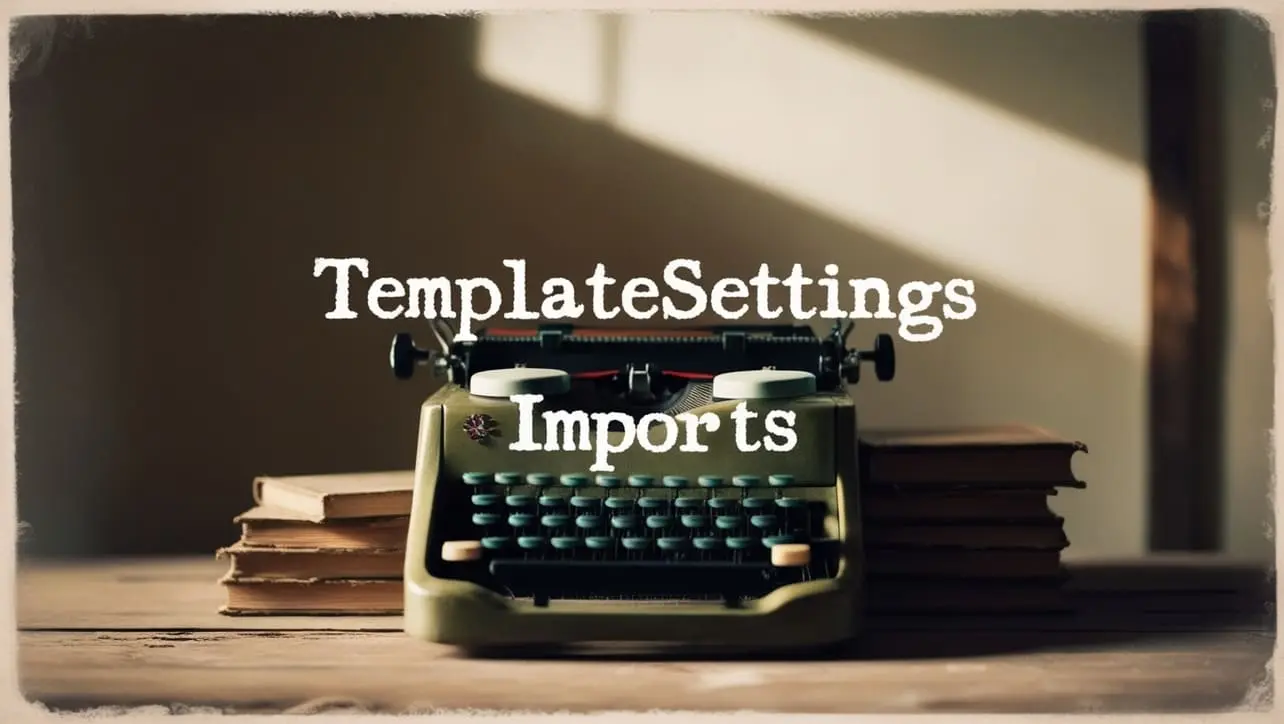
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- _.attempt
- _.bindAll
- _.cond
- _.conforms
- _.constant
- _.defaultTo
- _.flow
- _.flowRight
- _.identity
- _.iteratee
- _.matches
- _.matchesProperty
- _.method
- _.methodOf
- _.mixin
- _.noConflict
- _.noop
- _.nthArg
- _.over
- _.overEvery
- _.overSome
- _.property
- _.propertyOf
- _.range
- _.rangeRight
- _.runInContext
- _.stubArray
- _.stubFalse
- _.stubObject
- _.stubString
- _.stubTrue
- _.times
- _.toPath
- _.uniqueId
- Lodash Properties
- Lodash Methods
Lodash _.defaultTo() Util Method
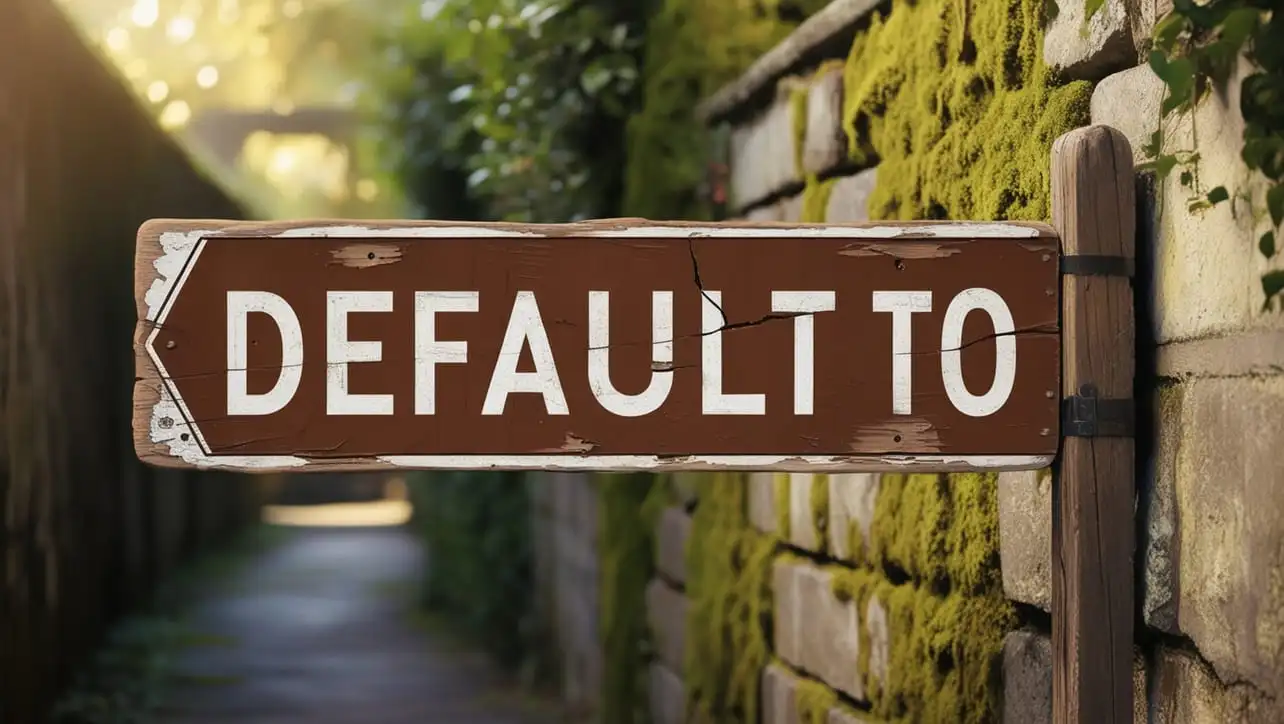
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript development, handling default values for variables or expressions is a common requirement. Lodash, a popular utility library, offers a wide range of functions to simplify JavaScript programming tasks. Among these functions is the _.defaultTo()
method, which provides a convenient way to assign default values to variables when they are null or undefined.
This method streamlines code and enhances readability, making it a valuable asset for developers.
🧠 Understanding _.defaultTo() Method
The _.defaultTo()
method in Lodash is designed to provide a default value for a variable if it is null or undefined. This eliminates the need for verbose null-checking code and simplifies logic, resulting in cleaner and more concise code.
💡 Syntax
The syntax for the _.defaultTo()
method is straightforward:
_.defaultTo(value, defaultValue)
- value: The value to check.
- defaultValue: The default value to use if value is null or undefined.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.defaultTo()
method:
const _ = require('lodash');
const userInput = ''; // Assume this value is obtained from user input
const sanitizedInput = _.defaultTo(userInput, 'No input provided');
console.log(sanitizedInput);
// Output: 'No input provided'
In this example, if userInput is null or undefined, _.defaultTo()
assigns the default value No input provided to sanitizedInput.
🏆 Best Practices
When working with the _.defaultTo()
method, consider the following best practices:
Ensure Clarity in Default Values:
When using
_.defaultTo()
, ensure that the default value provides clear information about the absence of the original value. This enhances code readability and helps maintain code logic.example.jsCopiedconst userRole = ''; // Assume this value is obtained from user data const role = _.defaultTo(userRole, 'guest'); console.log(`Current user role: ${role}`); // Output: 'Current user role: guest'
Avoid Nesting _.defaultTo():
To maintain code clarity and avoid confusion, refrain from nesting multiple
_.defaultTo()
calls. Instead, consider using a single_.defaultTo()
call for each variable where necessary.example.jsCopiedconst userInput = ''; // Assume this value is obtained from user input const defaultInput = 'No input provided'; // Avoid nesting const sanitizedInput = _.defaultTo(_.defaultTo(userInput, null), defaultInput); console.log(sanitizedInput); // Output: 'No input provided' // Prefer using a single _.defaultTo() call const sanitizedInput = _.defaultTo(userInput, defaultInput); console.log(sanitizedInput); // Output: 'No input provided'
Consistency in Default Values:
Maintain consistency in default values across your codebase to ensure predictability and ease of maintenance. Document default values where necessary to facilitate understanding for other developers.
example.jsCopied// Define default values in a centralized location const defaultValues = { role: 'guest', timeout: 5000, }; // Use consistent default values throughout the codebase const userRole = _.defaultTo(user.role, defaultValues.role); const apiTimeout = _.defaultTo(settings.timeout, defaultValues.timeout);
📚 Use Cases
User Input Sanitization:
_.defaultTo()
is commonly used to sanitize user input by providing default values when input fields are left blank or contain invalid data.example.jsCopiedconst userInput = ''; // Assume this value is obtained from user input const sanitizedInput = _.defaultTo(userInput, 'No input provided'); console.log(sanitizedInput); // Output: 'No input provided'
Configuration Settings:
When fetching configuration settings,
_.defaultTo()
ensures that default values are used when specific settings are not defined or are null/undefined.example.jsCopiedconst settings = { timeout: 3000, maxRetries: null, }; const apiTimeout = _.defaultTo(settings.timeout, 5000); const retries = _.defaultTo(settings.maxRetries, 3); console.log(`API Timeout: ${apiTimeout} ms, Max Retries: ${retries}`); // Output: 'API Timeout: 3000 ms, Max Retries: 3'
Data Transformation:
During data transformation operations,
_.defaultTo()
can ensure that default values are assigned to missing or invalid data points, maintaining data integrity.example.jsCopiedconst data = [ { id: 1, name: 'Alice', email: 'alice@example.com' }, { id: 2, name: 'Bob', email: null }, { id: 3, name: null, email: 'charlie@example.com' }, ]; const sanitizedData = data.map(entry => ({ id: entry.id, name: _.defaultTo(entry.name, 'Unknown'), email: _.defaultTo(entry.email, 'No email provided'), })); console.log(sanitizedData);
🎉 Conclusion
The _.defaultTo()
method in Lodash provides a convenient and efficient way to handle default values in JavaScript. By simplifying null-checking logic and ensuring consistency in default value assignment, _.defaultTo()
enhances code clarity and maintainability. Incorporate this method into your JavaScript projects to streamline variable assignment and improve code readability.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.defaultTo()
method in your Lodash projects.
👨💻 Join our Community:
Author
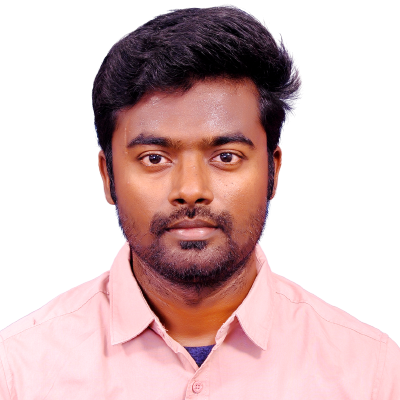
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
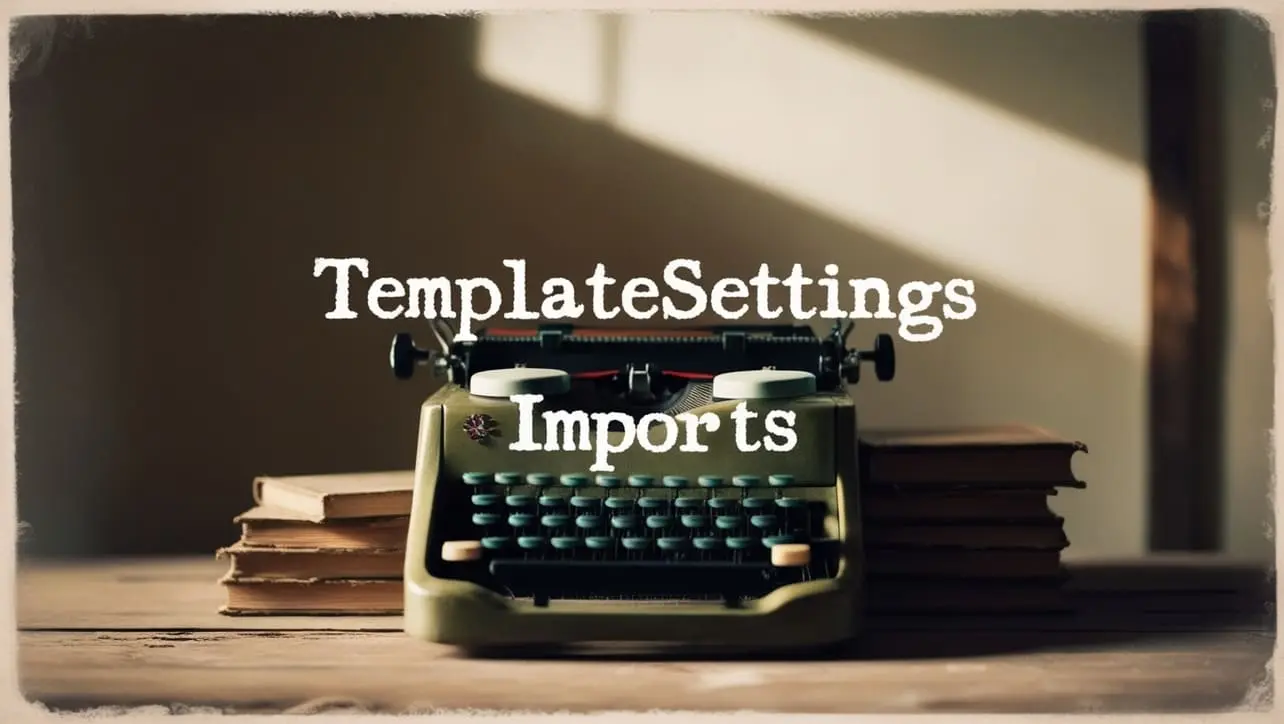
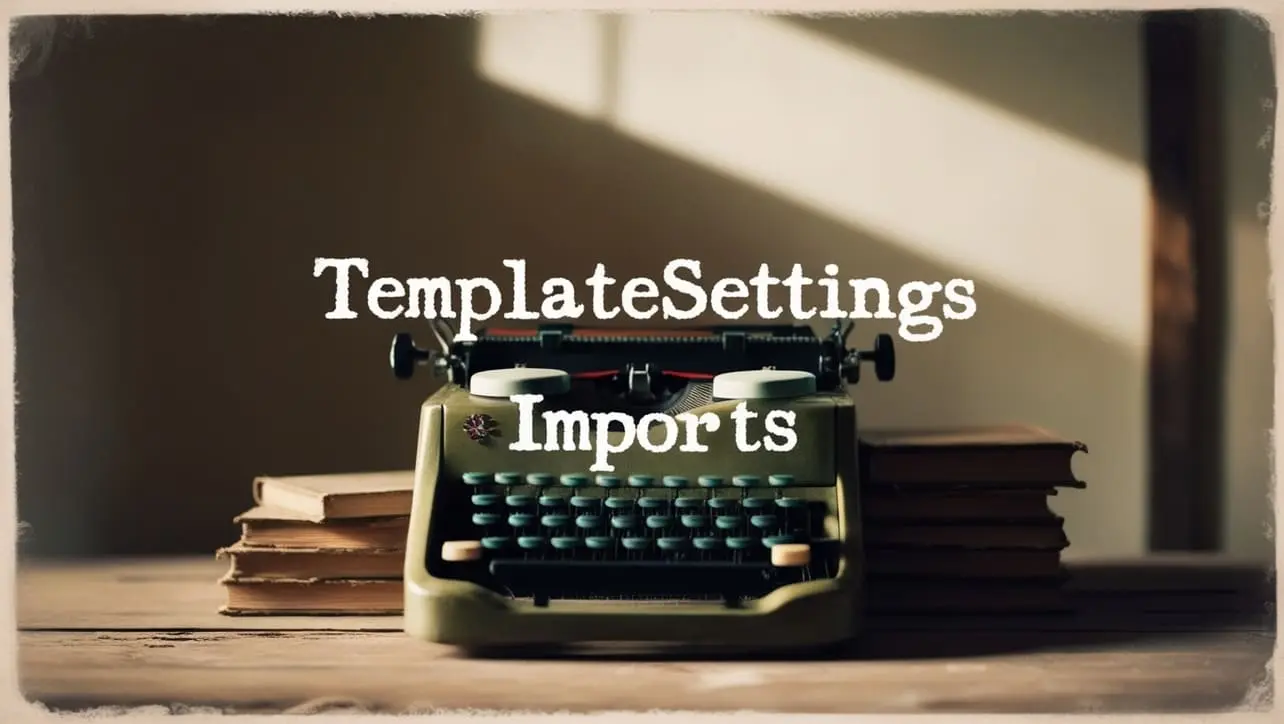
Lodash _.templateSettings.imports Property
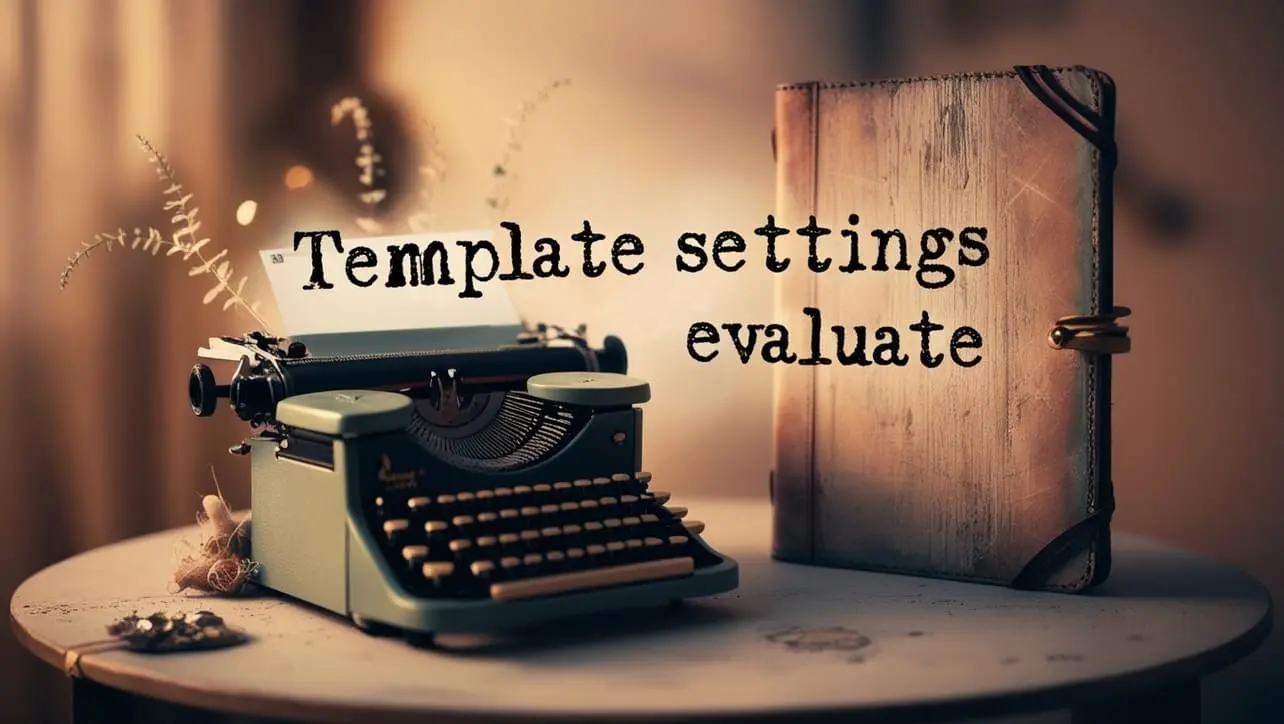
Lodash _.templateSettings.evaluate Property
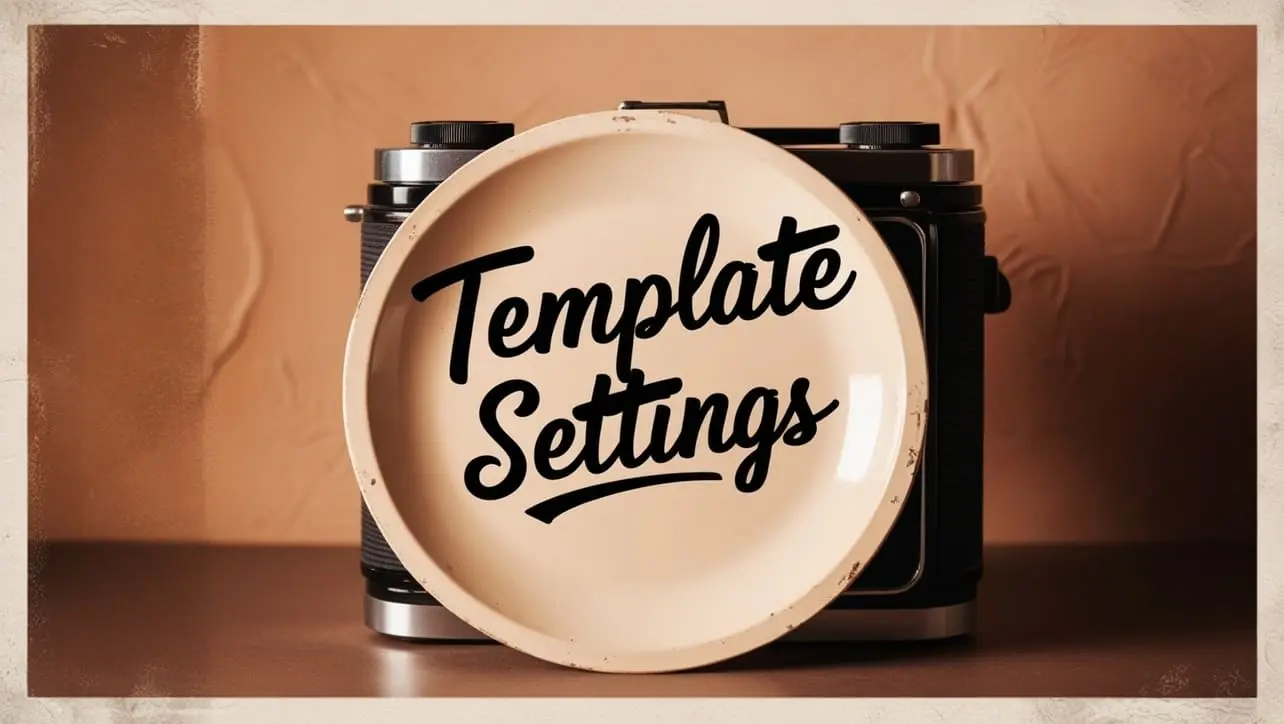
Lodash _.templateSettings Property
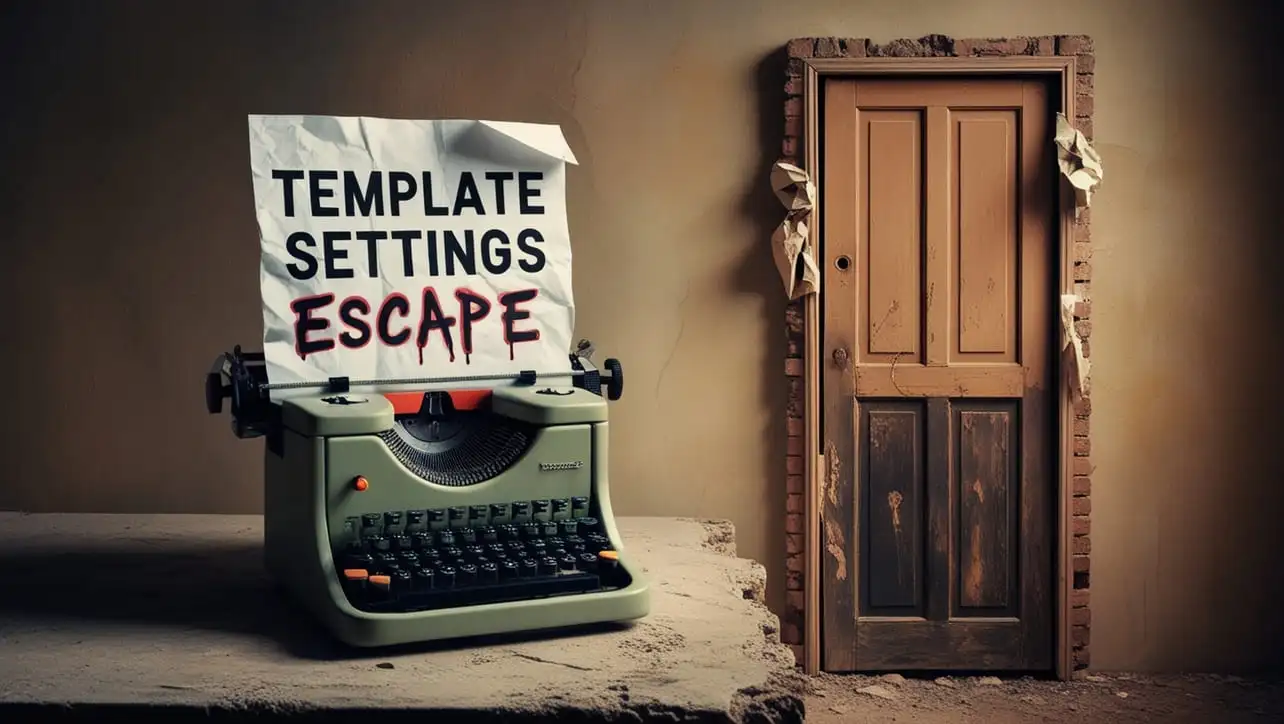
Lodash _.templateSettings.escape Property
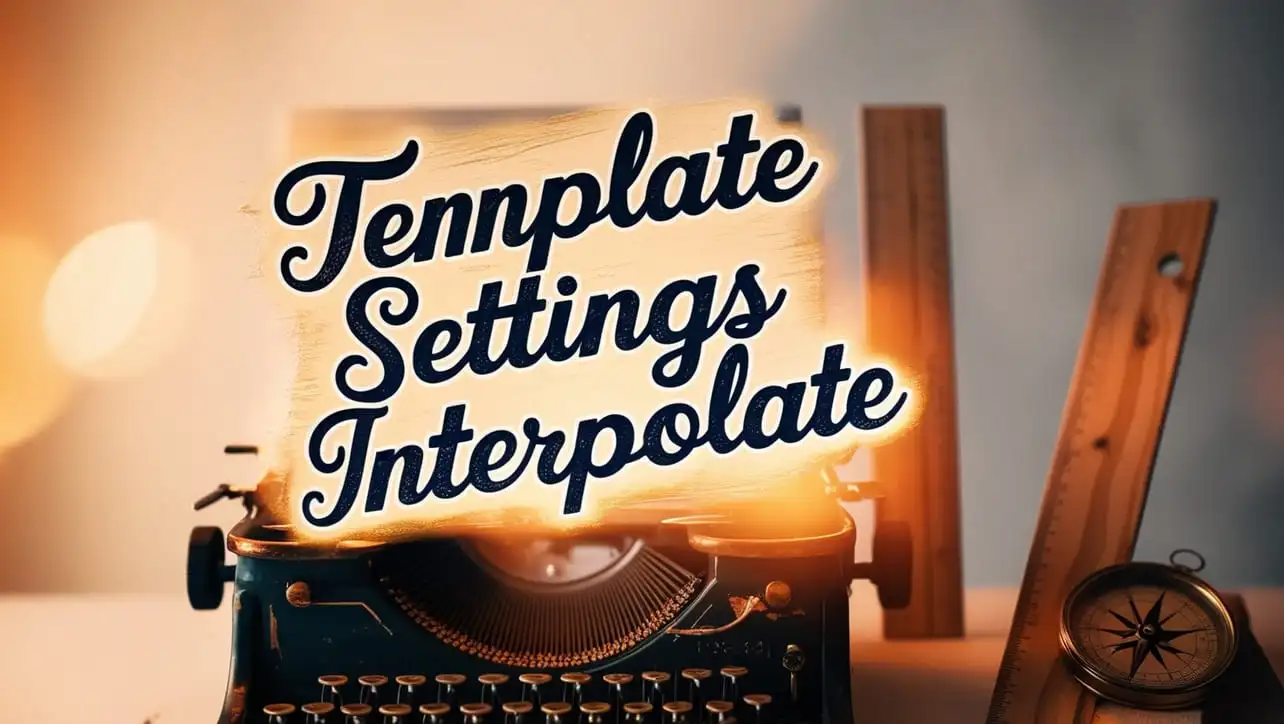
Lodash _.templateSettings.interpolate Property
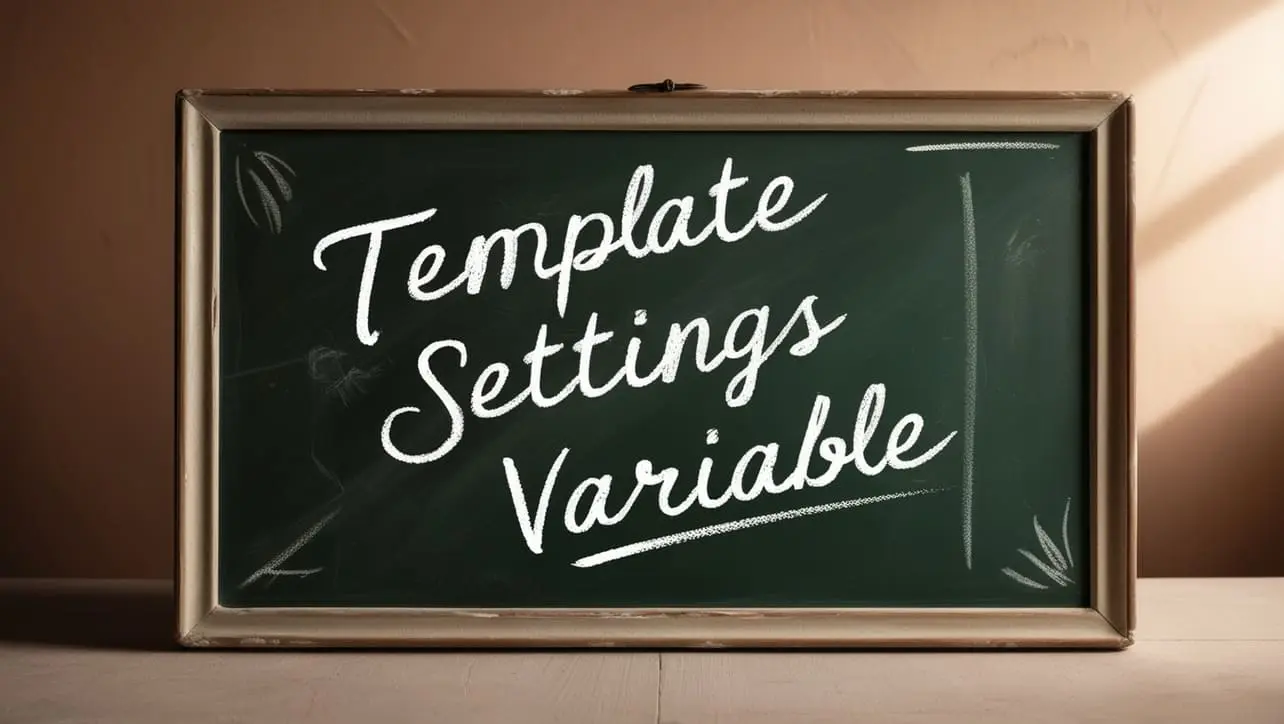
If you have any doubts regarding this article (Lodash _.defaultTo() Util Method), please comment here. I will help you immediately.