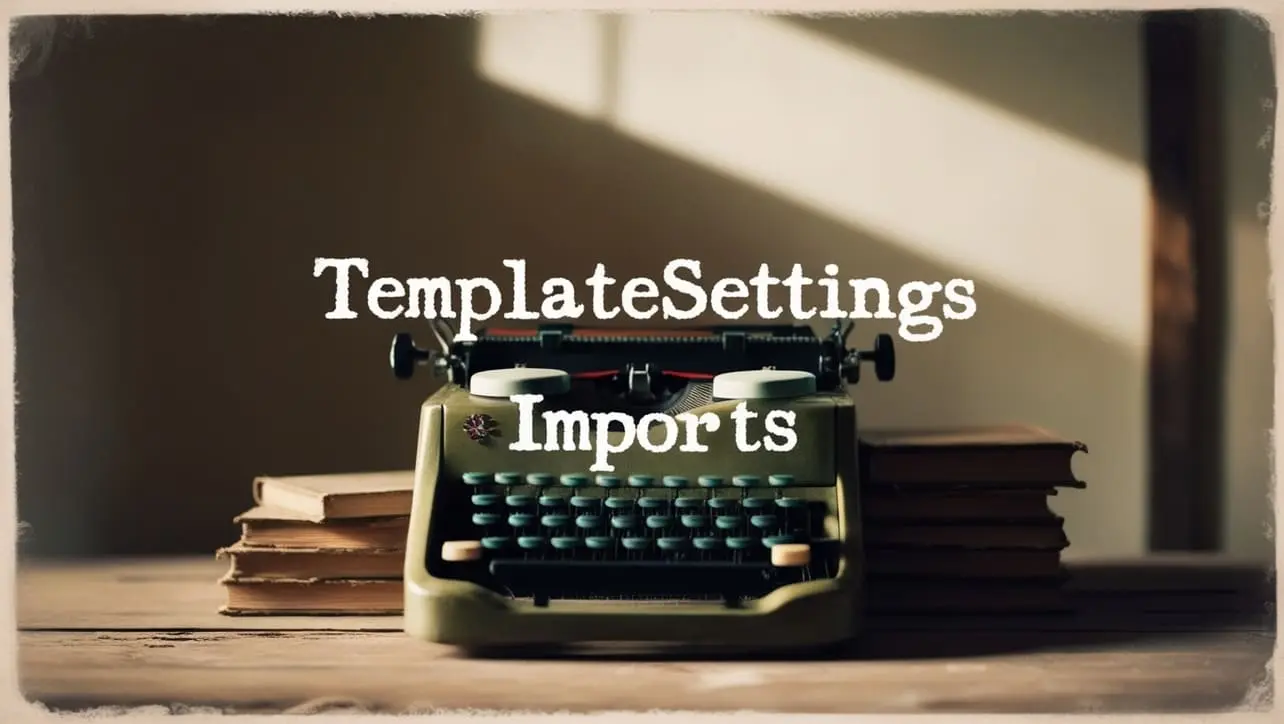
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- _.camelCase
- _.capitalize
- _.deburr
- _.endsWith
- _.escape
- _.escapeRegExp
- _.kebabCase
- _.lowerCase
- _.lowerFirst
- _.pad
- _.padEnd
- _.padStart
- _.parseInt
- _.repeat
- _.replace
- _.snakeCase
- _.split
- _.startCase
- _.startsWith
- _.template
- _.toLower
- _.toUpper
- _.trim
- _.trimEnd
- _.trimStart
- _.truncate
- _.unescape
- _.upperCase
- _.upperFirst
- _.words
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.upperCase() String Method
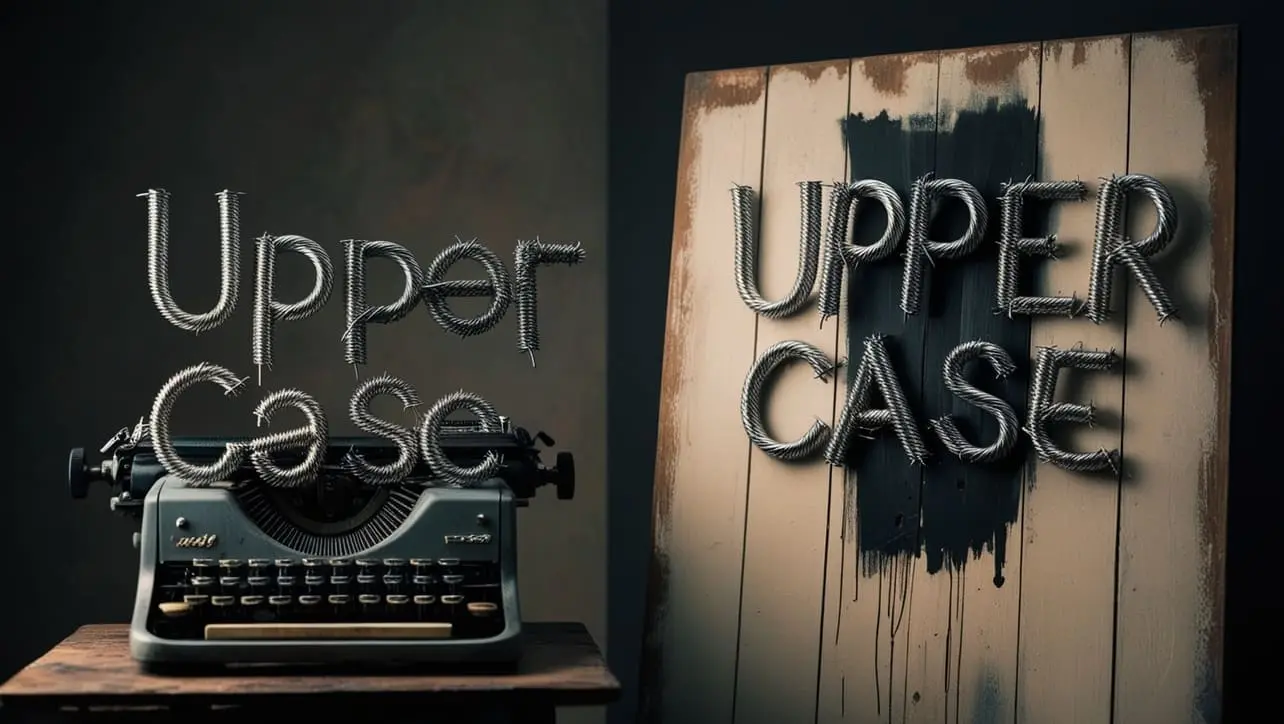
Photo Credit to CodeToFun
🙋 Introduction
In the domain of JavaScript programming, manipulating strings is a common task. Lodash, a comprehensive utility library, offers a multitude of functions to streamline string operations. Among these functions is the _.upperCase()
method, designed to convert a string to uppercase while handling special cases gracefully.
This method simplifies string manipulation tasks, enhancing code readability and developer productivity.
🧠 Understanding _.upperCase() Method
The _.upperCase()
method in Lodash transforms a string into uppercase format. Unlike native JavaScript methods such as toUpperCase(), _.upperCase()
intelligently handles special cases, ensuring consistent and accurate results across different input strings.
💡 Syntax
The syntax for the _.upperCase()
method is straightforward:
_.upperCase([string=''])
- string (Optional): The input string to convert to uppercase.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.upperCase()
method:
const _ = require('lodash');
const originalString = 'hello world';
const upperCaseString = _.upperCase(originalString);
console.log(upperCaseString);
// Output: 'HELLO WORLD'
In this example, the originalString is transformed into uppercase format using _.upperCase()
.
🏆 Best Practices
When working with the _.upperCase()
method, consider the following best practices:
Handling Empty Strings:
Ensure graceful handling of empty strings to prevent unexpected behavior when using
_.upperCase()
.example.jsCopiedconst emptyString = ''; const upperCaseEmptyString = _.upperCase(emptyString); console.log(upperCaseEmptyString); // Output: ''
Special Character Handling:
Be mindful of special characters and their behavior when converted to uppercase.
_.upperCase()
handles special cases intelligently, providing consistent results across various input strings.example.jsCopiedconst stringWithSpecialCharacters = 'hello-world'; const upperCaseSpecialString = _.upperCase(stringWithSpecialCharacters); console.log(upperCaseSpecialString); // Output: 'HELLO-WORLD'
Performance Considerations:
Consider performance implications, especially when processing large volumes of text.
_.upperCase()
offers optimized performance, making it suitable for string manipulation tasks in performance-sensitive applications.example.jsCopiedconst largeText = /* ...fetch large text data... */; const upperCaseText = _.upperCase(largeText); console.log(upperCaseText);
📚 Use Cases
Display Formatting:
_.upperCase()
is ideal for formatting text displayed to users, ensuring consistency in capitalization for a polished user experience.example.jsCopiedconst userName = /* ...fetch user name from database... */; const formattedUserName = _.upperCase(userName); console.log(`Welcome, ${formattedUserName}!`);
Data Normalization:
In scenarios requiring data normalization,
_.upperCase()
can be used to standardize text input, facilitating comparison and analysis.example.jsCopiedconst userInput = /* ...fetch user input... */; const normalizedInput = _.upperCase(userInput); console.log(`Normalized input: ${normalizedInput}`);
Text Processing:
For tasks involving text processing, such as generating reports or parsing documents,
_.upperCase()
simplifies the conversion of text to uppercase, improving code maintainability and readability.example.jsCopiedconst documentContent = /* ...fetch document content... */; const processedContent = _.upperCase(documentContent); console.log(processedContent);
🎉 Conclusion
The _.upperCase()
method in Lodash offers a straightforward solution for converting strings to uppercase, with intelligent handling of special cases. Whether you're formatting text for display, normalizing data, or processing large volumes of text, this method provides a versatile and reliable tool for string manipulation in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.upperCase()
method in your Lodash projects.
👨💻 Join our Community:
Author
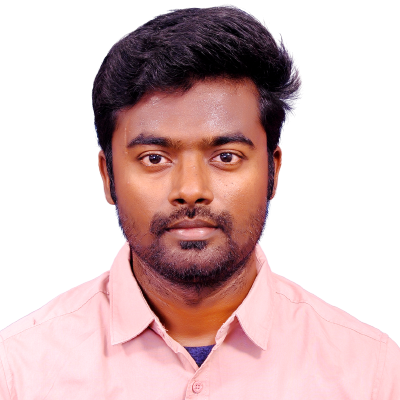
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
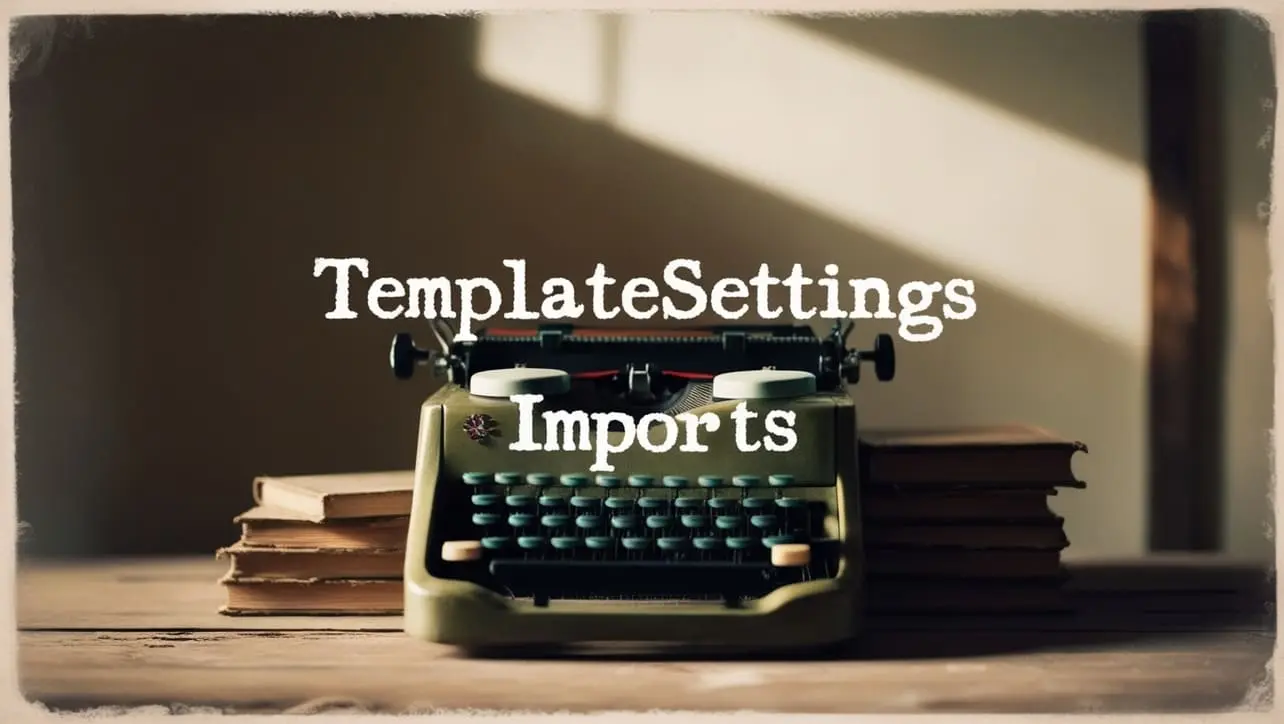
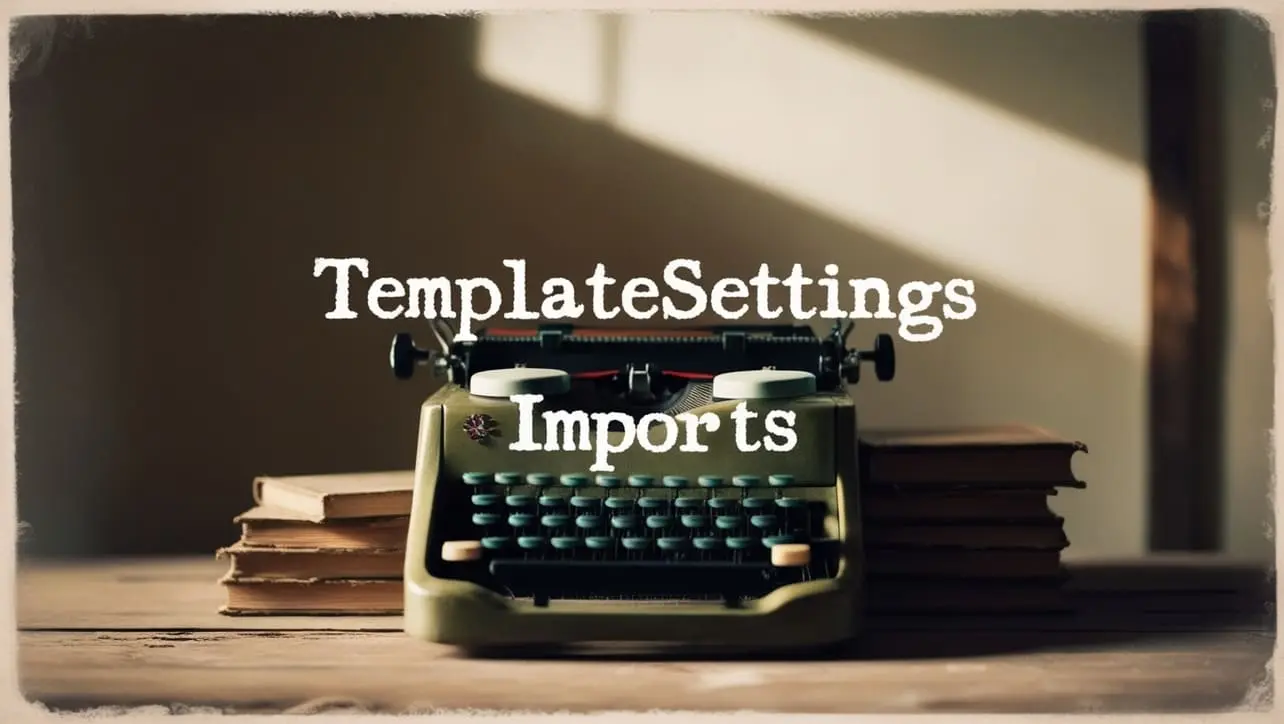
Lodash _.templateSettings.imports Property
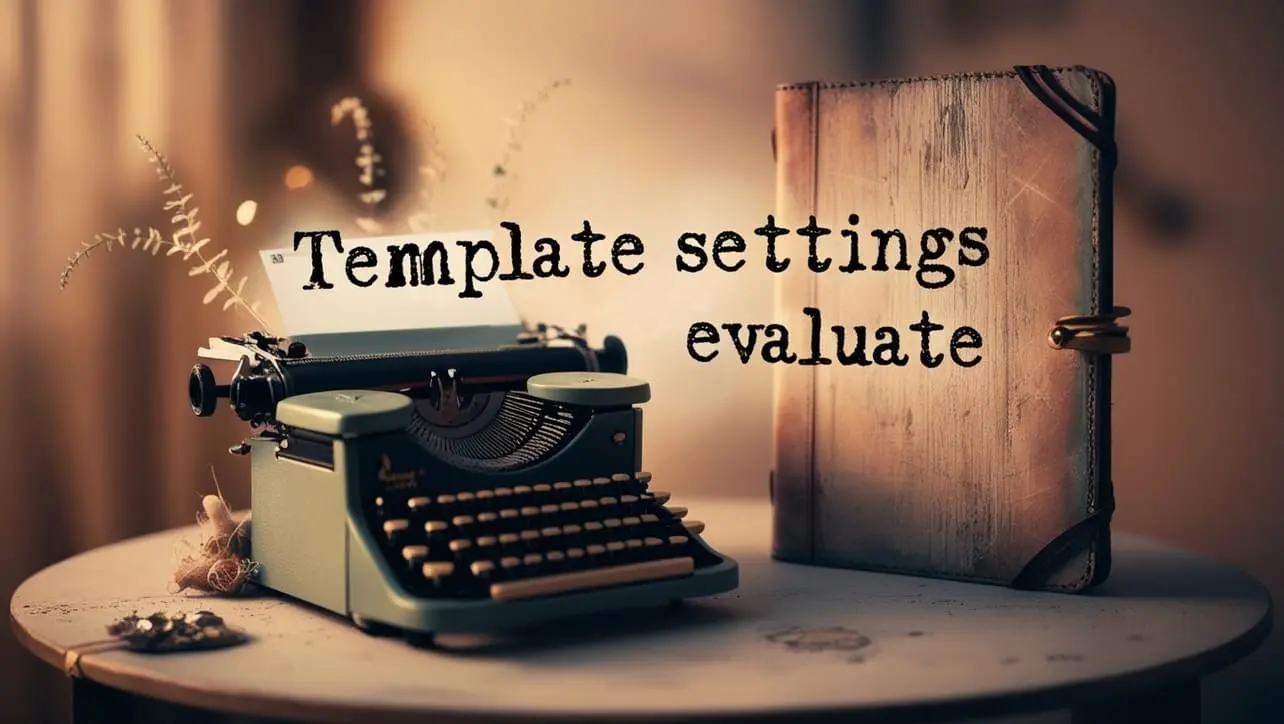
Lodash _.templateSettings.evaluate Property
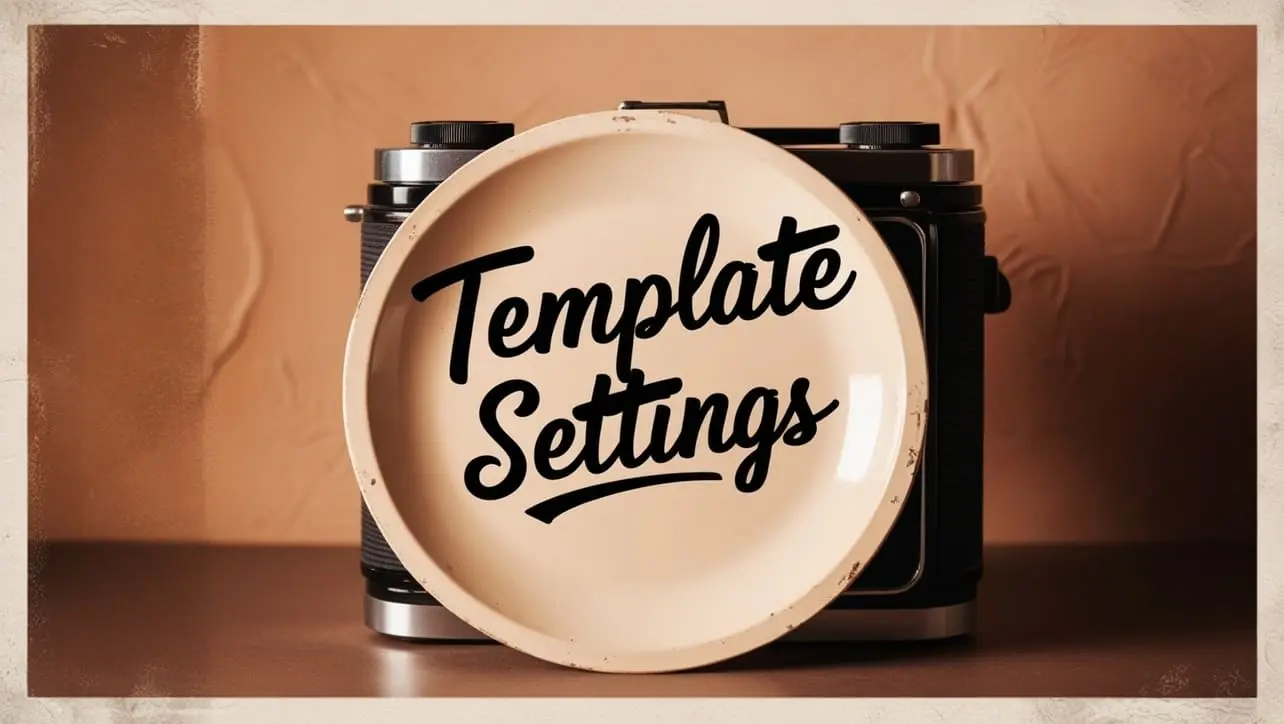
Lodash _.templateSettings Property
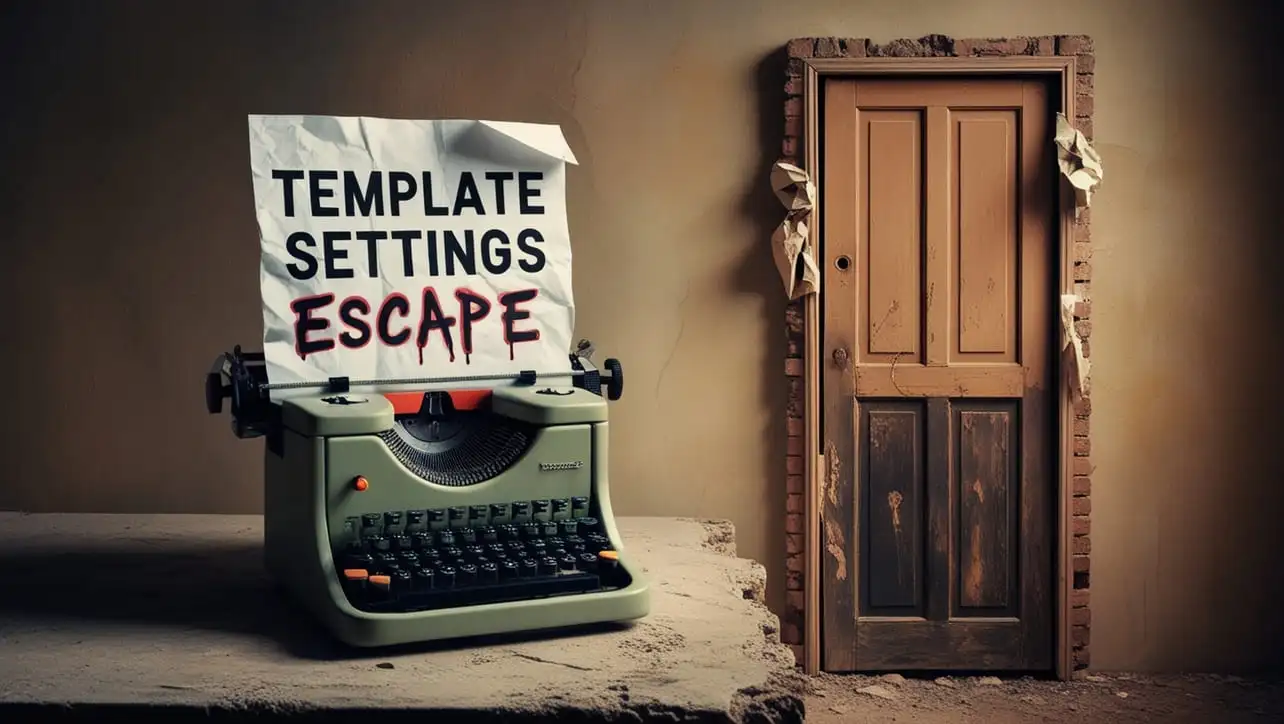
Lodash _.templateSettings.escape Property
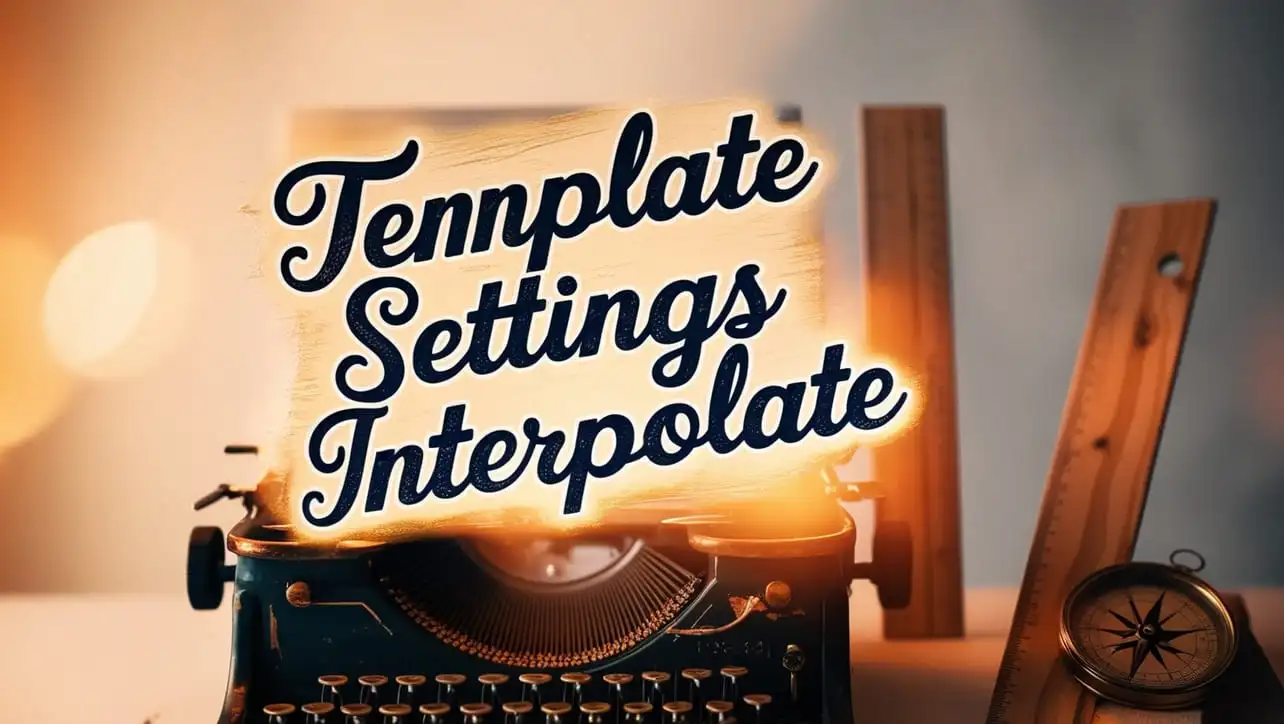
Lodash _.templateSettings.interpolate Property
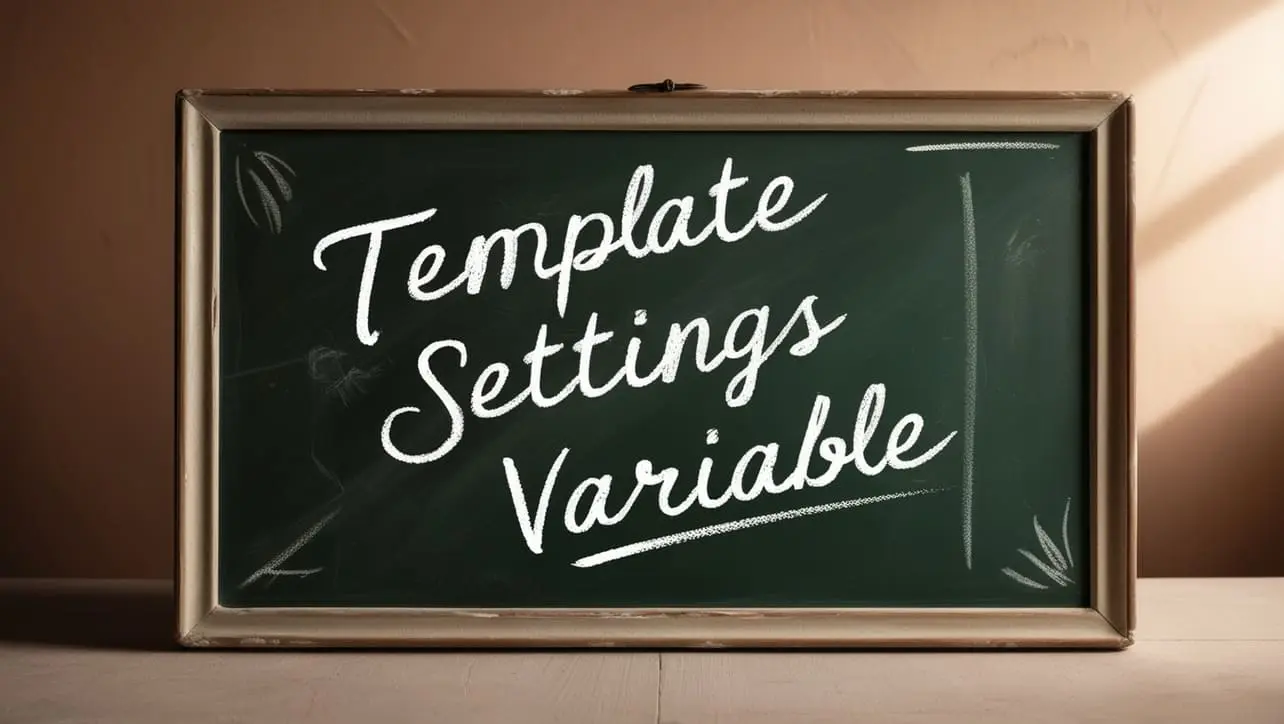
If you have any doubts regarding this article (Lodash _.upperCase() String Method), please comment here. I will help you immediately.