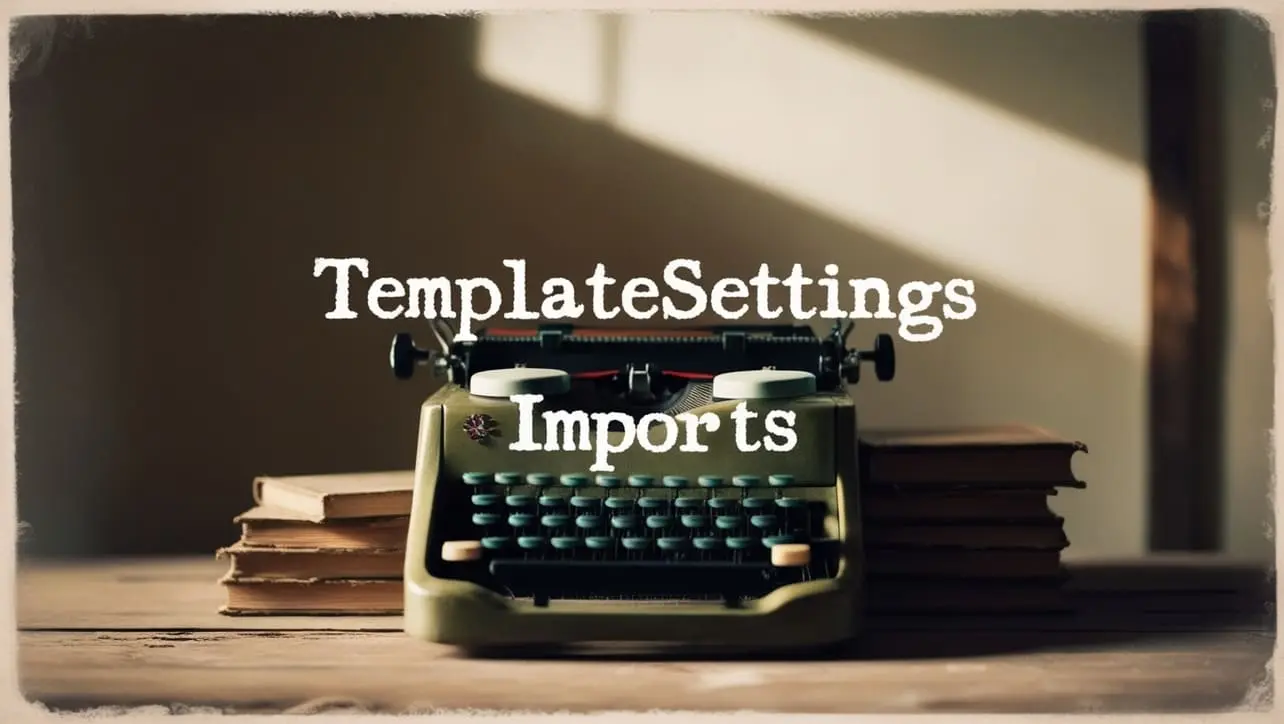
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- _.camelCase
- _.capitalize
- _.deburr
- _.endsWith
- _.escape
- _.escapeRegExp
- _.kebabCase
- _.lowerCase
- _.lowerFirst
- _.pad
- _.padEnd
- _.padStart
- _.parseInt
- _.repeat
- _.replace
- _.snakeCase
- _.split
- _.startCase
- _.startsWith
- _.template
- _.toLower
- _.toUpper
- _.trim
- _.trimEnd
- _.trimStart
- _.truncate
- _.unescape
- _.upperCase
- _.upperFirst
- _.words
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.repeat() String Method
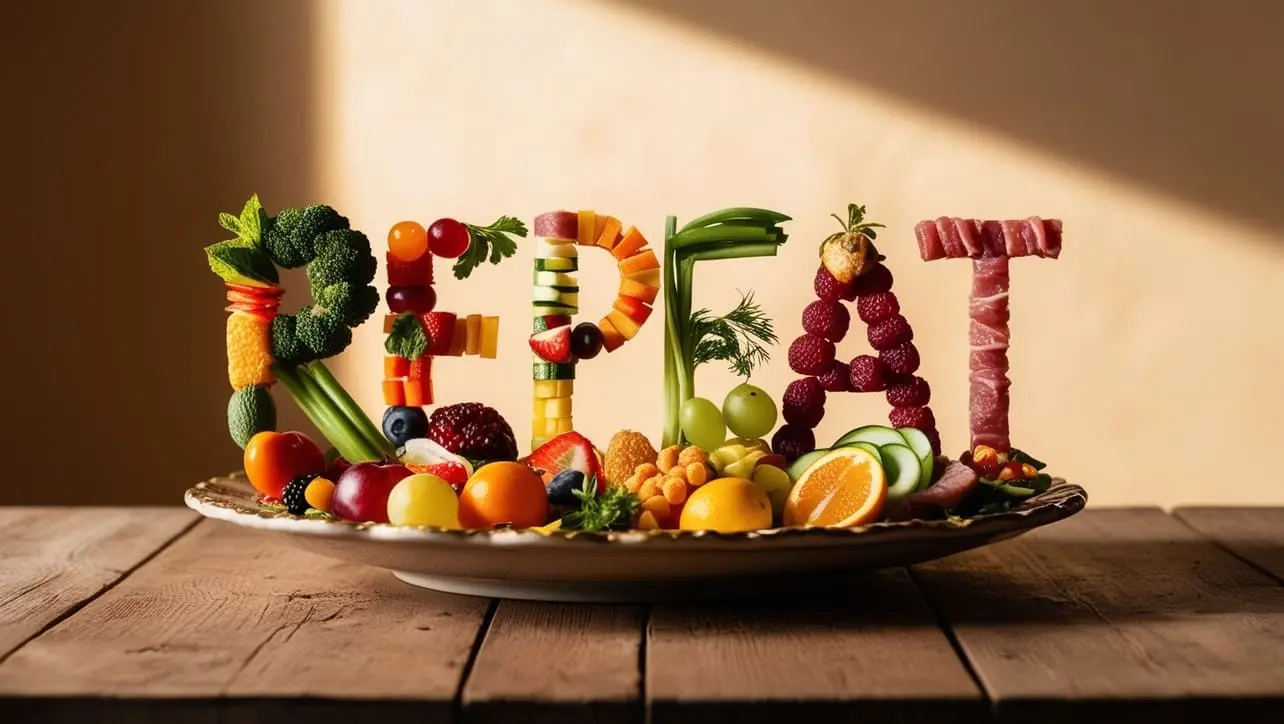
Photo Credit to CodeToFun
🙋 Introduction
String manipulation is a common task in JavaScript development, and the Lodash library provides a variety of utility functions to streamline this process. Among these functions is the _.repeat()
method, which allows developers to generate a new string by repeating a given string a specified number of times.
This method simplifies string generation tasks and enhances code readability, making it a valuable tool for JavaScript developers.
🧠 Understanding _.repeat() Method
The _.repeat()
method in Lodash generates a new string by repeating a specified string a given number of times. This provides a convenient way to create strings with repetitive patterns or sequences, reducing the need for manual string concatenation.
💡 Syntax
The syntax for the _.repeat()
method is straightforward:
_.repeat(string, [n])
- string: The string to repeat.
- n: The number of times to repeat the string.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.repeat()
method:
const _ = require('lodash');
const repeatedString = _.repeat('Hello ', 3);
console.log(repeatedString);
// Output: 'Hello Hello Hello '
In this example, the string Hello is repeated three times using _.repeat()
, resulting in the new string Hello Hello Hello .
🏆 Best Practices
When working with the _.repeat()
method, consider the following best practices:
Validating Inputs:
Before using
_.repeat()
, ensure that the input string is valid and the repetition count is a non-negative integer.example.jsCopiedconst inputString = 'Hello'; const repetitionCount = 5; if (typeof inputString !== 'string' || repetitionCount < 0 || !Number.isInteger(repetitionCount)) { console.error('Invalid input'); return; } const repeatedString = _.repeat(inputString, repetitionCount); console.log(repeatedString);
Handling Edge Cases:
Consider edge cases, such as an empty string or a repetition count of zero. Implement appropriate error handling or default behaviors to handle these scenarios gracefully.
example.jsCopiedconst emptyString = ''; const zeroRepetitions = 0; const result1 = _.repeat(emptyString, 3); // Returns: '' const result2 = _.repeat('Hello', zeroRepetitions); // Returns: '' console.log(result1); console.log(result2);
Performance Optimization:
Optimize performance by minimizing unnecessary repetitions and leveraging the built-in capabilities of
_.repeat()
.example.jsCopiedconst longString = 'A'.repeat(1000); // Using native JavaScript repeat method for performance console.log(longString);
📚 Use Cases
Generating Patterns:
_.repeat()
is useful for generating strings with repetitive patterns or sequences, such as borders, separators, or placeholders.example.jsCopiedconst border = _.repeat('-', 20); console.log(border); // Output: '--------------------'
Formatting Output:
When formatting output,
_.repeat()
can be employed to align or pad strings to achieve a visually appealing presentation.example.jsCopiedconst paddedString = '123'.padStart(10, '0'); console.log(paddedString); // Output: '0000000123'
Template Generation:
In template generation or string interpolation tasks,
_.repeat()
can be used to incorporate repeated elements into the generated strings.example.jsCopiedconst template = _.repeat('<li>Item</li>\n', 5); console.log(`<ul>\n${template}</ul>`);
🎉 Conclusion
The _.repeat()
method in Lodash provides a convenient way to generate strings by repeating a given string a specified number of times. Whether you're generating patterns, formatting output, or creating templates, _.repeat()
simplifies string manipulation tasks and enhances code clarity.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.repeat()
method in your Lodash projects.
👨💻 Join our Community:
Author
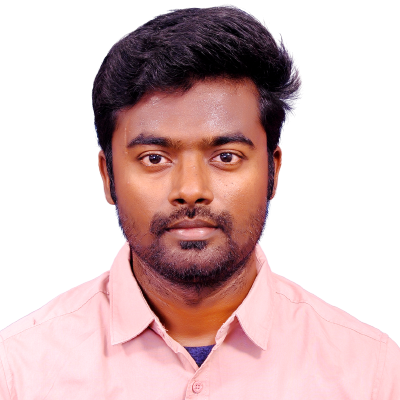
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
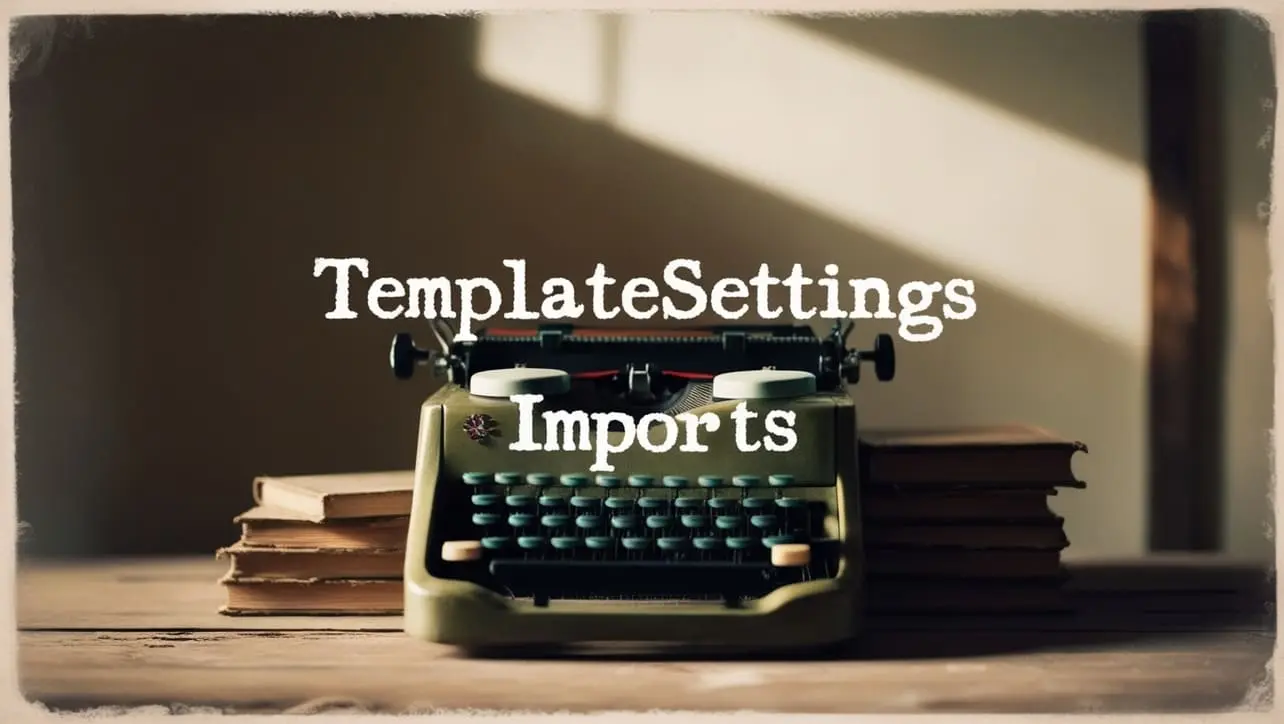
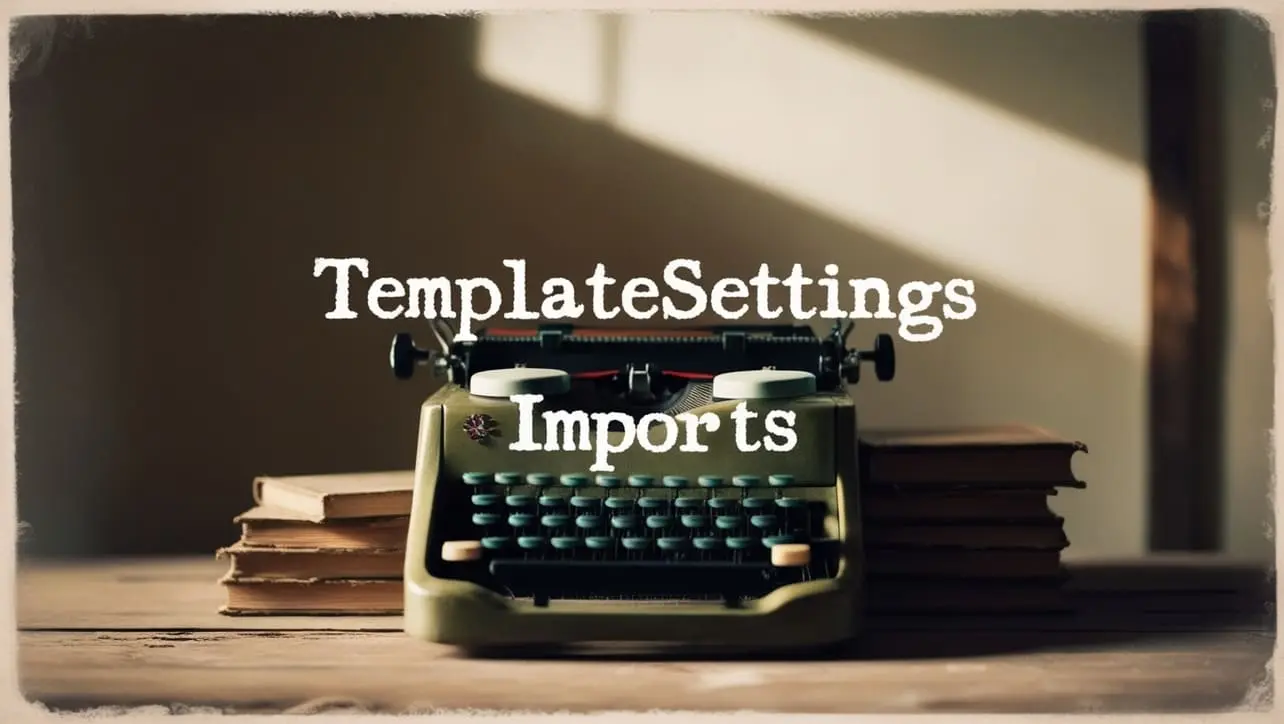
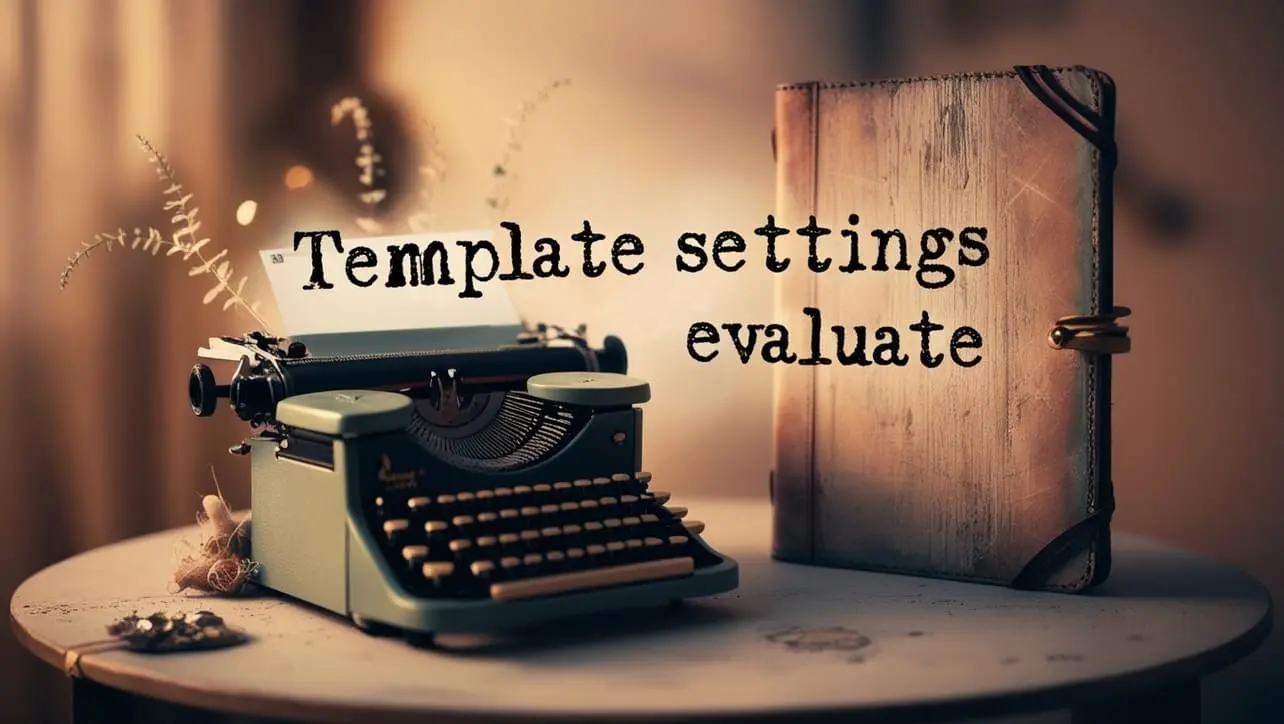
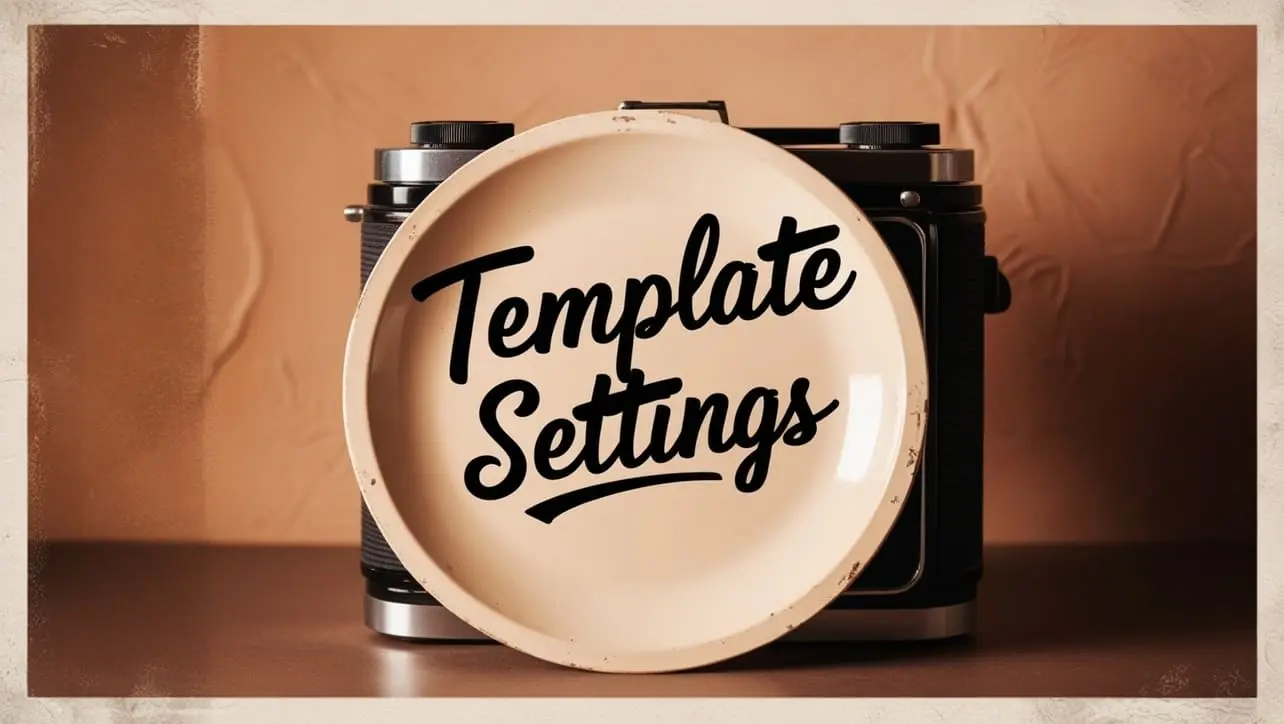
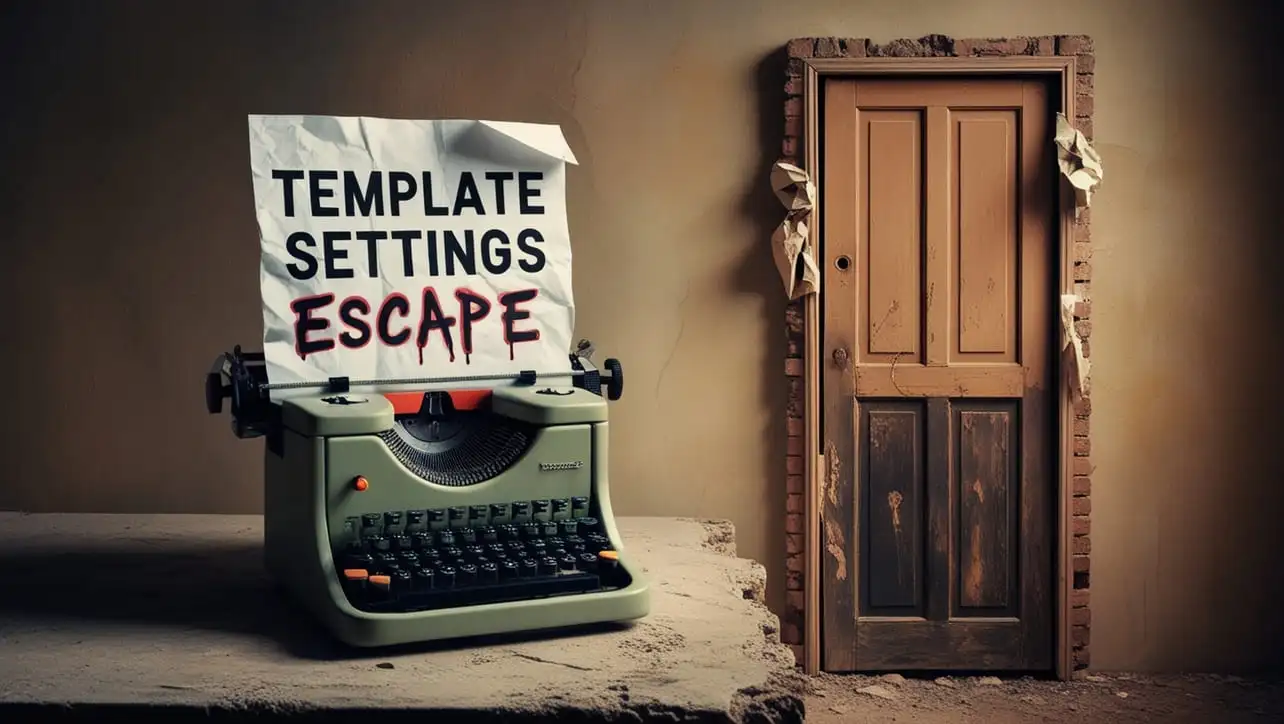
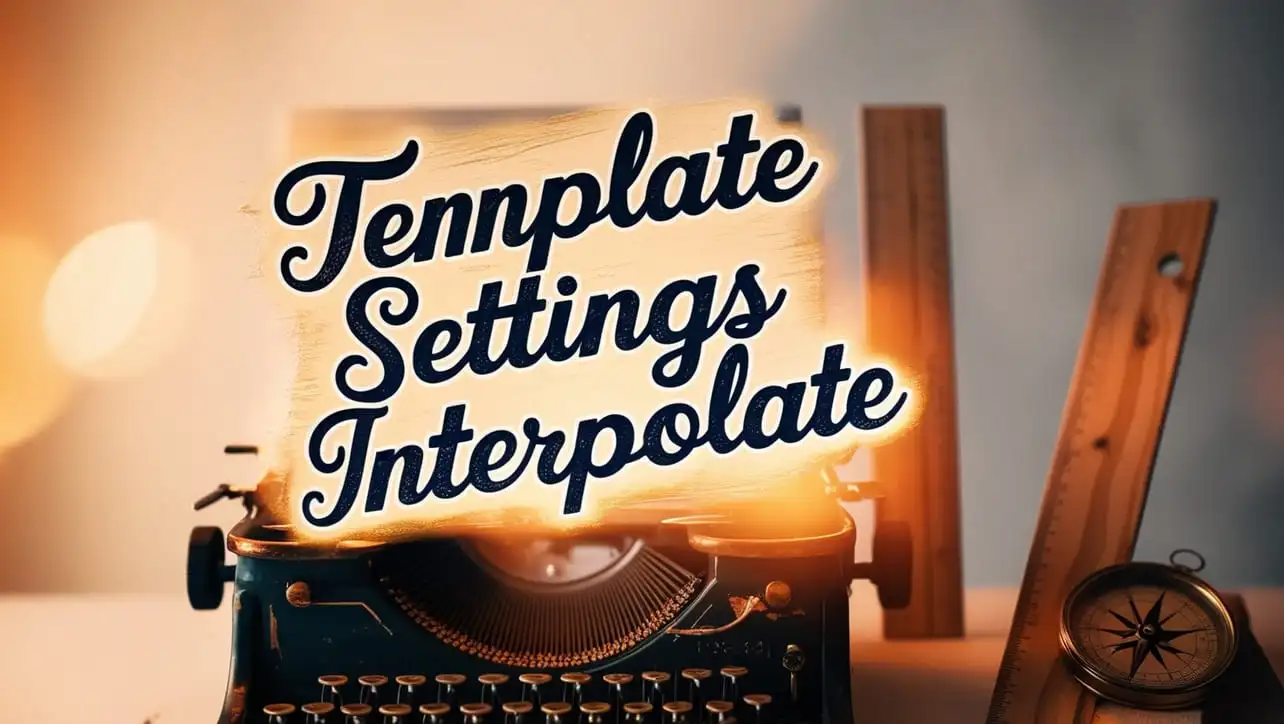
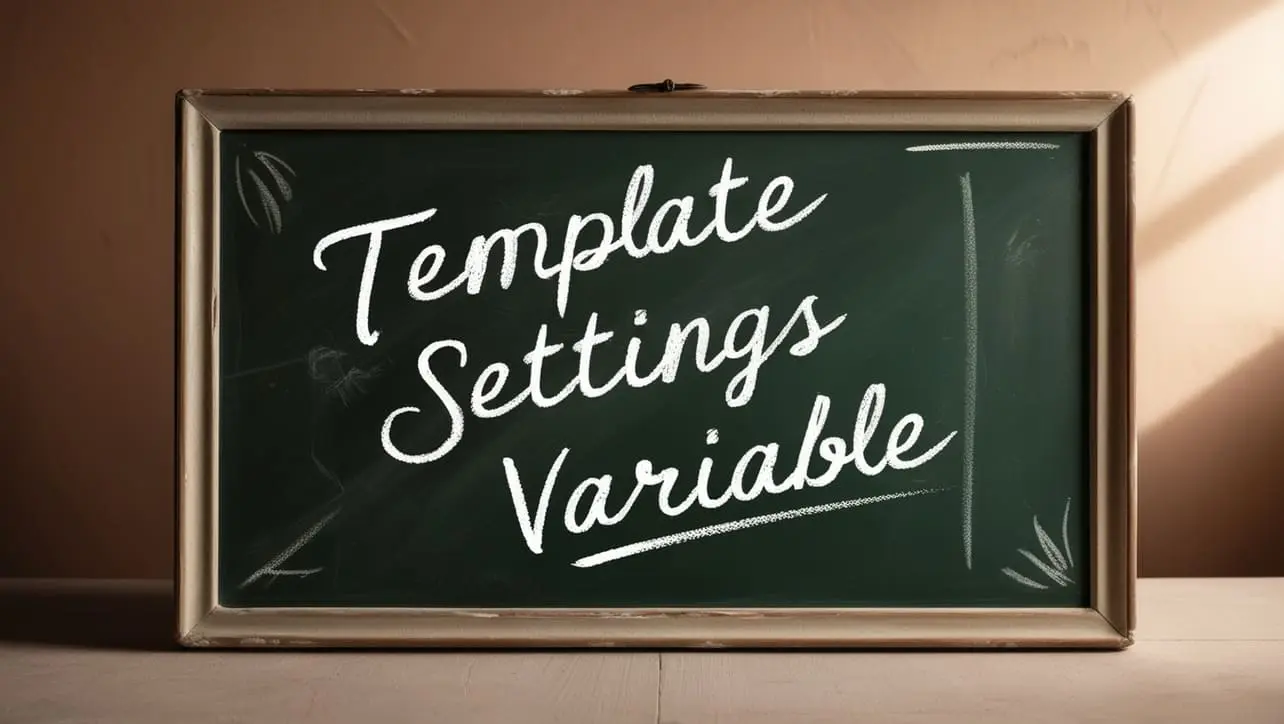
If you have any doubts regarding this article (Lodash _.repeat() String Method), please comment here. I will help you immediately.