.webp)
C++ Basic
C++ Alphabet Pattern Programs
- C++ Alphabet Pattern
- C++ Alphabet Pattern 1
- C++ Alphabet Pattern 2
- C++ Alphabet Pattern 3
- C++ Alphabet Pattern 4
- C++ Alphabet Pattern 5
- C++ Alphabet Pattern 6
- C++ Alphabet Pattern 7
- C++ Alphabet Pattern 8
- C++ Alphabet Pattern 9
- C++ Alphabet Pattern 10
- C++ Alphabet Pattern 11
- C++ Alphabet Pattern 12
- C++ Alphabet Pattern 13
- C++ Alphabet Pattern 14
- C++ Alphabet Pattern 15
- C++ Alphabet Pattern 16
- C++ Alphabet Pattern 17
- C++ Alphabet Pattern 18
- C++ Alphabet Pattern 19
- C++ Alphabet Pattern 20
- C++ Alphabet Pattern 21
- C++ Alphabet Pattern 22
- C++ Alphabet Pattern 23
- C++ Alphabet Pattern 24
- C++ Alphabet Pattern 25
- C++ Alphabet Pattern 26
- C++ Alphabet Pattern 27
- C++ Alphabet Pattern 28
- C++ Alphabet Pattern 29
- C++ Alphabet Pattern 30
- C++ Alphabet Pattern 31
- C++ Alphabet Pattern 32
- C++ Alphabet Pattern 33
- C++ Alphabet Pattern 34
C++ Alphabet Pattern 5
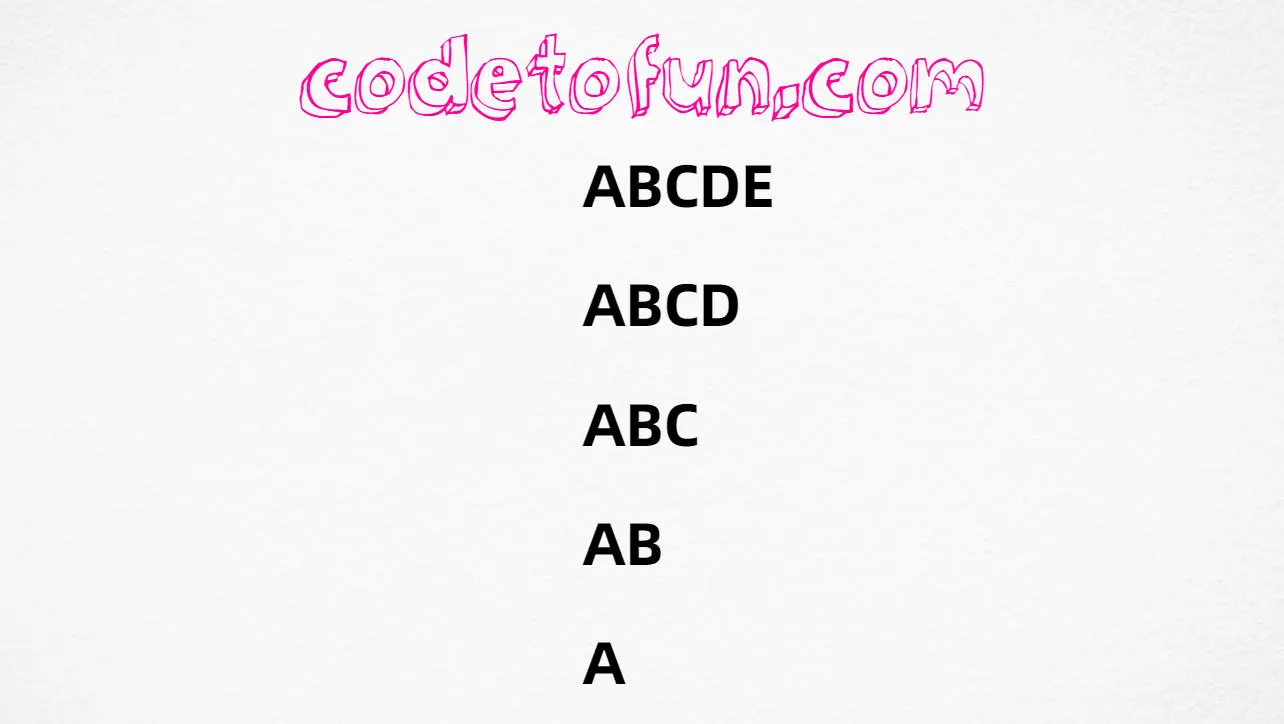
Photo Credit to CodeToFun
C++ Alphabet Pattern 5
Here`s a program that prints the above alphabet pattern using C++ Programming:
#include <iostream>
using namespace std;
int main() {
int i, j;
for (i = 69; i >= 65; i--) {
for (j = 65; j <= i; j++)
cout << (char) j;
cout << "\n";
}
return 0;
}
💻 Testing the Program
When you run the above program, it will print the following output:
ABCDE ABCD ABC AB A
🧠 How the Program Works
Let's break down the logic behind the code:
- The program starts with the inclusion of the <iostream> header file, which allows input and output operations.
- The using namespace std; statement tells the program to use the std namespace, which includes standard C++ library functions and objects.
- The int main() function is the starting point of the program. It declares two integer variables i and j.
- The for loop initializes i with a value of 69 and executes the loop as long as i is greater than or equal to 65. It decrements i by one in each iteration.
- Within the outer for loop, there is an inner for loop that initializes j with a value of 65 and continues as long as j is less than or equal to i. It increments j by one in each iteration.
- Inside the inner for loop, the program prints the character represented by the current value of j using the cout object, which represents the standard output stream.
- After the inner for loop finishes, the program prints a newline character ("\n") using cout to move the output to the next line.
- The outer for loop continues until i is no longer greater than or equal to 65.
- Finally, the return 0; statement ends the main() function and indicates that the program has executed successfully. The value 0 is conventionally used to indicate successful program execution.
💯 Tips for Enhancement:
Explore the versatility of this pattern by adjusting its parameters. Whether you increase or decrease the size, tweak the spacing, or modify the characters used, each change opens up a world of possibilities, allowing you to customize and create your unique visual effects.
✔ Conclusion:
Creating visually appealing patterns is not only a fun endeavour but also a great way to enhance your programming or design skills. We hope this tutorial has inspired you to explore the world of creative coding. Share your creations with us, and let your imagination run wild!
🤗 Closing Call-to-Action:
We'd love to see your unique interpretations of the alphabet pattern. Share your creations in the comments below, and don't hesitate to reach out if you have any questions or suggestions for future tutorials. Happy coding!
👨💻 Join our Community:
Author
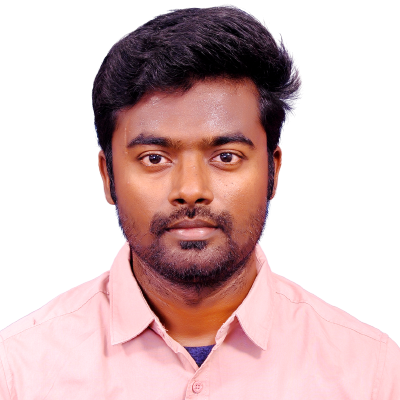
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C++ Alphabet Pattern 5) please comment here. I will help you immediately.