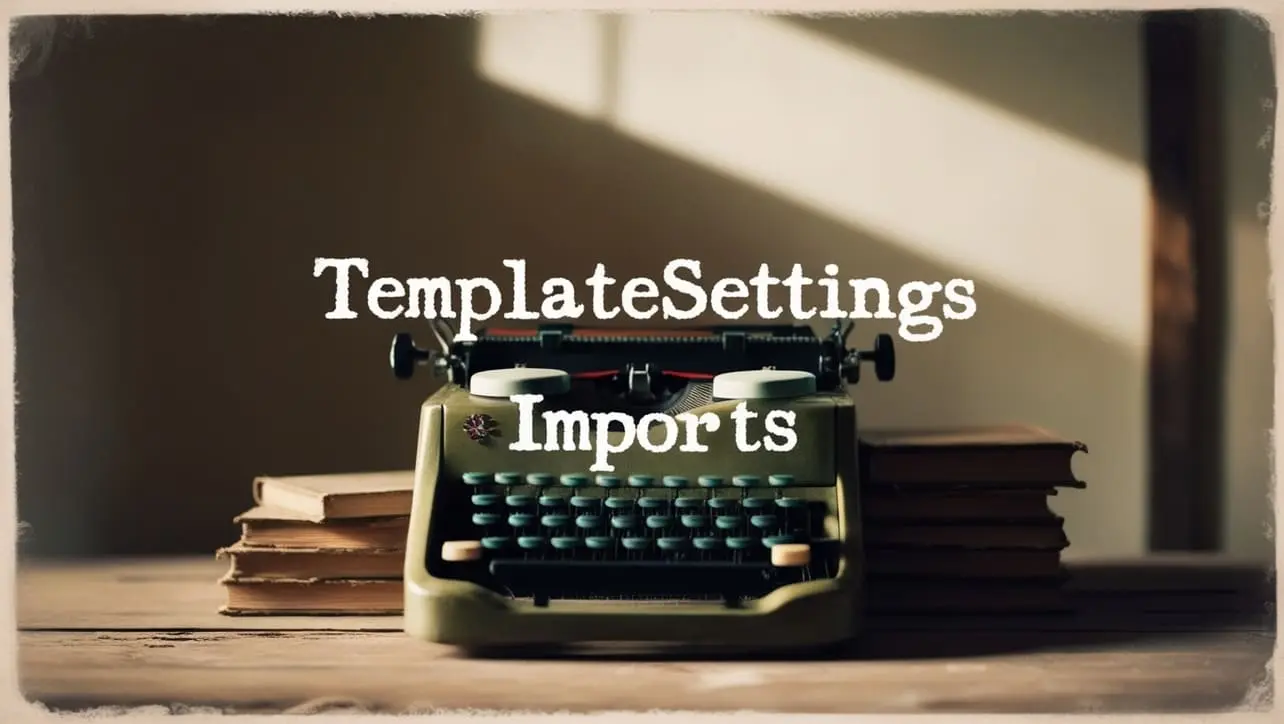
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- _.camelCase
- _.capitalize
- _.deburr
- _.endsWith
- _.escape
- _.escapeRegExp
- _.kebabCase
- _.lowerCase
- _.lowerFirst
- _.pad
- _.padEnd
- _.padStart
- _.parseInt
- _.repeat
- _.replace
- _.snakeCase
- _.split
- _.startCase
- _.startsWith
- _.template
- _.toLower
- _.toUpper
- _.trim
- _.trimEnd
- _.trimStart
- _.truncate
- _.unescape
- _.upperCase
- _.upperFirst
- _.words
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.split() String Method
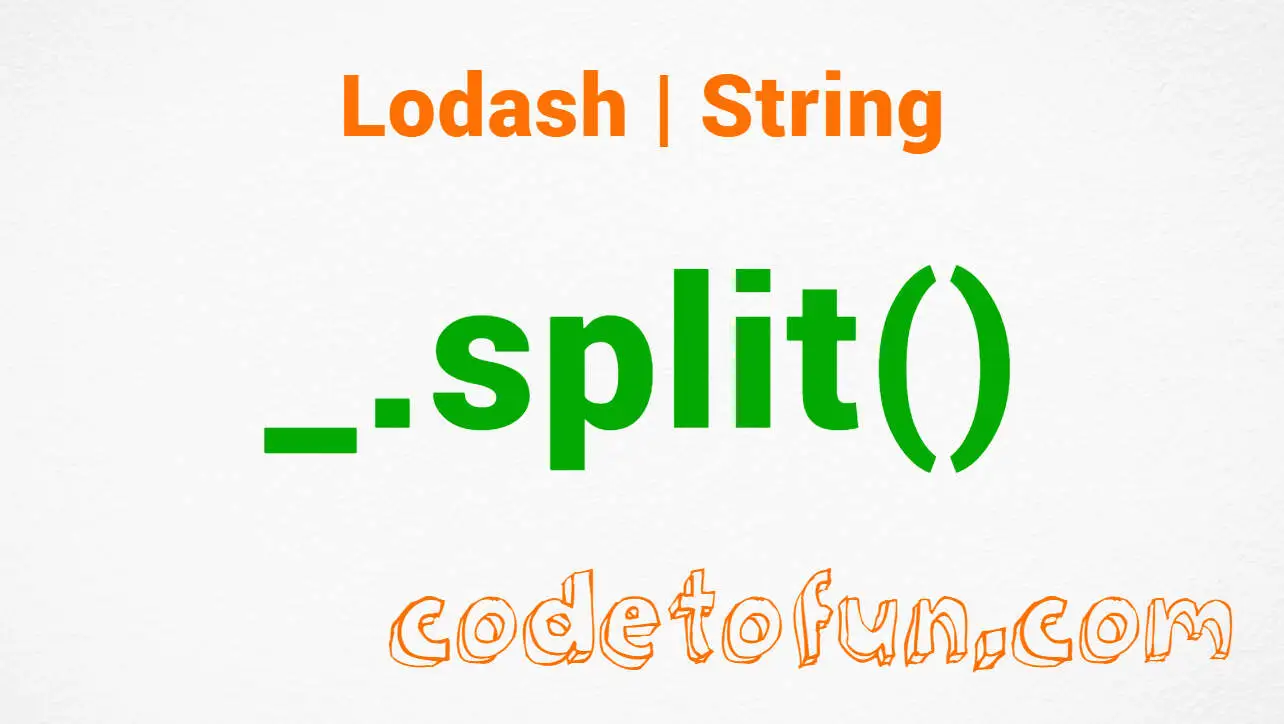
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript, manipulating strings is a common task, and having efficient tools can significantly improve development productivity. Lodash, a popular utility library, offers a wide range of functions to simplify string manipulation tasks. One such function is _.split()
, which provides a convenient way to split a string into an array of substrings based on a specified separator.
Understanding and utilizing this method can streamline string processing tasks in your JavaScript projects.
🧠 Understanding _.split() Method
The _.split()
method in Lodash is used to split a string into an array of substrings based on a specified separator. This method provides flexibility and ease of use, allowing developers to handle various string splitting scenarios with ease.
💡 Syntax
The syntax for the _.split()
method is straightforward:
_.split(string, separator, [limit])
- string: The string to split.
- separator: The separator used to determine where to split the string.
- limit (Optional): An integer specifying a limit on the number of splits to be performed.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.split()
method:
const _ = require('lodash');
const originalString = 'apple,banana,orange';
const splitArray = _.split(originalString, ',');
console.log(splitArray);
// Output: ['apple', 'banana', 'orange']
In this example, the originalString is split into an array of substrings using the comma , as the separator.
🏆 Best Practices
When working with the _.split()
method, consider the following best practices:
Handle Empty Strings:
Ensure your code gracefully handles cases where the input string is empty. Consider providing default values or implementing error handling logic to handle such scenarios.
example.jsCopiedconst emptyString = ''; const defaultSplit = _.split(emptyString, ',', 1); console.log(defaultSplit); // Output: ['']
Trim Whitespace:
When splitting strings based on a delimiter, consider trimming whitespace from the resulting substrings to ensure consistency and avoid unintended behavior.
example.jsCopiedconst stringWithWhitespace = 'apple, banana, orange'; const trimmedSplit = _.map(_.split(stringWithWhitespace, ','), _.trim); console.log(trimmedSplit); // Output: ['apple', 'banana', 'orange']
Specify Split Limit:
To control the number of splits performed, utilize the optional limit parameter. This can be useful when dealing with large strings or when you only need a certain number of splits.
example.jsCopiedconst longString = 'apple,banana,orange,grape,mango'; const limitedSplit = _.split(longString, ',', 3); console.log(limitedSplit); // Output: ['apple', 'banana', 'orange,grape,mango']
📚 Use Cases
Parsing CSV Data:
When working with comma-separated values (CSV) data,
_.split()
can be used to parse each row into an array of values, facilitating data processing and manipulation.example.jsCopiedconst csvData = 'John,Doe,30\nJane,Smith,25\n'; const parsedData = _.map(_.split(csvData, '\n'), row => _.split(row, ',')); console.log(parsedData); // Output: [['John', 'Doe', '30'], ['Jane', 'Smith', '25'], ['']]
Tokenization:
In lexical analysis and parsing tasks, tokenization involves splitting a string into individual tokens.
_.split()
can be a valuable tool in this process, enabling the extraction of tokens based on specific delimiters.example.jsCopiedconst expression = 'x + y - 10 * z'; const tokens = _.split(expression, /[\s+*-]/); console.log(tokens); // Output: ['x', '', 'y', '', '10', '', 'z']
URL Parsing:
When dealing with URLs,
_.split()
can help extract relevant components such as protocol, domain, and path by splitting the URL string based on predefined separators.example.jsCopiedconst url = 'https://www.example.com/path/to/resource'; const urlComponents = _.split(url, /[:/]/); console.log(urlComponents); // Output: ['https', '', 'www.example.com', 'path', 'to', 'resource']
🎉 Conclusion
The _.split()
method in Lodash provides a versatile solution for splitting strings into substrings based on specified delimiters. Whether you're parsing CSV data, tokenizing strings, or extracting components from URLs, _.split()
offers the flexibility and convenience needed to handle various string manipulation tasks effectively.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.split()
method in your Lodash projects.
👨💻 Join our Community:
Author
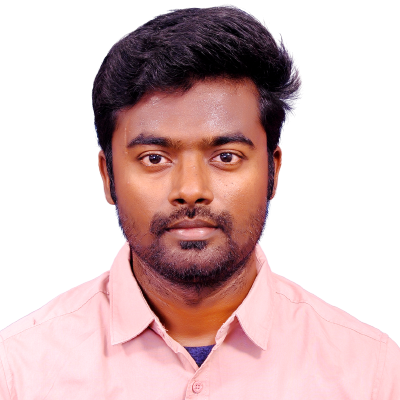
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
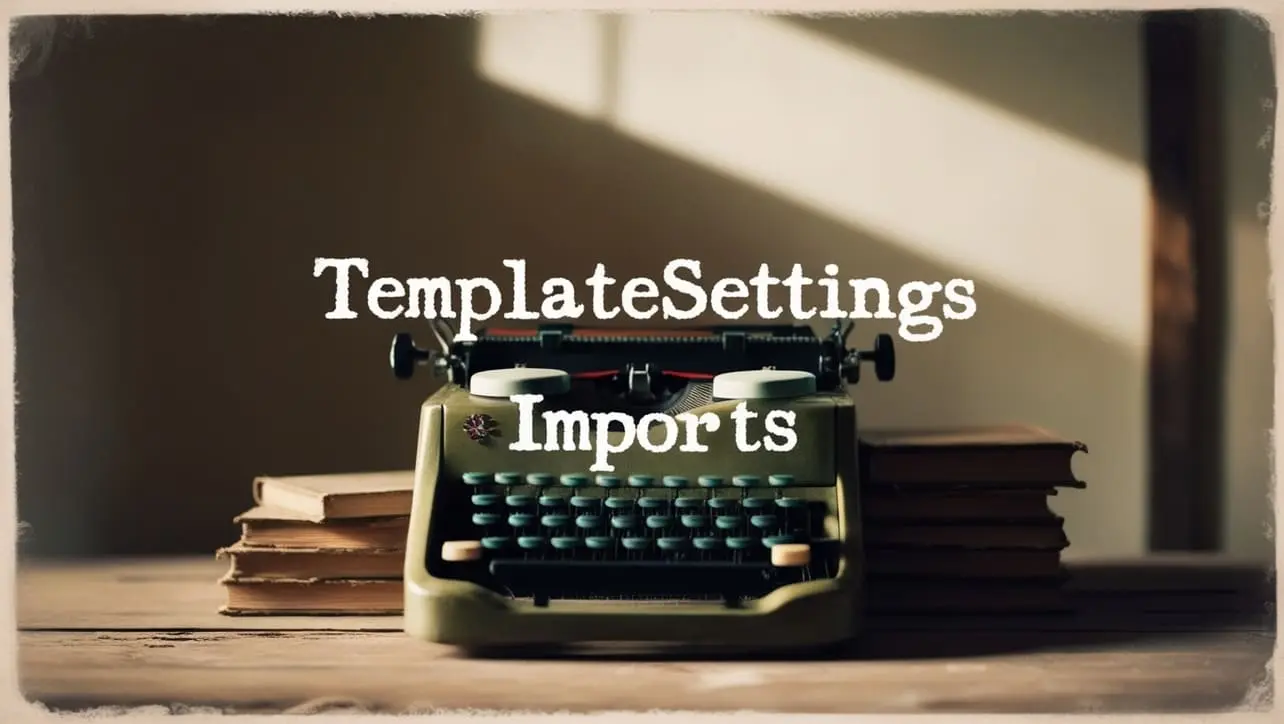
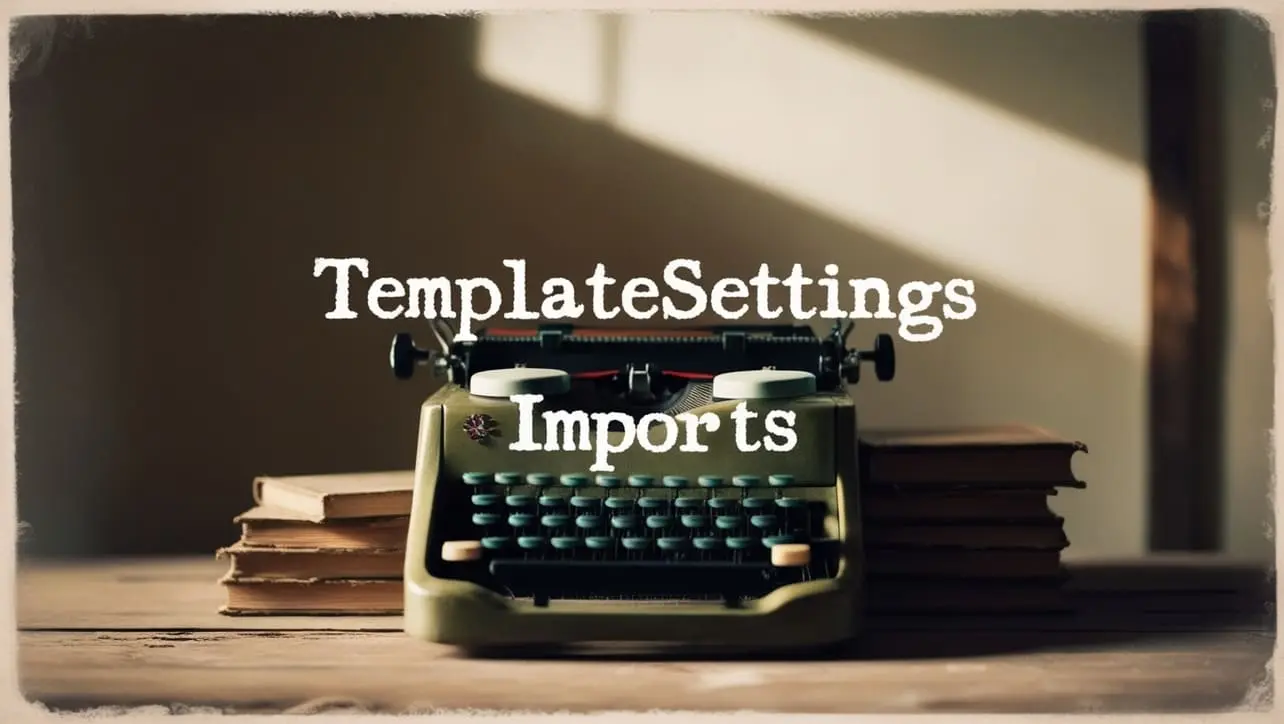
Lodash _.templateSettings.imports Property
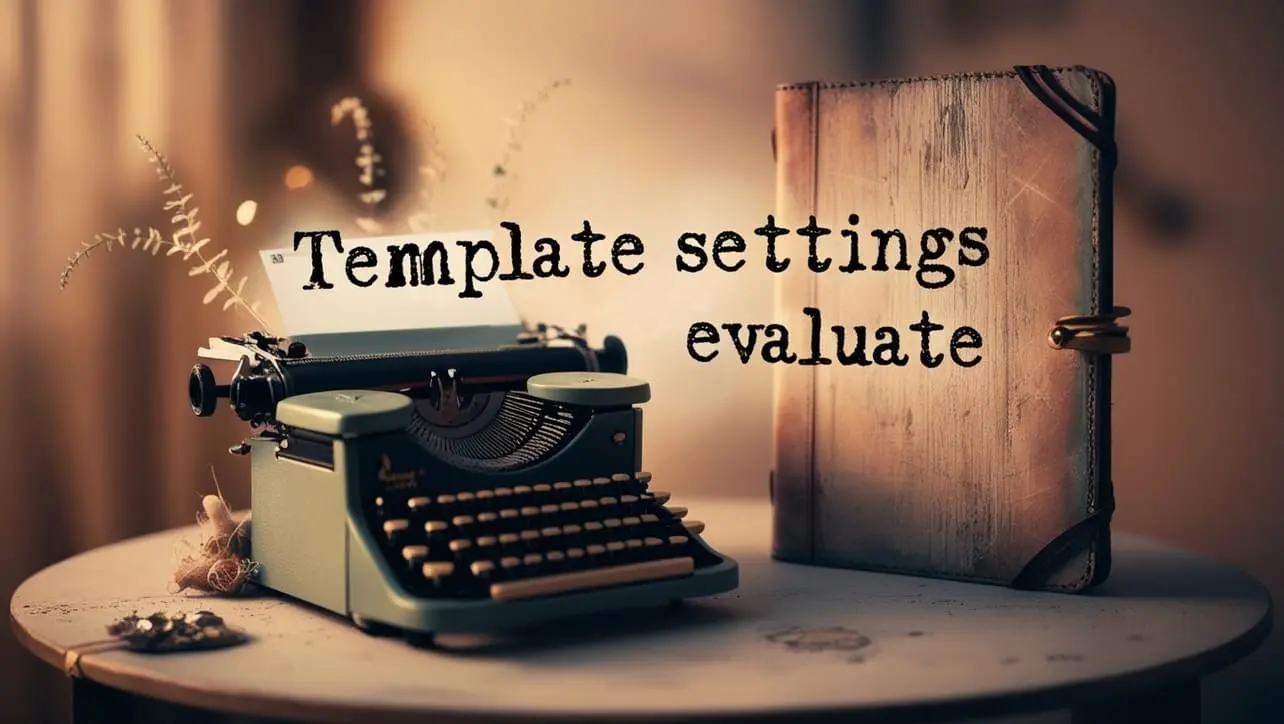
Lodash _.templateSettings.evaluate Property
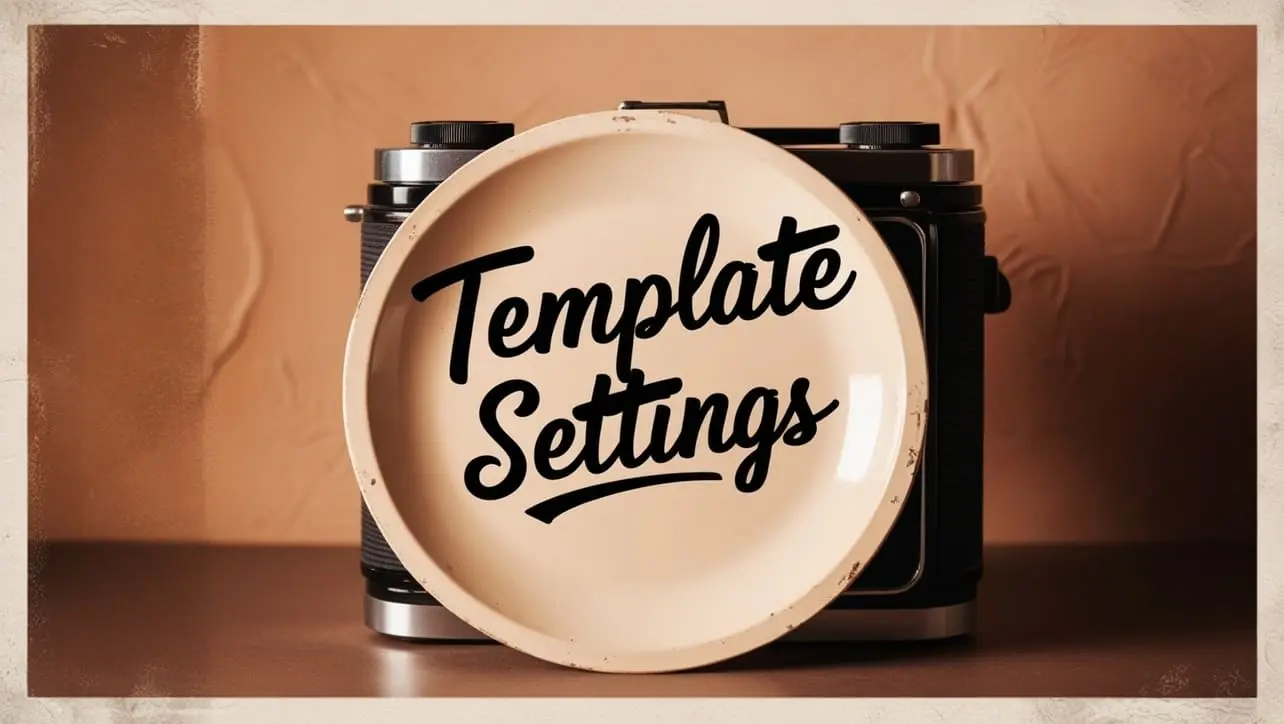
Lodash _.templateSettings Property
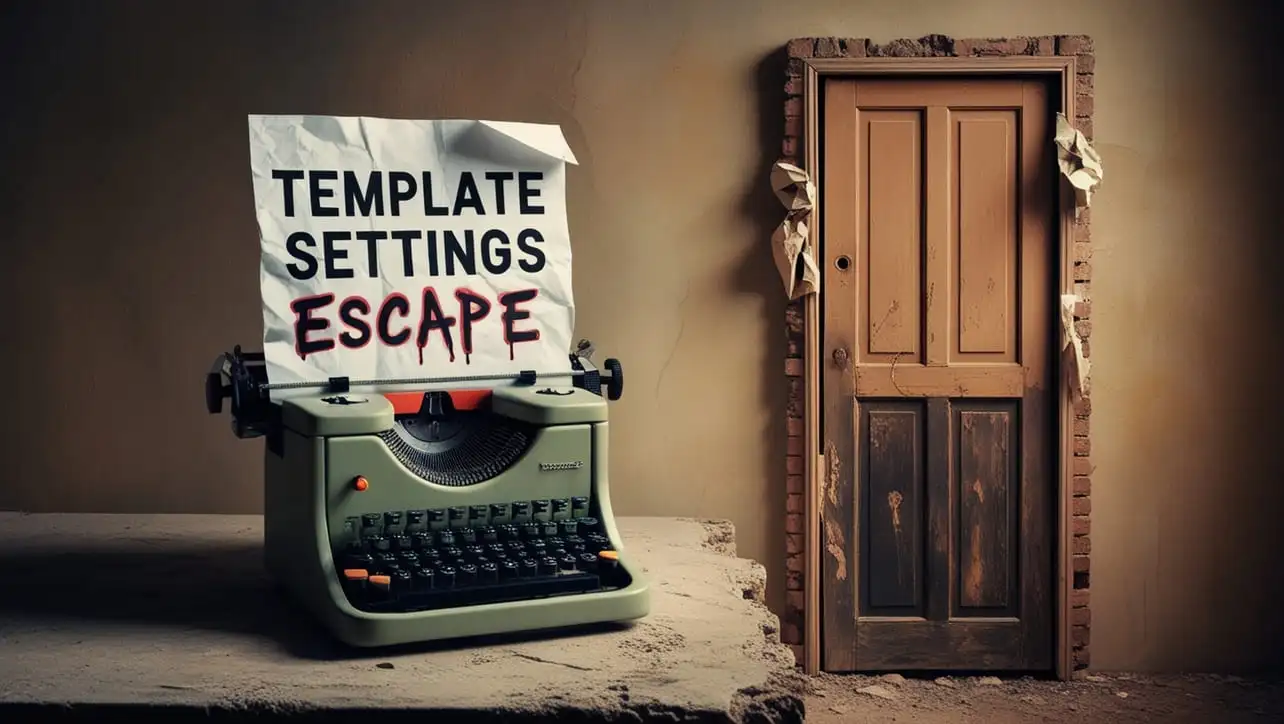
Lodash _.templateSettings.escape Property
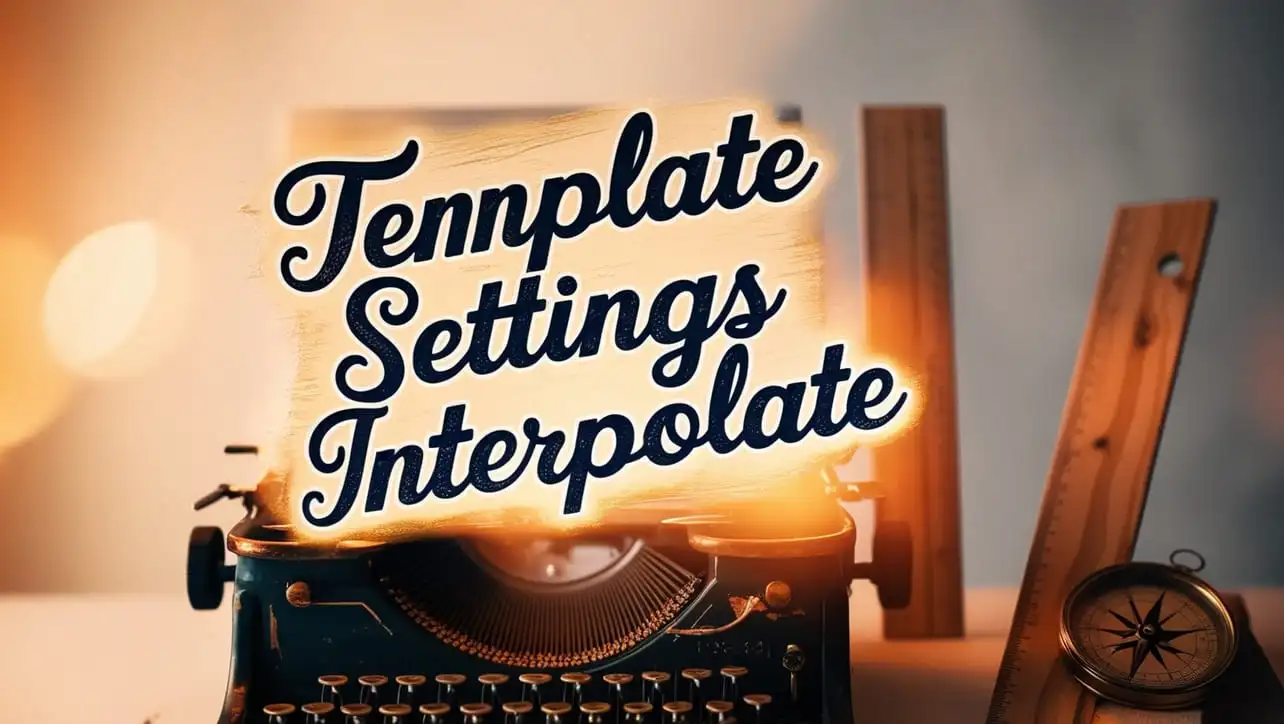
Lodash _.templateSettings.interpolate Property
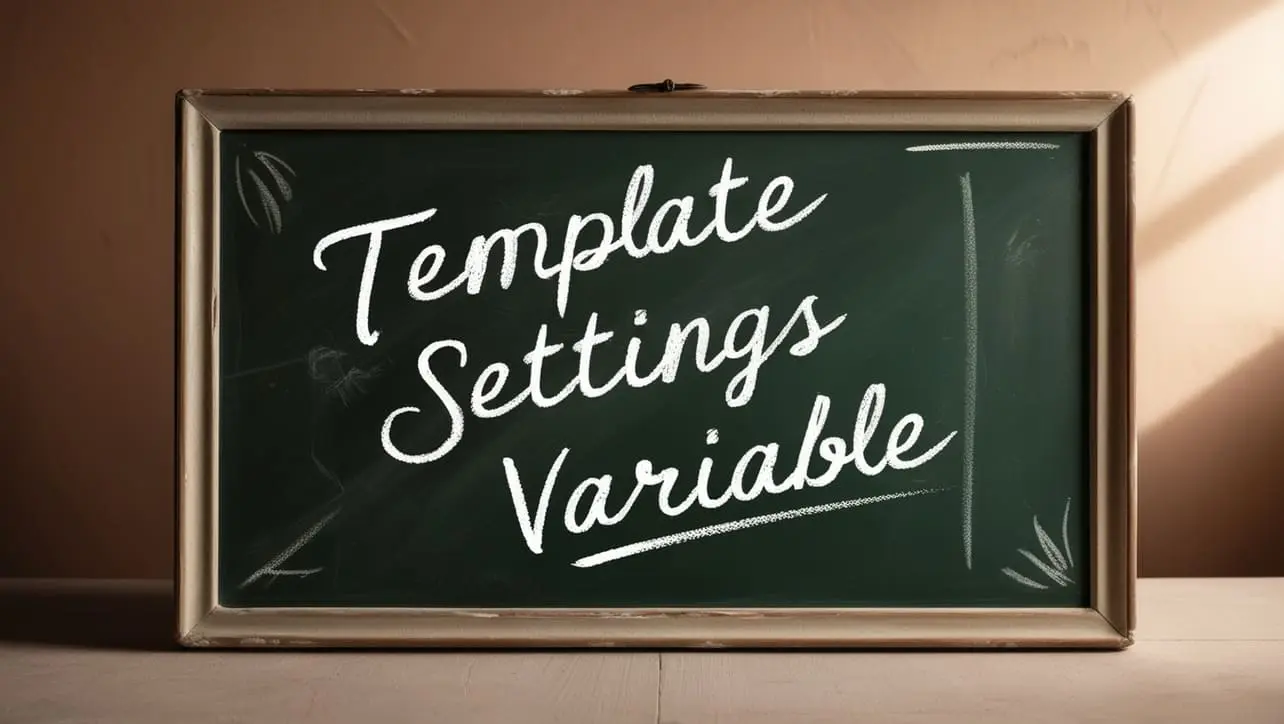
If you have any doubts regarding this article (Lodash _.split() String Method), please comment here. I will help you immediately.