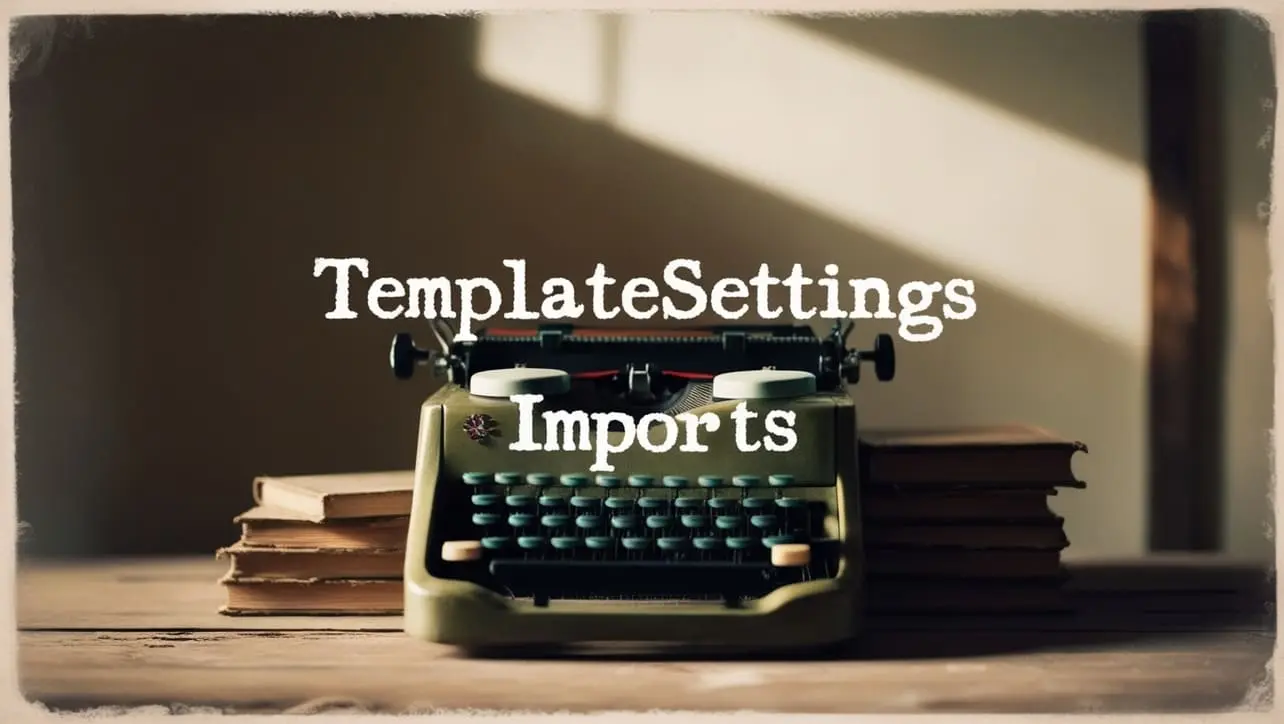
Lodash _() Seq Method
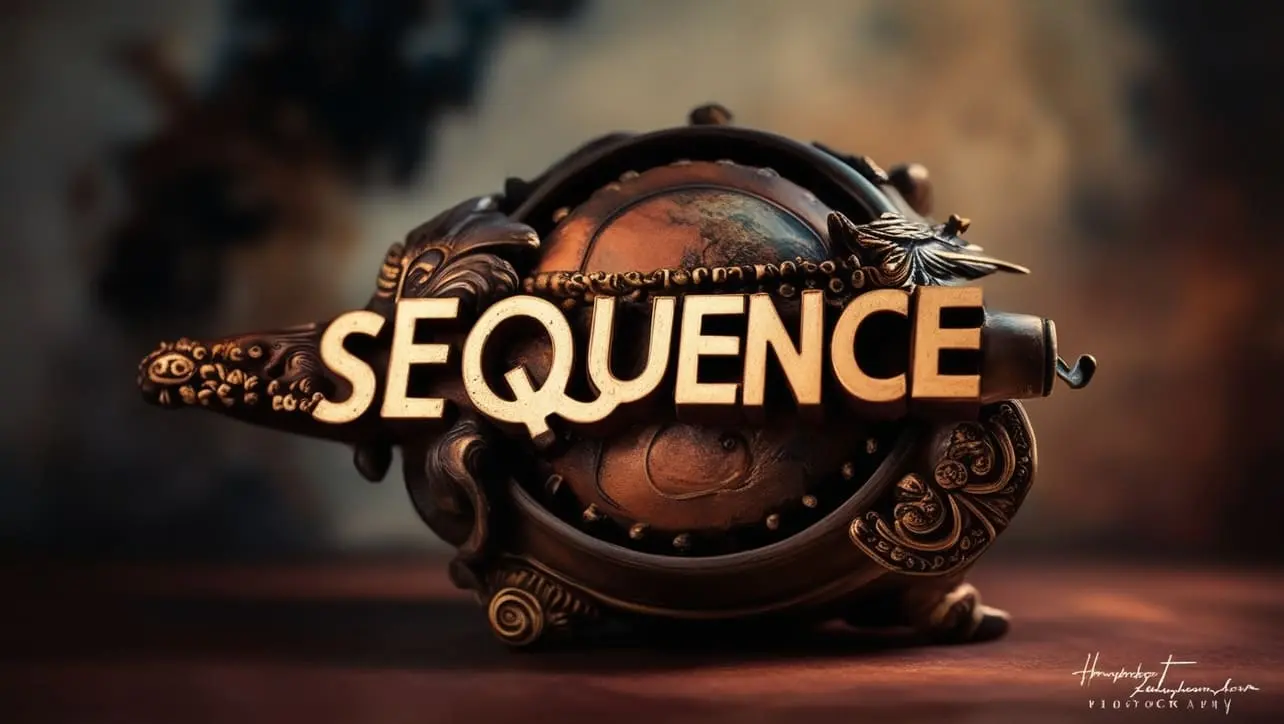
Photo Credit to CodeToFun
🙋 Introduction
The _.()
method in Lodash is the entry point for chaining and sequencing operations on arrays, objects, and other collections. By wrapping a value with _.()
, developers gain access to a wide range of powerful functions and methods provided by the Lodash library.
This method serves as the foundation for creating chained sequences of operations, enabling streamlined and expressive code for data manipulation tasks.
🧠 Understanding _.() Method
The _.()
method, often referred to as the "Lodash wrapper" or "chainable wrapper," transforms the given value into a Lodash wrapper object. This wrapper object provides access to numerous Lodash methods that can be chained together to perform a sequence of operations on the wrapped value.
💡 Syntax
The syntax for the _.()
method is straightforward:
_(value)
- value: The value to wrap.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.()
method:
const _ = require('lodash');
const wrappedArray = _([1, 2, 3]);
console.log(wrappedArray.map(x => x * 2).value());
// Output: [2, 4, 6]
In this example, the array [1, 2, 3] is wrapped with _.()
, allowing us to chain the map() method to double each element in the array.
🏆 Best Practices
When working with the _.()
method, consider the following best practices:
Chainable Operations:
Utilize the chaining capability of
_.()
to perform multiple operations in a single sequence. This enables concise and readable code for complex data manipulation tasks.example.jsCopiedconst wrappedArray = _([1, 2, 3]); const result = wrappedArray .map(x => x * 2) .filter(x => x > 3) .value(); console.log(result); // Output: [4, 6]
Reusability:
Reuse the Lodash wrapper object to perform multiple sequences of operations on the same value. This reduces code duplication and promotes modular design.
example.jsCopiedconst wrappedArray = _([1, 2, 3]); const doubleMap = wrappedArray.map(x => x * 2); const squaredMap = wrappedArray.map(x => x ** 2); console.log(doubleMap.value()); console.log(squaredMap.value());
Be Mindful of Performance:
While chaining operations with
_.()
can lead to elegant code, be mindful of performance implications, especially when dealing with large datasets. Consider the efficiency of each operation in the chain to optimize performance.example.jsCopiedconst largeArray = /* ...fetch large dataset... */ ; const wrappedArray = _(largeArray); console.time('chain'); const result = wrappedArray .map( /* ...operation 1... */ ) .filter( /* ...operation 2... */ ) .value(); console.timeEnd('chain'); console.log(result);
📚 Use Cases
Data Transformation:
Use
_.()
to create sequences of operations for transforming data structures, such as arrays or objects, into desired formats.example.jsCopiedconst data = /* ...fetch raw data... */ ; const wrappedData = _(data); const transformedData = wrappedData .map( /* ...transformations... */ ) .filter( /* ...filters... */ ) .value(); console.log(transformedData);
Fluent Interface:
Employ
_.()
to build fluent interfaces for APIs or libraries, allowing users to chain method calls in a natural and expressive manner.example.jsCopiedconst api = /* ...initialize API... */ ; const wrappedResponse = _(api.getData()); const formattedData = wrappedResponse .map( /* ...formatting... */ ) .sortBy( /* ...sorting... */ ) .value(); console.log(formattedData);
Composable Functions:
Leverage
_.()
to compose functions dynamically, combining reusable building blocks to create custom operations tailored to specific requirements.example.jsCopiedconst wrappedFunction = _( /* ...compose functions... */ ); const result = wrappedFunction .map( /* ...map function... */ ) .filter( /* ...filter function... */ ) .value(); console.log(result);
🎉 Conclusion
The _.()
method in Lodash serves as a powerful tool for chaining and sequencing operations on collections in JavaScript. By wrapping values with _.()
, developers gain access to a rich set of methods for transforming, filtering, and manipulating data with ease. Whether you're building complex data pipelines or crafting fluent interfaces, _.()
provides a flexible and expressive solution for enhancing your JavaScript code.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.()
method in your Lodash projects.
👨💻 Join our Community:
Author
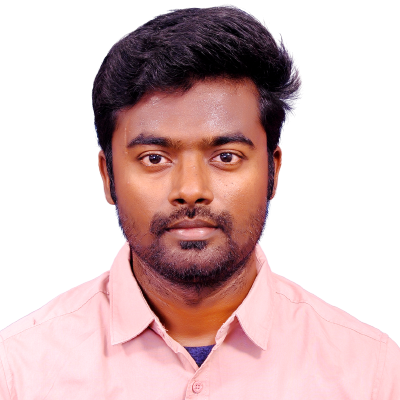
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
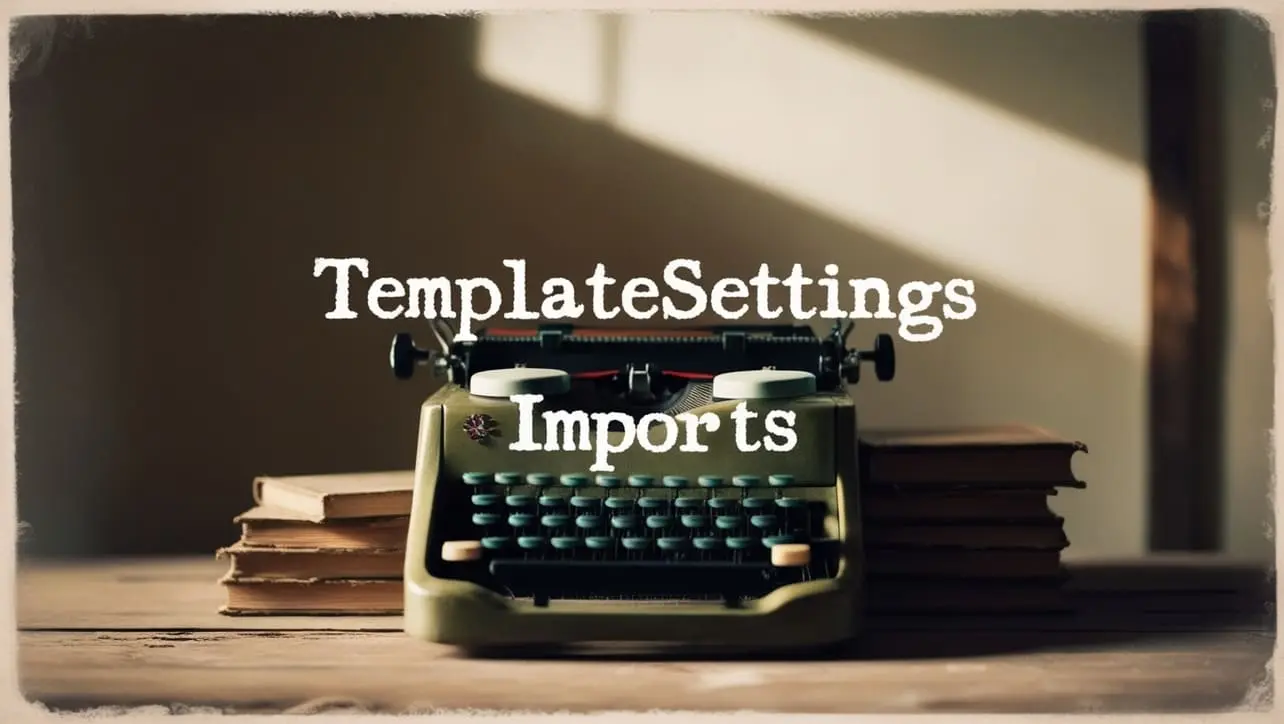
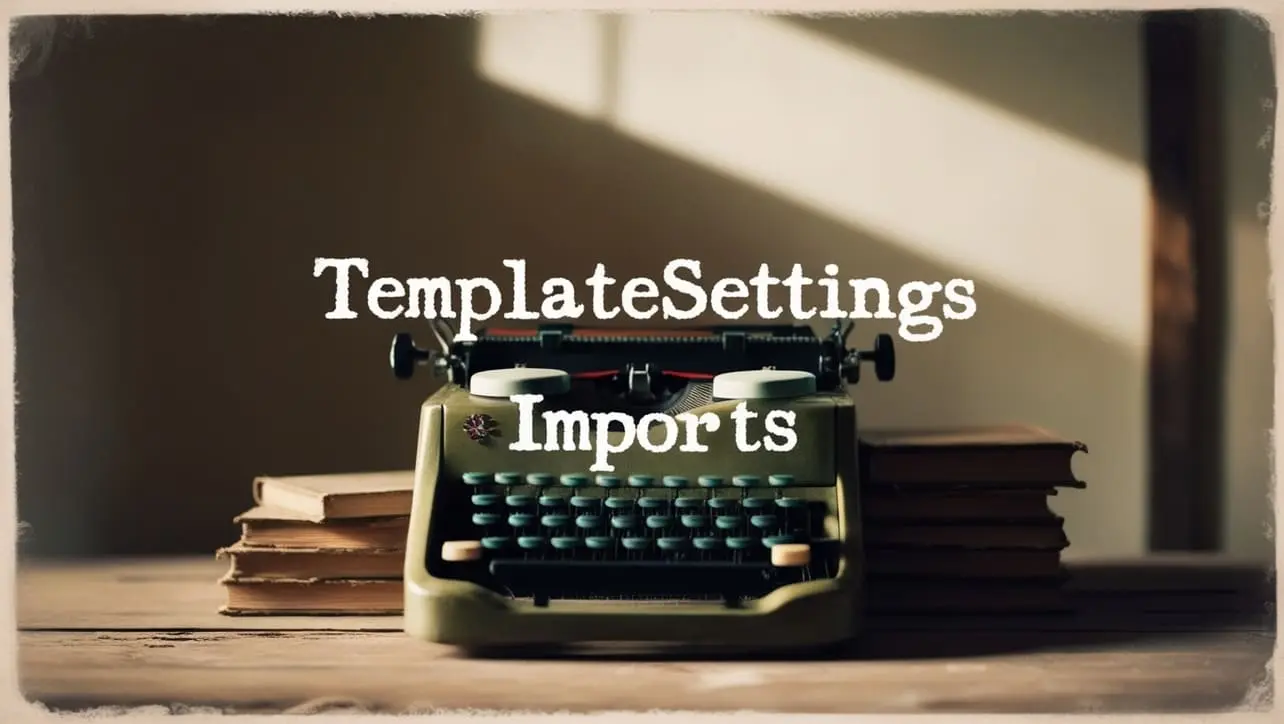
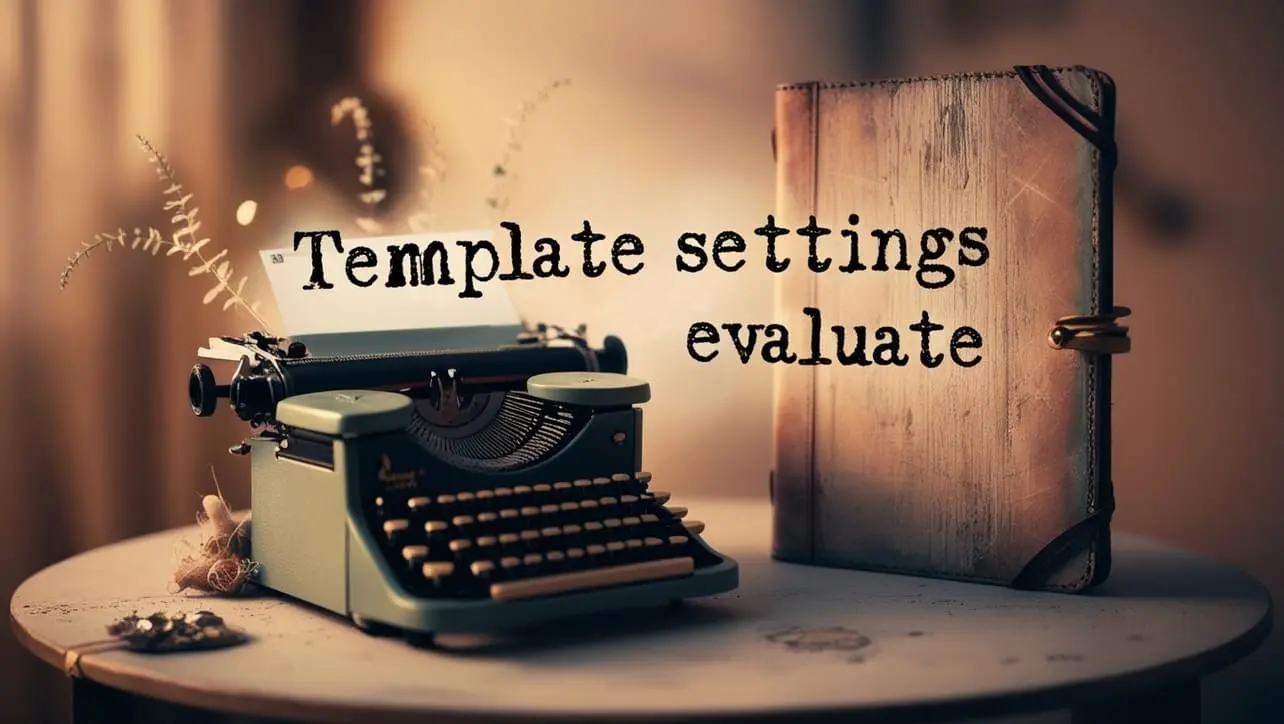
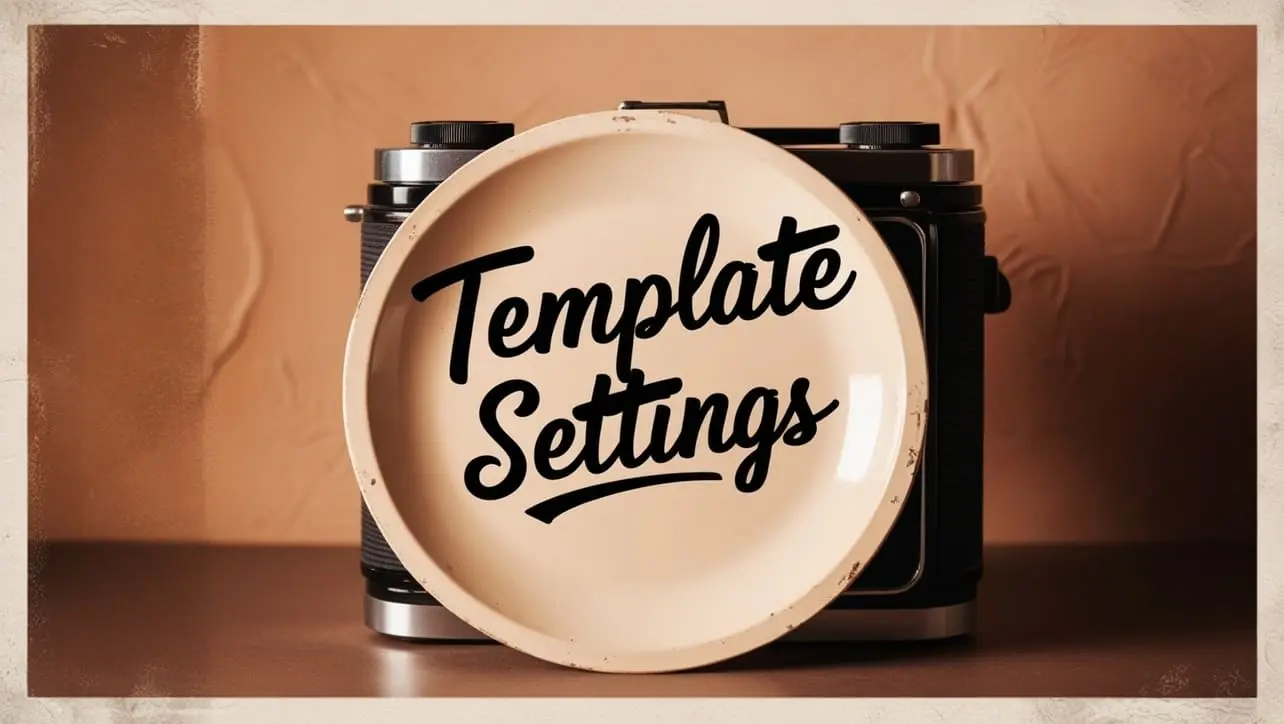
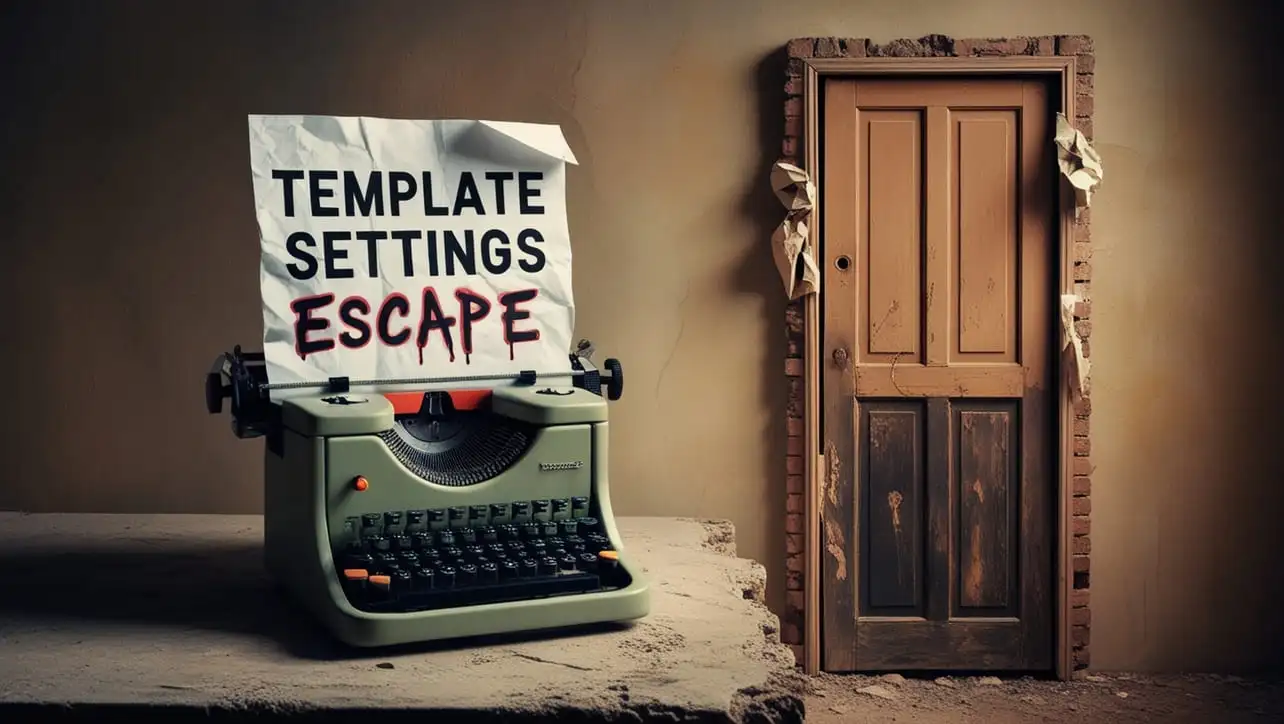
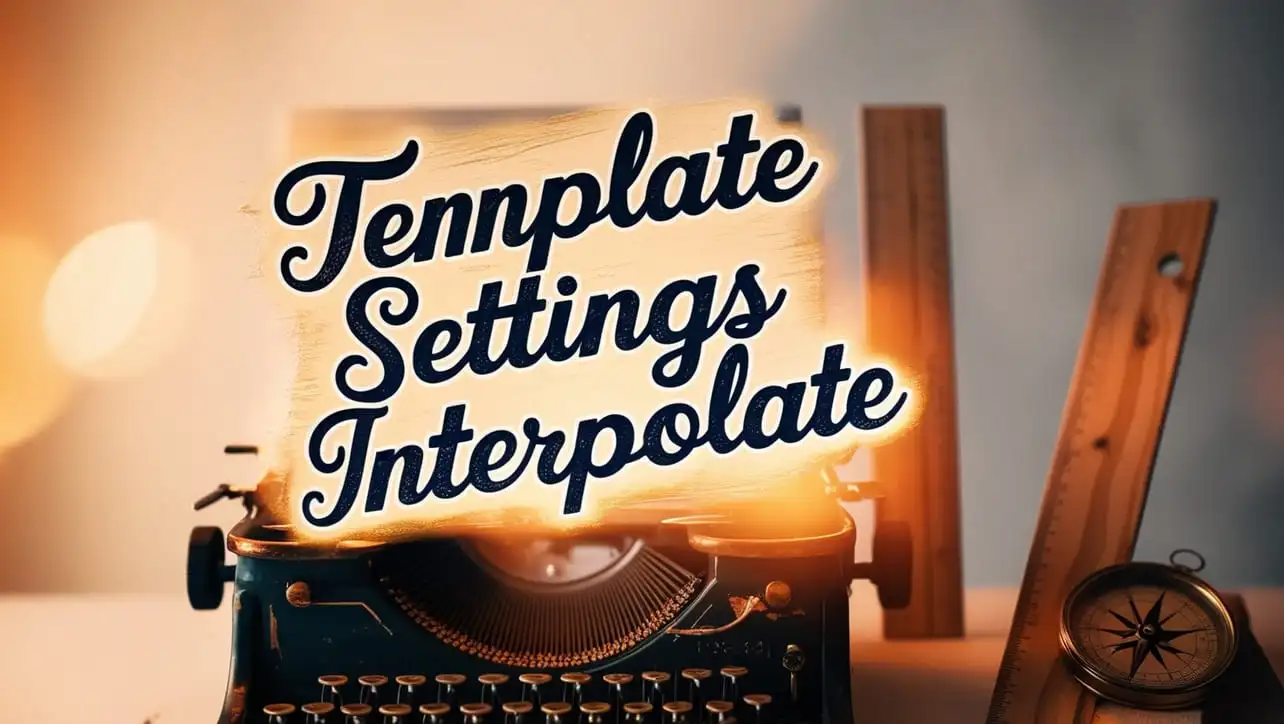
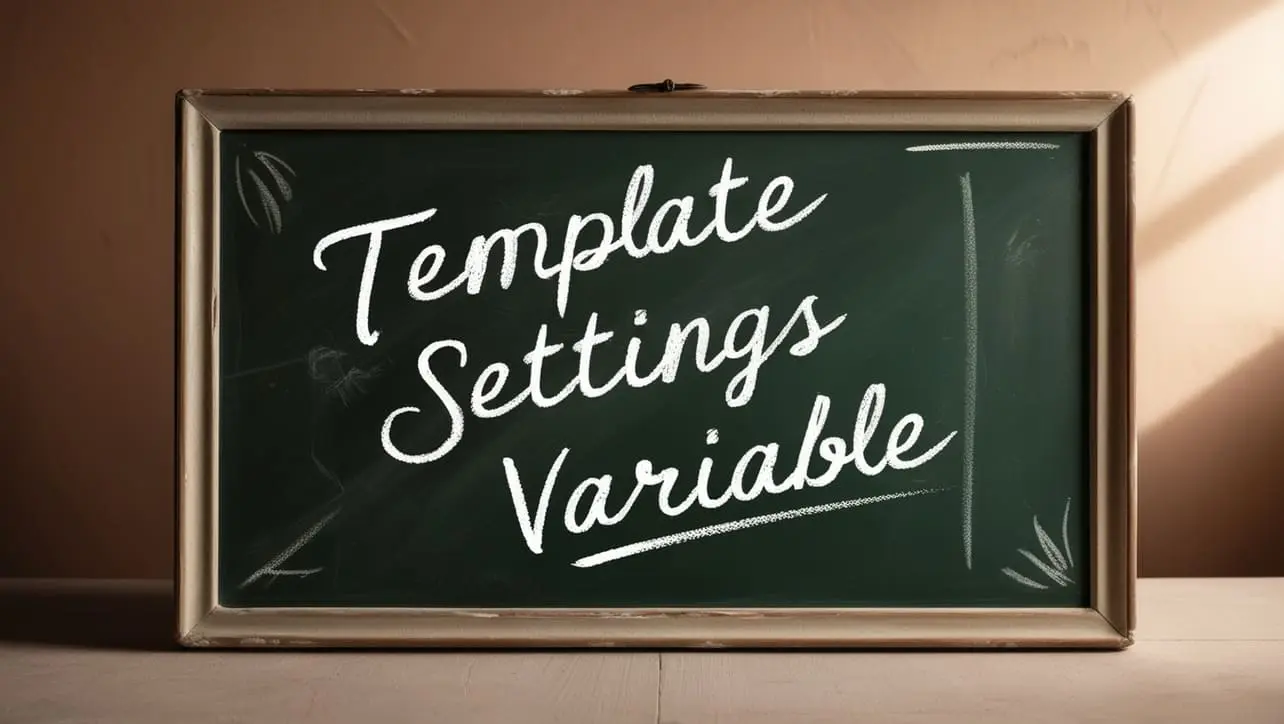
If you have any doubts regarding this article (Lodash _() Seq Method), please comment here. I will help you immediately.