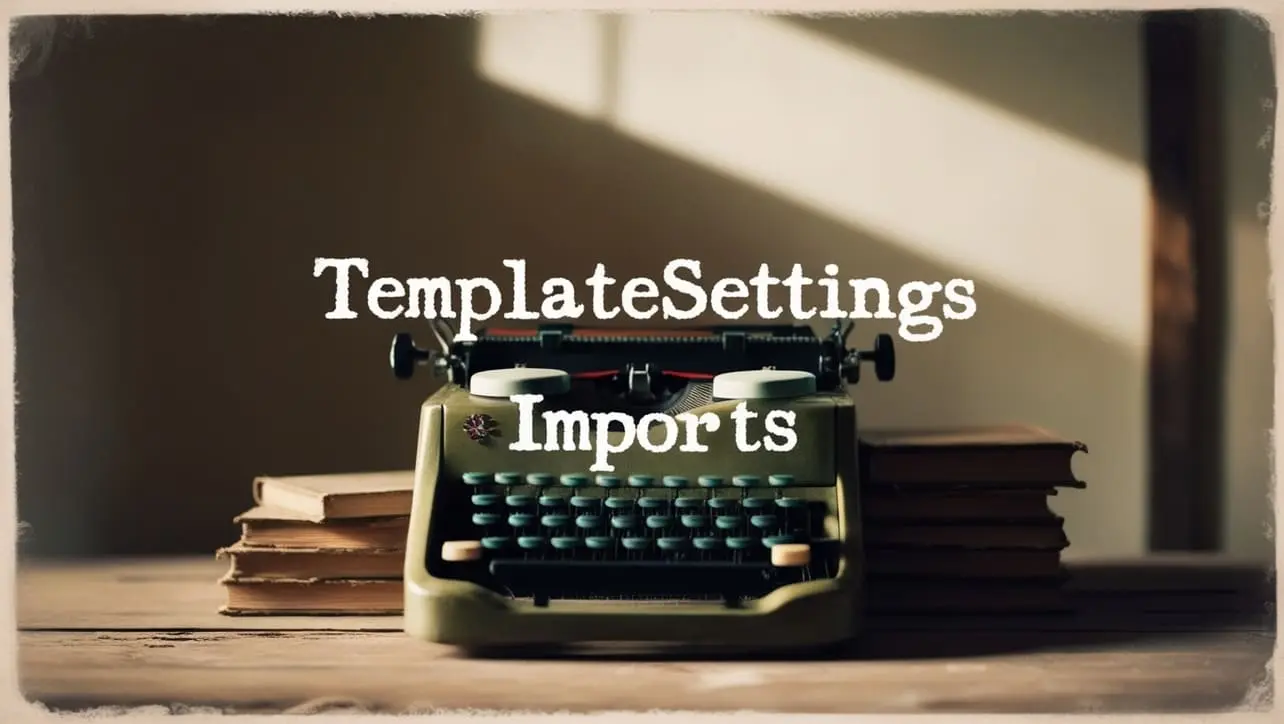
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- _.attempt
- _.bindAll
- _.cond
- _.conforms
- _.constant
- _.defaultTo
- _.flow
- _.flowRight
- _.identity
- _.iteratee
- _.matches
- _.matchesProperty
- _.method
- _.methodOf
- _.mixin
- _.noConflict
- _.noop
- _.nthArg
- _.over
- _.overEvery
- _.overSome
- _.property
- _.propertyOf
- _.range
- _.rangeRight
- _.runInContext
- _.stubArray
- _.stubFalse
- _.stubObject
- _.stubString
- _.stubTrue
- _.times
- _.toPath
- _.uniqueId
- Lodash Properties
- Lodash Methods
Lodash _.attempt() Util Method
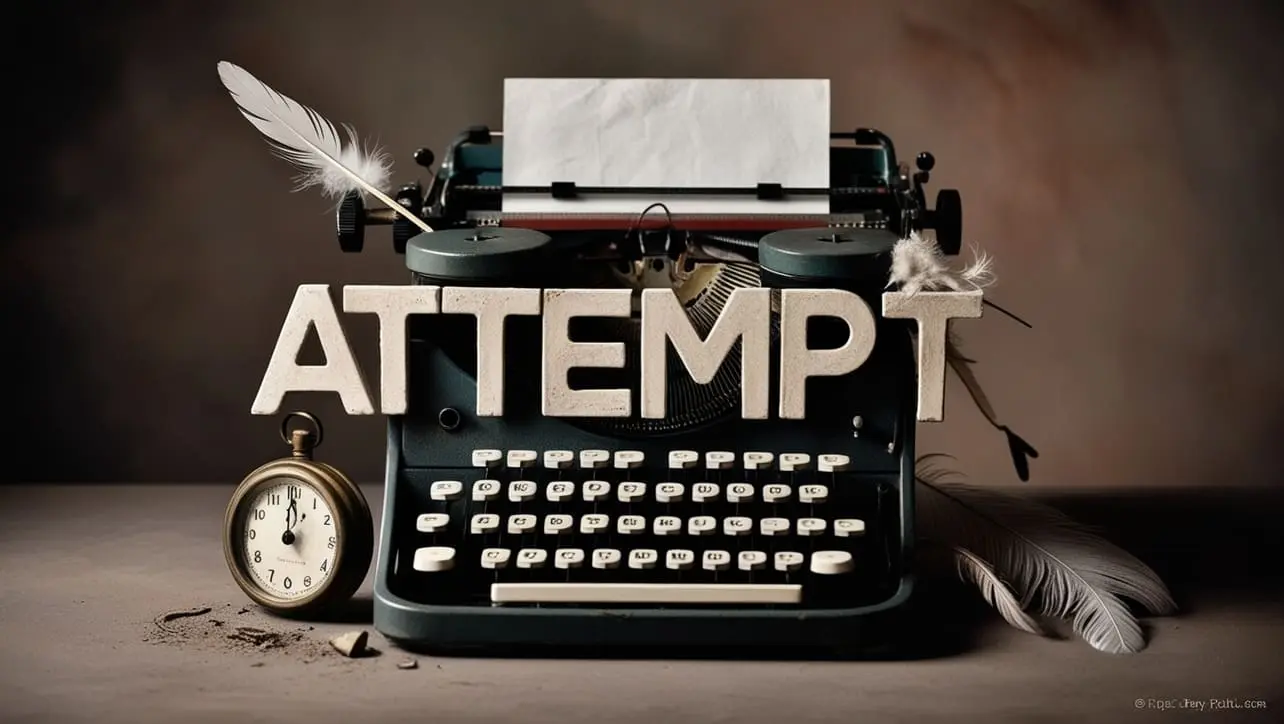
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript development, error handling is a critical aspect of writing robust and reliable code. Lodash, a popular utility library, provides a variety of functions to simplify common programming tasks. Among these functions is _.attempt()
, a powerful utility method designed to execute a function safely, catching any errors that may occur during execution.
Understanding and leveraging _.attempt()
can greatly enhance the resilience and stability of your codebase.
🧠 Understanding _.attempt() Method
The _.attempt()
method in Lodash is used to invoke a provided function while handling any potential errors gracefully. If the function executes successfully, _.attempt()
returns the result of the function. However, if an error occurs during execution, _.attempt()
returns the error object, allowing you to handle it appropriately without causing your application to crash.
💡 Syntax
The syntax for the _.attempt()
method is straightforward:
_.attempt(func, [args])
- func: The function to attempt to execute.
- args (Optional): Arguments to pass to the function.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.attempt()
method:
const _ = require('lodash');
// Define a function that may throw an error
function riskyOperation() {
throw new Error('Something went wrong');
}
// Attempt to execute the function safely
const result = _.attempt(riskyOperation);
if(_.isError(result)) {
console.error('An error occurred:', result.message);
} else {
console.log('Operation result:', result);
}
In this example, riskyOperation() is invoked using _.attempt()
. If the operation throws an error, _.attempt()
catches it and returns the error object, allowing you to handle it gracefully.
🏆 Best Practices
When working with the _.attempt()
method, consider the following best practices:
Handle Errors Gracefully:
Use
_.attempt()
to execute potentially risky operations and handle any resulting errors gracefully. This prevents your application from crashing and allows you to provide meaningful error messages to users.example.jsCopiedconst result = _.attempt(somePotentiallyRiskyOperation); if(_.isError(result)) { console.error('An error occurred:', result.message); } else { console.log('Operation result:', result); }
Use with Functional Programming:
_.attempt()
is particularly useful in functional programming paradigms, where error handling can be challenging. By encapsulating potentially error-prone operations within_.attempt()
, you can maintain the purity of your functional code.example.jsCopiedconst results = _.map(data, item => _.attempt(() => someOperation(item))); _.forEach(results, result => { if(_.isError(result)) { console.error('An error occurred:', result.message); } else { console.log('Operation result:', result); } });
Test Error Scenarios:
When using
_.attempt()
, ensure that you thoroughly test error scenarios to verify that your error handling logic behaves as expected. Mocking error-prone functions during testing can help simulate real-world conditions and identify potential issues early.example.jsCopied// Mocking error-prone function for testing const mockOperation = () => { throw new Error('Mocked error'); }; const result = _.attempt(mockOperation); if(_.isError(result)) { console.log('Test passed: Error handled correctly'); } else { console.error('Test failed: Expected error not caught'); }
📚 Use Cases
External API Calls:
When making external API calls, use
_.attempt()
to handle network errors or unexpected responses gracefully. This ensures that your application remains stable even in the face of unreliable network conditions.example.jsCopiedconst response = _.attempt(() => fetch('https://api.example.com/data')); if(_.isError(response)) { console.error('Failed to fetch data:', response.message); } else { console.log('Data fetched successfully:', response); }
File System Operations:
When performing file system operations, such as reading or writing files,
_.attempt()
can be used to handle file-related errors, such as file not found or permission denied errors.example.jsCopiedconst fileContent = _.attempt(() => fs.readFileSync('example.txt', 'utf8')); if(_.isError(fileContent)) { console.error('Failed to read file:', fileContent.message); } else { console.log('File content:', fileContent); }
Data Transformation:
During data transformation processes,
_.attempt()
can be used to safely execute transformation functions, ensuring that errors in individual transformations do not disrupt the entire process.example.jsCopiedconst transformedData = _.attempt(() => data.map(transformFunction)); if(_.isError(transformedData)) { console.error('Failed to transform data:', transformedData.message); } else { console.log('Data transformed successfully:', transformedData); }
🎉 Conclusion
The _.attempt()
method in Lodash is a valuable tool for executing potentially error-prone operations safely and handling any resulting errors gracefully. By leveraging _.attempt()
, you can enhance the resilience and stability of your JavaScript applications, ensuring a smooth user experience even in the face of unexpected errors.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.attempt()
method in your Lodash projects.
👨💻 Join our Community:
Author
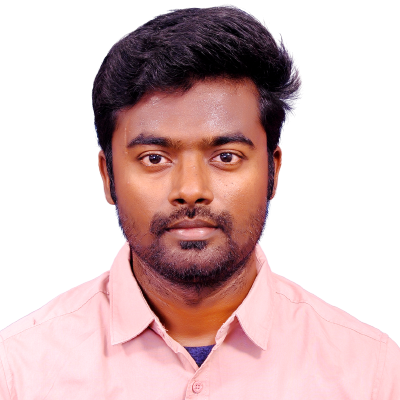
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
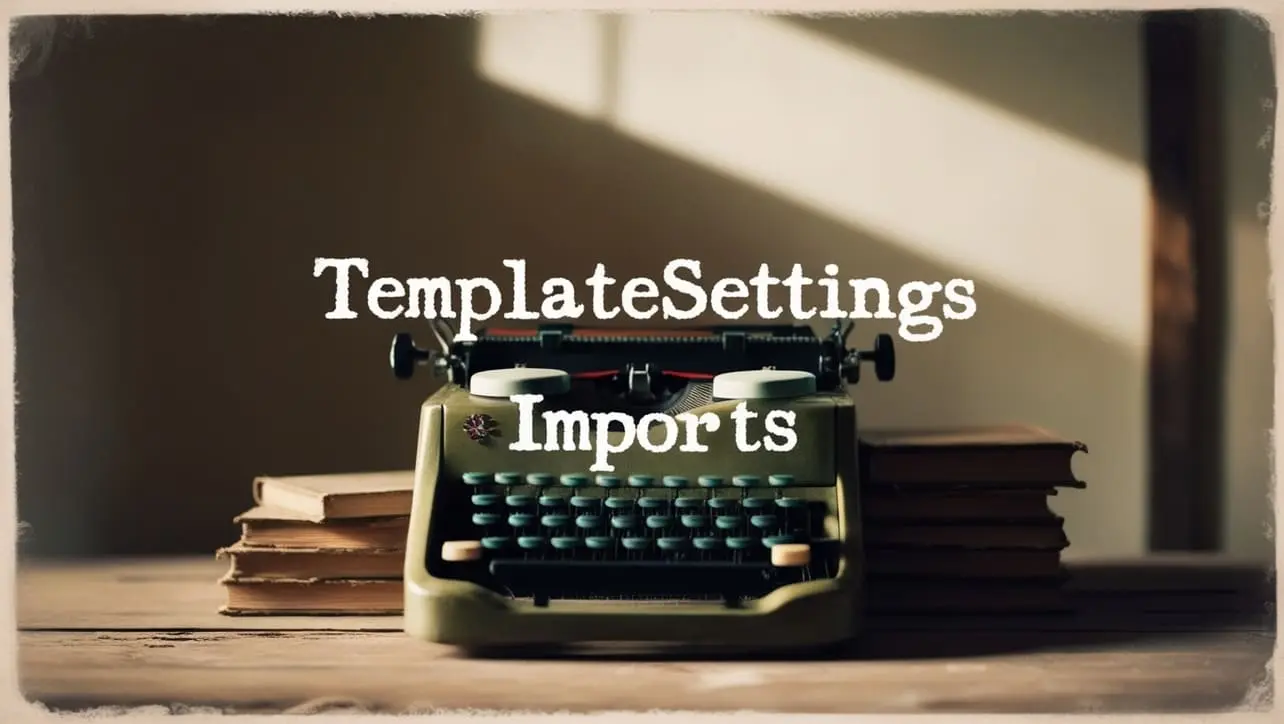
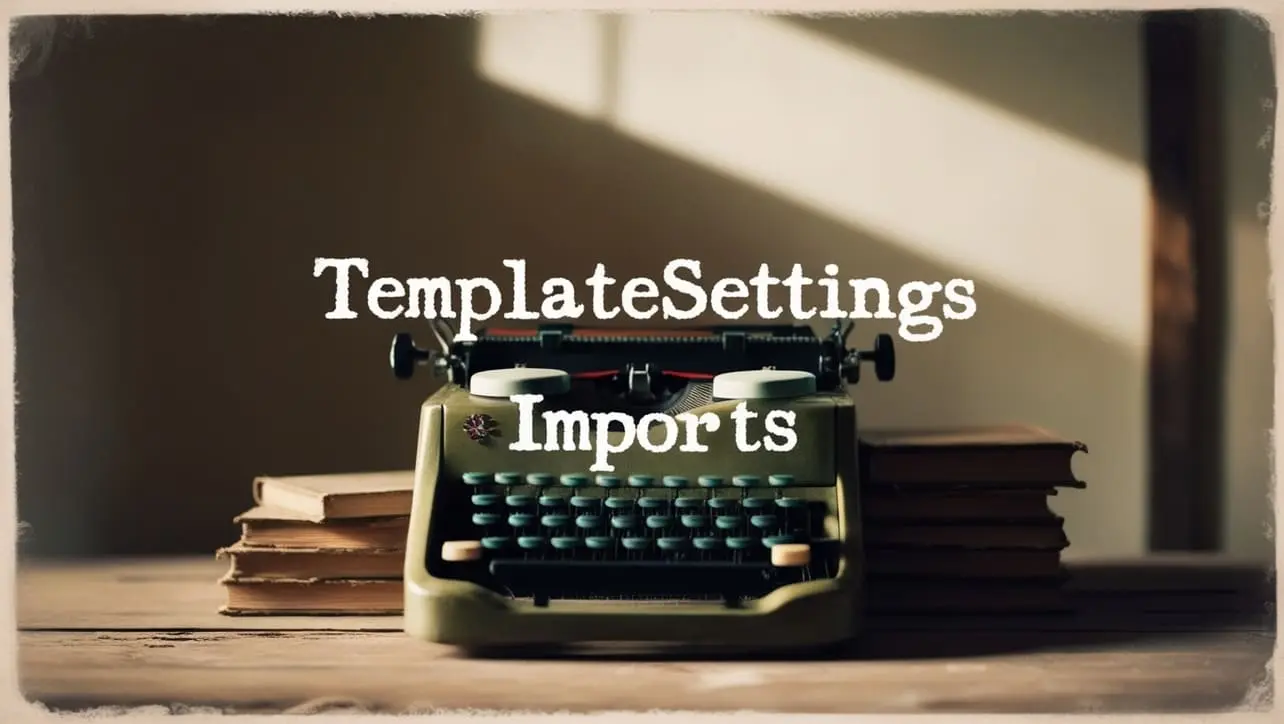
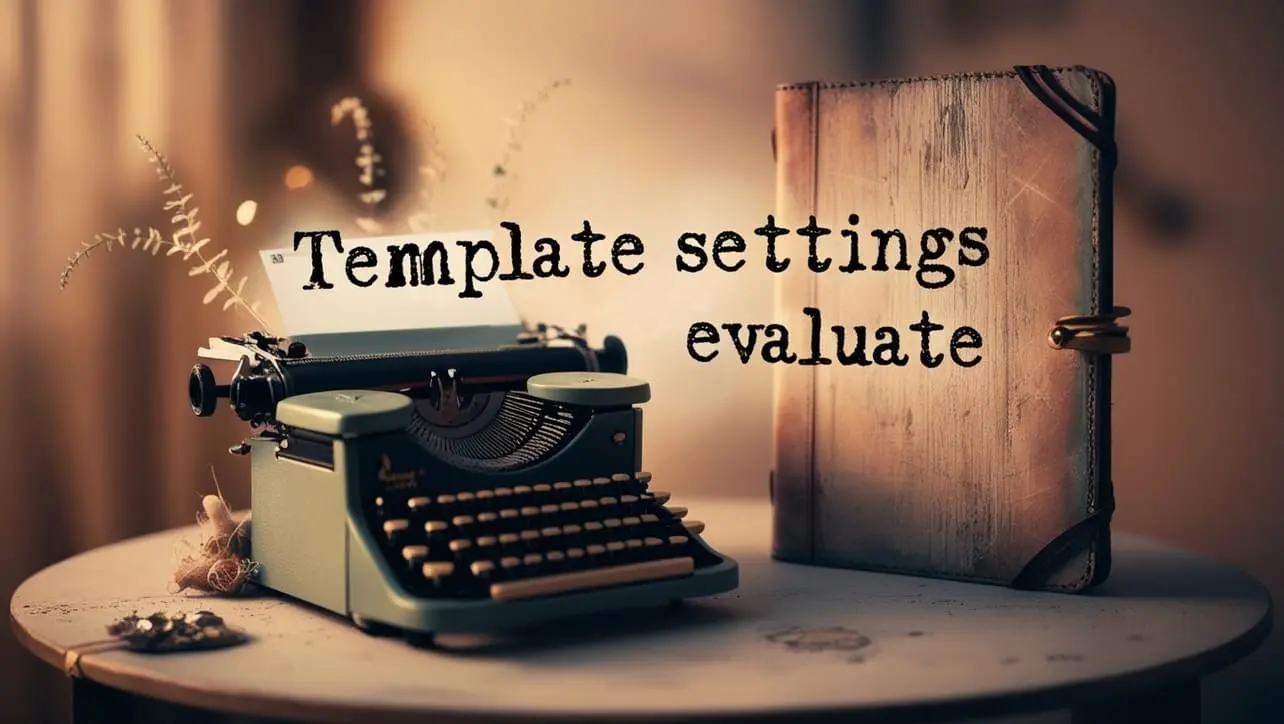
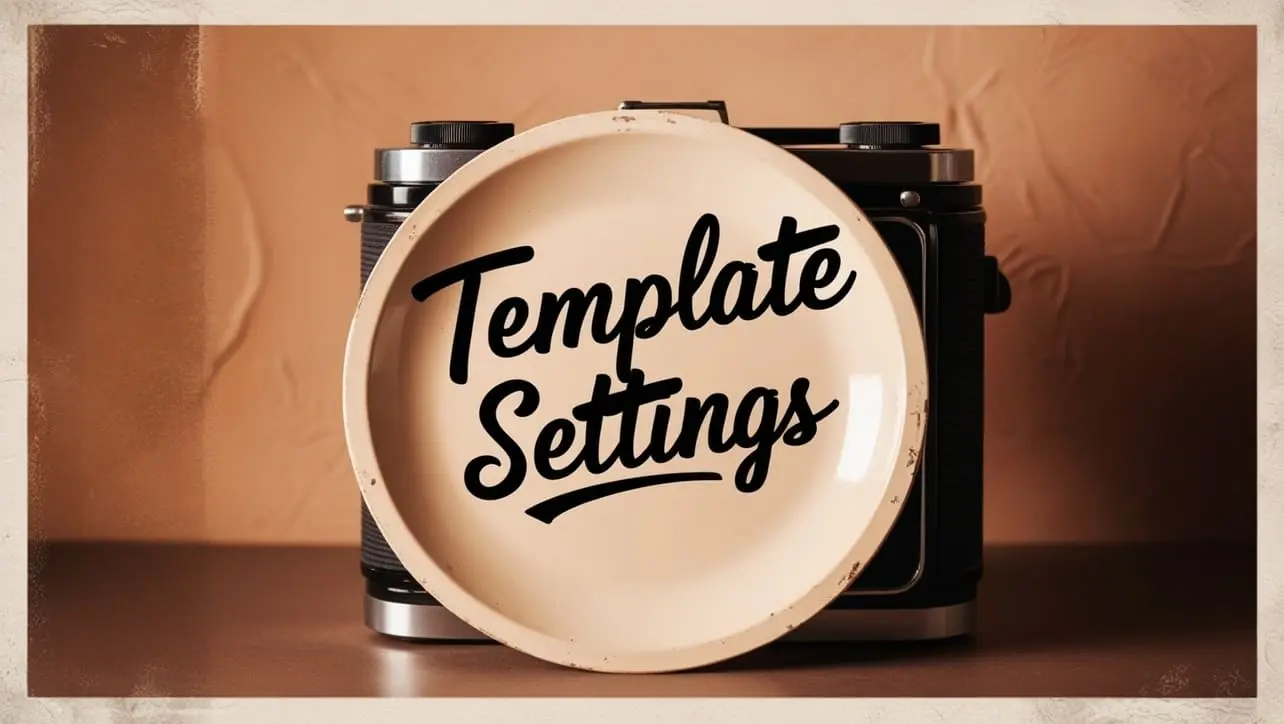
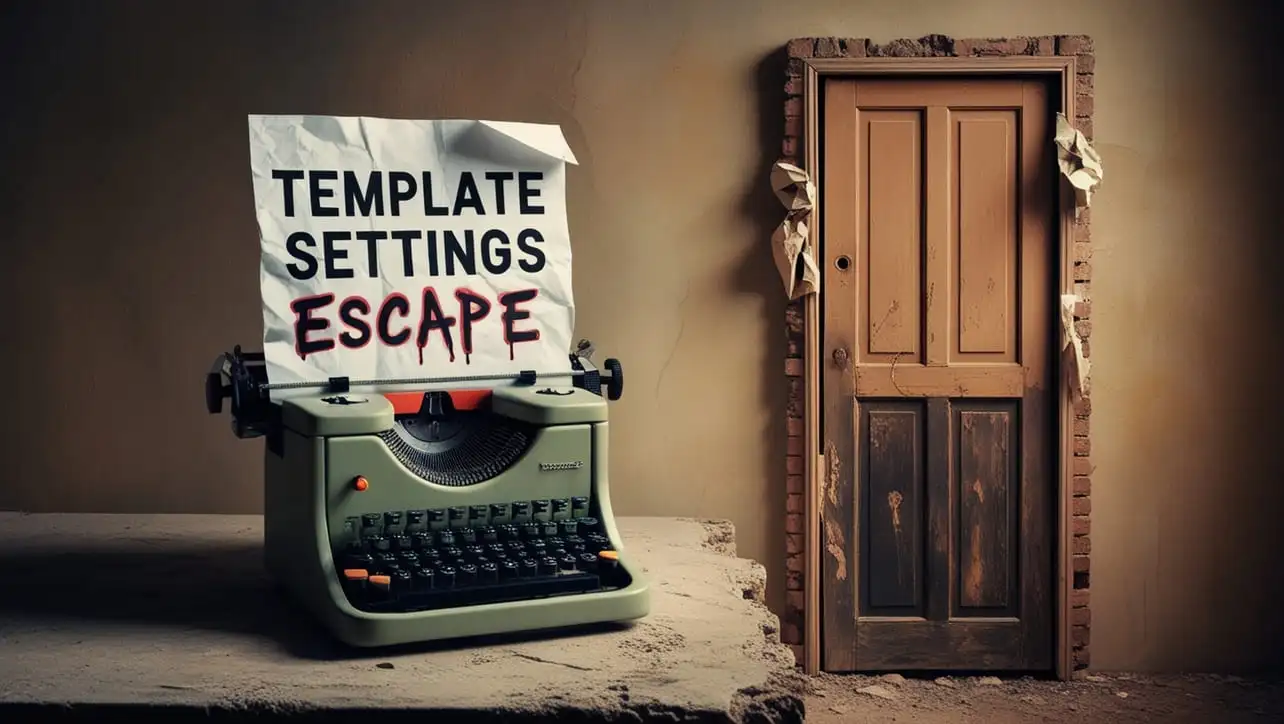
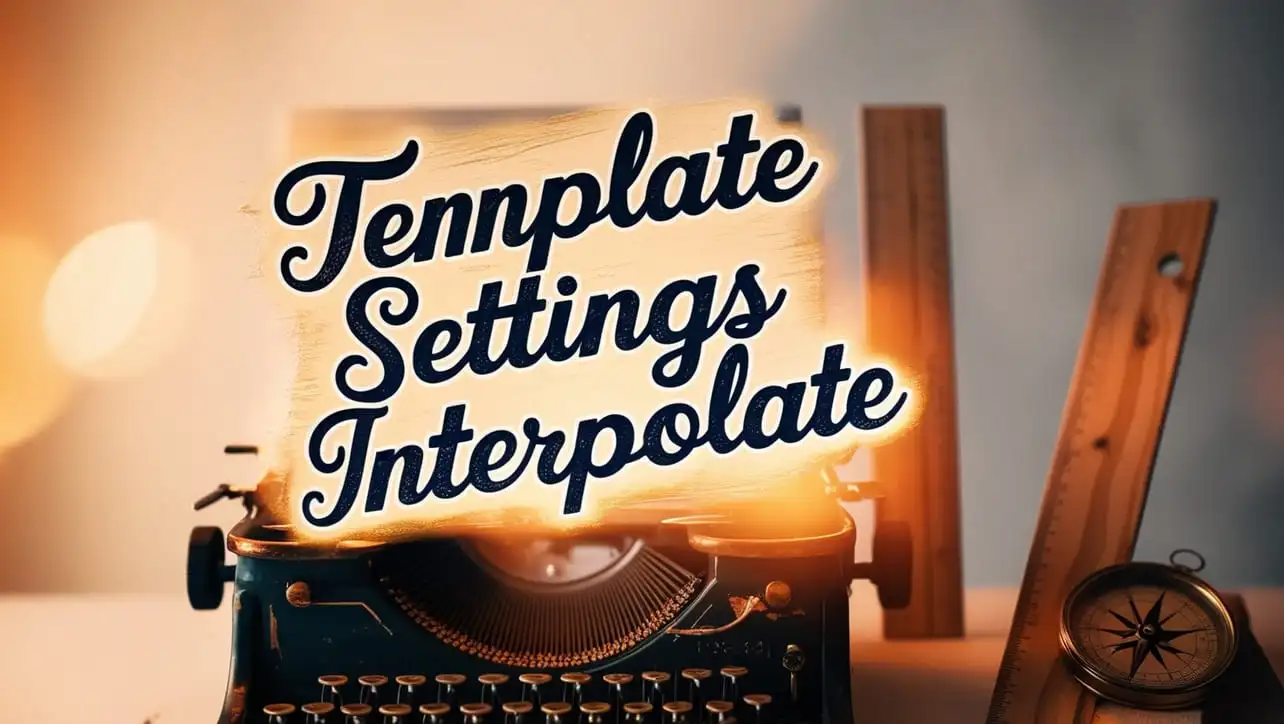
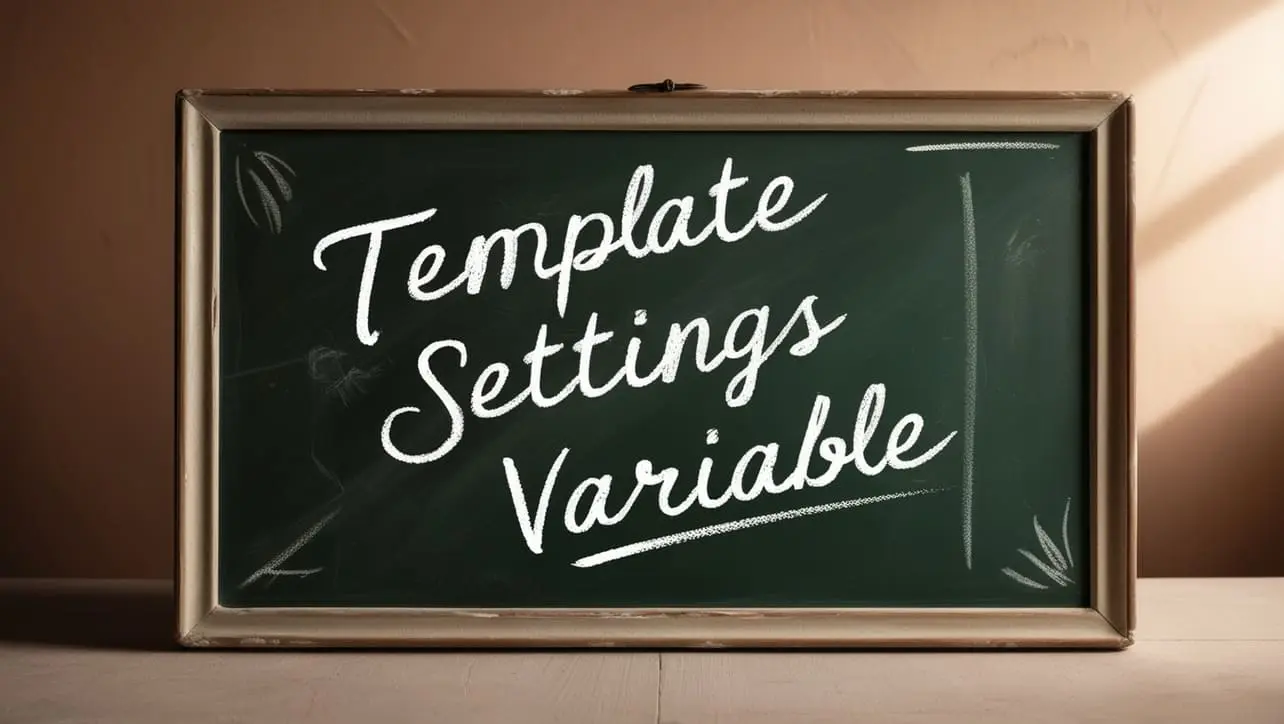
If you have any doubts regarding this article (Lodash _.attempt() Util Method), please comment here. I will help you immediately.