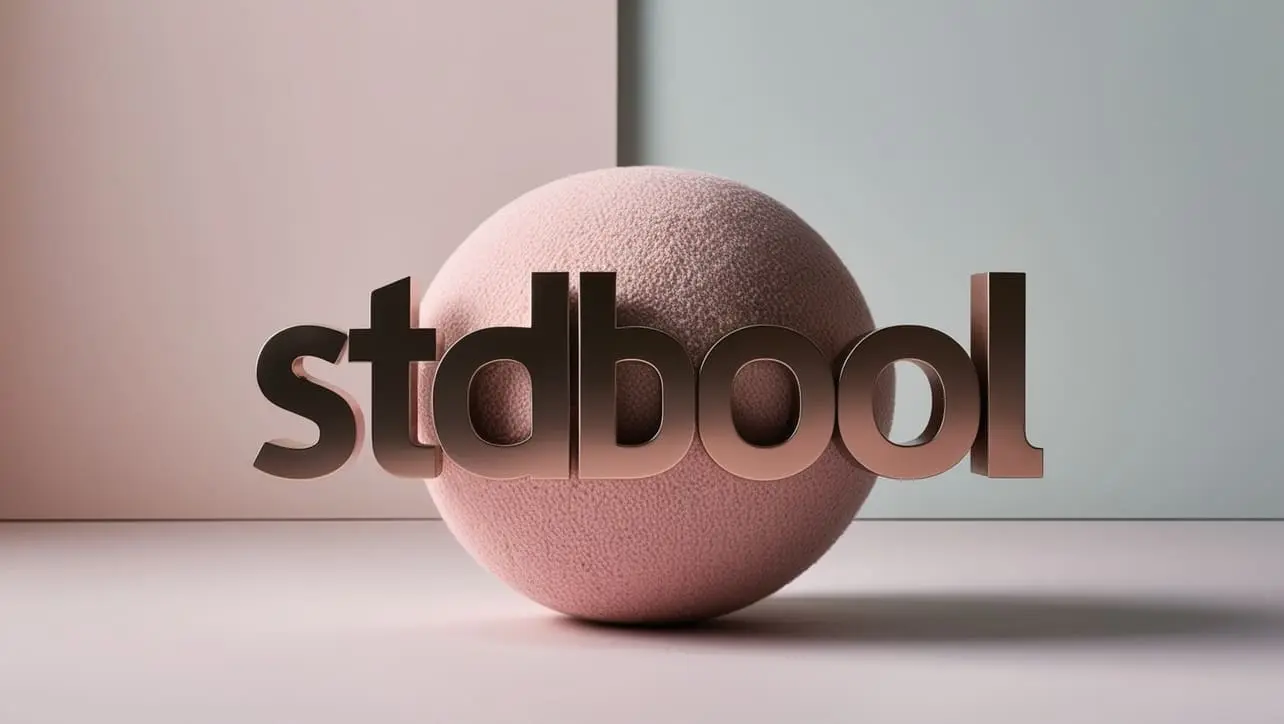
C Header Files
C Library – wchar.h
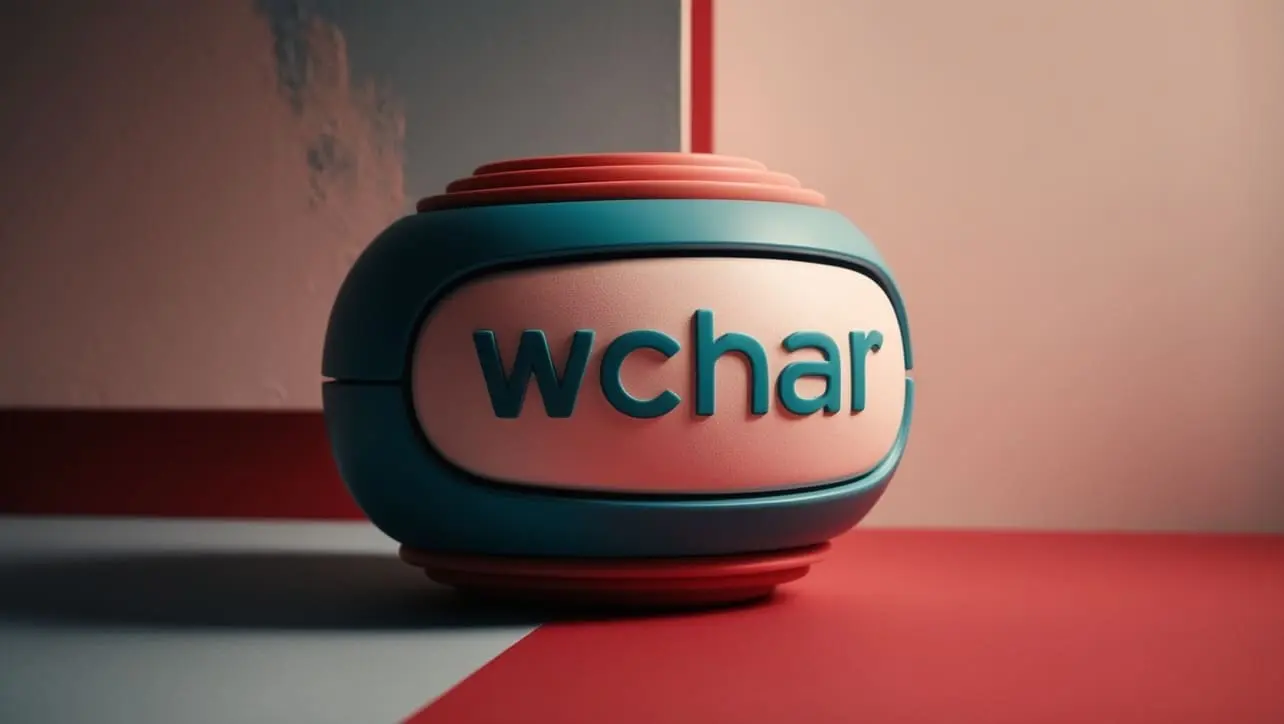
Photo Credit to CodeToFun
🙋 Introduction
The wchar.h
header in C provides facilities for manipulating wide characters and wide-character strings. Wide characters are useful for representing larger character sets, such as Unicode, which is essential for internationalization and handling multiple languages.
This header defines various data types, macros, and functions for dealing with wide characters and strings.
💡 Syntax
To use the wchar.h
library, you need to include it in your program:
#include <wchar.h>
Data Types Defined in wchar.h
The wchar.h
header defines several data types that facilitate working with wide characters:
- wchar_t: A type suitable for representing wide characters.
- mbstate_t: An object type suitable for storing conversion state information.
- size_t: An unsigned integral type.
Macros Defined in wchar.h
Some useful macros defined in wchar.h
include:
- WEOF: A macro that expands to a constant expression of type wint_t representing end-of-file.
- NULL: A macro that expands to a null pointer constant.
Functions Defined in wchar.h
The wchar.h
header defines a wide array of functions for handling wide characters and strings. Some of the most commonly used functions are:
Character Classification and Conversion Functions
iswalpha: Checks if a wide character is an alphabetic letter.
example.cCopiedint iswalpha(wint_t wc);
towlower: Converts a wide character to lowercase.
example.cCopiedwint_t towlower(wint_t wc);
Wide String Manipulation Functions
wcscpy: Copies a wide string.
example.cCopiedwchar_t *wcscpy(wchar_t *dest, const wchar_t *src);
wcslen: Computes the length of a wide string.
example.cCopiedsize_t wcslen(const wchar_t *s);
Multibyte and Wide Character Conversion Functions
mbstowcs: Converts a multibyte string to a wide-character string.
example.cCopiedsize_t mbstowcs(wchar_t *dest, const char *src, size_t n);
wcstombs: Converts a wide-character string to a multibyte string.
example.cCopiedsize_t wcstombs(char *dest, const wchar_t *src, size_t n);
📄 Example
Below is a simple example demonstrating how to use some of the functions in the wchar.h
library to handle wide strings.
#include <stdio.h>
#include <wchar.h>
int main() {
// Define a wide-character string
wchar_t wstr[] = L"Hello, World!";
// Print the wide-character string
wprintf(L"Wide String: %ls\n", wstr);
// Calculate and print the length of the wide-character string
size_t length = wcslen(wstr);
wprintf(L"Length: %zu\n", length);
// Convert the wide-character string to a multibyte string
char mbs[50];
wcstombs(mbs, wstr, sizeof(mbs));
printf("Multibyte String: %s\n", mbs);
return 0;
}
💻 Output
Wide String: Hello, World! Length: 13
🧠 How the Program Works
- Wide-character String Definition: The wstr array is defined as a wide-character string using the L prefix.
- Printing Wide String: The wprintf function is used to print the wide-character string.
- Length Calculation: The wcslen function calculates the length of the wide-character string.
- Conversion to Multibyte String: The wcstombs function converts the wide-character string to a multibyte string.
🚧 Common Pitfalls
- Locale Dependency: Functions like mbstowcs and wcstombs depend on the current locale. Ensure that the locale is set appropriately.
- Buffer Sizes: When converting between multibyte and wide-character strings, ensure that the destination buffer is large enough to hold the converted string.
- End-of-String Handling: Always ensure that wide-character strings are null-terminated to avoid undefined behavior.
✅ Best Practices
Locale Initialization: Use setlocale to set the appropriate locale at the beginning of your program.
example.cCopiedsetlocale(LC_ALL, "");
- Buffer Management: Always check the size of buffers and handle potential overflows gracefully.
- Consistent Use of Functions: Stick to wide-character functions when dealing with wide-character strings to maintain consistency and avoid potential issues with character encoding.
🎉 Conclusion
The wchar.h
header is an essential tool for C programmers dealing with internationalization and multiple character sets. It provides robust support for wide characters and strings, enabling the creation of applications that can handle a diverse range of languages and characters.
By understanding and utilizing the data types, macros, and functions defined in this header, you can write more flexible and internationalized code. However, be mindful of common pitfalls and adhere to best practices to ensure your code is both safe and efficient.
👨💻 Join our Community:
Author
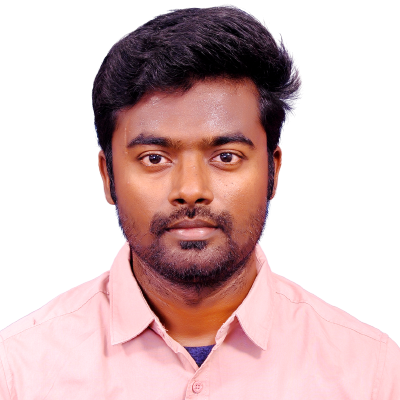
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C Library – wchar.h), please comment here. I will help you immediately.