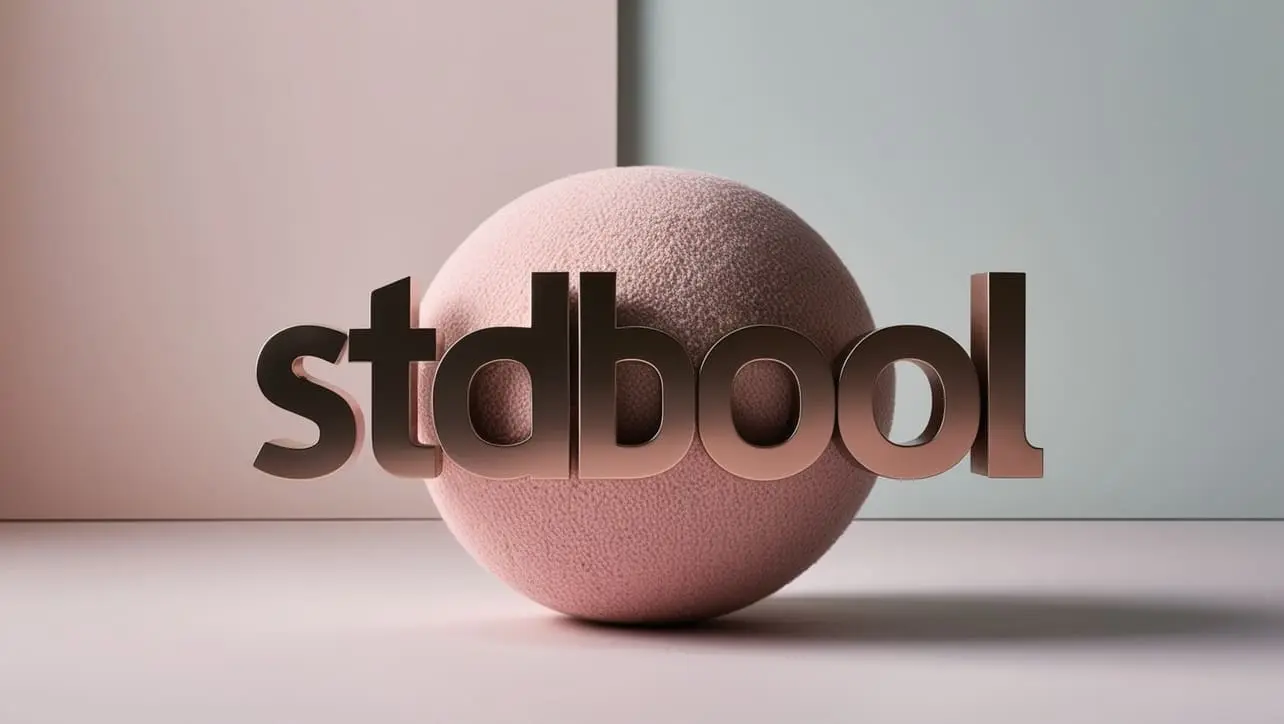
C Standard Library
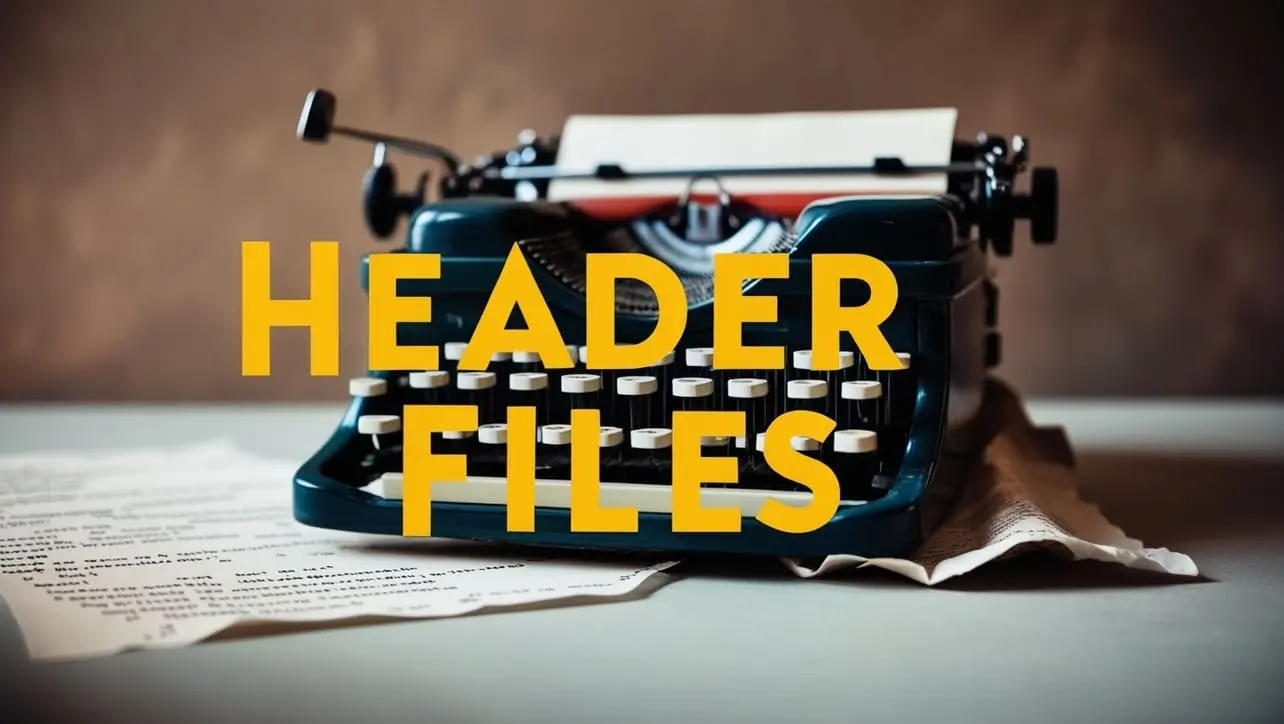
Photo Credit to CodeToFun
🙋 Introduction
The C Standard Library is a powerful set of predefined functions, macros, and types that are included in the C programming language. These libraries provide essential functionalities for performing input/output operations, string handling, mathematical computations, memory allocation, and more.
This reference guide provides an overview of the key components of the C Standard Library, helping you to effectively utilize these resources in your C programming projects.
📑 Table of Contents
Some of the commonly used standard libraries in C includes:
Library | Explanation |
---|---|
<assert.h> | Provides a macro for adding diagnostics to a program, allowing for runtime checks of expressions to help detect logical errors and assumptions. |
<ctype.h> | Provides functions for testing and mapping characters, such as checking if a character is a digit, letter, or whitespace, and converting between uppercase and lowercase. |
<errno.h> | Defines macros for reporting and handling error conditions through error codes, enabling standardized error checking and debugging. |
<float.h> | Provides macros that specify the characteristics of floating-point types, including their limits and precision. |
<limits.h> | Defines constants that specify the implementation-specific limits of fundamental data types, such as the maximum and minimum values for integers and the number of bits in a byte. |
<locale.h> | Provides functions and macros for setting and querying the program's locale, which affects how data such as dates, times, and numbers are formatted and interpreted. |
<math.h> | Provides functions for mathematical operations such as trigonometry, logarithms, and rounding. |
<setjmp.h> | Provides functions for non-local jumps, allowing control flow to move to a previously set program point. |
<signal.h> | Provides functions for handling signals, which are asynchronous events that can interrupt the normal flow of a program. |
<stdarg.h> | Provides functions for handling variable argument lists in functions. |
<stdbool.h> | Provides boolean data type support through the use of "true" and "false" constants. |
<stddef.h> | Provides definitions for common types and macros, including the NULL pointer and various size-related constants, facilitating portable code. |
<stdint.h> | Provides precise-width integer types in C programming, ensuring consistent data representation across different platforms. |
<stdio.h> | Provides functions for input and output operations, like printing to the console and reading from files. |
<stdlib.h> | Provides functions for memory allocation, conversion, and other utilities. |
<string.h> | Provides functions for manipulating strings in the C programming language. |
<time.h> | Provides functions for manipulating time and date information in C programs. |
<wchar.h> | Provides functions for handling wide characters and multibyte characters. |
<wctype.h> | Provides functions for character classification and mapping based on wide character types. |
🚀 Usage Tips
Here are some tips to make the most out of the C Standard Library:
- Include Headers: Ensure you include the appropriate header files at the beginning of your source files to access the library functions and macros.
- Read Documentation: Familiarize yourself with the official documentation for detailed information on each function, including parameters, return values, and potential errors.
- Error Handling: Implement proper error handling to manage and debug issues that may arise from library functions.
- Performance Considerations: Understand the performance implications of using certain library functions, especially in resource-constrained environments.
📄 Example
Let's look at a practical example of using C Standard Library functions to perform basic input/output operations and string manipulation:
#include <stdio.h>
#include <string.h>
int main() {
char str1[50], str2[50];
// Input strings from the user
printf("Enter the first string: ");
fgets(str1, sizeof(str1), stdin);
str1[strcspn(str1, "\n")] = '\0'; // Remove the trailing newline character
printf("Enter the second string: ");
fgets(str2, sizeof(str2), stdin);
str2[strcspn(str2, "\n")] = '\0'; // Remove the trailing newline character
// Compare the strings
if (strcmp(str1, str2) == 0) {
printf("The strings are equal.\n");
} else {
printf("The strings are not equal.\n");
}
// Concatenate the strings
strcat(str1, str2);
printf("Concatenated String: %s\n", str1);
return 0;
}
💻 Testing the Program
Enter the first string: codetofun Enter the second string: .com The strings are not equal. Concatenated String: codetofun.com
🧠 How the Program Works
This example demonstrates basic input/output operations using printf and fgets functions, and string manipulation using strcmp and strcat functions from the C Standard Library.
🎉 Conclusion
The C Standard Library provides a comprehensive suite of functions and macros that are essential for efficient C programming. By understanding and utilizing these libraries, you can significantly enhance the functionality and reliability of your code.
Explore the various components of the C Standard Library, experiment with different functions, and refer to the official documentation for detailed information. Happy coding!
👨💻 Join our Community:
Author
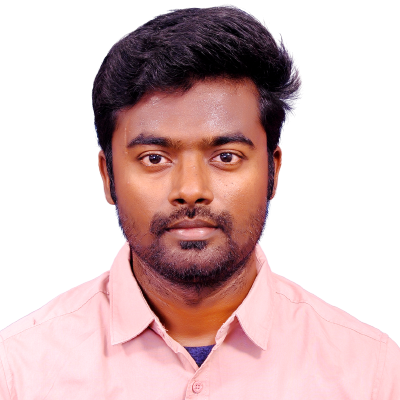
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C Standard Library), please comment here. I will help you immediately.