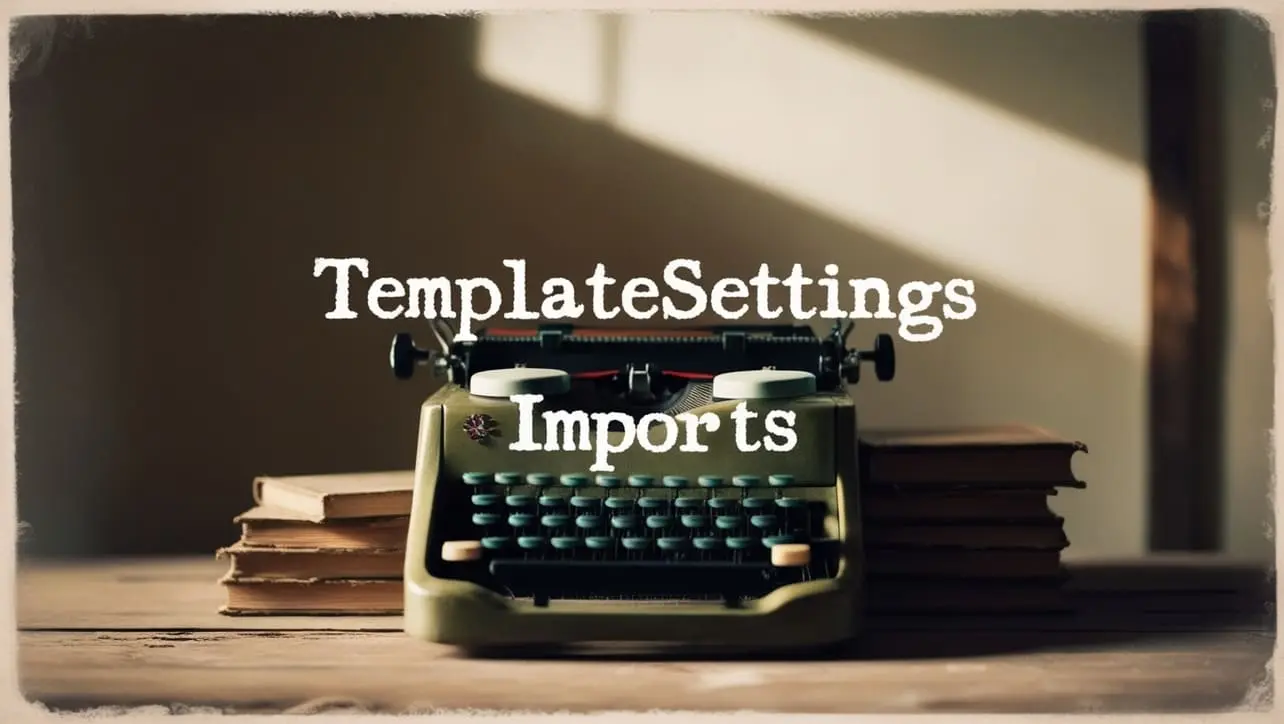
Lodash _.tap() Seq Method
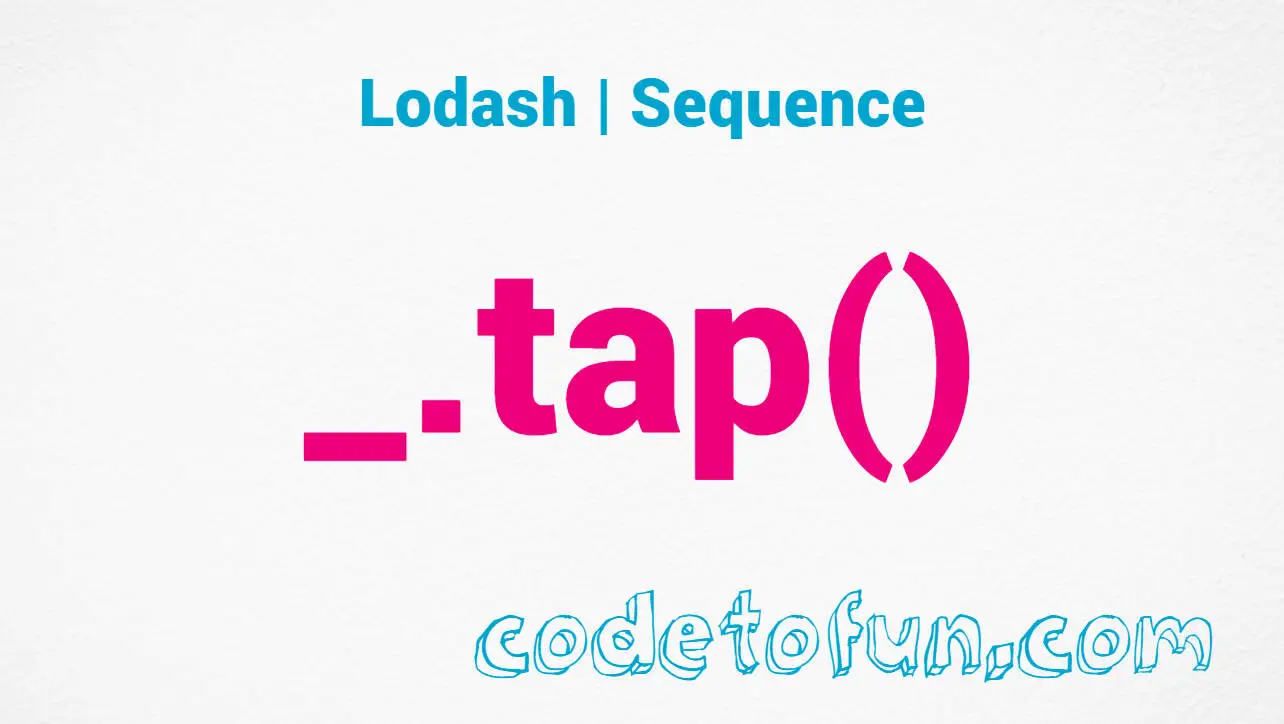
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript development, chaining operations on data is a common practice to perform multiple transformations in a concise and readable manner. Lodash offers a powerful utility method called _.tap()
in its Seq module, which allows developers to inspect and manipulate data within a chain without altering the chain's flow.
This method facilitates cleaner and more expressive code, enhancing the readability and maintainability of JavaScript projects.
🧠 Understanding _.tap() Method
The _.tap()
method in Lodash is part of the Seq module, designed to intercept values in a chain and apply side effects or additional operations without interrupting the chain's flow. It enables developers to inspect intermediate results, debug code, or perform auxiliary actions while maintaining the integrity of the chain.
💡 Syntax
The syntax for the _.tap()
method is straightforward:
_.tap(value, interceptor)
- value: The value to tap into.
- interceptor: The function invoked with the tapped value.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.tap()
method:
const _ = require('lodash');
const result = _.chain([1, 2, 3, 4])
.map(num => num * 2)
.tap(console.log)
.filter(num => num % 4 === 0)
.value();
console.log(result);
// Output:
// [2, 4, 6, 8]
// [4, 8]
In this example, the _.tap()
method intercepts the intermediate result of the map() operation, allowing developers to inspect the mapped array before proceeding with the filter() operation.
🏆 Best Practices
When working with the _.tap()
method, consider the following best practices:
Debugging Chains:
Use
_.tap()
for debugging chains by inspecting intermediate values or performing console logging within the chain. This can help identify issues or anomalies during data processing.example.jsCopiedconst result = _.chain(data) .map(transformFunction) .tap(console.log) // Debugging intermediate result .filter(filterFunction) .value();
Side Effects:
Employ
_.tap()
to apply side effects or perform auxiliary actions within a chain without altering its flow. This can include logging, data validation, or triggering external processes.example.jsCopiedconst result = _.chain(data) .map(transformFunction) .tap(validateData) .filter(filterFunction) .value();
Code Readability:
Enhance code readability by using
_.tap()
to segregate side effects or auxiliary operations from the main data processing logic. This improves code organization and makes the chain's purpose clearer.example.jsCopiedconst result = _.chain(data) .map(transformFunction) .tap(logTransformedData) .filter(filterFunction) .value();
📚 Use Cases
Data Transformation:
_.tap()
is valuable when transforming data in a chain while needing to inspect intermediate results or apply additional operations.example.jsCopiedconst processedData = _.chain(data) .map(transformFunction) .tap(console.log) // Inspect transformed data .filter(filterFunction) .value();
Logging and Debugging:
When debugging or logging data processing pipelines,
_.tap()
allows developers to insert logging statements without disrupting the chain's flow.example.jsCopiedconst result = _.chain(data) .map(transformFunction) .tap(console.log) // Debugging intermediate result .filter(filterFunction) .value();
Validation and Side Effects:
For scenarios requiring data validation or triggering side effects within a chain,
_.tap()
offers a convenient mechanism to perform such operations.example.jsCopiedconst result = _.chain(data) .map(transformFunction) .tap(validateData) .filter(filterFunction) .value();
🎉 Conclusion
The _.tap()
method in Lodash's Seq module provides a powerful tool for intercepting values within chains, enabling developers to inspect intermediate results, apply side effects, or perform auxiliary actions without disrupting the chain's flow. By leveraging _.tap()
, JavaScript developers can write cleaner, more expressive code and streamline data processing pipelines with confidence
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.tap()
method in your Lodash projects.
👨💻 Join our Community:
Author
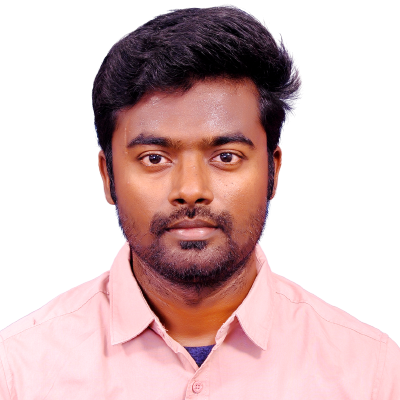
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
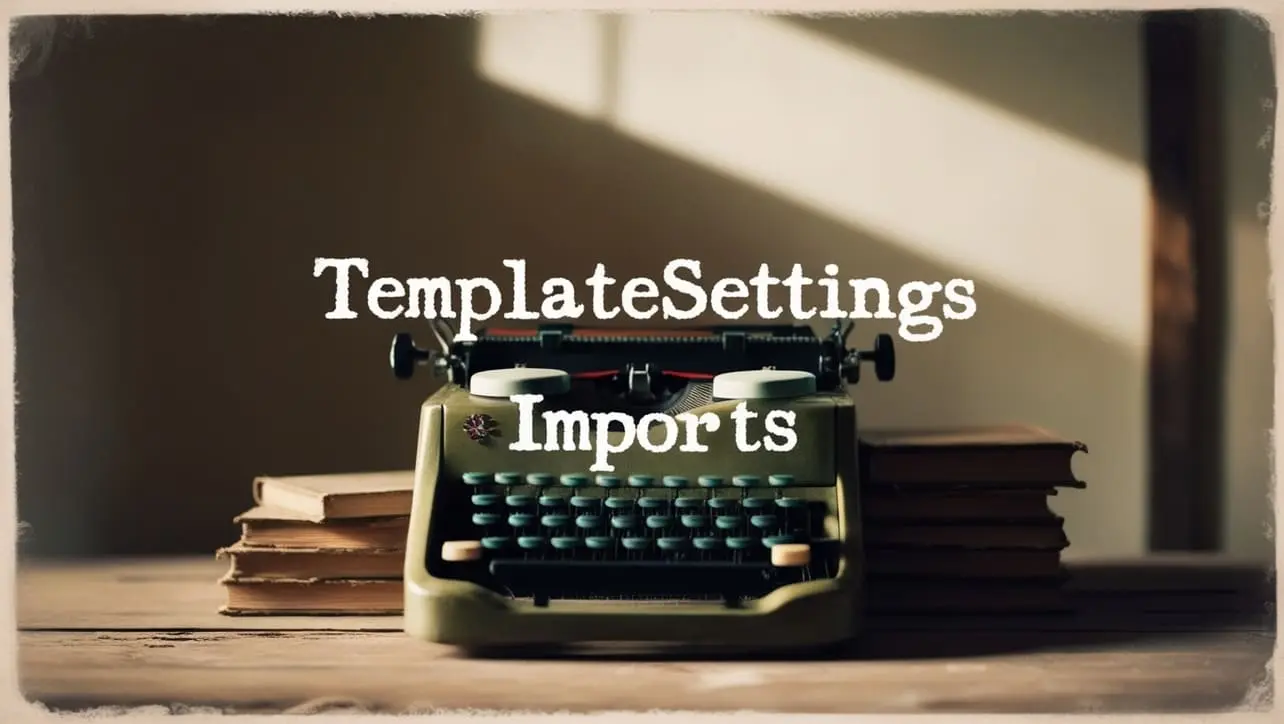
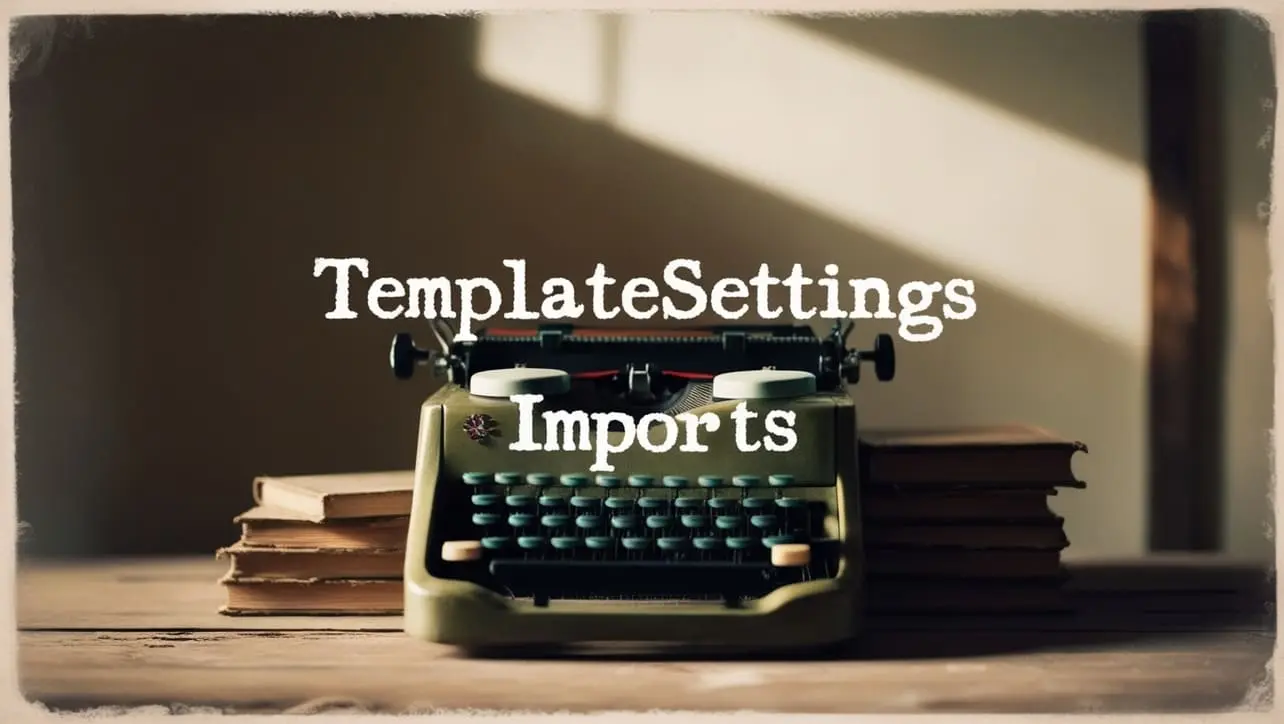
Lodash _.templateSettings.imports Property
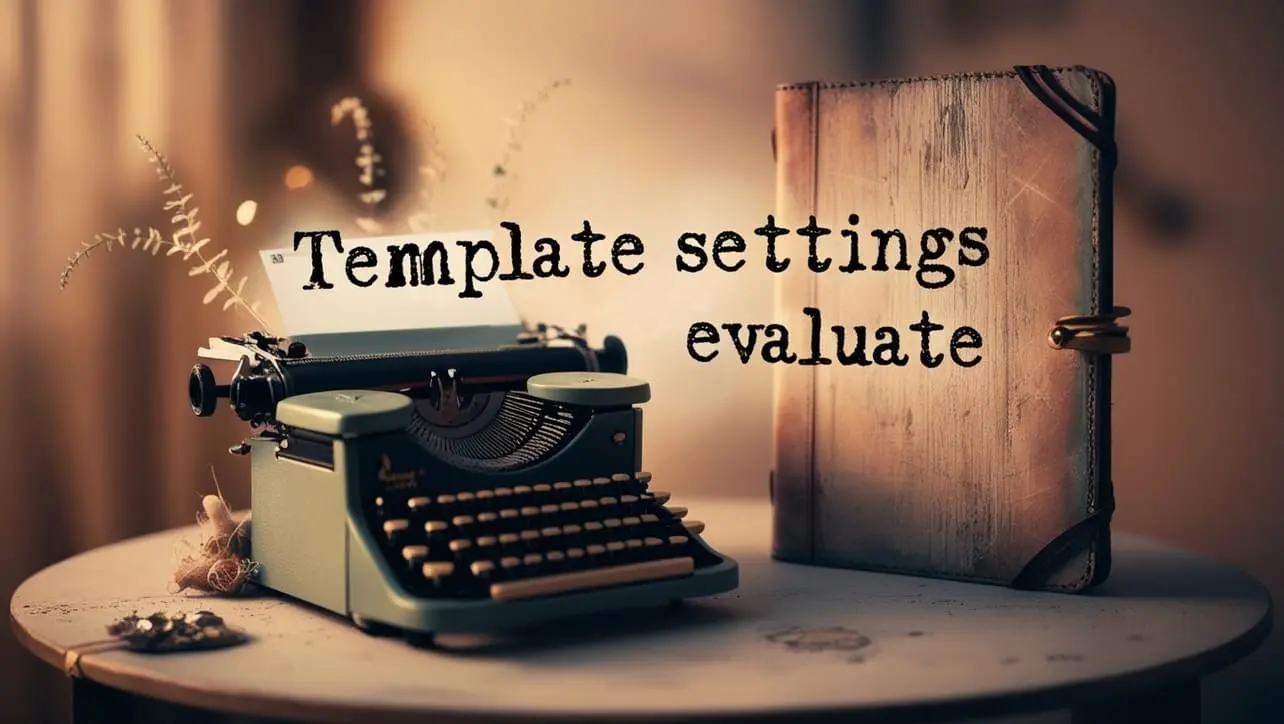
Lodash _.templateSettings.evaluate Property
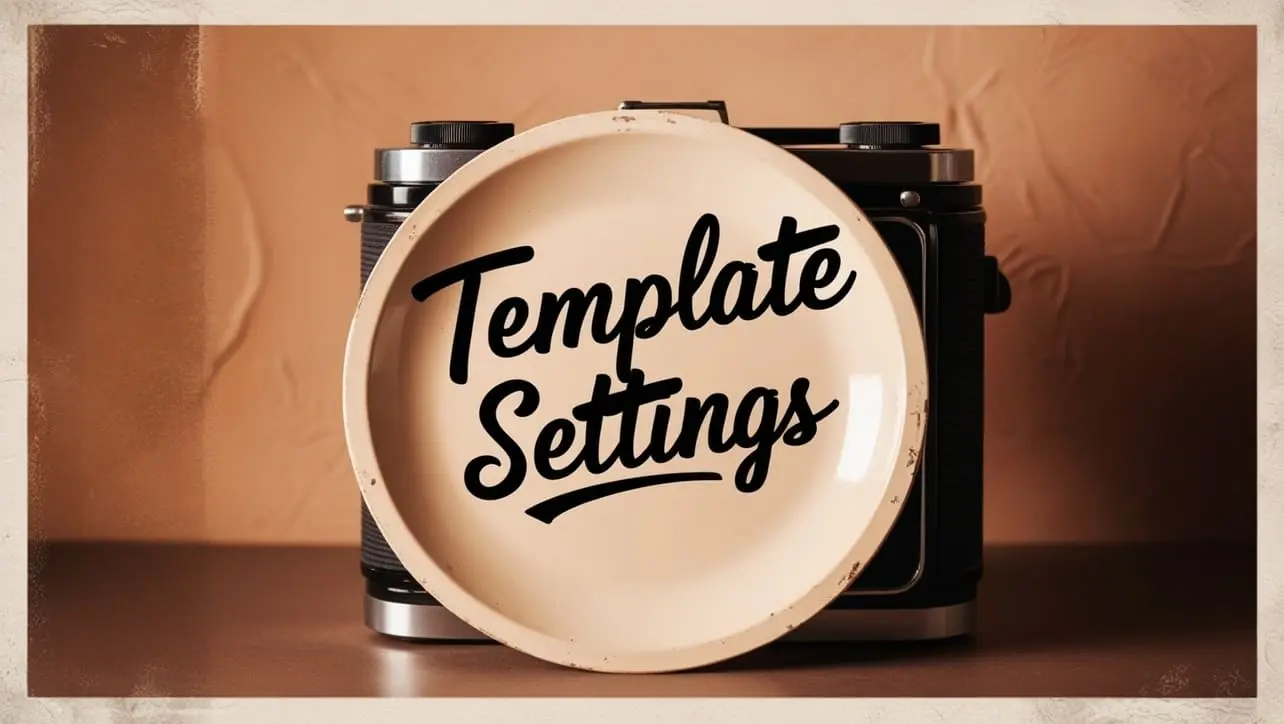
Lodash _.templateSettings Property
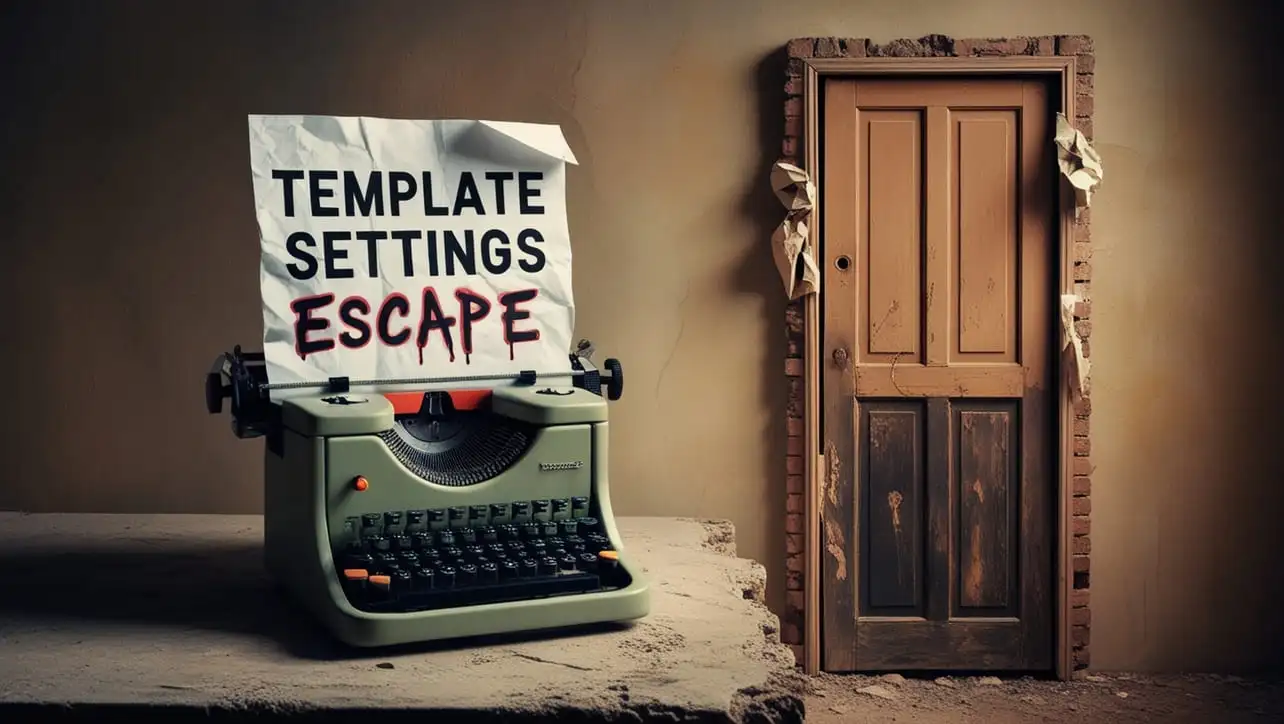
Lodash _.templateSettings.escape Property
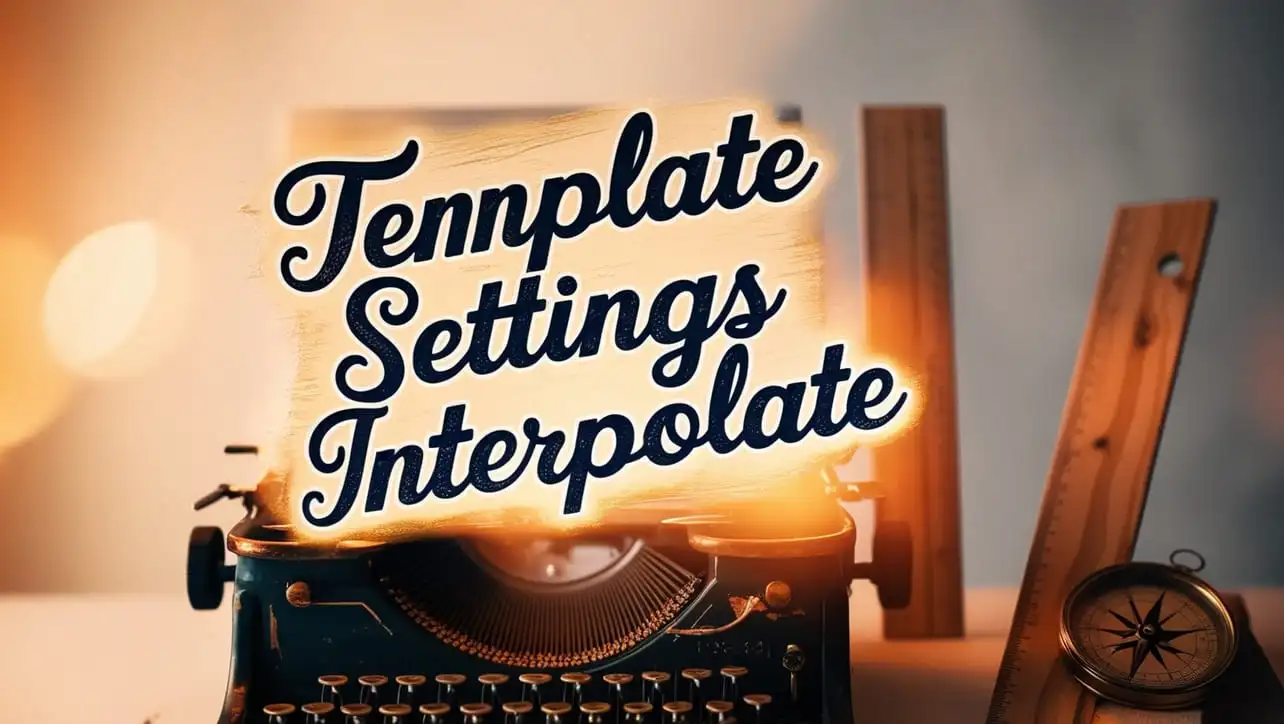
Lodash _.templateSettings.interpolate Property
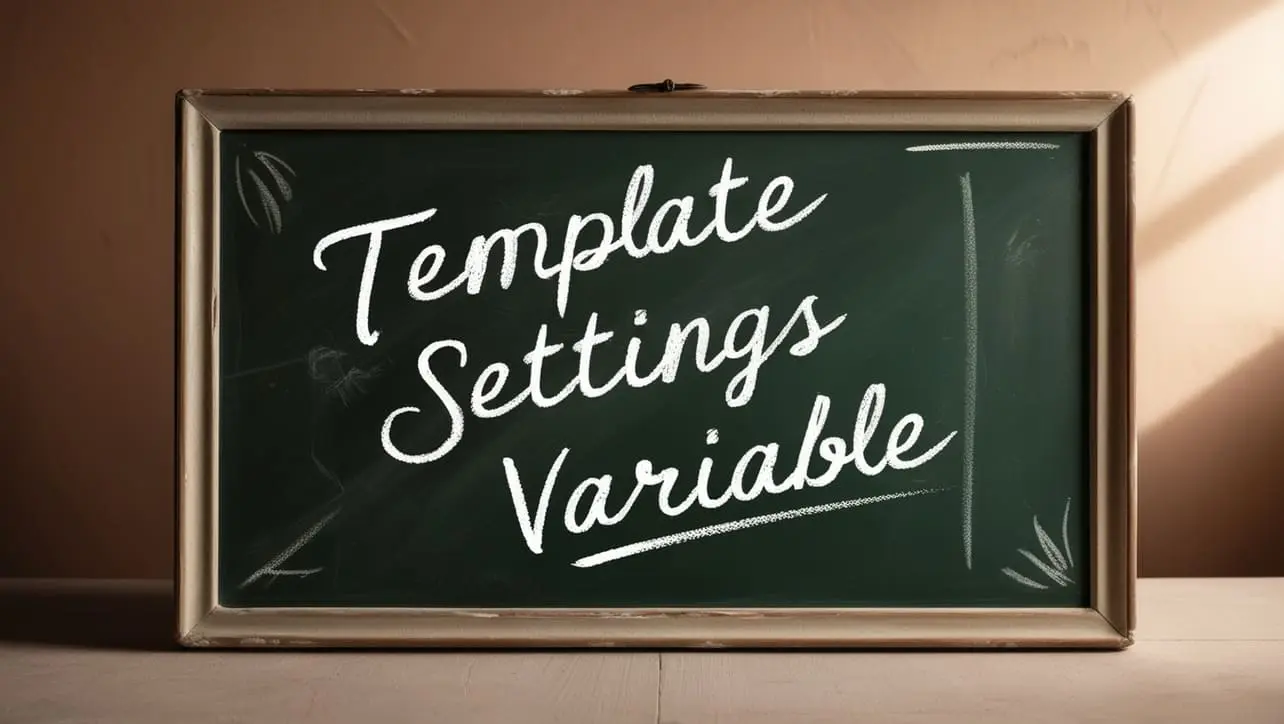
If you have any doubts regarding this article (Lodash _.tap() Seq Method), please comment here. I will help you immediately.