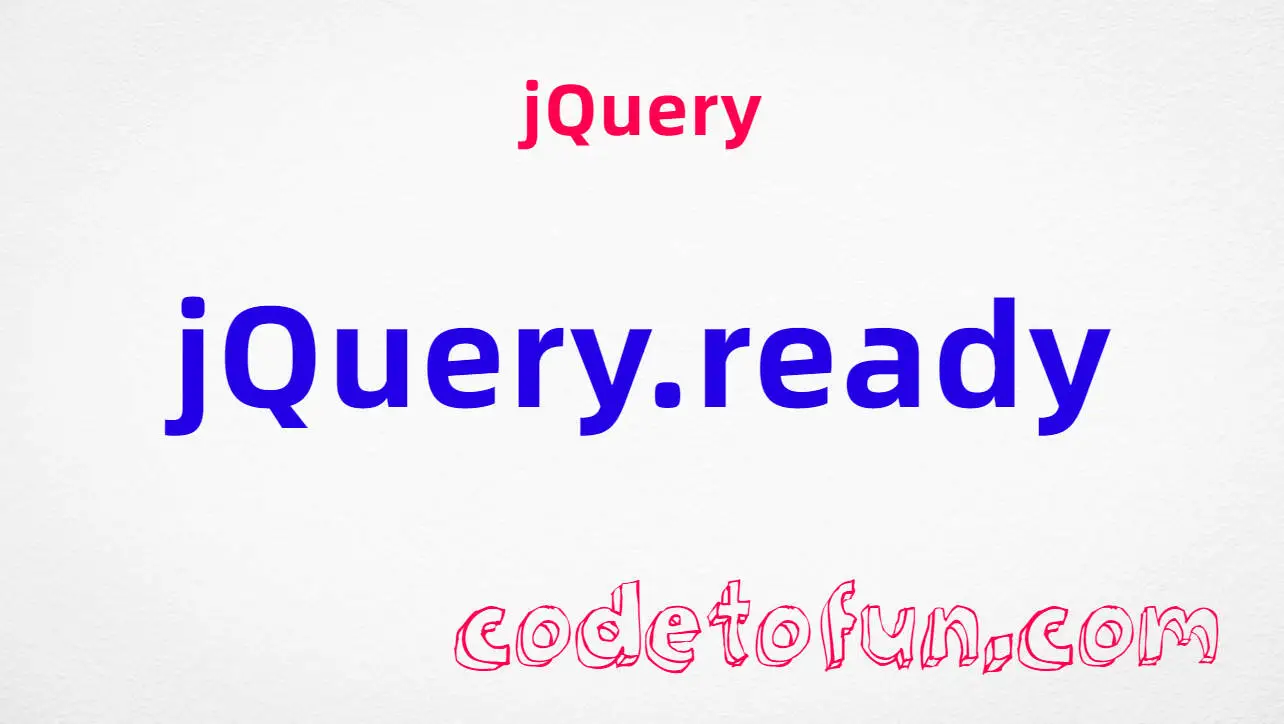
jQuery Basic
jQuery Selectors
- jQuery Selectors
- jQuery *
- jQuery :animated
- jQuery [name|=”value”]
- jQuery [name*=”value”]
- jQuery [name~=”value”]
- jQuery [name$=”value”]
- jQuery [name=”value”]
- jQuery [name!=”value”]
- jQuery [name^=”value”]
- jQuery :button
- jQuery :checkbox
- jQuery :checked
- jQuery Child Selector
- jQuery .class
- jQuery :contains()
- jQuery Descendant Selector
- jQuery :disabled
- jQuery Element
- jQuery :empty
- jQuery :enabled
- jQuery :eq()
- jQuery :even
- jQuery :file
- jQuery :first-child
- jQuery :first-of-type
- jQuery :first
- jQuery :focus
- jQuery :gt()
- jQuery Has Attribute
- jQuery :has()
- jQuery :header
- jQuery :hidden
- jQuery #id
- jQuery :image
- jQuery :input
- jQuery :lang()
- jQuery :last-child
- jQuery :last-of-type
- jQuery :last
- jQuery :lt()
- jQuery [name=”value”][name2=”value2″]
- jQuery (“selector1, selector2, selectorN”)
- jQuery (“prev + next”)
- jQuery (“prev ~ siblings”)
- jQuery :not()
- jQuery :nth-child()
- jQuery :nth-last-child()
- jQuery :nth-last-of-type()
- jQuery :nth-of-type()
- jQuery :odd
- jQuery :only-child
- jQuery :only-of-type
- jQuery :parent
- jQuery :password
- jQuery :radio
- jQuery :reset
- jQuery :root
- jQuery :selected
- jQuery :submit
- jQuery :target
- jQuery :text
- jQuery :visible
jQuery :only-child Selector
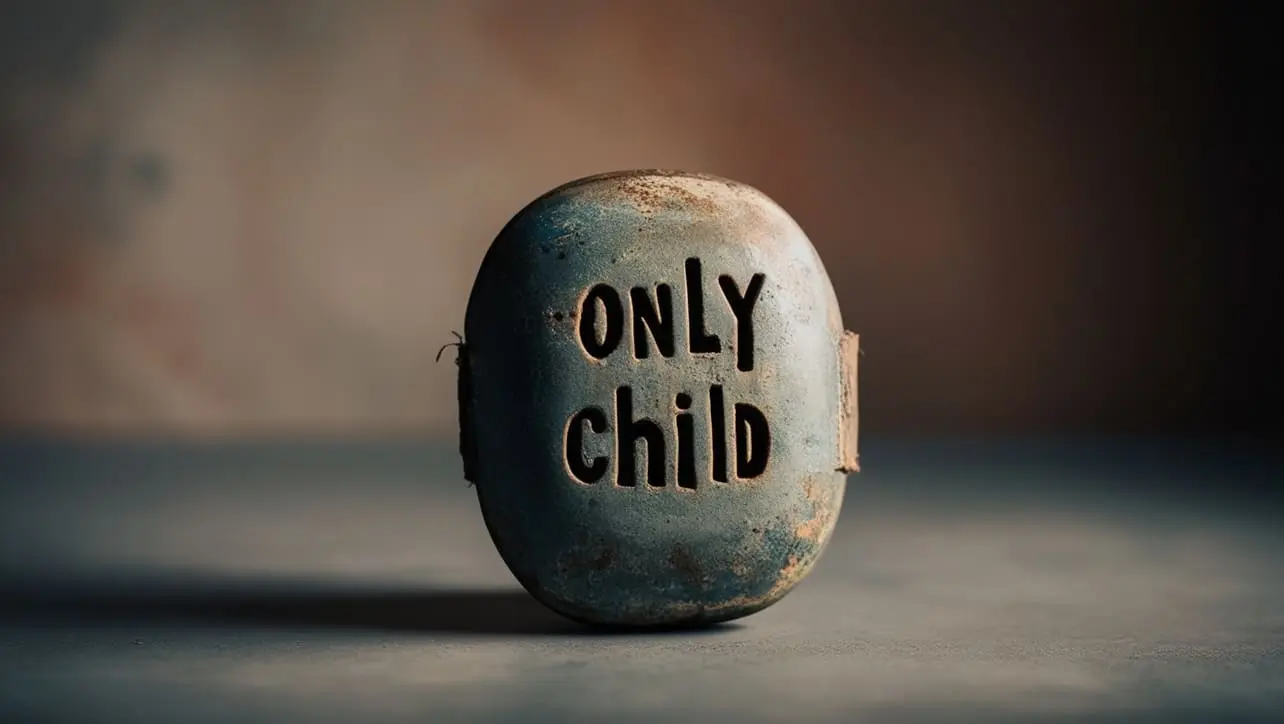
Photo Credit to CodeToFun
🙋 Introduction
In web development, jQuery offers a plethora of selectors to target specific elements within a document, facilitating dynamic interactions and styling. Among these selectors, the :only-child
selector stands out for its ability to target elements that are the only child of their parent. Understanding and leveraging this selector can streamline your jQuery code and enhance your website's functionality.
This guide explores the jQuery :only-child
selector with practical examples to illustrate its utility.
🧠 Understanding :only-child Selector
The :only-child
selector in jQuery allows you to target elements that are the only child of their parent element. This means that the selected elements have no siblings within the same parent container. It is particularly useful when you want to apply styles or perform actions exclusively on such singular elements.
💡 Syntax
The syntax for the :only-child
selector is straightforward:
$(":only-child")
📝 Example
Selecting Elements that are Only Child:
Consider a scenario where you have a list of items, and you want to select and style the <li> elements that are the only child within their respective <ul> containers:
index.htmlCopied<ul> <li>Item 1</li> <li>Item 2</li> </ul> <ul> <li>Single Item</li> </ul>
example.jsCopied$("ul li:only-child").css("font-weight", "bold");
This will make the <li> containing Single Item bold, as it is the only child within its parent <ul>.
Applying Styles to Sole Children in a Table:
Suppose you have a table structure, and you want to highlight cells that are the only children within their respective rows:
index.htmlCopied<table> <tr> <td>Cell 1</td> <td>Cell 2</td> </tr> <tr> <td>Only Cell</td> </tr> </table>
example.jsCopied$("tr td:only-child").css("background-color", "lightblue");
This will set the background color of the cell containing Only Cell to light blue.
Adding Functionality to Exclusive Elements:
You can also bind events or perform actions on elements that are the sole children within their parent containers. For instance, let's alert a message when the only <div> within a <section> is clicked:
index.htmlCopied<section> <div>Exclusive Content</div> </section>
example.jsCopied$("section div:only-child").click(function() { alert("You clicked the exclusive content!"); });
🎉 Conclusion
The jQuery :only-child
selector provides a convenient way to target elements that are the sole children within their parent containers. Whether you need to style, manipulate, or add functionality to such exclusive elements, this selector offers a straightforward solution.
By incorporating it into your jQuery toolkit, you can enhance the interactivity and aesthetics of your web pages with ease.
👨💻 Join our Community:
Author
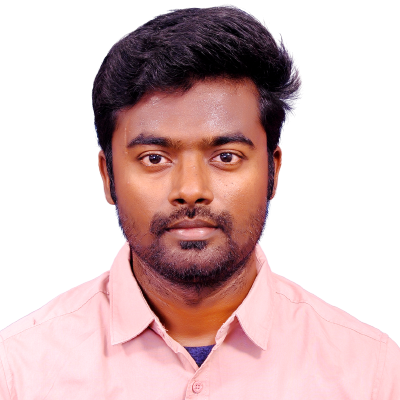
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery :only-child Selector), please comment here. I will help you immediately.