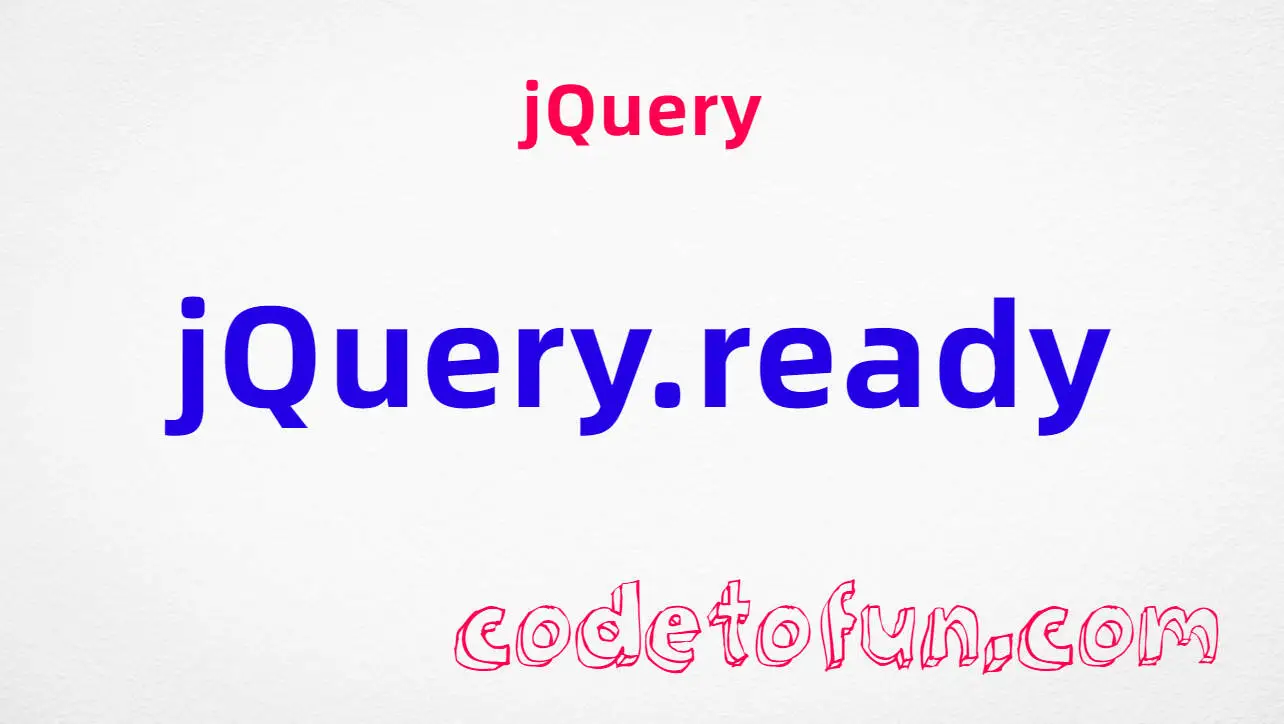
jQuery Basic
jQuery Deferred
- jQuery Deferred
- deferred.always()
- deferred.catch()
- deferred.done()
- deferred.fail()
- deferred.isRejected()
- deferred.isResolved()
- deferred.notify()
- deferred.notifyWith()
- deferred.pipe()
- deferred.progress()
- deferred.promise()
- deferred.reject()
- deferred.rejectWith()
- deferred.resolve()
- deferred.resolveWith()
- deferred.state()
- deferred.then()
- jQuery.Deferred()
- jQuery.when()
- .promise()
jQuery deferred.resolveWith() Method
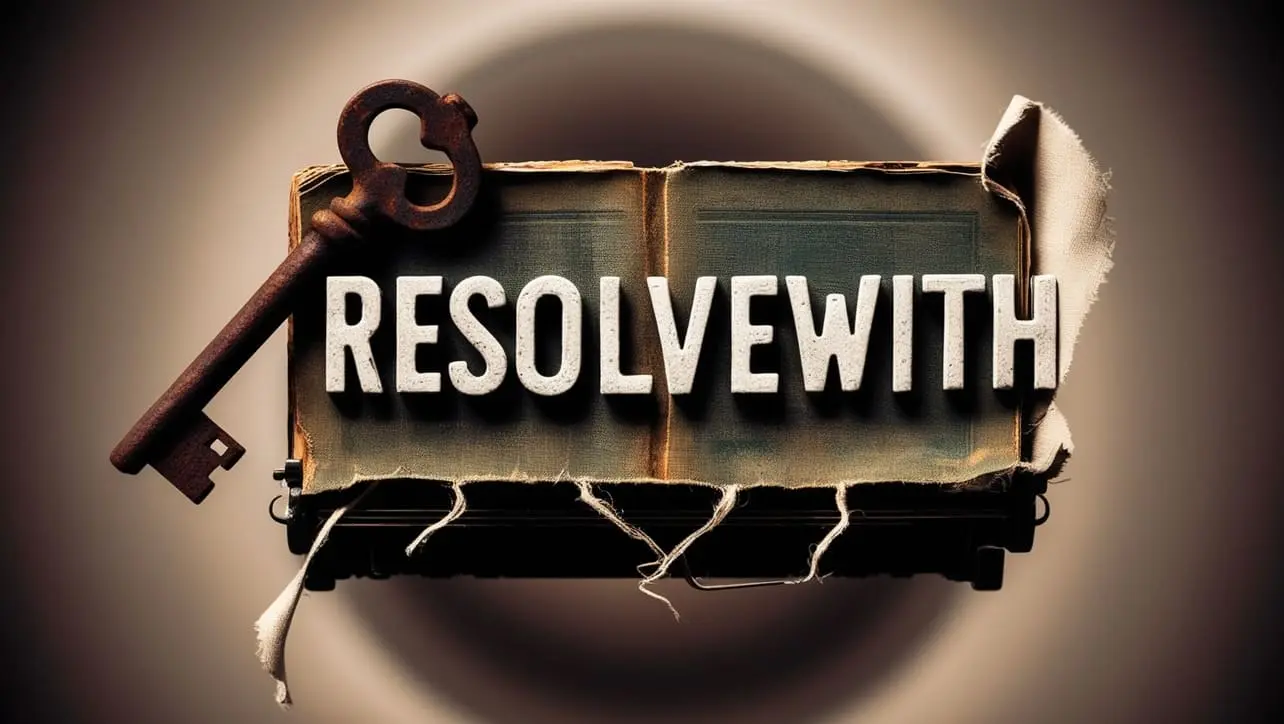
Photo Credit to CodeToFun
🙋 Introduction
In the realm of asynchronous JavaScript programming, managing the flow of execution and coordinating multiple tasks is paramount. jQuery's Deferred Object API offers a suite of methods to streamline this process, among which the deferred.resolveWith()
method stands out. This method enables developers to control the resolution of deferred objects with precision, empowering them to orchestrate complex asynchronous operations effortlessly.
Join us as we delve into the intricacies of deferred.resolveWith()
, exploring its syntax, applications, and best practices.
🧠 Understanding deferred.resolveWith() Method
The deferred.resolveWith()
method is a fundamental component of jQuery's Deferred Object API, designed to trigger the successful resolution of a deferred object and execute associated success callbacks with a specific context (this value) and optional arguments.
💡 Syntax
The syntax for the deferred.resolveWith()
method is straightforward:
deferred.resolveWith( context [, args ] )
- context: The context (i.e., the value of this) in which success callbacks should be executed.
- args (optional): An array or array-like object containing arguments to be passed to success callbacks.
📝 Example
Let's dive into a simple example to illustrate the usage of the deferred.resolveWith()
method:
var deferred = $.Deferred();
function successCallback(value) {
console.log("Success:", value);
}
deferred.resolveWith({
message: "Hello"
}, ["World"]);
deferred.done(successCallback); // Output: Success: World
🏆 Best Practices
When working with the deferred.resolveWith()
method, consider the following best practices:
Contextual Execution:
Choose the appropriate context for success callbacks using
deferred.resolveWith()
to ensure that callback functions operate within the desired scope.Parameter Passing:
Pass relevant data or arguments to success callbacks via the optional args parameter, facilitating seamless communication between asynchronous tasks.
Error Handling:
Implement complementary error handling mechanisms alongside
deferred.resolveWith()
to gracefully manage exceptional conditions and ensure the reliability of your applications.Code Organization:
Structure your code in a modular and cohesive manner, leveraging
deferred.resolveWith()
to encapsulate asynchronous logic and promote code reusability and maintainability.Documentation:
Document usage patterns and conventions surrounding
deferred.resolveWith()
within your codebase to facilitate collaboration and enhance code comprehension for future developers.
📚 Use Cases
AJAX Requests:
Utilize
deferred.resolveWith()
to handle successful responses from AJAX requests, facilitating seamless data retrieval and integration into your web applications.Promise Chains:
Integrate
deferred.resolveWith()
within promise chains to propagate resolved values with custom contexts, enabling more flexible and expressive asynchronous workflows.Event Handling:
Employ
deferred.resolveWith()
in event-driven architectures to trigger success callbacks with specific contexts and arguments, enhancing event handling capabilities.Animation Sequences:
Coordinate animations and transitions on webpages by leveraging
deferred.resolveWith()
to synchronize the execution of callbacks within animation sequences.Data Processing Pipelines:
Construct robust data processing pipelines using
deferred.resolveWith()
to propagate intermediate results and trigger subsequent processing steps with appropriate contexts and parameters.
🎉 Conclusion
jQuery's deferred.resolveWith()
method empowers developers to orchestrate asynchronous workflows with finesse, enabling precise control over the resolution of deferred objects and the execution of success callbacks.
By mastering its syntax, exploring diverse applications, and adhering to best practices, you can leverage deferred.resolveWith()
to craft robust and responsive web applications that excel in handling complex asynchronous tasks. Incorporate this powerful method into your toolkit to unlock new possibilities in asynchronous JavaScript programming and elevate the quality of your code.
👨💻 Join our Community:
Author
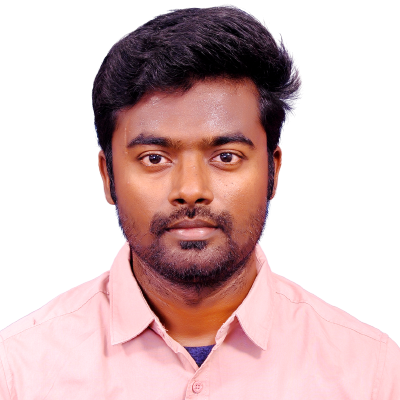
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery deferred.resolveWith() Method), please comment here. I will help you immediately.