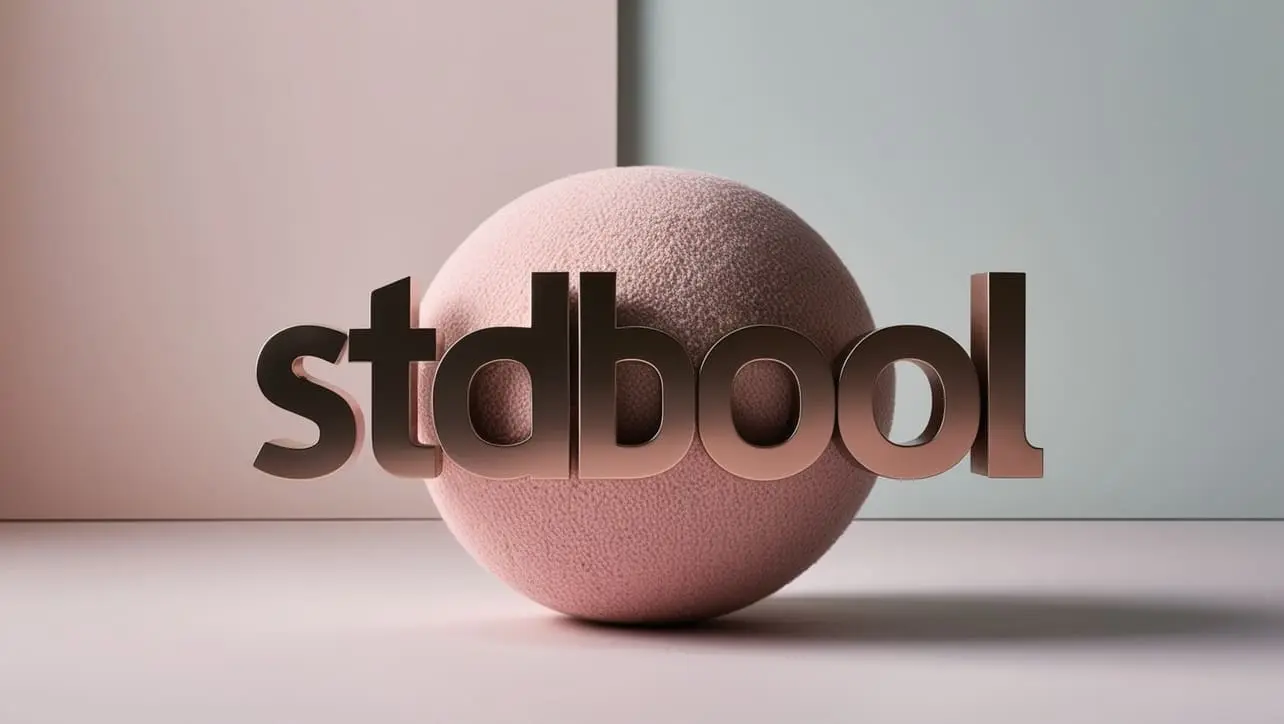
C nested if Statement
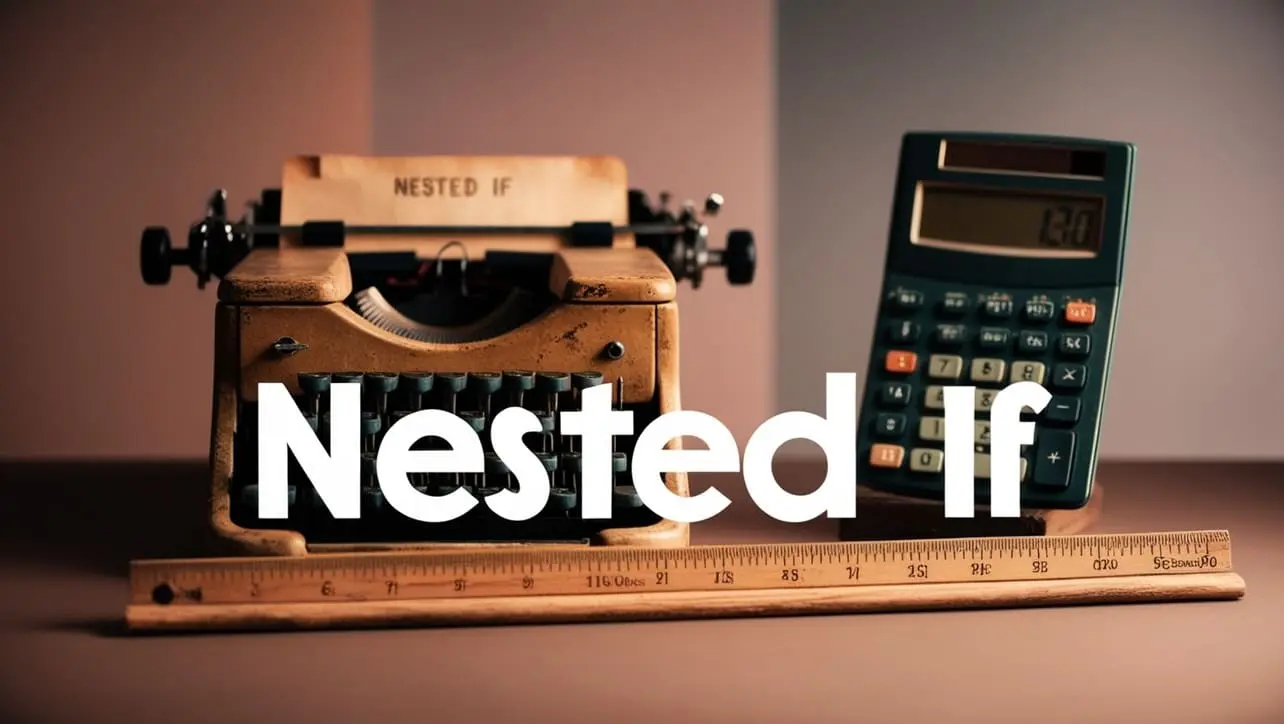
Photo Credit to CodeToFun
π Introduction
The if statement is fundamental to decision-making in C programming. Sometimes, you may need to test multiple conditions in a program, which can be achieved using nested if statements.
This guide will provide an in-depth look at nested if statements, their syntax, and practical examples of their usage.
π€ What is a Nested if Statement?
A nested if statement is an if statement placed inside another if statement. This allows you to check multiple conditions and perform different actions based on those conditions.
π Key Features
- Multiple Condition Checking: Allows testing of multiple conditions.
- Hierarchical Decision Making: Supports complex decision-making processes.
- Flexibility: Offers flexibility in defining conditions and actions.
π‘ Syntax
The syntax for nested if statements involves placing one if statement inside another. Hereβs a general structure:
if (condition1) {
// Executes when condition1 is true
if (condition2) {
// Executes when condition2 is true
}
}
Example: Basic Nested if Statement
#include <stdio.h>
int main() {
int num = 10;
if (num > 0) {
printf("Number is positive\n");
if (num % 2 == 0) {
printf("Number is even\n");
}
}
return 0;
}
π» Output
In this example, the program checks if num is positive and then checks if it is even.
Number is positive Number is even
Practical Examples
Example 1: Checking Multiple Conditions
example.cCopied#include <stdio.h> int main() { int age = 20; int grade = 85; if (age >= 18) { if (grade >= 60) { printf("Eligible for admission\n"); } else { printf("Not eligible due to low grade\n"); } } else { printf("Not eligible due to age\n"); } return 0; }
This program checks if a person is eligible for admission based on age and grade.
Example 2: Nested if-else Statements
example.cCopied#include <stdio.h> int main() { int number = -5; if (number >= 0) { if (number == 0) { printf("Number is zero\n"); } else { printf("Number is positive\n"); } } else { printf("Number is negative\n"); } return 0; }
In this example, the program distinguishes between zero, positive, and negative numbers using nested if-else statements.
Common Pitfalls and Best Practices
Readability:
Nested if statements can become complex and hard to read. To improve readability:
- Indentation: Use proper indentation to highlight nested structures.
- Comments: Add comments to explain the logic of complex conditions.
Logical Complexity:
Avoid excessive nesting to prevent logical complexity. Consider using logical operators (&&, ||) or else if for simpler conditions.
example.cCopied#include <stdio.h> int main() { int num = 10; if (num > 0 && num % 2 == 0) { printf("Number is positive and even\n"); } return 0; }
This example simplifies the nested if statement by combining conditions using the logical && operator.
Nested if-else-if Ladder:
In cases with multiple conditions, consider using an if-else-if ladder for better readability and structure.
example.cCopied#include <stdio.h> int main() { int marks = 75; if (marks >= 90) { printf("Grade: A\n"); } else if (marks >= 80) { printf("Grade: B\n"); } else if (marks >= 70) { printf("Grade: C\n"); } else if (marks >= 60) { printf("Grade: D\n"); } else { printf("Grade: F\n"); } return 0; }
This example uses an if-else-if ladder to assign grades based on marks.
π Conclusion
Nested if statements are a powerful tool in C programming for handling multiple conditions and making complex decisions. By understanding their syntax and best practices, you can write more efficient and readable code.
π¨βπ» Join our Community:
Author
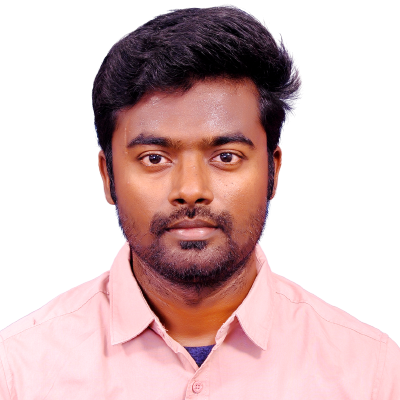
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C nested if Statement), please comment here. I will help you immediately.