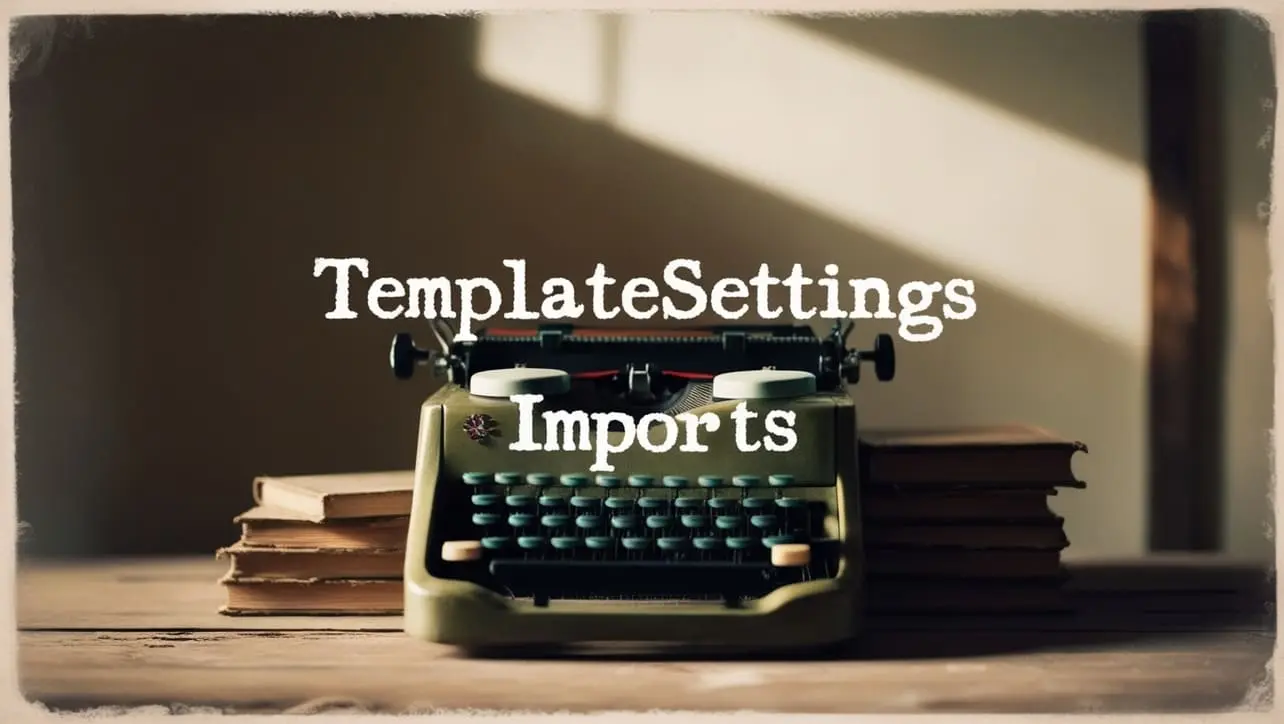
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- _.camelCase
- _.capitalize
- _.deburr
- _.endsWith
- _.escape
- _.escapeRegExp
- _.kebabCase
- _.lowerCase
- _.lowerFirst
- _.pad
- _.padEnd
- _.padStart
- _.parseInt
- _.repeat
- _.replace
- _.snakeCase
- _.split
- _.startCase
- _.startsWith
- _.template
- _.toLower
- _.toUpper
- _.trim
- _.trimEnd
- _.trimStart
- _.truncate
- _.unescape
- _.upperCase
- _.upperFirst
- _.words
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.template() String Method
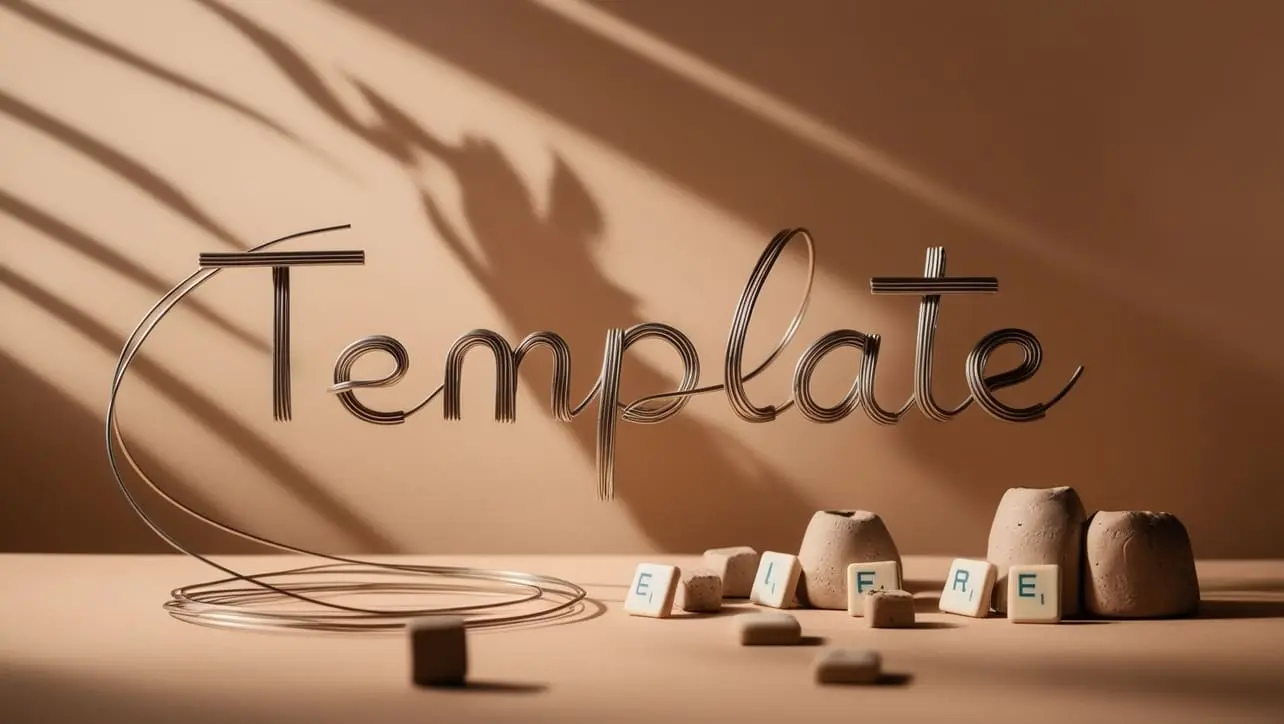
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript development, string interpolation and template rendering are common tasks. Lodash, a popular utility library, provides the _.template()
method, offering a flexible and powerful solution for string templating.
This method allows developers to create dynamic strings by embedding variables and expressions, making it a valuable tool for generating HTML, emails, and other text-based content dynamically.
🧠 Understanding _.template() Method
The _.template()
method in Lodash enables the creation of template functions that can be used to render strings with embedded variables and expressions. This method supports various delimiters and escape characters, providing extensive customization options for string interpolation.
💡 Syntax
The syntax for the _.template()
method is straightforward:
_.template(string, [options])
- string: The template string.
- options (Optional) : An object specifying options for template compilation.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.template()
method:
const _ = require('lodash');
const templateString = 'Hello, <%= name %>!';
const compiledTemplate = _.template(templateString);
const renderedString = compiledTemplate({ name: 'John' });
console.log(renderedString);
// Output: Hello, John!
In this example, the templateString containing <%= name %> is compiled into a template function using _.template()
. The compiled template is then invoked with an object containing the name variable, resulting in the rendered string "Hello, John!".
🏆 Best Practices
When working with the _.template()
method, consider the following best practices:
Avoid HTML Injection:
When embedding user input in templates, be cautious of HTML injection vulnerabilities. Sanitize user-provided data or use appropriate escape mechanisms to mitigate security risks.
example.jsCopiedconst userInput = '<script>alert("Dangerous script!");</script>'; const templateString = 'User input: <%= userInput %>'; const compiledTemplate = _.template(templateString); const renderedString = compiledTemplate({ userInput }); console.log(renderedString); // Output: User input: <script>alert("Dangerous script!");</script>
Customize Delimiters:
Customize delimiters to avoid conflicts with existing template syntax or improve code readability. Lodash allows you to define custom opening and closing delimiters to suit your preferences.
example.jsCopiedconst templateString = 'Hello, ${ name }!'; const compiledTemplate = _.template(templateString, { interpolate: /#{([\s\S]+?)}/g }); const renderedString = compiledTemplate({ name: 'Jane' }); console.log(renderedString); // Output: Hello, Jane!
Reuse Compiled Templates:
For improved performance, consider reusing compiled templates instead of recompiling them for each rendering. Store compiled templates in variables or functions to avoid unnecessary overhead.
example.jsCopiedconst templateString = 'Hello, <%= name %>!'; const compiledTemplate = _.template(templateString); function renderGreeting(name) { return compiledTemplate({ name }); } console.log(renderGreeting('Alice')); console.log(renderGreeting('Bob'));
📚 Use Cases
Generating HTML Content:
_.template()
is commonly used for generating HTML content dynamically, allowing developers to create reusable templates for generating complex HTML structures.example.jsCopiedconst user = { name: 'Alice', email: 'alice@example.com' }; const userTemplate = _.template(` <div class="user-profile"> <h2><%= user.name %></h2> <p>Email: <%= user.email %></p> </div> `); const renderedUserProfile = userTemplate({ user }); document.body.innerHTML = renderedUserProfile;
Email Templating:
When sending dynamic emails,
_.template()
can be utilized to create email templates with personalized content, such as user names, dates, and custom messages.example.jsCopiedconst emailTemplate = _.template(` <p>Dear <%= recipientName %>,</p> <p>This is a notification regarding your recent purchase.</p> <p>Order ID: <%= orderId %></p> <p>Thank you for shopping with us!</p> `); const emailContent = emailTemplate({ recipientName: 'Alice', orderId: '123456' }); sendEmail(recipientEmail, 'Order Notification', emailContent);
Dynamic Text Generation:
For dynamic text generation, such as generating reports or generating text-based documents,
_.template()
can be used to create customizable templates with placeholders for dynamic data.example.jsCopiedconst reportTemplate = _.template(` Report for <%= month %> <%= year %> ----------------------------- Total Sales: <%= totalSales %> Total Expenses: <%= totalExpenses %> Net Profit: <%= netProfit %> `); const monthlyReport = reportTemplate({ month: 'January', year: 2023, totalSales: 50000, totalExpenses: 30000, netProfit: 20000 }); console.log(monthlyReport);
🎉 Conclusion
The _.template()
method in Lodash offers a versatile and powerful solution for string templating in JavaScript. Whether you're generating HTML content, composing dynamic emails, or creating text-based documents, _.template()
provides extensive customization options and efficient rendering capabilities.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.template()
method in your Lodash projects.
👨💻 Join our Community:
Author
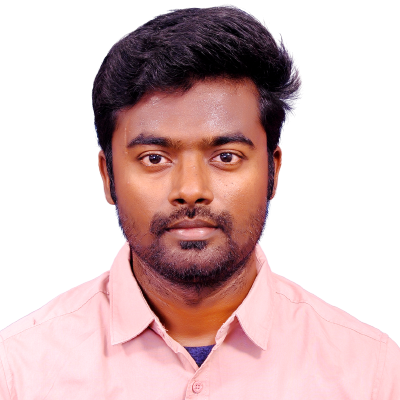
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
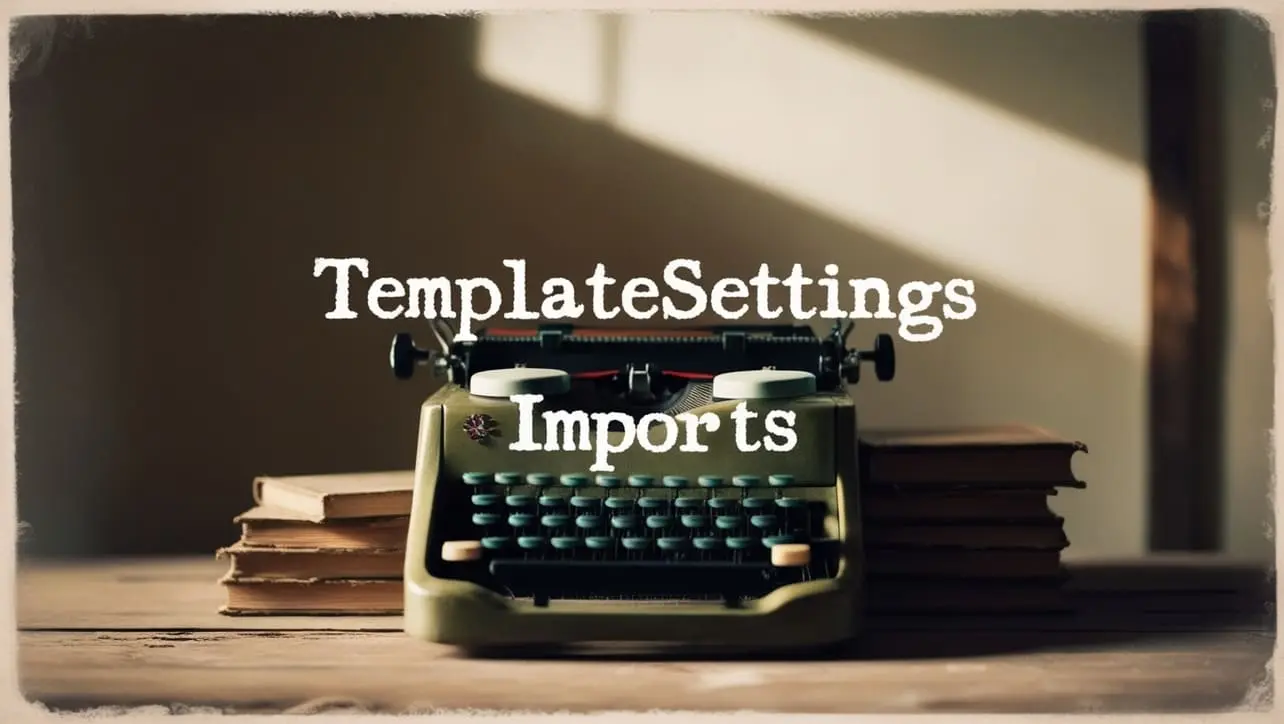
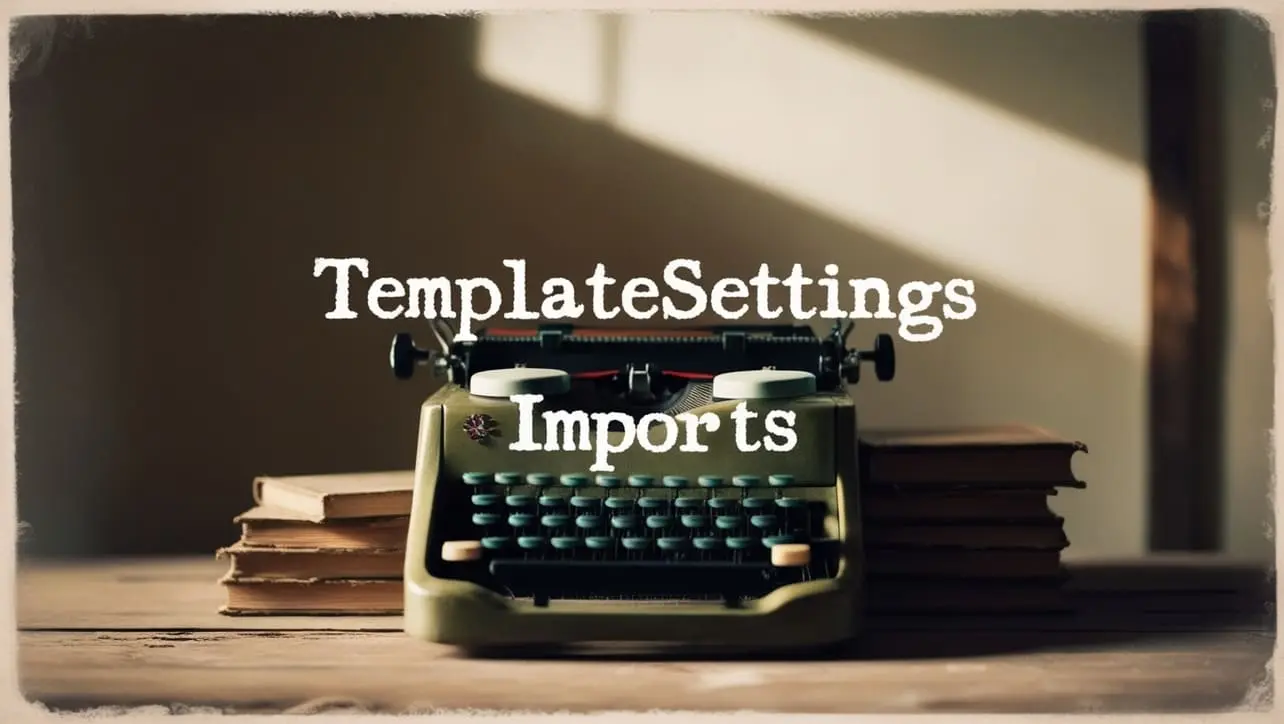
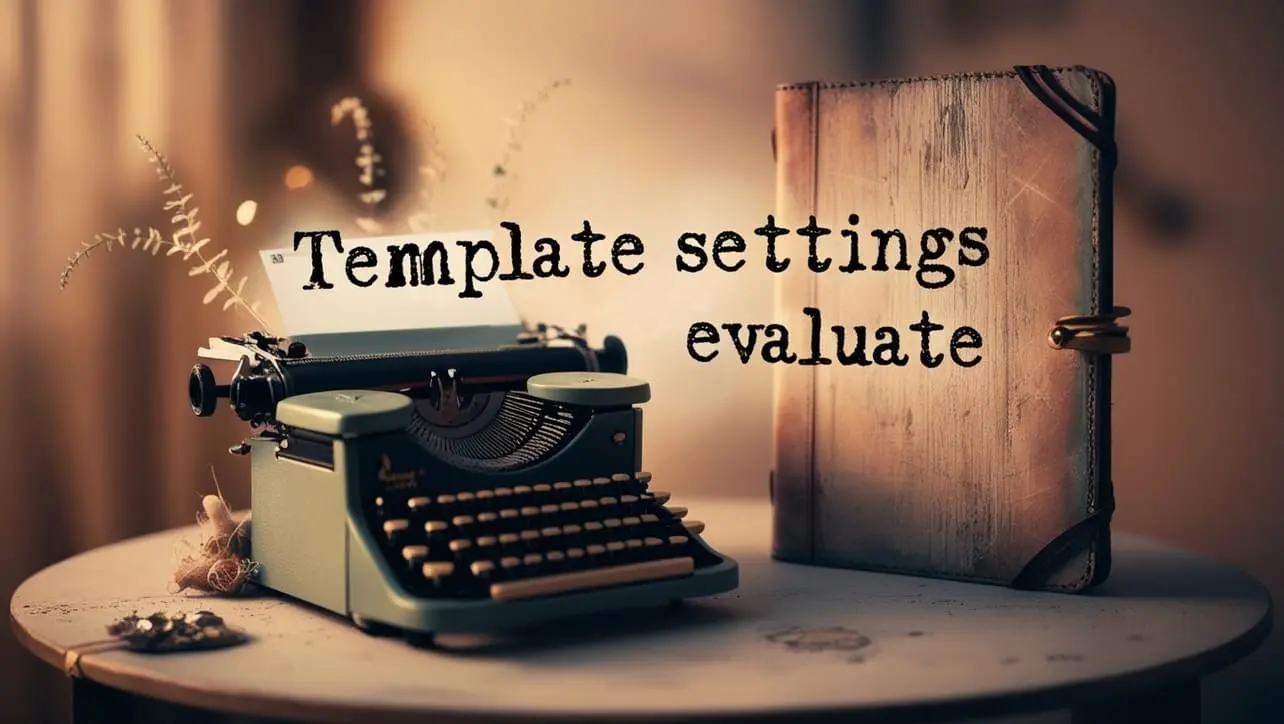
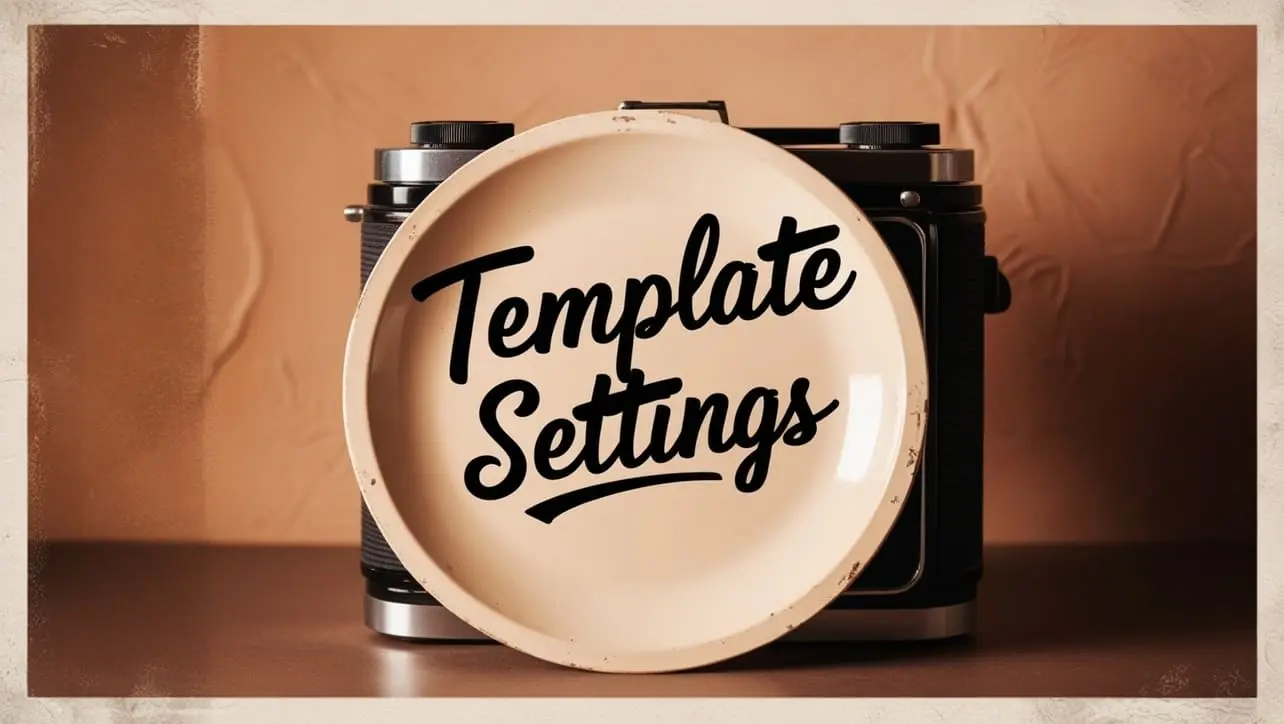
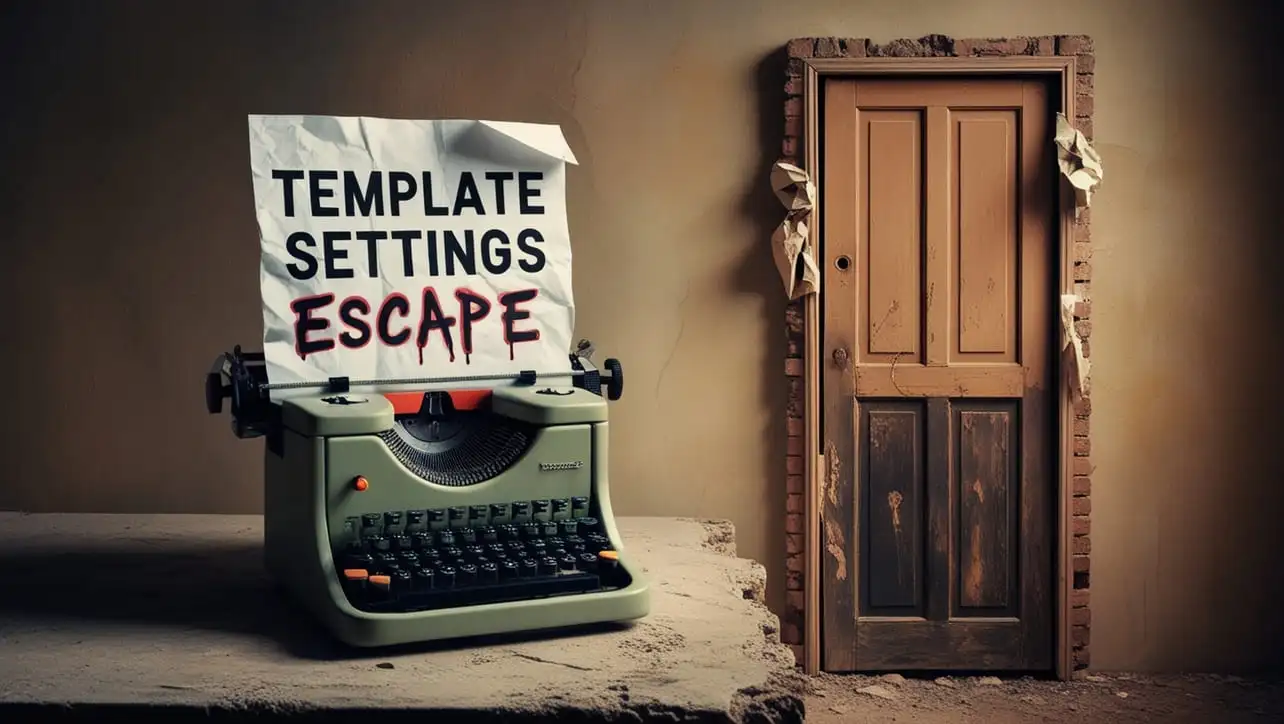
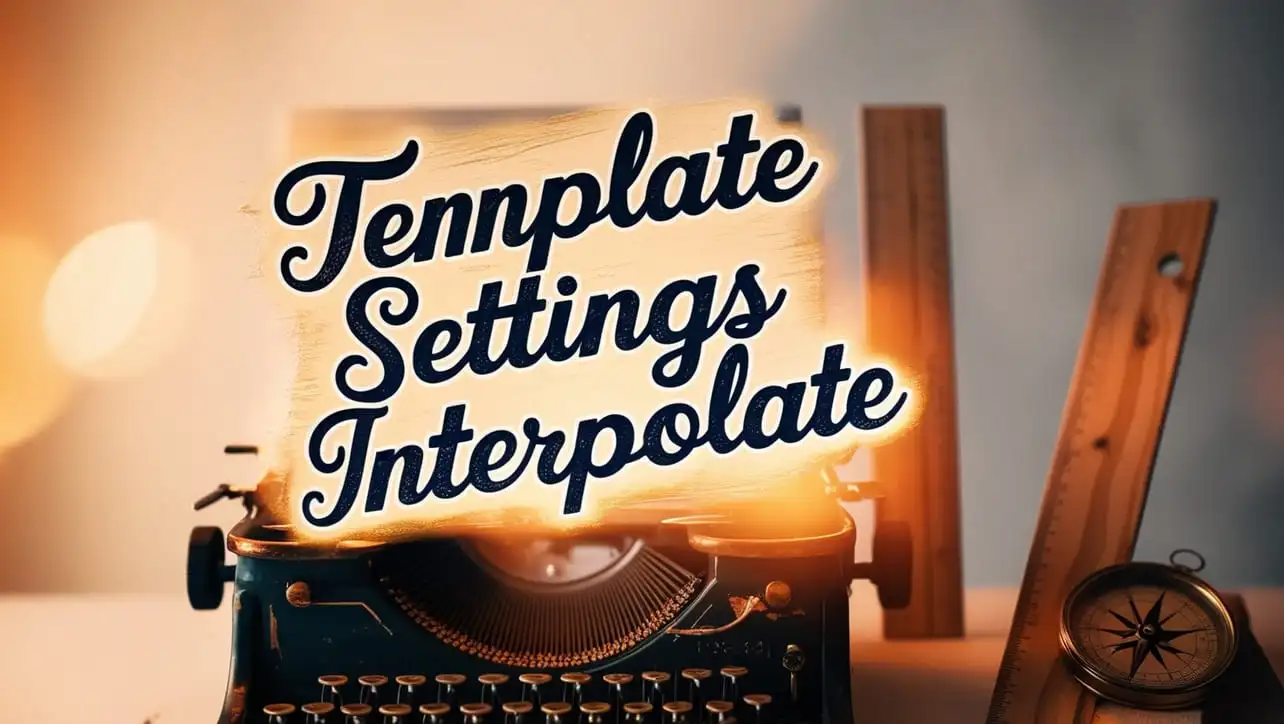
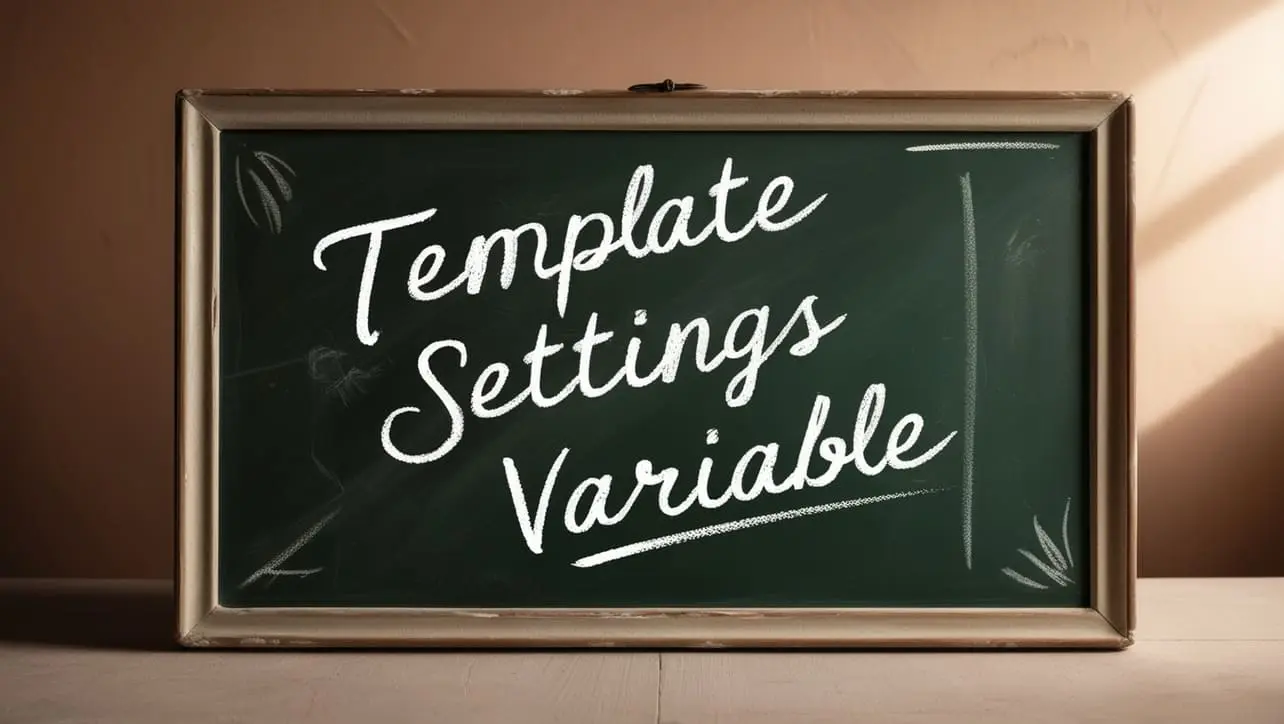
If you have any doubts regarding this article (Lodash _.template() String Method), please comment here. I will help you immediately.