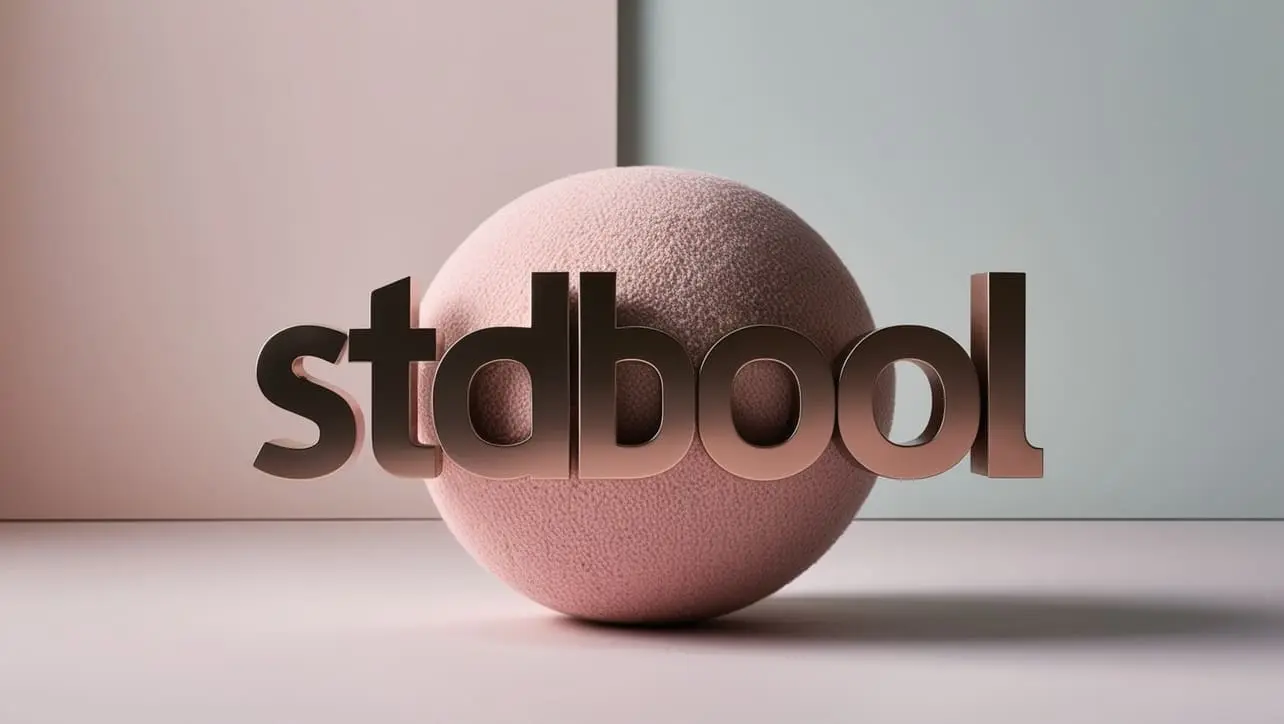
C break Statement
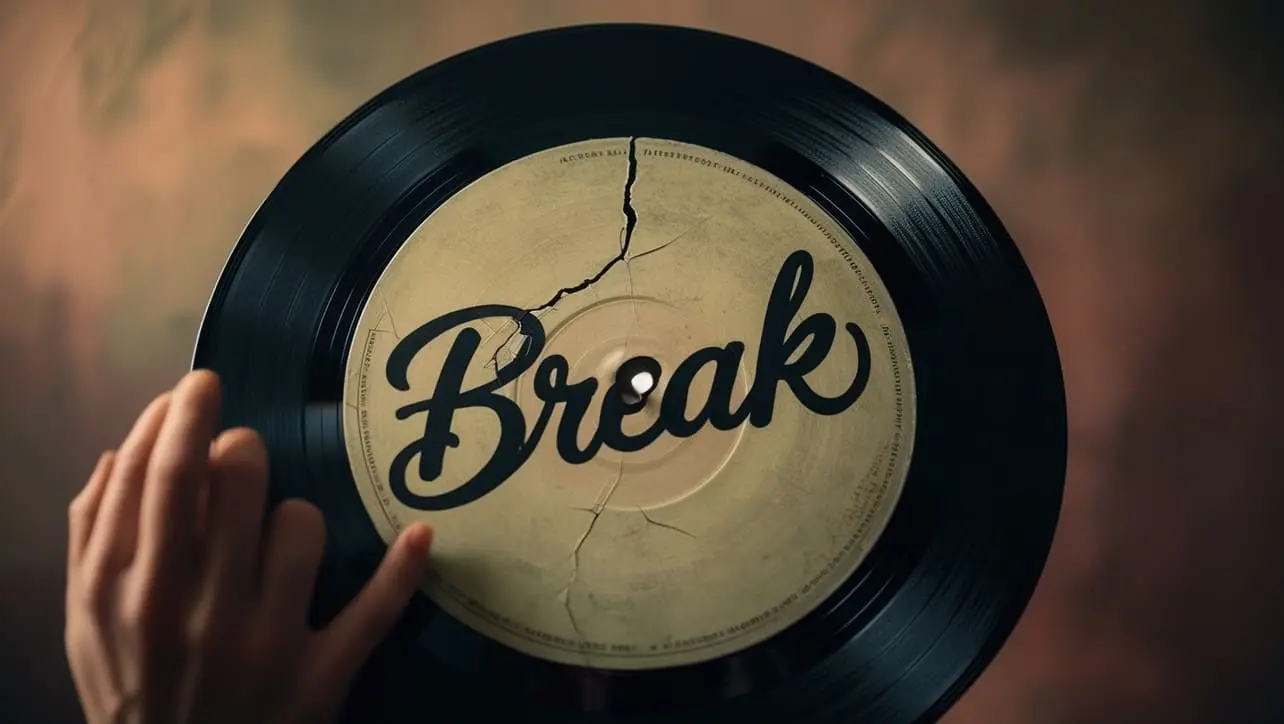
Photo Credit to CodeToFun
🙋 Introduction
The break
statement in C is a powerful control statement that allows you to exit from loops or switch statements prematurely.
This guide will provide an in-depth look at how the break
statement works, its syntax, and practical examples of its usage.
🤔 What is the break Statement?
In C programming, the break
statement is used to terminate the execution of the nearest enclosing loop or switch statement. When a break
statement is encountered, control is transferred immediately to the statement following the terminated statement.
🔑 Key Features of the break Statement
- Control Flow: Alters the normal sequence of execution.
- Loop Termination: Exits loops (for, while, do-while) prematurely.
- Switch Case Exit: Exits a switch statement once a case is executed.
💡 Syntax
The syntax for the break
statement is straightforward:
break;
Usage in Loops
The break
statement is commonly used within for, while, and do-while loops to exit the loop when a certain condition is met.
Example: Using break in a for Loop
#include <stdio.h>
int main() {
for (int i = 0; i < 10; i++) {
if (i == 5) {
break; // Exit the loop when i equals 5
}
printf("%d\n", i);
}
return 0;
}
💻 Output
In this example, the loop will terminate when i equals 5, resulting in the output:
0 1 2 3 4
Example: Using break in a while Loop
#include <stdio.h>
int main() {
int i = 0;
while (i < 10) {
if (i == 5) {
break; // Exit the loop when i equals 5
}
printf("%d\n", i);
i++;
}
return 0;
}
💻 Output
Similar to the for loop example, this will output:
0 1 2 3 4
Usage in Switch Statements
The break
statement is essential in switch statements to prevent fall-through, which occurs when control flows from one case to subsequent cases.
Example: Using break in a switch Statement
#include <stdio.h>
int main() {
int day = 3;
switch (day) {
case 1:
printf("Monday\n");
break;
case 2:
printf("Tuesday\n");
break;
case 3:
printf("Wednesday\n");
break;
default:
printf("Invalid day\n");
}
return 0;
}
💻 Output
In this example, when day equals 3, "Wednesday" is printed, and the break
statement prevents the execution of subsequent cases.
Wednesday
Common Pitfalls and Best Practices
Avoid Unnecessary Breaks:
Use
break
statements judiciously to maintain code readability and logic flow. Overusingbreak
can make code harder to understand and debug.Ensure Clear Logic:
Ensure the condition leading to a
break
is clear and intentional. This avoids unexpected behavior in loops and switch statements.Break in Nested Loops:
When using
break
in nested loops, it only exits the nearest enclosing loop. Consider using labels or flags for more complex control flow.Example: Break in Nested Loops
example.cCopied#include <stdio.h> int main() { for (int i = 0; i < 3; i++) { for (int j = 0; j < 3; j++) { if (j == 1) { break; // Exit the inner loop } printf("i = %d, j = %d\n", i, j); } } return 0; }
💻 Output
In this example, the inner loop exits when j equals 1, but the outer loop continues.
Outputi = 0, j = 0 i = 1, j = 0 i = 2, j = 0
🎉 Conclusion
The break
statement is a fundamental control statement in C programming that provides a way to alter the flow of loops and switch statements.
By understanding its usage and best practices, you can write more efficient and readable code.
👨💻 Join our Community:
Author
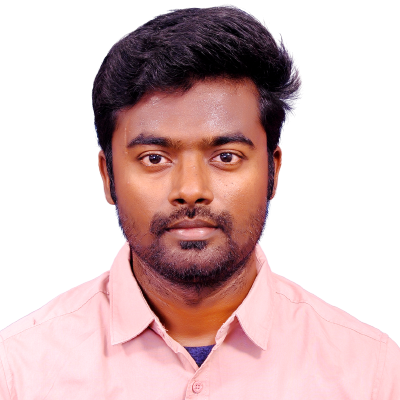
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C break Statement), please comment here. I will help you immediately.