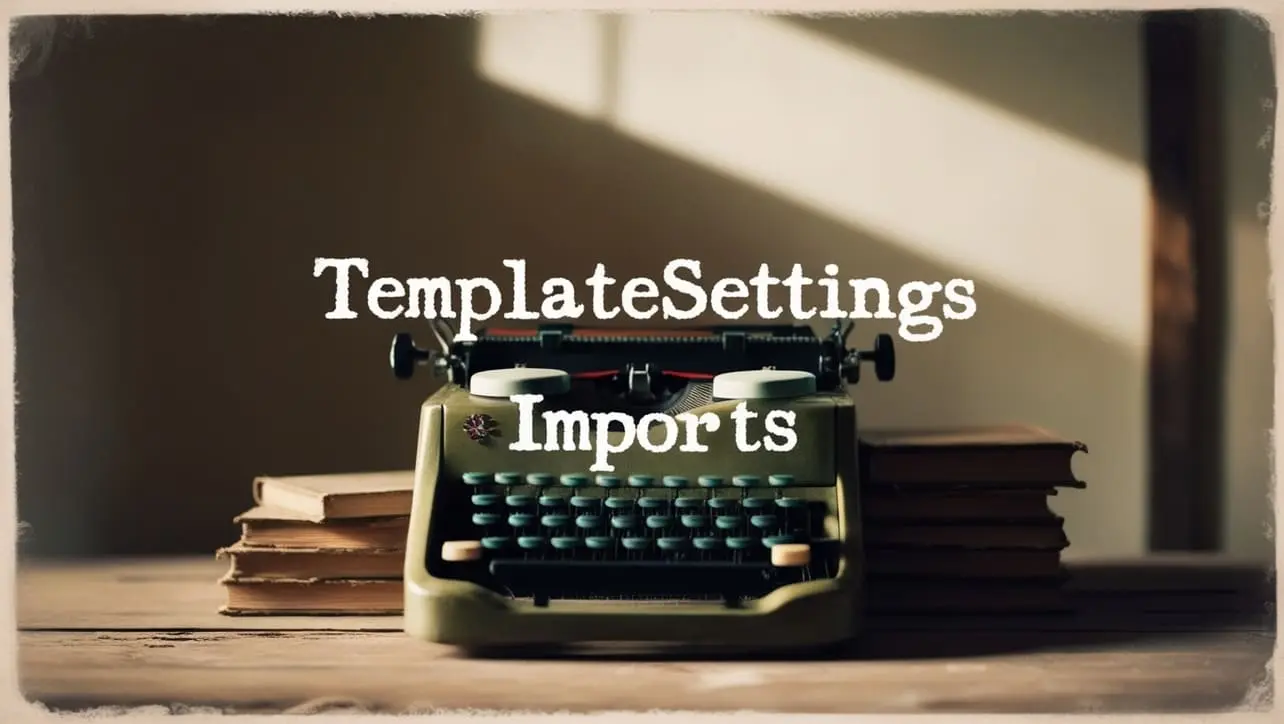
Lodash _.thru() Seq Method
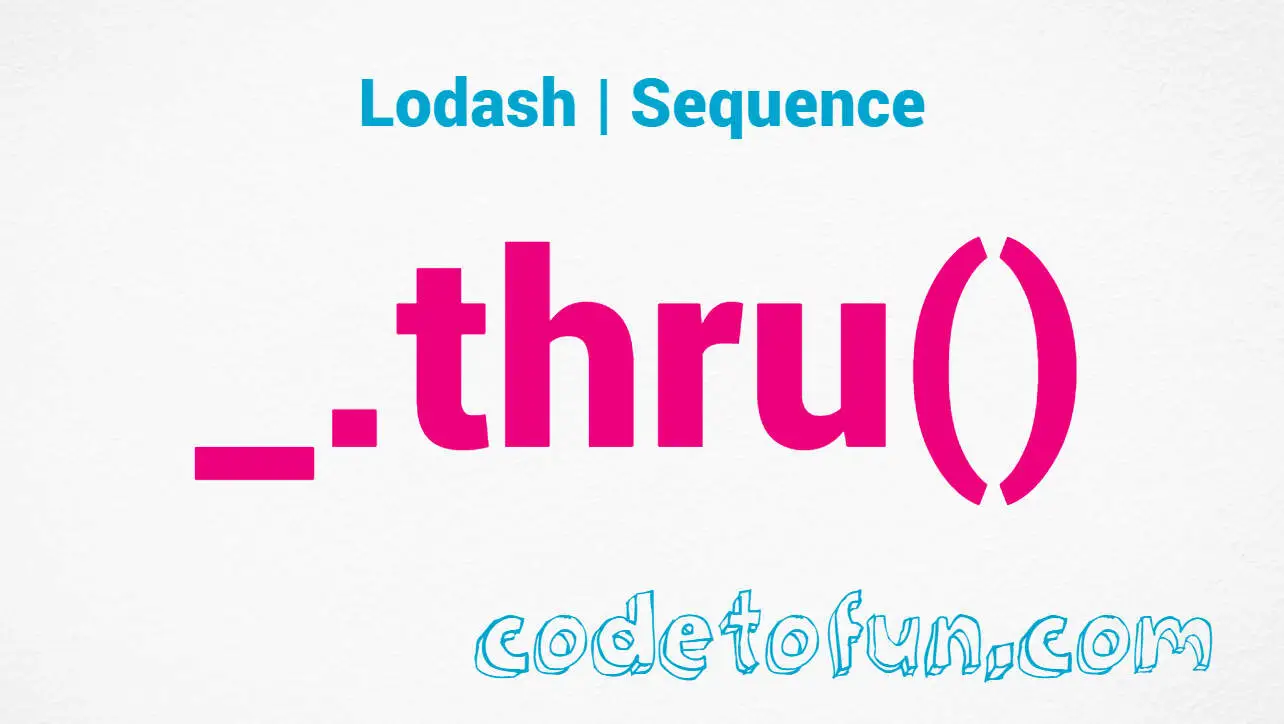
Photo Credit to CodeToFun
🙋 Introduction
In the landscape of JavaScript programming, working with sequences of operations on data collections is a common task. Lodash, a popular utility library, provides a variety of methods to streamline this process. Among these methods is _.thru()
, a Seq method that serves as a versatile tool for chaining and transforming data through a sequence of operations.
Understanding how to leverage _.thru()
can significantly enhance code readability and maintainability.
🧠 Understanding _.thru() Method
The _.thru()
method in Lodash is part of the Seq object, which represents a sequence of chained operations on a data collection. _.thru()
allows you to pass the current value in the sequence through a function, enabling intermediate transformations or inspections while preserving the chain.
💡 Syntax
The syntax for the _.thru()
method is straightforward:
_(value).thru(interceptor)
- value: The initial value or collection to process.
- interceptor: The function invoked per iteration.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.thru()
method:
const _ = require('lodash');
const result = _([1, 2, 3])
.map(x => x * 2)
.thru(collection => {
console.log('Intermediate:', collection);
return collection.filter(x => x > 3);
})
.value();
console.log('Result:', result);
// Output:
// Intermediate: [2, 4, 6]
// Result: [4, 6]
In this example, the array [1, 2, 3] is first mapped to [2, 4, 6]. Then, _.thru()
intercepts the sequence, allowing for intermediate processing (filtering values greater than 3). Finally, the result is obtained after the chain is completed.
🏆 Best Practices
When working with the _.thru()
method, consider the following best practices:
Intermediate Transformations:
Utilize
_.thru()
for intermediate transformations within a sequence to modify or inspect the data at different stages of processing.example.jsCopiedconst result = _([1, 2, 3]) .map(x => x * 2) .thru(collection => { console.log('Intermediate:', collection); return collection.filter(x => x > 3); }) .value(); console.log('Result:', result);
Debugging and Inspection:
Leverage
_.thru()
for debugging purposes by inserting logging statements or inspection logic within the sequence.example.jsCopiedconst result = _([1, 2, 3]) .map(x => x * 2) .thru(collection => { console.log('Intermediate:', collection); return collection.filter(x => x > 3); }) .value(); console.log('Result:', result);
Error Handling:
Implement error handling or validation checks within the sequence using
_.thru()
to ensure data integrity and handle edge cases.example.jsCopiedconst result = _([1, 2, 3]) .map(x => x * 2) .thru(collection => { if(!Array.isArray(collection)) { throw new Error('Invalid data format'); } return collection.filter(x => x > 3); }) .value(); console.log('Result:', result);
📚 Use Cases
Data Transformation Pipelines:
_.thru()
is well-suited for constructing data transformation pipelines where data undergoes multiple stages of processing.example.jsCopiedconst transformedData = _(initialData) .thru(data => preprocess(data)) .thru(data => applyBusinessLogic(data)) .thru(data => postProcess(data)) .value();
Conditional Processing:
In scenarios where conditional processing is required based on intermediate results,
_.thru()
can be employed to dynamically adjust the sequence.example.jsCopiedconst result = _(data) .thru(data => condition ? processData(data) : data) .value();
Functional Programming Paradigm:
Adopt a functional programming approach by composing sequences of operations using
_.thru()
to enhance code modularity and reusability.example.jsCopiedconst result = _([1, 2, 3]) .thru(data => transform(data)) .thru(data => filter(data)) .thru(data => process(data)) .value();
🎉 Conclusion
The _.thru()
Seq method in Lodash offers a powerful mechanism for chaining and transforming data through a sequence of operations. By strategically incorporating _.thru()
into your code, you can enhance readability, facilitate debugging, and ensure data integrity within your data processing pipelines.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.thru()
method in your Lodash projects.
👨💻 Join our Community:
Author
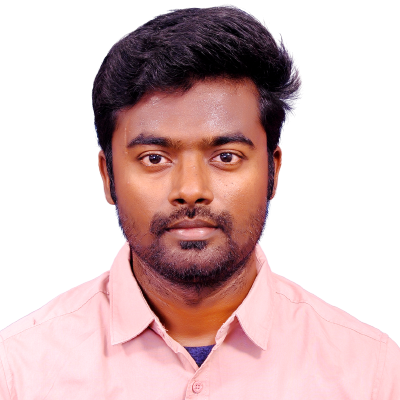
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
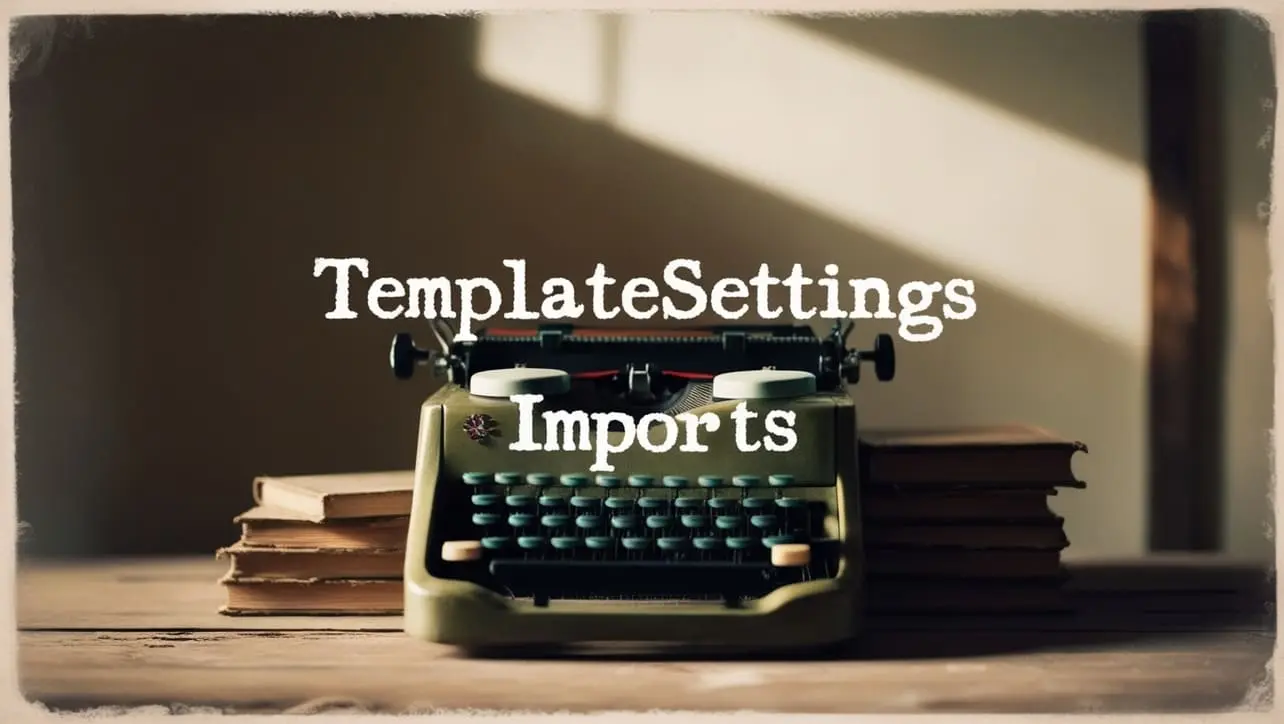
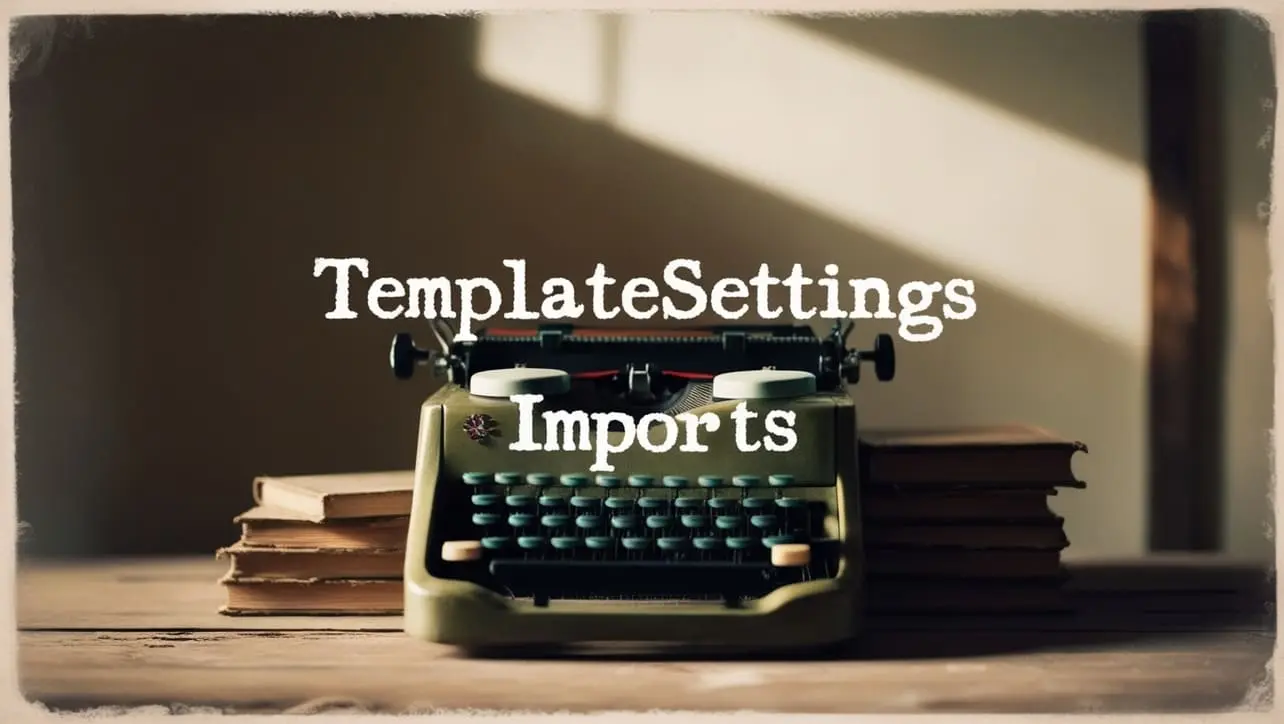
Lodash _.templateSettings.imports Property
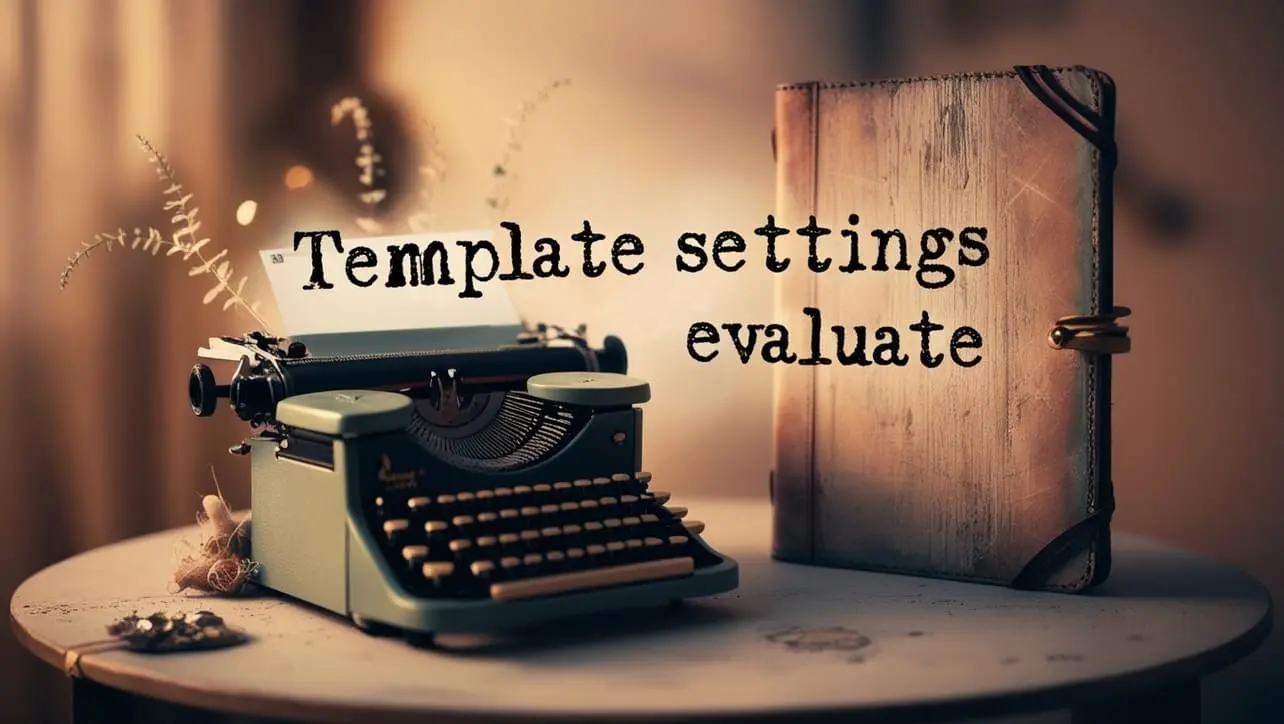
Lodash _.templateSettings.evaluate Property
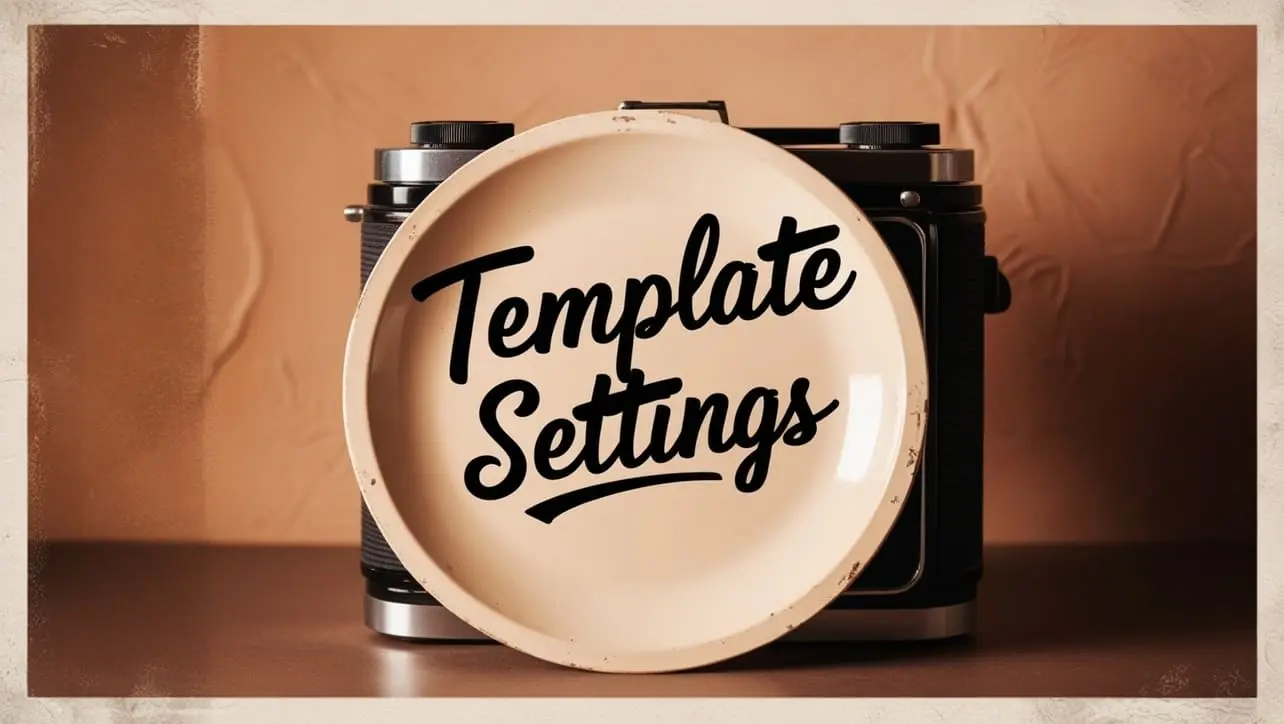
Lodash _.templateSettings Property
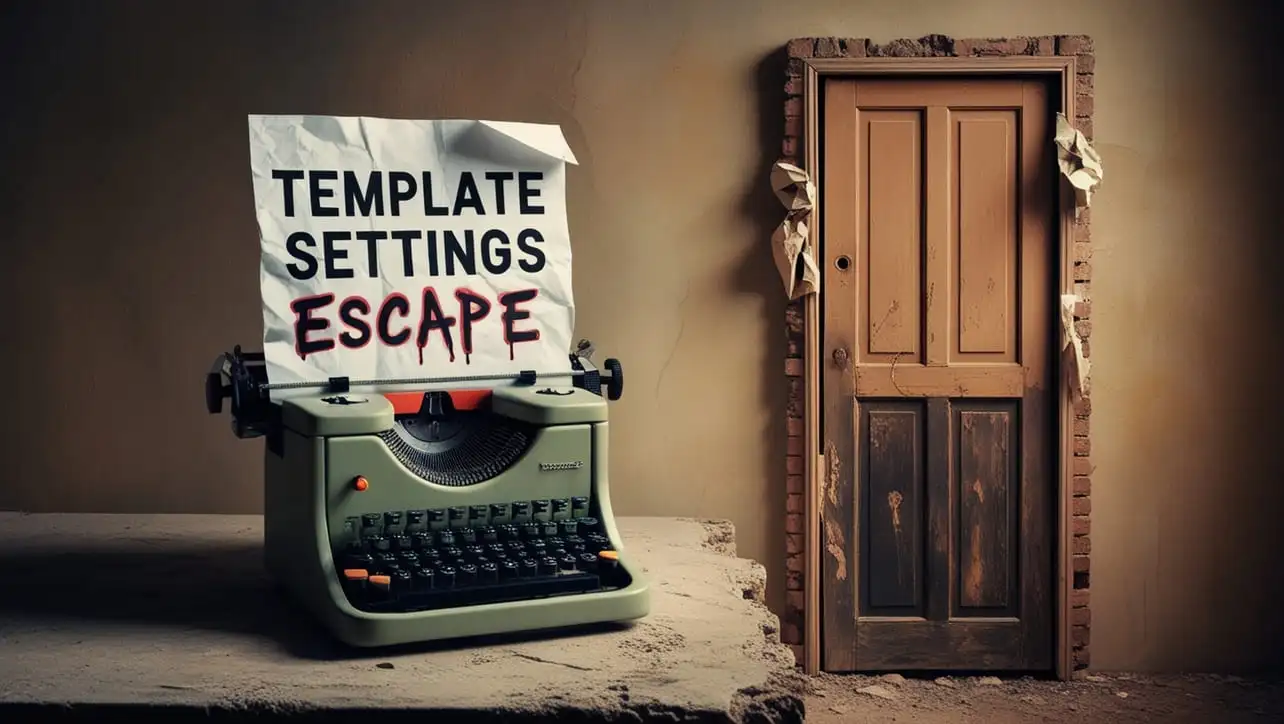
Lodash _.templateSettings.escape Property
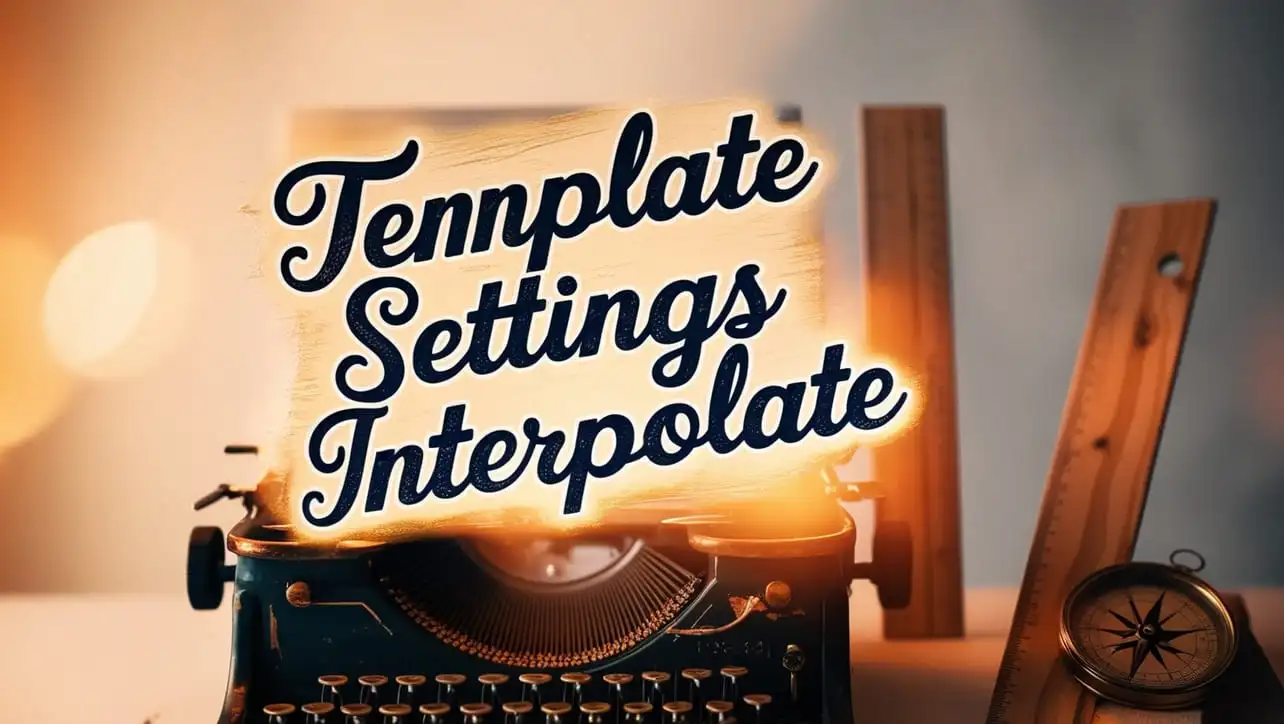
Lodash _.templateSettings.interpolate Property
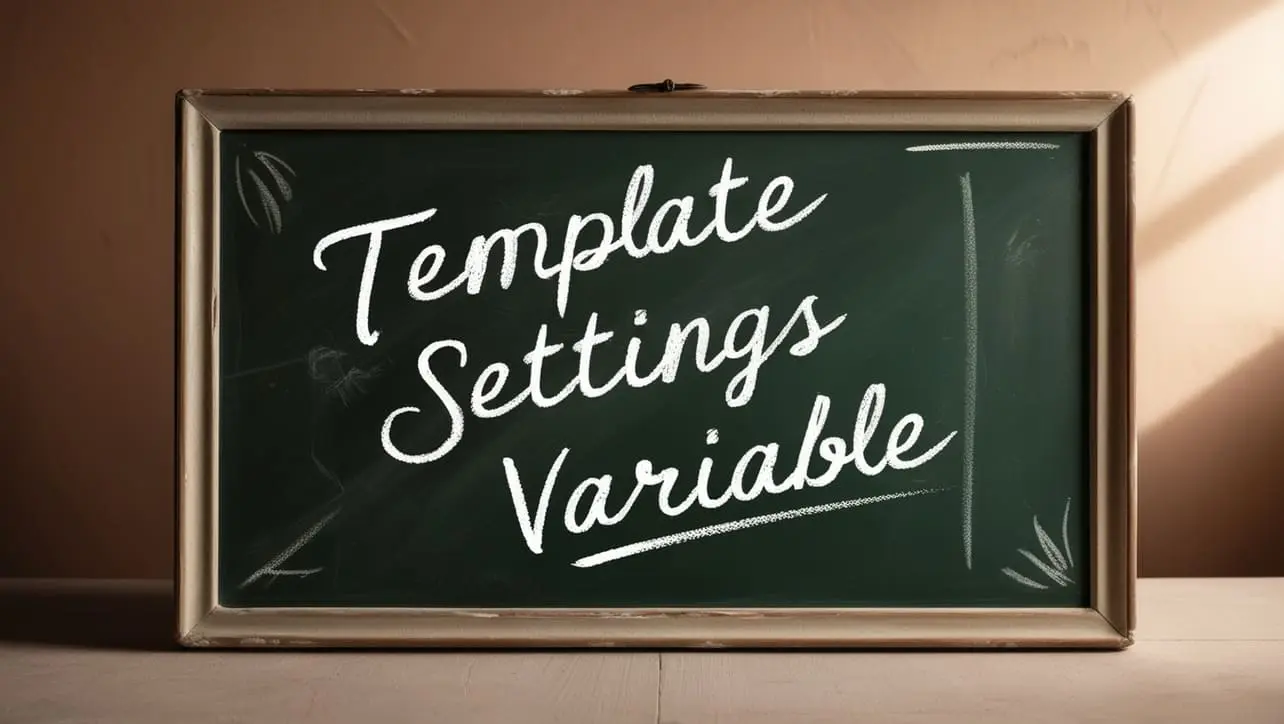
If you have any doubts regarding this article (Lodash _.thru() Seq Method), please comment here. I will help you immediately.