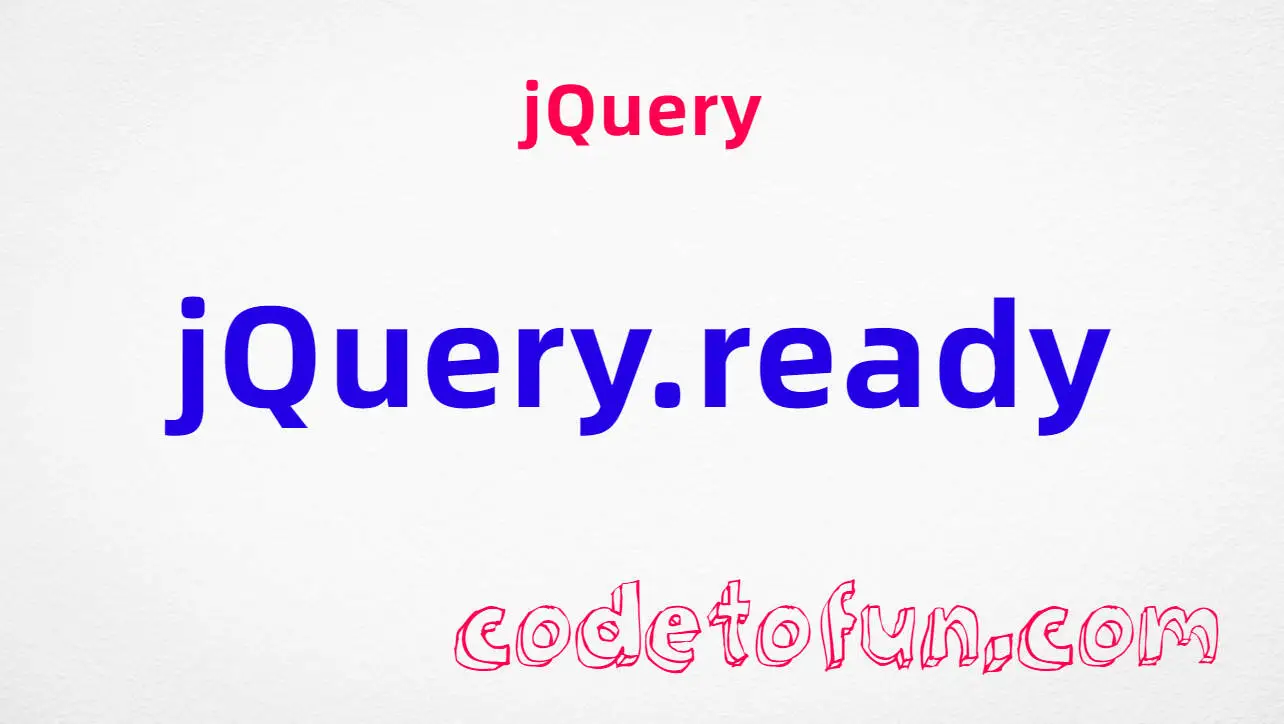
jQuery Basic
jQuery Deferred
- jQuery Deferred
- deferred.always()
- deferred.catch()
- deferred.done()
- deferred.fail()
- deferred.isRejected()
- deferred.isResolved()
- deferred.notify()
- deferred.notifyWith()
- deferred.pipe()
- deferred.progress()
- deferred.promise()
- deferred.reject()
- deferred.rejectWith()
- deferred.resolve()
- deferred.resolveWith()
- deferred.state()
- deferred.then()
- jQuery.Deferred()
- jQuery.when()
- .promise()
jQuery deferred.progress() Method
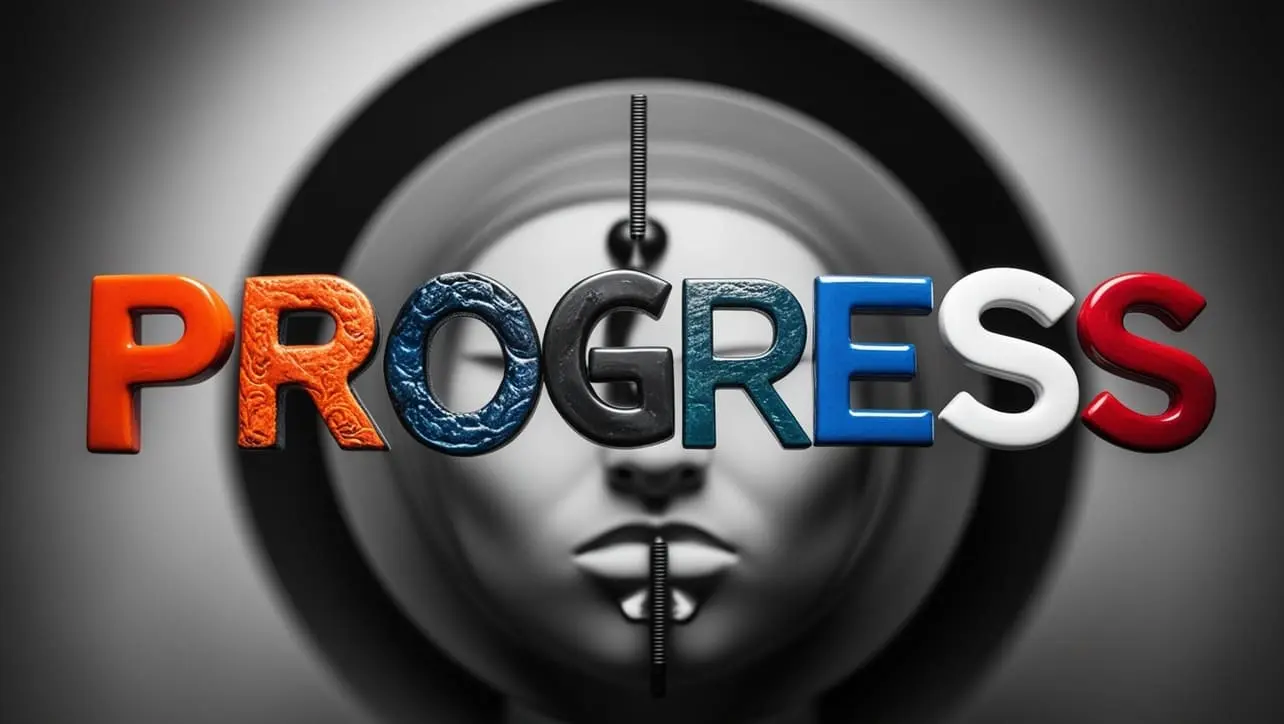
Photo Credit to CodeToFun
🙋 Introduction
Asynchronous programming plays a vital role in modern web development, enabling the creation of responsive and dynamic user interfaces. jQuery offers a suite of tools to manage asynchronous operations effectively, including the deferred.progress()
method.
In this guide, we'll explore the deferred.progress()
method, its purpose, syntax, and practical applications in building robust asynchronous workflows.
🧠 Understanding deferred.progress() Method
The deferred.progress()
method is part of jQuery's Deferred Object API, designed to manage asynchronous operations such as AJAX requests, animations, and timeouts. Unlike deferred.done() and deferred.fail(), which handle success and error states respectively, deferred.progress()
allows you to track the progress of an asynchronous operation.
💡 Syntax
The syntax for the deferred.progress()
method is straightforward:
deferred.progress( progressHandler )
- progressHandler: A function that is executed when the deferred object notifies progress updates. It can accept arguments providing information about the progress.
📝 Example
Let's dive into a simple example to illustrate the usage of the deferred.progress()
method:
var deferred = $.Deferred();
deferred.progress(function(progressData) {
console.log("Progress:", progressData);
});
// Simulating a long-running operation
setTimeout(function() {
deferred.notify("50% complete");
}, 1000);
setTimeout(function() {
deferred.notify("90% complete");
}, 2000);
setTimeout(function() {
deferred.resolve("Operation completed successfully");
}, 3000);
🏆 Best Practices
When working with the deferred.progress()
method, consider the following best practices:
Granular Updates:
Notify progress with sufficient granularity to keep users informed without overwhelming them with excessive notifications.
Consistent Feedback:
Provide consistent feedback throughout the duration of an operation, ensuring users remain engaged and informed.
Clear Messaging:
Use descriptive messages to convey progress updates effectively, avoiding ambiguity or confusion.
Graceful Handling:
Handle scenarios where progress notifications may not be applicable or relevant, ensuring graceful degradation of functionality.
Performance Considerations:
Be mindful of performance implications, especially in scenarios involving frequent progress updates or large datasets.
📚 Use Cases
Long-running Operations:
Track the progress of time-consuming tasks such as file uploads, data processing, or complex calculations to provide feedback to users.
Multi-step Processes:
Monitor the progress of multi-step processes or workflows, updating the user interface dynamically as each step is completed.
Real-time Updates:
Implement real-time updates in web applications, such as live chat systems or collaborative editing environments, by notifying clients of changes as they occur.
Loading Indicators:
Display loading indicators or progress bars to indicate ongoing background processes, enhancing the user experience during resource-intensive operations.
🎉 Conclusion
The deferred.progress()
method in jQuery offers a powerful mechanism for tracking the progress of asynchronous operations in web development.
By incorporating it into your projects, you can create responsive and intuitive user experiences, keeping users informed and engaged during long-running tasks or multi-step processes. Experiment with deferred.progress()
to unlock new possibilities in asynchronous workflows and elevate the interactivity of your web applications.
👨💻 Join our Community:
Author
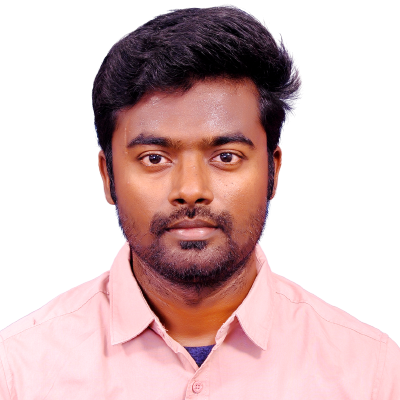
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery deferred.progress() Method), please comment here. I will help you immediately.