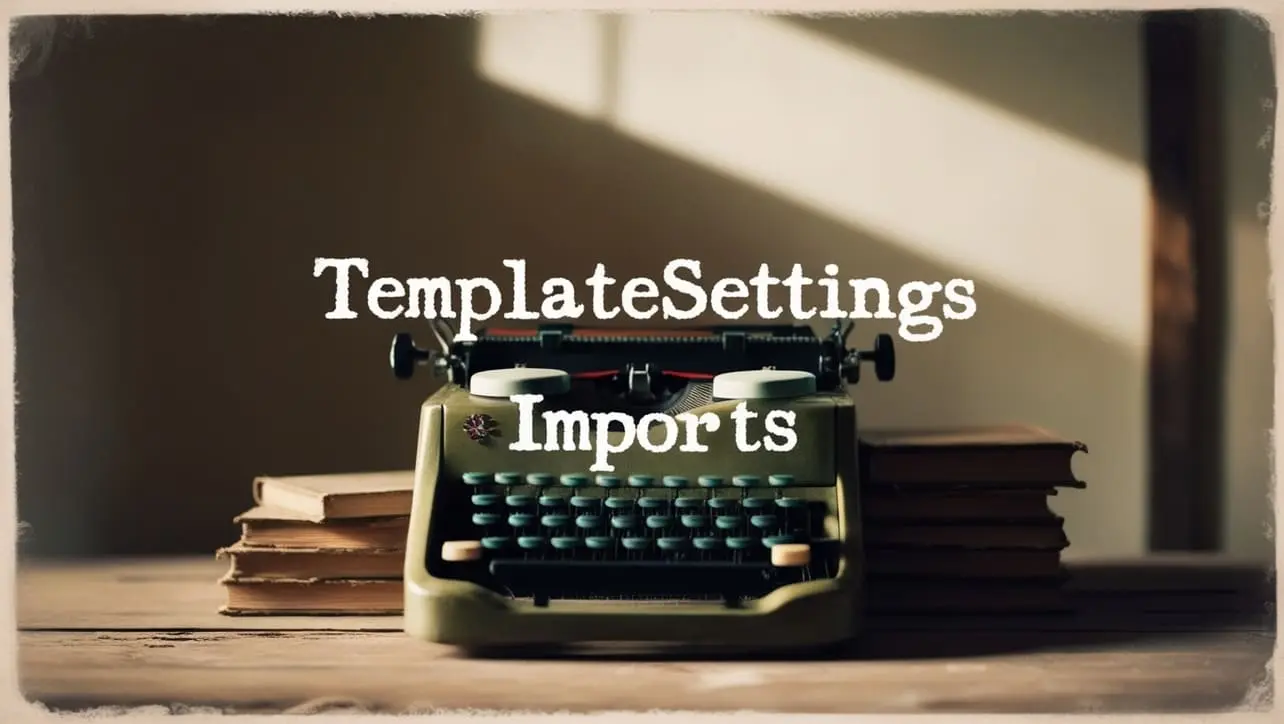
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- _.camelCase
- _.capitalize
- _.deburr
- _.endsWith
- _.escape
- _.escapeRegExp
- _.kebabCase
- _.lowerCase
- _.lowerFirst
- _.pad
- _.padEnd
- _.padStart
- _.parseInt
- _.repeat
- _.replace
- _.snakeCase
- _.split
- _.startCase
- _.startsWith
- _.template
- _.toLower
- _.toUpper
- _.trim
- _.trimEnd
- _.trimStart
- _.truncate
- _.unescape
- _.upperCase
- _.upperFirst
- _.words
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.trim() String Method
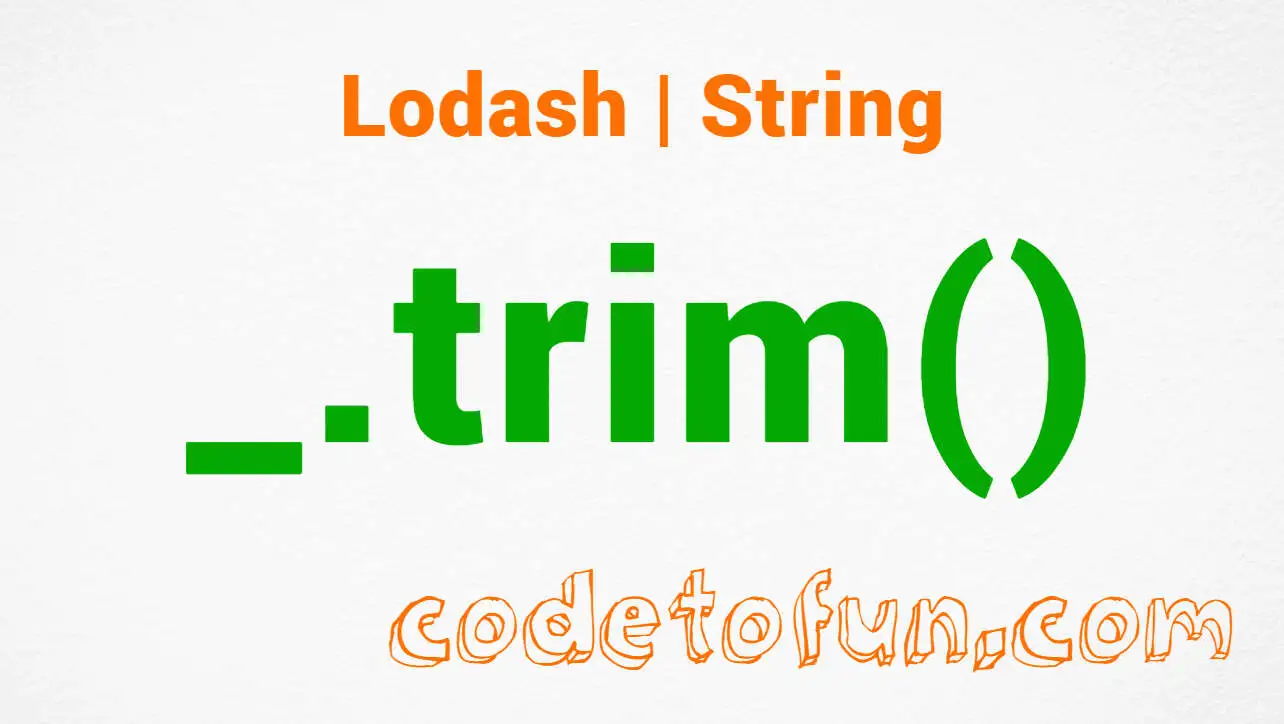
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript, managing strings effectively is crucial for many applications, from data processing to user interface manipulation. The Lodash library provides a wide array of utility functions to streamline string manipulation tasks, including the _.trim()
method.
This method offers a convenient way to remove leading and trailing whitespace from strings, improving data cleanliness and enhancing user experience.
🧠 Understanding _.trim() Method
The _.trim()
method in Lodash simplifies the process of removing leading and trailing whitespace characters (such as spaces, tabs, and newlines) from strings. This functionality is particularly useful when dealing with user input, data parsing, or formatting strings for display.
💡 Syntax
The syntax for the _.trim()
method is straightforward:
_.trim(string)
- string: The string to trim whitespace from.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.trim()
method:
const _ = require('lodash');
const untrimmedString = ' Hello, world! ';
const trimmedString = _.trim(untrimmedString);
console.log(trimmedString);
// Output: 'Hello, world!'
In this example, the untrimmedString is processed by _.trim()
, resulting in a new string without leading and trailing whitespace.
🏆 Best Practices
When working with the _.trim()
method, consider the following best practices:
Consistent Input Validation:
Ensure that input strings are properly validated before applying
_.trim()
. This helps prevent unexpected behavior and ensures consistent data processing.example.jsCopiedconst userInput = /* ...retrieve user input... */; const trimmedInput = _.trim(userInput); console.log(trimmedInput);
Preserve Inner Whitespace:
Consider using _.trimStart() and _.trimEnd() if you need to preserve whitespace within the string while removing leading or trailing whitespace.
example.jsCopiedconst stringWithInnerWhitespace = ' Hello, world! '; const trimmedStart = _.trimStart(stringWithInnerWhitespace); const trimmedEnd = _.trimEnd(stringWithInnerWhitespace); console.log(trimmedStart); console.log(trimmedEnd);
Chaining Methods:
Leverage method chaining to combine
_.trim()
with other string manipulation methods for concise and expressive code.example.jsCopiedconst messyString = ' This is a messy string. '; const cleanString = _.trim(_.toUpper(messyString)); console.log(cleanString);
📚 Use Cases
User Input Sanitization:
When processing user input,
_.trim()
can be used to remove leading and trailing whitespace, ensuring consistency and improving data integrity.example.jsCopiedconst userInput = /* ...retrieve user input... */; const sanitizedInput = _.trim(userInput); console.log(sanitizedInput);
Data Formatting:
In scenarios where data formatting is necessary,
_.trim()
can help ensure that strings are properly formatted for display or further processing.example.jsCopiedconst unformattedData = /* ...retrieve unformatted data... */; const formattedData = _.map(unformattedData, _.trim); console.log(formattedData);
String Comparison:
When comparing strings, it's often beneficial to remove extraneous whitespace to ensure accurate comparisons.
_.trim()
can facilitate this process.example.jsCopiedconst string1 = ' Hello '; const string2 = 'Hello'; console.log(_.isEqual(_.trim(string1), string2)); // Output: true
🎉 Conclusion
The _.trim()
method in Lodash provides a convenient solution for removing leading and trailing whitespace from strings in JavaScript. Whether you're sanitizing user input, formatting data, or comparing strings, _.trim()
offers a simple yet powerful tool for enhancing string manipulation tasks.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.trim()
method in your Lodash projects.
👨💻 Join our Community:
Author
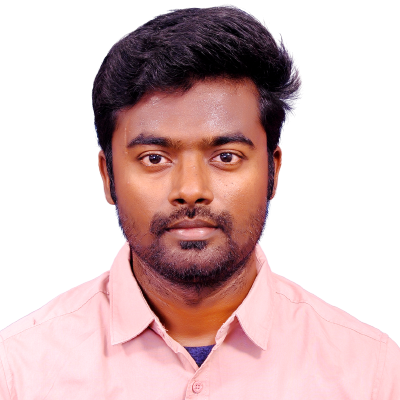
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
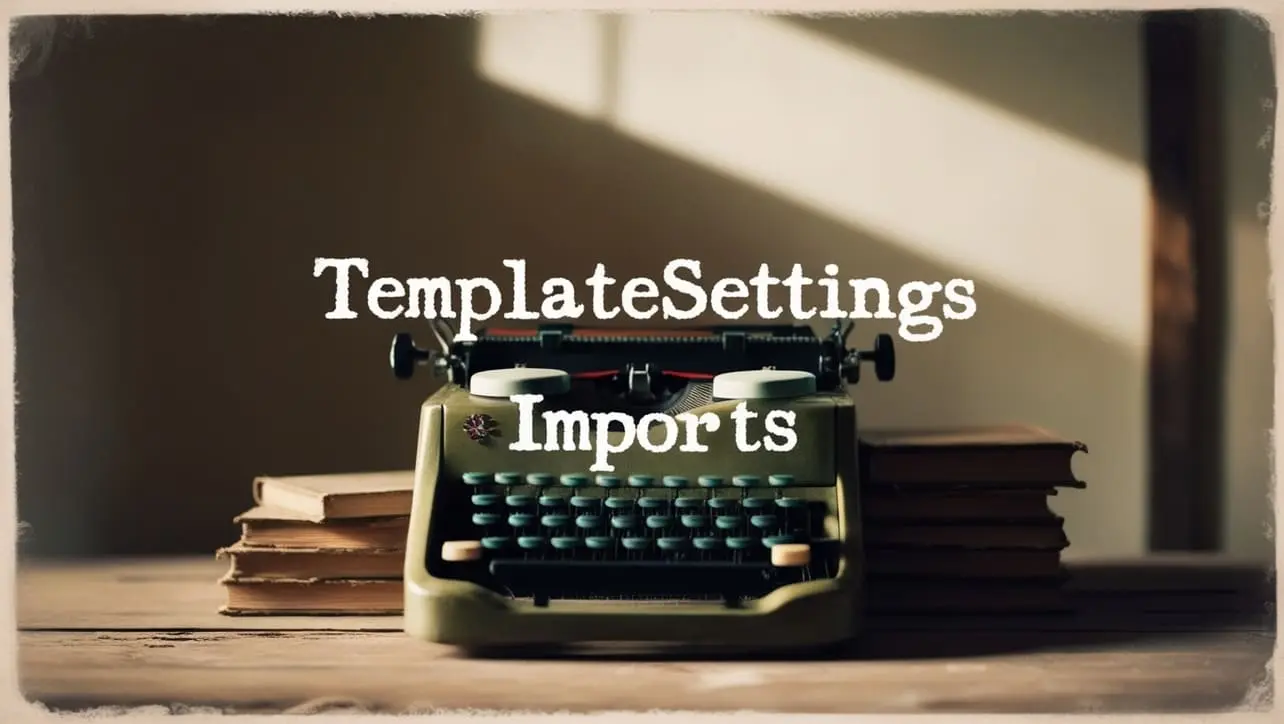
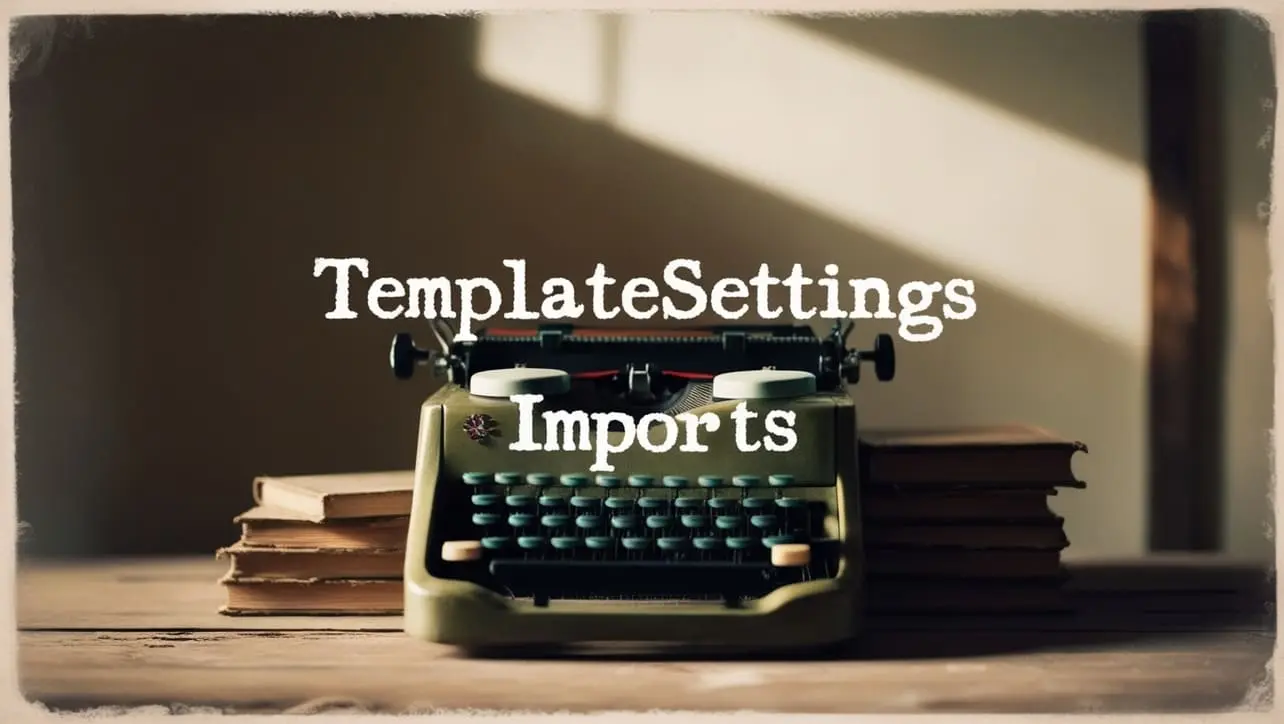
Lodash _.templateSettings.imports Property
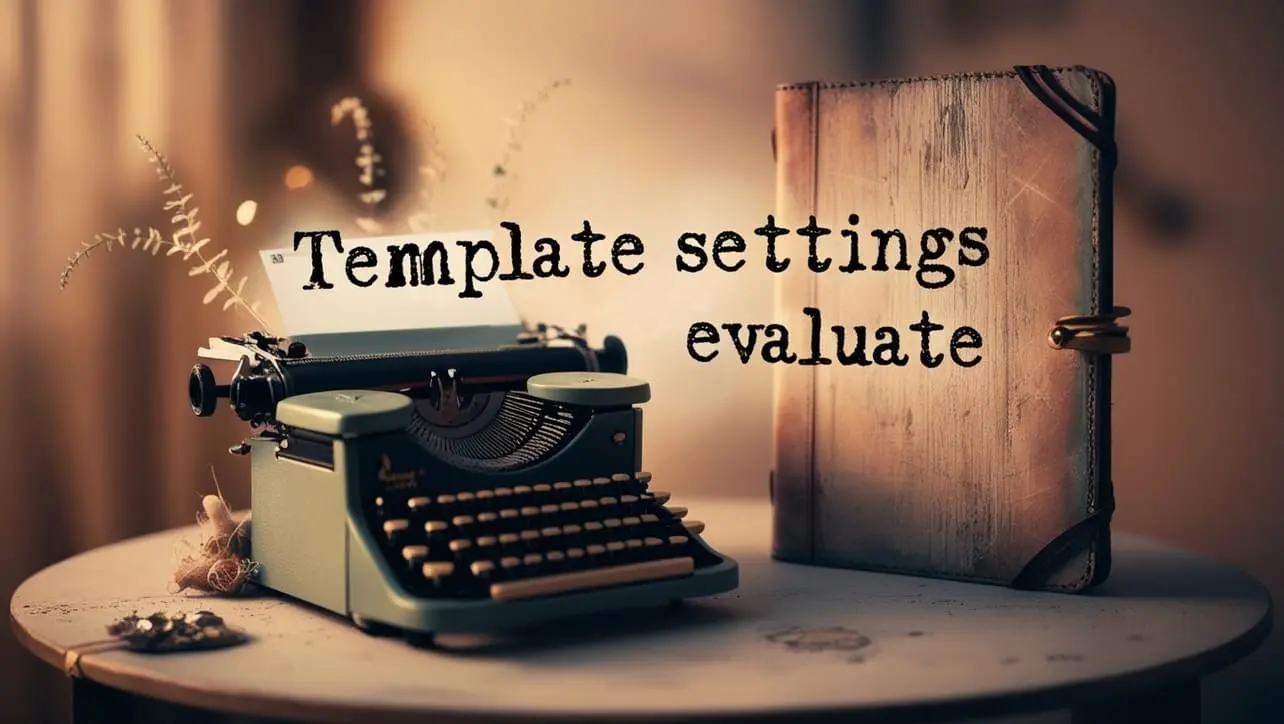
Lodash _.templateSettings.evaluate Property
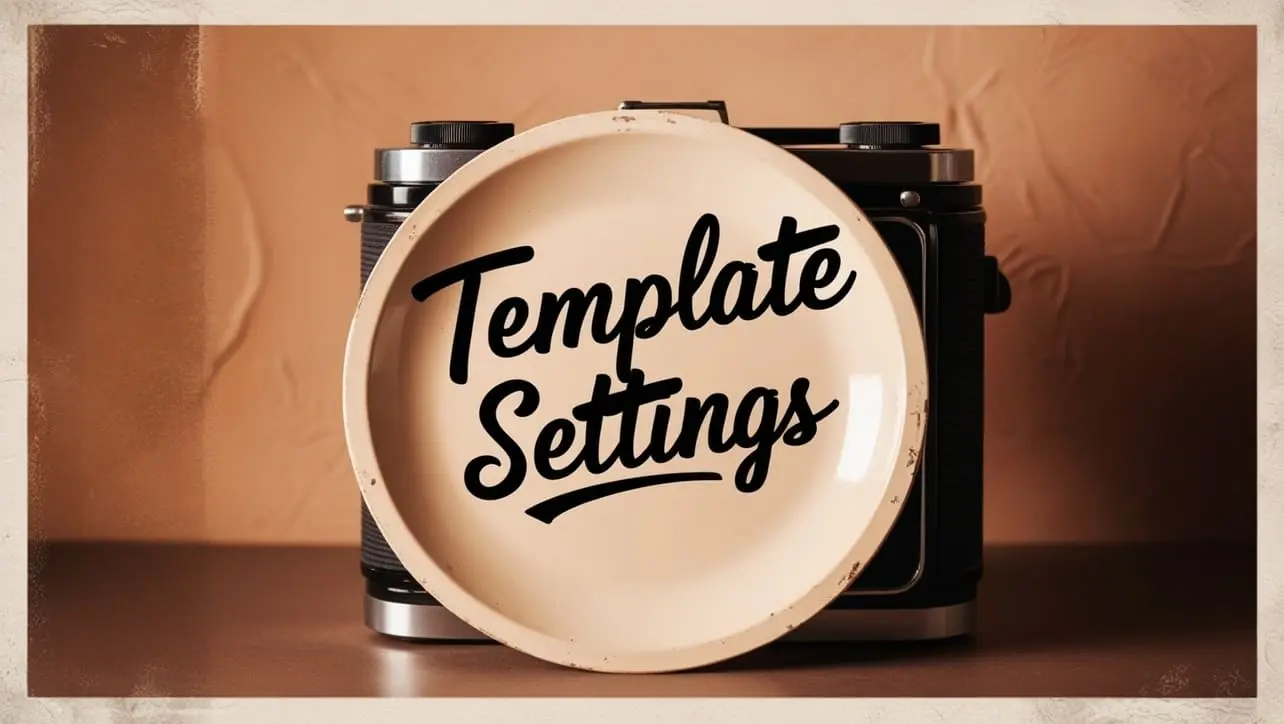
Lodash _.templateSettings Property
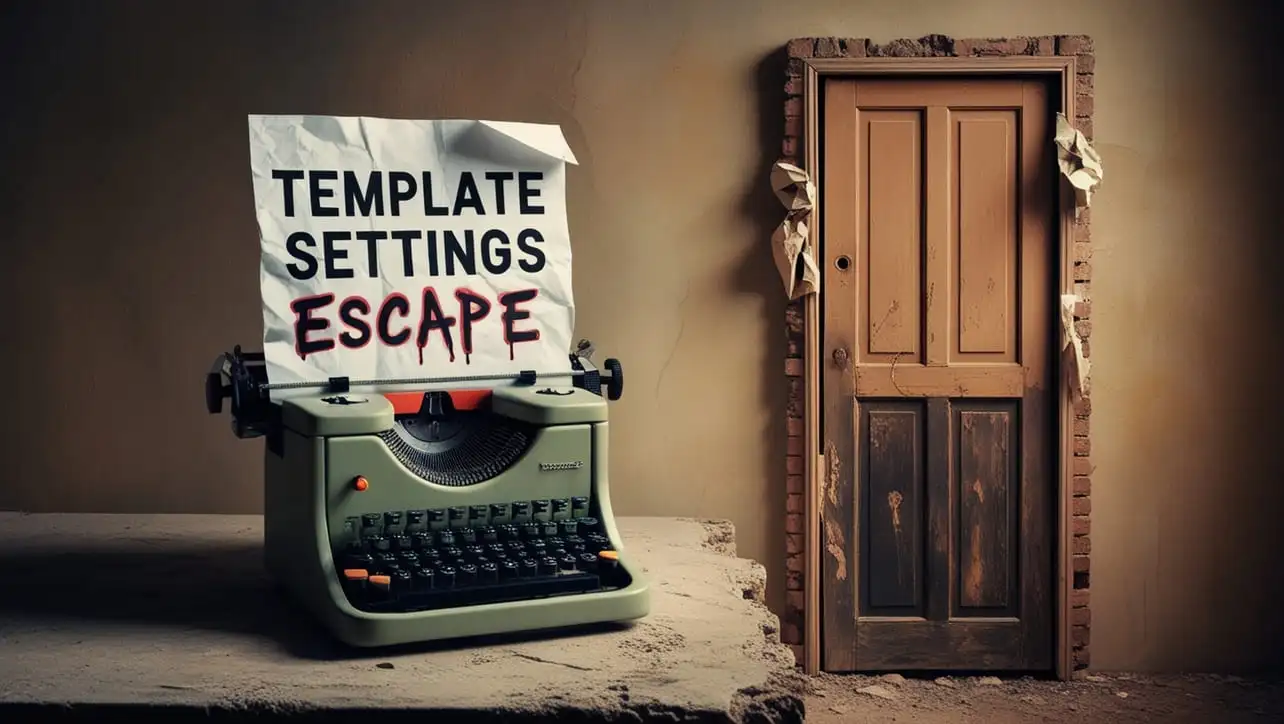
Lodash _.templateSettings.escape Property
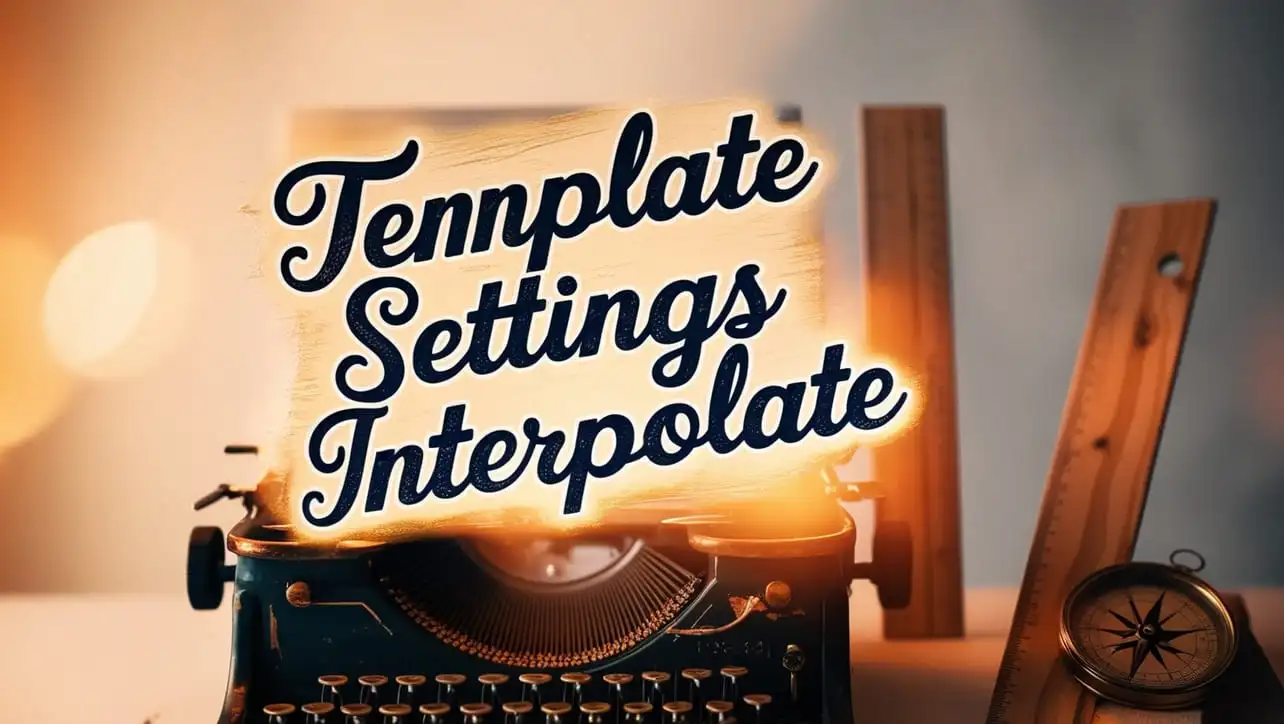
Lodash _.templateSettings.interpolate Property
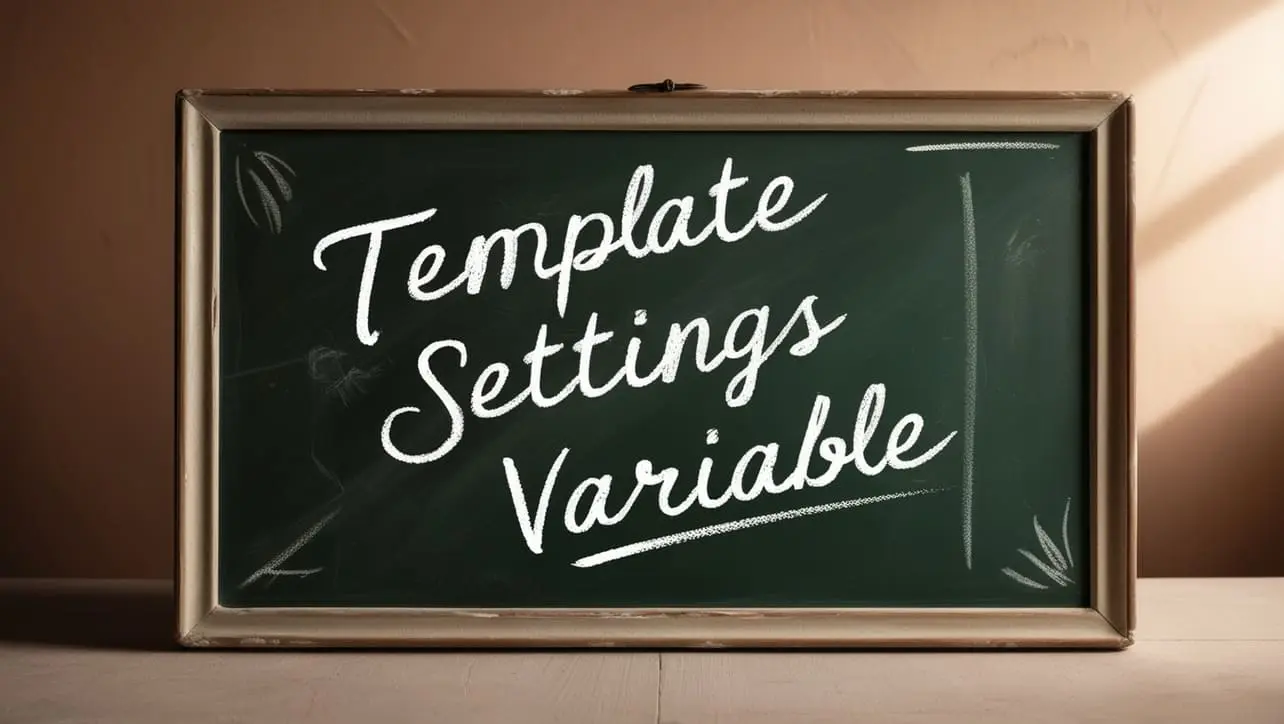
If you have any doubts regarding this article (Lodash _.trim() String Method), please comment here. I will help you immediately.