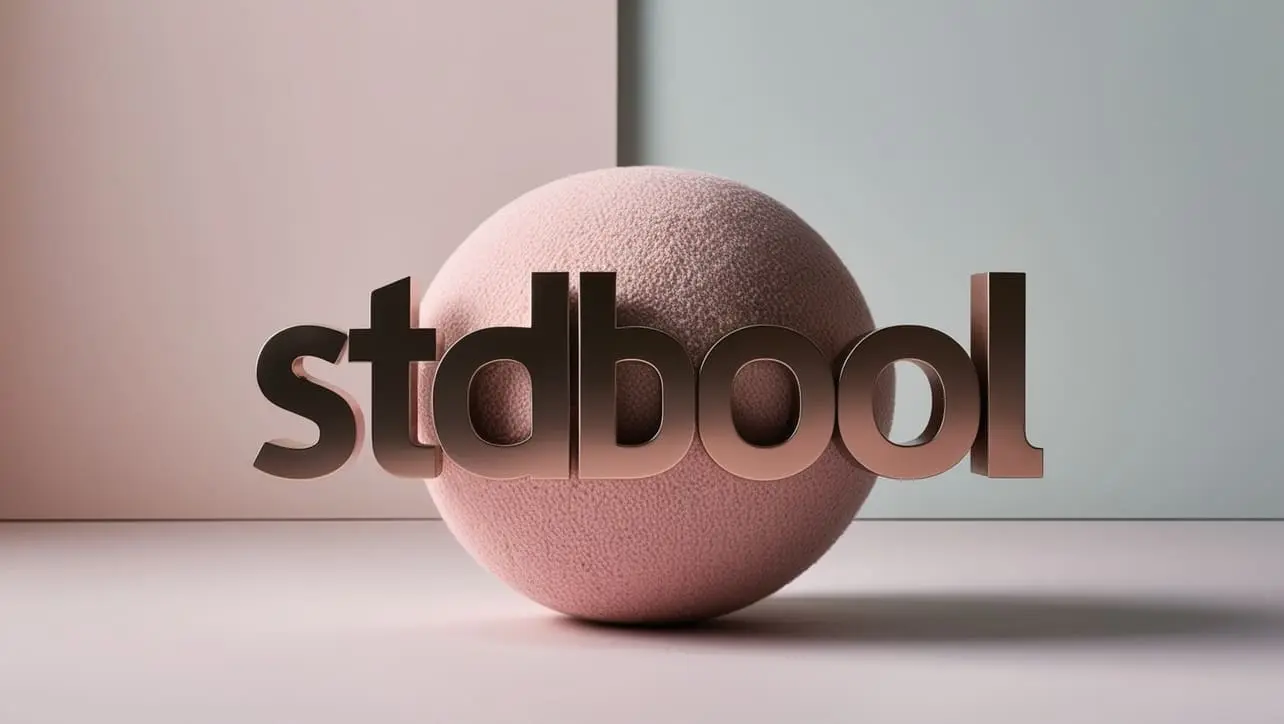
C Library โ math.h
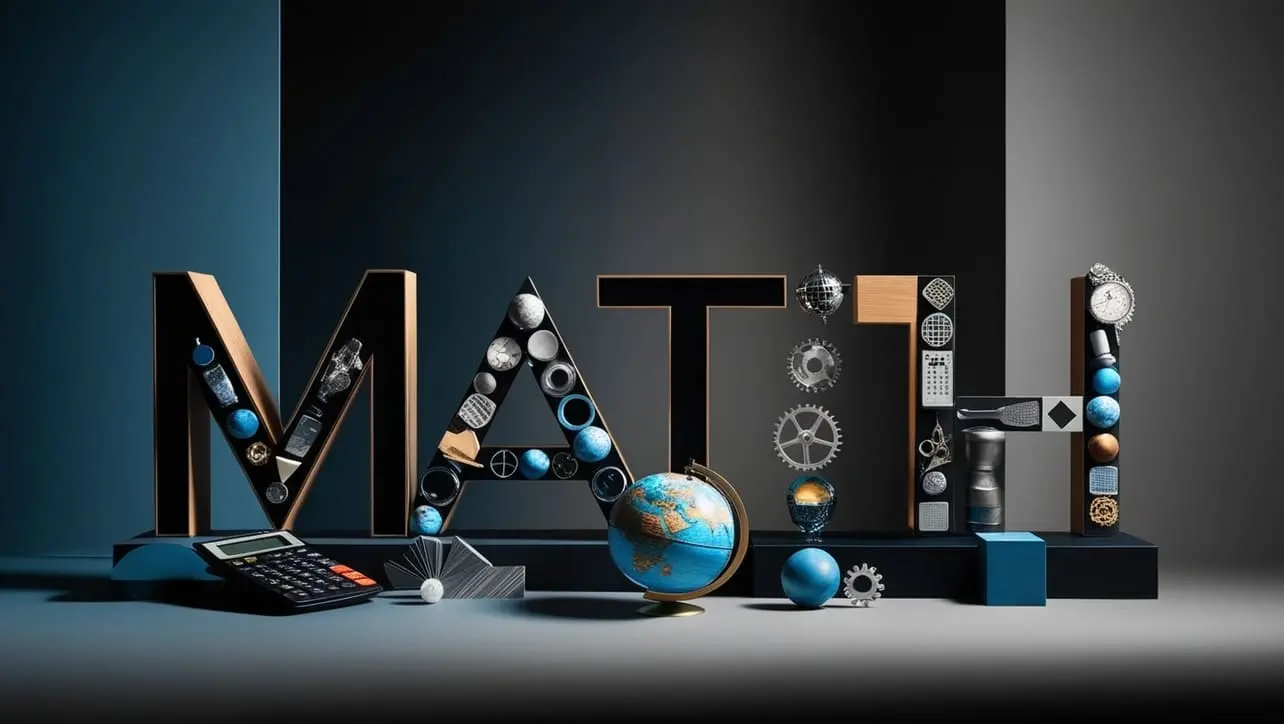
Photo Credit to CodeToFun
Introduction
The <math.h>
header file in the C programming language provides a variety of mathematical functions for performing complex calculations. This library is essential for applications that require mathematical operations such as trigonometry, logarithms, power functions, and more.
Understanding and using <math.h>
can greatly enhance your ability to handle mathematical computations in C.
Syntax
To use the functions and macros provided by <math.h>
, include the header file at the beginning of your C program:
#include <math.h>
Key Functions and Macros
Here are some of the key functions and macros available in <math.h>
:
pow():
Computes the power of a number.
example.cCopieddouble result; result = pow(base, exponent); // Computes base^exponent
sqrt():
Calculates the square root of a number.
example.cCopieddouble result; result = sqrt(number); // Computes the square root of number
sin(), cos(), tan():
Compute the sine, cosine, and tangent of an angle (in radians).
example.cCopieddouble result; result = sin(angle); // Computes the sine of angle result = cos(angle); // Computes the cosine of angle result = tan(angle); // Computes the tangent of angle
log(), log10():
Compute the natural logarithm and base-10 logarithm of a number.
example.cCopieddouble result; result = log(number); // Computes the natural logarithm of number result = log10(number); // Computes the base-10 logarithm of number
exp():
Calculates the exponential value of a number (e^x).
example.cCopieddouble result; result = exp(exponent); // Computes e^exponent
fabs():
Computes the absolute value of a floating-point number.
example.cCopieddouble result; result = fabs(number); // Computes the absolute value of number
Basic Example
Here's a complete example demonstrating the use of several <math.h>
functions:
#include <stdio.h>
#include <math.h>
int main() {
double x = 2.0, y = 8.0;
double result;
// Power function
result = pow(x, y);
printf("pow(%.2f, %.2f) = %.2f\n", x, y, result);
// Square root function
result = sqrt(y);
printf("sqrt(%.2f) = %.2f\n", y, result);
// Trigonometric functions
double angle = M_PI / 4; // 45 degrees in radians
printf("sin(%.2f) = %.2f\n", angle, sin(angle));
printf("cos(%.2f) = %.2f\n", angle, cos(angle));
printf("tan(%.2f) = %.2f\n", angle, tan(angle));
// Logarithmic functions
result = log(x);
printf("log(%.2f) = %.2f\n", x, result);
result = log10(y);
printf("log10(%.2f) = %.2f\n", y, result);
// Exponential function
result = exp(x);
printf("exp(%.2f) = %.2f\n", x, result);
// Absolute value function
result = fabs(-x);
printf("fabs(%.2f) = %.2f\n", -x, result);
return 0;
}
Output
pow(2.00, 8.00) = 256.00 sqrt(8.00) = 2.83 sin(0.79) = 0.71 cos(0.79) = 0.71 tan(0.79) = 1.00 log(2.00) = 0.69 log10(8.00) = 0.90 exp(2.00) = 7.39 fabs(-2.00) = 2.00
Benefits
Using the <math.h>
library offers several benefits:
- Standardization: As part of the C standard library,
<math.h>
provides a consistent and portable way to perform mathematical operations across different platforms and compilers. - Comprehensive Functionality: The library offers a wide range of mathematical functions, covering basic arithmetic, trigonometry, logarithms, and more, making it versatile for various applications.
- Performance: The functions in
<math.h>
are optimized for performance, ensuring efficient calculations, which is crucial for computation-heavy applications. - Ease of Use: The functions are designed to be easy to use, allowing even beginners to perform complex mathematical operations with simple function calls.
- Interoperability: The functions in
<math.h>
use standard data types and return values, facilitating easy integration with other parts of your program and other libraries.
Conclusion
The <math.h>
library in C is a powerful tool for handling a wide range of mathematical operations. By leveraging the functions and macros it provides, you can perform complex calculations with ease and efficiency.
Understanding and utilizing <math.h>
is crucial for developing applications that require accurate and fast mathematical computations.
Join our Community:
Author
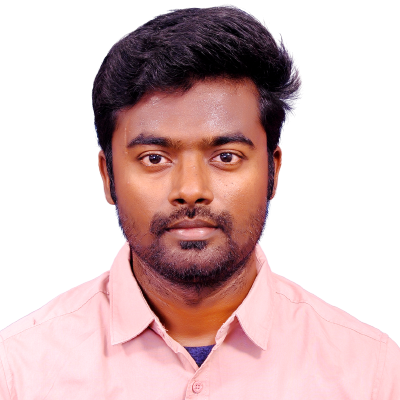
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C Library โ math.h), please comment here. I will help you immediately.