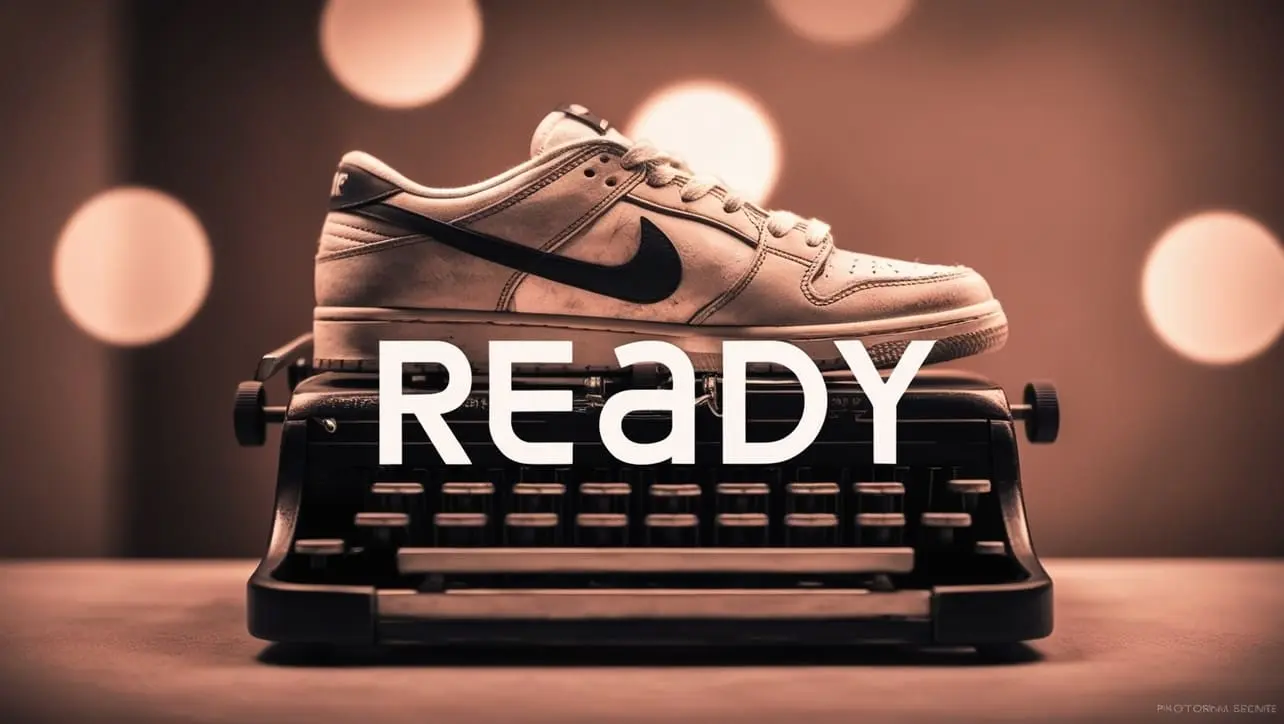
jQuery Topics
- jQuery Introduction
- jQuery Callbacks
- jQuery deferred
- jQuery selectors
- jQuery Ajax Events
- jQuery Ajax Methods
- jQuery Keyboard Events
- jQuery Keyboard Methods
- jQuery Form Events
- jQuery Form Methods
- jQuery Mouse Events
- jQuery Mouse Methods
- jQuery Event Properties
- jQuery Event Methods
- jQuery HTML
- jQuery CSS
- jQuery Fading
- jQuery Traversing
- jQuery Utilities
- jQuery Properties
jQuery .size() Method
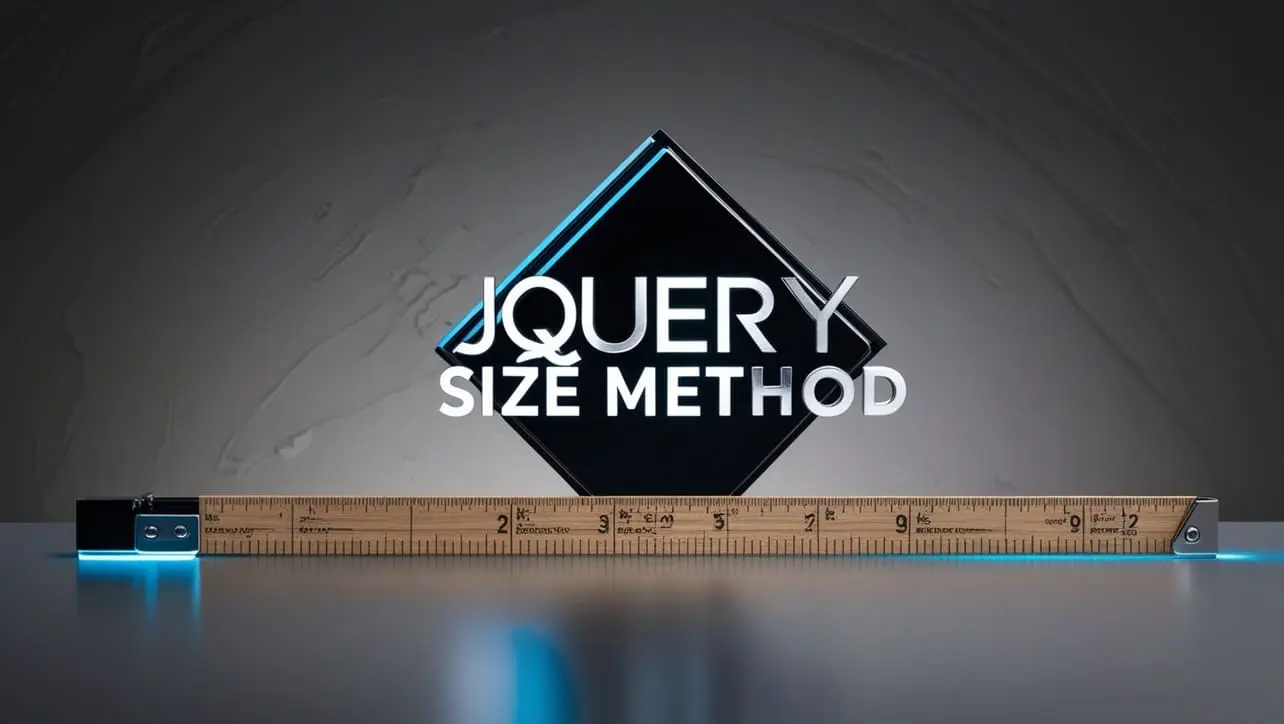
Photo Credit to CodeToFun
🙋 Introduction
In jQuery, the .size()
method serves as a handy tool for determining the number of elements in a jQuery object. This method provides a simple and efficient way to obtain the size of a collection of DOM elements selected by a jQuery selector.
In this guide, we'll explore the functionality of the .size()
method and demonstrate its usage through practical examples.
🧠 Understanding .size() Method
The .size()
method returns the number of elements contained within the jQuery object to which it is applied. It effectively provides a count of the matched elements, allowing you to perform actions based on the size of the selection.
💡 Syntax
The syntax for the .size()
method is straightforward:
$(selector).size()
$(selector).length
(Note: .length is an alternative to .size()
and provides the same functionality.)
📝 Example
Determining the Size of a Selection:
Consider a scenario where you want to determine the number of paragraphs (<p>) in a document:
example.jsCopiedvar paragraphCount = $("p").size(); console.log("Number of paragraphs: " + paragraphCount);
This will log the number of paragraphs in the document to the console.
Conditionally Executing Code Based on Selection Size:
You can use the
.size()
method to execute code conditionally based on the size of the selection. For instance, let's check if there are any list items (<li>) in an unordered list (<ul>) and display a message accordingly:example.jsCopiedif ($("ul li").size() > 0) { console.log("List contains items."); } else { console.log("List is empty."); }
This will log either List contains items. or List is empty. based on the presence or absence of list items.
Combining with Other Methods:
The
.size()
method can be combined with other jQuery methods for more complex operations. For example, you can use it with .each() to iterate over a collection of elements:example.jsCopied$("div").each(function(index) { console.log("Index: " + index + ", Size: " + $(this).size()); });
This will log the index and size of each <div> element in the document.
🎉 Conclusion
The jQuery .size()
method provides a convenient way to determine the size of a jQuery selection, allowing you to perform various actions based on the number of matched elements.
Whether you need to count elements, execute code conditionally, or iterate over a collection, the .size()
method proves to be a valuable asset in your jQuery toolkit.
👨💻 Join our Community:
Author
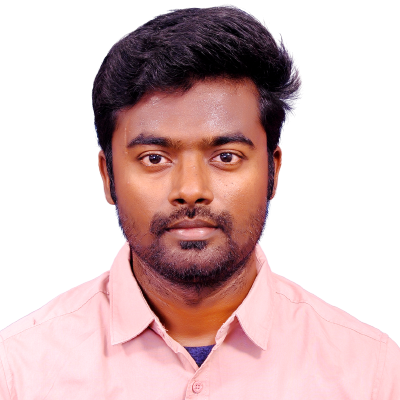
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery .size() Method), please comment here. I will help you immediately.