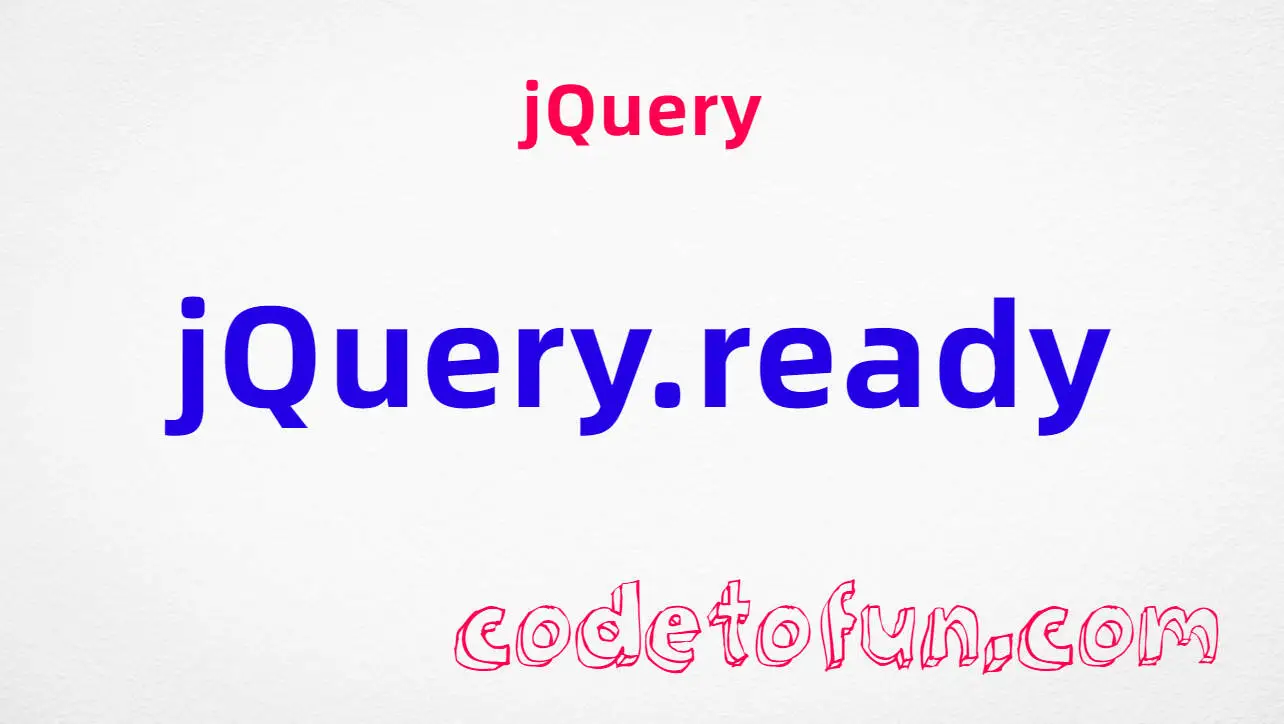
jQuery Basic
jQuery Deferred
- jQuery Deferred
- deferred.always()
- deferred.catch()
- deferred.done()
- deferred.fail()
- deferred.isRejected()
- deferred.isResolved()
- deferred.notify()
- deferred.notifyWith()
- deferred.pipe()
- deferred.progress()
- deferred.promise()
- deferred.reject()
- deferred.rejectWith()
- deferred.resolve()
- deferred.resolveWith()
- deferred.state()
- deferred.then()
- jQuery.Deferred()
- jQuery.when()
- .promise()
jQuery deferred.pipe() Method
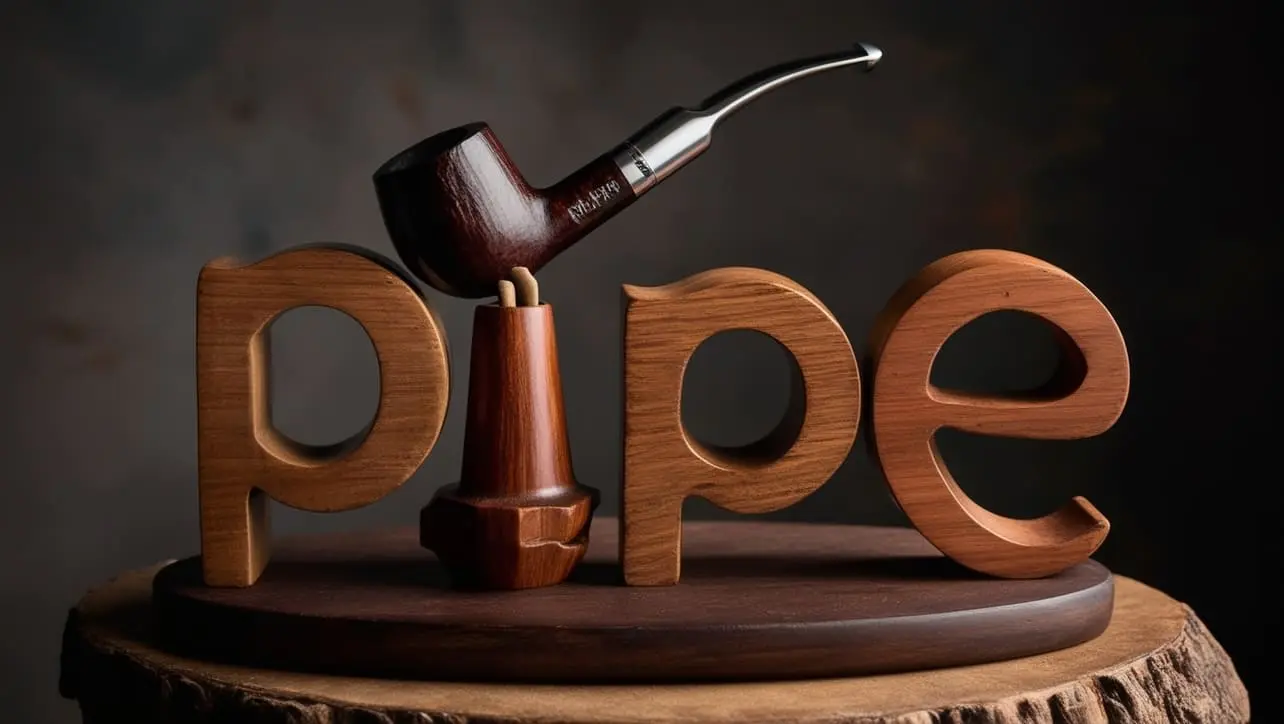
Photo Credit to CodeToFun
🙋 Introduction
In the realm of asynchronous JavaScript programming, managing complex workflows can be challenging. Fortunately, jQuery provides a versatile toolkit to simplify these tasks. One such tool is the deferred.pipe()
method, which enables developers to streamline asynchronous operations by chaining promises in a concise and elegant manner.
In this comprehensive guide, we'll delve into the intricacies of the deferred.pipe()
method, exploring its syntax, practical applications, and best practices.
🧠 Understanding deferred.pipe() Method
The deferred.pipe()
method is a valuable addition to jQuery's Deferred Object API, designed to enhance the management of asynchronous workflows. It allows developers to chain multiple deferred objects together, passing the results of one operation as input to the next, creating a seamless pipeline of asynchronous tasks.
💡 Syntax
The syntax for the deferred.pipe()
method is straightforward:
deferred.pipe( doneFilter [, failFilter [, progressFilter ] ] )
- doneFilter: A function that is executed when the deferred object is resolved successfully. It accepts the result of the previous operation as its argument and returns a new value or promise.
- failFilter (Optional): A function that is executed when the deferred object encounters an error. It allows for handling and transforming errors within the pipeline.
- progressFilter (Optional): A function that is executed when the deferred object notifies progress. This is typically used for reporting the progress of long-running operations.
📝 Example
Let's dive into a simple example to illustrate the usage of the deferred.pipe()
method:
// Example 1: Chaining AJAX requests
$.ajax({
url: "example.com/data",
method: "GET"
}).pipe(function(data) {
// Process the data and return a modified result
return $.ajax({
url: "example.com/process",
method: "POST",
data: data
});
}).done(function(response) {
// Handle the final result
}).fail(function(xhr, status, error) {
// Handle errors
});
// Example 2: Chaining promises
firstAsyncOperation().pipe(function(result) {
// Process the result and return a new promise
return secondAsyncOperation(result);
}).done(function(finalResult) {
// Handle the final result
}).fail(function(error) {
// Handle errors
});
🏆 Best Practices
When working with the deferred.pipe()
method, consider the following best practices:
Modularization:
Break down complex workflows into smaller, modular functions, making it easier to compose and maintain asynchronous pipelines.
Error Propagation:
Ensure that errors are propagated through the pipeline appropriately, allowing for centralized error handling and debugging.
Testing:
Thoroughly test each step of the pipeline to validate functionality and identify potential edge cases or failure scenarios.
Documentation:
Document the purpose and behavior of each step in the pipeline, along with any assumptions or requirements, to aid understanding and collaboration.
📚 Use Cases
Sequential Operations:
Perform a series of asynchronous tasks sequentially, passing the result of each operation to the next one in the pipeline.
Data Transformation:
Transform data or perform additional processing on the results of asynchronous operations before proceeding to the next step.
Conditional Execution:
Conditionally execute asynchronous operations based on the result of previous tasks, allowing for dynamic and flexible workflows.
Error Handling:
Implement custom error handling logic within the pipeline to gracefully manage errors and failures.
🎉 Conclusion
The deferred.pipe()
method in jQuery empowers developers to create elegant and efficient asynchronous workflows, allowing for seamless integration of multiple operations.
By understanding its syntax, exploring common use cases, and following best practices, you can leverage deferred.pipe()
to streamline your asynchronous JavaScript code effectively. Incorporate this powerful tool into your toolkit to build robust and responsive web applications with ease.
👨💻 Join our Community:
Author
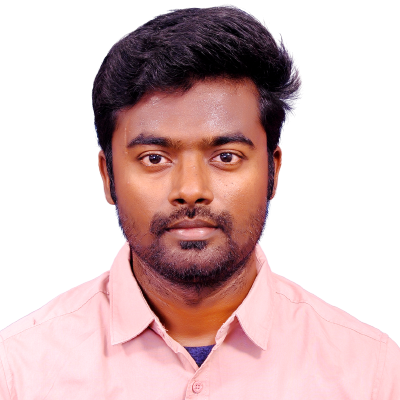
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (jQuery deferred.pipe() Method), please comment here. I will help you immediately.