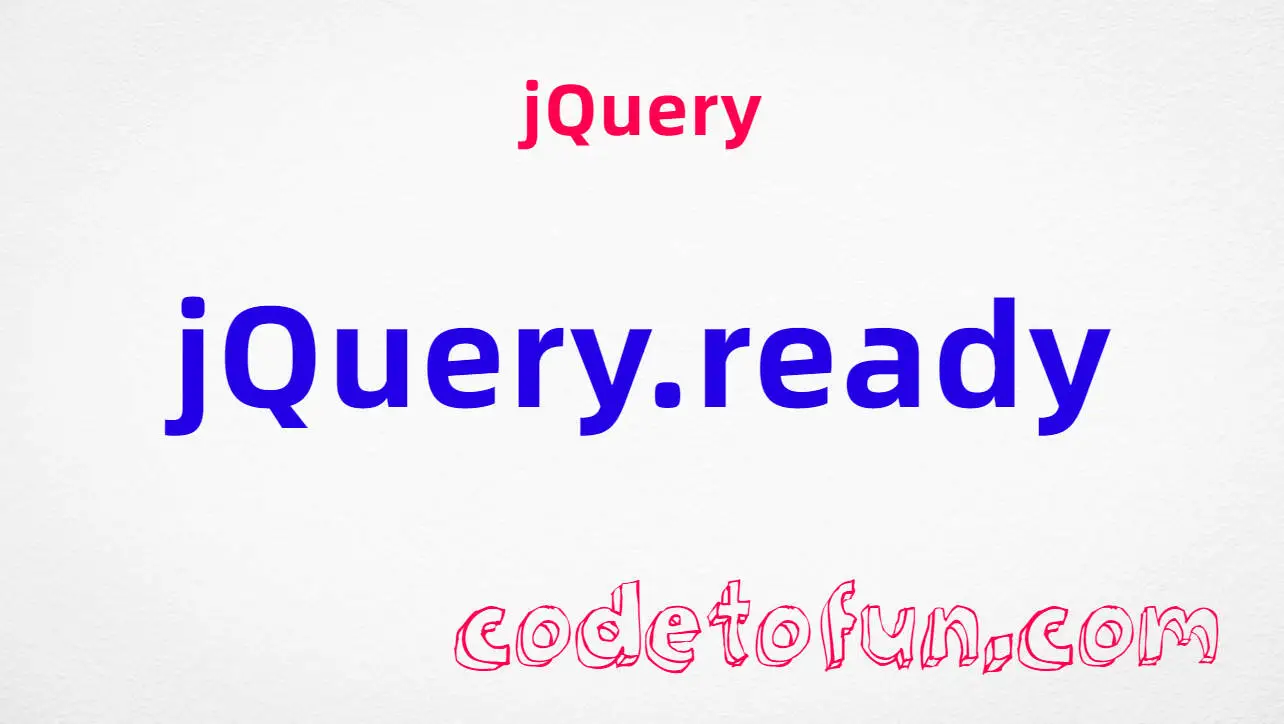
jQuery Basic
jQuery Deferred
- jQuery Deferred
- deferred.always()
- deferred.catch()
- deferred.done()
- deferred.fail()
- deferred.isRejected()
- deferred.isResolved()
- deferred.notify()
- deferred.notifyWith()
- deferred.pipe()
- deferred.progress()
- deferred.promise()
- deferred.reject()
- deferred.rejectWith()
- deferred.resolve()
- deferred.resolveWith()
- deferred.state()
- deferred.then()
- jQuery.Deferred()
- jQuery.when()
- .promise()
jQuery jQuery.when() Method
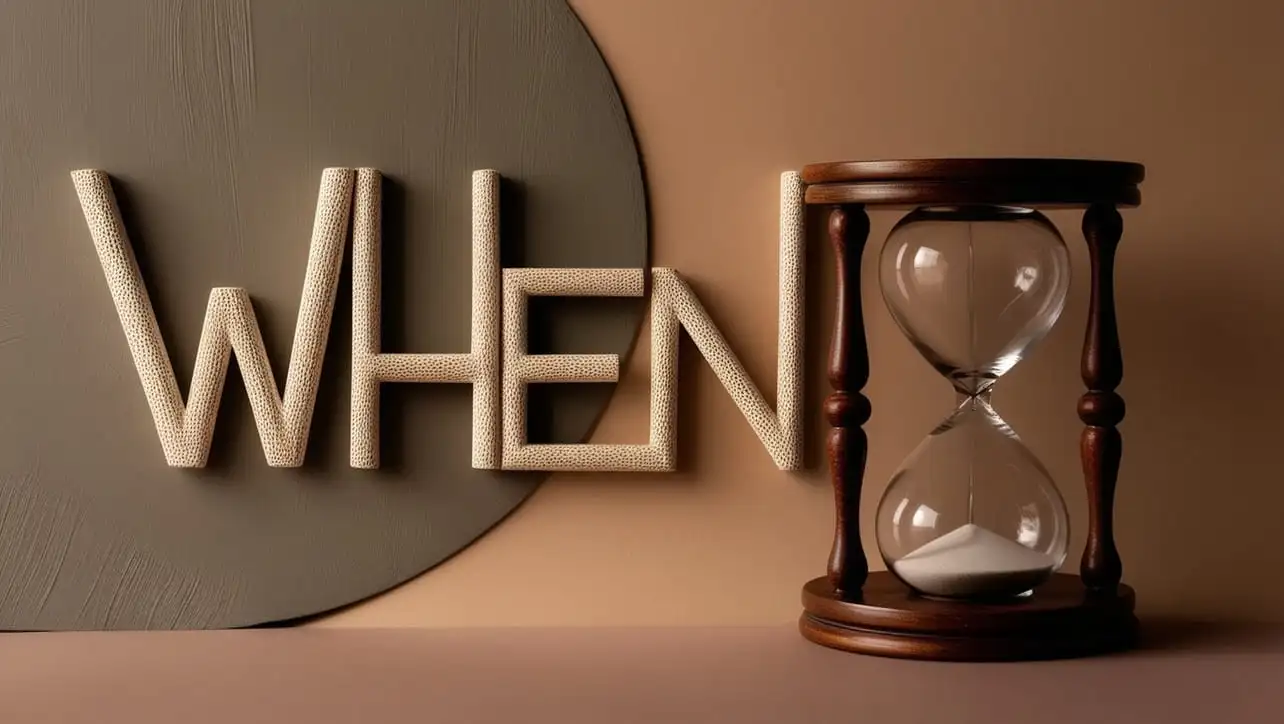
Photo Credit to CodeToFun
🙋 Introduction
Asynchronous programming is a fundamental aspect of modern web development, enabling developers to execute tasks concurrently and improve user experience. jQuery offers a versatile solution for managing asynchronous operations through its jQuery.when()
method.
In this guide, we'll explore the jQuery.when()
method in depth, uncovering its syntax, usage patterns, and practical applications.
🧠 Understanding jQuery.when() Method
The jQuery.when()
method is a powerful tool for synchronizing and coordinating multiple asynchronous operations in JavaScript. It allows you to execute a callback function only when all specified deferred objects have either succeeded or failed.
💡 Syntax
The syntax for the jQuery.when()
method is straightforward:
jQuery.when( deferred1 [, deferred2 [, ... ] ] )
.done( function1 [, function2 [, ... ] ] )
.fail( function1 [, function2 [, ... ] ] );
- deferred1, deferred2, ...: One or more deferred objects representing asynchronous tasks.
- function1, function2, ...: Callback functions to execute when all deferred objects are resolved successfully (.done()) or when any of them is rejected (.fail()).
📝 Example
Let's dive into a simple example to illustrate the usage of the jQuery.when()
method:
var request1 = $.ajax({
url: "example.com/api",
method: "GET"
});
var request2 = $.ajax({
url: "example.com/another_api",
method: "GET"
});
jQuery.when(request1, request2)
.done(function(response1, response2) {
// Process both responses
})
.fail(function(xhr, status, error) {
// Handle any failed requests
console.error("Request failed:", status, error);
});
🏆 Best Practices
When working with the jQuery.when()
method, consider the following best practices:
Error Handling:
Implement error handling logic within the .fail() callback to gracefully manage any failures or errors in the asynchronous operations.
Promise Chaining:
Chain additional methods, such as .then(), onto the
jQuery.when()
method to execute further actions based on the aggregated results.Parameter Handling:
Understand the order and structure of parameters passed to the .done() callback function, ensuring proper handling of responses or data.
Performance Considerations:
Be mindful of performance implications when synchronizing multiple asynchronous tasks, especially in scenarios involving large datasets or complex computations.
Testing:
Thoroughly test the behavior of your code under various conditions, including different combinations of successful and failed asynchronous operations.
📚 Use Cases
Parallel AJAX Requests:
Execute multiple AJAX requests simultaneously and process the responses collectively.
Dependency Management:
Ensure that dependent asynchronous tasks, such as data loading or initialization, are completed before proceeding.
Animation Sequencing:
Synchronize animations or transitions on a webpage to create cohesive user experiences.
Promise Aggregation:
Consolidate promises from various sources and execute a callback once all promises are resolved.
Resource Loading:
Load multiple external resources, such as scripts or stylesheets, and trigger actions once all resources are loaded.
🎉 Conclusion
The jQuery.when()
method empowers developers to orchestrate and synchronize asynchronous tasks with ease, enhancing the efficiency and responsiveness of web applications.
By mastering its syntax, leveraging common use cases, and adhering to best practices, you can harness the full potential of asynchronous programming in JavaScript. Incorporate jQuery.when()
into your development workflow to build dynamic and interactive web experiences that delight users and streamline workflows.
👨💻 Join our Community:
Author
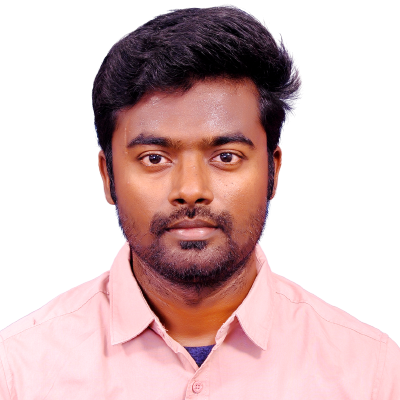
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery jQuery.when() Method), please comment here. I will help you immediately.