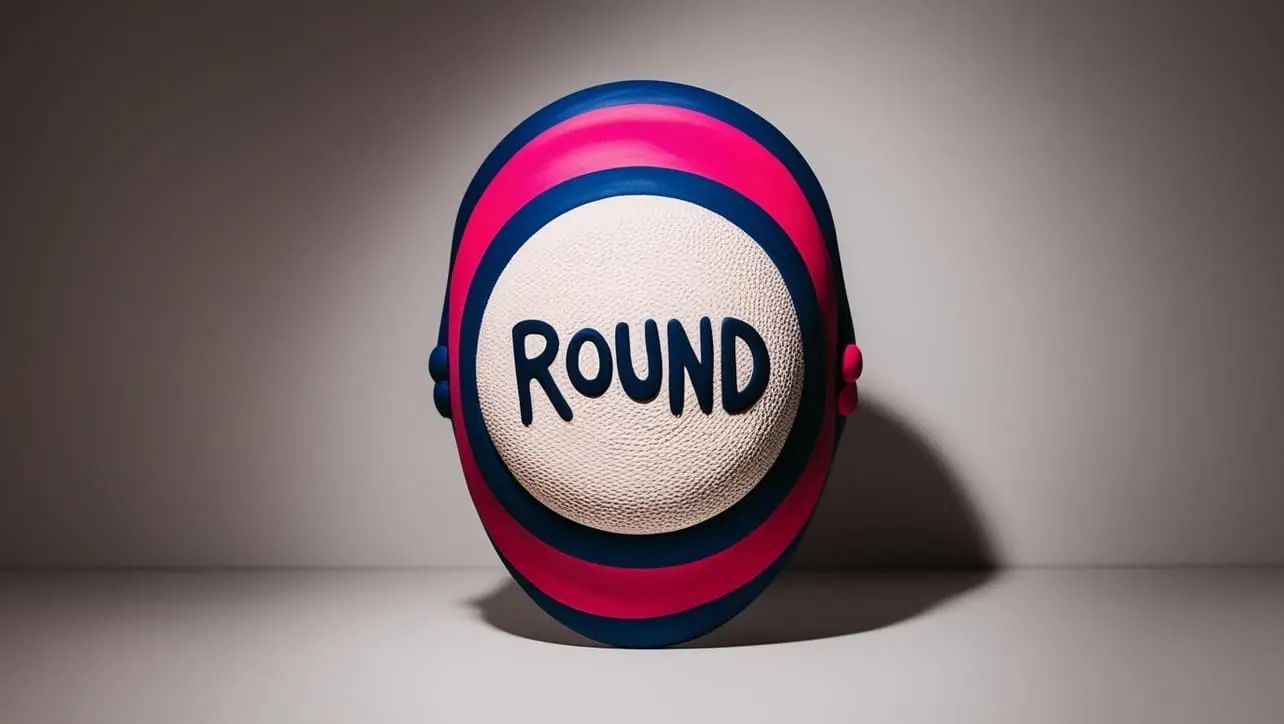
MongoDB Basic
MongoDB $accumulator Operator
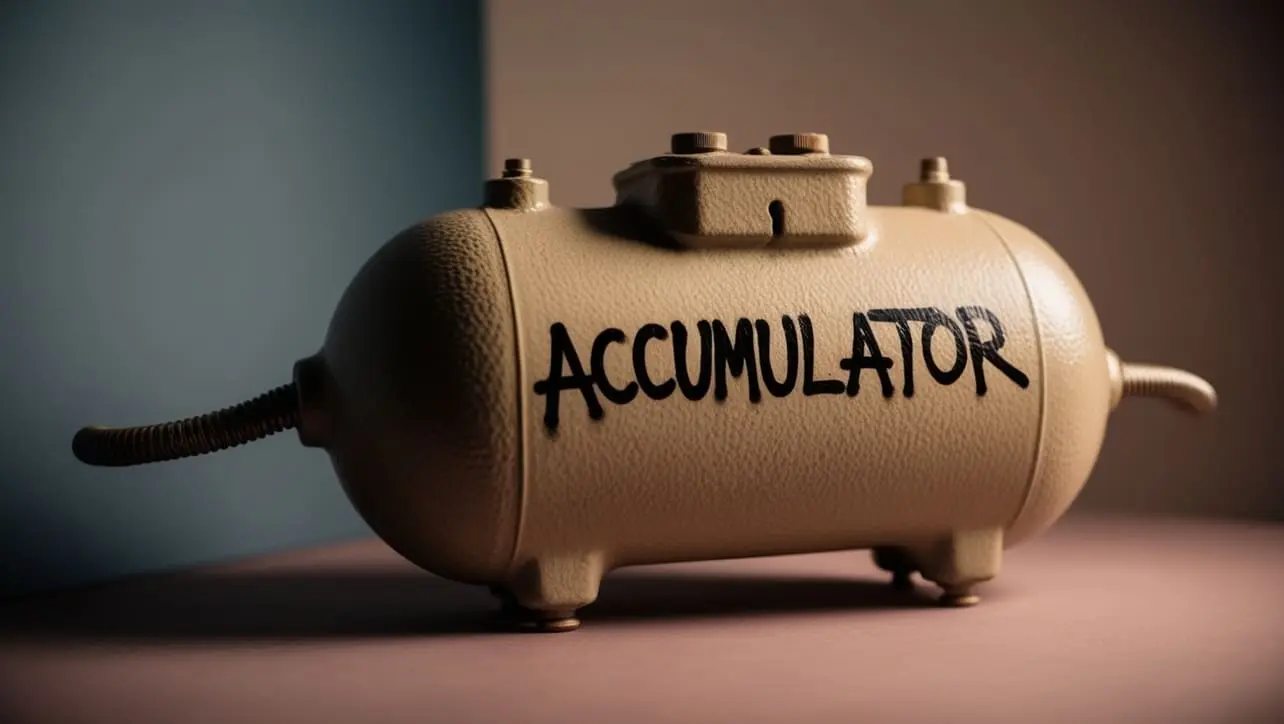
Photo Credit to CodeToFun
🙋 Introduction
In MongoDB's aggregation framework, the $accumulator
operator serves as a powerful tool for performing custom aggregation operations. This operator allows users to define and execute JavaScript functions within aggregation pipelines, enabling complex data transformations and computations.
Let's dive into the details of how the $accumulator
operator functions and its practical applications within MongoDB's aggregation pipelines.
💡 Syntax
The syntax for the $accumulator
method is straightforward:
{ $accumulator: {
init: <function>,
accumulate: <function>,
accumulateArgs: <array>,
merge: <function>,
finalize: <function>,
lang: <string>
}
}
- $accumulator: This operator indicates that the subsequent operation will involve custom accumulation.
- init: This is the initialization function that sets up the initial state of the accumulator.
- accumulate: This function accumulates values based on the specified logic.
- accumulateArgs: This array contains additional arguments for the accumulate function.
- merge: This function merges the intermediate states of accumulators.
- finalize: This function performs final processing on the accumulated result.
- lang: This specifies the language of the accumulator functions, typically "js" for JavaScript.
📝 Example
⌨️ Input
Consider a collection named transactions containing documents with fields amount representing transaction amounts. Here are sample documents from the transactions collection:
[
{ "_id": ObjectId("609c26812e9274a86871bc6a"), "amount": 100 },
{ "_id": ObjectId("609c26812e9274a86871bc6b"), "amount": 150 },
{ "_id": ObjectId("609c26812e9274a86871bc6c"), "amount": 200 }
]
🔄 Aggregation
Suppose we want to calculate the cumulative sum of the transaction amounts using a custom accumulator. Here's how you can achieve this using the $accumulator
operator:
db.transactions.aggregate([
{
$group: {
_id: null,
totalAmount: {
$accumulator: {
init: function() { return 0; },
accumulate: function(state, amount) { return state + amount; },
accumulateArgs: ["$amount"],
merge: function(state1, state2) { return state1 + state2; },
finalize: function(state) { return state; },
lang: "js"
}
}
}
}
])
🧩 Explanation
- $group: This stage groups all documents into a single group (since _id: null) for accumulation.
- $accumulator: This operator defines a custom accumulator to calculate the cumulative sum of transaction amounts.
- init: Initializes the accumulator state to 0.
- accumulate: Accumulates values by adding the current amount to the accumulator state.
- accumulateArgs: Specifies the field ($amount) to be passed as an argument to the accumulate function.
- merge: Merges intermediate states of accumulators (not applicable in this example).
- finalize: Performs final processing on the accumulated result (not applicable in this example).
- lang: Specifies the language of the accumulator functions as JavaScript.
When discussing how the above aggregation works:
- The total amount is the sum of all amount fields from the input documents, which is 100 + 150 + 200 = 450.
💻 Output
Now, let's take a look at the output generated by the aggregation pipeline:
{ "_id": null, "totalAmount": 450 }
📚 Use Cases
Complex Aggregations:
The
$accumulator
operator allows for the execution of complex aggregation logic that cannot be expressed using standard aggregation stages.Custom Transformations:
Users can define custom accumulator functions to perform specific data transformations or computations within aggregation pipelines.
Advanced Analytics:
Advanced analytics tasks, such as cumulative sum calculations, moving averages, or weighted averages, can be achieved using custom accumulators.
🎉 Conclusion
The $accumulator
operator in MongoDB's aggregation framework provides a flexible and powerful mechanism for executing custom aggregation logic within pipelines. Whether you need to perform complex calculations, implement custom transformations, or conduct advanced analytics, mastering the usage of $accumulator
empowers you to achieve sophisticated data processing tasks with ease.
With its expressive syntax and extensive capabilities, the $accumulator
operator unlocks new possibilities for data manipulation and analysis within MongoDB. Incorporate it into your aggregation pipelines to tackle complex aggregation challenges and extract meaningful insights from your datasets.
👨💻 Join our Community:
Author
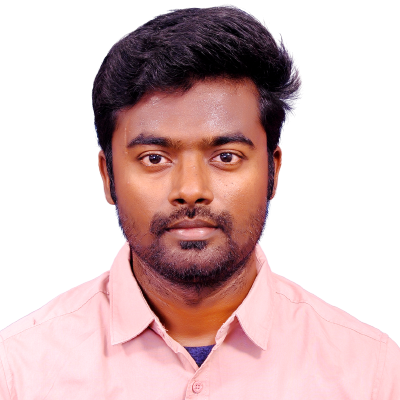
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (MongoDB $accumulator Operator), please comment here. I will help you immediately.