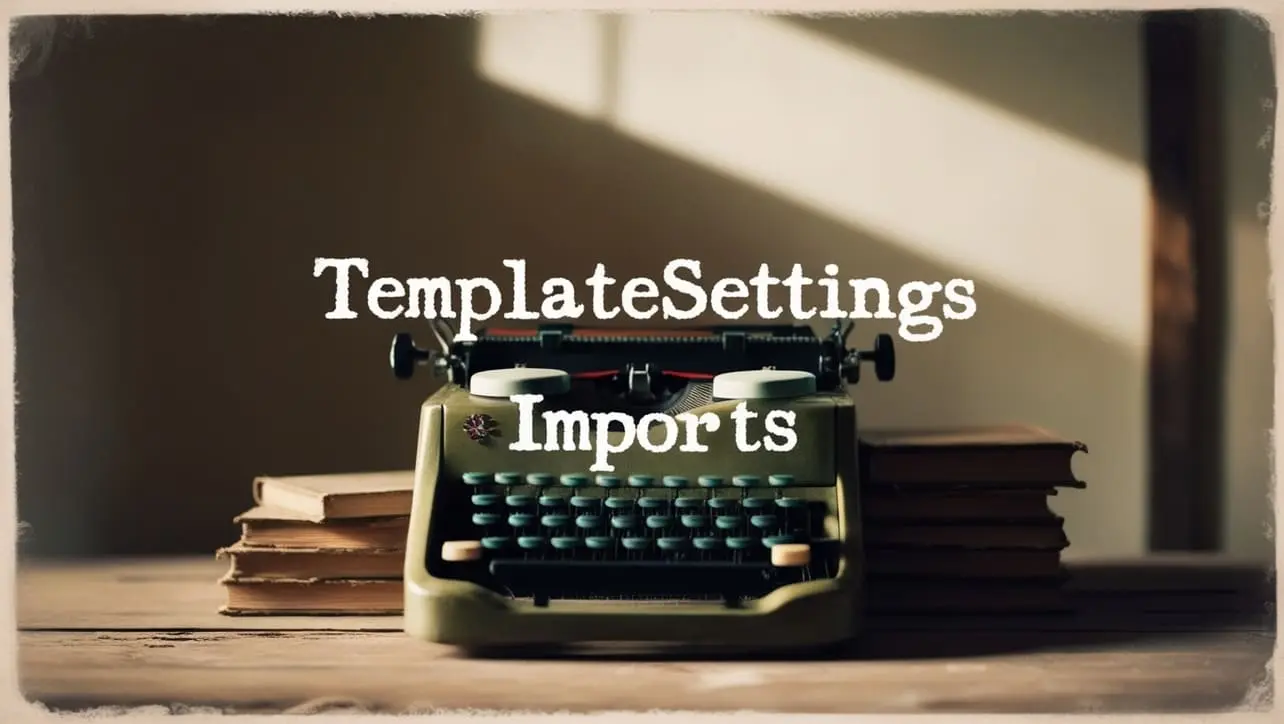
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.valuesIn() Object Method
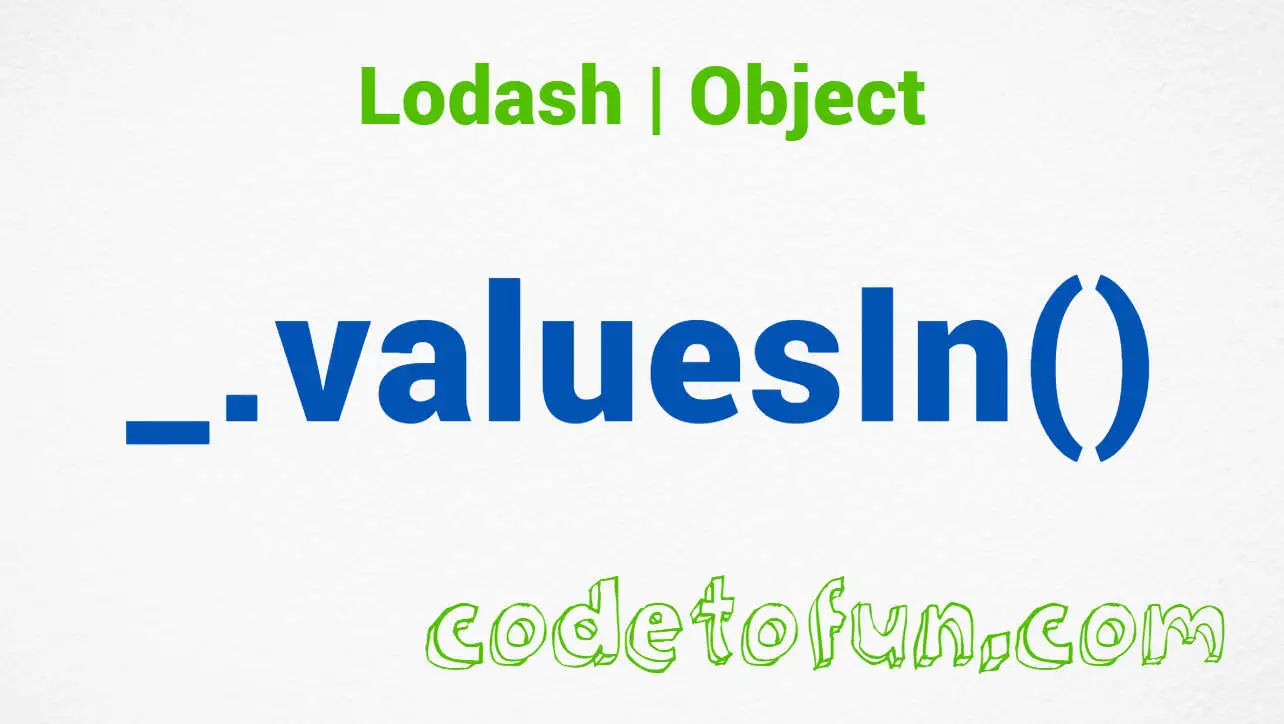
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript programming, effective manipulation of objects is essential for building robust applications. Lodash, a popular utility library, offers a plethora of functions to streamline object handling tasks. Among these functions is the _.valuesIn()
method, which provides a convenient way to extract the property values of an object, including inherited ones.
This method enhances code clarity and simplifies data extraction, making it a valuable tool for JavaScript developers.
🧠 Understanding _.valuesIn() Method
The _.valuesIn()
method in Lodash retrieves the values of an object's own and inherited properties. Unlike the native Object.values() method, _.valuesIn()
includes properties from the object's prototype chain. This comprehensive approach to value extraction can be particularly useful when dealing with complex object structures or when you need to access inherited properties.
💡 Syntax
The syntax for the _.valuesIn()
method is straightforward:
_.valuesIn(object)
- object: The object whose values are to be retrieved.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.valuesIn()
method:
const _ = require('lodash');
function Person(name, age) {
this.name = name;
this.age = age;
}
Person.prototype.sayHello = function() {
console.log(`Hello, my name is ${this.name}`);
};
const john = new Person('John', 30);
const values = _.valuesIn(john);
console.log(values);
// Output: ['John', 30, function() { console.log(`Hello, my name is ${this.name}`); }]
In this example, the _.valuesIn()
method is used to extract the values of the john object, including its own properties (name, age) and the inherited method (sayHello).
🏆 Best Practices
When working with the _.valuesIn()
method, consider the following best practices:
Handle Nested Objects:
When working with nested objects, ensure that
_.valuesIn()
is applied recursively to extract values from all levels of the object hierarchy.example.jsCopiedconst nestedObject = { a: 1, b: { c: 2, d: { e: 3 } } }; const allValues = _.flattenDeep(_.valuesIn(nestedObject)); console.log(allValues); // Output: [1, 2, 3]
Avoid Circular References:
Be cautious when using
_.valuesIn()
with objects containing circular references, as it may lead to infinite recursion. Implement appropriate checks or use alternative approaches to handle such scenarios.example.jsCopiedconst circularObject = { a: 1 }; circularObject.b = circularObject; const valuesWithoutCircularRefs = _.values(_.omitBy(circularObject, _.isCircular)); console.log(valuesWithoutCircularRefs); // Output: [1]
Performance Considerations:
Consider the performance implications of using
_.valuesIn()
on large objects or in performance-sensitive code. Evaluate alternatives or optimize the usage based on the specific requirements of your application.example.jsCopiedconst largeObject = /* ...large object data... */; const values = _.valuesIn(largeObject); console.log(values);
📚 Use Cases
Serialization:
_.valuesIn()
can be utilized to serialize an object's properties, including inherited ones, for storage or transmission purposes.example.jsCopiedconst user = { name: 'Alice', age: 25 }; const serializedData = JSON.stringify(_.valuesIn(user)); console.log(serializedData);
Object Inspection:
When inspecting or debugging objects,
_.valuesIn()
provides a comprehensive view of an object's properties, facilitating easier analysis and troubleshooting.example.jsCopiedconst objectToInspect = /* ...object data... */; const allPropertyValues = _.valuesIn(objectToInspect); console.log(allPropertyValues);
Inheritance Exploration:
By including inherited properties,
_.valuesIn()
enables exploration of an object's inheritance chain, allowing developers to gain insights into its structure and behavior.example.jsCopiedfunction Animal(species) { this.species = species; } Animal.prototype.makeSound = function() { console.log('Animal sound'); }; function Dog(breed) { Animal.call(this, 'Canine'); this.breed = breed; } Dog.prototype = Object.create(Animal.prototype); Dog.prototype.constructor = Dog; const myDog = new Dog('Labrador'); const allValues = _.valuesIn(myDog); console.log(allValues);
🎉 Conclusion
The _.valuesIn()
method in Lodash provides a convenient way to extract the values of an object, including its inherited properties. Whether you're serializing data, inspecting objects, or exploring inheritance chains, _.valuesIn()
offers versatility and simplicity in object manipulation tasks.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.valuesIn()
method in your Lodash projects.
👨💻 Join our Community:
Author
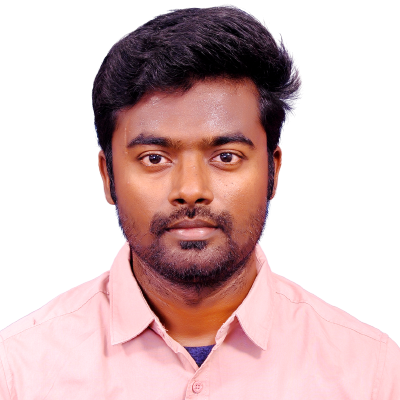
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
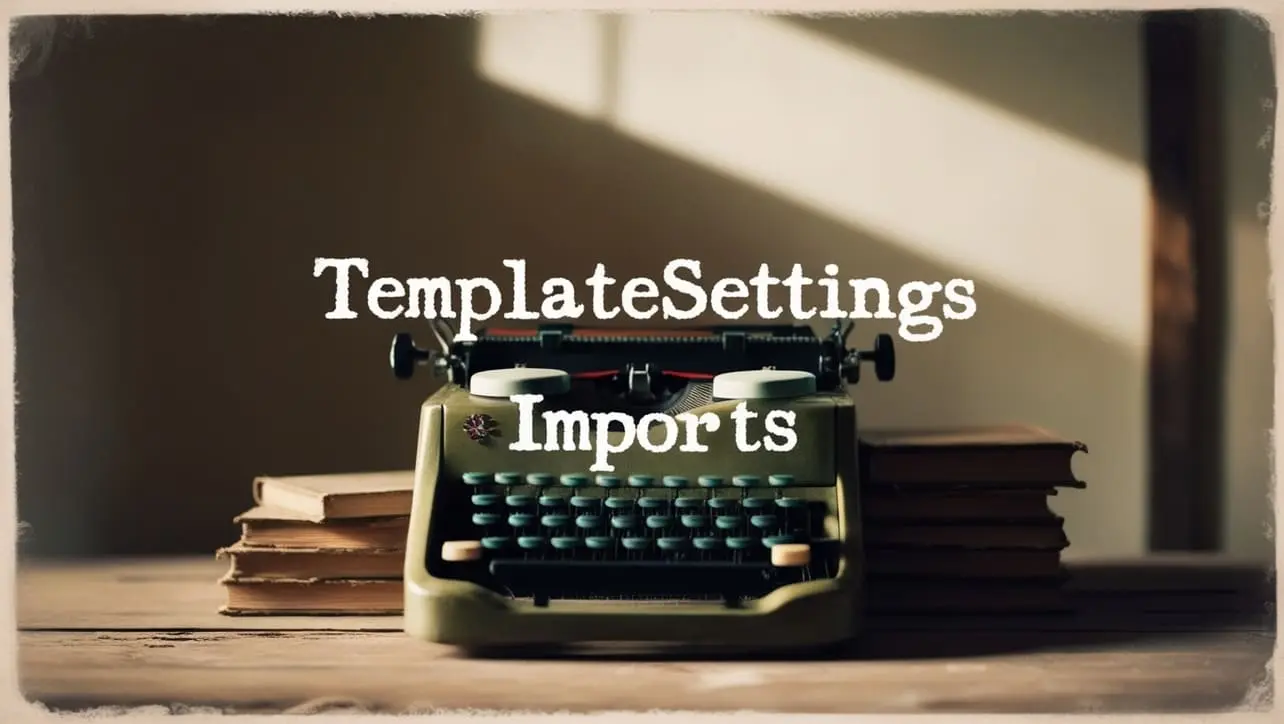
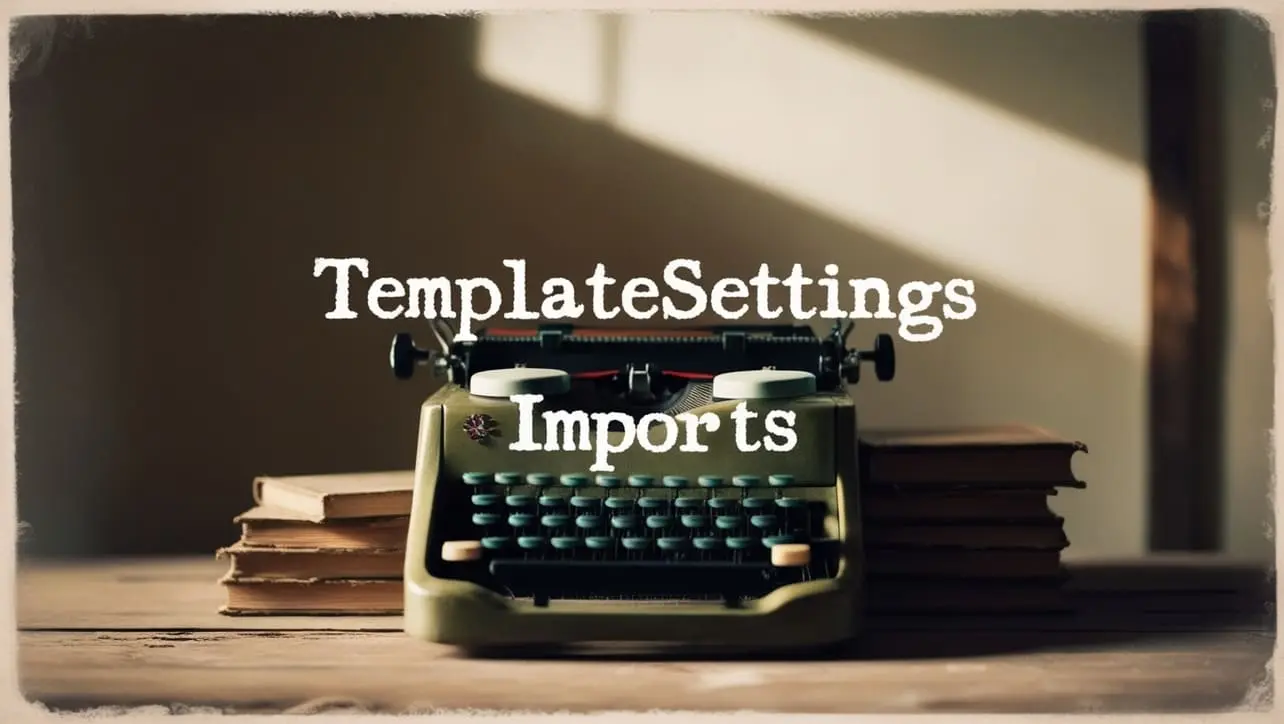
Lodash _.templateSettings.imports Property
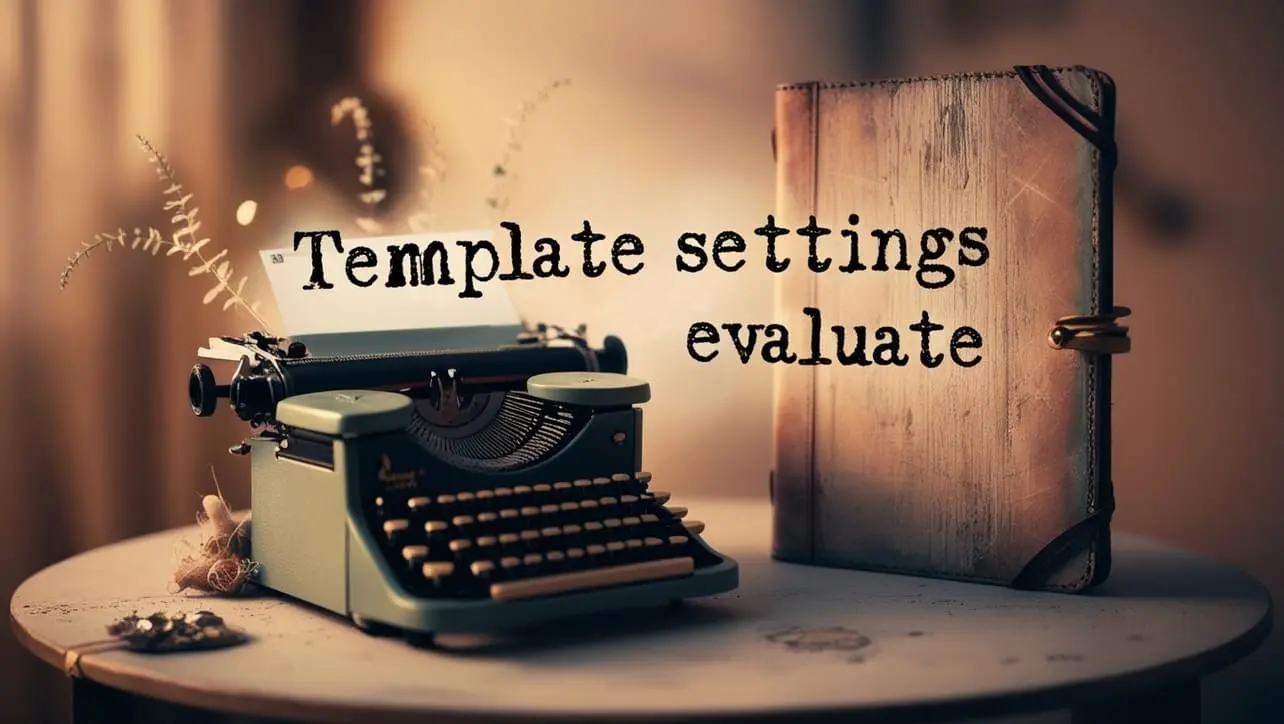
Lodash _.templateSettings.evaluate Property
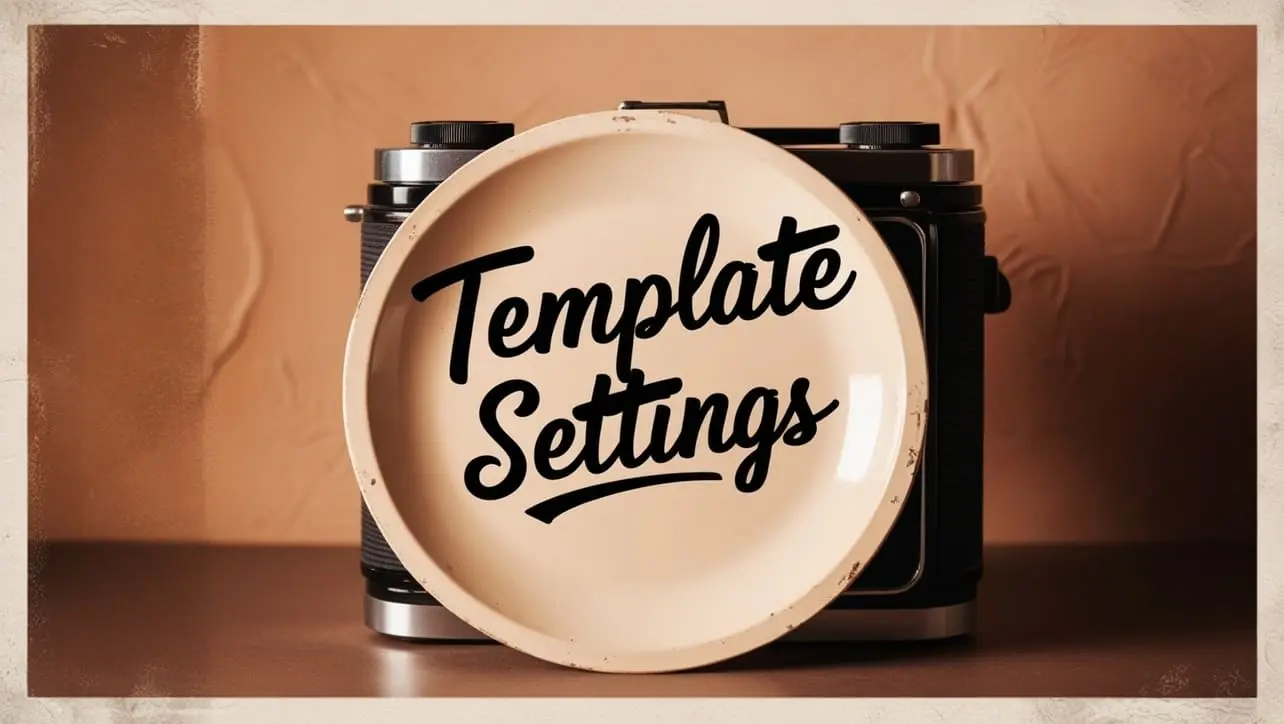
Lodash _.templateSettings Property
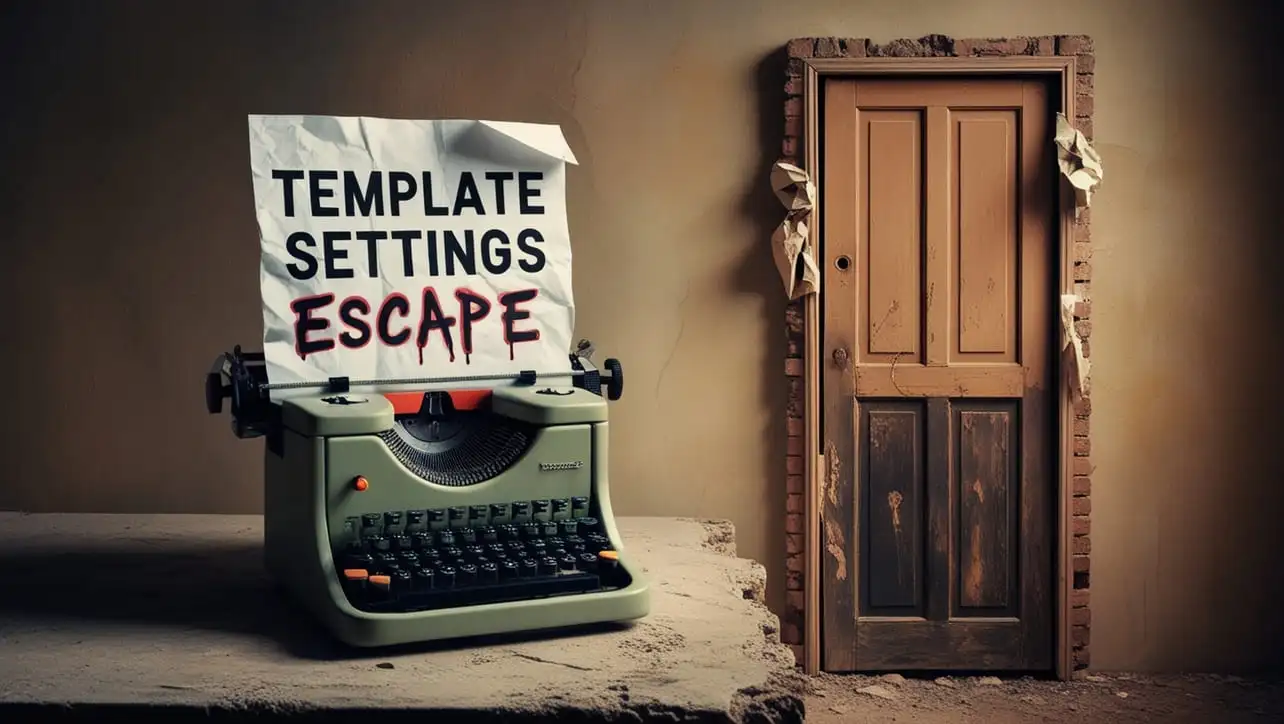
Lodash _.templateSettings.escape Property
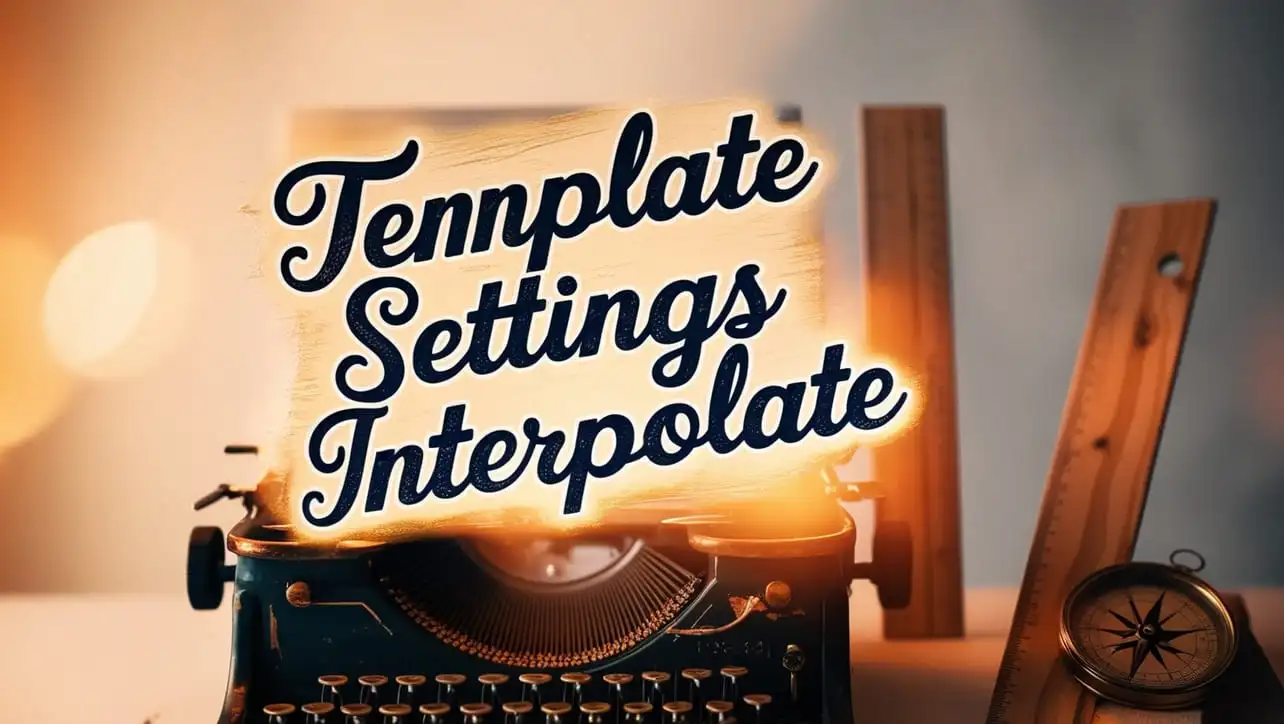
Lodash _.templateSettings.interpolate Property
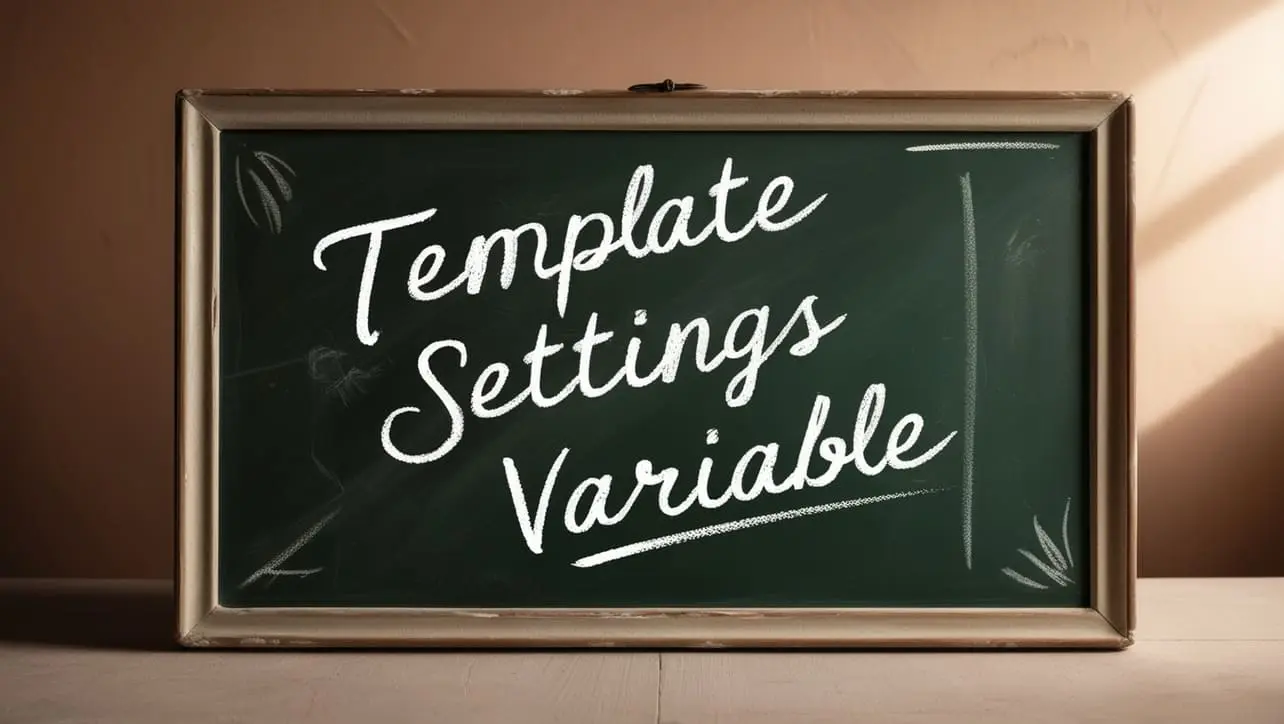
If you have any doubts regarding this article (Lodash _.valuesIn() Object Method), please comment here. I will help you immediately.