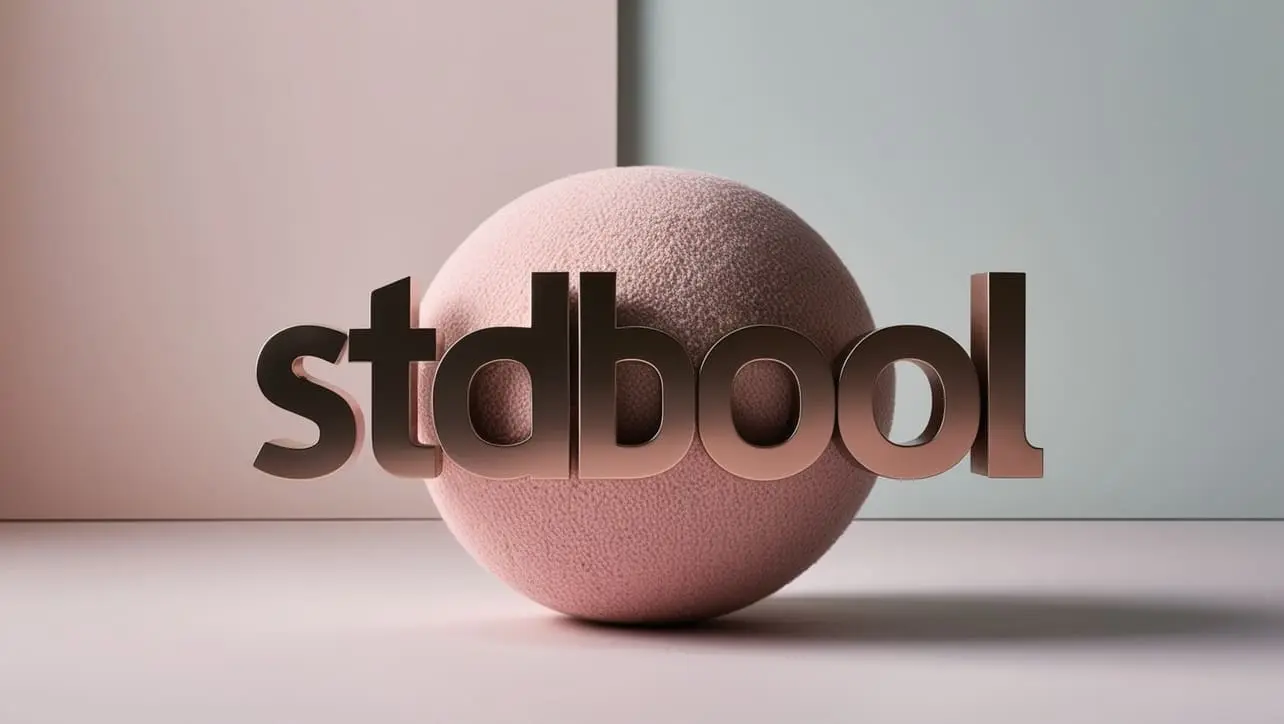
C if else Statement
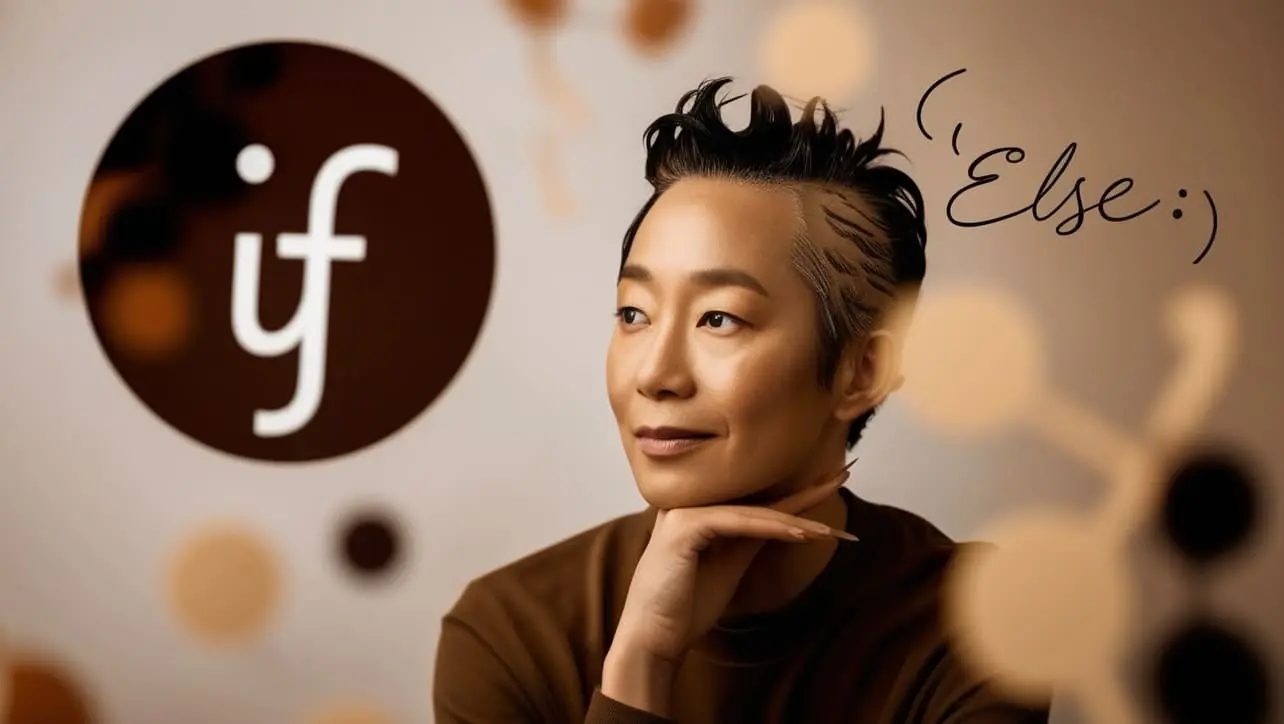
Photo Credit to CodeToFun
🙋 Introduction
The else
statement in C is a fundamental part of conditional programming. It allows you to specify a block of code that will execute if the condition in an if statement is false.
This guide will provide an in-depth look at how the else
statement works, its syntax, and practical examples of its usage.
🤔 What is the else Statement?
In C programming, the else
statement is used in conjunction with the if statement to define an alternative block of code to be executed when the condition in the if statement is false. This helps in handling different cases based on specific conditions.
🔑 Key Features
- Conditional Execution: Executes a block of code when the if condition is not met.
- Control Flow: Enhances the decision-making capabilities of your program.
- Readability: Improves code readability by clearly defining alternative paths.
💡 Syntax
The syntax for the else
statement is simple and pairs with the if statement:
if (condition) {
// Code to execute if condition is true
} else {
// Code to execute if condition is false
}
Usage
The else
statement follows an if statement and provides an alternative path of execution.
Example:
#include <stdio.h>
int main() {
int number = 10;
if (number > 0) {
printf("The number is positive.\n");
} else {
printf("The number is non-positive.\n");
}
return 0;
}
💻 Output
In this example, since number is 10, which is greater than 0, the output will be:
The number is positive.
If number were -5, the output would be:
The number is non-positive.
Combining else with if Statements
The else
statement can be used with multiple if statements to handle multiple conditions.
Example: else if Ladder
#include <stdio.h>
int main() {
int number = 0;
if (number > 0) {
printf("The number is positive.\n");
} else if (number < 0) {
printf("The number is negative.\n");
} else {
printf("The number is zero.\n");
}
return 0;
}
In this example, the program checks if the number is positive, negative, or zero and prints the corresponding message. Since number is 0, the output will be:
The number is zero.
Nested else Statements
You can nest else
statements within other if or else blocks to handle more complex conditions.
Example: Nested else
#include <stdio.h>
int main() {
int age = 25;
char gender = 'F';
if (age > 18) {
if (gender == 'M') {
printf("Adult Male.\n");
} else {
printf("Adult Female.\n");
}
} else {
printf("Not an adult.\n");
}
return 0;
}
💻 Output
In this example, the output will be:
Adult Female.
because age is greater than 18 and gender is 'F'.
📚 Common Pitfalls and Best Practices
Ensure Proper Pairing:
Each else should follow an if statement to ensure correct logic flow and avoid compilation errors.
Avoid Overusing else:
While else is useful, overusing it can make code harder to read. Use
else
statements judiciously and consider other structures like switch for multiple conditions.Maintain Readability:
Use proper indentation and spacing to make your if-else blocks easy to read and understand.
🎉 Conclusion
The else
statement is a crucial component of conditional programming in C. It allows you to handle alternative cases when an if condition is not met, enhancing the decision-making capabilities of your programs.
By understanding how to use the else
statement effectively, you can write more robust and readable code.
👨💻 Join our Community:
Author
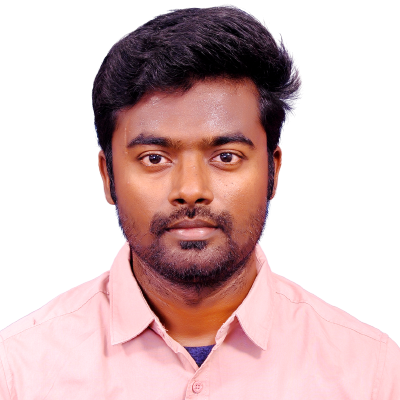
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C if else Statement), please comment here. I will help you immediately.