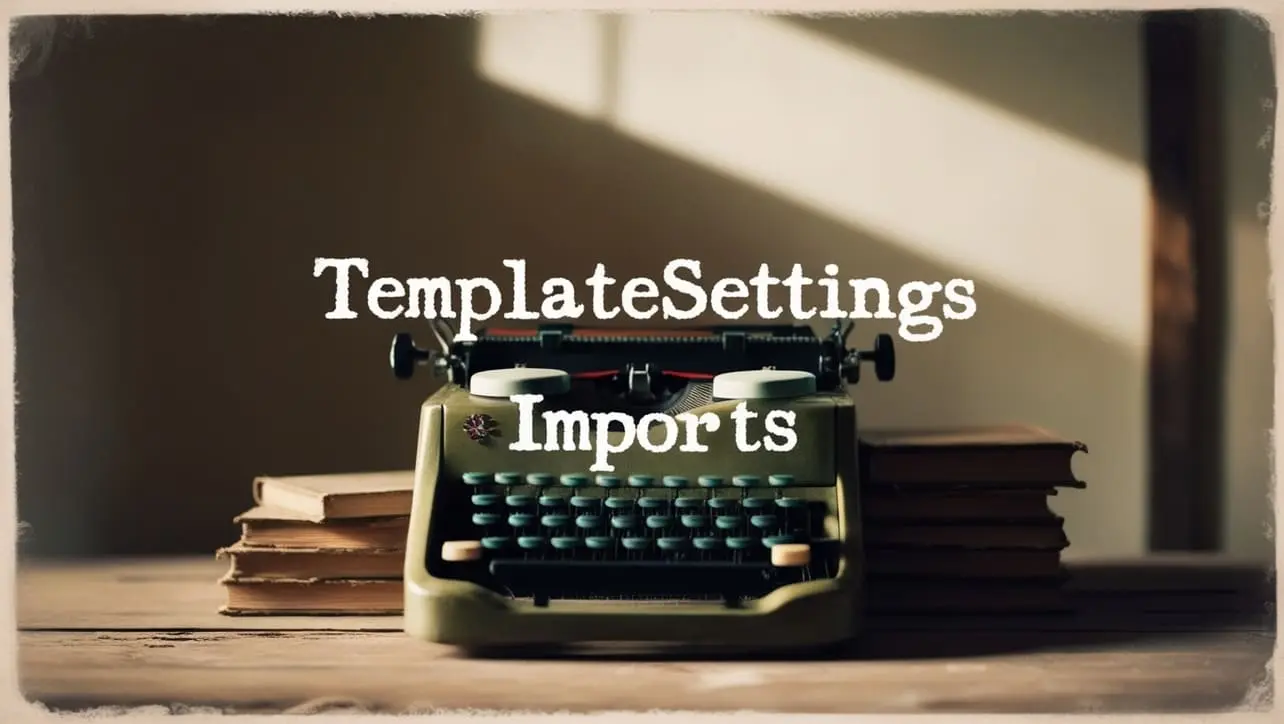
Lodash _.prototype.chain() Seq Method
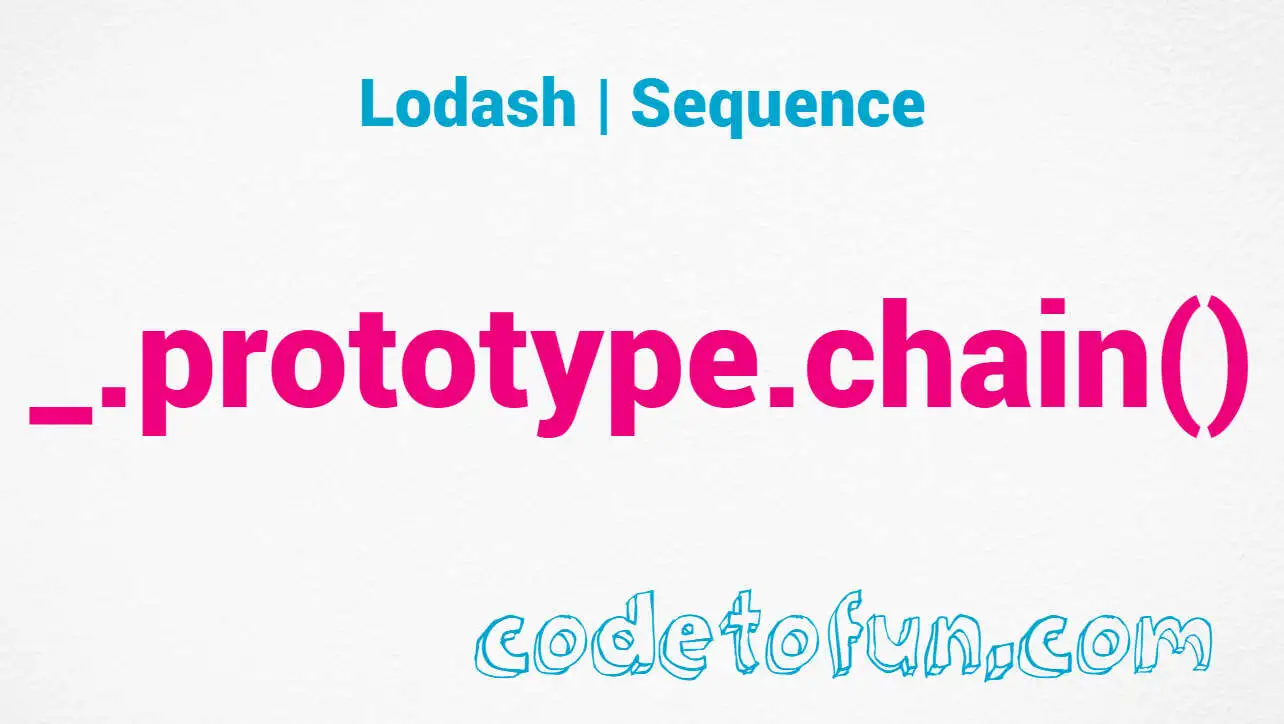
Photo Credit to CodeToFun
🙋 Introduction
In the world of JavaScript development, streamlining data transformations is a common challenge. The Lodash library addresses this challenge with its powerful sequence methods, including _.prototype.chain()
. Unlike the regular _.chain() method, _.prototype.chain()
allows for explicit chaining, providing a more flexible and intuitive approach to data manipulation.
By leveraging this method, developers can create elegant and readable code for complex transformation pipelines.
🧠 Understanding _.prototype.chain() Method
The _.prototype.chain()
method in Lodash enables explicit chaining of sequence operations. It allows developers to create a sequence of operations on a wrapped object, providing a fluent interface for data transformation. This method is particularly useful when dealing with multiple transformations or when clarity and readability are paramount.
💡 Syntax
The syntax for the _.prototype.chain()
method is straightforward:
_(value).chain()
- value: The value to wrap and chain sequence operations.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.prototype.chain()
method:
const _ = require('lodash');
const users = [
{
'user': 'barney',
'age': 36
},
{
'user': 'fred',
'age': 40
}];
// A sequence without explicit chaining.
const result1 = _(users).head();
console.log(result1);
// Output: { 'user': 'barney', 'age': 36 }
// A sequence with explicit chaining.
const result2 = _(users)
.chain()
.head()
.pick('user')
.value();
console.log(result2);
// Output: { 'user': 'barney' }
In this example, we demonstrate both implicit and explicit chaining using _.prototype.chain()
. The explicit chaining approach provides a more structured and readable way to express data transformations.
🏆 Best Practices
When working with the _.prototype.chain()
method, consider the following best practices:
Clarity Over Conciseness:
Prioritize clarity when using explicit chaining with
_.prototype.chain()
. While concise code is desirable, it should not come at the expense of readability.example.jsCopiedconst result = _(users) .chain() .filter({ 'active': true }) .map('name') .take(5) .value();
Avoid Excessive Chaining:
Avoid excessive chaining to maintain code readability and ease of debugging. Break down complex transformations into smaller, more manageable steps when necessary.
example.jsCopiedconst result = _(users) .chain() .map(user => user.age * 2) .filter(age => age > 30) .take(3) .value();
Utilize Helper Functions:
Leverage helper functions within chained sequences to encapsulate reusable logic and improve code maintainability.
example.jsCopiedconst isAdult = user => user.age >= 18; const result = _(users) .chain() .filter(isAdult) .map('name') .value();
📚 Use Cases
Data Transformation Pipelines:
_.prototype.chain()
is ideal for constructing data transformation pipelines, where multiple operations are applied sequentially to transform input data into desired output.example.jsCopiedconst transformedData = _(data) .chain() .filter(...) .map(...) .sortBy(...) .value();
Fluent Interface for Data Manipulation:
Use explicit chaining with
_.prototype.chain()
to create fluent interfaces for data manipulation, enhancing code readability and maintainability.example.jsCopiedconst userNames = _(users) .chain() .map('name') .sortBy() .value();
Complex Filtering and Mapping:
When dealing with complex filtering and mapping requirements, explicit chaining offers a structured and intuitive approach to expressing data transformations.
example.jsCopiedconst processedData = _(data) .chain() .filter(...) .map(...) .groupBy(...) .value();
🎉 Conclusion
The _.prototype.chain()
method in Lodash empowers developers to create explicit chaining sequences for data transformation tasks. By following best practices and leveraging this method effectively, developers can write clean, readable code for complex data manipulation scenarios.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.prototype.chain()
method in your Lodash projects.
👨💻 Join our Community:
Author
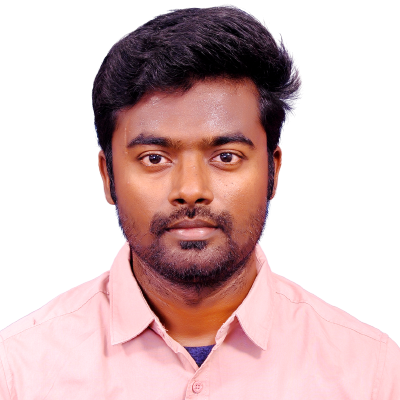
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
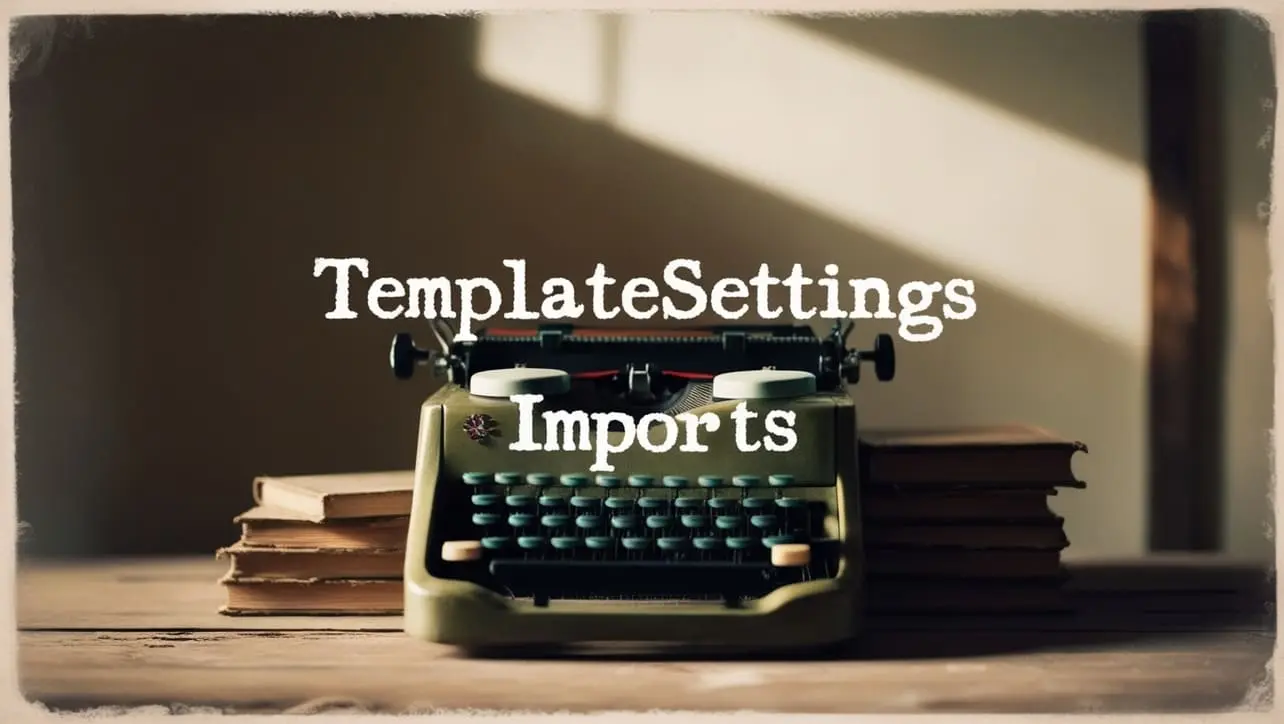
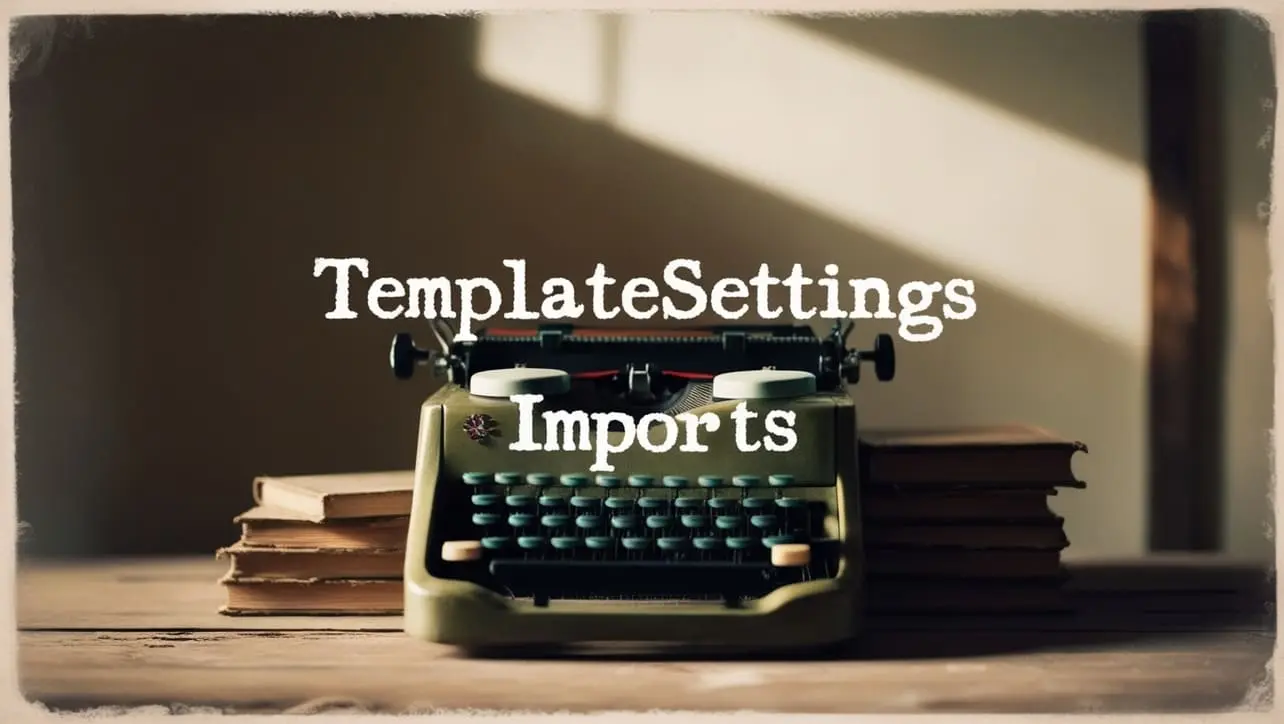
Lodash _.templateSettings.imports Property
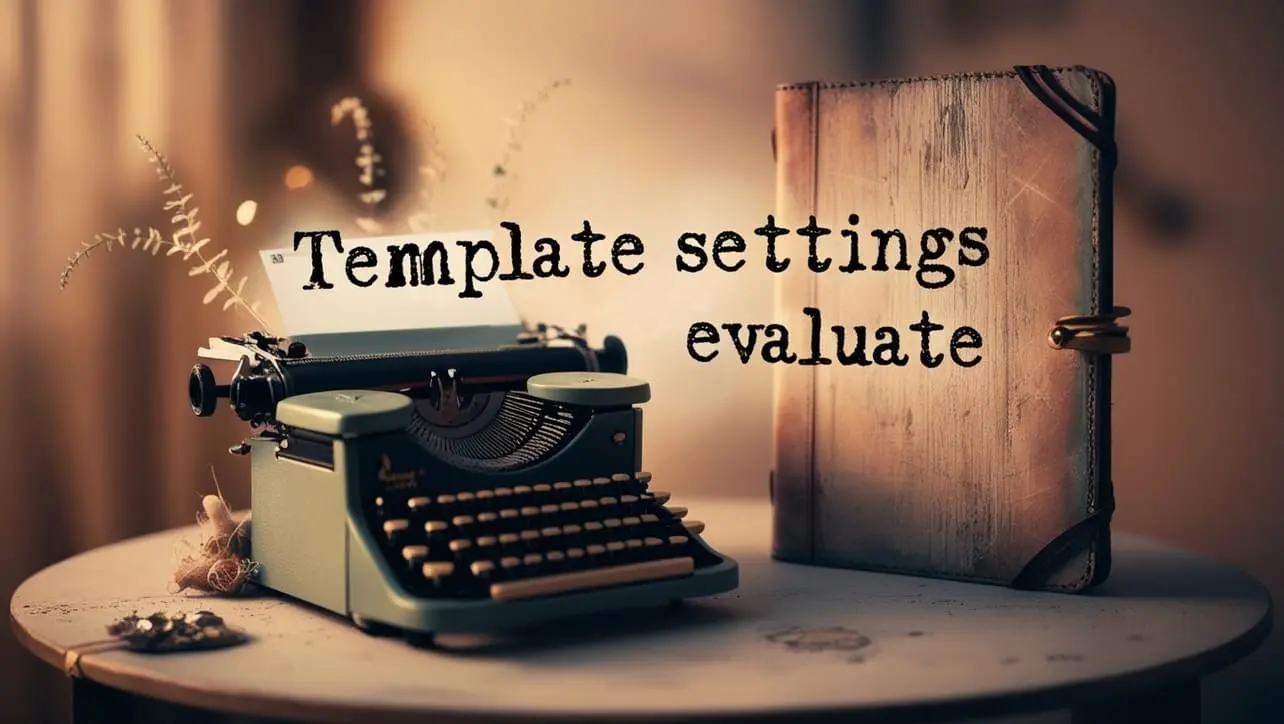
Lodash _.templateSettings.evaluate Property
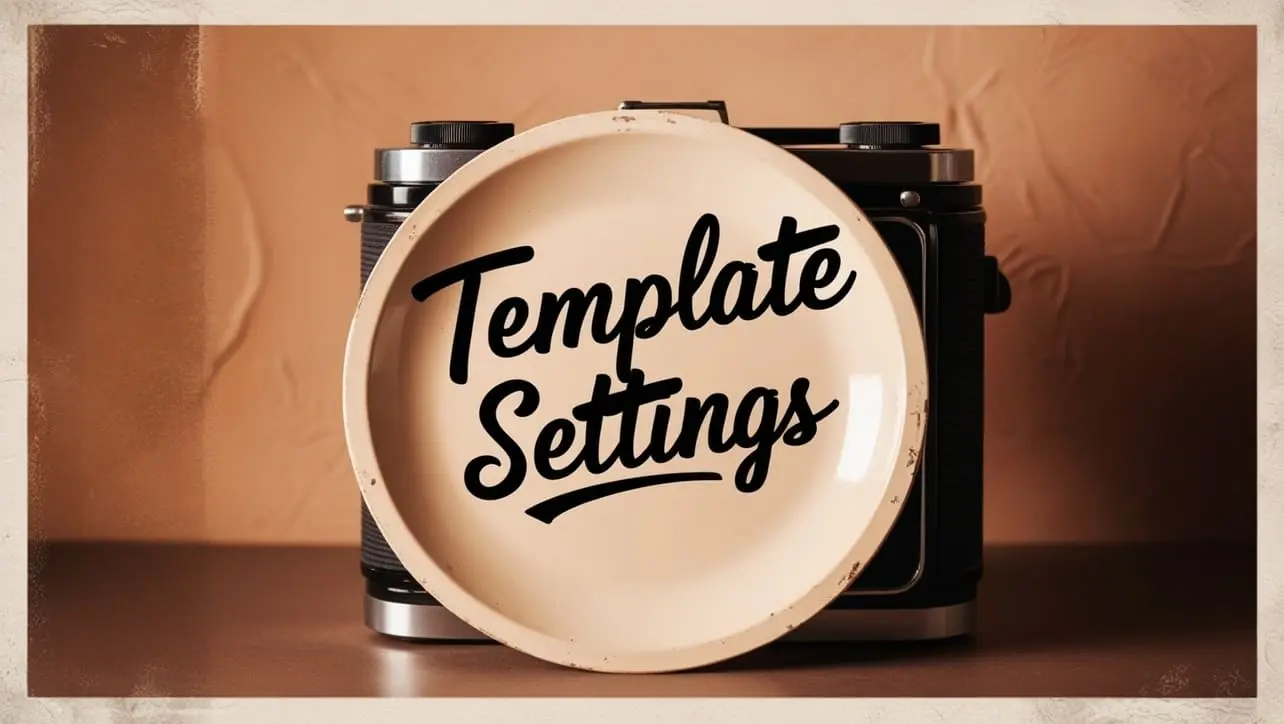
Lodash _.templateSettings Property
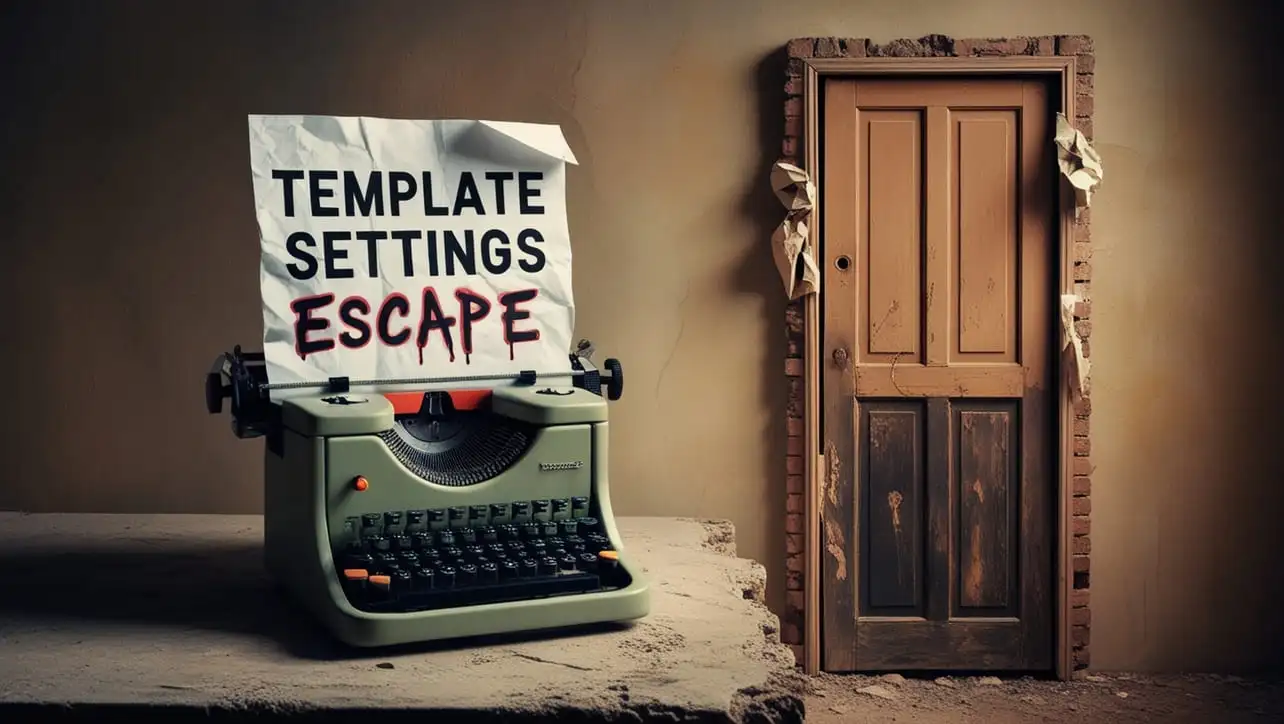
Lodash _.templateSettings.escape Property
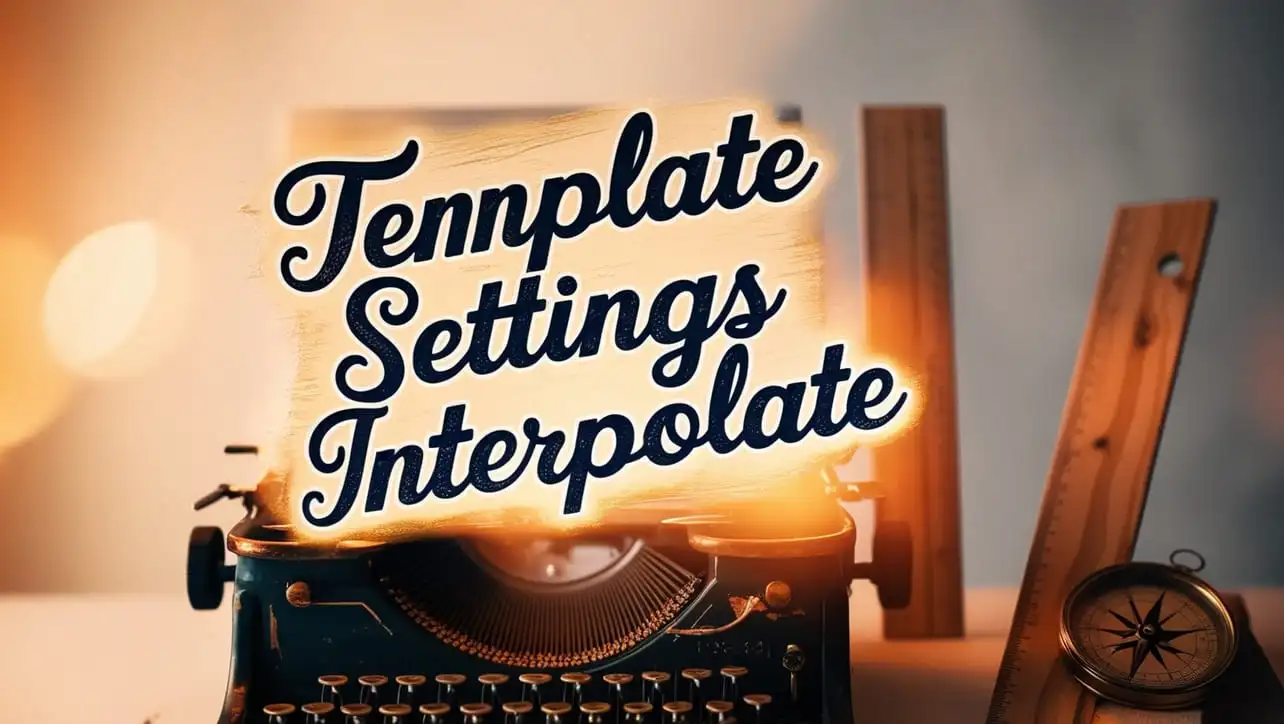
Lodash _.templateSettings.interpolate Property
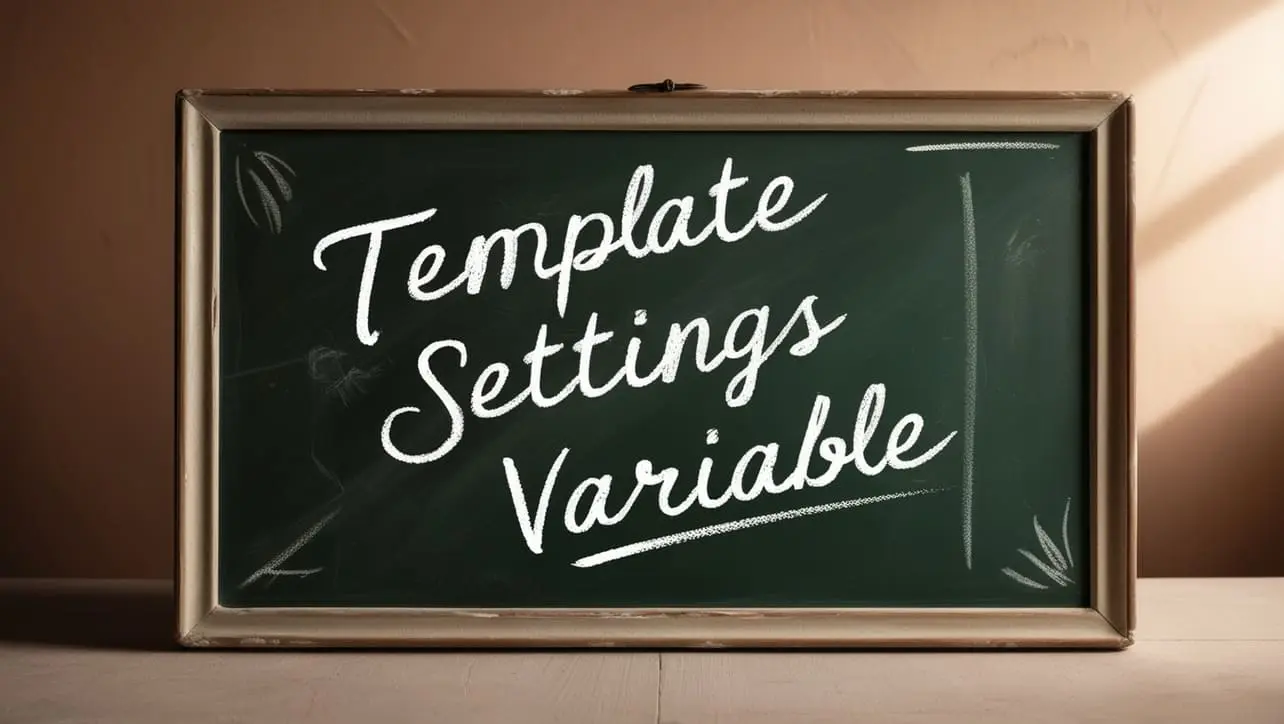
If you have any doubts regarding this article (Lodash _.prototype.chain() Seq Method), please comment here. I will help you immediately.