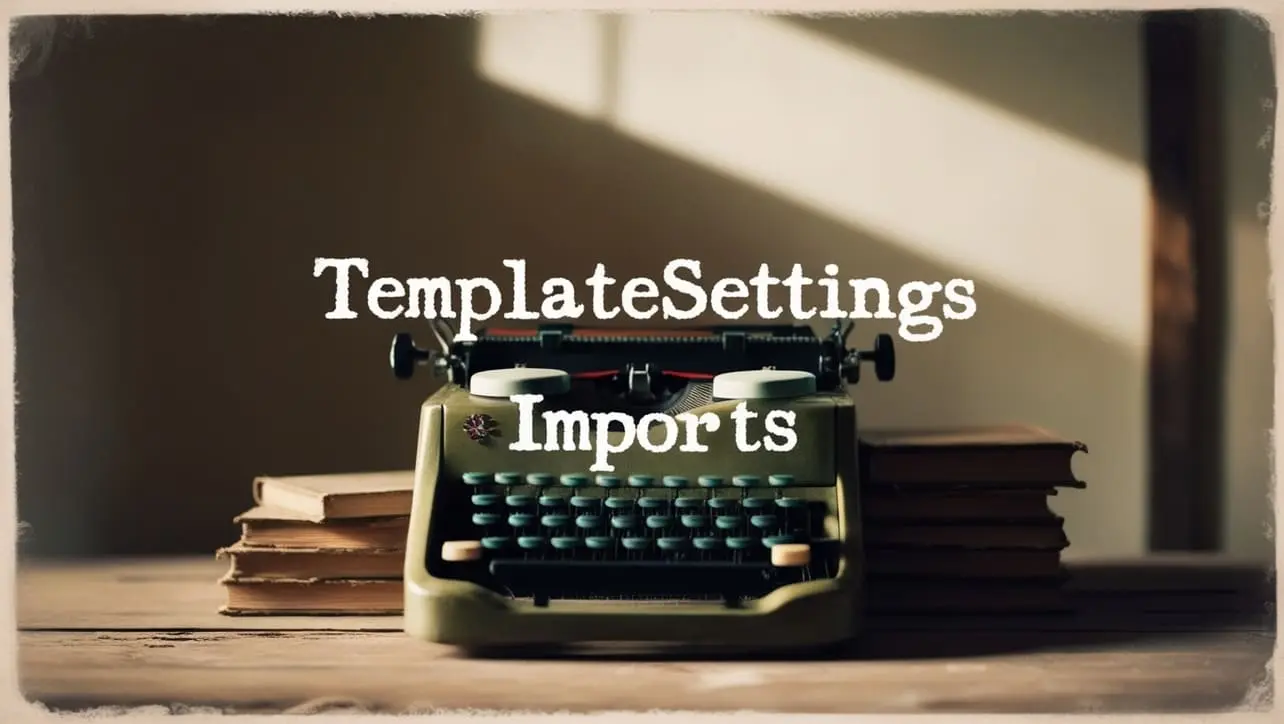
Lodash _.prototype.value() Seq Method
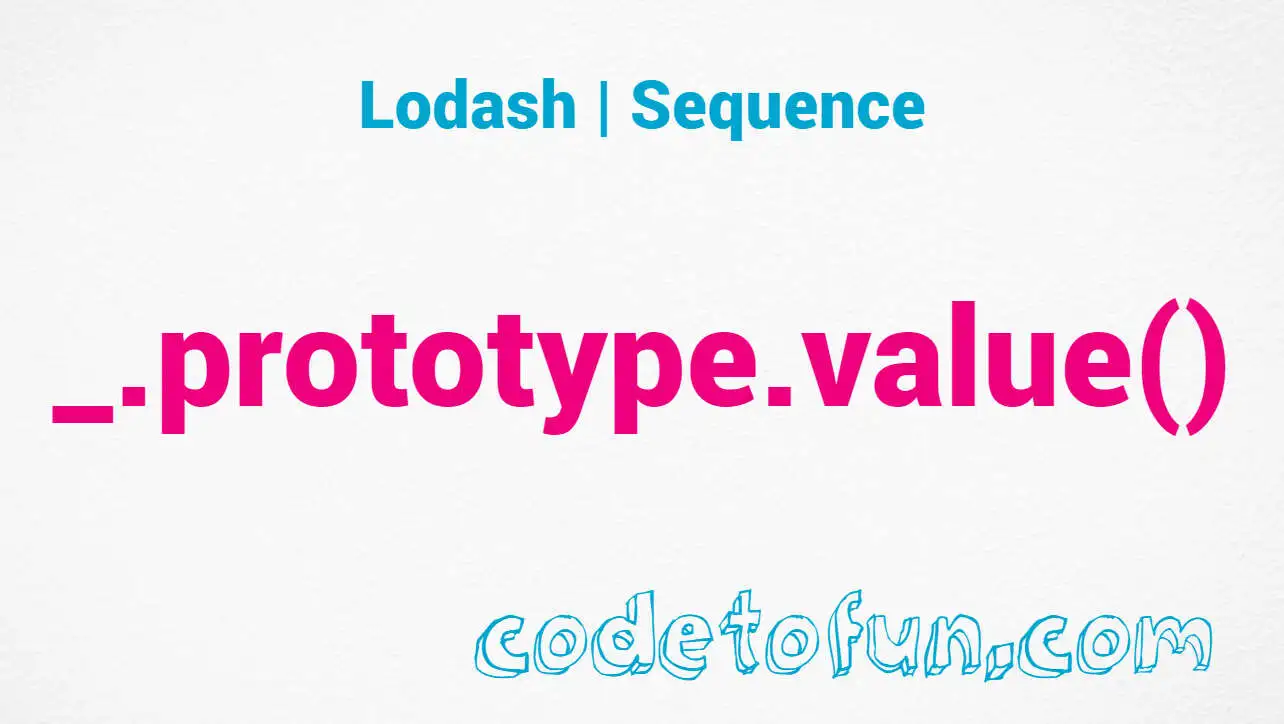
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript development, efficient data manipulation is essential. Lodash, a widely-used utility library, provides a plethora of methods to streamline array and object operations. Among these methods lies the _.prototype.value()
method, specifically designed for sequences.
This method extracts the wrapped value from a sequence object, offering convenience and flexibility in data processing.
🧠 Understanding _.prototype.value() Method
The _.prototype.value()
method in Lodash is utilized to retrieve the wrapped value from a sequence object. It serves as the final step in chaining sequence methods, allowing developers to obtain the resulting value for further processing or consumption.
💡 Syntax
The syntax for the _.prototype.value()
method is straightforward:
_([value]).value()
- value: The value to wrap.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.prototype.value()
method:
const _ = require('lodash');
const wrappedArray = _([1, 2, 3]);
const unwrappedArray = wrappedArray.value();
console.log(unwrappedArray);
// Output: [1, 2, 3]
In this example, the _.prototype.value()
method extracts the wrapped array value, returning [1, 2, 3].
🏆 Best Practices
When working with the _.prototype.value()
method, consider the following best practices:
Chaining Sequences:
Utilize chaining with sequence methods to perform a series of operations on a wrapped value before extracting it with
_.prototype.value()
. This enables concise and expressive data processing workflows.example.jsCopiedconst result = _([1, 2, 3]) .map(num => num * 2) .filter(num => num > 3) .value(); console.log(result); // Output: [4, 6]
Error Handling:
Ensure error handling for cases where the wrapped value might be undefined or null. Implement defensive programming techniques to handle such scenarios gracefully.
example.jsCopiedconst wrappedValue = _(/* ...generate wrapped value dynamically... */); const unwrappedValue = wrappedValue ? wrappedValue.value() : []; console.log(unwrappedValue);
Performance Considerations:
Be mindful of performance implications when working with large datasets. While chaining sequence methods offers convenience, excessive chaining can impact performance. Optimize sequences for efficiency, considering factors such as algorithm complexity and data volume.
example.jsCopiedconst largeDataset = /* ...fetch data from API or elsewhere... */ ; const result = _(largeDataset) .filter( /* ...filter condition... */ ) .map( /* ...mapping function... */ ) .value(); console.log(result);
📚 Use Cases
Data Transformation:
The
_.prototype.value()
method is commonly used to finalize data transformations applied through sequence chaining. It allows developers to obtain the processed data in its final form for consumption or further manipulation.example.jsCopiedconst transformedData = _([ /* ...data to transform... */ ]) .map( /* ...mapping function... */ ) .filter( /* ...filter condition... */ ) .value(); console.log(transformedData);
Fluent API Design:
By leveraging sequence chaining with
_.prototype.value()
, developers can design fluent APIs that facilitate intuitive and readable code. This promotes code maintainability and enhances developer productivity.example.jsCopiedconst result = _( /* ...data source... */ ) .doSomething() .doAnotherThing() .value(); console.log(result);
Asynchronous Processing:
The
_.prototype.value()
method can be employed in asynchronous workflows to obtain the final result after a series of asynchronous operations. This simplifies asynchronous code structure and improves readability.example.jsCopiedasync function processDataAsync() { const result = await _( /* ...async data source... */ ) .asyncOperation1() .asyncOperation2() .value(); console.log(result); } processDataAsync();
🎉 Conclusion
The _.prototype.value()
method in Lodash is a versatile tool for extracting the wrapped value from a sequence object. Whether you're performing data transformations, designing fluent APIs, or handling asynchronous workflows, this method offers convenience and flexibility in JavaScript development.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.prototype.value()
method in your Lodash projects.
👨💻 Join our Community:
Author
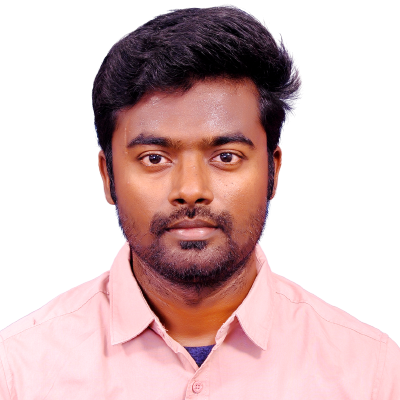
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
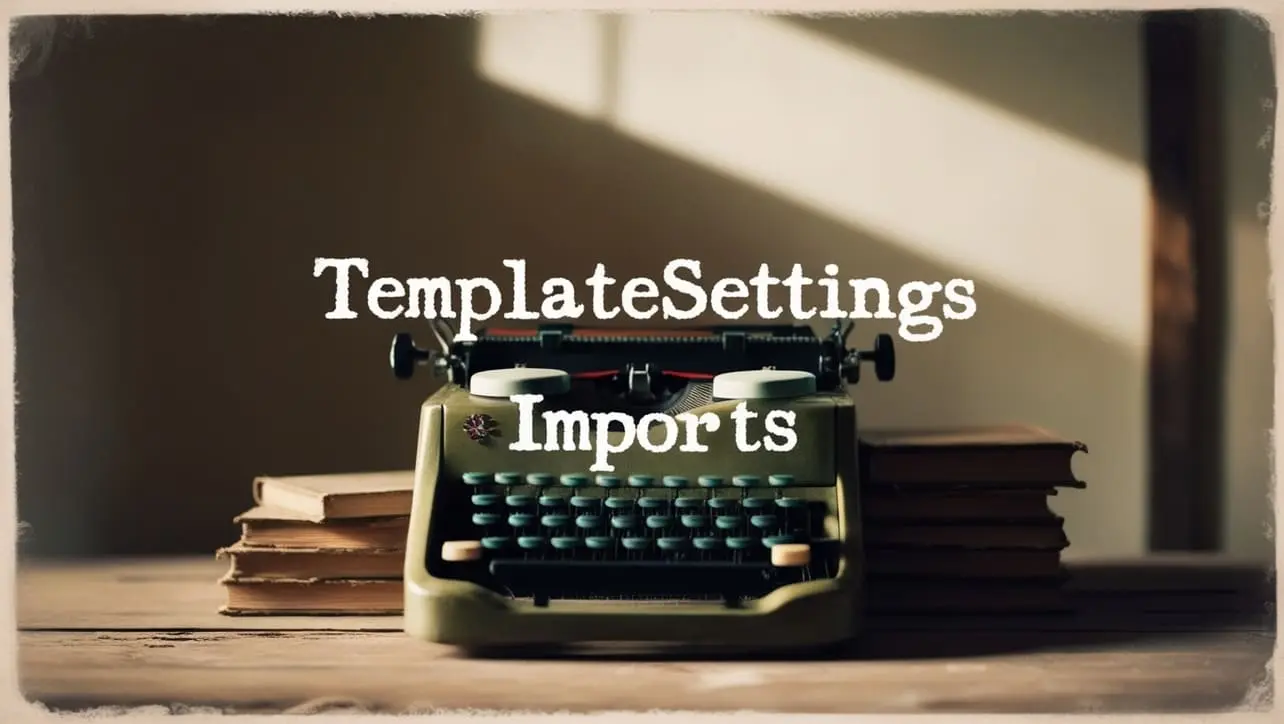
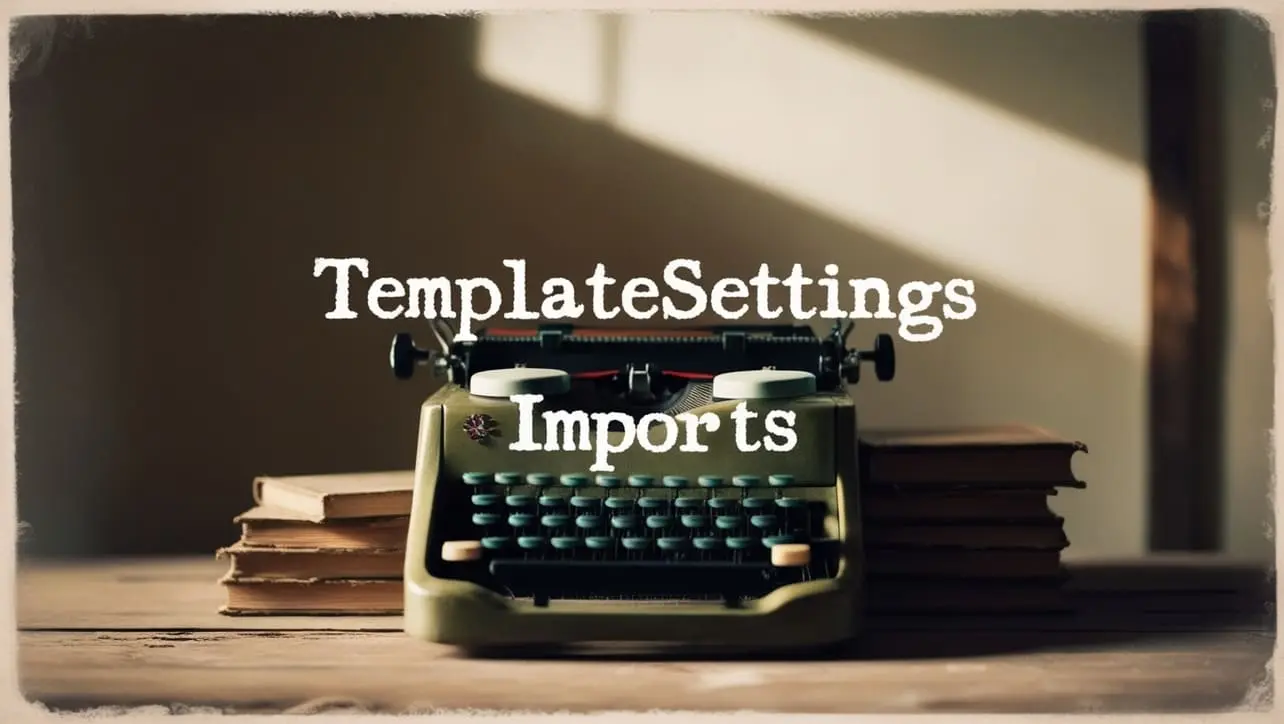
Lodash _.templateSettings.imports Property
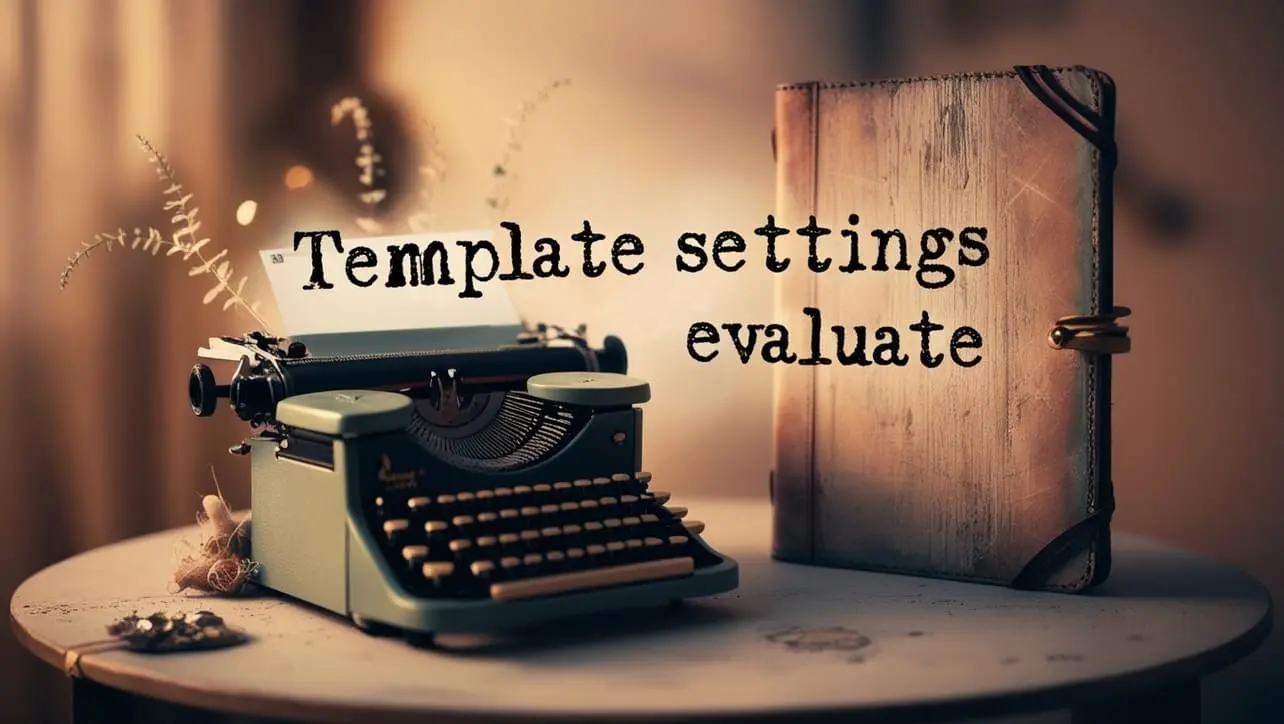
Lodash _.templateSettings.evaluate Property
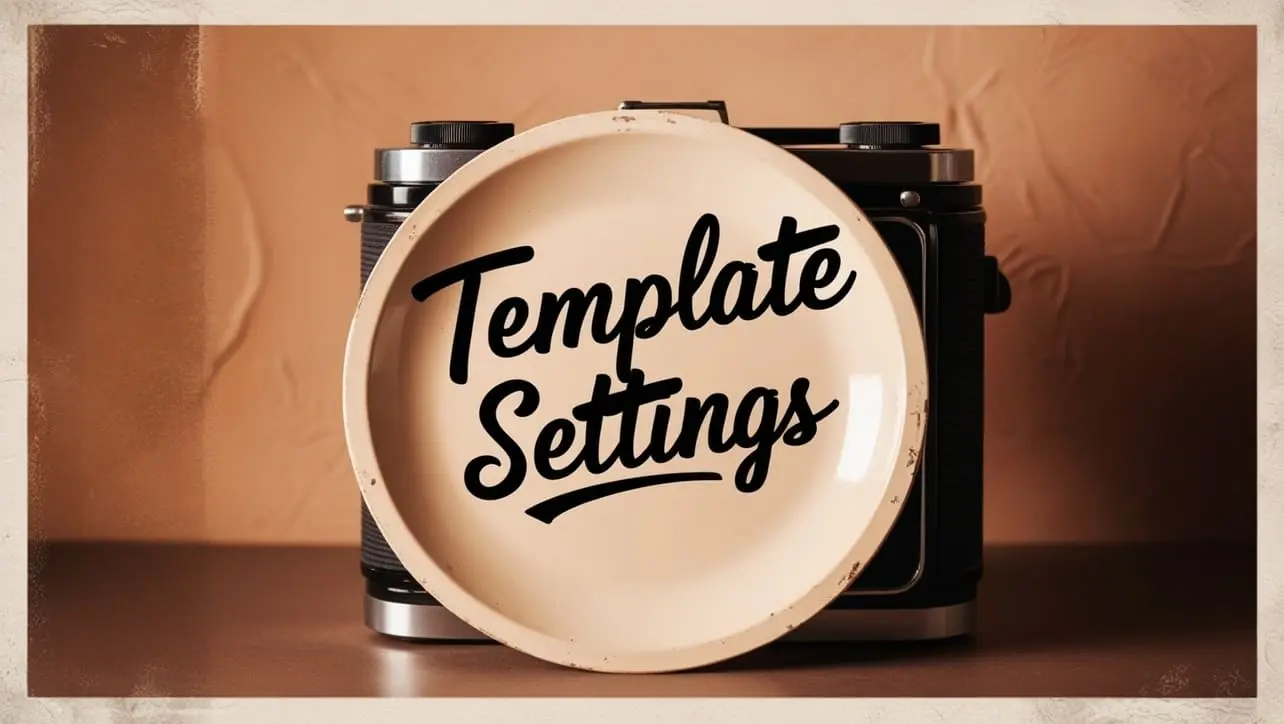
Lodash _.templateSettings Property
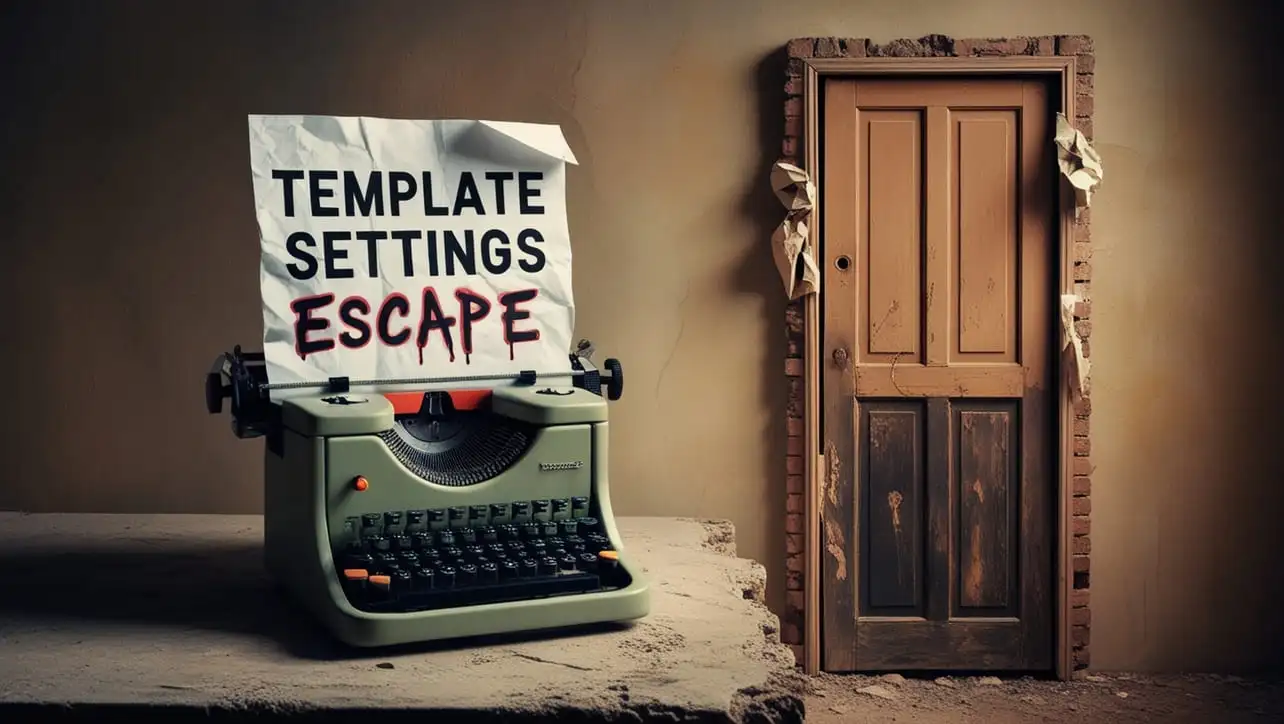
Lodash _.templateSettings.escape Property
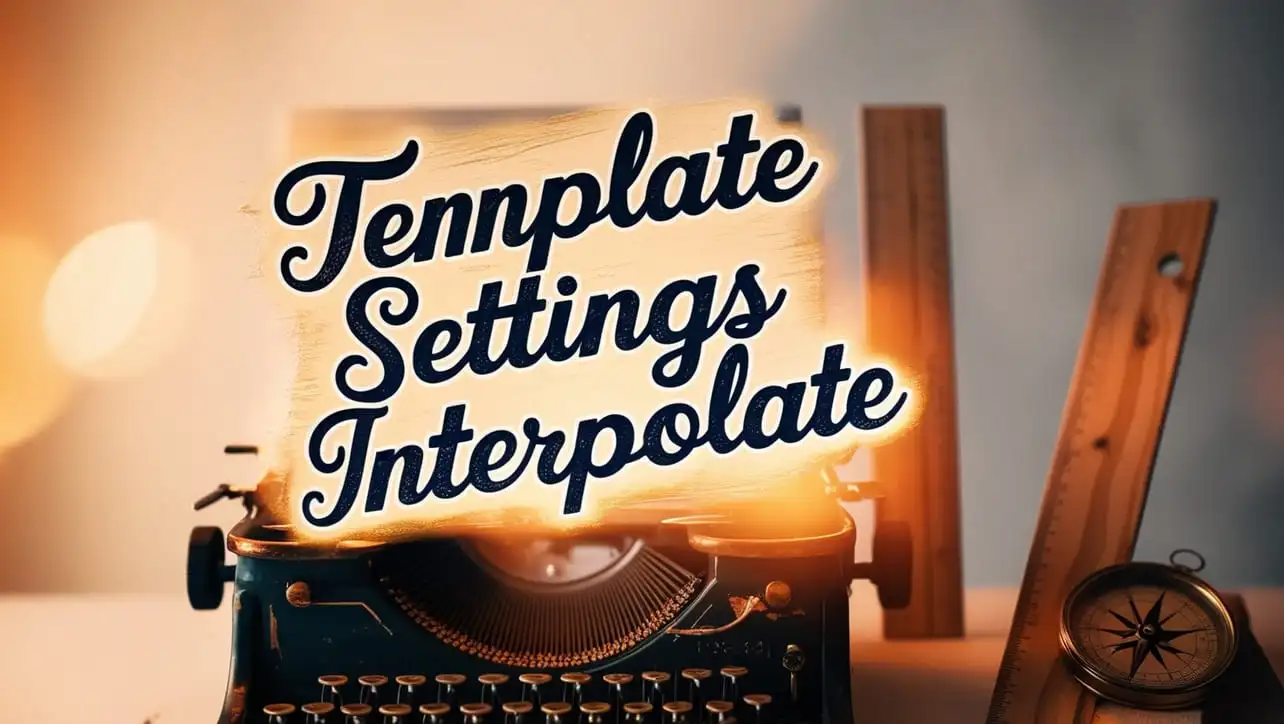
Lodash _.templateSettings.interpolate Property
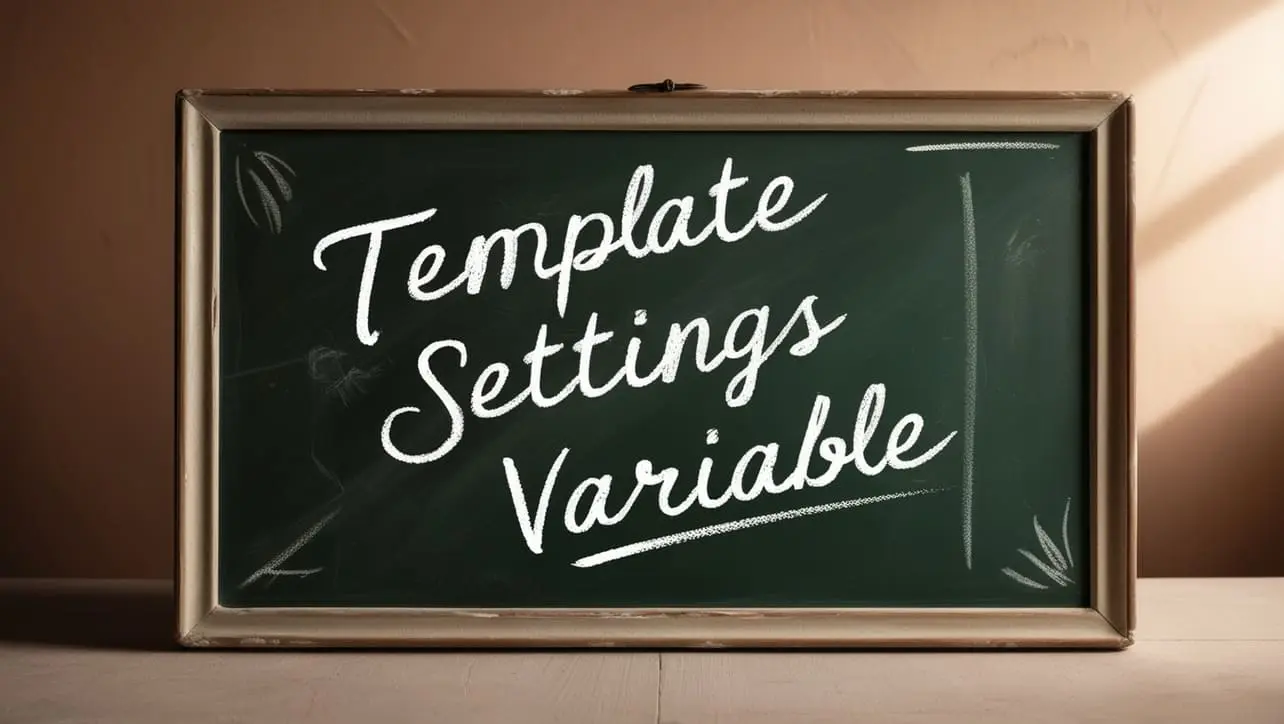
If you have any doubts regarding this article (Lodash _.prototype.value() Seq Method), please comment here. I will help you immediately.