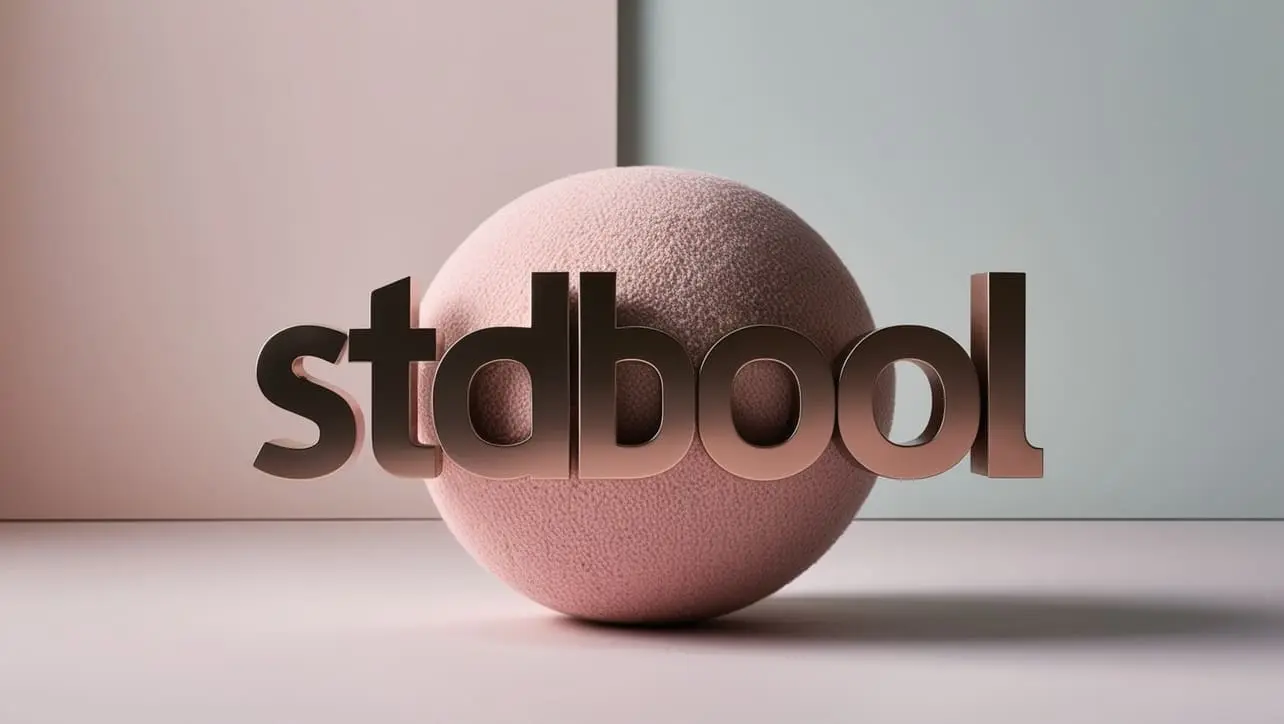
C Library – time.h
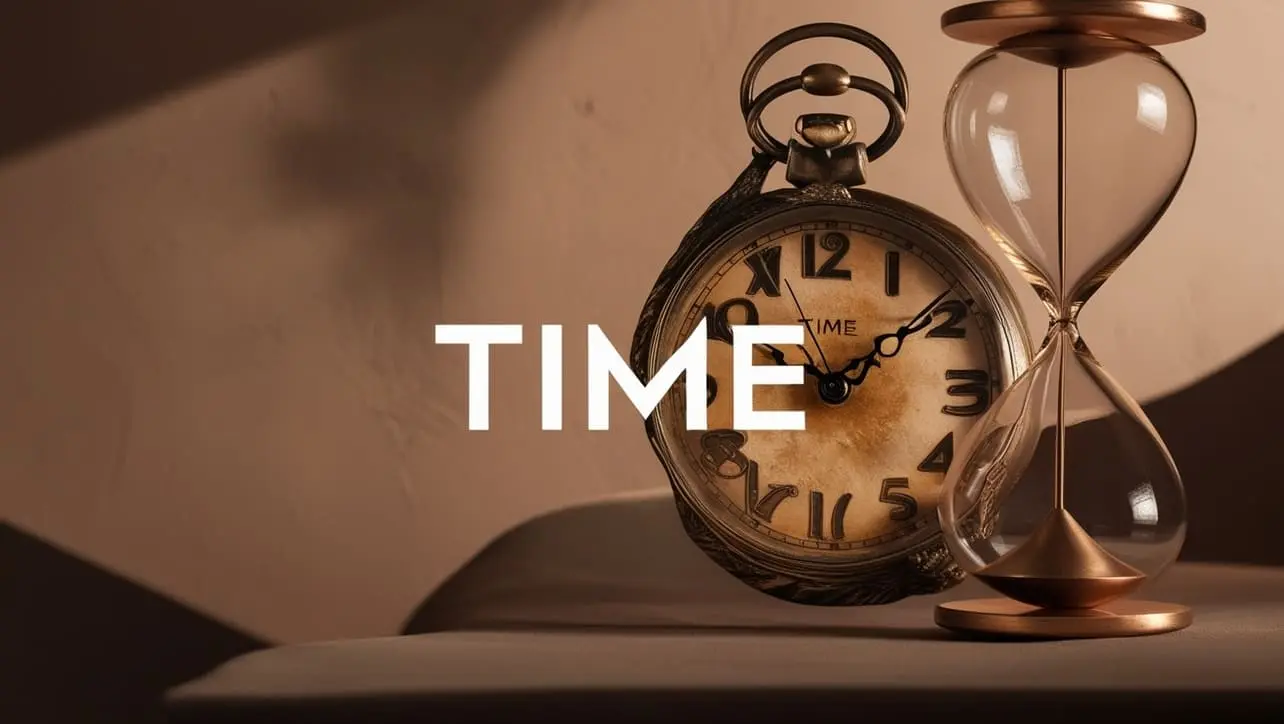
Photo Credit to CodeToFun
🙋 Introduction
The <time.h>
header file in the C programming language provides functions and macros for manipulating date and time.
This library is essential for applications that require time-related functionalities, such as timestamping events, measuring intervals, and formatting dates and times.
💡 Syntax
To use the functions and macros provided by <time.h>
, include the header file at the beginning of your C program:
#include <time.h>
📚 Key Functions and Macros
Here are some of the key functions and macros available in <time.h>
:
time():
Returns the current calendar time as a time_t object.
example.cCopiedtime_t current_time; current_time = time(NULL);
difftime():
Returns the difference in seconds between two time_t values.
example.cCopieddouble difference; difference = difftime(time1, time2);
clock():
Returns the processor time consumed by the program.
example.cCopiedclock_t start, end; start = clock(); /* Some computation */ end = clock(); double cpu_time_used = ((double) (end - start)) / CLOCKS_PER_SEC;
gmtime():
Converts a time_t value to a tm structure representing Coordinated Universal Time (UTC).
example.cCopiedstruct tm *utc_time; time_t current_time = time(NULL); utc_time = gmtime(¤t_time);
localtime():
Converts a time_t value to a tm structure representing local time.
example.cCopiedstruct tm *local_time; time_t current_time = time(NULL); local_time = localtime(¤t_time);
strftime():
Formats a tm structure as a string.
example.cCopiedchar buffer[80]; struct tm *time_info; time_t rawtime = time(NULL); time_info = localtime(&rawtime); strftime(buffer, 80, "Now it's %I:%M%p.", time_info);
📝 Basic Example:
Here's a complete example demonstrating the use of several <time.h>
functions to print the current date and time:
#include <stdio.h>
#include <time.h>
int main() {
time_t current_time;
struct tm *local_time;
char buffer[80];
// Get the current time
time(¤t_time);
// Convert to local time format
local_time = localtime(¤t_time);
// Format time as a string
strftime(buffer, 80, "Current date and time: %Y-%m-%d %H:%M:%S", local_time);
printf("%s\n", buffer);
return 0;
}
💻 Output
Current date and time: 2024-06-02 10:51:02
💰 Benefits
Using the <time.h>
library offers several benefits:
- Standardization: As part of the C standard library,
<time.h>
provides a consistent and portable way to handle time-related functions across different platforms and compilers. - Ease of Use: The functions and macros in
<time.h>
are straightforward and easy to use, making it accessible even for beginners. - Comprehensive Functionality: With a wide range of functions,
<time.h>
covers various aspects of time manipulation, from simple time retrieval to complex formatting and interval calculations. - Performance: The library functions are optimized for performance, ensuring efficient time calculations and manipulations, which is crucial for time-sensitive applications.
- Interoperability: The time_t and tm structures provide a standardized way to store and manipulate time data, facilitating interoperability with other libraries and systems that use similar time representations.
🎉 Conclusion
The <time.h>
library in C is a powerful tool for handling various time-related operations. By leveraging the functions and macros it provides, you can perform complex time manipulations and formatting with ease.
Understanding and utilizing <time.h>
is crucial for developing applications that depend on accurate and efficient time management.
👨💻 Join our Community:
Author
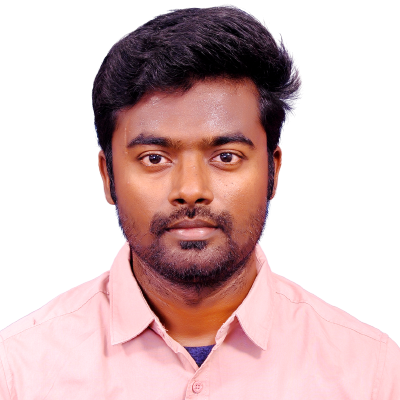
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C Library – time.h), please comment here. I will help you immediately.