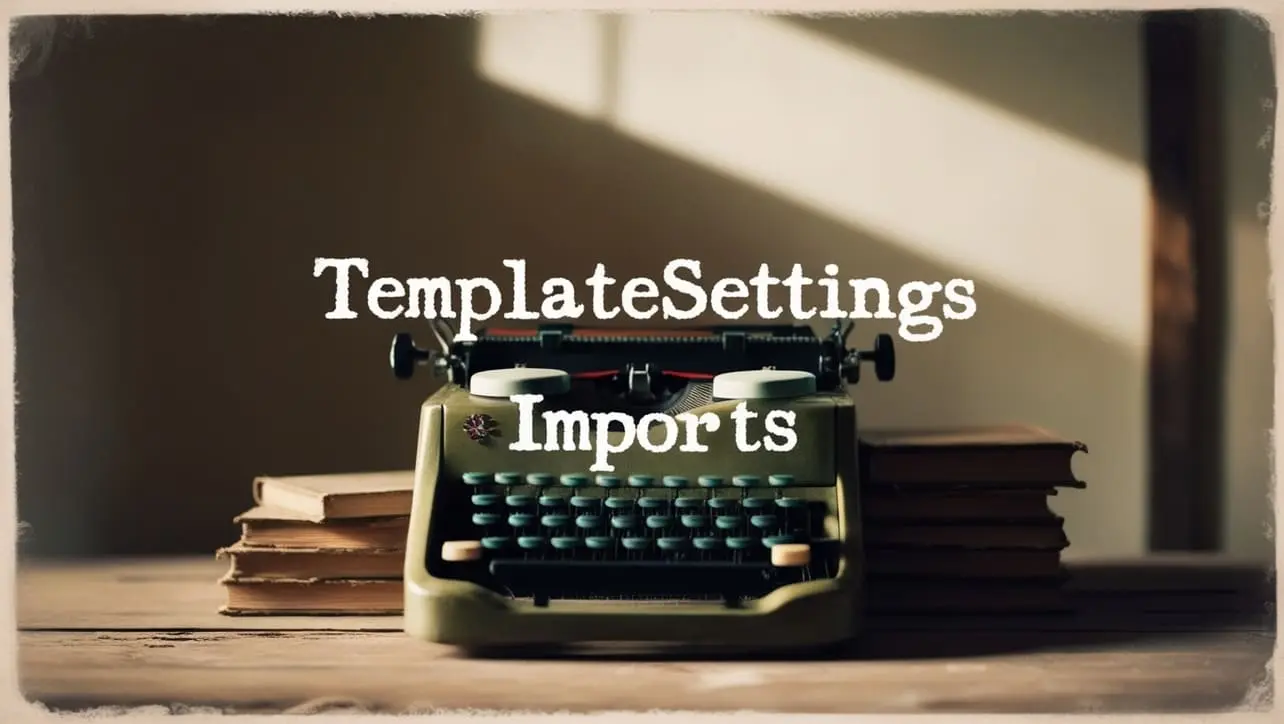
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.transform() Object Method
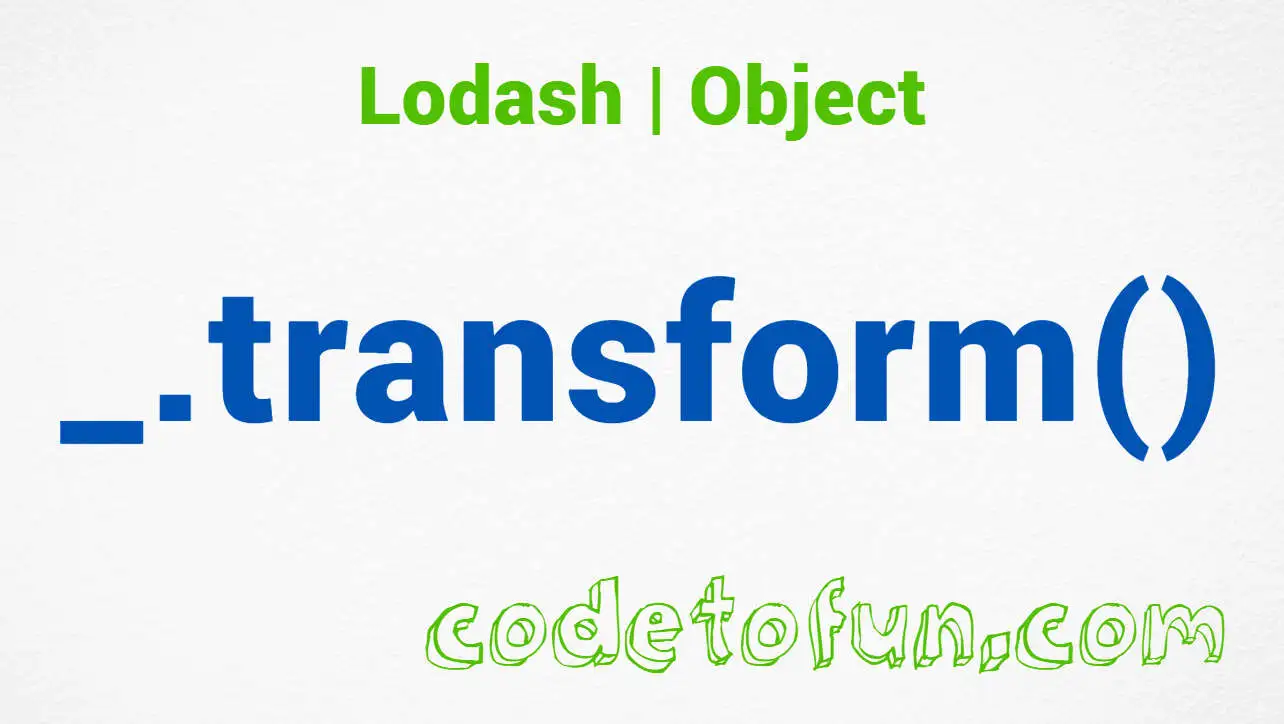
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript development, efficient manipulation of objects is crucial for various tasks ranging from data processing to algorithm implementation. Lodash, a popular utility library, provides a rich set of functions to streamline object manipulation. Among these functions is the _.transform()
method, a versatile tool for iteratively transforming objects based on custom logic.
This method empowers developers to handle complex object transformations with ease and precision.
🧠 Understanding _.transform() Method
The _.transform()
method in Lodash facilitates the iterative transformation of objects. Unlike some other methods that operate on objects, _.transform()
allows for intricate modifications, enabling developers to apply custom logic at each iteration. This flexibility makes it invaluable for tasks such as data normalization, filtering, and aggregation.
💡 Syntax
The syntax for the _.transform()
method is straightforward:
_.transform(object, [iteratee], [accumulator])
- object: The object to iterate over.
- iteratee (Optional): The function invoked per iteration.
- accumulator (Optional): The initial value of the accumulator.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.transform()
method:
const _ = require('lodash');
const data = {
a: 1,
b: 2,
c: 3
};
const transformedObject = _.transform(data, (result, value, key) => {
result[key.toUpperCase()] = value * 2;
}, {});
console.log(transformedObject);
// Output: { A: 2, B: 4, C: 6 }
In this example, the data object is iterated over, with each key-value pair transformed according to the provided iteratee function. The transformed object is then returned with keys converted to uppercase and values doubled.
🏆 Best Practices
When working with the _.transform()
method, consider the following best practices:
Understand Iteration Order:
Be aware of the iteration order when using
_.transform()
. By default, iteration occurs in ascending order of object keys. If a specific order is required, consider pre-sorting the keys or providing a custom iteratee function.example.jsCopiedconst data = { b: 2, a: 1, c: 3 }; const transformedObject = _.transform(data, (result, value, key) => { result[key.toUpperCase()] = value * 2; }, {}); console.log(transformedObject); // Output: { A: 2, B: 4, C: 6 }
Handle Complex Transformations:
Utilize
_.transform()
for complex object transformations involving conditional logic, data aggregation, or nested structures. Its iterative nature and customizable iteratee function provide the flexibility needed for such tasks.example.jsCopiedconst data = { a: { value: 1 }, b: { value: 2 }, c: { value: 3 } }; const transformedObject = _.transform(data, (result, obj, key) => { if (obj.value > 1) { result[key.toUpperCase()] = obj.value * 2; } }, {}); console.log(transformedObject); // Output: { B: 4, C: 6 }
Initialize Accumulator Appropriately:
Ensure that the accumulator is initialized appropriately, especially when performing cumulative operations. Depending on the use case, the accumulator can be initialized as an empty object, array, or any other suitable value.
example.jsCopiedconst data = [1, 2, 3]; const sum = _.transform(data, (result, value) => { result.total += value; }, { total: 0 }).total; console.log(sum); // Output: 6
📚 Use Cases
Data Transformation and Normalization:
_.transform()
is ideal for transforming and normalizing data structures, especially when dealing with diverse or irregular data formats. It enables developers to apply consistent formatting or extract specific information from objects.example.jsCopiedconst rawData = /* ...fetch data from API or elsewhere... */; const normalizedData = _.transform(rawData, (result, obj) => { // Apply data normalization logic here }, []);
Filtering Objects:
For tasks requiring selective inclusion or exclusion of object properties based on specific criteria,
_.transform()
can be used to filter objects dynamically.example.jsCopiedconst data = { a: 1, b: 2, c: 3 }; const filteredObject = _.transform(data, (result, value, key) => { if(value > 1) { result[key] = value; } }, {}); console.log(filteredObject); // Output: { b: 2, c: 3 }
Aggregating Data:
When aggregating data from multiple sources or organizing information into a structured format,
_.transform()
offers a powerful mechanism for accumulating and organizing data.example.jsCopiedconst dataSet = /* ...fetch data from multiple sources... */; const aggregatedData = _.transform(dataSet, (result, data) => { // Aggregate data into result object/array }, {});
🎉 Conclusion
The _.transform()
method in Lodash empowers developers to perform intricate object transformations with ease and precision. By leveraging its iterative nature and customizable iteratee function, developers can handle a wide range of object manipulation tasks efficiently. Whether it's data normalization, filtering, or aggregation, _.transform()
offers a versatile solution for JavaScript developers.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.transform()
method in your Lodash projects.
👨💻 Join our Community:
Author
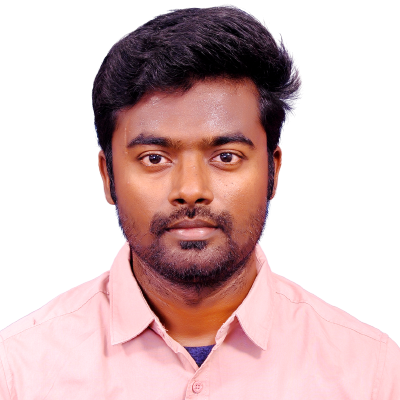
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
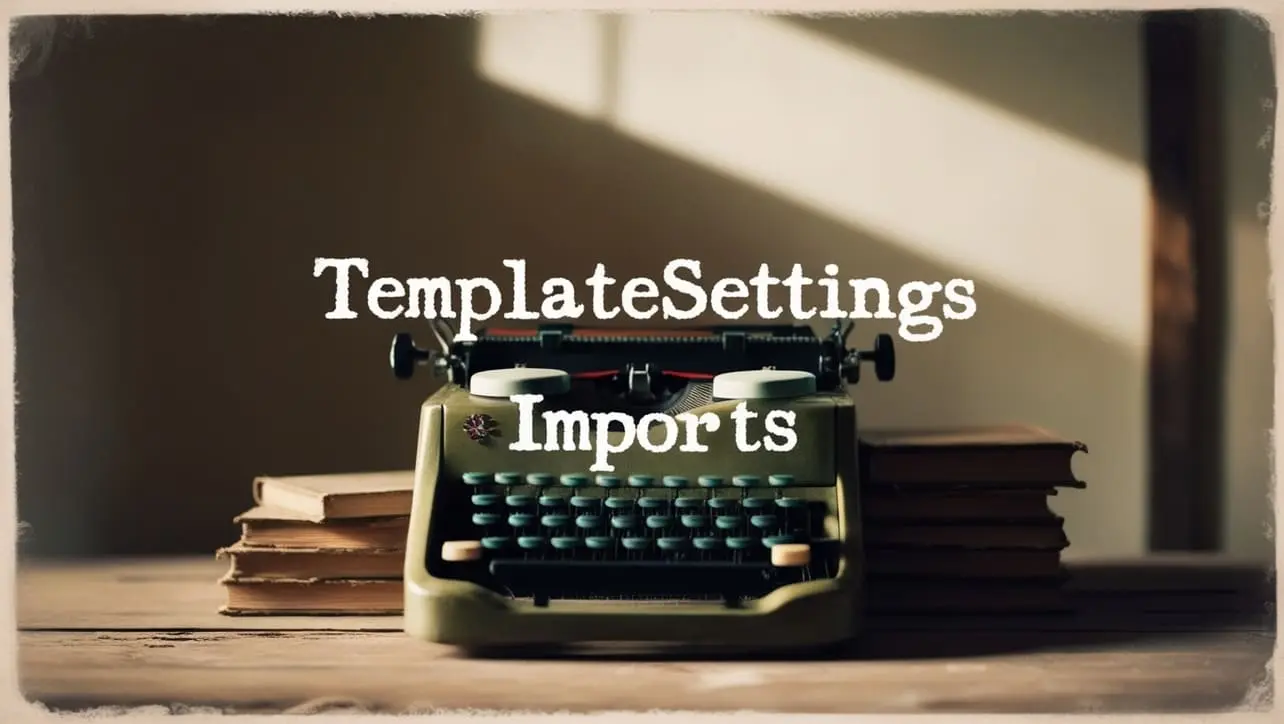
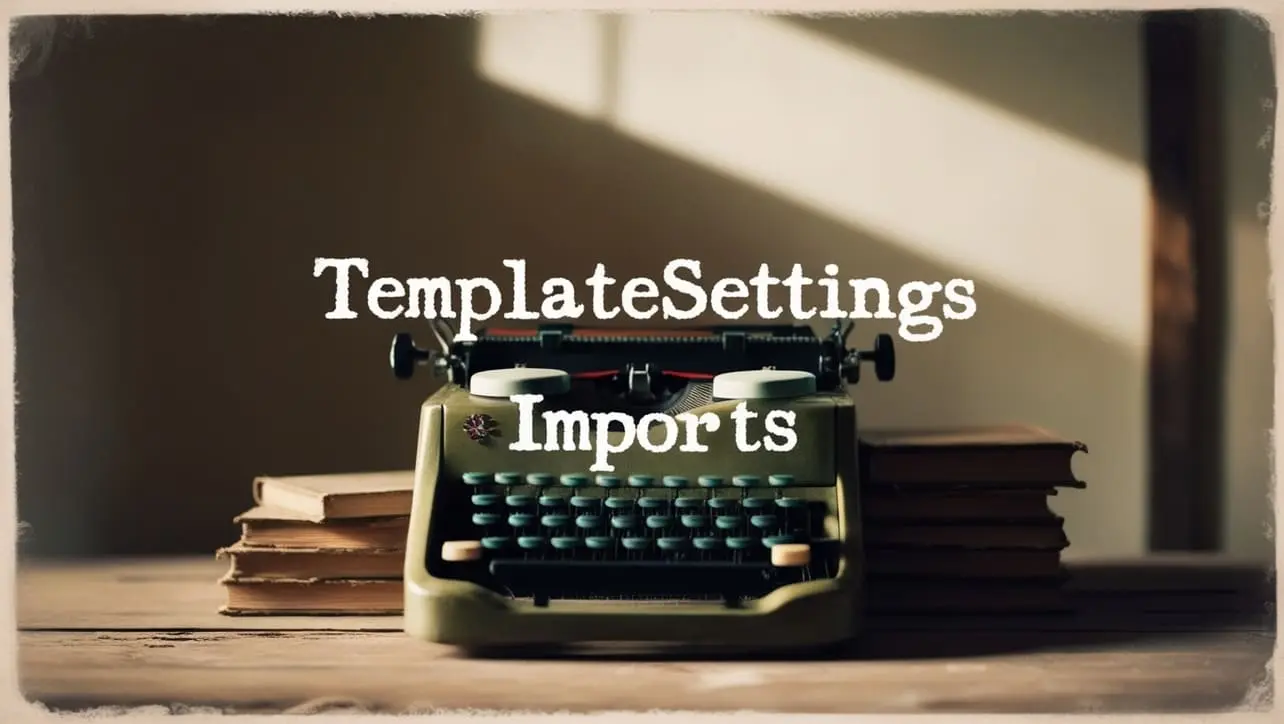
Lodash _.templateSettings.imports Property
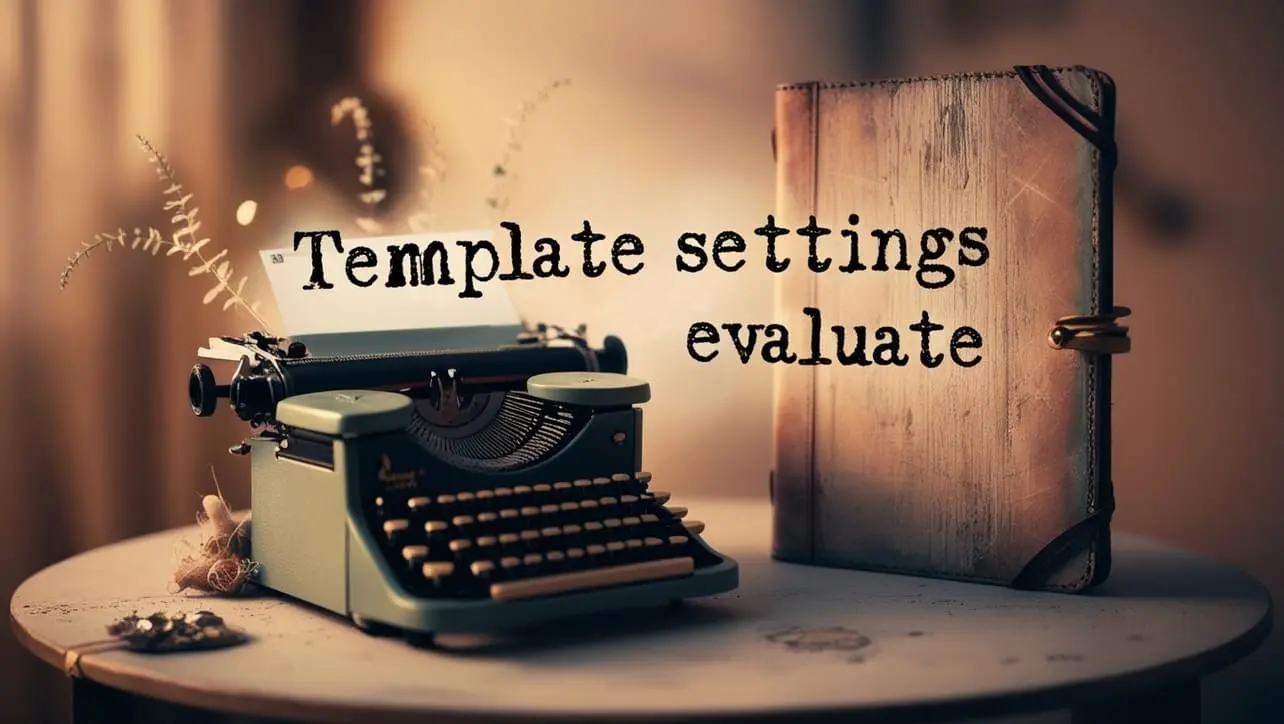
Lodash _.templateSettings.evaluate Property
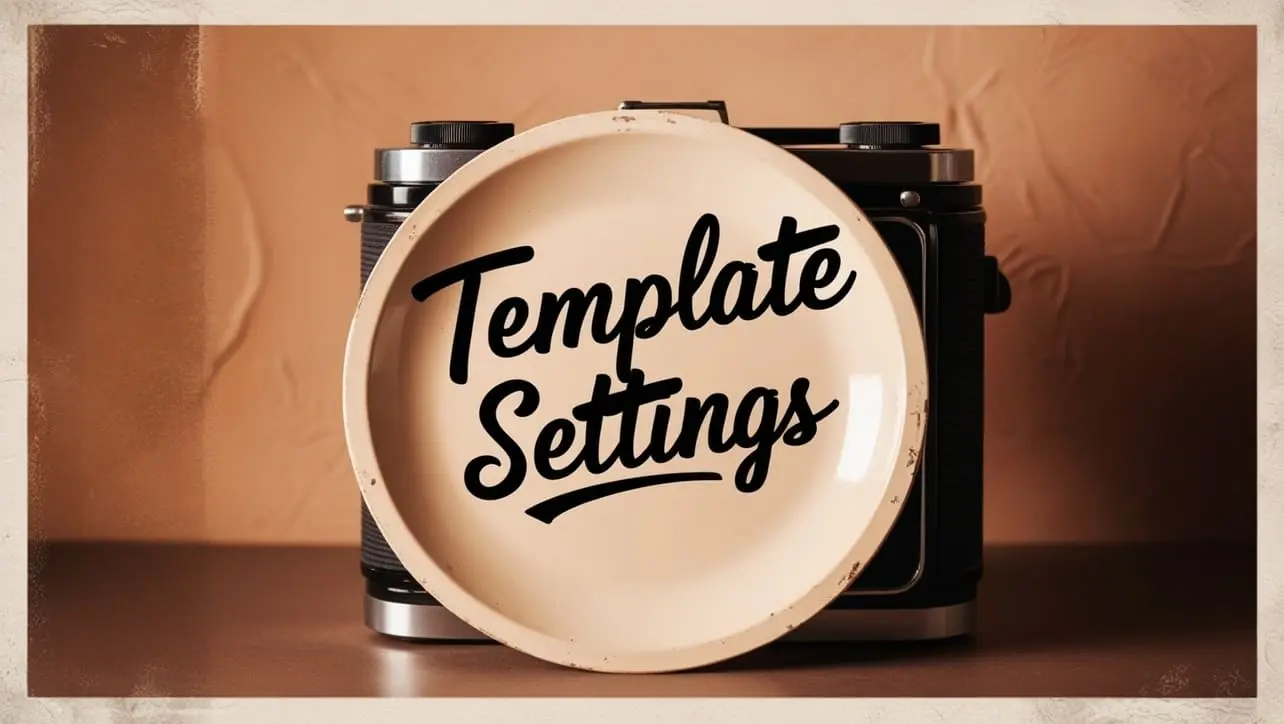
Lodash _.templateSettings Property
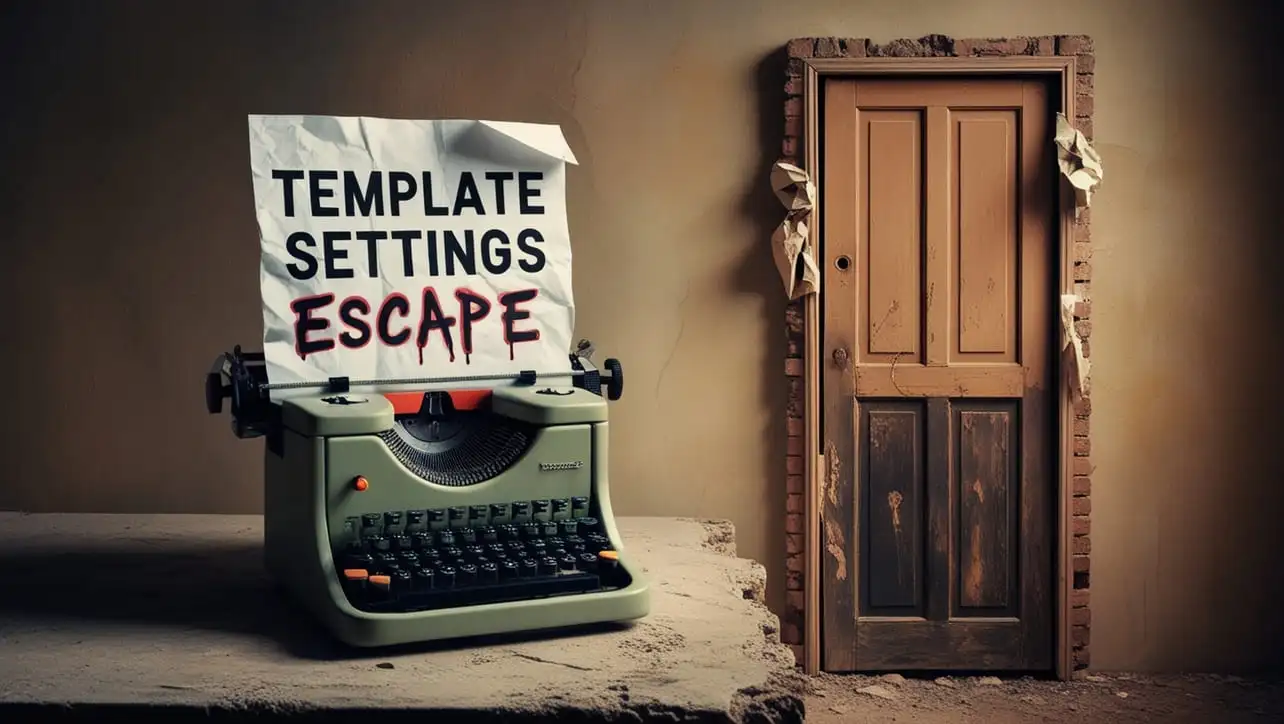
Lodash _.templateSettings.escape Property
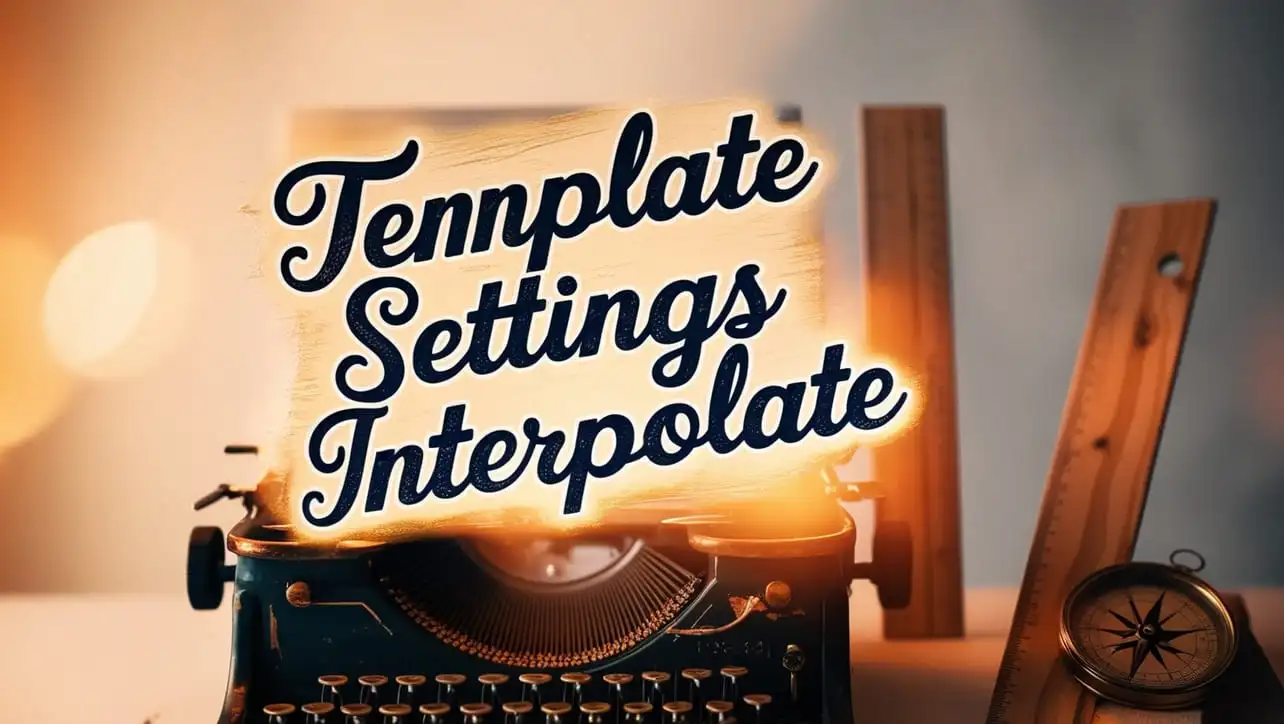
Lodash _.templateSettings.interpolate Property
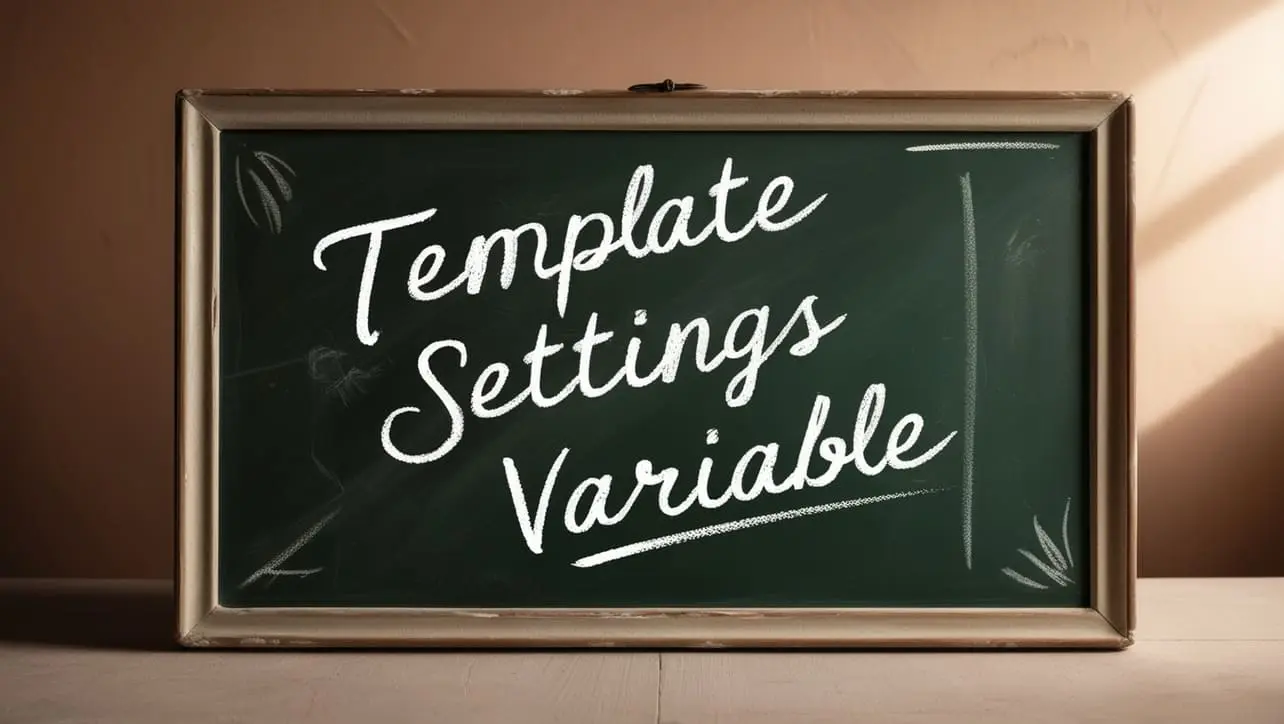
If you have any doubts regarding this article (Lodash _.transform() Object Method), please comment here. I will help you immediately.