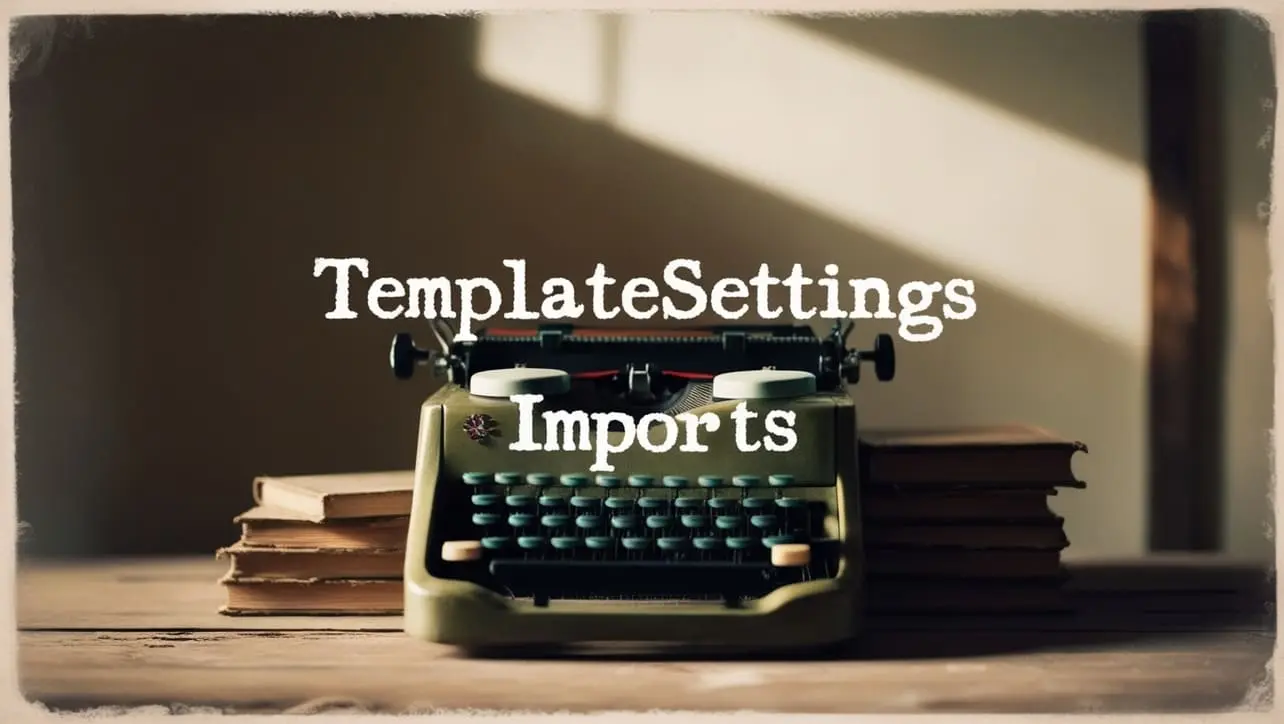
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- _.attempt
- _.bindAll
- _.cond
- _.conforms
- _.constant
- _.defaultTo
- _.flow
- _.flowRight
- _.identity
- _.iteratee
- _.matches
- _.matchesProperty
- _.method
- _.methodOf
- _.mixin
- _.noConflict
- _.noop
- _.nthArg
- _.over
- _.overEvery
- _.overSome
- _.property
- _.propertyOf
- _.range
- _.rangeRight
- _.runInContext
- _.stubArray
- _.stubFalse
- _.stubObject
- _.stubString
- _.stubTrue
- _.times
- _.toPath
- _.uniqueId
- Lodash Properties
- Lodash Methods
Lodash _.property() Util Method
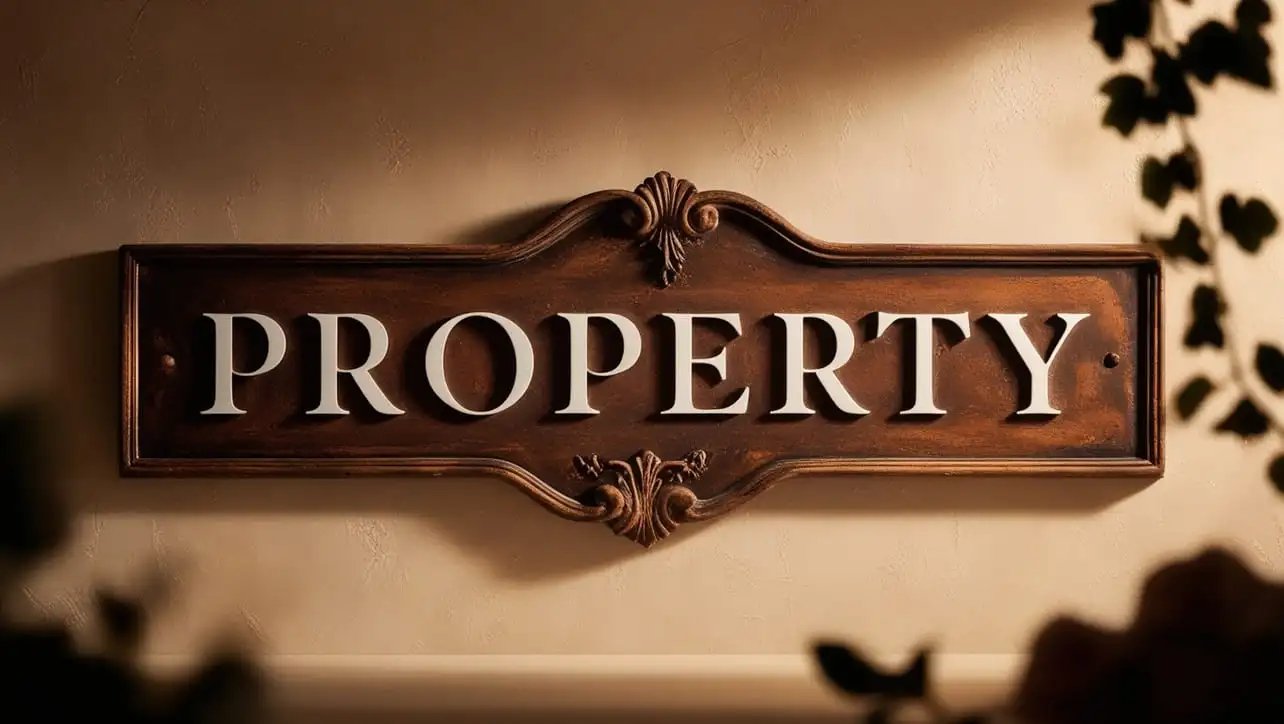
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript development, accessing nested properties of objects often requires verbose code or error-prone manual checks. Enter Lodash, a powerful utility library that simplifies common programming tasks. Among its arsenal of functions lies the _.property()
method, a concise and efficient solution for accessing nested properties of objects.
This method streamlines code, improves readability, and reduces the risk of errors, making it a valuable tool for JavaScript developers.
🧠 Understanding _.property() Method
The _.property()
method in Lodash allows you to create a function that returns the value of a specified property path within an object. This eliminates the need for manual checks and provides a clean and concise way to access nested properties.
💡 Syntax
The syntax for the _.property()
method is straightforward:
_.property(path)
- path: The property path to retrieve.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.property()
method:
const _ = require('lodash');
const user = {
id: 1,
name: 'John Doe',
address: {
city: 'New York',
zip: '10001'
}
};
const getCity = _.property('address.city');
console.log(getCity(user));
// Output: 'New York'
In this example, the getCity function created by _.property('address.city') accesses the nested property address.city of the user object, returning its value.
🏆 Best Practices
When working with the _.property()
method, consider the following best practices:
Simplify Property Access:
Use
_.property()
to simplify property access, especially when dealing with nested objects. This method reduces the need for manual checks and makes code more concise.example.jsCopiedconst getAddressZip = _.property('address.zip'); console.log(getAddressZip(user)); // Output: '10001'
Error Handling:
Handle potential errors gracefully when using
_.property()
. Ensure that the property path provided exists in the object to avoid runtime errors.example.jsCopiedconst getCountry = _.property('address.country'); console.log(getCountry(user)); // Output: undefined (No error thrown)
Dynamic Property Access:
Utilize
_.property()
dynamically by passing property paths as variables or using it within functions to access properties based on runtime conditions.example.jsCopiedconst propertyName = 'address.zip'; const getPropertyValue = _.property(propertyName); console.log(getPropertyValue(user)); // Output: '10001'
📚 Use Cases
Data Extraction:
_.property()
is ideal for extracting specific data from objects, especially when dealing with complex nested structures.example.jsCopiedconst getProductPrice = _.property('details.price'); console.log(getProductPrice(product)); // Output: 19.99
Conditional Rendering:
In scenarios where conditional rendering based on object properties is required,
_.property()
provides a convenient way to access those properties.example.jsCopiedconst isUserAdmin = _.property('role.admin'); if(isUserAdmin(user)) { console.log('User is an admin.'); } else { console.log('User is not an admin.'); }
Data Transformation:
_.property()
can be leveraged in data transformation operations to extract and manipulate specific properties of objects efficiently.example.jsCopiedconst getUserFullName = _.property('name'); const userNames = users.map(getUserFullName); console.log(userNames);
🎉 Conclusion
The _.property()
method in Lodash simplifies property access in JavaScript objects, providing a concise and efficient way to handle nested properties. Whether you're extracting data, performing conditional checks, or transforming objects, _.property()
offers a versatile solution that enhances code readability and reduces complexity.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.property()
method in your Lodash projects.
👨💻 Join our Community:
Author
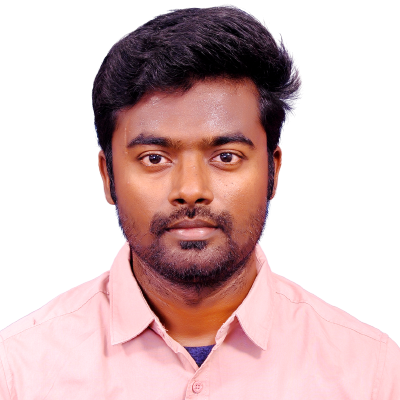
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
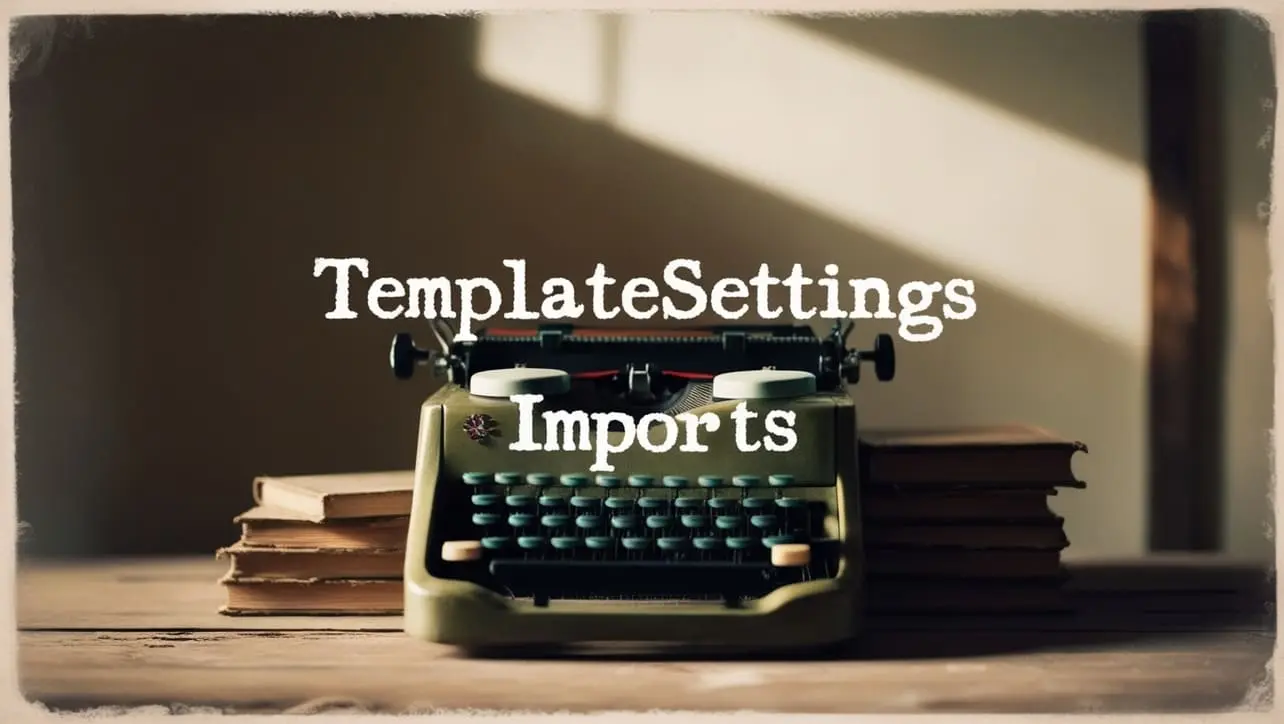
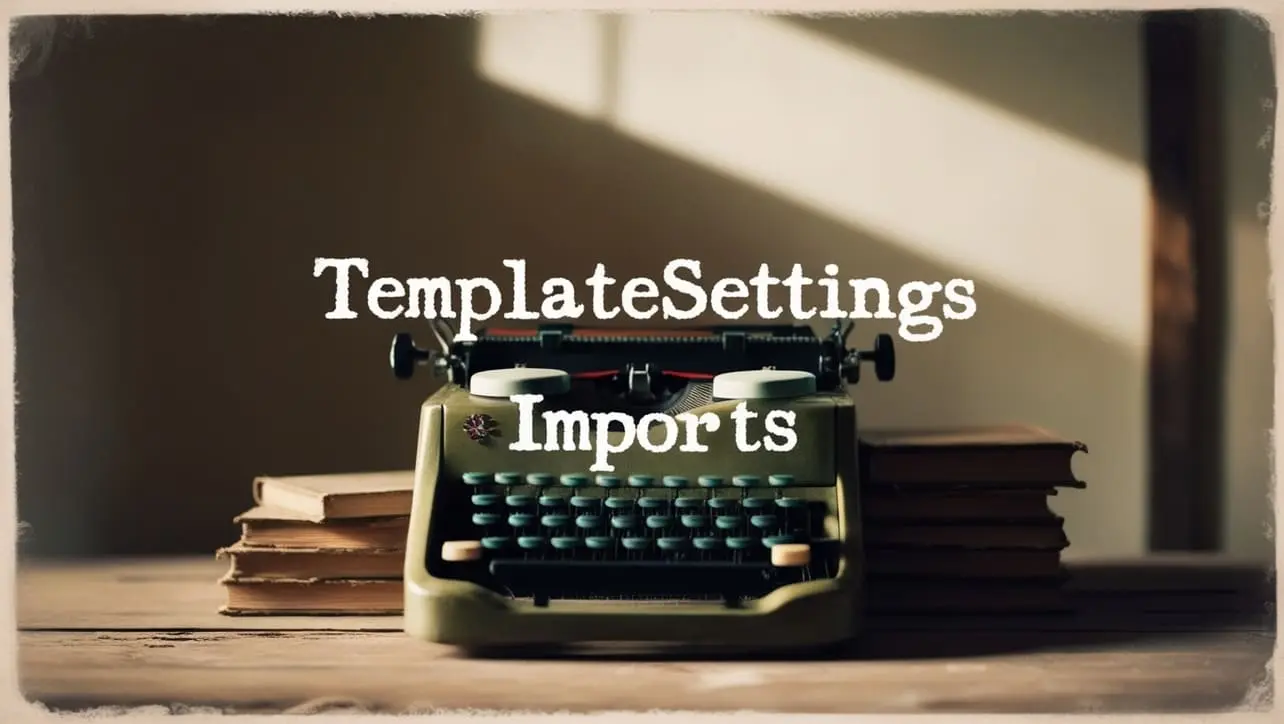
Lodash _.templateSettings.imports Property
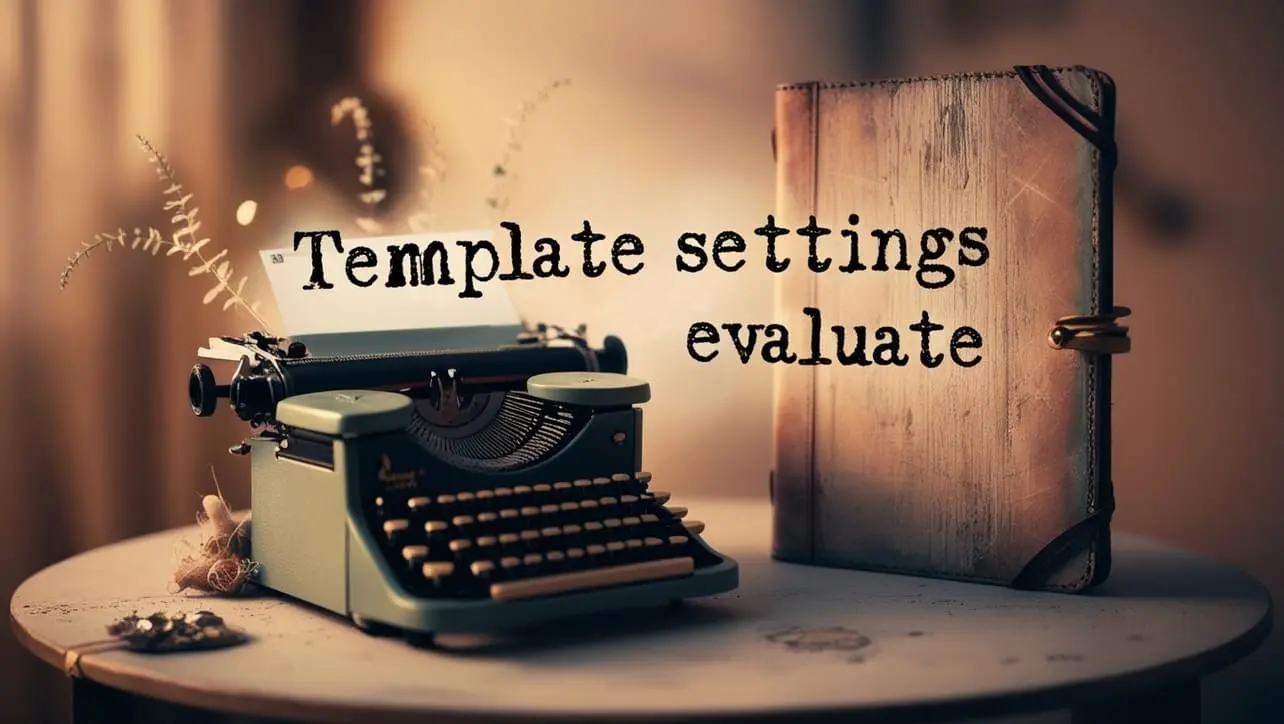
Lodash _.templateSettings.evaluate Property
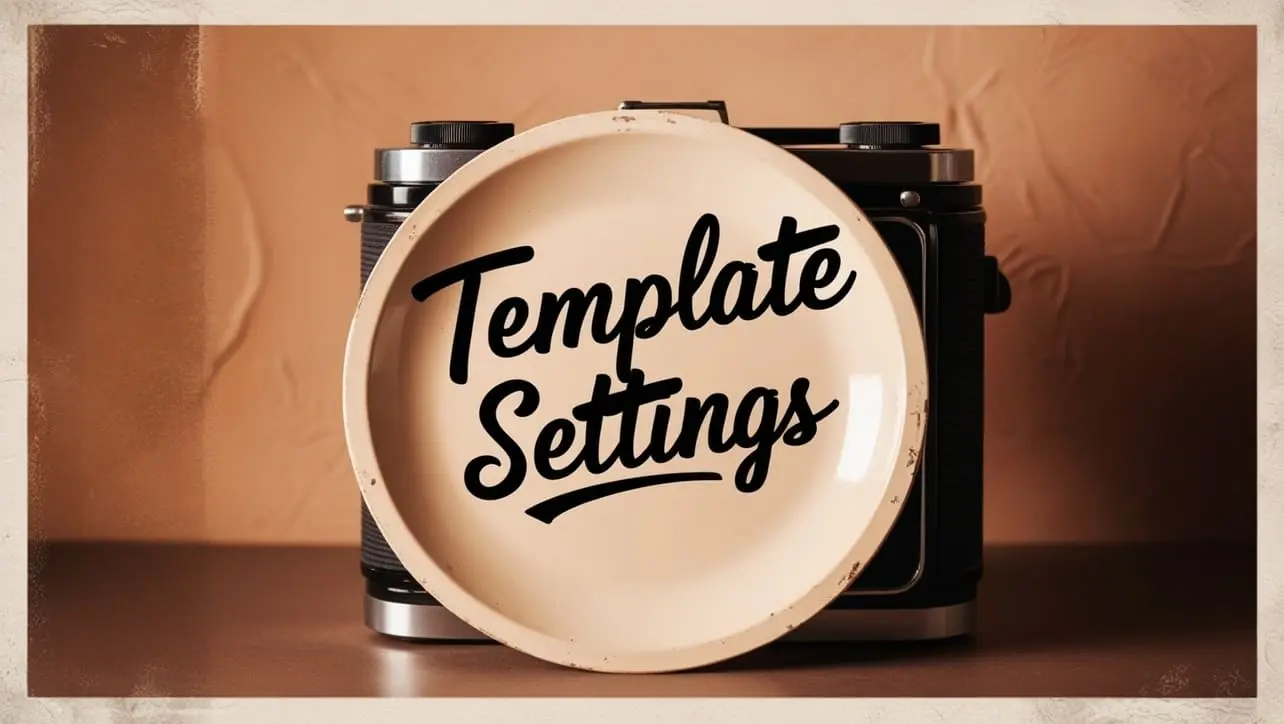
Lodash _.templateSettings Property
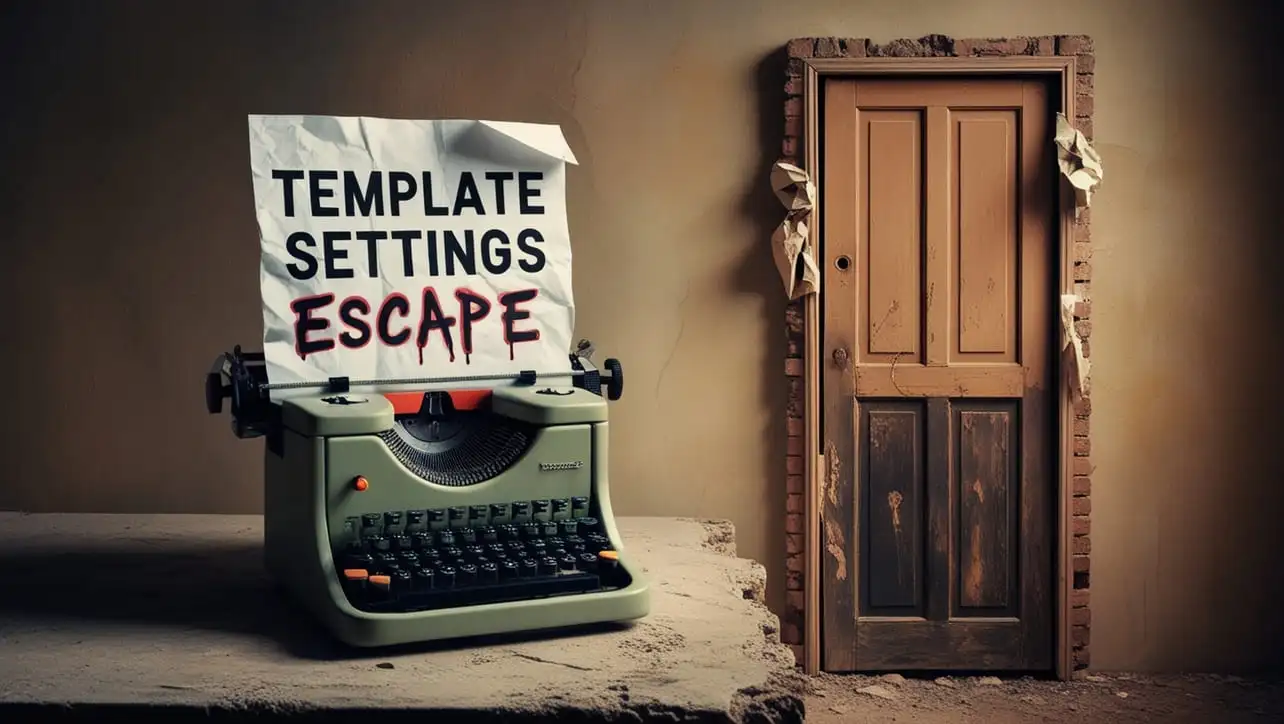
Lodash _.templateSettings.escape Property
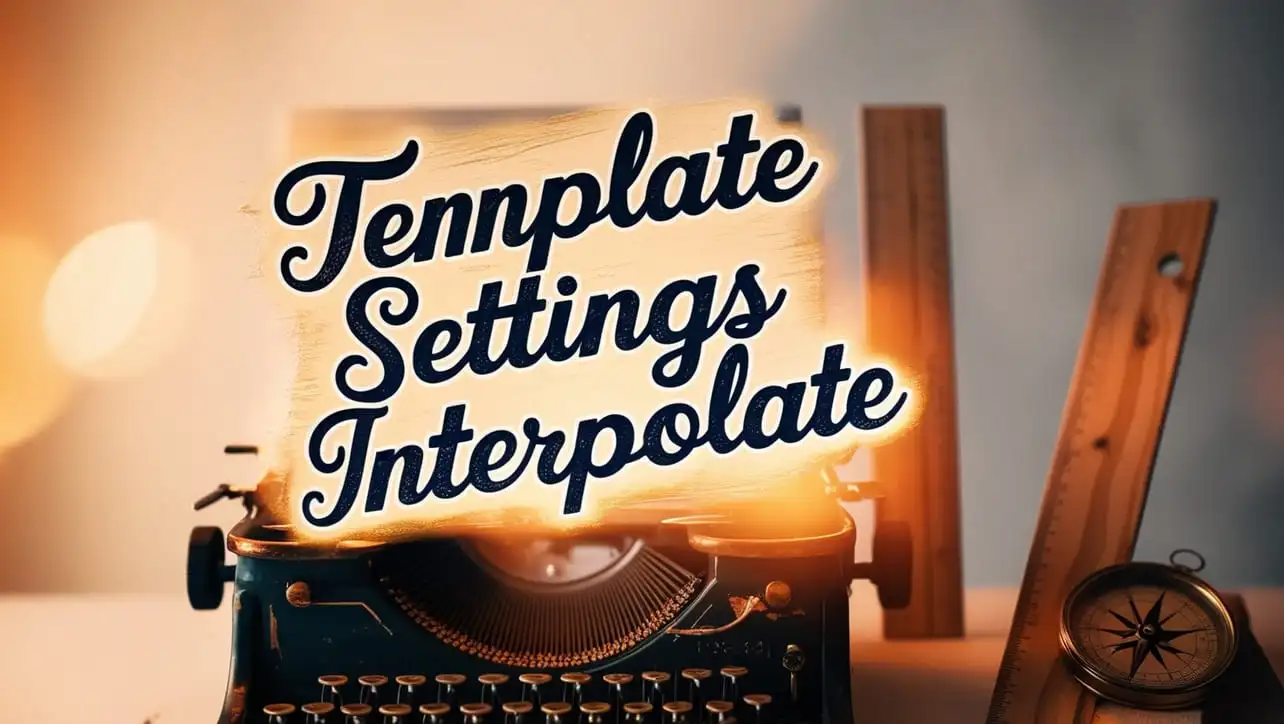
Lodash _.templateSettings.interpolate Property
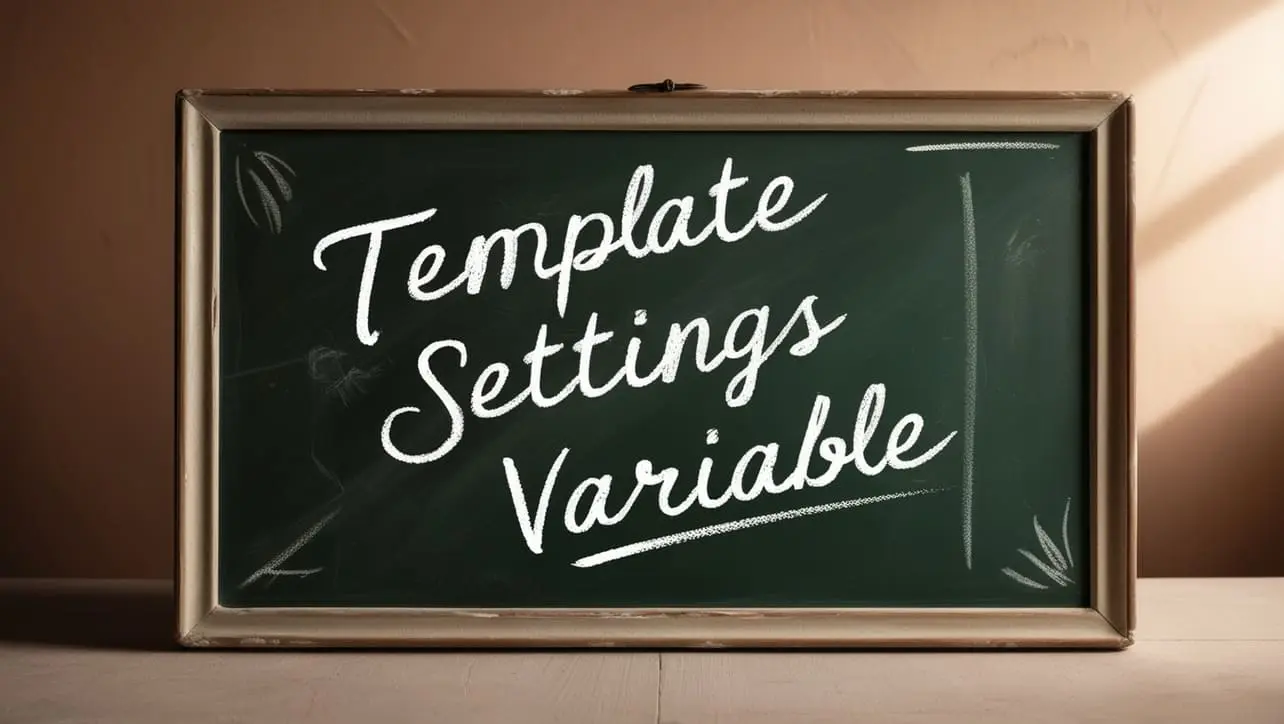
If you have any doubts regarding this article (Lodash _.property() Util Method), please comment here. I will help you immediately.