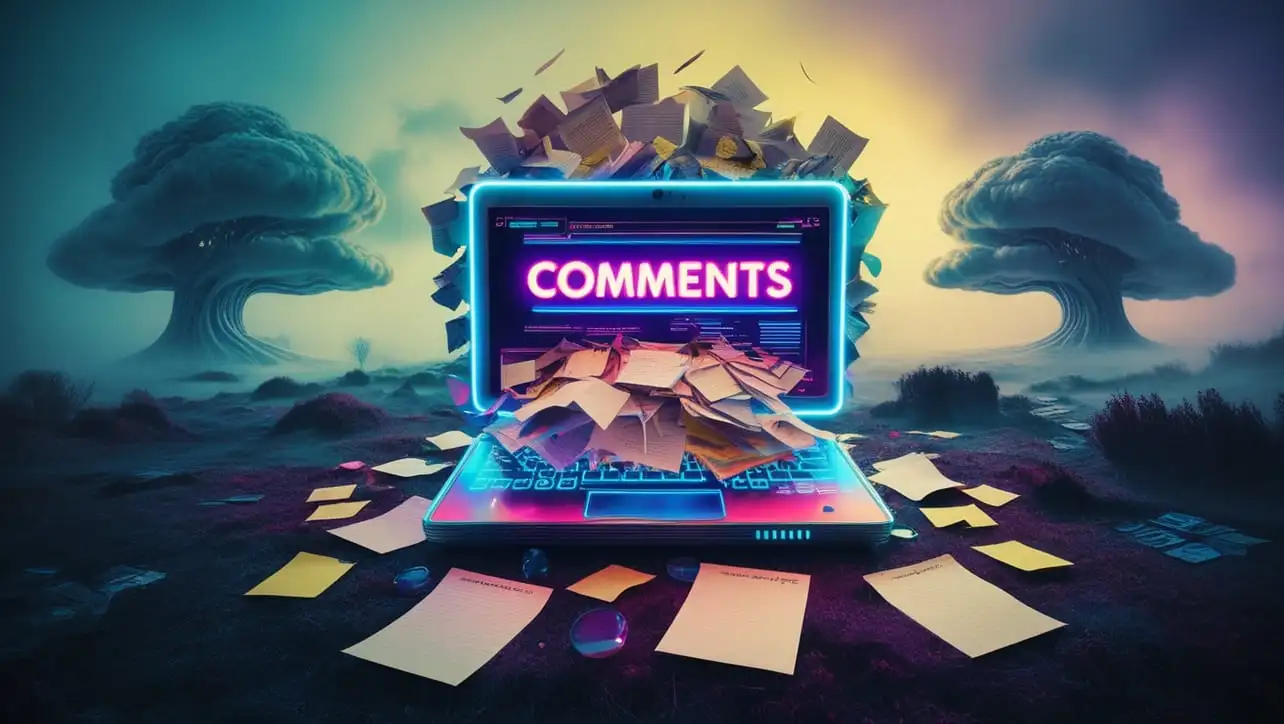
JSONP
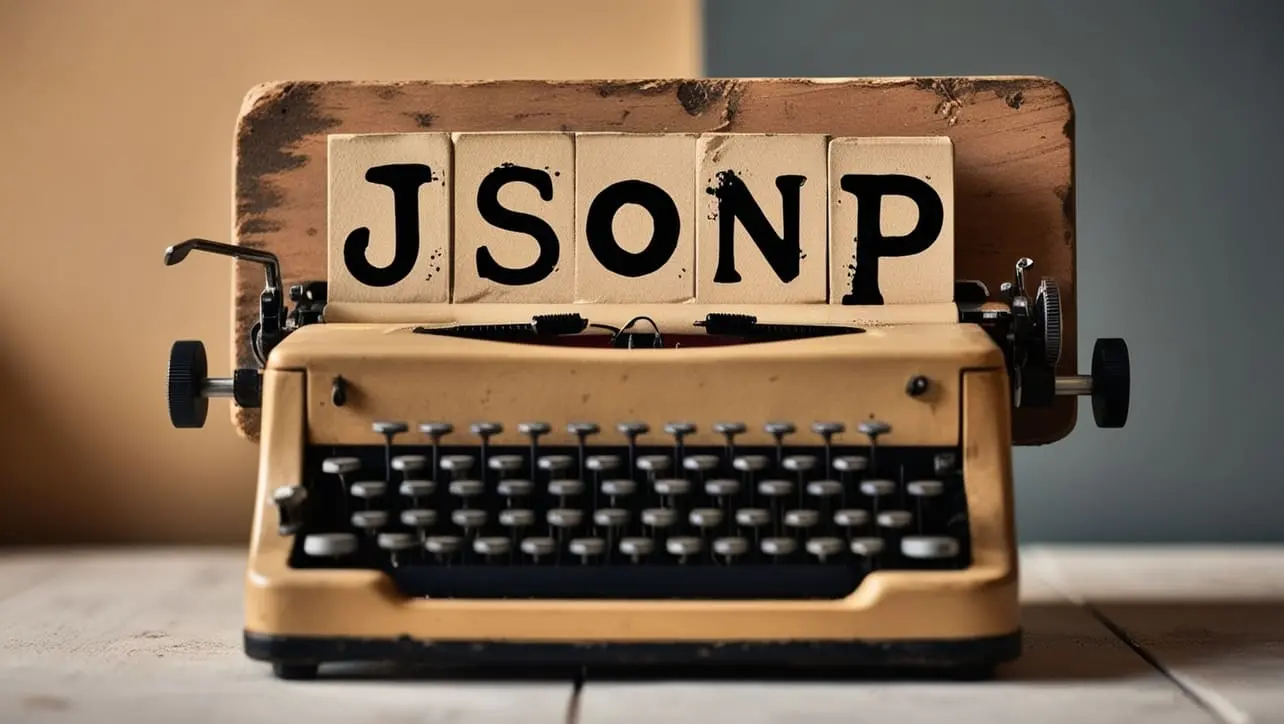
Photo Credit to CodeToFun
π Introduction
JSONP (JSON with Padding) is a technique used to overcome the limitations of the same-origin policy in web browsers. This policy restricts web pages from making requests to a domain different from the one that served the web page.
JSONP allows web pages to request data from a server in a different domain, enabling cross-domain communication in a way that doesn't violate the same-origin policy.
π€ What is JSONP?
JSONP stands for JSON with Padding. It is a method used to request data from a server residing in a different domain than the client. Unlike regular AJAX requests, which are restricted by the same-origin policy, JSONP works by dynamically injecting a <script> tag into the document. This script tag points to the URL of the data provider, and the server responds with a script containing the requested data wrapped in a callback function.
π§ How JSONP Works
- Client Request: The client creates a <script> tag and sets its src attribute to the URL of the server, including a callback function name as a query parameter.
- Server Response: The server generates a response that includes the data wrapped in the specified callback function.
- Callback Execution: The browser executes the script, calling the callback function and passing the data to it.
π§ Why Use JSONP?
JSONP is primarily used to overcome the limitations of the same-origin policy, allowing web applications to fetch data from different domains. Some reasons to use JSONP include:
- Cross-Domain Requests: JSONP enables fetching data from third-party APIs that reside on different domains.
- No Need for CORS: JSONP works without requiring Cross-Origin Resource Sharing (CORS) headers.
- Simple to Implement: JSONP is relatively straightforward to implement and can be a quick solution for cross-domain data requests.
π§± Basic Structure of a JSONP Request
Hereβs an example of how to make a JSONP request:
π» Client-Side Code:
<!DOCTYPE html>
<html>
<head>
<title>JSONP Example</title>
<script type="text/javascript">
function handleResponse(data) {
console.log(data);
}
function fetchData() {
var script = document.createElement('script');
script.src = 'https://example.com/api?callback=handleResponse';
document.body.appendChild(script);
}
</script>
</head>
<body onload="fetchData()">
</body>
</html>
π½ Server-Side Code (Pseudo Code)
// Example in Node.js
app.get('/api', function(req, res) {
const callback = req.query.callback;
const data = { message: 'Hello, world!' };
res.send(`${callback}(${JSON.stringify(data)})`);
});
π§ Explanation:
- Client-Side: The client creates a <script> tag with the src attribute pointing to the server's API endpoint, including a callback function name (handleResponse).
- Server-Side: The server wraps the response data in the callback function and sends it back as a script.
π’ Advantages
- Cross-Domain Requests: Allows making requests to different domains easily.
- Browser Compatibility: Supported by all major browsers without needing additional configurations.
π Disadvantages
- Security Risks: JSONP can expose your application to security vulnerabilities such as Cross-Site Scripting (XSS).
- Only GET Requests: JSONP only supports GET requests, limiting its use for other types of HTTP methods like POST, PUT, DELETE.
- Limited Error Handling: Error handling with JSONP is not as robust as with XMLHttpRequest or Fetch API.
βοΈ JSONP vs. CORS
While JSONP is useful for making cross-domain requests, it's not the only solution. Cross-Origin Resource Sharing (CORS) is a more secure and flexible way to handle cross-origin requests.
CORS allows servers to specify who can access their resources and how they can be accessed, offering better control and security.
π Conclusion
JSONP is a useful technique for overcoming the same-origin policy and making cross-domain requests. Although it has its limitations and security concerns, it provides a simple way to fetch data from different domains without requiring CORS configuration.
Understanding how JSONP works and when to use it can help you in situations where cross-domain data fetching is necessary.
π¨βπ» Join our Community:
Author
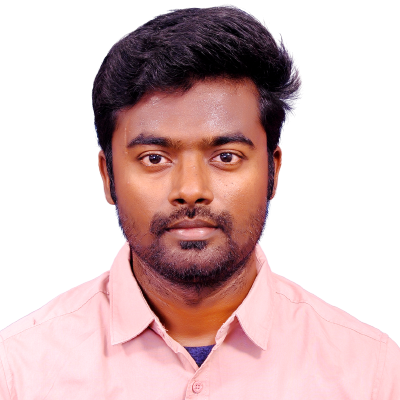
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JSONP), please comment here. I will help you immediately.