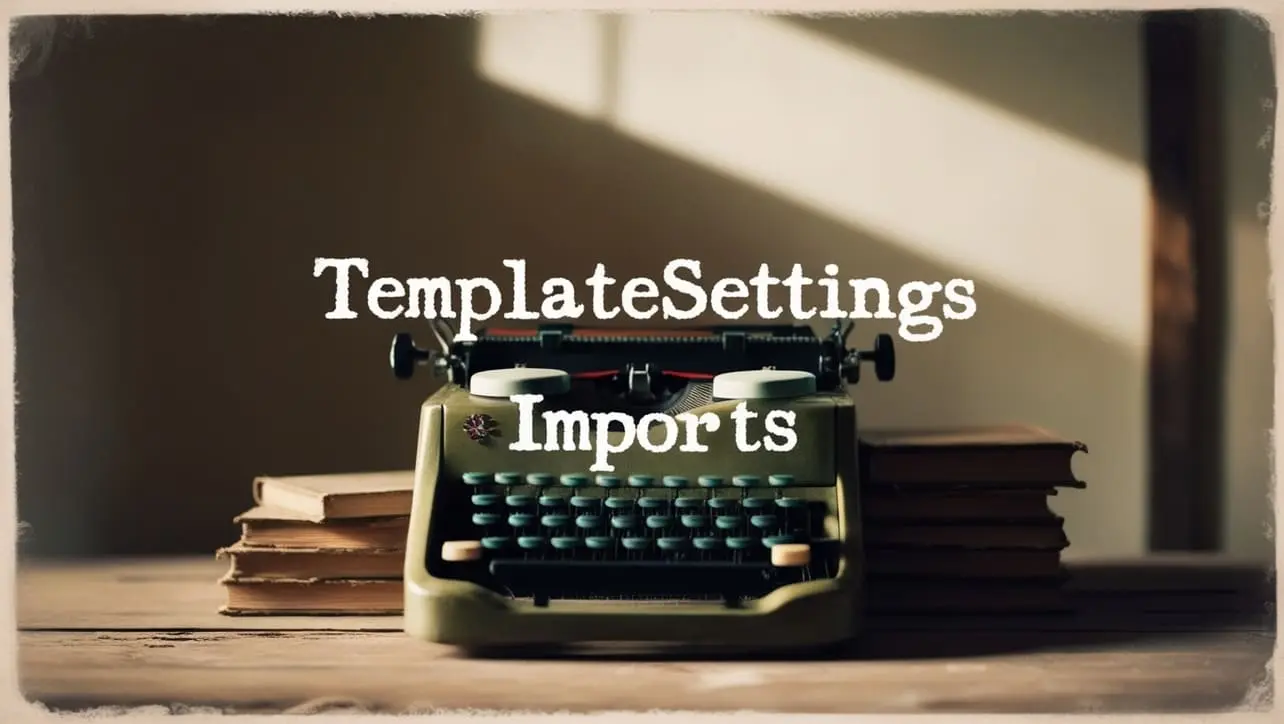
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.values() Object Method
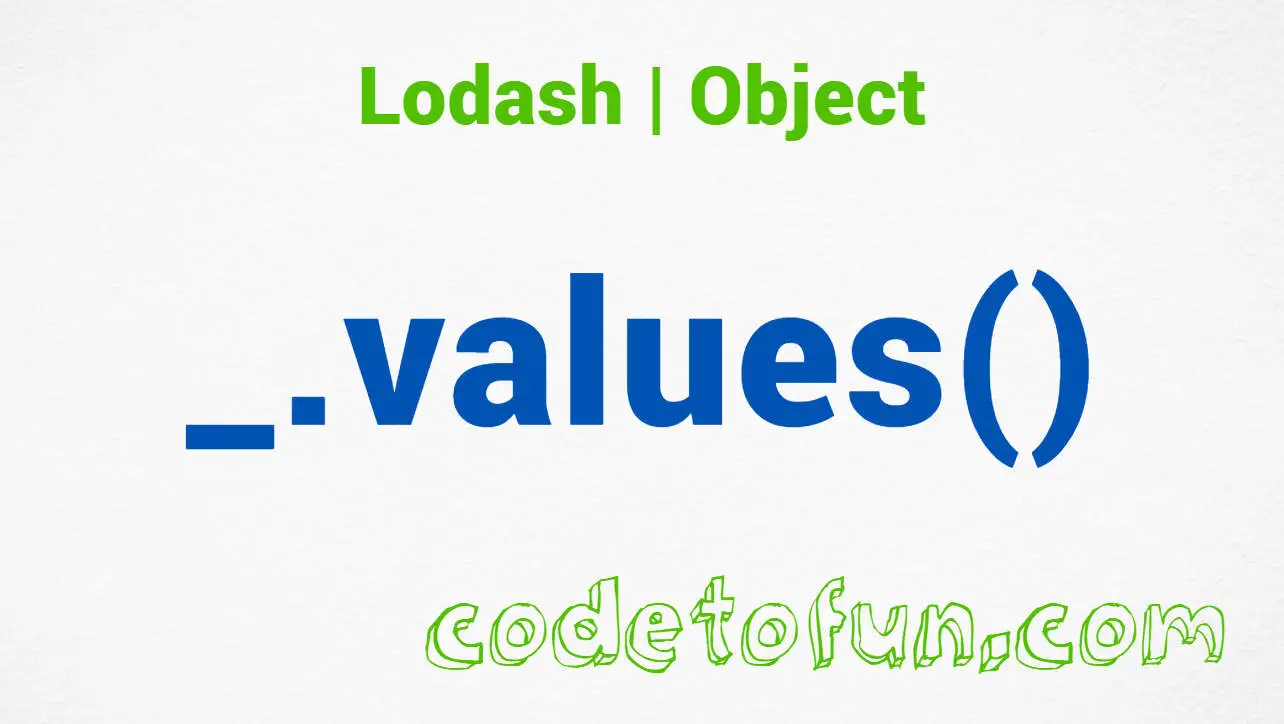
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript, objects are fundamental data structures used to store key-value pairs. While working with objects, developers often need to extract the values associated with the keys for various operations. The Lodash library simplifies this task with its _.values()
method, which retrieves the values of an object's own enumerable properties into an array.
This method provides a convenient and efficient way to access and manipulate object values in JavaScript applications.
🧠 Understanding _.values() Method
The _.values()
method in Lodash allows developers to extract the values of an object and store them in an array. This array can then be used for further processing, such as iteration, filtering, or transformation. By abstracting the process of extracting object values into a single method, Lodash streamlines code and enhances readability.
💡 Syntax
The syntax for the _.values()
method is straightforward:
_.values(object)
- object: The object whose values will be retrieved.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.values()
method:
const _ = require('lodash');
const sampleObject = { a: 1, b: 2, c: 3 };
const objectValues = _.values(sampleObject);
console.log(objectValues);
// Output: [1, 2, 3]
In this example, _.values()
is applied to the sampleObject, resulting in an array containing its values.
🏆 Best Practices
When working with the _.values()
method, consider the following best practices:
Check for Object Validity:
Before applying
_.values()
, ensure that the input is a valid object to prevent runtime errors.example.jsCopiedconst invalidObject = 'This is not an object'; const objectValues = _.values(invalidObject); console.log(objectValues); // Output: []
Enumerate Own Properties:
_.values()
retrieves the values of an object's own enumerable properties. Be aware that inherited properties or non-enumerable properties will not be included in the result.example.jsCopiedfunction SampleClass() { this.a = 1; this.b = 2; } SampleClass.prototype.c = 3; const sampleInstance = new SampleClass(); const instanceValues = _.values(sampleInstance); console.log(instanceValues); // Output: [1, 2]
Use with Object Destructuring:
Combine
_.values()
with object destructuring for concise and expressive code when extracting values from objects.example.jsCopiedconst user = { id: 1, name: 'John Doe', email: 'john@example.com' }; const { name, email } = user; console.log(name, email); // Output: John Doe john@example.com
📚 Use Cases
Iteration and Transformation:
_.values()
facilitates iteration over object values, enabling transformations or computations based on those values.example.jsCopiedconst salesData = { product1: 100, product2: 200, product3: 150, }; const totalSales = _.sum(_.values(salesData)); console.log(totalSales); // Output: 450
Data Manipulation:
Object values extracted with
_.values()
can be manipulated or processed further to derive insights or prepare data for display.example.jsCopiedconst userData = { user1: { name: 'Alice', age: 30 }, user2: { name: 'Bob', age: 25 }, user3: { name: 'Charlie', age: 35 }, }; const userAges = _.mapValues(userData, user => user.age); const averageAge = _.mean(_.values(userAges)); console.log(averageAge); // Output: 30
🎉 Conclusion
The _.values()
method in Lodash simplifies the extraction of object values, providing developers with a concise and efficient solution for accessing and manipulating object data in JavaScript applications. By leveraging this method, developers can streamline their code and enhance productivity when working with object-oriented data structures.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.values()
method in your Lodash projects.
👨💻 Join our Community:
Author
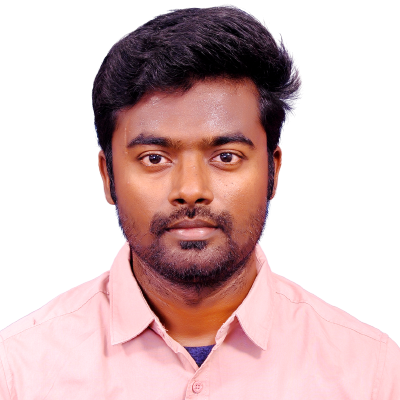
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
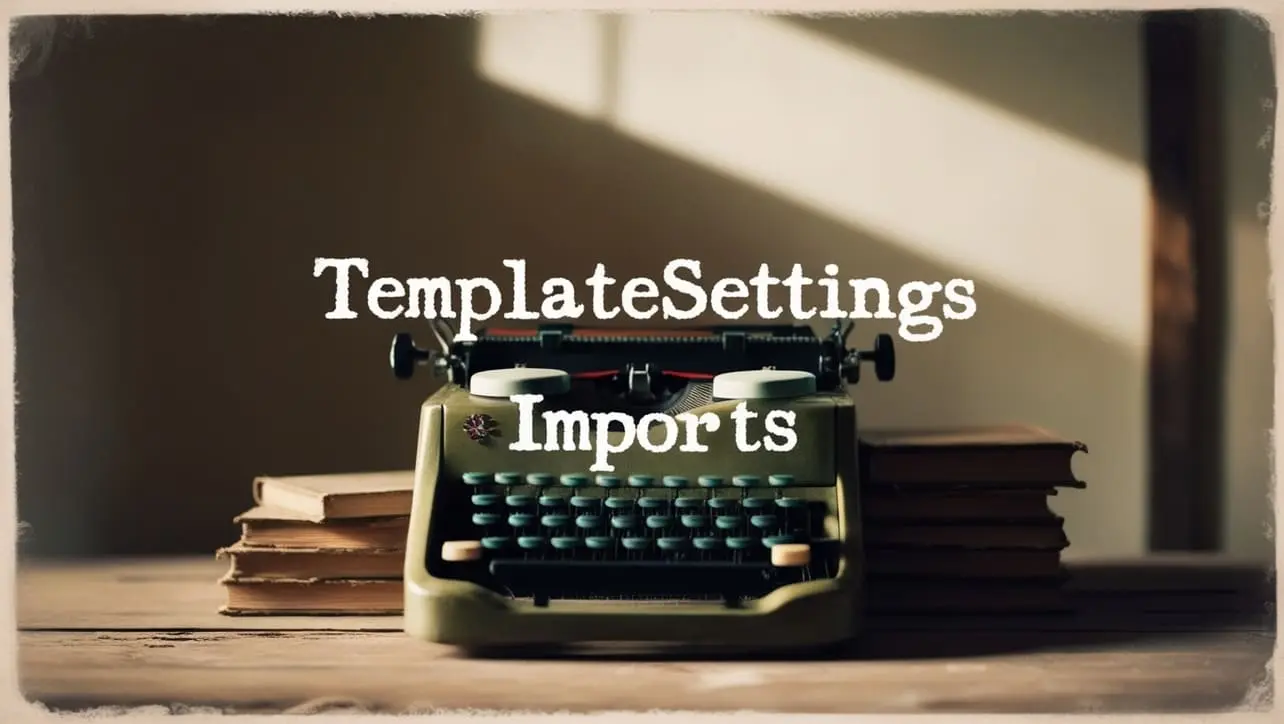
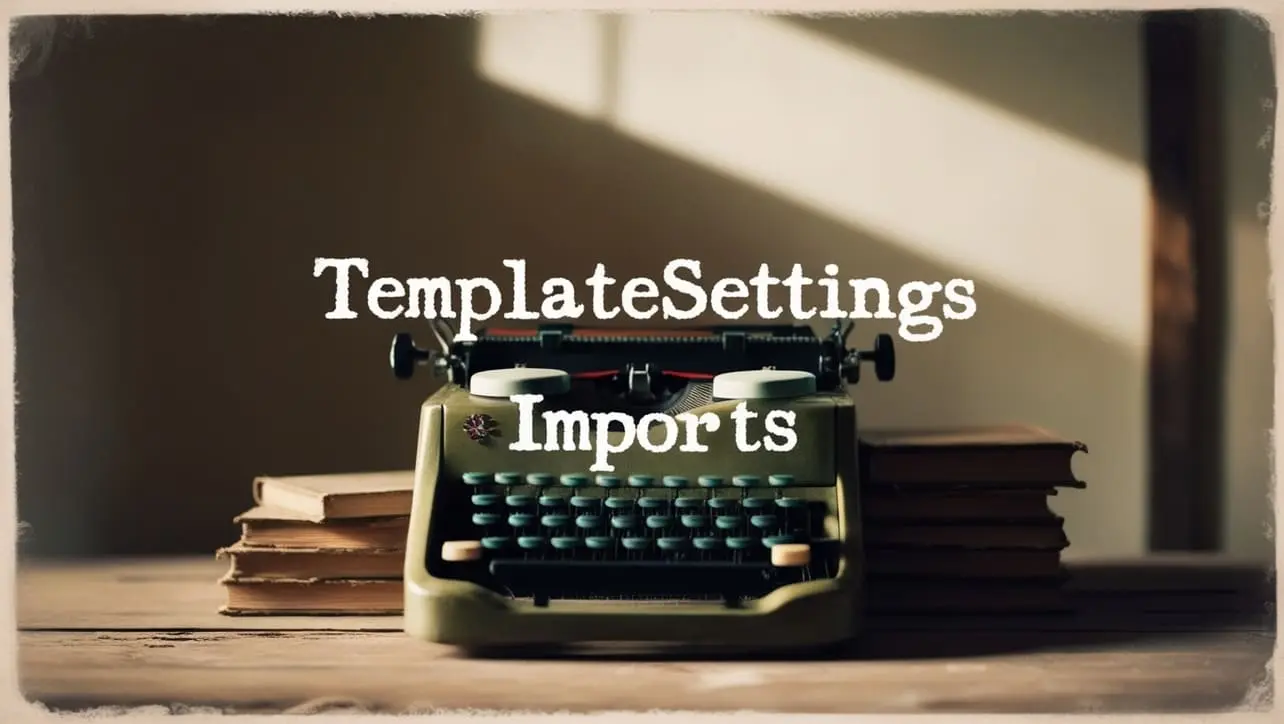
Lodash _.templateSettings.imports Property
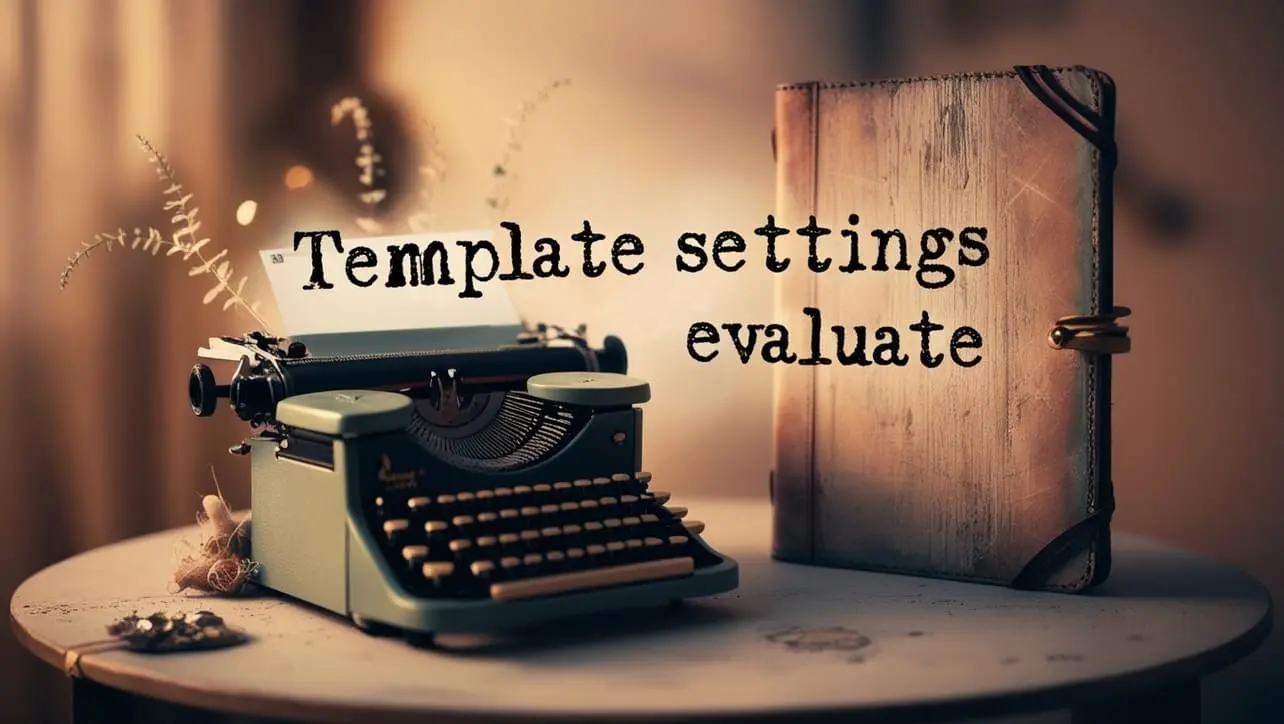
Lodash _.templateSettings.evaluate Property
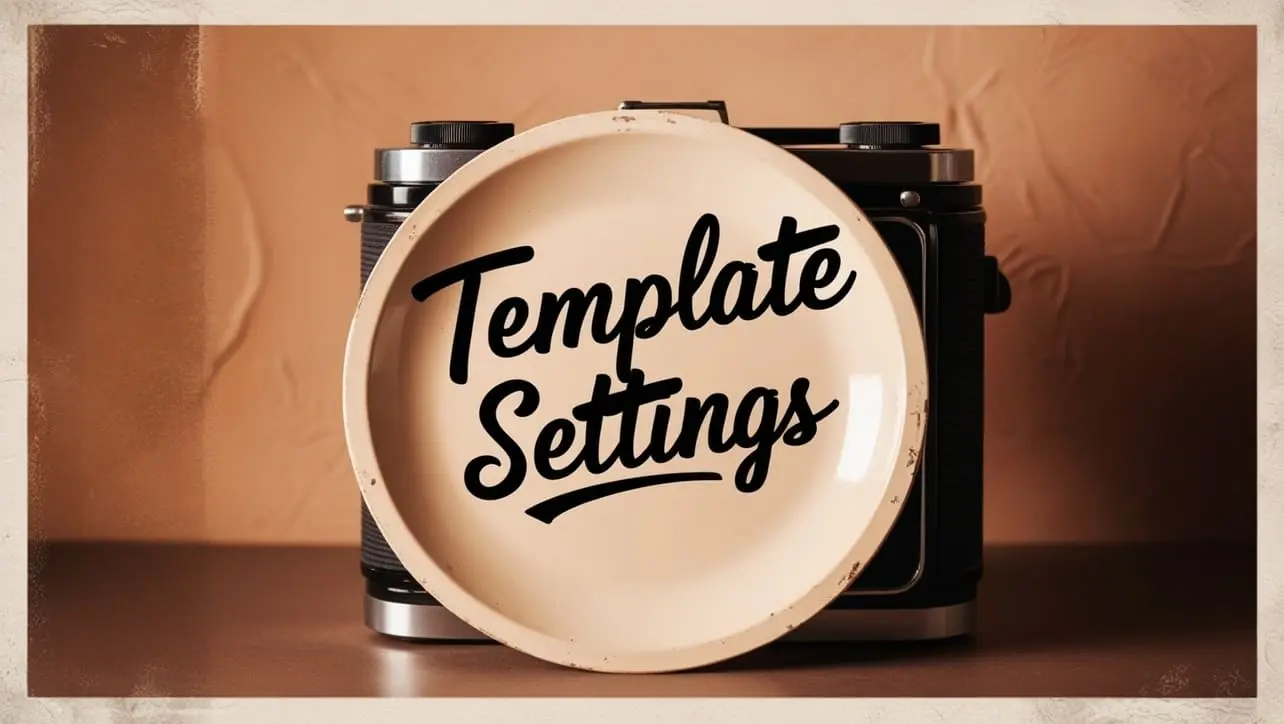
Lodash _.templateSettings Property
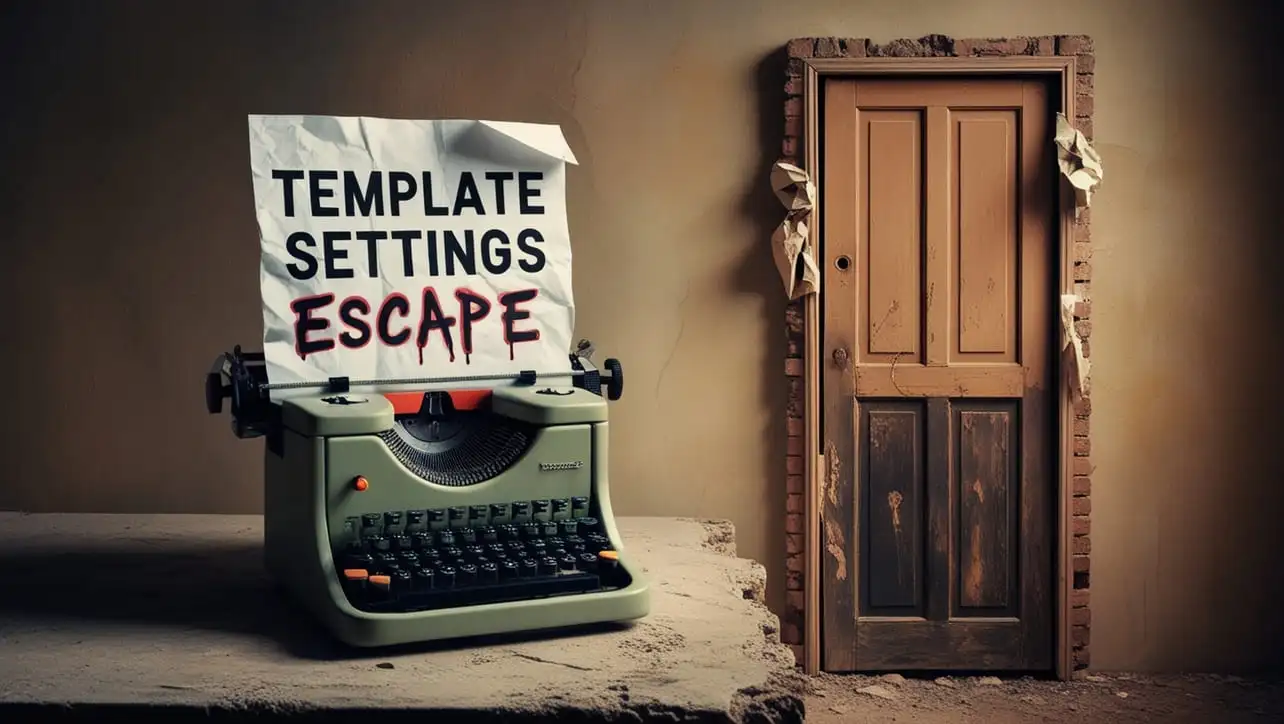
Lodash _.templateSettings.escape Property
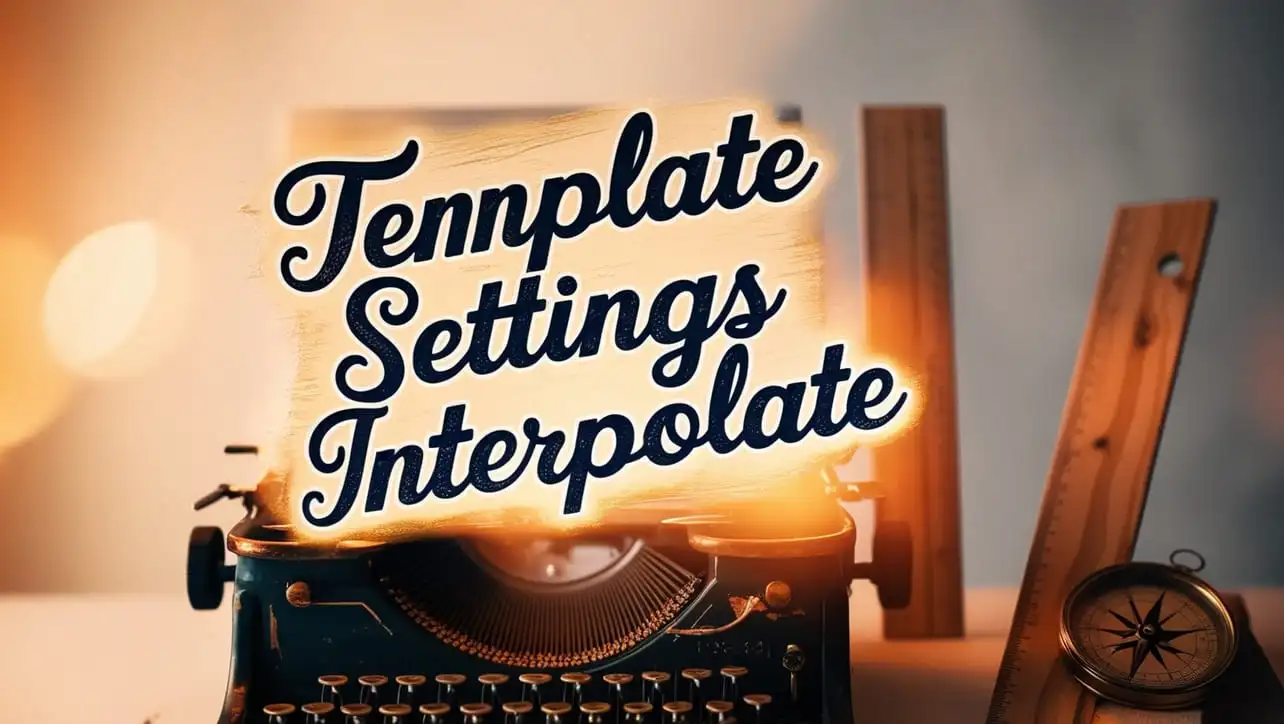
Lodash _.templateSettings.interpolate Property
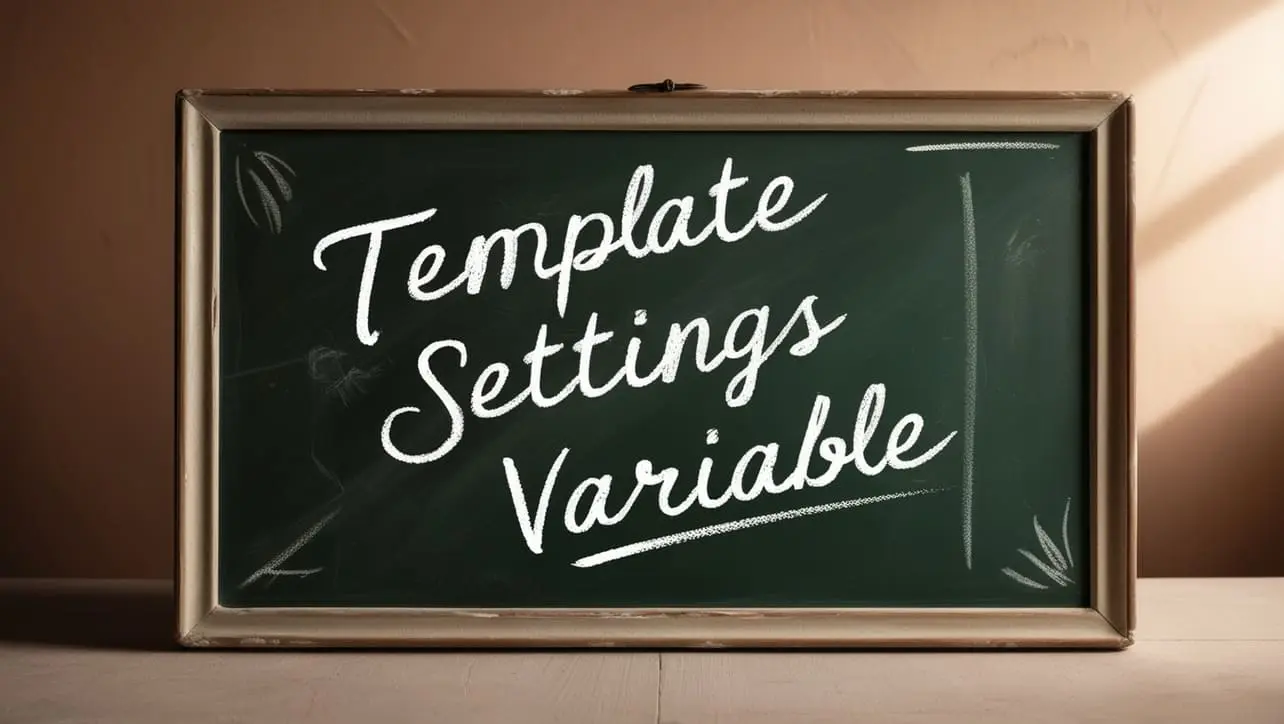
If you have any doubts regarding this article (Lodash _.values() Object Method), please comment here. I will help you immediately.