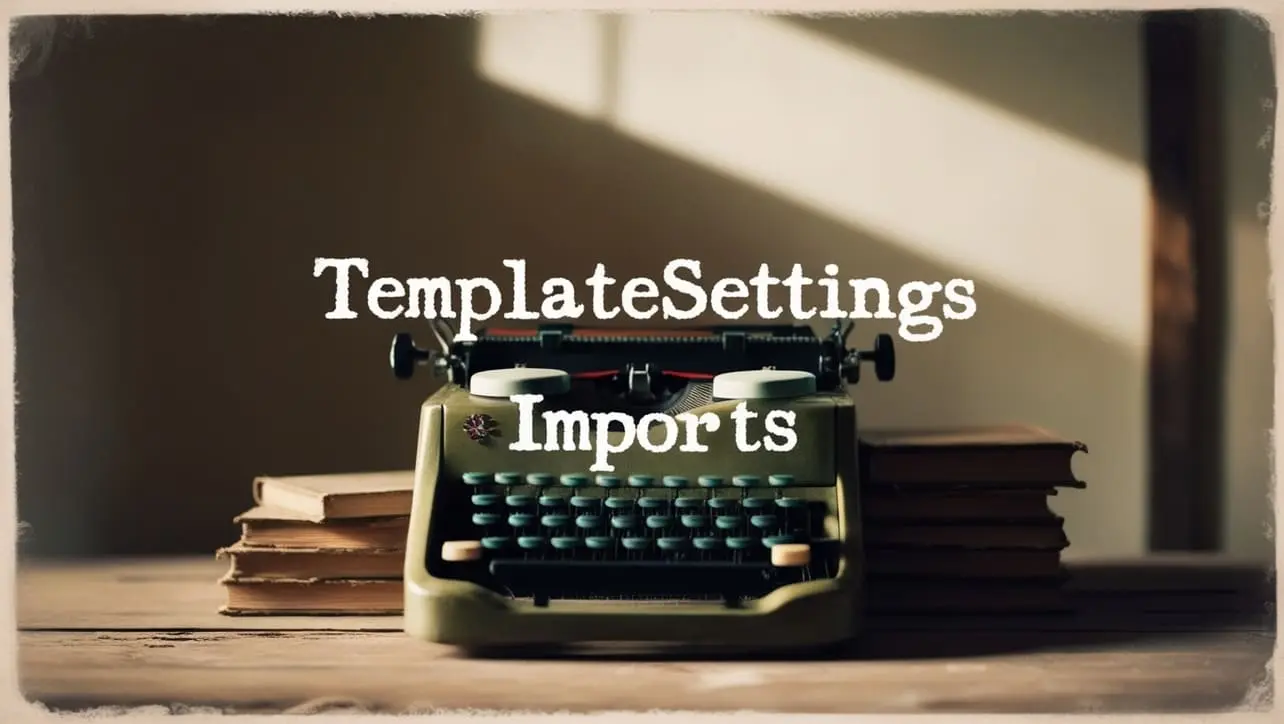
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.unset() Object Method
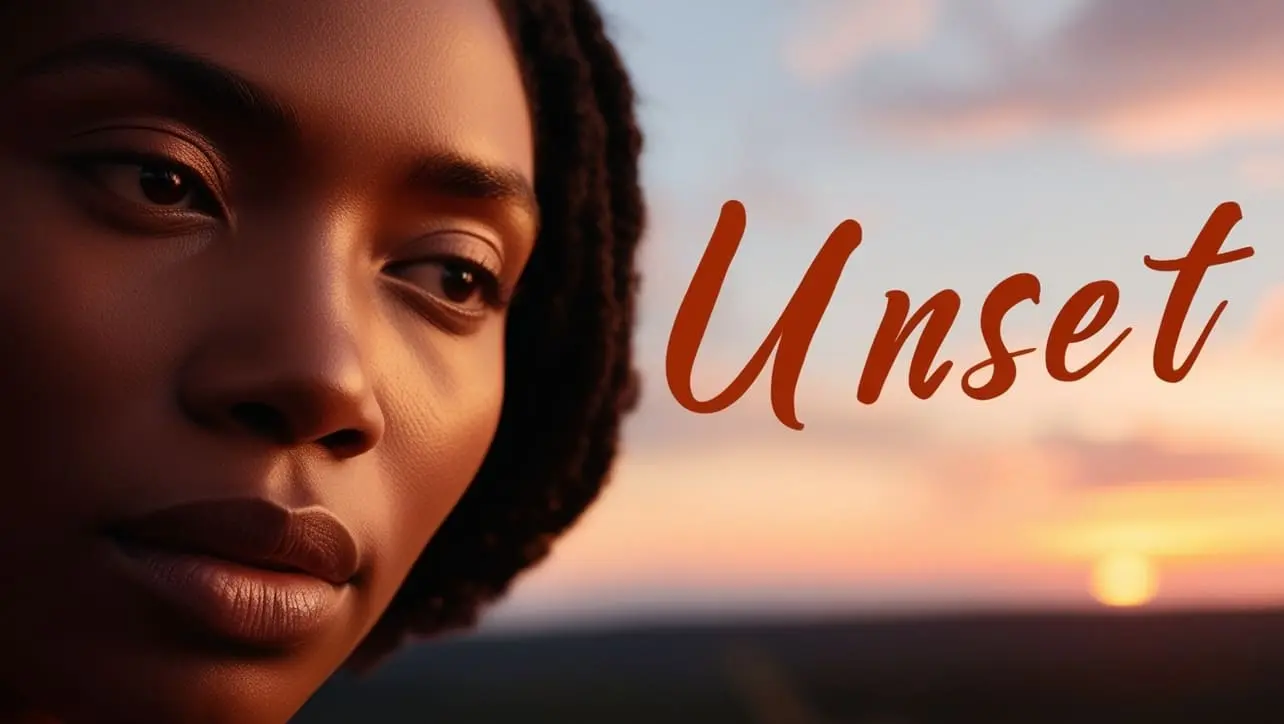
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript development, effective manipulation of objects is essential for building robust applications. Lodash, a popular utility library, provides a multitude of functions to simplify object manipulation tasks. One such function is _.unset()
, which allows developers to safely remove properties from nested objects.
This method enhances code readability and maintainability, making it a valuable tool for managing complex data structures.
🧠 Understanding _.unset() Method
The _.unset()
method in Lodash facilitates the removal of properties from nested objects, ensuring that the operation is performed safely without causing errors if the property or its parent objects do not exist. This provides a convenient way to clean up object structures and manage dynamic data.
💡 Syntax
The syntax for the _.unset()
method is straightforward:
_.unset(object, path)
- object: The object from which to remove the property.
- path: The path of the property to remove.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.unset()
method:
const _ = require('lodash');
let user = {
id: 1,
name: 'John',
address: {
city: 'New York',
zip: '10001'
}
};
_.unset(user, 'address.zip');
console.log(user);
// Output: { id: 1, name: 'John', address: { city: 'New York' } }
In this example, the zip property nested within the address object is removed using _.unset()
.
🏆 Best Practices
When working with the _.unset()
method, consider the following best practices:
Safety Checks:
Perform safety checks before using
_.unset()
to ensure that the property exists in the object. This prevents errors and unexpected behavior.example.jsCopiedif (_.has(user, 'address.zip')) { _.unset(user, 'address.zip'); }
Handling Nested Properties:
Handle nested properties with care and ensure that the path provided to
_.unset()
accurately reflects the property's location within the object.example.jsCopiedlet nestedObject = { a: { b: { c: 'Nested Value' } } }; _.unset(nestedObject, 'a.b.c'); console.log(nestedObject); // Output: { a: { b: {} } }
Immutable Operations:
Keep in mind that
_.unset()
operates immutably, meaning it does not modify the original object but returns a new object with the specified property removed. Assign the result to a new variable or use it accordingly.example.jsCopiedlet updatedUser = _.unset(user, 'address.zip'); console.log(updatedUser); // Output: { id: 1, name: 'John', address: { city: 'New York' } }
📚 Use Cases
Data Cleaning:
_.unset()
is useful for cleaning up object data by removing unwanted or obsolete properties, ensuring data integrity and consistency.example.jsCopiedlet data = { id: 1, name: 'Alice', email: 'alice@example.com', password: 'hashedpassword123' }; _.unset(data, 'password'); console.log(data); // Output: { id: 1, name: 'Alice', email: 'alice@example.com' }
Dynamic Object Manipulation:
When dealing with dynamically generated object structures,
_.unset()
provides a reliable way to manage and modify object properties as needed.example.jsCopiedlet dynamicData = { settings: { theme: 'light', language: 'en' } }; let propertyToRemove = 'settings.theme'; _.unset(dynamicData, propertyToRemove); console.log(dynamicData); // Output: { settings: { language: 'en' } }
Conditional Property Removal:
In scenarios where certain properties need to be removed based on specific conditions,
_.unset()
offers a straightforward solution for conditional property removal.example.jsCopiedlet settings = { theme: 'dark', showNotifications: true, enableAnalytics: false }; if(!settings.showNotifications) { _.unset(settings, 'showNotifications'); } console.log(settings); // Output: { theme: 'dark', enableAnalytics: false }
🎉 Conclusion
The _.unset()
method in Lodash provides a convenient and safe way to remove properties from nested objects in JavaScript. By following best practices and leveraging its versatility, developers can effectively manage object structures and maintain code cleanliness. Incorporate _.unset()
into your projects to streamline object manipulation tasks and enhance code quality.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.unset()
method in your Lodash projects.
👨💻 Join our Community:
Author
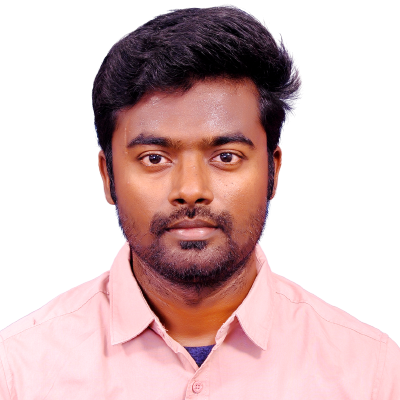
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
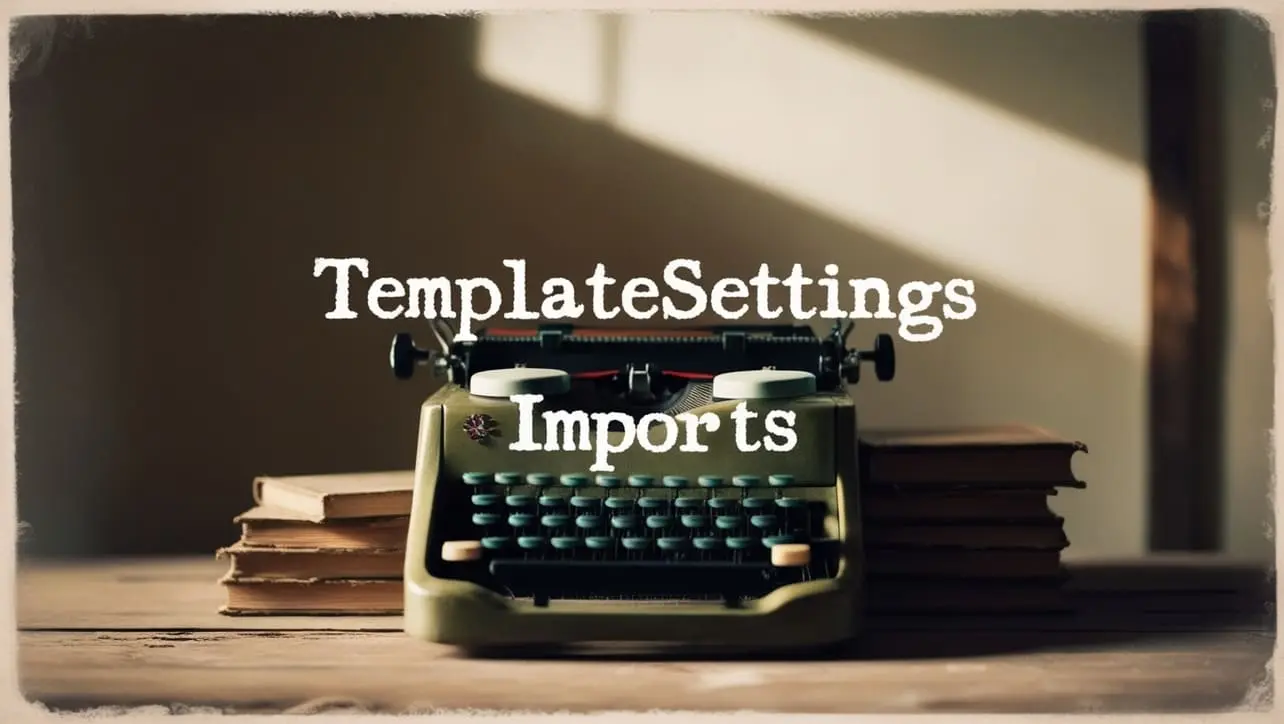
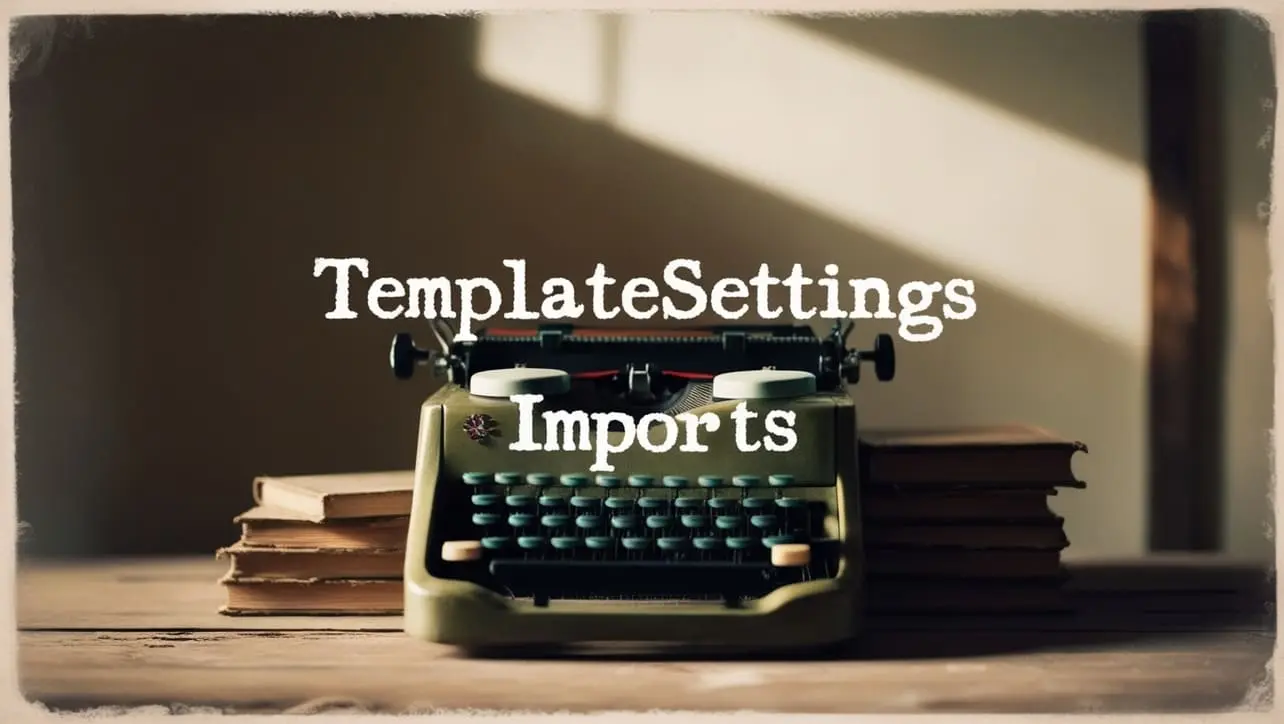
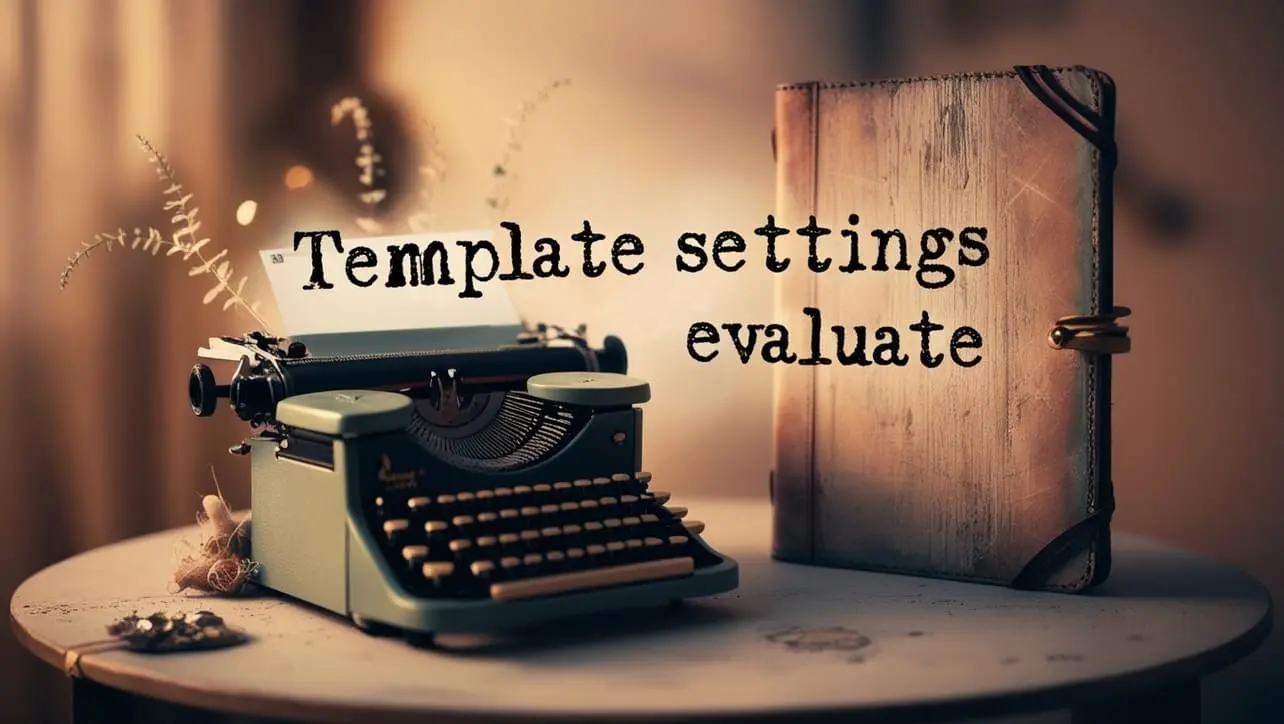
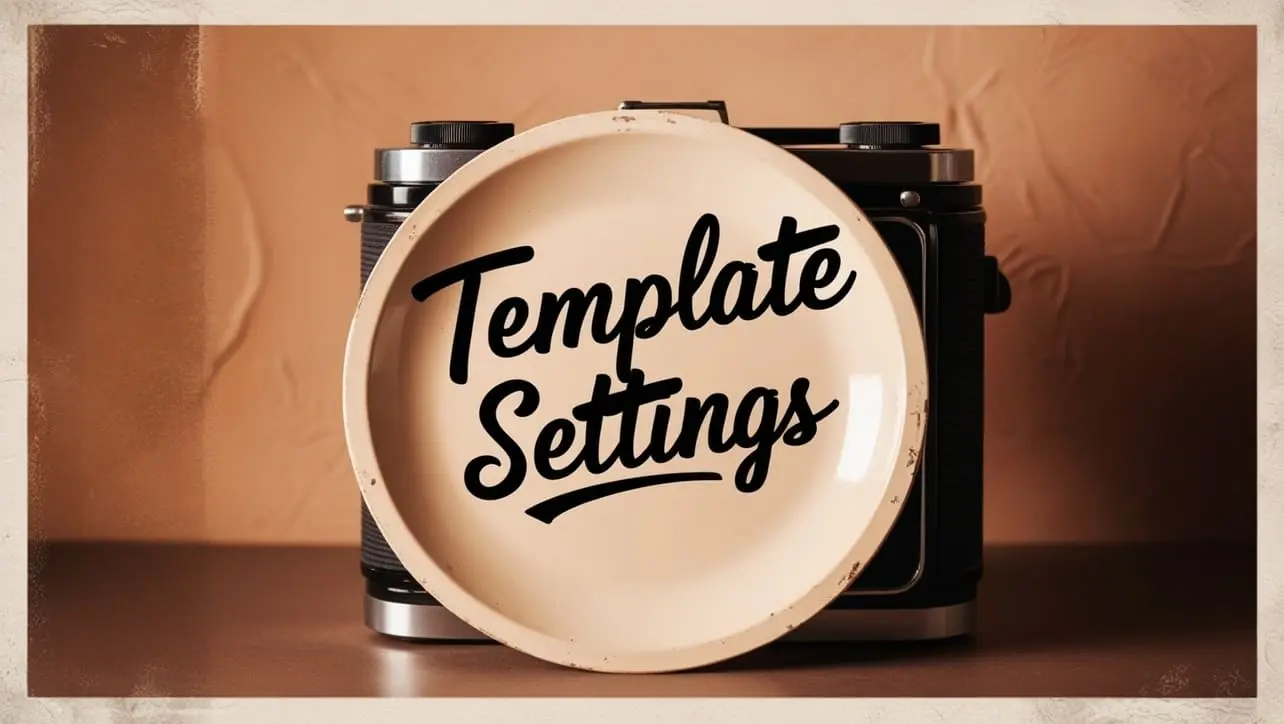
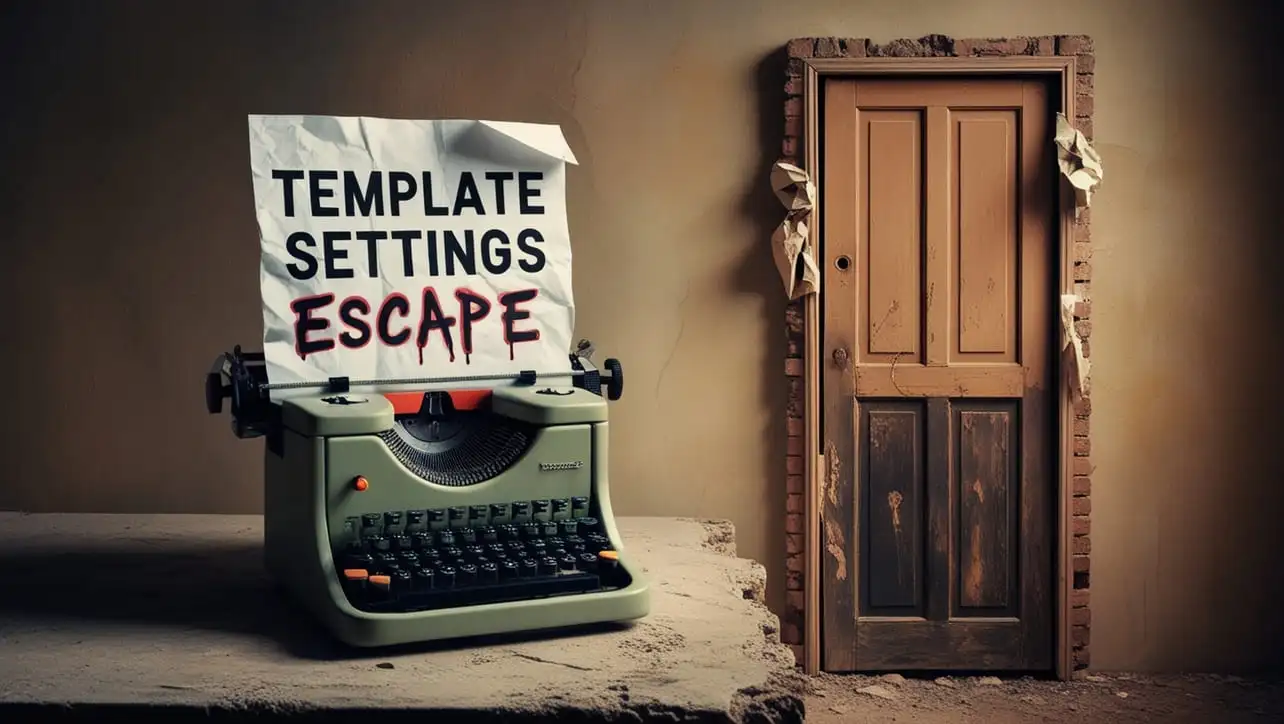
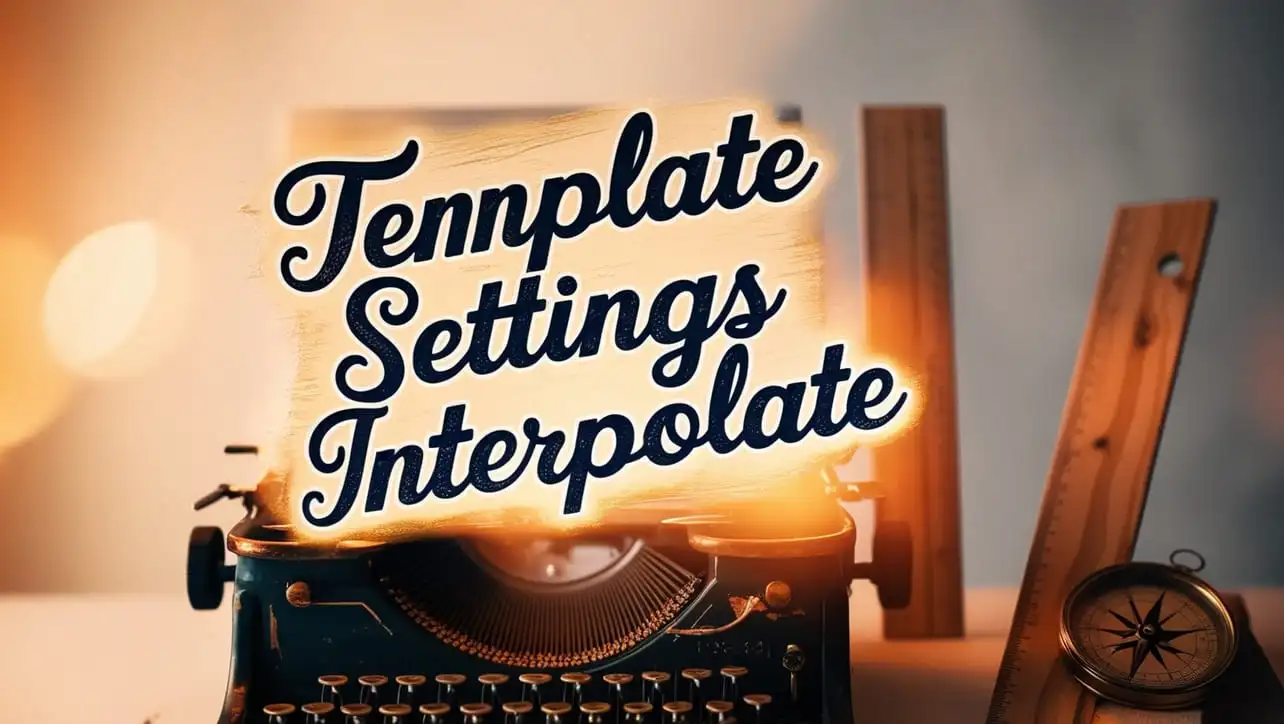
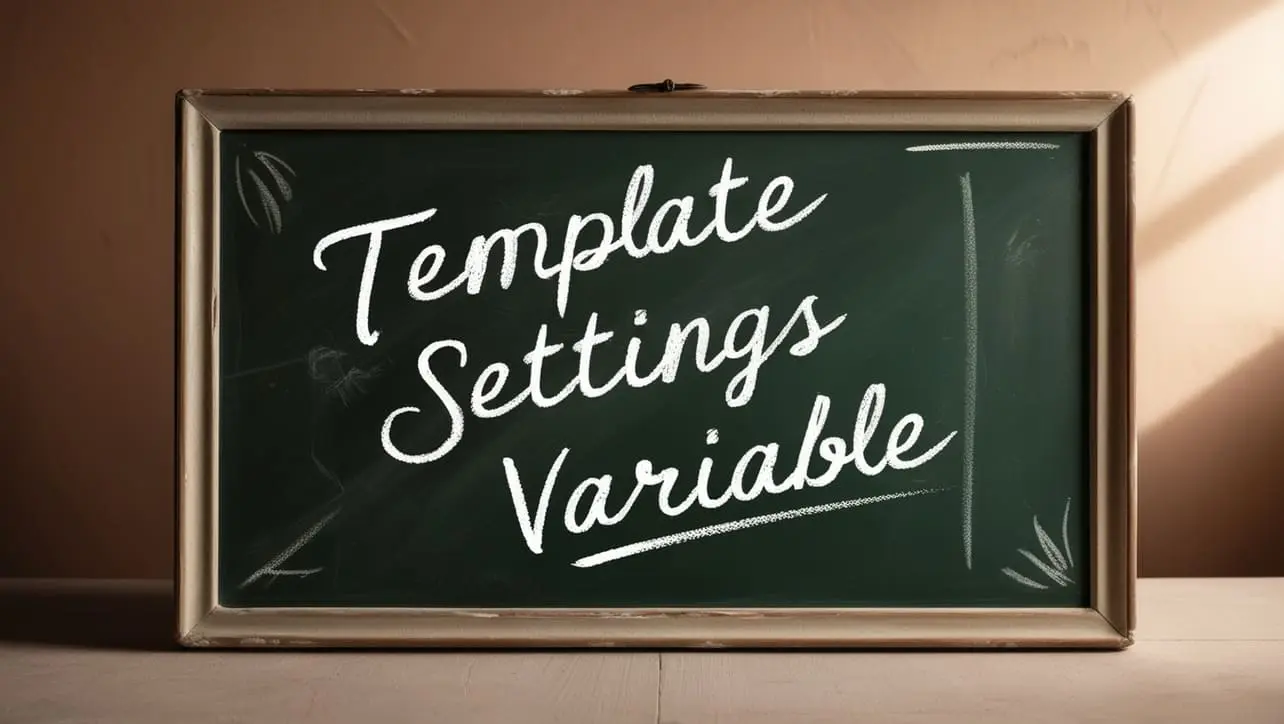
If you have any doubts regarding this article (Lodash _.unset() Object Method), please comment here. I will help you immediately.