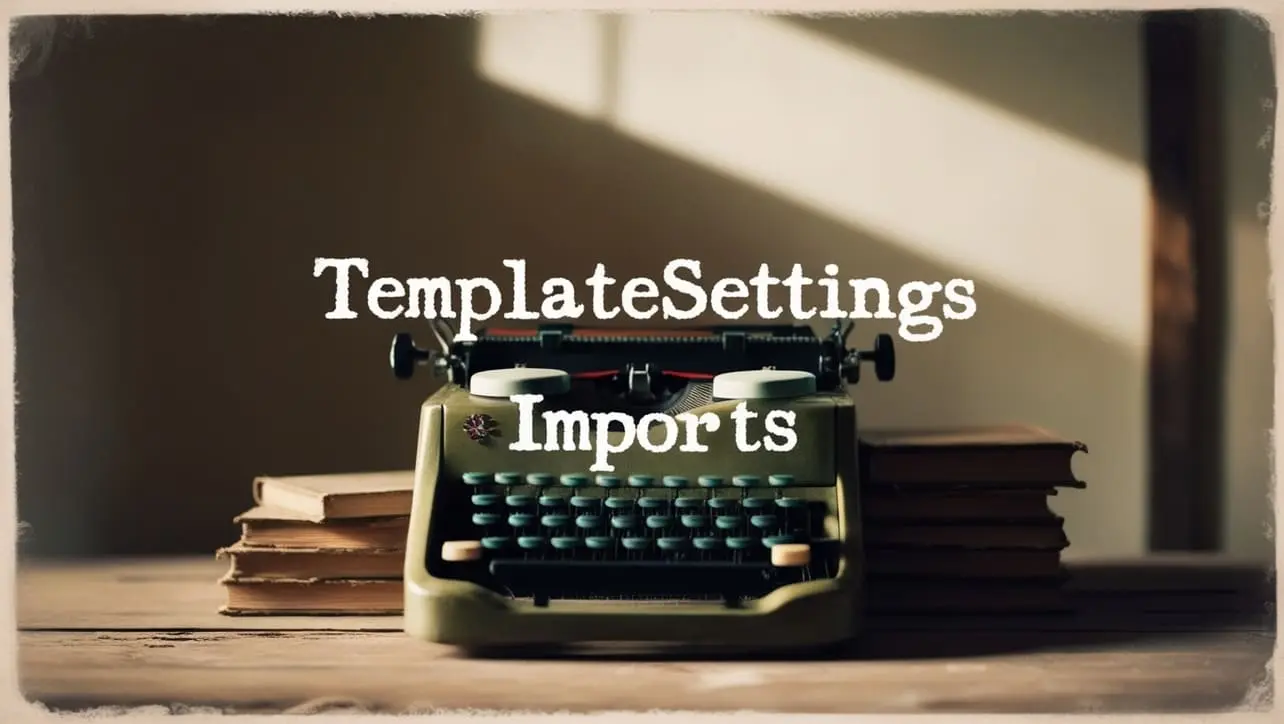
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.result() Object Method
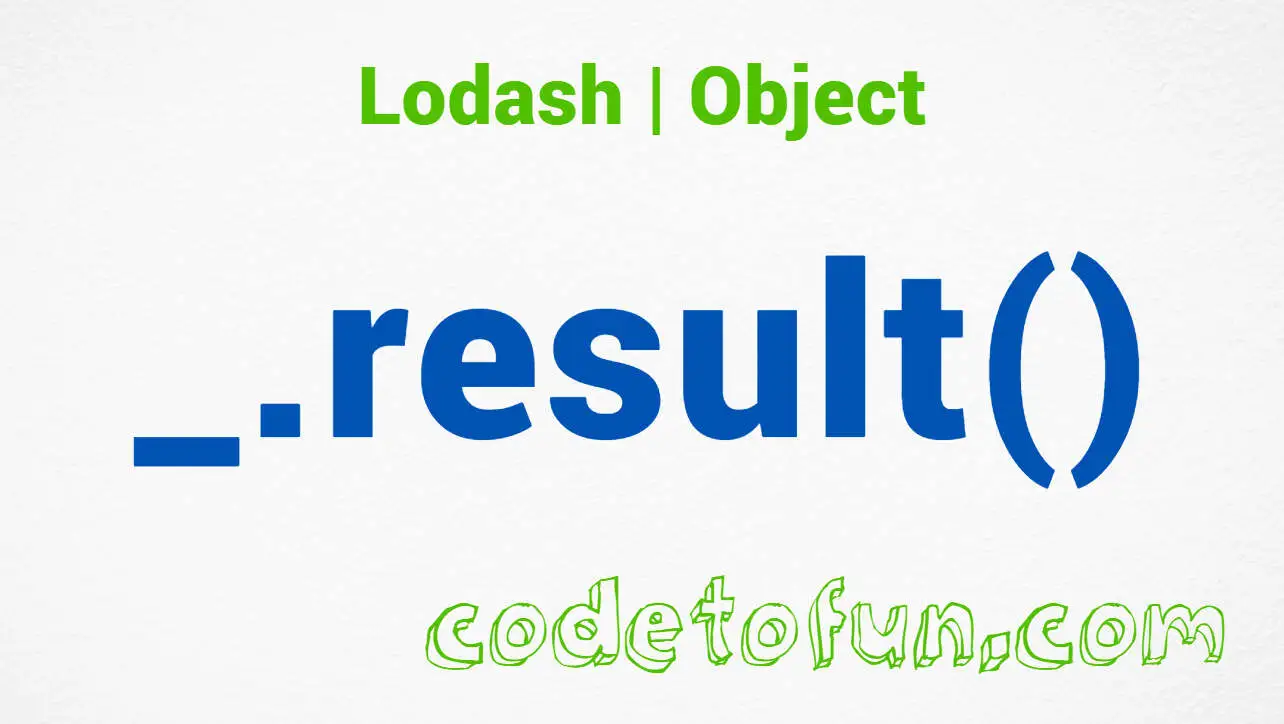
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript development, working with objects is a common task, and having utilities to simplify object manipulation can greatly enhance productivity. Enter Lodash, a popular utility library offering a wide range of functions for various programming tasks. Among these functions is _.result()
, a versatile method designed to retrieve the value of a specified property from an object.
This method provides flexibility and convenience, making it a valuable tool for JavaScript developers.
🧠 Understanding _.result() Method
The _.result()
method in Lodash allows you to retrieve the value of a property from an object, providing a default value if the property does not exist or if it is a function. This method simplifies access to object properties and facilitates error handling when dealing with potentially undefined properties.
💡 Syntax
The syntax for the _.result()
method is straightforward:
_.result(object, path, [defaultValue])
- object: The object to query.
- path: The path of the property to retrieve.
- defaultValue (Optional): The default value returned if the property is undefined or a function.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.result()
method:
const _ = require('lodash');
const user = {
name: 'John Doe',
age: 30,
greet: function() {
return `Hello, my name is ${this.name}.`;
}
};
const userName = _.result(user, 'name');
const userGreeting = _.result(user, 'greet', 'Greetings not available');
console.log(userName);
// Output: 'John Doe'
console.log(userGreeting);
// Output: 'Hello, my name is John Doe.'
In this example, _.result()
retrieves the value of the name property from the user object and invokes the greet function to generate a personalized greeting.
🏆 Best Practices
When working with the _.result()
method, consider the following best practices:
Handling Undefined Properties:
Use
_.result()
to safely retrieve properties from objects, providing a default value if the property is undefined or a function.example.jsCopiedconst user = { name: 'Jane Doe' }; const userAge = _.result(user, 'age', 'Age not available'); console.log(userAge); // Output: 'Age not available'
Default Values for Functions:
When retrieving properties that are functions, specify a default value to handle cases where the property is not a function.
example.jsCopiedconst user = { name: 'Jane Doe', greet: 'Hello' }; const userGreeting = _.result(user, 'greet', 'Greetings not available'); console.log(userGreeting); // Output: 'Greetings not available'
Nested Properties:
_.result()
supports accessing nested properties using dot notation or an array of keys.example.jsCopiedconst car = { details: { make: 'Toyota', model: 'Camry' } }; const carMake = _.result(car, 'details.make'); console.log(carMake); // Output: 'Toyota'
📚 Use Cases
Default Values for Object Properties:
_.result()
is useful for providing default values for object properties, ensuring consistent behavior in your applications.example.jsCopiedconst user = { name: 'John Doe' }; const userEmail = _.result(user, 'email', 'Email not provided'); console.log(userEmail); // Output: 'Email not provided'
Accessing Object Methods:
You can use
_.result()
to invoke object methods dynamically, providing a default value if the method is not available.example.jsCopiedconst user = { name: 'John Doe', greet: function() { return `Hello, my name is ${this.name}.`; } }; const userGreeting = _.result(user, 'greet', 'Greetings not available'); console.log(userGreeting); // Output: 'Hello, my name is John Doe.'
Dynamic Property Access:
When working with dynamic property names or nested objects,
_.result()
offers a convenient way to access properties without encountering errors.example.jsCopiedconst user = { profile: { firstName: 'John', lastName: 'Doe' } }; const firstName = _.result(user, 'profile.firstName'); console.log(firstName); // Output: 'John'
🎉 Conclusion
The _.result()
method in Lodash provides a powerful and flexible solution for accessing object properties and invoking methods with ease. Whether you need to handle undefined properties, set default values, or access nested properties, _.result()
offers a versatile tool to streamline your JavaScript development workflow.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.result()
method in your Lodash projects.
👨💻 Join our Community:
Author
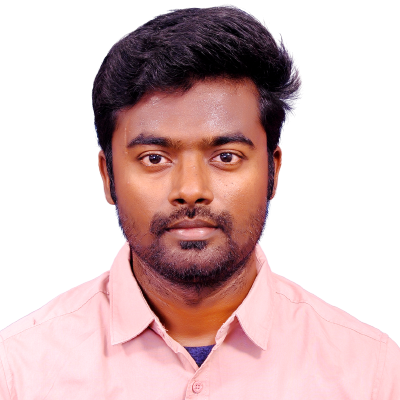
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
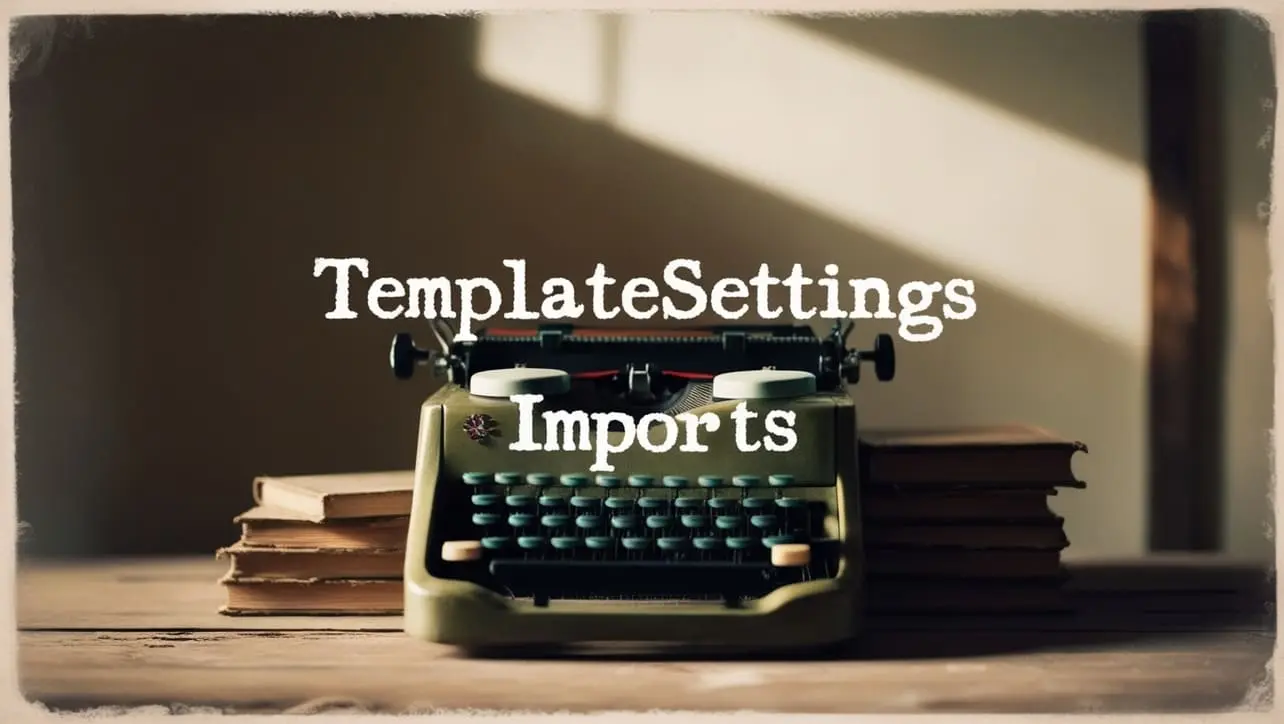
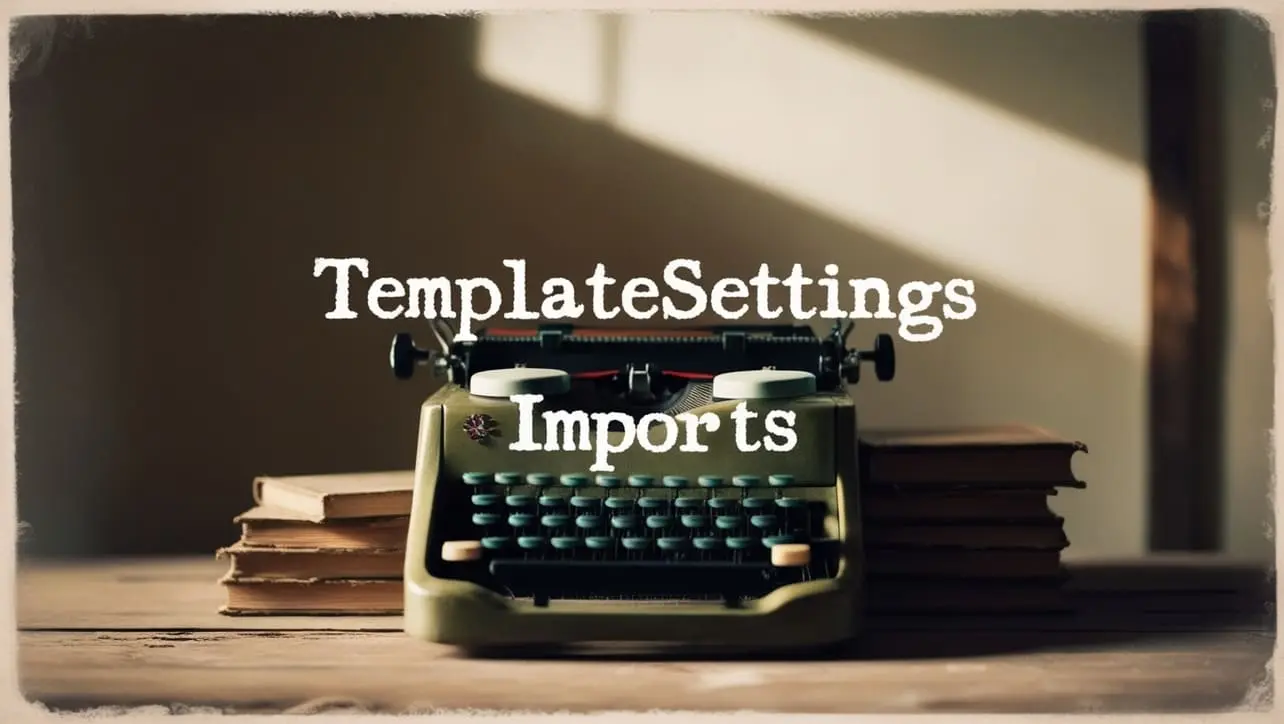
Lodash _.templateSettings.imports Property
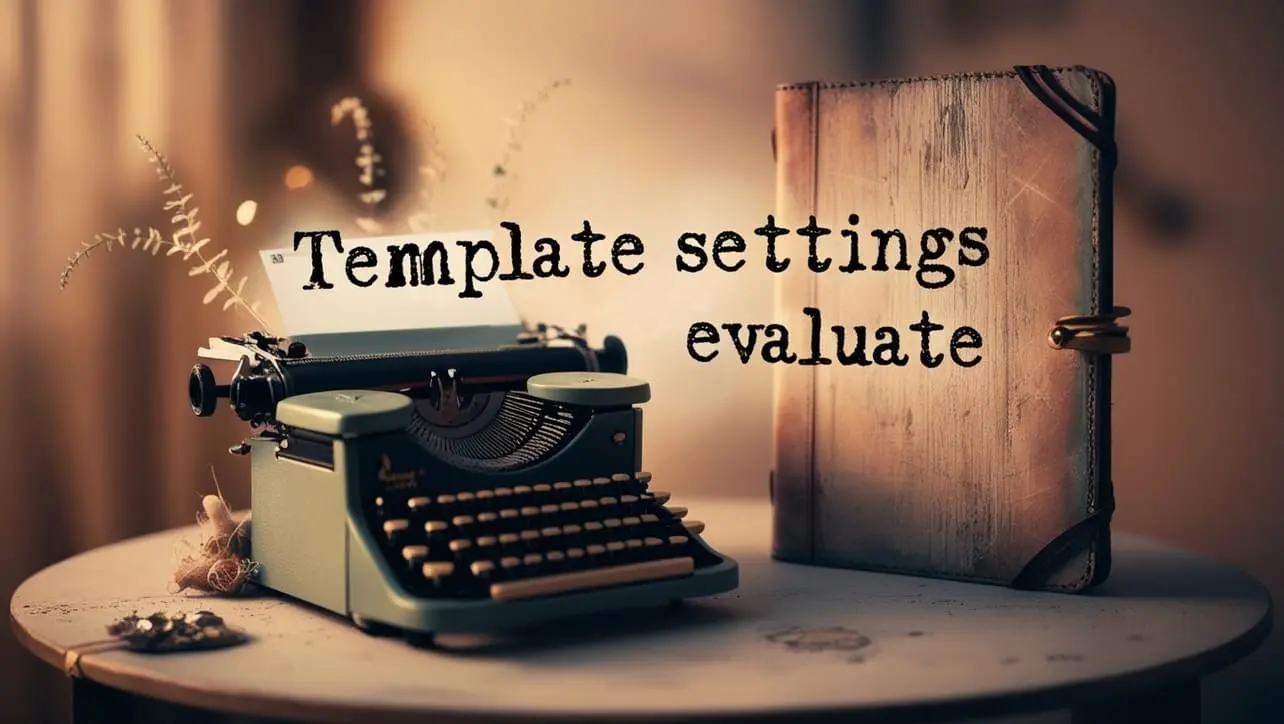
Lodash _.templateSettings.evaluate Property
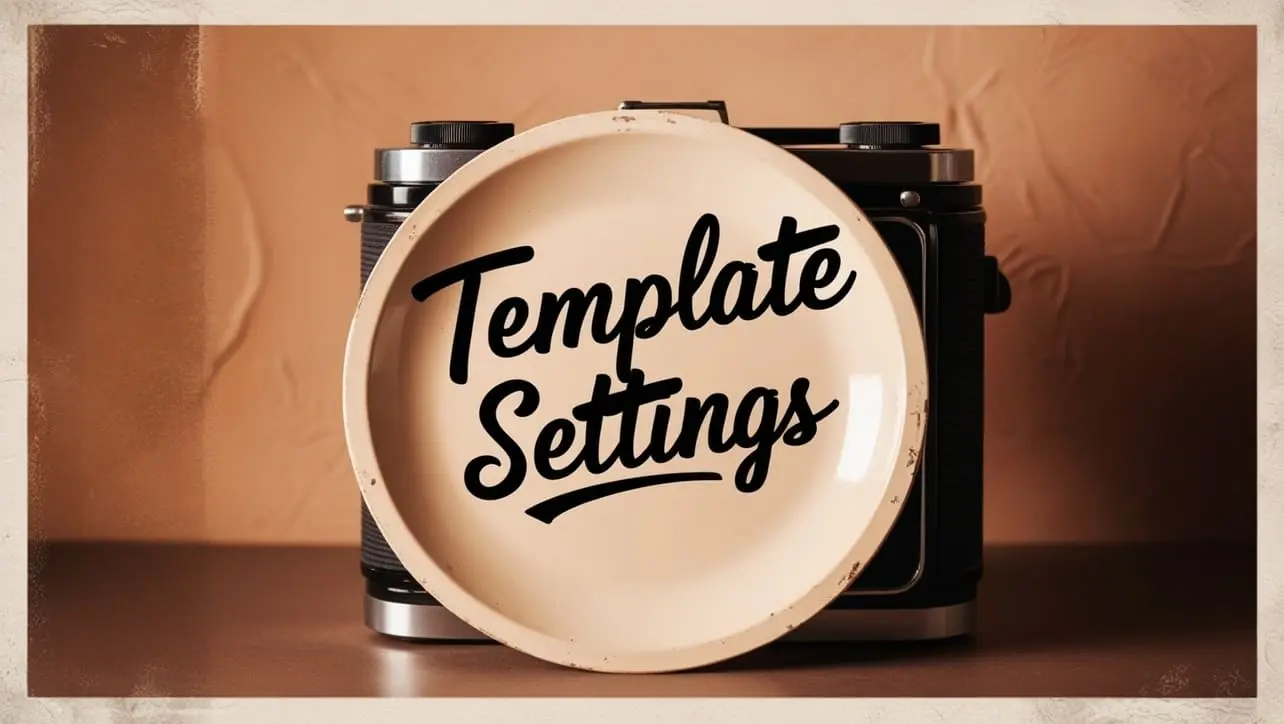
Lodash _.templateSettings Property
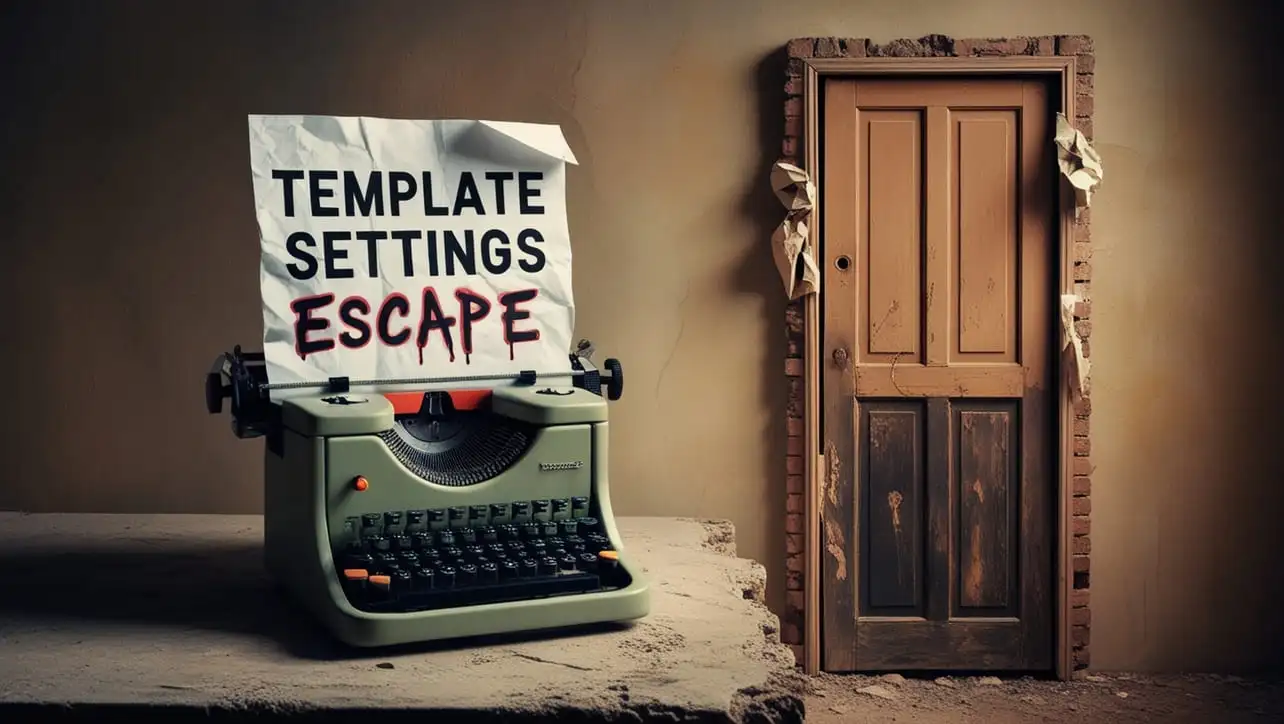
Lodash _.templateSettings.escape Property
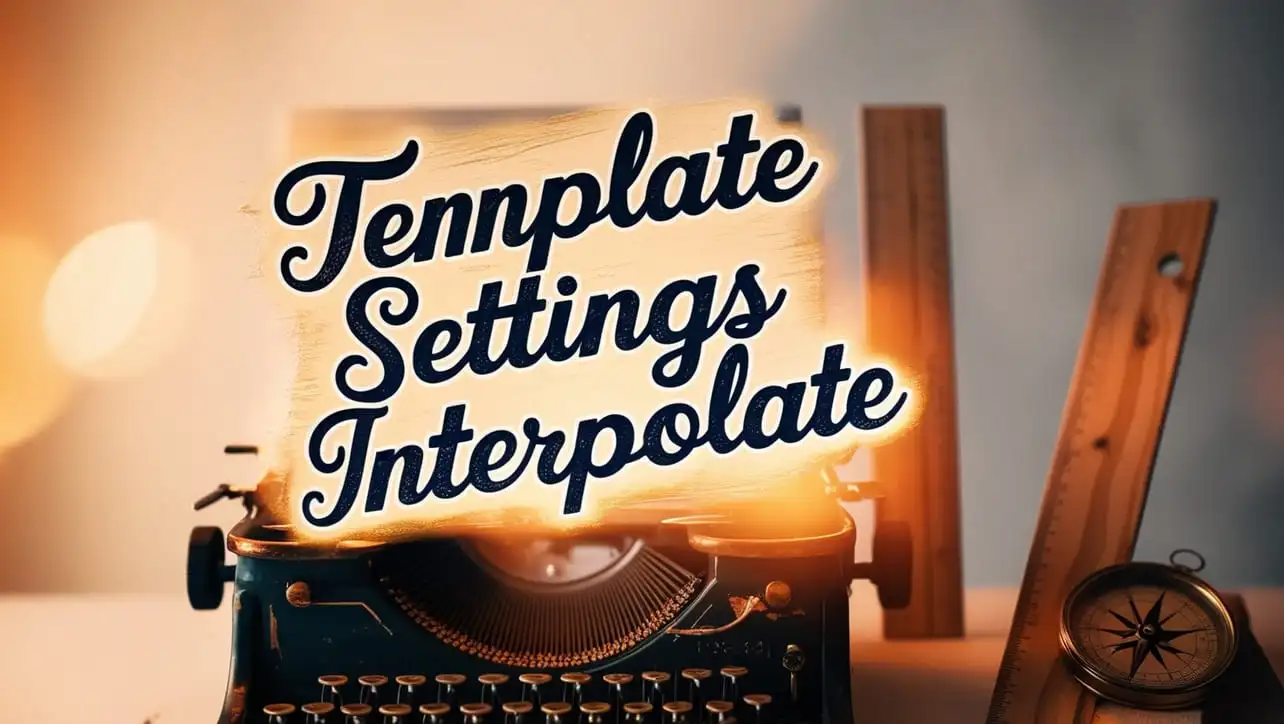
Lodash _.templateSettings.interpolate Property
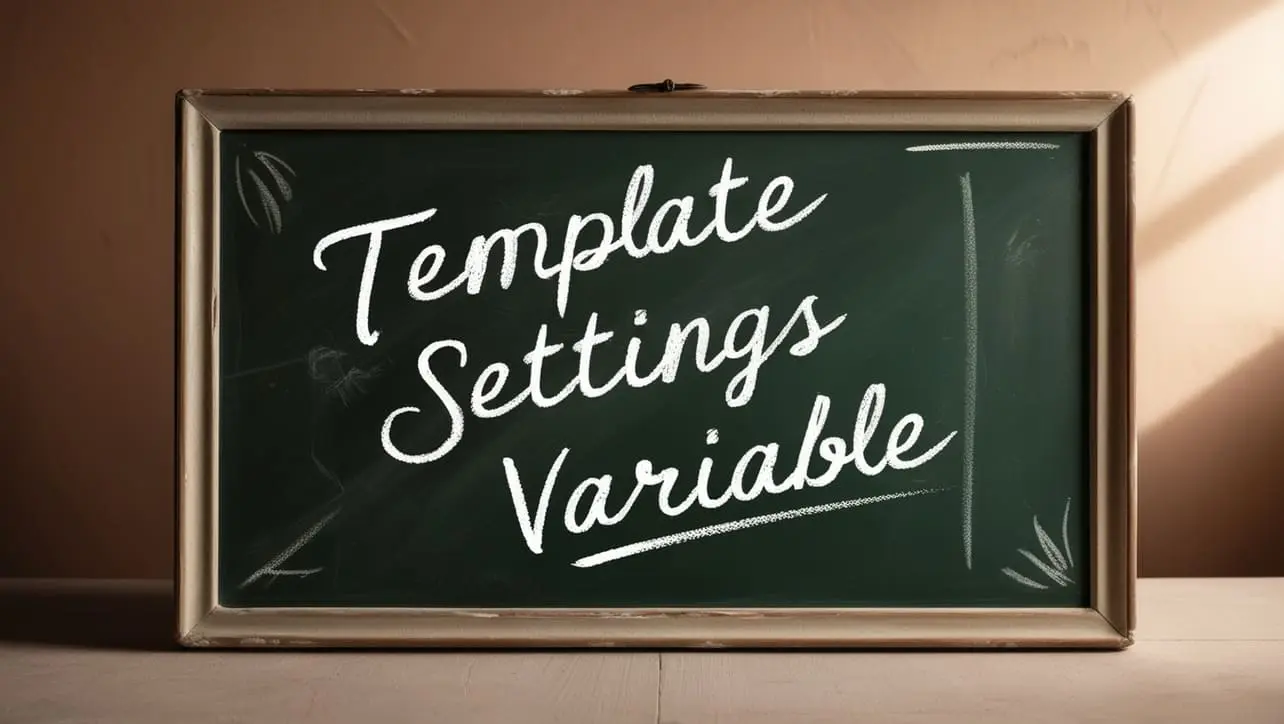
If you have any doubts regarding this article (Lodash _.result() Object Method), please comment here. I will help you immediately.