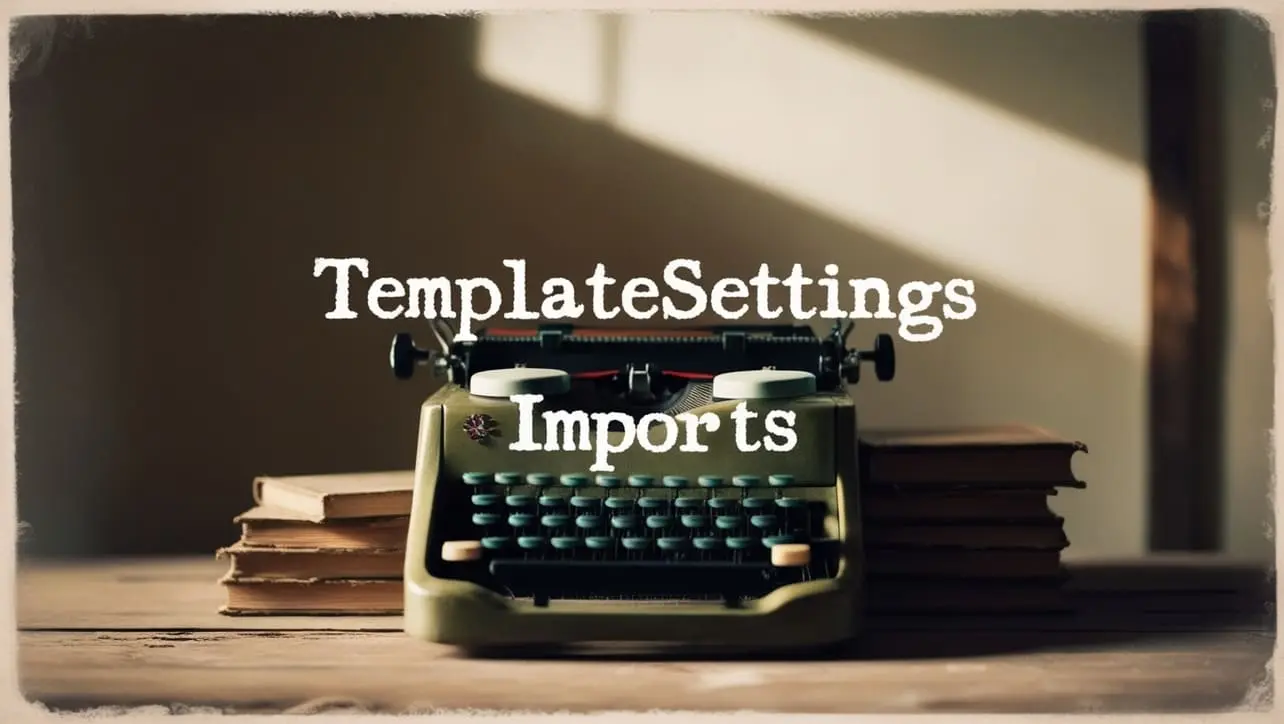
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- _.attempt
- _.bindAll
- _.cond
- _.conforms
- _.constant
- _.defaultTo
- _.flow
- _.flowRight
- _.identity
- _.iteratee
- _.matches
- _.matchesProperty
- _.method
- _.methodOf
- _.mixin
- _.noConflict
- _.noop
- _.nthArg
- _.over
- _.overEvery
- _.overSome
- _.property
- _.propertyOf
- _.range
- _.rangeRight
- _.runInContext
- _.stubArray
- _.stubFalse
- _.stubObject
- _.stubString
- _.stubTrue
- _.times
- _.toPath
- _.uniqueId
- Lodash Properties
- Lodash Methods
Lodash _.cond() Util Method
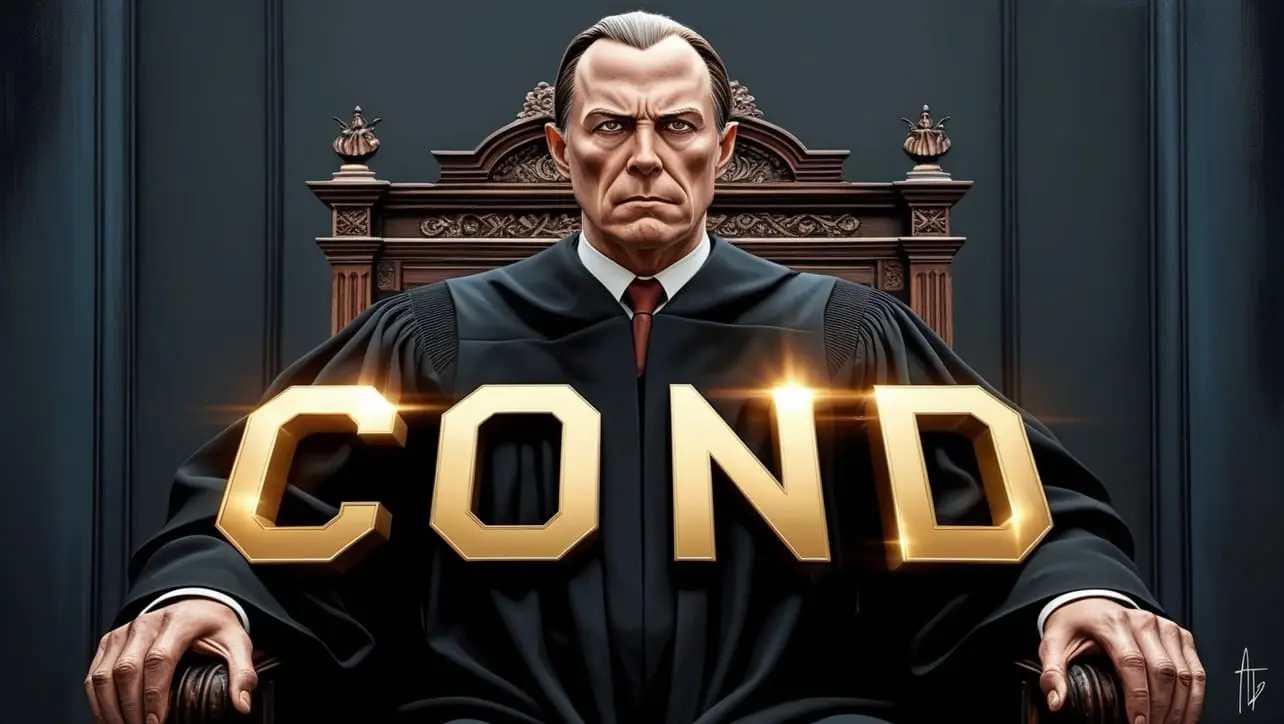
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript development, efficient and concise code is essential. Lodash, a popular utility library, offers a variety of functions to streamline common programming tasks. Among these functions is _.cond()
, a powerful utility method that allows developers to create custom conditional functions in a succinct and expressive manner.
Understanding how to leverage _.cond()
can enchance code readability and maintainability, making it a valuable tool in any JavaScript developer's toolkit.
🧠 Understanding _.cond() Method
The _.cond()
method in Lodash is designed to create a conditional function based on an array of predicate-function pairs. Each pair consists of a predicate function that evaluates a condition and a corresponding function to execute if the condition is met. This enables developers to define complex conditional logic in a clear and concise manner.
💡 Syntax
The syntax for the _.cond()
method is straightforward:
_.cond(pairs)
- pairs: An array of predicate-function pairs.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.cond()
method:
const _ = require('lodash');
// Define predicate-function pairs
const conditions = [
[value => value < 10, value => 'Small'],
[value => value < 20, value => 'Medium'],
[value => value < 30, value => 'Large'],
[_.stubTrue, value => 'Huge']
];
// Create a conditional function
const getSizeCategory = _.cond(conditions);
// Test the conditional function
console.log(getSizeCategory(5)); // Output: 'Small'
console.log(getSizeCategory(15)); // Output: 'Medium'
console.log(getSizeCategory(25)); // Output: 'Large'
console.log(getSizeCategory(35)); // Output: 'Huge'
In this example, getSizeCategory is a conditional function created using _.cond()
. It evaluates the input value against the defined predicate-function pairs and returns the corresponding result.
🏆 Best Practices
When working with the _.cond()
method, consider the following best practices:
Organize Predicate-Function Pairs:
Organize predicate-function pairs in a logical order to ensure that conditions are evaluated correctly. Place more specific conditions before general ones to prevent unintended matches.
example.jsCopiedconst conditions = [ [value => value < 10, value => 'Small'], [value => value < 20, value => 'Medium'], [value => value < 30, value => 'Large'], [_.stubTrue, value => 'Huge'] // Default condition should be the last one ];
Use Predicate Functions Wisely:
Use predicate functions to encapsulate complex conditions and logic, promoting code reusability and readability. Break down complex conditions into smaller, more manageable functions for better maintainability.
example.jsCopiedconst isEven = value => value % 2 === 0; const isPositive = value => value > 0; const conditions = [ [isEven, value => 'Even'], [isPositive, value => 'Positive'], [_.stubTrue, value => 'Other'] ];
Handle Edge Cases:
Consider edge cases and handle them appropriately within predicate functions. Ensure that the conditional function behaves as expected for all possible input values.
example.jsCopiedconst conditions = [ [_.isNil, value => 'Undefined or Null'], [_.isNaN, value => 'Not a Number'], [_.stubTrue, value => 'Other'] ];
📚 Use Cases
Dynamic Function Execution:
_.cond()
is ideal for scenarios where you need to execute different functions based on varying conditions. This flexibility enables dynamic behavior in your applications.example.jsCopiedconst conditions = [ [value => value === 'foo', () => console.log('Function 1')], [value => value === 'bar', () => console.log('Function 2')], [_.stubTrue, () => console.log('Default Function')] ]; const executeFunction = _.cond(conditions); executeFunction('foo'); // Output: 'Function 1' executeFunction('bar'); // Output: 'Function 2' executeFunction('baz'); // Output: 'Default Function'
Data Transformation:
_.cond()
can be used for transforming data based on specified conditions. This is particularly useful for categorizing or processing data dynamically.example.jsCopiedconst temperatureConditions = [ [temp => temp < 0, () => 'Freezing'], [temp => temp < 10, () => 'Cold'], [temp => temp < 25, () => 'Moderate'], [_.stubTrue, () => 'Hot'] ]; const getTemperatureCategory = _.cond(temperatureConditions); console.log(getTemperatureCategory(5)); // Output: 'Cold' console.log(getTemperatureCategory(20)); // Output: 'Moderate' console.log(getTemperatureCategory(30)); // Output: 'Hot'
Error Handling:
_.cond()
can facilitate error handling by executing different error-handling functions based on specific error conditions. This promotes modularity and maintainability in error-handling logic.example.jsCopiedconst errorConditions = [ [error => error.code === 404, () => console.error('Resource Not Found')], [error => error.code === 500, () => console.error('Internal Server Error')], [_.stubTrue, () => console.error('Unknown Error')] ]; const handleServerError = _.cond(errorConditions); // Simulate an error object const error = { code: 404, message: 'Resource not found' }; handleServerError(error); // Output: 'Resource Not Found'
🎉 Conclusion
The _.cond()
method in Lodash empowers developers to create custom conditional functions with ease, enabling dynamic behavior and promoting code readability. By leveraging _.cond()
, you can streamline complex conditional logic and enhance the maintainability of your JavaScript applications.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.cond()
method in your Lodash projects.
👨💻 Join our Community:
Author
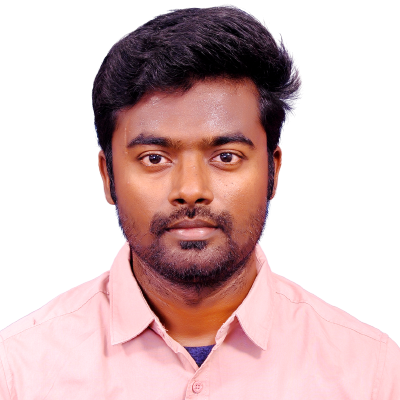
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
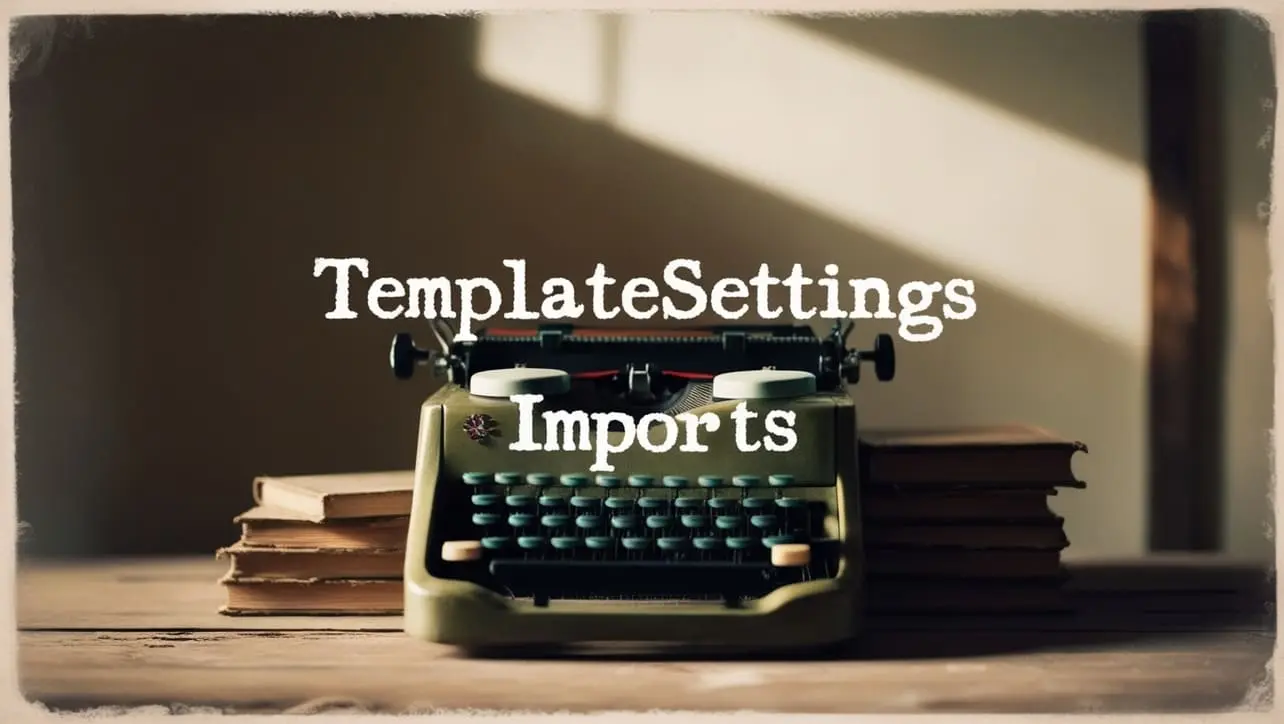
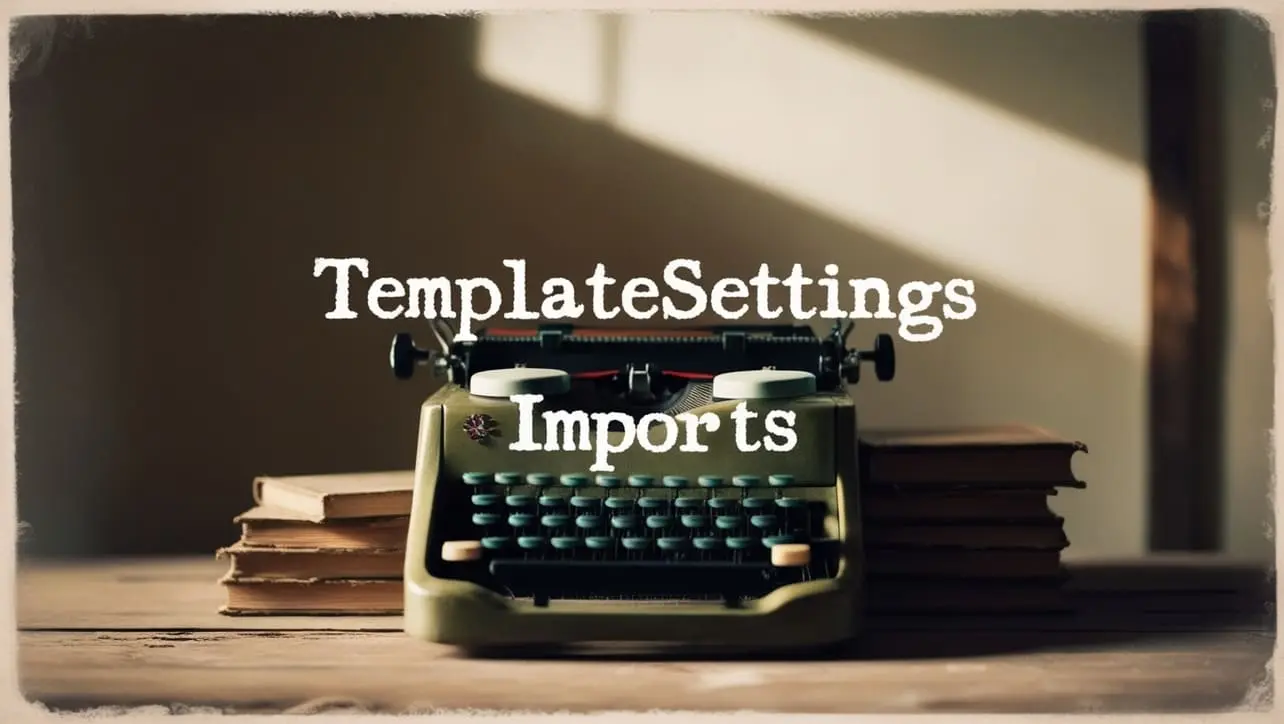
Lodash _.templateSettings.imports Property
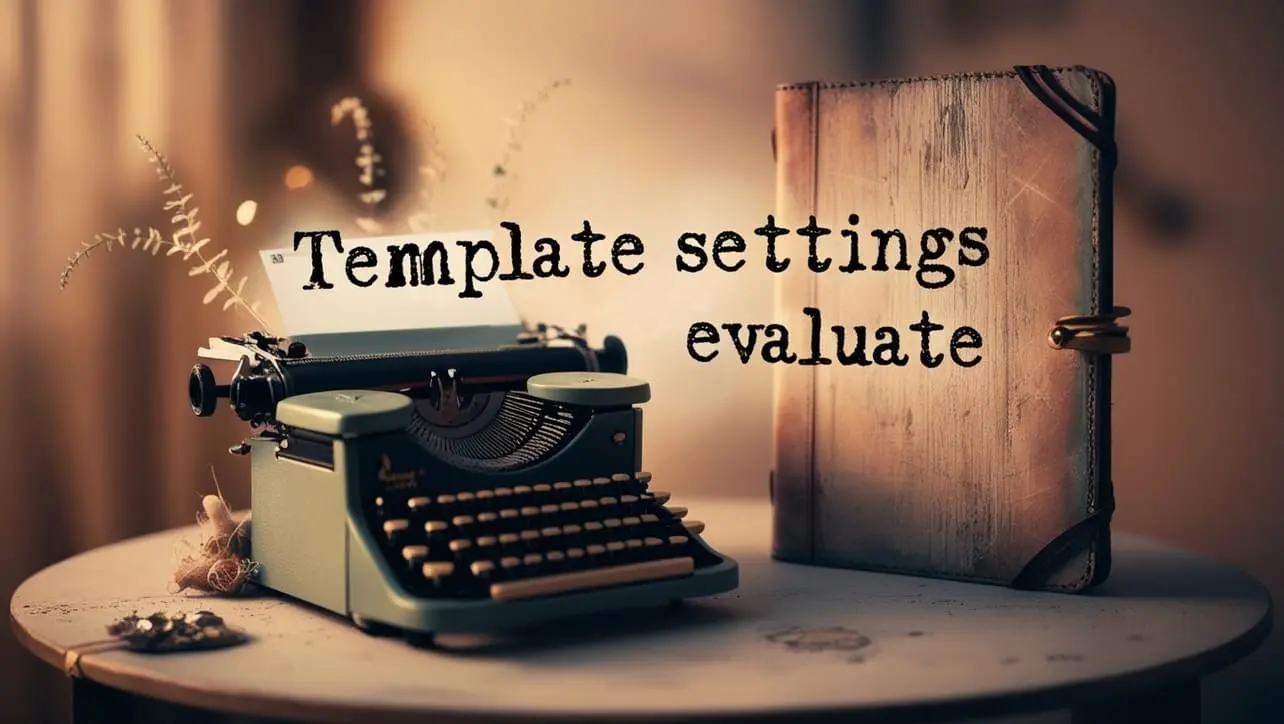
Lodash _.templateSettings.evaluate Property
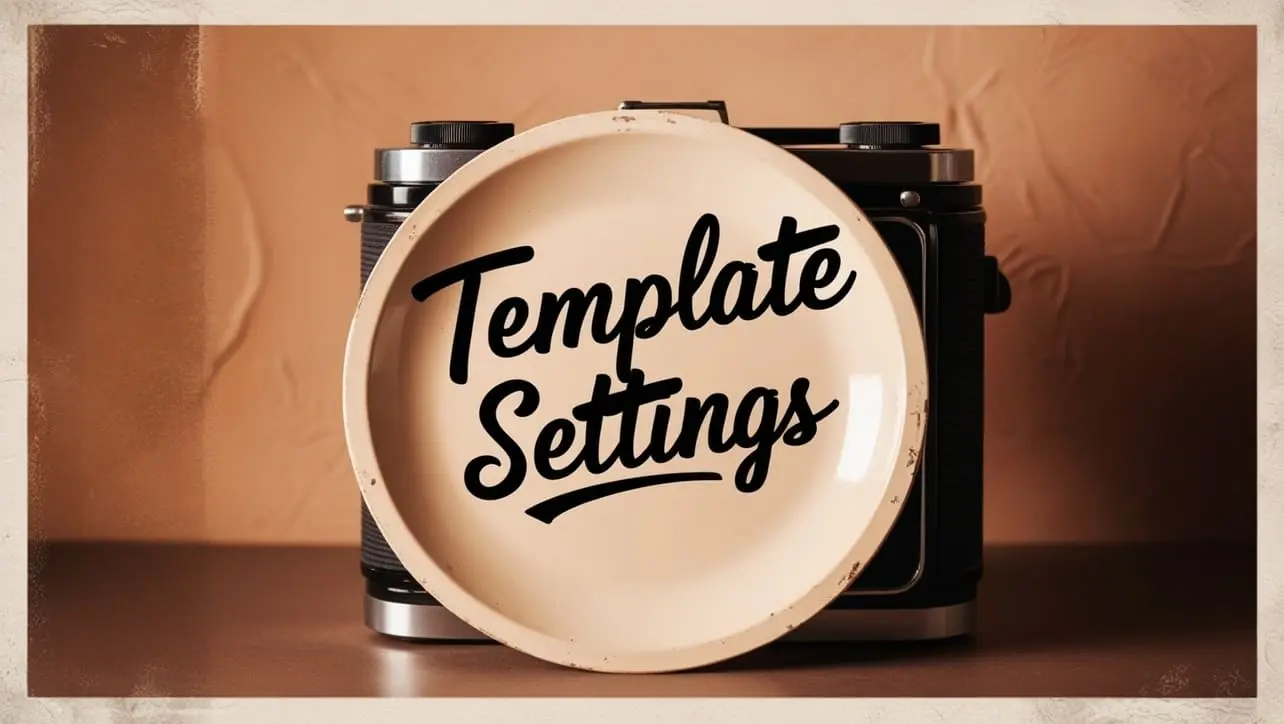
Lodash _.templateSettings Property
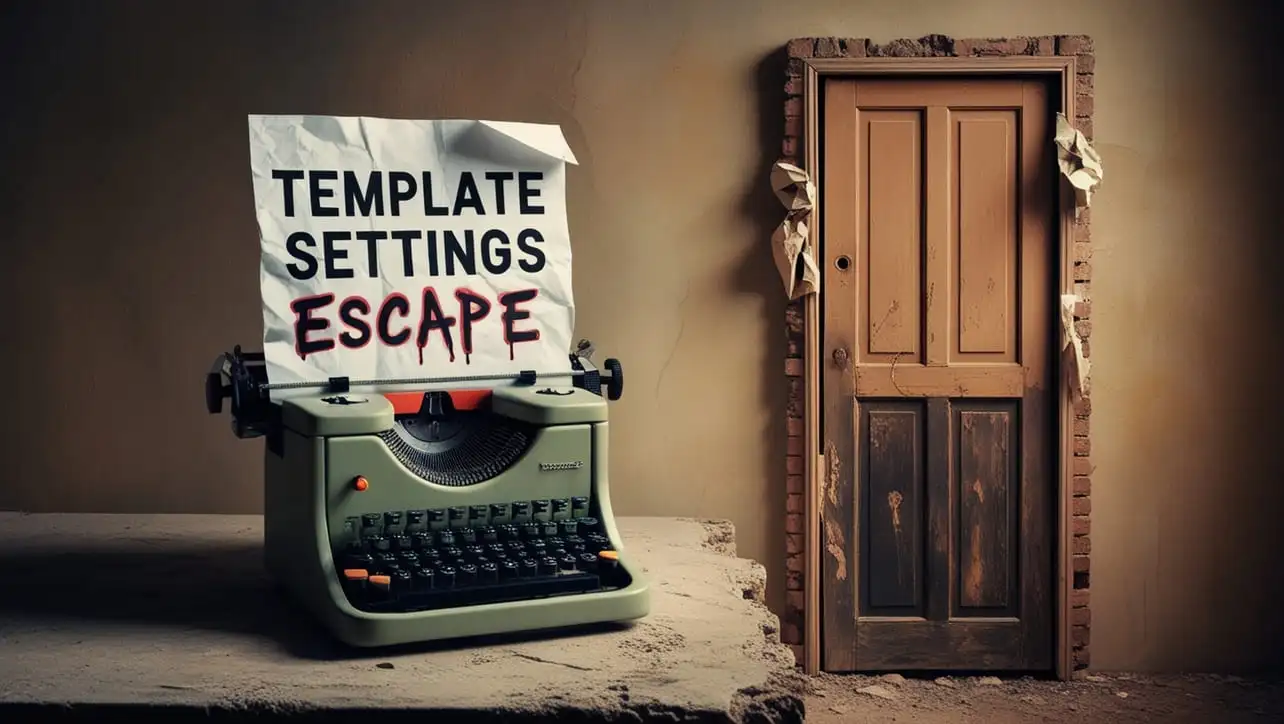
Lodash _.templateSettings.escape Property
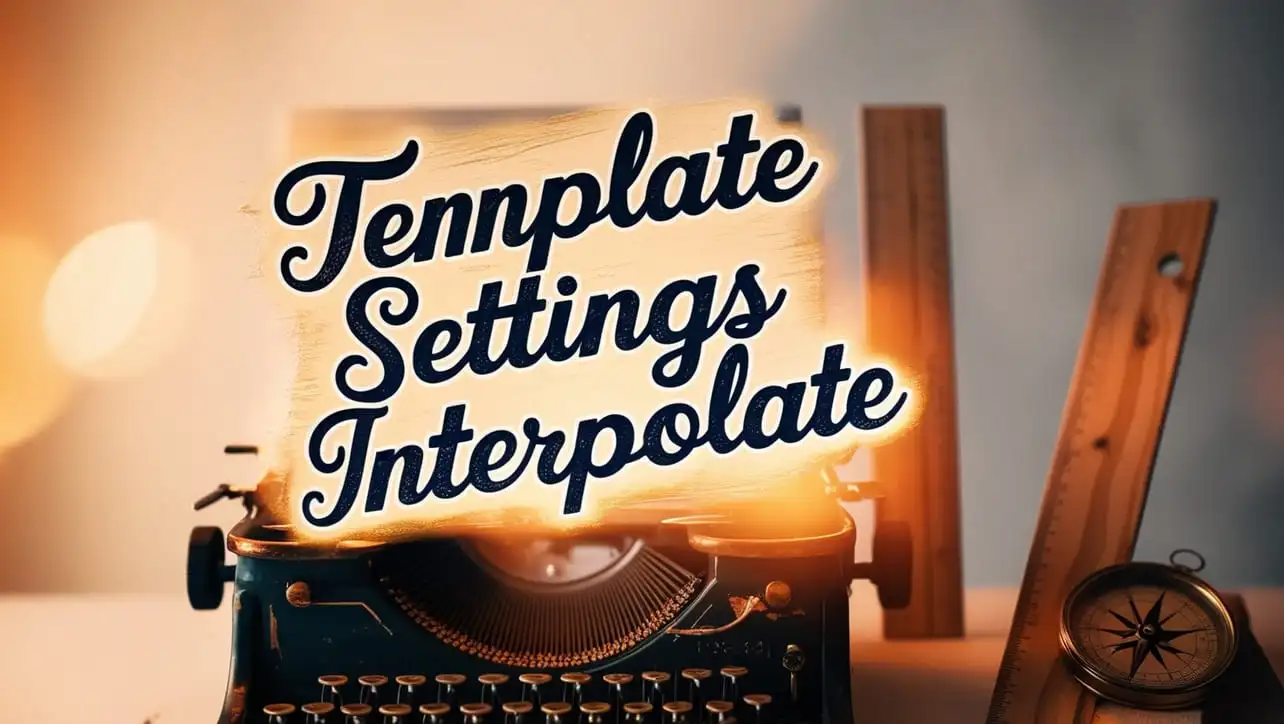
Lodash _.templateSettings.interpolate Property
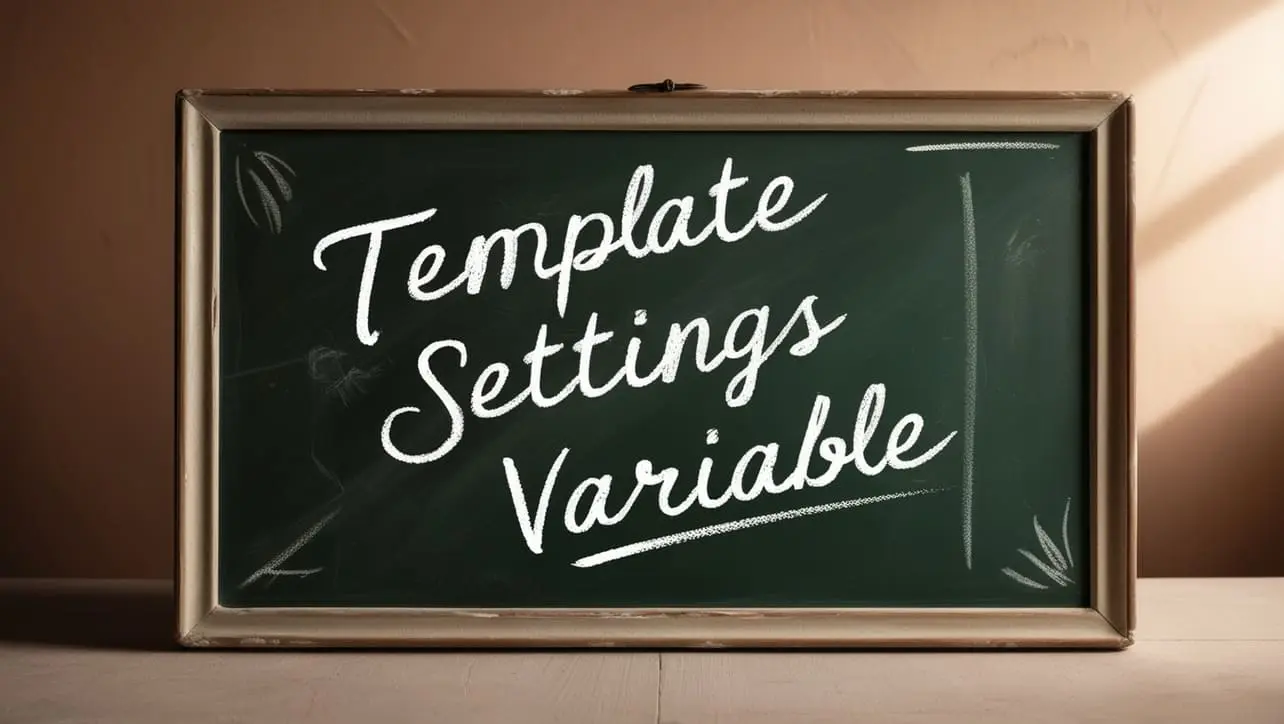
If you have any doubts regarding this article (Lodash _.cond() Util Method), please comment here. I will help you immediately.