initialization: A wide character wc is initialized with a character value.
- Character Classification: The iswalpha function checks if wc is an alphabetic character.
- Character Conversion: The towlower function converts wc to its lowercase equivalent, and the towupper function converts it back to its uppercase form.
🚧 Common Pitfalls
- Locale Dependence: Functions in
wctype.h
are locale-dependent, which means their behavior can change based on the current locale settings. Ensure the correct locale is set when using these functions. - Wide Character Representation: Be cautious about the representation of wide characters, especially when dealing with different character sets or encoding standards.
- Library Support: Ensure your compiler and standard library support wide characters and the
wctype.h
functions, as some older systems may have limited support.
✅ Best Practices
-
Set Locale: Always set the appropriate locale using setlocale at the beginning of your program to ensure consistent behavior of wide character functions.
example.cCopiedsetlocale(LC_ALL, "");
- Check Return Values: Always check the return values of classification and conversion functions to handle unexpected characters or errors gracefully.
- Consistent Use: Use wide character functions consistently when working with wide strings to avoid mixing wide and narrow character functions, which can lead to bugs.
🎉 Conclusion
The wctype.h
header is a vital part of the C standard library for handling wide characters and supporting internationalization in C programs.
By using the functions provided in this header, you can effectively classify and convert wide characters, enabling your programs to handle diverse character sets. However, it is crucial to be aware of common pitfalls and follow best practices to ensure your programs are robust and locale-independent.
👨💻 Join our Community:
Author
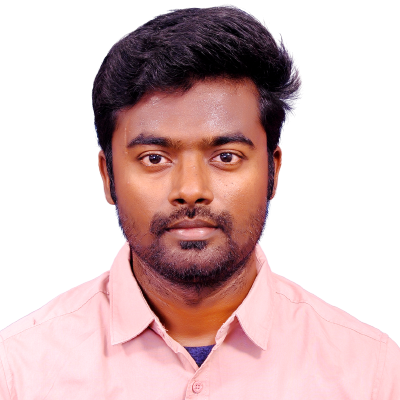
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C Library – wctype.h), please comment here. I will help you immediately.