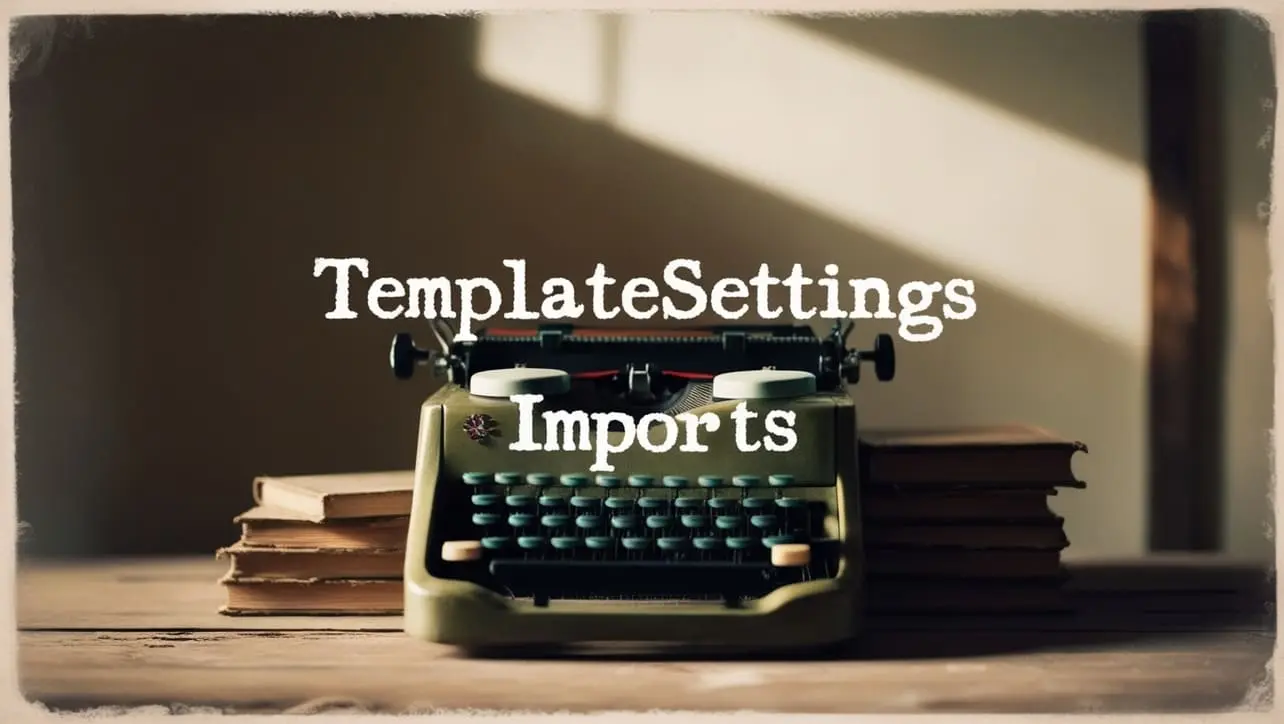
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- _.camelCase
- _.capitalize
- _.deburr
- _.endsWith
- _.escape
- _.escapeRegExp
- _.kebabCase
- _.lowerCase
- _.lowerFirst
- _.pad
- _.padEnd
- _.padStart
- _.parseInt
- _.repeat
- _.replace
- _.snakeCase
- _.split
- _.startCase
- _.startsWith
- _.template
- _.toLower
- _.toUpper
- _.trim
- _.trimEnd
- _.trimStart
- _.truncate
- _.unescape
- _.upperCase
- _.upperFirst
- _.words
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.startsWith() String Method
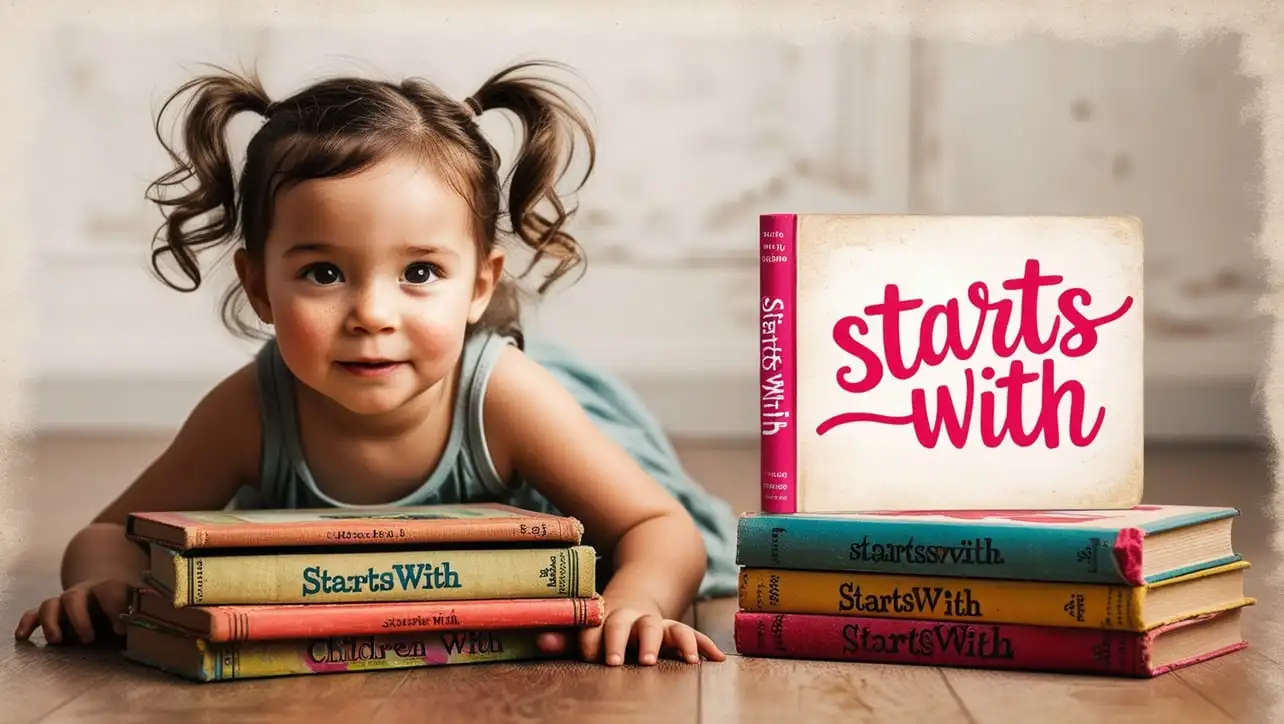
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript development, string manipulation is a common task, and having efficient tools to perform such operations is crucial. Enter Lodash, a comprehensive utility library that offers a plethora of functions to simplify JavaScript programming. Among its arsenal is the _.startsWith()
method, designed to determine whether a string begins with a specified prefix.
This method provides developers with a convenient and reliable way to handle string comparisons, enhancing code readability and efficiency.
🧠 Understanding _.startsWith() Method
The _.startsWith()
method in Lodash enables developers to check if a string starts with a specified prefix. This functionality is particularly useful in scenarios where string comparison or validation is required, such as input validation, parsing, or pattern matching.
💡 Syntax
The syntax for the _.startsWith()
method is straightforward:
_.startsWith(string, target, [position=0])
- string: The string to inspect.
- target: The string prefix to search for.
- position: The position to start searching within string.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.startsWith()
method:
const _ = require('lodash');
const str = 'Hello, world!';
const prefix = 'Hello';
const startsWithPrefix = _.startsWith(str, prefix);
console.log(startsWithPrefix);
// Output: true
In this example, _.startsWith()
is used to determine whether the string str starts with the prefix prefix, resulting in true.
🏆 Best Practices
When working with the _.startsWith()
method, consider the following best practices:
Specify Position Parameter:
Utilize the position parameter to specify the position within the string to start searching for the prefix. This allows for more granular control over the search operation.
example.jsCopiedconst str = 'Hello, world!'; const prefix = 'world'; const startsWithPrefix = _.startsWith(str, prefix, 7); // Start searching from position 7 console.log(startsWithPrefix); // Output: true
Handle Case Sensitivity:
Consider the case sensitivity of the comparison based on your specific use case. Use appropriate methods to handle case sensitivity or insensitivity as required.
example.jsCopiedconst str = 'Hello, World!'; const prefix = 'hello'; const startsWithPrefix = _.startsWith(_.toLower(str), _.toLower(prefix)); console.log(startsWithPrefix); // Output: true
Validate Inputs:
Before using
_.startsWith()
, ensure that the input string and prefix are valid and of the expected data type. Implement appropriate error handling to address invalid inputs.example.jsCopiedconst validateInput = (str, prefix) => { if(typeof str !== 'string' || typeof prefix !== 'string') { throw new Error('Invalid input types. Expected strings.'); } }; const str = 'Hello, World!'; const prefix = 'Hello'; validateInput(str, prefix); const startsWithPrefix = _.startsWith(str, prefix); console.log(startsWithPrefix);
📚 Use Cases
Input Validation:
_.startsWith()
can be used for input validation to ensure that user input begins with an expected prefix, such as a URL protocol or a specific format identifier.example.jsCopiedconst validateUrl = (url) => { if(!_.startsWith(url, 'http://') && !_.startsWith(url, 'https://')) { throw new Error('Invalid URL. URL must start with "http://" or "https://".'); } }; const userInputUrl = 'https://example.com'; validateUrl(userInputUrl); console.log('Valid URL:', userInputUrl);
Pattern Matching:
When dealing with textual data,
_.startsWith()
can be utilized for pattern matching to identify strings that begin with specific sequences or prefixes.example.jsCopiedconst files = ['app.js', 'index.html', 'styles.css']; const jsFiles = files.filter(file => _.startsWith(file, 'app')); console.log('JavaScript files:', jsFiles); // Output: ['app.js']
String Parsing:
In parsing tasks, such as extracting substrings or analyzing textual data,
_.startsWith()
can help identify and process strings based on their initial segments.example.jsCopiedconst rawData = 'Temperature: 25°C, Humidity: 60%'; const isTemperatureData = _.startsWith(rawData, 'Temperature'); console.log('Is temperature data:', isTemperatureData); // Output: true
🎉 Conclusion
The _.startsWith()
method in Lodash offers a convenient and efficient solution for determining whether a string starts with a specified prefix. Whether you're performing input validation, pattern matching, or string parsing, this method provides a reliable tool for handling string comparisons in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.startsWith()
method in your Lodash projects.
👨💻 Join our Community:
Author
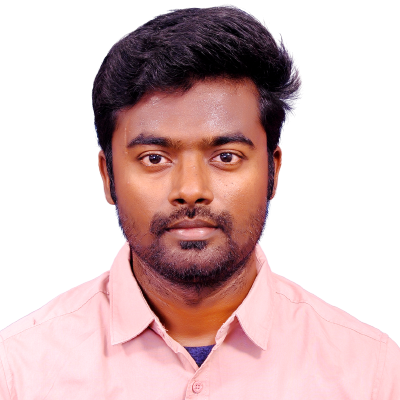
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
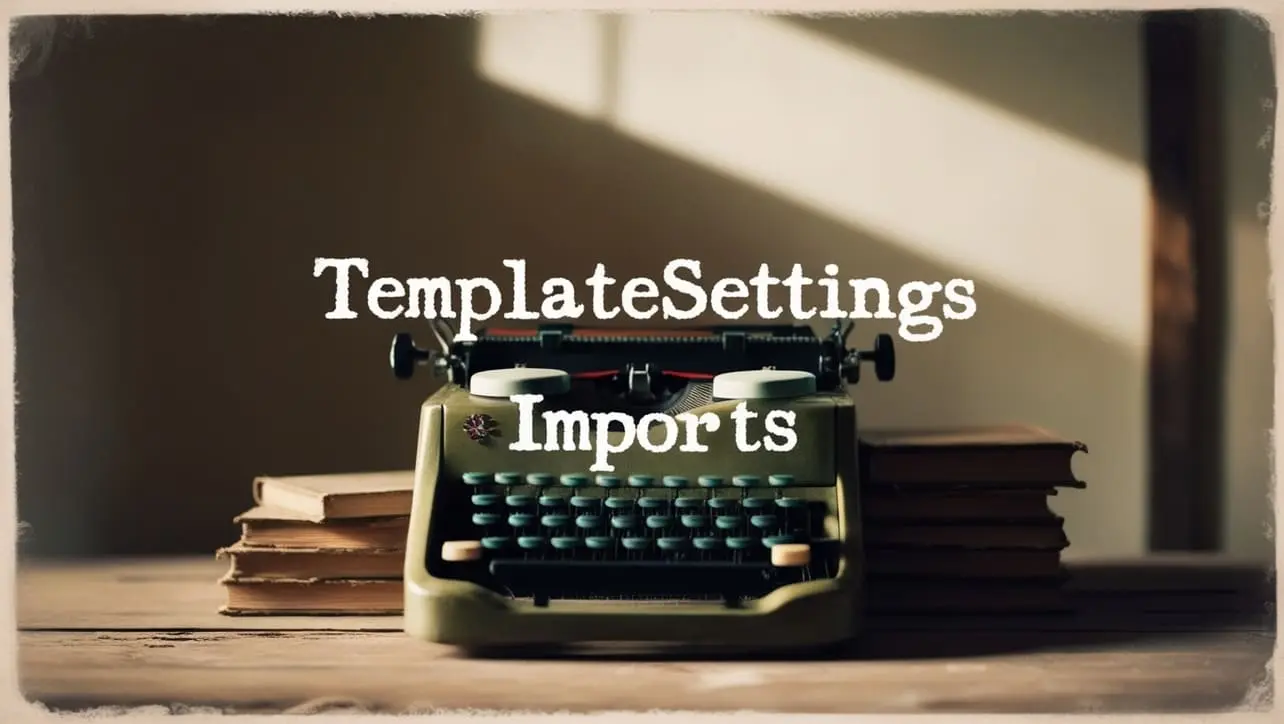
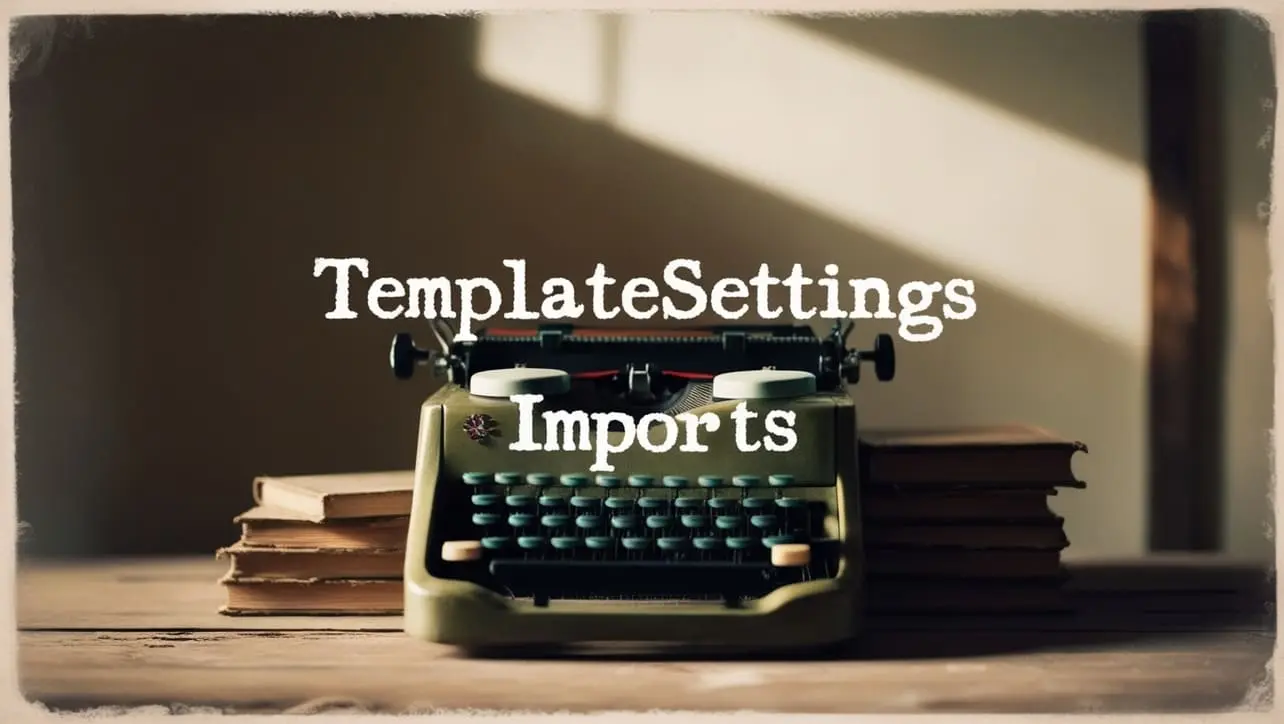
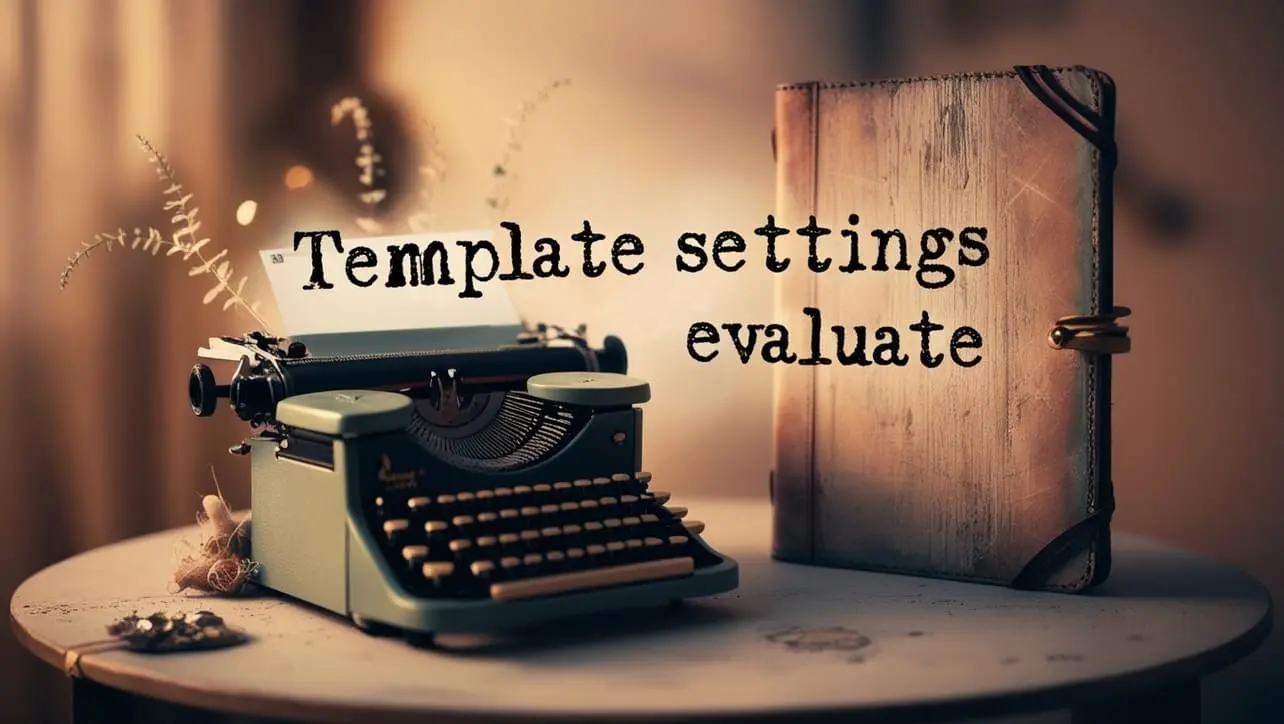
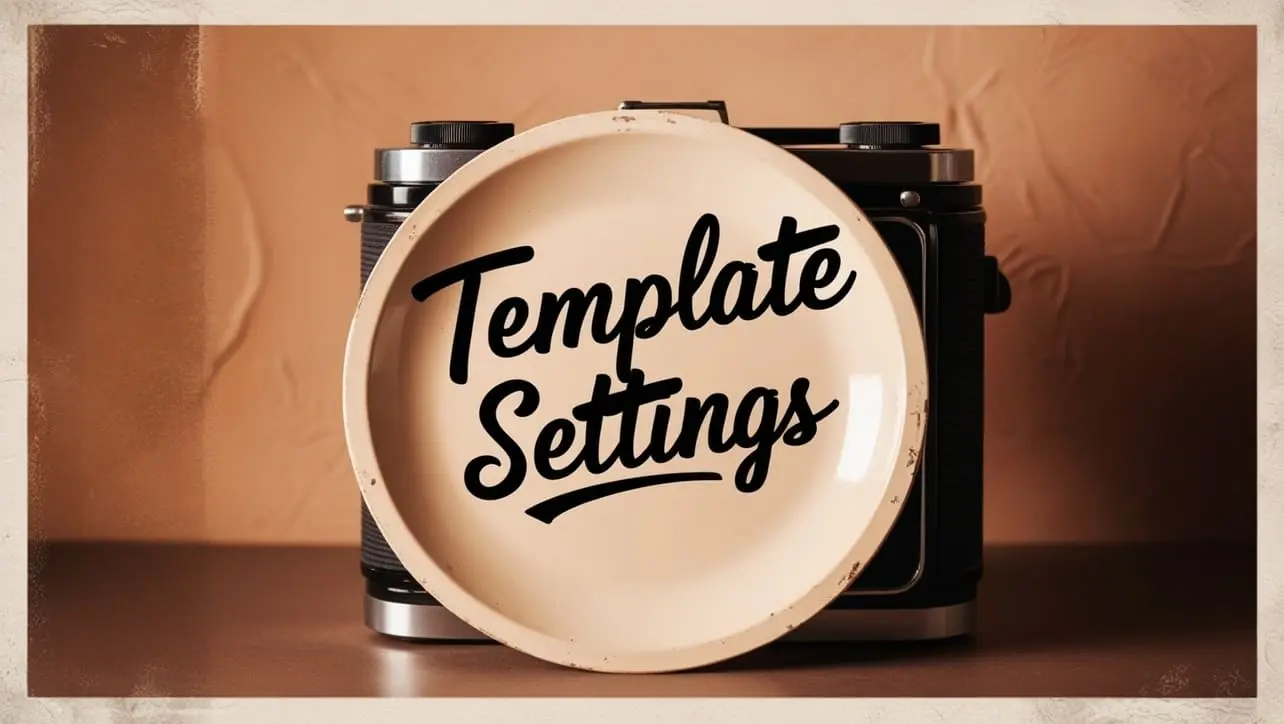
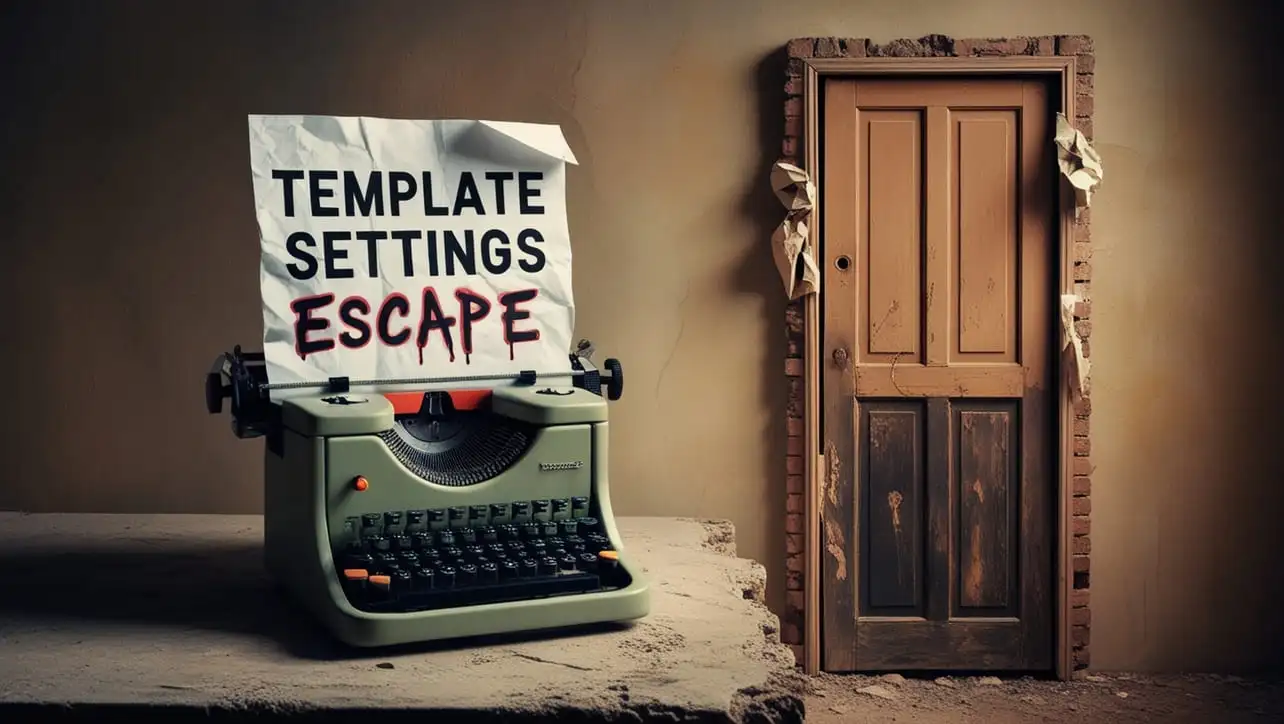
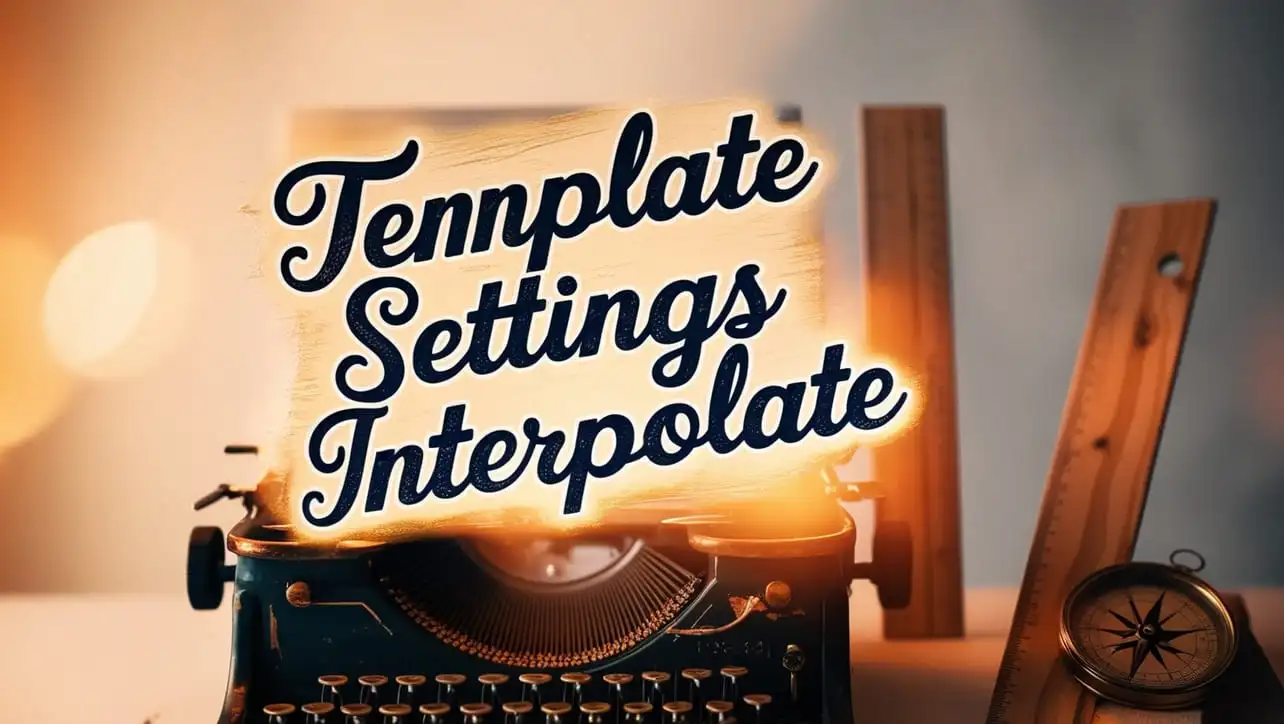
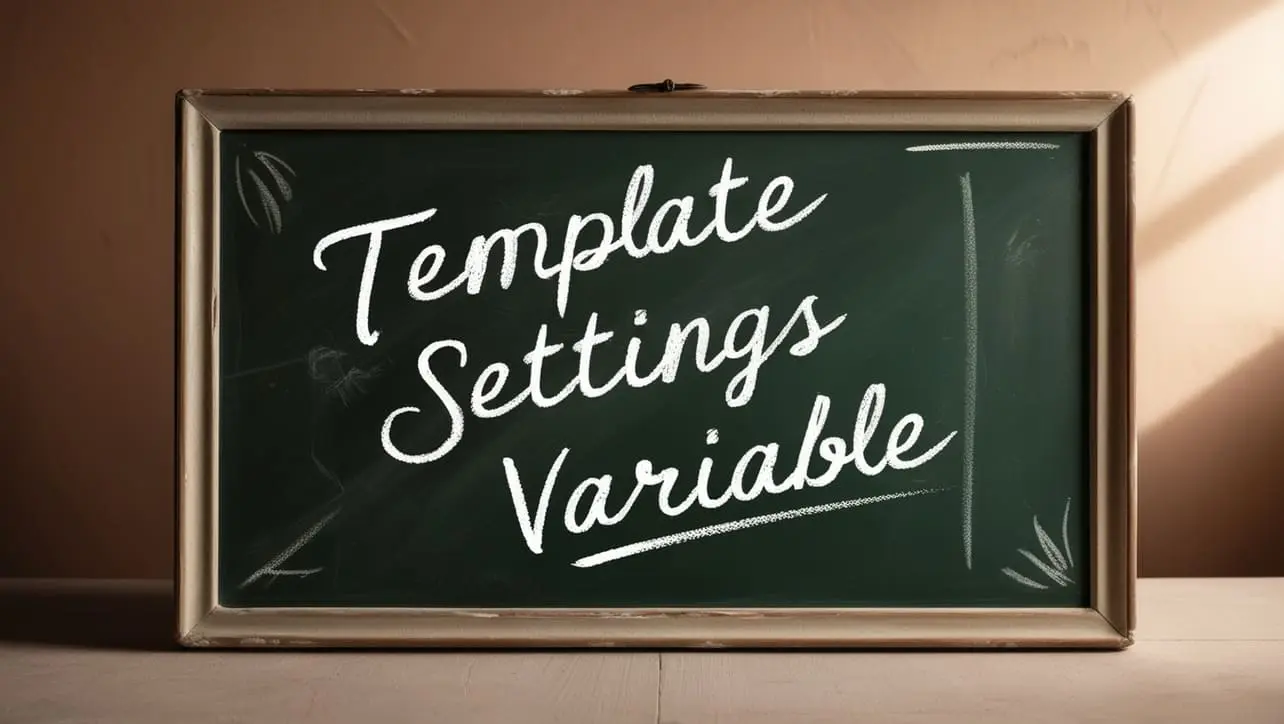
If you have any doubts regarding this article (Lodash _.startsWith() String Method), please comment here. I will help you immediately.