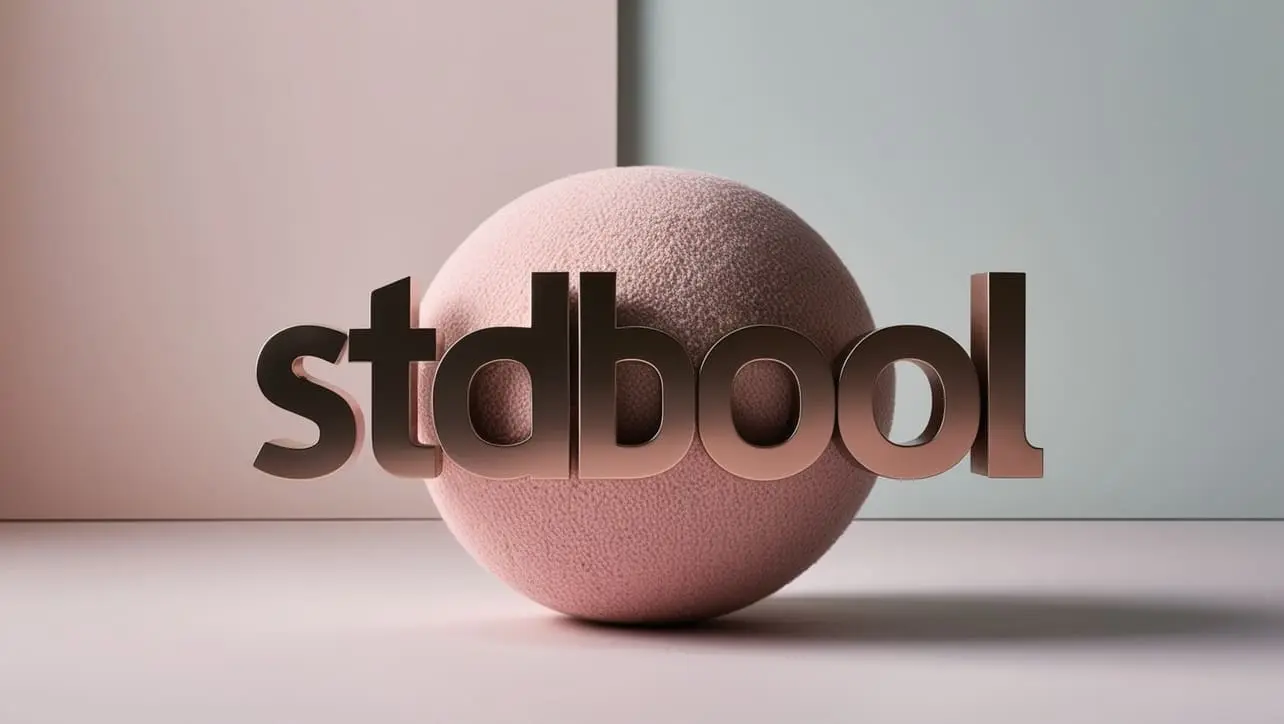
C Library – stdlib.h
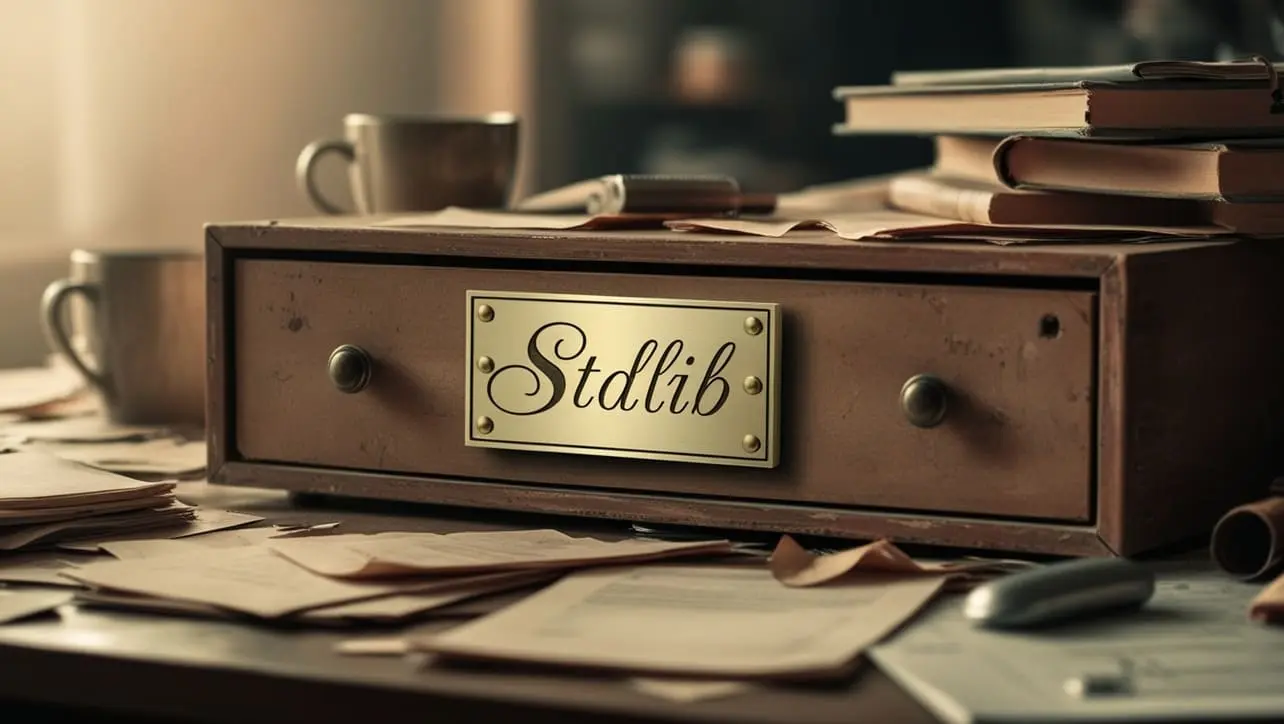
Photo Credit to CodeToFun
🙋 Introduction
The <stdlib.h>
header file in the C programming language is a vital part of the standard library, providing a range of functions for performing general utility operations. These include memory allocation, process control, conversions, and other miscellaneous functions. Understanding the capabilities of <stdlib.h>
is essential for effective C programming.
💡 Syntax
To use the functions and macros provided by <stdlib.h>
, include the header file at the beginning of your C program:
#include <stdlib.h>
📚 Key Functions and Macros
The following are the list of Memory Allocation Functions and macros
malloc():
Allocates a specified number of bytes and returns a pointer to the allocated memory.
example.cCopiedvoid *ptr = malloc(size_t size);
calloc():
Allocates memory for an array of elements, initializes them to zero, and returns a pointer to the memory.
example.cCopiedvoid *ptr = calloc(size_t num, size_t size);
realloc():
Resizes previously allocated memory.
example.cCopiedvoid *ptr = realloc(void *ptr, size_t size);
free():
Frees previously allocated memory.
example.cCopiedfree(void *ptr);
The following are the list of Process Control Functions and macros
exit():
Terminates the calling process.
example.cCopiedvoid exit(int status);
abort():
Abnormally terminates the process.
example.cCopiedvoid abort(void);
The following are the list of Conversion functions and macros
atoi():
Converts a string to an integer.
example.cCopiedint num = atoi(const char *str);
atof():
Converts a string to a floating-point number.
example.cCopieddouble num = atof(const char *str);
strtol():
Converts a string to a long integer.
example.cCopiedlong int num = strtol(const char *str, char **endptr, int base);
strtod():
Converts a string to a double.
example.cCopieddouble num = strtod(const char *str, char **endptr);
The following are the list of Miscellaneous Functions and macros
rand():
Returns a pseudo-random number.
example.cCopiedint random_number = rand(void);
srand():
Seeds the random number generator used by rand().
example.cCopiedvoid srand(unsigned int seed);
📝 Basic Example
Here's a complete example demonstrating the use of several <stdlib.h>
functions:
#include <stdio.h>
#include <stdlib.h>
int main() {
// Memory allocation
int *arr = (int *)malloc(5 * sizeof(int));
if (arr == NULL) {
fprintf(stderr, "Memory allocation failed\n");
exit(1);
}
// Initialize array
for (int i = 0; i < 5; i++) {
arr[i] = i + 1;
}
// Print array elements
for (int i = 0; i < 5; i++) {
printf("%d ", arr[i]);
}
printf("\n");
// Free allocated memory
free(arr);
// String to integer conversion
char str[] = "12345";
int num = atoi(str);
printf("The integer value is %d\n", num);
return 0;
}
💻 Output
1 2 3 4 5 The integer value is 12345
💰 Benefits
Using the <stdlib.h>
library offers several benefits:
- Versatility: Provides a wide range of functions that cover various aspects of programming, from memory management to process control and numerical conversions.
- Standardization: As part of the C standard library,
<stdlib.h>
ensures that your code is portable and adheres to standard practices across different platforms and compilers. - Efficiency: The functions in
<stdlib.h>
are optimized for performance, which is crucial for developing efficient and responsive applications. - Ease of Use: The straightforward nature of the functions and macros in
<stdlib.h>
makes it accessible to both beginners and experienced programmers. - Robustness: Offers robust utilities for handling common programming tasks, reducing the likelihood of errors and improving code reliability.
🎉 Conclusion
The <stdlib.h>
library in C is an indispensable resource for developers, providing essential functions for memory management, process control, conversions, and more.
Mastering the use of <stdlib.h>
functions enhances your ability to write efficient, portable, and reliable C programs.
👨💻 Join our Community:
Author
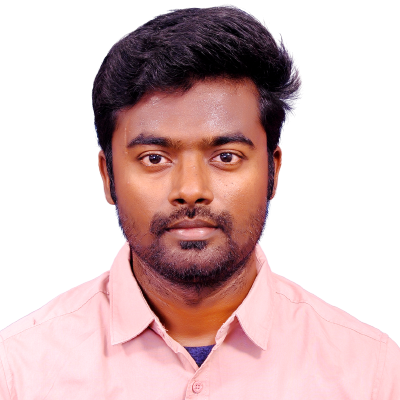
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C Library – stdlib.h), please comment here. I will help you immediately.