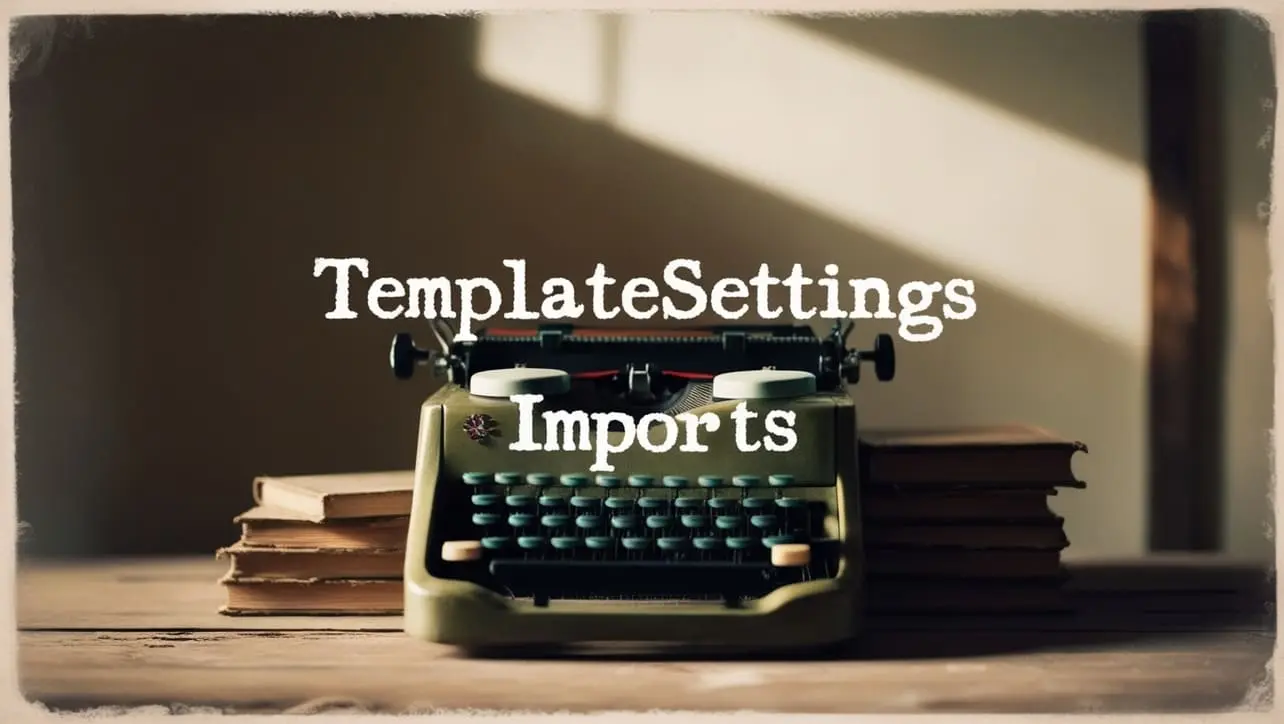
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- _.attempt
- _.bindAll
- _.cond
- _.conforms
- _.constant
- _.defaultTo
- _.flow
- _.flowRight
- _.identity
- _.iteratee
- _.matches
- _.matchesProperty
- _.method
- _.methodOf
- _.mixin
- _.noConflict
- _.noop
- _.nthArg
- _.over
- _.overEvery
- _.overSome
- _.property
- _.propertyOf
- _.range
- _.rangeRight
- _.runInContext
- _.stubArray
- _.stubFalse
- _.stubObject
- _.stubString
- _.stubTrue
- _.times
- _.toPath
- _.uniqueId
- Lodash Properties
- Lodash Methods
Lodash _.range() Util Method
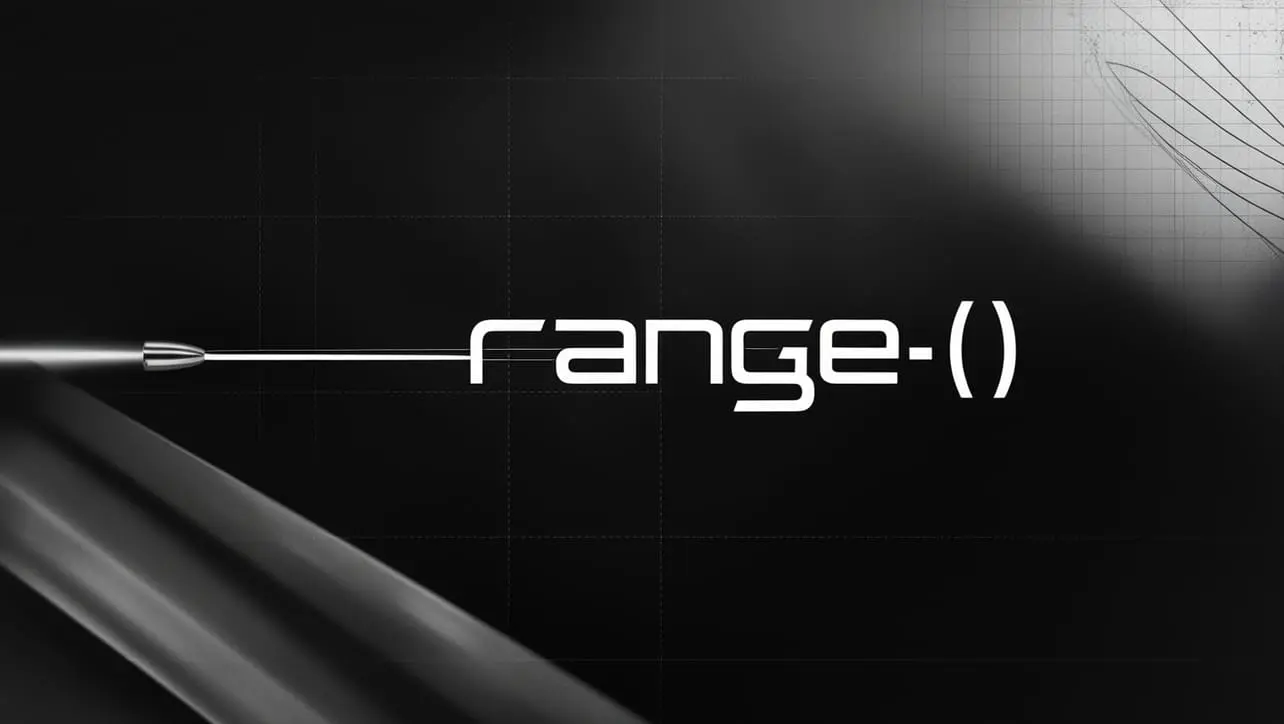
Photo Credit to CodeToFun
🙋 Introduction
When it comes to generating a range of numbers in JavaScript, developers often face challenges, especially when needing to create sequences with specific starting points, ending points, and step values. Lodash, a popular utility library, provides a solution to this with its _.range()
method.
This method simplifies the process of generating sequences of numbers with customizable parameters, offering flexibility and convenience for JavaScript developers.
🧠 Understanding _.range() Method
The _.range()
method in Lodash allows you to create an array of numbers forming a specified arithmetic progression. This progression can be defined by providing parameters such as the start, end, and step values, enabling precise control over the generated sequence.
💡 Syntax
The syntax for the _.range()
method is straightforward:
_.range([start=0], end, [step=1])
- start: The starting value of the sequence (default is 0).
- end: The end value (exclusive) of the sequence.
- step: The value to increment or decrement by (default is 1).
📝 Example
Let's dive into a simple example to illustrate the usage of the _.range()
method:
const _ = require('lodash');
const sequence = _.range(1, 6, 2);
console.log(sequence);
// Output: [1, 3, 5]
In this example, _.range(1, 6, 2) generates a sequence starting from 1 (inclusive), ending at 6 (exclusive), and incrementing by 2.
🏆 Best Practices
When working with the _.range()
method, consider the following best practices:
Understanding Parameters:
Ensure clarity on the parameters provided to
_.range()
, especially regarding the start, end, and step values. This understanding is crucial for generating the desired sequence accurately.example.jsCopiedconst sequenceWithDefaults = _.range(5); const sequenceWithStep = _.range(1, 10, 2); console.log(sequenceWithDefaults); // Output: [0, 1, 2, 3, 4] console.log(sequenceWithStep); // Output: [1, 3, 5, 7, 9]
Handling Reversed Sequences:
Take into account the behavior of
_.range()
when generating reversed sequences (i.e., when the start value is greater than the end value). Adjust parameters accordingly to obtain the desired result.example.jsCopiedconst reversedSequence = _.range(5, 1, -1); console.log(reversedSequence); // Output: [5, 4, 3, 2]
Performance Considerations:
Consider the performance implications when generating large sequences using
_.range()
. While Lodash is optimized for performance, generating extremely large arrays may impact memory usage and execution time.example.jsCopiedconst largeSequence = _.range(1000000); console.log(largeSequence.length); // Output: 1000000
📚 Use Cases
Loop Iteration:
_.range()
is commonly used in conjunction with loops to iterate over a range of values, facilitating repetitive tasks such as data processing and calculations.example.jsCopiedconst numIterations = 5; for(let i of _.range(numIterations)) { console.log(`Iteration ${i + 1}`); }
Generating Number Ranges:
When dealing with scenarios requiring the generation of number ranges, such as pagination or data visualization,
_.range()
simplifies the process by providing a convenient way to create sequences of numbers.example.jsCopiedconst currentPage = 1; const itemsPerPage = 10; const totalItems = 87; const pageNumbers = _.range(1, Math.ceil(totalItems / itemsPerPage) + 1); console.log(pageNumbers);
Custom Sequence Generation:
For specialized tasks requiring custom sequences of numbers, such as creating arrays for mathematical computations or simulations,
_.range()
offers the flexibility to define precise sequences tailored to specific requirements.example.jsCopiedconst fibonacciSequence = [0, 1, ..._.range(2, 10).map(i => fibonacciSequence[i - 1] + fibonacciSequence[i - 2])]; console.log(fibonacciSequence);
🎉 Conclusion
The _.range()
method in Lodash provides a versatile solution for generating sequences of numbers with customizable parameters. Whether you need to iterate over a range of values, generate number ranges, or create custom sequences, _.range()
offers a convenient and efficient way to accomplish these tasks in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.range()
method in your Lodash projects.
👨💻 Join our Community:
Author
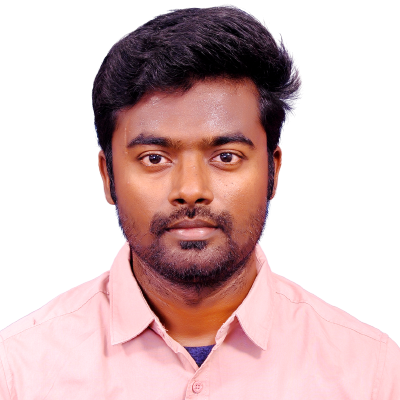
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
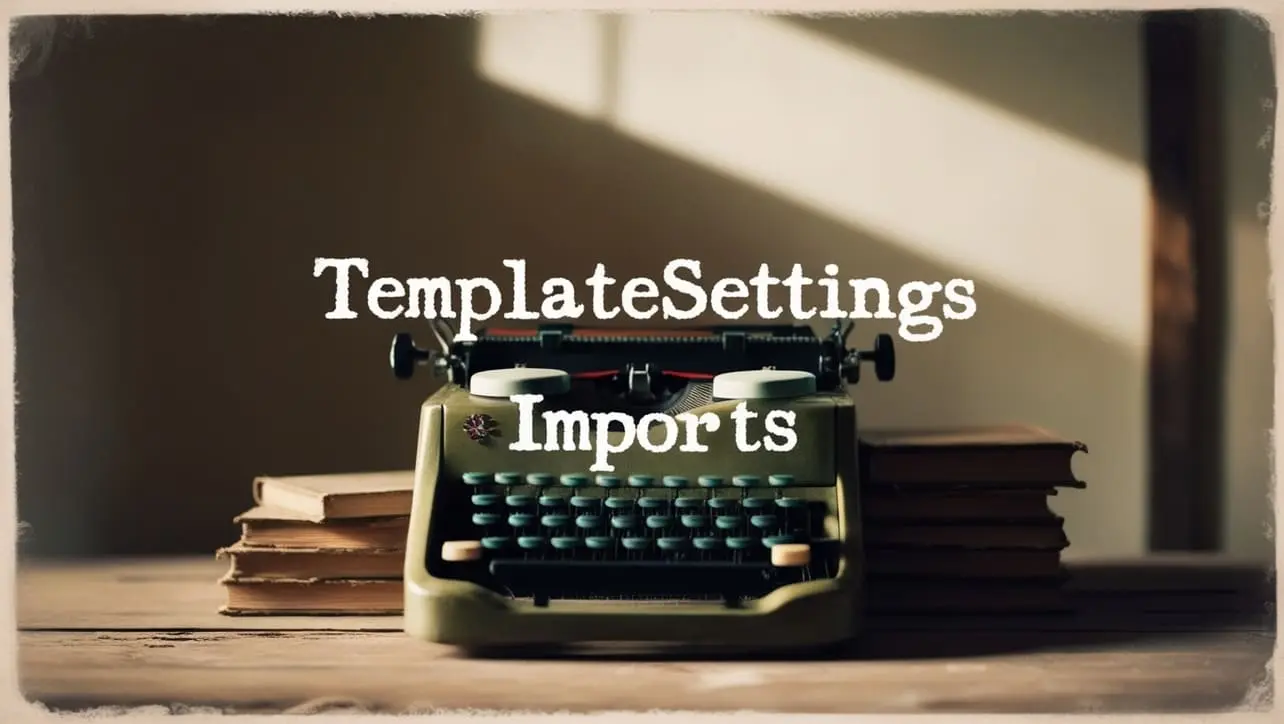
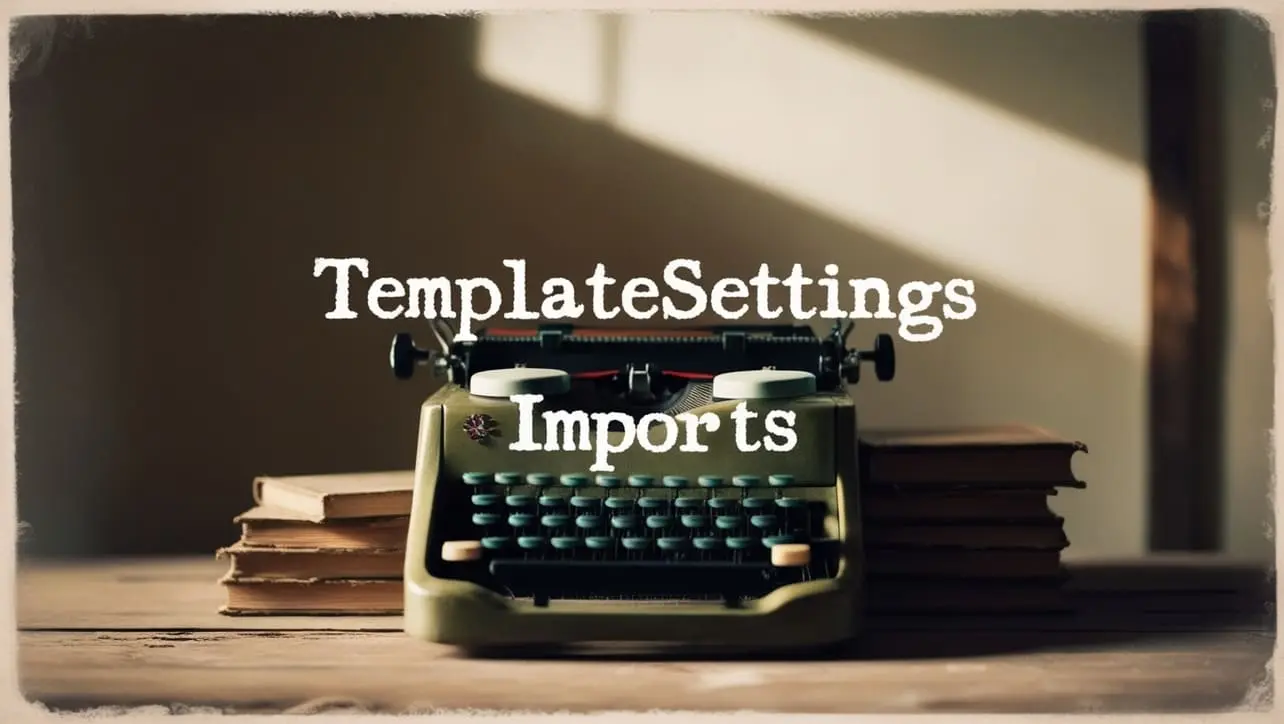
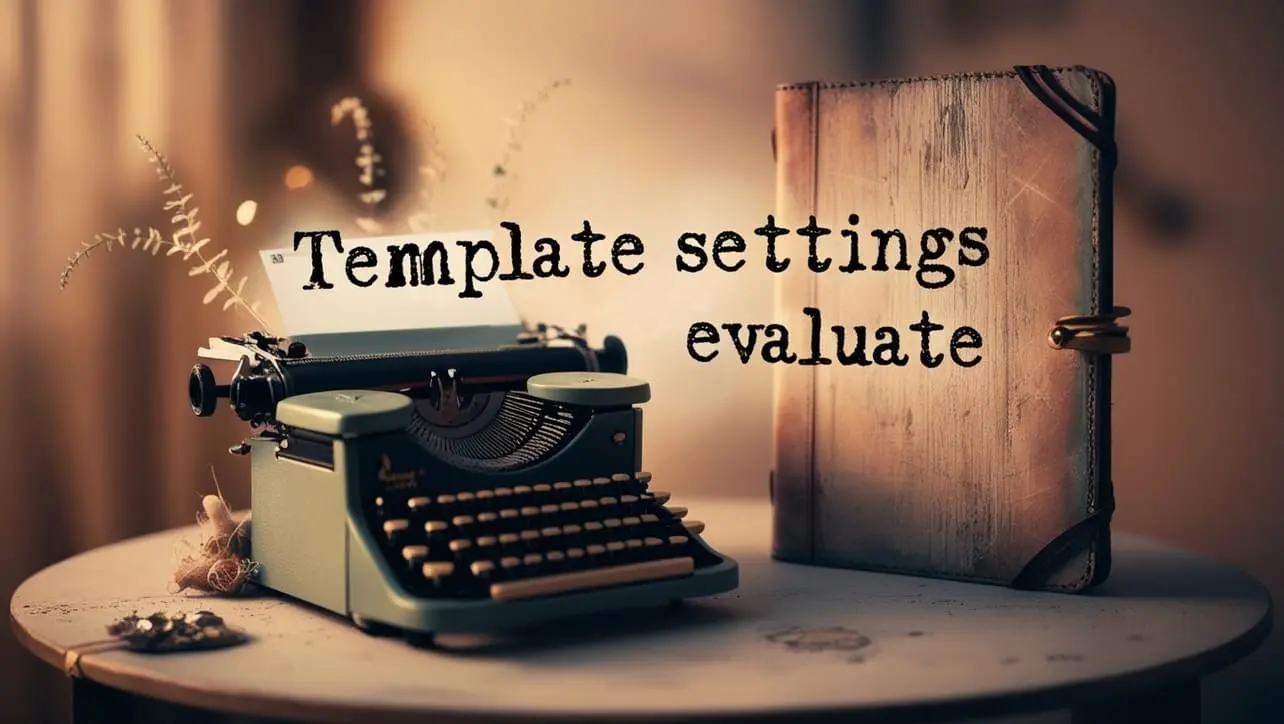
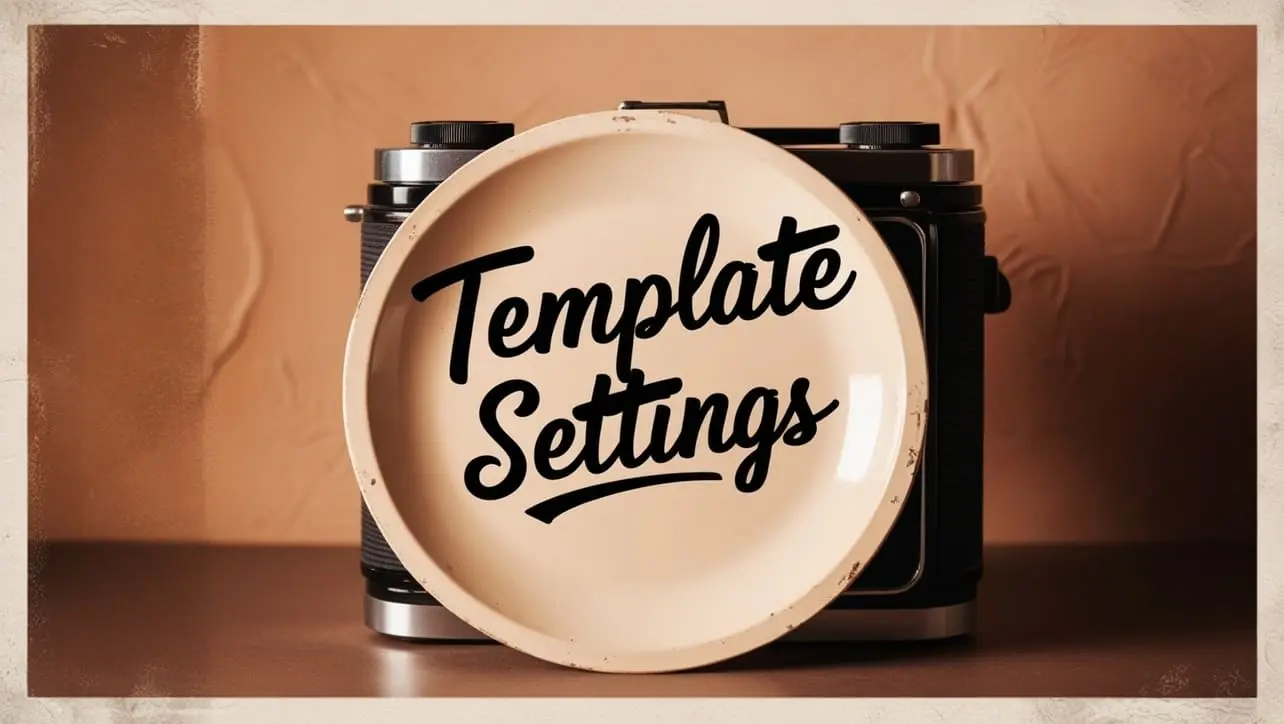
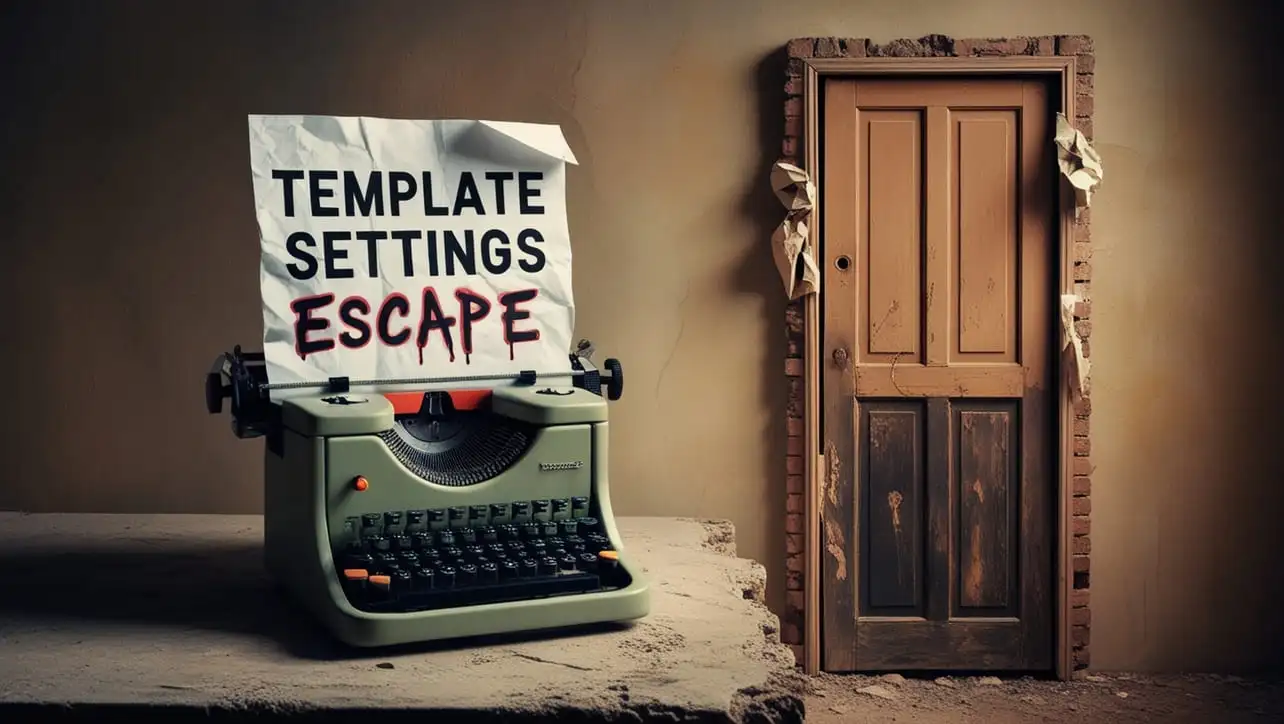
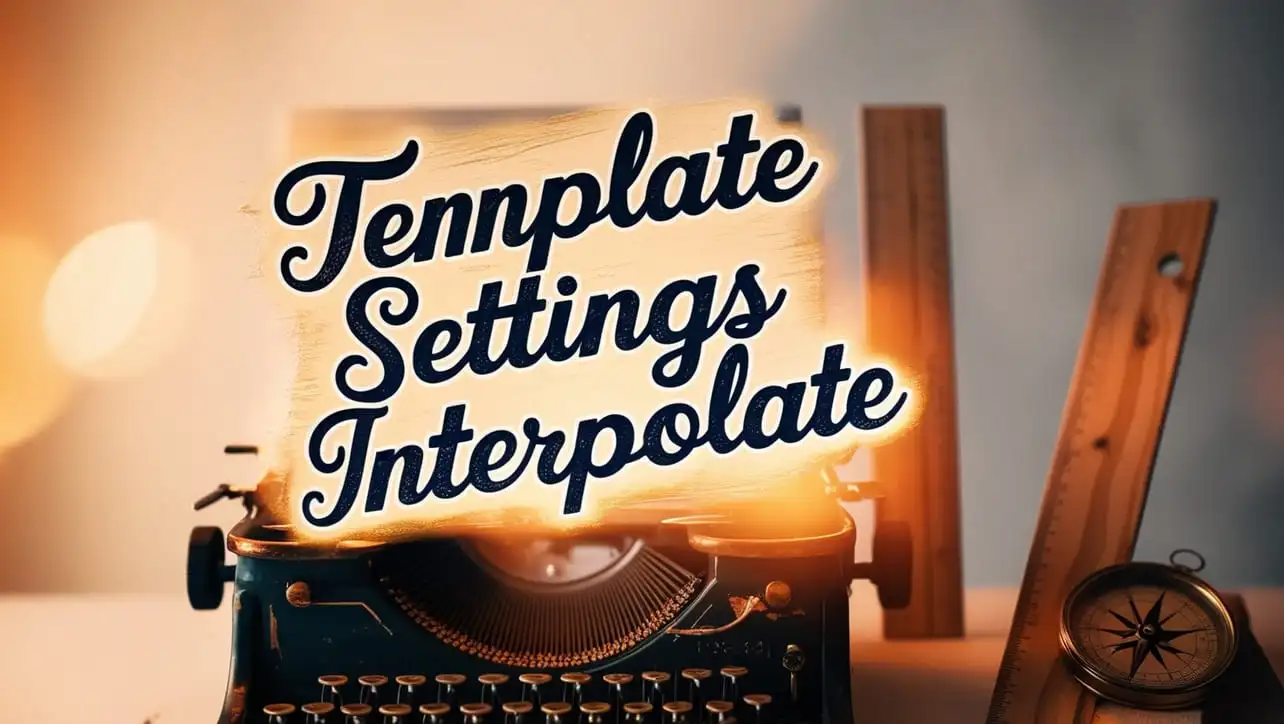
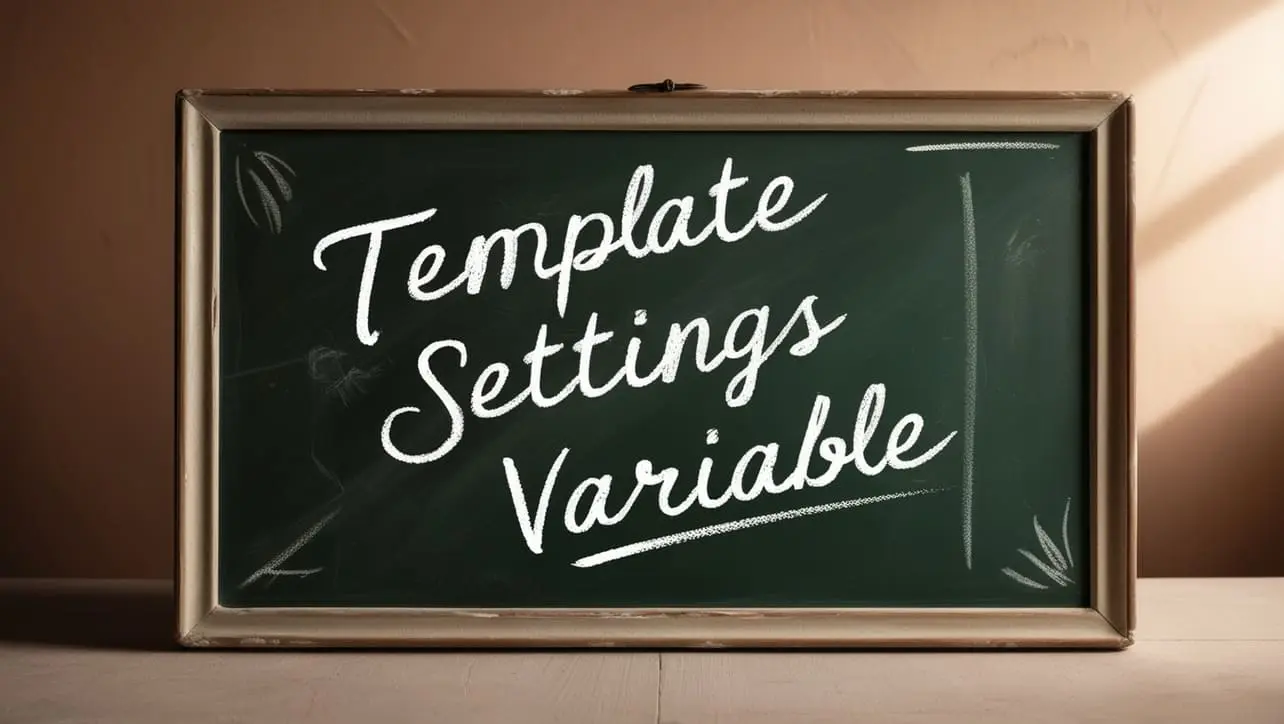
If you have any doubts regarding this article (Lodash _.range() Util Method), please comment here. I will help you immediately.